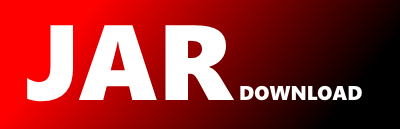
software.amazon.awssdk.services.fms.model.NetworkAclEntry Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a rule in a network ACL.
*
*
* Each network ACL has a set of numbered ingress rules and a separate set of numbered egress rules. When determining
* whether a packet should be allowed in or out of a subnet associated with the network ACL, Amazon Web Services
* processes the entries in the network ACL according to the rule numbers, in ascending order.
*
*
* When you manage an individual network ACL, you explicitly specify the rule numbers. When you specify the network ACL
* rules in a Firewall Manager policy, you provide the rules to run first, in the order that you want them to run, and
* the rules to run last, in the order that you want them to run. Firewall Manager assigns the rule numbers for you when
* you save the network ACL policy specification.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkAclEntry implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ICMP_TYPE_CODE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("IcmpTypeCode")
.getter(getter(NetworkAclEntry::icmpTypeCode)).setter(setter(Builder::icmpTypeCode))
.constructor(NetworkAclIcmpTypeCode::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IcmpTypeCode").build()).build();
private static final SdkField PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Protocol").getter(getter(NetworkAclEntry::protocol)).setter(setter(Builder::protocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Protocol").build()).build();
private static final SdkField PORT_RANGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PortRange")
.getter(getter(NetworkAclEntry::portRange)).setter(setter(Builder::portRange))
.constructor(NetworkAclPortRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PortRange").build()).build();
private static final SdkField CIDR_BLOCK_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CidrBlock").getter(getter(NetworkAclEntry::cidrBlock)).setter(setter(Builder::cidrBlock))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CidrBlock").build()).build();
private static final SdkField IPV6_CIDR_BLOCK_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Ipv6CidrBlock").getter(getter(NetworkAclEntry::ipv6CidrBlock)).setter(setter(Builder::ipv6CidrBlock))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6CidrBlock").build()).build();
private static final SdkField RULE_ACTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RuleAction").getter(getter(NetworkAclEntry::ruleActionAsString)).setter(setter(Builder::ruleAction))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuleAction").build()).build();
private static final SdkField EGRESS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Egress").getter(getter(NetworkAclEntry::egress)).setter(setter(Builder::egress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Egress").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ICMP_TYPE_CODE_FIELD,
PROTOCOL_FIELD, PORT_RANGE_FIELD, CIDR_BLOCK_FIELD, IPV6_CIDR_BLOCK_FIELD, RULE_ACTION_FIELD, EGRESS_FIELD));
private static final long serialVersionUID = 1L;
private final NetworkAclIcmpTypeCode icmpTypeCode;
private final String protocol;
private final NetworkAclPortRange portRange;
private final String cidrBlock;
private final String ipv6CidrBlock;
private final String ruleAction;
private final Boolean egress;
private NetworkAclEntry(BuilderImpl builder) {
this.icmpTypeCode = builder.icmpTypeCode;
this.protocol = builder.protocol;
this.portRange = builder.portRange;
this.cidrBlock = builder.cidrBlock;
this.ipv6CidrBlock = builder.ipv6CidrBlock;
this.ruleAction = builder.ruleAction;
this.egress = builder.egress;
}
/**
*
* ICMP protocol: The ICMP type and code.
*
*
* @return ICMP protocol: The ICMP type and code.
*/
public final NetworkAclIcmpTypeCode icmpTypeCode() {
return icmpTypeCode;
}
/**
*
* The protocol number. A value of "-1" means all protocols.
*
*
* @return The protocol number. A value of "-1" means all protocols.
*/
public final String protocol() {
return protocol;
}
/**
*
* TCP or UDP protocols: The range of ports the rule applies to.
*
*
* @return TCP or UDP protocols: The range of ports the rule applies to.
*/
public final NetworkAclPortRange portRange() {
return portRange;
}
/**
*
* The IPv4 network range to allow or deny, in CIDR notation.
*
*
* @return The IPv4 network range to allow or deny, in CIDR notation.
*/
public final String cidrBlock() {
return cidrBlock;
}
/**
*
* The IPv6 network range to allow or deny, in CIDR notation.
*
*
* @return The IPv6 network range to allow or deny, in CIDR notation.
*/
public final String ipv6CidrBlock() {
return ipv6CidrBlock;
}
/**
*
* Indicates whether to allow or deny the traffic that matches the rule.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ruleAction} will
* return {@link NetworkAclRuleAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #ruleActionAsString}.
*
*
* @return Indicates whether to allow or deny the traffic that matches the rule.
* @see NetworkAclRuleAction
*/
public final NetworkAclRuleAction ruleAction() {
return NetworkAclRuleAction.fromValue(ruleAction);
}
/**
*
* Indicates whether to allow or deny the traffic that matches the rule.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ruleAction} will
* return {@link NetworkAclRuleAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #ruleActionAsString}.
*
*
* @return Indicates whether to allow or deny the traffic that matches the rule.
* @see NetworkAclRuleAction
*/
public final String ruleActionAsString() {
return ruleAction;
}
/**
*
* Indicates whether the rule is an egress, or outbound, rule (applied to traffic leaving the subnet). If it's not
* an egress rule, then it's an ingress, or inbound, rule.
*
*
* @return Indicates whether the rule is an egress, or outbound, rule (applied to traffic leaving the subnet). If
* it's not an egress rule, then it's an ingress, or inbound, rule.
*/
public final Boolean egress() {
return egress;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(icmpTypeCode());
hashCode = 31 * hashCode + Objects.hashCode(protocol());
hashCode = 31 * hashCode + Objects.hashCode(portRange());
hashCode = 31 * hashCode + Objects.hashCode(cidrBlock());
hashCode = 31 * hashCode + Objects.hashCode(ipv6CidrBlock());
hashCode = 31 * hashCode + Objects.hashCode(ruleActionAsString());
hashCode = 31 * hashCode + Objects.hashCode(egress());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkAclEntry)) {
return false;
}
NetworkAclEntry other = (NetworkAclEntry) obj;
return Objects.equals(icmpTypeCode(), other.icmpTypeCode()) && Objects.equals(protocol(), other.protocol())
&& Objects.equals(portRange(), other.portRange()) && Objects.equals(cidrBlock(), other.cidrBlock())
&& Objects.equals(ipv6CidrBlock(), other.ipv6CidrBlock())
&& Objects.equals(ruleActionAsString(), other.ruleActionAsString()) && Objects.equals(egress(), other.egress());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NetworkAclEntry").add("IcmpTypeCode", icmpTypeCode()).add("Protocol", protocol())
.add("PortRange", portRange()).add("CidrBlock", cidrBlock()).add("Ipv6CidrBlock", ipv6CidrBlock())
.add("RuleAction", ruleActionAsString()).add("Egress", egress()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IcmpTypeCode":
return Optional.ofNullable(clazz.cast(icmpTypeCode()));
case "Protocol":
return Optional.ofNullable(clazz.cast(protocol()));
case "PortRange":
return Optional.ofNullable(clazz.cast(portRange()));
case "CidrBlock":
return Optional.ofNullable(clazz.cast(cidrBlock()));
case "Ipv6CidrBlock":
return Optional.ofNullable(clazz.cast(ipv6CidrBlock()));
case "RuleAction":
return Optional.ofNullable(clazz.cast(ruleActionAsString()));
case "Egress":
return Optional.ofNullable(clazz.cast(egress()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function