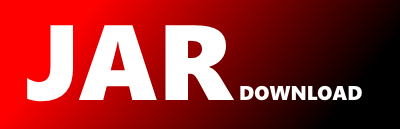
software.amazon.awssdk.services.fms.FmsClient Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.AssociateThirdPartyFirewallRequest;
import software.amazon.awssdk.services.fms.model.AssociateThirdPartyFirewallResponse;
import software.amazon.awssdk.services.fms.model.BatchAssociateResourceRequest;
import software.amazon.awssdk.services.fms.model.BatchAssociateResourceResponse;
import software.amazon.awssdk.services.fms.model.BatchDisassociateResourceRequest;
import software.amazon.awssdk.services.fms.model.BatchDisassociateResourceResponse;
import software.amazon.awssdk.services.fms.model.DeleteAppsListRequest;
import software.amazon.awssdk.services.fms.model.DeleteAppsListResponse;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.DeletePolicyRequest;
import software.amazon.awssdk.services.fms.model.DeletePolicyResponse;
import software.amazon.awssdk.services.fms.model.DeleteProtocolsListRequest;
import software.amazon.awssdk.services.fms.model.DeleteProtocolsListResponse;
import software.amazon.awssdk.services.fms.model.DeleteResourceSetRequest;
import software.amazon.awssdk.services.fms.model.DeleteResourceSetResponse;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.DisassociateThirdPartyFirewallRequest;
import software.amazon.awssdk.services.fms.model.DisassociateThirdPartyFirewallResponse;
import software.amazon.awssdk.services.fms.model.FmsException;
import software.amazon.awssdk.services.fms.model.GetAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.GetAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.GetAdminScopeRequest;
import software.amazon.awssdk.services.fms.model.GetAdminScopeResponse;
import software.amazon.awssdk.services.fms.model.GetAppsListRequest;
import software.amazon.awssdk.services.fms.model.GetAppsListResponse;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailRequest;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailResponse;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.GetPolicyRequest;
import software.amazon.awssdk.services.fms.model.GetPolicyResponse;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusRequest;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusResponse;
import software.amazon.awssdk.services.fms.model.GetProtocolsListRequest;
import software.amazon.awssdk.services.fms.model.GetProtocolsListResponse;
import software.amazon.awssdk.services.fms.model.GetResourceSetRequest;
import software.amazon.awssdk.services.fms.model.GetResourceSetResponse;
import software.amazon.awssdk.services.fms.model.GetThirdPartyFirewallAssociationStatusRequest;
import software.amazon.awssdk.services.fms.model.GetThirdPartyFirewallAssociationStatusResponse;
import software.amazon.awssdk.services.fms.model.GetViolationDetailsRequest;
import software.amazon.awssdk.services.fms.model.GetViolationDetailsResponse;
import software.amazon.awssdk.services.fms.model.InternalErrorException;
import software.amazon.awssdk.services.fms.model.InvalidInputException;
import software.amazon.awssdk.services.fms.model.InvalidOperationException;
import software.amazon.awssdk.services.fms.model.InvalidTypeException;
import software.amazon.awssdk.services.fms.model.LimitExceededException;
import software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest;
import software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationResponse;
import software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest;
import software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountResponse;
import software.amazon.awssdk.services.fms.model.ListAppsListsRequest;
import software.amazon.awssdk.services.fms.model.ListAppsListsResponse;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse;
import software.amazon.awssdk.services.fms.model.ListDiscoveredResourcesRequest;
import software.amazon.awssdk.services.fms.model.ListDiscoveredResourcesResponse;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse;
import software.amazon.awssdk.services.fms.model.ListPoliciesRequest;
import software.amazon.awssdk.services.fms.model.ListPoliciesResponse;
import software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest;
import software.amazon.awssdk.services.fms.model.ListProtocolsListsResponse;
import software.amazon.awssdk.services.fms.model.ListResourceSetResourcesRequest;
import software.amazon.awssdk.services.fms.model.ListResourceSetResourcesResponse;
import software.amazon.awssdk.services.fms.model.ListResourceSetsRequest;
import software.amazon.awssdk.services.fms.model.ListResourceSetsResponse;
import software.amazon.awssdk.services.fms.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.fms.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest;
import software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesResponse;
import software.amazon.awssdk.services.fms.model.PutAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.PutAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.PutAppsListRequest;
import software.amazon.awssdk.services.fms.model.PutAppsListResponse;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.PutPolicyRequest;
import software.amazon.awssdk.services.fms.model.PutPolicyResponse;
import software.amazon.awssdk.services.fms.model.PutProtocolsListRequest;
import software.amazon.awssdk.services.fms.model.PutProtocolsListResponse;
import software.amazon.awssdk.services.fms.model.PutResourceSetRequest;
import software.amazon.awssdk.services.fms.model.PutResourceSetResponse;
import software.amazon.awssdk.services.fms.model.ResourceNotFoundException;
import software.amazon.awssdk.services.fms.model.TagResourceRequest;
import software.amazon.awssdk.services.fms.model.TagResourceResponse;
import software.amazon.awssdk.services.fms.model.UntagResourceRequest;
import software.amazon.awssdk.services.fms.model.UntagResourceResponse;
import software.amazon.awssdk.services.fms.paginators.ListAdminAccountsForOrganizationIterable;
import software.amazon.awssdk.services.fms.paginators.ListAdminsManagingAccountIterable;
import software.amazon.awssdk.services.fms.paginators.ListAppsListsIterable;
import software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable;
import software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable;
import software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable;
import software.amazon.awssdk.services.fms.paginators.ListProtocolsListsIterable;
import software.amazon.awssdk.services.fms.paginators.ListThirdPartyFirewallFirewallPoliciesIterable;
/**
* Service client for accessing FMS. This can be created using the static {@link #builder()} method.
*
*
* This is the Firewall Manager API Reference. This guide is for developers who need detailed information about
* the Firewall Manager API actions, data types, and errors. For detailed information about Firewall Manager features,
* see the Firewall Manager Developer
* Guide.
*
*
* Some API actions require explicit resource permissions. For information, see the developer guide topic Service roles for Firewall Manager.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface FmsClient extends AwsClient {
String SERVICE_NAME = "fms";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "fms";
/**
*
* Sets a Firewall Manager default administrator account. The Firewall Manager default administrator account can
* manage third-party firewalls and has full administrative scope that allows administration of all policy types,
* accounts, organizational units, and Regions. This account must be a member account of the organization in
* Organizations whose resources you want to protect.
*
*
* For information about working with Firewall Manager administrator accounts, see Managing Firewall
* Manager administrators in the Firewall Manager Developer Guide.
*
*
* @param associateAdminAccountRequest
* @return Result of the AssociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.AssociateAdminAccount
* @see AWS API
* Documentation
*/
default AssociateAdminAccountResponse associateAdminAccount(AssociateAdminAccountRequest associateAdminAccountRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
LimitExceededException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Sets a Firewall Manager default administrator account. The Firewall Manager default administrator account can
* manage third-party firewalls and has full administrative scope that allows administration of all policy types,
* accounts, organizational units, and Regions. This account must be a member account of the organization in
* Organizations whose resources you want to protect.
*
*
* For information about working with Firewall Manager administrator accounts, see Managing Firewall
* Manager administrators in the Firewall Manager Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateAdminAccountRequest.Builder} avoiding the
* need to create one manually via {@link AssociateAdminAccountRequest#builder()}
*
*
* @param associateAdminAccountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.AssociateAdminAccountRequest.Builder} to create a
* request.
* @return Result of the AssociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.AssociateAdminAccount
* @see AWS API
* Documentation
*/
default AssociateAdminAccountResponse associateAdminAccount(
Consumer associateAdminAccountRequest) throws InvalidOperationException,
InvalidInputException, ResourceNotFoundException, InternalErrorException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
return associateAdminAccount(AssociateAdminAccountRequest.builder().applyMutation(associateAdminAccountRequest).build());
}
/**
*
* Sets the Firewall Manager policy administrator as a tenant administrator of a third-party firewall service. A
* tenant is an instance of the third-party firewall service that's associated with your Amazon Web Services
* customer account.
*
*
* @param associateThirdPartyFirewallRequest
* @return Result of the AssociateThirdPartyFirewall operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.AssociateThirdPartyFirewall
* @see AWS API Documentation
*/
default AssociateThirdPartyFirewallResponse associateThirdPartyFirewall(
AssociateThirdPartyFirewallRequest associateThirdPartyFirewallRequest) throws InvalidOperationException,
InvalidInputException, ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the Firewall Manager policy administrator as a tenant administrator of a third-party firewall service. A
* tenant is an instance of the third-party firewall service that's associated with your Amazon Web Services
* customer account.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateThirdPartyFirewallRequest.Builder}
* avoiding the need to create one manually via {@link AssociateThirdPartyFirewallRequest#builder()}
*
*
* @param associateThirdPartyFirewallRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.AssociateThirdPartyFirewallRequest.Builder} to create a
* request.
* @return Result of the AssociateThirdPartyFirewall operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.AssociateThirdPartyFirewall
* @see AWS API Documentation
*/
default AssociateThirdPartyFirewallResponse associateThirdPartyFirewall(
Consumer associateThirdPartyFirewallRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return associateThirdPartyFirewall(AssociateThirdPartyFirewallRequest.builder()
.applyMutation(associateThirdPartyFirewallRequest).build());
}
/**
*
* Associate resources to a Firewall Manager resource set.
*
*
* @param batchAssociateResourceRequest
* @return Result of the BatchAssociateResource operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.BatchAssociateResource
* @see AWS
* API Documentation
*/
default BatchAssociateResourceResponse batchAssociateResource(BatchAssociateResourceRequest batchAssociateResourceRequest)
throws InvalidOperationException, InternalErrorException, InvalidInputException, LimitExceededException,
ResourceNotFoundException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Associate resources to a Firewall Manager resource set.
*
*
*
* This is a convenience which creates an instance of the {@link BatchAssociateResourceRequest.Builder} avoiding the
* need to create one manually via {@link BatchAssociateResourceRequest#builder()}
*
*
* @param batchAssociateResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.BatchAssociateResourceRequest.Builder} to create a
* request.
* @return Result of the BatchAssociateResource operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.BatchAssociateResource
* @see AWS
* API Documentation
*/
default BatchAssociateResourceResponse batchAssociateResource(
Consumer batchAssociateResourceRequest) throws InvalidOperationException,
InternalErrorException, InvalidInputException, LimitExceededException, ResourceNotFoundException,
AwsServiceException, SdkClientException, FmsException {
return batchAssociateResource(BatchAssociateResourceRequest.builder().applyMutation(batchAssociateResourceRequest)
.build());
}
/**
*
* Disassociates resources from a Firewall Manager resource set.
*
*
* @param batchDisassociateResourceRequest
* @return Result of the BatchDisassociateResource operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.BatchDisassociateResource
* @see AWS
* API Documentation
*/
default BatchDisassociateResourceResponse batchDisassociateResource(
BatchDisassociateResourceRequest batchDisassociateResourceRequest) throws InvalidOperationException,
InternalErrorException, InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates resources from a Firewall Manager resource set.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDisassociateResourceRequest.Builder} avoiding
* the need to create one manually via {@link BatchDisassociateResourceRequest#builder()}
*
*
* @param batchDisassociateResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.BatchDisassociateResourceRequest.Builder} to create a
* request.
* @return Result of the BatchDisassociateResource operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.BatchDisassociateResource
* @see AWS
* API Documentation
*/
default BatchDisassociateResourceResponse batchDisassociateResource(
Consumer batchDisassociateResourceRequest)
throws InvalidOperationException, InternalErrorException, InvalidInputException, ResourceNotFoundException,
AwsServiceException, SdkClientException, FmsException {
return batchDisassociateResource(BatchDisassociateResourceRequest.builder()
.applyMutation(batchDisassociateResourceRequest).build());
}
/**
*
* Permanently deletes an Firewall Manager applications list.
*
*
* @param deleteAppsListRequest
* @return Result of the DeleteAppsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteAppsList
* @see AWS API
* Documentation
*/
default DeleteAppsListResponse deleteAppsList(DeleteAppsListRequest deleteAppsListRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes an Firewall Manager applications list.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAppsListRequest.Builder} avoiding the need to
* create one manually via {@link DeleteAppsListRequest#builder()}
*
*
* @param deleteAppsListRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.DeleteAppsListRequest.Builder} to create a request.
* @return Result of the DeleteAppsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteAppsList
* @see AWS API
* Documentation
*/
default DeleteAppsListResponse deleteAppsList(Consumer deleteAppsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return deleteAppsList(DeleteAppsListRequest.builder().applyMutation(deleteAppsListRequest).build());
}
/**
*
* Deletes an Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS) topic
* that is used to record Firewall Manager SNS logs.
*
*
* @param deleteNotificationChannelRequest
* @return Result of the DeleteNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
default DeleteNotificationChannelResponse deleteNotificationChannel(
DeleteNotificationChannelRequest deleteNotificationChannelRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS) topic
* that is used to record Firewall Manager SNS logs.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNotificationChannelRequest.Builder} avoiding
* the need to create one manually via {@link DeleteNotificationChannelRequest#builder()}
*
*
* @param deleteNotificationChannelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.DeleteNotificationChannelRequest.Builder} to create a
* request.
* @return Result of the DeleteNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
default DeleteNotificationChannelResponse deleteNotificationChannel(
Consumer deleteNotificationChannelRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return deleteNotificationChannel(DeleteNotificationChannelRequest.builder()
.applyMutation(deleteNotificationChannelRequest).build());
}
/**
*
* Permanently deletes an Firewall Manager policy.
*
*
* @param deletePolicyRequest
* @return Result of the DeletePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeletePolicy
* @see AWS API
* Documentation
*/
default DeletePolicyResponse deletePolicy(DeletePolicyRequest deletePolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidInputException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes an Firewall Manager policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePolicyRequest.Builder} avoiding the need to
* create one manually via {@link DeletePolicyRequest#builder()}
*
*
* @param deletePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.DeletePolicyRequest.Builder} to create a request.
* @return Result of the DeletePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeletePolicy
* @see AWS API
* Documentation
*/
default DeletePolicyResponse deletePolicy(Consumer deletePolicyRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, InvalidInputException,
LimitExceededException, AwsServiceException, SdkClientException, FmsException {
return deletePolicy(DeletePolicyRequest.builder().applyMutation(deletePolicyRequest).build());
}
/**
*
* Permanently deletes an Firewall Manager protocols list.
*
*
* @param deleteProtocolsListRequest
* @return Result of the DeleteProtocolsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteProtocolsList
* @see AWS API
* Documentation
*/
default DeleteProtocolsListResponse deleteProtocolsList(DeleteProtocolsListRequest deleteProtocolsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes an Firewall Manager protocols list.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProtocolsListRequest.Builder} avoiding the
* need to create one manually via {@link DeleteProtocolsListRequest#builder()}
*
*
* @param deleteProtocolsListRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.DeleteProtocolsListRequest.Builder} to create a request.
* @return Result of the DeleteProtocolsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteProtocolsList
* @see AWS API
* Documentation
*/
default DeleteProtocolsListResponse deleteProtocolsList(
Consumer deleteProtocolsListRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return deleteProtocolsList(DeleteProtocolsListRequest.builder().applyMutation(deleteProtocolsListRequest).build());
}
/**
*
* Deletes the specified ResourceSet.
*
*
* @param deleteResourceSetRequest
* @return Result of the DeleteResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteResourceSet
* @see AWS API
* Documentation
*/
default DeleteResourceSetResponse deleteResourceSet(DeleteResourceSetRequest deleteResourceSetRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, InvalidInputException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified ResourceSet.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteResourceSetRequest.Builder} avoiding the need
* to create one manually via {@link DeleteResourceSetRequest#builder()}
*
*
* @param deleteResourceSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.DeleteResourceSetRequest.Builder} to create a request.
* @return Result of the DeleteResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteResourceSet
* @see AWS API
* Documentation
*/
default DeleteResourceSetResponse deleteResourceSet(Consumer deleteResourceSetRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, InvalidInputException,
AwsServiceException, SdkClientException, FmsException {
return deleteResourceSet(DeleteResourceSetRequest.builder().applyMutation(deleteResourceSetRequest).build());
}
/**
*
* Disassociates an Firewall Manager administrator account. To set a different account as an Firewall Manager
* administrator, submit a PutAdminAccount request. To set an account as a default administrator account, you
* must submit an AssociateAdminAccount request.
*
*
* Disassociation of the default administrator account follows the first in, last out principle. If you are the
* default administrator, all Firewall Manager administrators within the organization must first disassociate their
* accounts before you can disassociate your account.
*
*
* @param disassociateAdminAccountRequest
* @return Result of the DisassociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
default DisassociateAdminAccountResponse disassociateAdminAccount(
DisassociateAdminAccountRequest disassociateAdminAccountRequest) throws InvalidOperationException,
ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates an Firewall Manager administrator account. To set a different account as an Firewall Manager
* administrator, submit a PutAdminAccount request. To set an account as a default administrator account, you
* must submit an AssociateAdminAccount request.
*
*
* Disassociation of the default administrator account follows the first in, last out principle. If you are the
* default administrator, all Firewall Manager administrators within the organization must first disassociate their
* accounts before you can disassociate your account.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateAdminAccountRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateAdminAccountRequest#builder()}
*
*
* @param disassociateAdminAccountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.DisassociateAdminAccountRequest.Builder} to create a
* request.
* @return Result of the DisassociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
default DisassociateAdminAccountResponse disassociateAdminAccount(
Consumer disassociateAdminAccountRequest) throws InvalidOperationException,
ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return disassociateAdminAccount(DisassociateAdminAccountRequest.builder().applyMutation(disassociateAdminAccountRequest)
.build());
}
/**
*
* Disassociates a Firewall Manager policy administrator from a third-party firewall tenant. When you call
* DisassociateThirdPartyFirewall
, the third-party firewall vendor deletes all of the firewalls that
* are associated with the account.
*
*
* @param disassociateThirdPartyFirewallRequest
* @return Result of the DisassociateThirdPartyFirewall operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DisassociateThirdPartyFirewall
* @see AWS API Documentation
*/
default DisassociateThirdPartyFirewallResponse disassociateThirdPartyFirewall(
DisassociateThirdPartyFirewallRequest disassociateThirdPartyFirewallRequest) throws InvalidOperationException,
InvalidInputException, ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a Firewall Manager policy administrator from a third-party firewall tenant. When you call
* DisassociateThirdPartyFirewall
, the third-party firewall vendor deletes all of the firewalls that
* are associated with the account.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateThirdPartyFirewallRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateThirdPartyFirewallRequest#builder()}
*
*
* @param disassociateThirdPartyFirewallRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.DisassociateThirdPartyFirewallRequest.Builder} to create
* a request.
* @return Result of the DisassociateThirdPartyFirewall operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DisassociateThirdPartyFirewall
* @see AWS API Documentation
*/
default DisassociateThirdPartyFirewallResponse disassociateThirdPartyFirewall(
Consumer disassociateThirdPartyFirewallRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return disassociateThirdPartyFirewall(DisassociateThirdPartyFirewallRequest.builder()
.applyMutation(disassociateThirdPartyFirewallRequest).build());
}
/**
*
* Returns the Organizations account that is associated with Firewall Manager as the Firewall Manager default
* administrator.
*
*
* @param getAdminAccountRequest
* @return Result of the GetAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAdminAccount
* @see AWS API
* Documentation
*/
default GetAdminAccountResponse getAdminAccount(GetAdminAccountRequest getAdminAccountRequest)
throws InvalidOperationException, ResourceNotFoundException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the Organizations account that is associated with Firewall Manager as the Firewall Manager default
* administrator.
*
*
*
* This is a convenience which creates an instance of the {@link GetAdminAccountRequest.Builder} avoiding the need
* to create one manually via {@link GetAdminAccountRequest#builder()}
*
*
* @param getAdminAccountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetAdminAccountRequest.Builder} to create a request.
* @return Result of the GetAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAdminAccount
* @see AWS API
* Documentation
*/
default GetAdminAccountResponse getAdminAccount(Consumer getAdminAccountRequest)
throws InvalidOperationException, ResourceNotFoundException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return getAdminAccount(GetAdminAccountRequest.builder().applyMutation(getAdminAccountRequest).build());
}
/**
*
* Returns information about the specified account's administrative scope. The administrative scope defines the
* resources that an Firewall Manager administrator can manage.
*
*
* @param getAdminScopeRequest
* @return Result of the GetAdminScope operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAdminScope
* @see AWS API
* Documentation
*/
default GetAdminScopeResponse getAdminScope(GetAdminScopeRequest getAdminScopeRequest) throws InvalidOperationException,
InvalidInputException, ResourceNotFoundException, InternalErrorException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified account's administrative scope. The administrative scope defines the
* resources that an Firewall Manager administrator can manage.
*
*
*
* This is a convenience which creates an instance of the {@link GetAdminScopeRequest.Builder} avoiding the need to
* create one manually via {@link GetAdminScopeRequest#builder()}
*
*
* @param getAdminScopeRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetAdminScopeRequest.Builder} to create a request.
* @return Result of the GetAdminScope operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAdminScope
* @see AWS API
* Documentation
*/
default GetAdminScopeResponse getAdminScope(Consumer getAdminScopeRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
LimitExceededException, AwsServiceException, SdkClientException, FmsException {
return getAdminScope(GetAdminScopeRequest.builder().applyMutation(getAdminScopeRequest).build());
}
/**
*
* Returns information about the specified Firewall Manager applications list.
*
*
* @param getAppsListRequest
* @return Result of the GetAppsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAppsList
* @see AWS API
* Documentation
*/
default GetAppsListResponse getAppsList(GetAppsListRequest getAppsListRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified Firewall Manager applications list.
*
*
*
* This is a convenience which creates an instance of the {@link GetAppsListRequest.Builder} avoiding the need to
* create one manually via {@link GetAppsListRequest#builder()}
*
*
* @param getAppsListRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetAppsListRequest.Builder} to create a request.
* @return Result of the GetAppsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAppsList
* @see AWS API
* Documentation
*/
default GetAppsListResponse getAppsList(Consumer getAppsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return getAppsList(GetAppsListRequest.builder().applyMutation(getAppsListRequest).build());
}
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy.
*
*
* The reasons for resources being considered compliant depend on the Firewall Manager policy type.
*
*
* @param getComplianceDetailRequest
* @return Result of the GetComplianceDetail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetComplianceDetail
* @see AWS API
* Documentation
*/
default GetComplianceDetailResponse getComplianceDetail(GetComplianceDetailRequest getComplianceDetailRequest)
throws ResourceNotFoundException, InternalErrorException, InvalidInputException, InvalidOperationException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy.
*
*
* The reasons for resources being considered compliant depend on the Firewall Manager policy type.
*
*
*
* This is a convenience which creates an instance of the {@link GetComplianceDetailRequest.Builder} avoiding the
* need to create one manually via {@link GetComplianceDetailRequest#builder()}
*
*
* @param getComplianceDetailRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetComplianceDetailRequest.Builder} to create a request.
* @return Result of the GetComplianceDetail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetComplianceDetail
* @see AWS API
* Documentation
*/
default GetComplianceDetailResponse getComplianceDetail(
Consumer getComplianceDetailRequest) throws ResourceNotFoundException,
InternalErrorException, InvalidInputException, InvalidOperationException, AwsServiceException, SdkClientException,
FmsException {
return getComplianceDetail(GetComplianceDetailRequest.builder().applyMutation(getComplianceDetailRequest).build());
}
/**
*
* Information about the Amazon Simple Notification Service (SNS) topic that is used to record Firewall Manager SNS
* logs.
*
*
* @param getNotificationChannelRequest
* @return Result of the GetNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetNotificationChannel
* @see AWS
* API Documentation
*/
default GetNotificationChannelResponse getNotificationChannel(GetNotificationChannelRequest getNotificationChannelRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Information about the Amazon Simple Notification Service (SNS) topic that is used to record Firewall Manager SNS
* logs.
*
*
*
* This is a convenience which creates an instance of the {@link GetNotificationChannelRequest.Builder} avoiding the
* need to create one manually via {@link GetNotificationChannelRequest#builder()}
*
*
* @param getNotificationChannelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetNotificationChannelRequest.Builder} to create a
* request.
* @return Result of the GetNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetNotificationChannel
* @see AWS
* API Documentation
*/
default GetNotificationChannelResponse getNotificationChannel(
Consumer getNotificationChannelRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return getNotificationChannel(GetNotificationChannelRequest.builder().applyMutation(getNotificationChannelRequest)
.build());
}
/**
*
* Returns information about the specified Firewall Manager policy.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetPolicy
* @see AWS API
* Documentation
*/
default GetPolicyResponse getPolicy(GetPolicyRequest getPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidTypeException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified Firewall Manager policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetPolicyRequest#builder()}
*
*
* @param getPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetPolicyRequest.Builder} to create a request.
* @return Result of the GetPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetPolicy
* @see AWS API
* Documentation
*/
default GetPolicyResponse getPolicy(Consumer getPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidTypeException, AwsServiceException, SdkClientException,
FmsException {
return getPolicy(GetPolicyRequest.builder().applyMutation(getPolicyRequest).build());
}
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack. Other policy types are currently unsupported.
*
*
* @param getProtectionStatusRequest
* @return Result of the GetProtectionStatus operation returned by the service.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetProtectionStatus
* @see AWS API
* Documentation
*/
default GetProtectionStatusResponse getProtectionStatus(GetProtectionStatusRequest getProtectionStatusRequest)
throws InvalidInputException, ResourceNotFoundException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack. Other policy types are currently unsupported.
*
*
*
* This is a convenience which creates an instance of the {@link GetProtectionStatusRequest.Builder} avoiding the
* need to create one manually via {@link GetProtectionStatusRequest#builder()}
*
*
* @param getProtectionStatusRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetProtectionStatusRequest.Builder} to create a request.
* @return Result of the GetProtectionStatus operation returned by the service.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetProtectionStatus
* @see AWS API
* Documentation
*/
default GetProtectionStatusResponse getProtectionStatus(
Consumer getProtectionStatusRequest) throws InvalidInputException,
ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return getProtectionStatus(GetProtectionStatusRequest.builder().applyMutation(getProtectionStatusRequest).build());
}
/**
*
* Returns information about the specified Firewall Manager protocols list.
*
*
* @param getProtocolsListRequest
* @return Result of the GetProtocolsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetProtocolsList
* @see AWS API
* Documentation
*/
default GetProtocolsListResponse getProtocolsList(GetProtocolsListRequest getProtocolsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified Firewall Manager protocols list.
*
*
*
* This is a convenience which creates an instance of the {@link GetProtocolsListRequest.Builder} avoiding the need
* to create one manually via {@link GetProtocolsListRequest#builder()}
*
*
* @param getProtocolsListRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetProtocolsListRequest.Builder} to create a request.
* @return Result of the GetProtocolsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetProtocolsList
* @see AWS API
* Documentation
*/
default GetProtocolsListResponse getProtocolsList(Consumer getProtocolsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return getProtocolsList(GetProtocolsListRequest.builder().applyMutation(getProtocolsListRequest).build());
}
/**
*
* Gets information about a specific resource set.
*
*
* @param getResourceSetRequest
* @return Result of the GetResourceSet operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetResourceSet
* @see AWS API
* Documentation
*/
default GetResourceSetResponse getResourceSet(GetResourceSetRequest getResourceSetRequest) throws InvalidOperationException,
InvalidInputException, ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a specific resource set.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourceSetRequest.Builder} avoiding the need to
* create one manually via {@link GetResourceSetRequest#builder()}
*
*
* @param getResourceSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetResourceSetRequest.Builder} to create a request.
* @return Result of the GetResourceSet operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetResourceSet
* @see AWS API
* Documentation
*/
default GetResourceSetResponse getResourceSet(Consumer getResourceSetRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return getResourceSet(GetResourceSetRequest.builder().applyMutation(getResourceSetRequest).build());
}
/**
*
* The onboarding status of a Firewall Manager admin account to third-party firewall vendor tenant.
*
*
* @param getThirdPartyFirewallAssociationStatusRequest
* @return Result of the GetThirdPartyFirewallAssociationStatus operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetThirdPartyFirewallAssociationStatus
* @see AWS API Documentation
*/
default GetThirdPartyFirewallAssociationStatusResponse getThirdPartyFirewallAssociationStatus(
GetThirdPartyFirewallAssociationStatusRequest getThirdPartyFirewallAssociationStatusRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* The onboarding status of a Firewall Manager admin account to third-party firewall vendor tenant.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetThirdPartyFirewallAssociationStatusRequest.Builder} avoiding the need to create one manually via
* {@link GetThirdPartyFirewallAssociationStatusRequest#builder()}
*
*
* @param getThirdPartyFirewallAssociationStatusRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetThirdPartyFirewallAssociationStatusRequest.Builder} to
* create a request.
* @return Result of the GetThirdPartyFirewallAssociationStatus operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetThirdPartyFirewallAssociationStatus
* @see AWS API Documentation
*/
default GetThirdPartyFirewallAssociationStatusResponse getThirdPartyFirewallAssociationStatus(
Consumer getThirdPartyFirewallAssociationStatusRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return getThirdPartyFirewallAssociationStatus(GetThirdPartyFirewallAssociationStatusRequest.builder()
.applyMutation(getThirdPartyFirewallAssociationStatusRequest).build());
}
/**
*
* Retrieves violations for a resource based on the specified Firewall Manager policy and Amazon Web Services
* account.
*
*
* @param getViolationDetailsRequest
* @return Result of the GetViolationDetails operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetViolationDetails
* @see AWS API
* Documentation
*/
default GetViolationDetailsResponse getViolationDetails(GetViolationDetailsRequest getViolationDetailsRequest)
throws ResourceNotFoundException, InvalidInputException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves violations for a resource based on the specified Firewall Manager policy and Amazon Web Services
* account.
*
*
*
* This is a convenience which creates an instance of the {@link GetViolationDetailsRequest.Builder} avoiding the
* need to create one manually via {@link GetViolationDetailsRequest#builder()}
*
*
* @param getViolationDetailsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.GetViolationDetailsRequest.Builder} to create a request.
* @return Result of the GetViolationDetails operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetViolationDetails
* @see AWS API
* Documentation
*/
default GetViolationDetailsResponse getViolationDetails(
Consumer getViolationDetailsRequest) throws ResourceNotFoundException,
InvalidInputException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return getViolationDetails(GetViolationDetailsRequest.builder().applyMutation(getViolationDetailsRequest).build());
}
/**
*
* Returns a AdminAccounts
object that lists the Firewall Manager administrators within the
* organization that are onboarded to Firewall Manager by AssociateAdminAccount.
*
*
* This operation can be called only from the organization's management account.
*
*
* @param listAdminAccountsForOrganizationRequest
* @return Result of the ListAdminAccountsForOrganization operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminAccountsForOrganization
* @see AWS API Documentation
*/
default ListAdminAccountsForOrganizationResponse listAdminAccountsForOrganization(
ListAdminAccountsForOrganizationRequest listAdminAccountsForOrganizationRequest) throws InvalidOperationException,
ResourceNotFoundException, InternalErrorException, LimitExceededException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a AdminAccounts
object that lists the Firewall Manager administrators within the
* organization that are onboarded to Firewall Manager by AssociateAdminAccount.
*
*
* This operation can be called only from the organization's management account.
*
*
*
* This is a convenience which creates an instance of the {@link ListAdminAccountsForOrganizationRequest.Builder}
* avoiding the need to create one manually via {@link ListAdminAccountsForOrganizationRequest#builder()}
*
*
* @param listAdminAccountsForOrganizationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest.Builder} to
* create a request.
* @return Result of the ListAdminAccountsForOrganization operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminAccountsForOrganization
* @see AWS API Documentation
*/
default ListAdminAccountsForOrganizationResponse listAdminAccountsForOrganization(
Consumer listAdminAccountsForOrganizationRequest)
throws InvalidOperationException, ResourceNotFoundException, InternalErrorException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
return listAdminAccountsForOrganization(ListAdminAccountsForOrganizationRequest.builder()
.applyMutation(listAdminAccountsForOrganizationRequest).build());
}
/**
*
* This is a variant of
* {@link #listAdminAccountsForOrganization(software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminAccountsForOrganizationIterable responses = client.listAdminAccountsForOrganizationPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListAdminAccountsForOrganizationIterable responses = client
* .listAdminAccountsForOrganizationPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminAccountsForOrganizationIterable responses = client.listAdminAccountsForOrganizationPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAdminAccountsForOrganization(software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest)}
* operation.
*
*
* @param listAdminAccountsForOrganizationRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminAccountsForOrganization
* @see AWS API Documentation
*/
default ListAdminAccountsForOrganizationIterable listAdminAccountsForOrganizationPaginator(
ListAdminAccountsForOrganizationRequest listAdminAccountsForOrganizationRequest) throws InvalidOperationException,
ResourceNotFoundException, InternalErrorException, LimitExceededException, AwsServiceException, SdkClientException,
FmsException {
return new ListAdminAccountsForOrganizationIterable(this, listAdminAccountsForOrganizationRequest);
}
/**
*
* This is a variant of
* {@link #listAdminAccountsForOrganization(software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminAccountsForOrganizationIterable responses = client.listAdminAccountsForOrganizationPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListAdminAccountsForOrganizationIterable responses = client
* .listAdminAccountsForOrganizationPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminAccountsForOrganizationIterable responses = client.listAdminAccountsForOrganizationPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAdminAccountsForOrganization(software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAdminAccountsForOrganizationRequest.Builder}
* avoiding the need to create one manually via {@link ListAdminAccountsForOrganizationRequest#builder()}
*
*
* @param listAdminAccountsForOrganizationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminAccountsForOrganization
* @see AWS API Documentation
*/
default ListAdminAccountsForOrganizationIterable listAdminAccountsForOrganizationPaginator(
Consumer listAdminAccountsForOrganizationRequest)
throws InvalidOperationException, ResourceNotFoundException, InternalErrorException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
return listAdminAccountsForOrganizationPaginator(ListAdminAccountsForOrganizationRequest.builder()
.applyMutation(listAdminAccountsForOrganizationRequest).build());
}
/**
*
* Lists the accounts that are managing the specified Organizations member account. This is useful for any member
* account so that they can view the accounts who are managing their account. This operation only returns the
* managing administrators that have the requested account within their AdminScope.
*
*
* @param listAdminsManagingAccountRequest
* @return Result of the ListAdminsManagingAccount operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminsManagingAccount
* @see AWS
* API Documentation
*/
default ListAdminsManagingAccountResponse listAdminsManagingAccount(
ListAdminsManagingAccountRequest listAdminsManagingAccountRequest) throws ResourceNotFoundException,
InvalidInputException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the accounts that are managing the specified Organizations member account. This is useful for any member
* account so that they can view the accounts who are managing their account. This operation only returns the
* managing administrators that have the requested account within their AdminScope.
*
*
*
* This is a convenience which creates an instance of the {@link ListAdminsManagingAccountRequest.Builder} avoiding
* the need to create one manually via {@link ListAdminsManagingAccountRequest#builder()}
*
*
* @param listAdminsManagingAccountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest.Builder} to create a
* request.
* @return Result of the ListAdminsManagingAccount operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminsManagingAccount
* @see AWS
* API Documentation
*/
default ListAdminsManagingAccountResponse listAdminsManagingAccount(
Consumer listAdminsManagingAccountRequest)
throws ResourceNotFoundException, InvalidInputException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return listAdminsManagingAccount(ListAdminsManagingAccountRequest.builder()
.applyMutation(listAdminsManagingAccountRequest).build());
}
/**
*
* This is a variant of
* {@link #listAdminsManagingAccount(software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminsManagingAccountIterable responses = client.listAdminsManagingAccountPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListAdminsManagingAccountIterable responses = client
* .listAdminsManagingAccountPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminsManagingAccountIterable responses = client.listAdminsManagingAccountPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAdminsManagingAccount(software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest)}
* operation.
*
*
* @param listAdminsManagingAccountRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminsManagingAccount
* @see AWS
* API Documentation
*/
default ListAdminsManagingAccountIterable listAdminsManagingAccountPaginator(
ListAdminsManagingAccountRequest listAdminsManagingAccountRequest) throws ResourceNotFoundException,
InvalidInputException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return new ListAdminsManagingAccountIterable(this, listAdminsManagingAccountRequest);
}
/**
*
* This is a variant of
* {@link #listAdminsManagingAccount(software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminsManagingAccountIterable responses = client.listAdminsManagingAccountPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListAdminsManagingAccountIterable responses = client
* .listAdminsManagingAccountPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAdminsManagingAccountIterable responses = client.listAdminsManagingAccountPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAdminsManagingAccount(software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAdminsManagingAccountRequest.Builder} avoiding
* the need to create one manually via {@link ListAdminsManagingAccountRequest#builder()}
*
*
* @param listAdminsManagingAccountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAdminsManagingAccount
* @see AWS
* API Documentation
*/
default ListAdminsManagingAccountIterable listAdminsManagingAccountPaginator(
Consumer listAdminsManagingAccountRequest)
throws ResourceNotFoundException, InvalidInputException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return listAdminsManagingAccountPaginator(ListAdminsManagingAccountRequest.builder()
.applyMutation(listAdminsManagingAccountRequest).build());
}
/**
*
* Returns an array of AppsListDataSummary
objects.
*
*
* @param listAppsListsRequest
* @return Result of the ListAppsLists operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAppsLists
* @see AWS API
* Documentation
*/
default ListAppsListsResponse listAppsLists(ListAppsListsRequest listAppsListsRequest) throws ResourceNotFoundException,
InvalidOperationException, LimitExceededException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of AppsListDataSummary
objects.
*
*
*
* This is a convenience which creates an instance of the {@link ListAppsListsRequest.Builder} avoiding the need to
* create one manually via {@link ListAppsListsRequest#builder()}
*
*
* @param listAppsListsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListAppsListsRequest.Builder} to create a request.
* @return Result of the ListAppsLists operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAppsLists
* @see AWS API
* Documentation
*/
default ListAppsListsResponse listAppsLists(Consumer listAppsListsRequest)
throws ResourceNotFoundException, InvalidOperationException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listAppsLists(ListAppsListsRequest.builder().applyMutation(listAppsListsRequest).build());
}
/**
*
* This is a variant of {@link #listAppsLists(software.amazon.awssdk.services.fms.model.ListAppsListsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAppsListsIterable responses = client.listAppsListsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListAppsListsIterable responses = client.listAppsListsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListAppsListsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAppsListsIterable responses = client.listAppsListsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAppsLists(software.amazon.awssdk.services.fms.model.ListAppsListsRequest)} operation.
*
*
* @param listAppsListsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAppsLists
* @see AWS API
* Documentation
*/
default ListAppsListsIterable listAppsListsPaginator(ListAppsListsRequest listAppsListsRequest)
throws ResourceNotFoundException, InvalidOperationException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return new ListAppsListsIterable(this, listAppsListsRequest);
}
/**
*
* This is a variant of {@link #listAppsLists(software.amazon.awssdk.services.fms.model.ListAppsListsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAppsListsIterable responses = client.listAppsListsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListAppsListsIterable responses = client.listAppsListsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListAppsListsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListAppsListsIterable responses = client.listAppsListsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAppsLists(software.amazon.awssdk.services.fms.model.ListAppsListsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAppsListsRequest.Builder} avoiding the need to
* create one manually via {@link ListAppsListsRequest#builder()}
*
*
* @param listAppsListsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListAppsListsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListAppsLists
* @see AWS API
* Documentation
*/
default ListAppsListsIterable listAppsListsPaginator(Consumer listAppsListsRequest)
throws ResourceNotFoundException, InvalidOperationException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listAppsListsPaginator(ListAppsListsRequest.builder().applyMutation(listAppsListsRequest).build());
}
/**
*
* Returns an array of PolicyComplianceStatus
objects. Use PolicyComplianceStatus
to get a
* summary of which member accounts are protected by the specified policy.
*
*
* @param listComplianceStatusRequest
* @return Result of the ListComplianceStatus operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusResponse listComplianceStatus(ListComplianceStatusRequest listComplianceStatusRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicyComplianceStatus
objects. Use PolicyComplianceStatus
to get a
* summary of which member accounts are protected by the specified policy.
*
*
*
* This is a convenience which creates an instance of the {@link ListComplianceStatusRequest.Builder} avoiding the
* need to create one manually via {@link ListComplianceStatusRequest#builder()}
*
*
* @param listComplianceStatusRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest.Builder} to create a request.
* @return Result of the ListComplianceStatus operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusResponse listComplianceStatus(
Consumer listComplianceStatusRequest) throws ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listComplianceStatus(ListComplianceStatusRequest.builder().applyMutation(listComplianceStatusRequest).build());
}
/**
*
* This is a variant of
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client
* .listComplianceStatusPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)}
* operation.
*
*
* @param listComplianceStatusRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusIterable listComplianceStatusPaginator(ListComplianceStatusRequest listComplianceStatusRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return new ListComplianceStatusIterable(this, listComplianceStatusRequest);
}
/**
*
* This is a variant of
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client
* .listComplianceStatusPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListComplianceStatusRequest.Builder} avoiding the
* need to create one manually via {@link ListComplianceStatusRequest#builder()}
*
*
* @param listComplianceStatusRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusIterable listComplianceStatusPaginator(
Consumer listComplianceStatusRequest) throws ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listComplianceStatusPaginator(ListComplianceStatusRequest.builder().applyMutation(listComplianceStatusRequest)
.build());
}
/**
*
* Returns an array of resources in the organization's accounts that are available to be associated with a resource
* set.
*
*
* @param listDiscoveredResourcesRequest
* @return Result of the ListDiscoveredResources operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListDiscoveredResources
* @see AWS
* API Documentation
*/
default ListDiscoveredResourcesResponse listDiscoveredResources(ListDiscoveredResourcesRequest listDiscoveredResourcesRequest)
throws InvalidOperationException, InvalidInputException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of resources in the organization's accounts that are available to be associated with a resource
* set.
*
*
*
* This is a convenience which creates an instance of the {@link ListDiscoveredResourcesRequest.Builder} avoiding
* the need to create one manually via {@link ListDiscoveredResourcesRequest#builder()}
*
*
* @param listDiscoveredResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListDiscoveredResourcesRequest.Builder} to create a
* request.
* @return Result of the ListDiscoveredResources operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListDiscoveredResources
* @see AWS
* API Documentation
*/
default ListDiscoveredResourcesResponse listDiscoveredResources(
Consumer listDiscoveredResourcesRequest) throws InvalidOperationException,
InvalidInputException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listDiscoveredResources(ListDiscoveredResourcesRequest.builder().applyMutation(listDiscoveredResourcesRequest)
.build());
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's Amazon Web
* Services organization.
*
*
* Either an Firewall Manager administrator or the organization's management account can make this request.
*
*
* @param listMemberAccountsRequest
* @return Result of the ListMemberAccounts operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsResponse listMemberAccounts(ListMemberAccountsRequest listMemberAccountsRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's Amazon Web
* Services organization.
*
*
* Either an Firewall Manager administrator or the organization's management account can make this request.
*
*
*
* This is a convenience which creates an instance of the {@link ListMemberAccountsRequest.Builder} avoiding the
* need to create one manually via {@link ListMemberAccountsRequest#builder()}
*
*
* @param listMemberAccountsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest.Builder} to create a request.
* @return Result of the ListMemberAccounts operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsResponse listMemberAccounts(Consumer listMemberAccountsRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listMemberAccounts(ListMemberAccountsRequest.builder().applyMutation(listMemberAccountsRequest).build());
}
/**
*
* This is a variant of
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client
* .listMemberAccountsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation.
*
*
* @param listMemberAccountsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsIterable listMemberAccountsPaginator(ListMemberAccountsRequest listMemberAccountsRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return new ListMemberAccountsIterable(this, listMemberAccountsRequest);
}
/**
*
* This is a variant of
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client
* .listMemberAccountsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListMemberAccountsRequest.Builder} avoiding the
* need to create one manually via {@link ListMemberAccountsRequest#builder()}
*
*
* @param listMemberAccountsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsIterable listMemberAccountsPaginator(
Consumer listMemberAccountsRequest) throws ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listMemberAccountsPaginator(ListMemberAccountsRequest.builder().applyMutation(listMemberAccountsRequest).build());
}
/**
*
* Returns an array of PolicySummary
objects.
*
*
* @param listPoliciesRequest
* @return Result of the ListPolicies operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesResponse listPolicies(ListPoliciesRequest listPoliciesRequest) throws ResourceNotFoundException,
InvalidOperationException, LimitExceededException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicySummary
objects.
*
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListPoliciesRequest.Builder} to create a request.
* @return Result of the ListPolicies operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesResponse listPolicies(Consumer listPoliciesRequest)
throws ResourceNotFoundException, InvalidOperationException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listPolicies(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)} operation.
*
*
* @param listPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesIterable listPoliciesPaginator(ListPoliciesRequest listPoliciesRequest) throws ResourceNotFoundException,
InvalidOperationException, LimitExceededException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
return new ListPoliciesIterable(this, listPoliciesRequest);
}
/**
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListPoliciesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesIterable listPoliciesPaginator(Consumer listPoliciesRequest)
throws ResourceNotFoundException, InvalidOperationException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listPoliciesPaginator(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* Returns an array of ProtocolsListDataSummary
objects.
*
*
* @param listProtocolsListsRequest
* @return Result of the ListProtocolsLists operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListProtocolsLists
* @see AWS API
* Documentation
*/
default ListProtocolsListsResponse listProtocolsLists(ListProtocolsListsRequest listProtocolsListsRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of ProtocolsListDataSummary
objects.
*
*
*
* This is a convenience which creates an instance of the {@link ListProtocolsListsRequest.Builder} avoiding the
* need to create one manually via {@link ListProtocolsListsRequest#builder()}
*
*
* @param listProtocolsListsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest.Builder} to create a request.
* @return Result of the ListProtocolsLists operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListProtocolsLists
* @see AWS API
* Documentation
*/
default ListProtocolsListsResponse listProtocolsLists(Consumer listProtocolsListsRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return listProtocolsLists(ListProtocolsListsRequest.builder().applyMutation(listProtocolsListsRequest).build());
}
/**
*
* This is a variant of
* {@link #listProtocolsLists(software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListProtocolsListsIterable responses = client.listProtocolsListsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListProtocolsListsIterable responses = client
* .listProtocolsListsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListProtocolsListsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListProtocolsListsIterable responses = client.listProtocolsListsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProtocolsLists(software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest)} operation.
*
*
* @param listProtocolsListsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListProtocolsLists
* @see AWS API
* Documentation
*/
default ListProtocolsListsIterable listProtocolsListsPaginator(ListProtocolsListsRequest listProtocolsListsRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return new ListProtocolsListsIterable(this, listProtocolsListsRequest);
}
/**
*
* This is a variant of
* {@link #listProtocolsLists(software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListProtocolsListsIterable responses = client.listProtocolsListsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListProtocolsListsIterable responses = client
* .listProtocolsListsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListProtocolsListsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListProtocolsListsIterable responses = client.listProtocolsListsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProtocolsLists(software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListProtocolsListsRequest.Builder} avoiding the
* need to create one manually via {@link ListProtocolsListsRequest#builder()}
*
*
* @param listProtocolsListsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListProtocolsLists
* @see AWS API
* Documentation
*/
default ListProtocolsListsIterable listProtocolsListsPaginator(
Consumer listProtocolsListsRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listProtocolsListsPaginator(ListProtocolsListsRequest.builder().applyMutation(listProtocolsListsRequest).build());
}
/**
*
* Returns an array of resources that are currently associated to a resource set.
*
*
* @param listResourceSetResourcesRequest
* @return Result of the ListResourceSetResources operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListResourceSetResources
* @see AWS
* API Documentation
*/
default ListResourceSetResourcesResponse listResourceSetResources(
ListResourceSetResourcesRequest listResourceSetResourcesRequest) throws InvalidOperationException,
InternalErrorException, InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of resources that are currently associated to a resource set.
*
*
*
* This is a convenience which creates an instance of the {@link ListResourceSetResourcesRequest.Builder} avoiding
* the need to create one manually via {@link ListResourceSetResourcesRequest#builder()}
*
*
* @param listResourceSetResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListResourceSetResourcesRequest.Builder} to create a
* request.
* @return Result of the ListResourceSetResources operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListResourceSetResources
* @see AWS
* API Documentation
*/
default ListResourceSetResourcesResponse listResourceSetResources(
Consumer listResourceSetResourcesRequest) throws InvalidOperationException,
InternalErrorException, InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException,
FmsException {
return listResourceSetResources(ListResourceSetResourcesRequest.builder().applyMutation(listResourceSetResourcesRequest)
.build());
}
/**
*
* Returns an array of ResourceSetSummary
objects.
*
*
* @param listResourceSetsRequest
* @return Result of the ListResourceSets operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListResourceSets
* @see AWS API
* Documentation
*/
default ListResourceSetsResponse listResourceSets(ListResourceSetsRequest listResourceSetsRequest)
throws InvalidOperationException, InvalidInputException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of ResourceSetSummary
objects.
*
*
*
* This is a convenience which creates an instance of the {@link ListResourceSetsRequest.Builder} avoiding the need
* to create one manually via {@link ListResourceSetsRequest#builder()}
*
*
* @param listResourceSetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListResourceSetsRequest.Builder} to create a request.
* @return Result of the ListResourceSets operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListResourceSets
* @see AWS API
* Documentation
*/
default ListResourceSetsResponse listResourceSets(Consumer listResourceSetsRequest)
throws InvalidOperationException, InvalidInputException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return listResourceSets(ListResourceSetsRequest.builder().applyMutation(listResourceSetsRequest).build());
}
/**
*
* Retrieves the list of tags for the specified Amazon Web Services resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, InvalidInputException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the list of tags for the specified Amazon Web Services resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListTagsForResourceRequest.Builder} to create a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidInputException, AwsServiceException, SdkClientException,
FmsException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Retrieves a list of all of the third-party firewall policies that are associated with the third-party firewall
* administrator's account.
*
*
* @param listThirdPartyFirewallFirewallPoliciesRequest
* @return Result of the ListThirdPartyFirewallFirewallPolicies operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListThirdPartyFirewallFirewallPolicies
* @see AWS API Documentation
*/
default ListThirdPartyFirewallFirewallPoliciesResponse listThirdPartyFirewallFirewallPolicies(
ListThirdPartyFirewallFirewallPoliciesRequest listThirdPartyFirewallFirewallPoliciesRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of all of the third-party firewall policies that are associated with the third-party firewall
* administrator's account.
*
*
*
* This is a convenience which creates an instance of the
* {@link ListThirdPartyFirewallFirewallPoliciesRequest.Builder} avoiding the need to create one manually via
* {@link ListThirdPartyFirewallFirewallPoliciesRequest#builder()}
*
*
* @param listThirdPartyFirewallFirewallPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest.Builder} to
* create a request.
* @return Result of the ListThirdPartyFirewallFirewallPolicies operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListThirdPartyFirewallFirewallPolicies
* @see AWS API Documentation
*/
default ListThirdPartyFirewallFirewallPoliciesResponse listThirdPartyFirewallFirewallPolicies(
Consumer listThirdPartyFirewallFirewallPoliciesRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listThirdPartyFirewallFirewallPolicies(ListThirdPartyFirewallFirewallPoliciesRequest.builder()
.applyMutation(listThirdPartyFirewallFirewallPoliciesRequest).build());
}
/**
*
* This is a variant of
* {@link #listThirdPartyFirewallFirewallPolicies(software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListThirdPartyFirewallFirewallPoliciesIterable responses = client.listThirdPartyFirewallFirewallPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListThirdPartyFirewallFirewallPoliciesIterable responses = client
* .listThirdPartyFirewallFirewallPoliciesPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListThirdPartyFirewallFirewallPoliciesIterable responses = client.listThirdPartyFirewallFirewallPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listThirdPartyFirewallFirewallPolicies(software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest)}
* operation.
*
*
* @param listThirdPartyFirewallFirewallPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListThirdPartyFirewallFirewallPolicies
* @see AWS API Documentation
*/
default ListThirdPartyFirewallFirewallPoliciesIterable listThirdPartyFirewallFirewallPoliciesPaginator(
ListThirdPartyFirewallFirewallPoliciesRequest listThirdPartyFirewallFirewallPoliciesRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return new ListThirdPartyFirewallFirewallPoliciesIterable(this, listThirdPartyFirewallFirewallPoliciesRequest);
}
/**
*
* This is a variant of
* {@link #listThirdPartyFirewallFirewallPolicies(software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListThirdPartyFirewallFirewallPoliciesIterable responses = client.listThirdPartyFirewallFirewallPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListThirdPartyFirewallFirewallPoliciesIterable responses = client
* .listThirdPartyFirewallFirewallPoliciesPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListThirdPartyFirewallFirewallPoliciesIterable responses = client.listThirdPartyFirewallFirewallPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listThirdPartyFirewallFirewallPolicies(software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the
* {@link ListThirdPartyFirewallFirewallPoliciesRequest.Builder} avoiding the need to create one manually via
* {@link ListThirdPartyFirewallFirewallPoliciesRequest#builder()}
*
*
* @param listThirdPartyFirewallFirewallPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListThirdPartyFirewallFirewallPolicies
* @see AWS API Documentation
*/
default ListThirdPartyFirewallFirewallPoliciesIterable listThirdPartyFirewallFirewallPoliciesPaginator(
Consumer listThirdPartyFirewallFirewallPoliciesRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listThirdPartyFirewallFirewallPoliciesPaginator(ListThirdPartyFirewallFirewallPoliciesRequest.builder()
.applyMutation(listThirdPartyFirewallFirewallPoliciesRequest).build());
}
/**
*
* Creates or updates an Firewall Manager administrator account. The account must be a member of the organization
* that was onboarded to Firewall Manager by AssociateAdminAccount. Only the organization's management
* account can create an Firewall Manager administrator account. When you create an Firewall Manager administrator
* account, the service checks to see if the account is already a delegated administrator within Organizations. If
* the account isn't a delegated administrator, Firewall Manager calls Organizations to delegate the account within
* Organizations. For more information about administrator accounts within Organizations, see Managing the Amazon
* Web Services Accounts in Your Organization.
*
*
* @param putAdminAccountRequest
* @return Result of the PutAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutAdminAccount
* @see AWS API
* Documentation
*/
default PutAdminAccountResponse putAdminAccount(PutAdminAccountRequest putAdminAccountRequest)
throws InvalidOperationException, InvalidInputException, InternalErrorException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates an Firewall Manager administrator account. The account must be a member of the organization
* that was onboarded to Firewall Manager by AssociateAdminAccount. Only the organization's management
* account can create an Firewall Manager administrator account. When you create an Firewall Manager administrator
* account, the service checks to see if the account is already a delegated administrator within Organizations. If
* the account isn't a delegated administrator, Firewall Manager calls Organizations to delegate the account within
* Organizations. For more information about administrator accounts within Organizations, see Managing the Amazon
* Web Services Accounts in Your Organization.
*
*
*
* This is a convenience which creates an instance of the {@link PutAdminAccountRequest.Builder} avoiding the need
* to create one manually via {@link PutAdminAccountRequest#builder()}
*
*
* @param putAdminAccountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.PutAdminAccountRequest.Builder} to create a request.
* @return Result of the PutAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutAdminAccount
* @see AWS API
* Documentation
*/
default PutAdminAccountResponse putAdminAccount(Consumer putAdminAccountRequest)
throws InvalidOperationException, InvalidInputException, InternalErrorException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
return putAdminAccount(PutAdminAccountRequest.builder().applyMutation(putAdminAccountRequest).build());
}
/**
*
* Creates an Firewall Manager applications list.
*
*
* @param putAppsListRequest
* @return Result of the PutAppsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutAppsList
* @see AWS API
* Documentation
*/
default PutAppsListResponse putAppsList(PutAppsListRequest putAppsListRequest) throws ResourceNotFoundException,
InvalidOperationException, InvalidInputException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Firewall Manager applications list.
*
*
*
* This is a convenience which creates an instance of the {@link PutAppsListRequest.Builder} avoiding the need to
* create one manually via {@link PutAppsListRequest#builder()}
*
*
* @param putAppsListRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.PutAppsListRequest.Builder} to create a request.
* @return Result of the PutAppsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutAppsList
* @see AWS API
* Documentation
*/
default PutAppsListResponse putAppsList(Consumer putAppsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InvalidInputException, LimitExceededException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return putAppsList(PutAppsListRequest.builder().applyMutation(putAppsListRequest).build());
}
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that Firewall Manager uses to record
* SNS logs.
*
*
* To perform this action outside of the console, you must first configure the SNS topic's access policy to allow
* the SnsRoleName
to publish SNS logs. If the SnsRoleName
provided is a role other than
* the AWSServiceRoleForFMS
service-linked role, this role must have a trust relationship configured to
* allow the Firewall Manager service principal fms.amazonaws.com
to assume this role. For information
* about configuring an SNS access policy, see Service roles for Firewall Manager in the Firewall Manager Developer Guide.
*
*
* @param putNotificationChannelRequest
* @return Result of the PutNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutNotificationChannel
* @see AWS
* API Documentation
*/
default PutNotificationChannelResponse putNotificationChannel(PutNotificationChannelRequest putNotificationChannelRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that Firewall Manager uses to record
* SNS logs.
*
*
* To perform this action outside of the console, you must first configure the SNS topic's access policy to allow
* the SnsRoleName
to publish SNS logs. If the SnsRoleName
provided is a role other than
* the AWSServiceRoleForFMS
service-linked role, this role must have a trust relationship configured to
* allow the Firewall Manager service principal fms.amazonaws.com
to assume this role. For information
* about configuring an SNS access policy, see Service roles for Firewall Manager in the Firewall Manager Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutNotificationChannelRequest.Builder} avoiding the
* need to create one manually via {@link PutNotificationChannelRequest#builder()}
*
*
* @param putNotificationChannelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.PutNotificationChannelRequest.Builder} to create a
* request.
* @return Result of the PutNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutNotificationChannel
* @see AWS
* API Documentation
*/
default PutNotificationChannelResponse putNotificationChannel(
Consumer putNotificationChannelRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return putNotificationChannel(PutNotificationChannelRequest.builder().applyMutation(putNotificationChannelRequest)
.build());
}
/**
*
* Creates an Firewall Manager policy.
*
*
* A Firewall Manager policy is specific to the individual policy type. If you want to enforce multiple policy types
* across accounts, you can create multiple policies. You can create more than one policy for each type.
*
*
* If you add a new account to an organization that you created with Organizations, Firewall Manager automatically
* applies the policy to the resources in that account that are within scope of the policy.
*
*
* Firewall Manager provides the following types of policies:
*
*
* -
*
* WAF policy - This policy applies WAF web ACL protections to specified accounts and resources.
*
*
* -
*
* Shield Advanced policy - This policy applies Shield Advanced protection to specified accounts and
* resources.
*
*
* -
*
* Security Groups policy - This type of policy gives you control over security groups that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of rules across your
* organization.
*
*
* -
*
* Network ACL policy - This type of policy gives you control over the network ACLs that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of first and last network ACL
* rules across your organization.
*
*
* -
*
* Network Firewall policy - This policy applies Network Firewall protection to your organization's VPCs.
*
*
* -
*
* DNS Firewall policy - This policy applies Amazon Route 53 Resolver DNS Firewall protections to your
* organization's VPCs.
*
*
* -
*
* Third-party firewall policy - This policy applies third-party firewall protections. Third-party firewalls
* are available by subscription through the Amazon Web Services Marketplace console at Amazon Web Services Marketplace.
*
*
* -
*
* Palo Alto Networks Cloud NGFW policy - This policy applies Palo Alto Networks Cloud Next Generation
* Firewall (NGFW) protections and Palo Alto Networks Cloud NGFW rulestacks to your organization's VPCs.
*
*
* -
*
* Fortigate CNF policy - This policy applies Fortigate Cloud Native Firewall (CNF) protections. Fortigate
* CNF is a cloud-centered solution that blocks Zero-Day threats and secures cloud infrastructures with
* industry-leading advanced threat prevention, smart web application firewalls (WAF), and API protection.
*
*
*
*
*
*
* @param putPolicyRequest
* @return Result of the PutPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutPolicy
* @see AWS API
* Documentation
*/
default PutPolicyResponse putPolicy(PutPolicyRequest putPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InvalidInputException, LimitExceededException, InternalErrorException,
InvalidTypeException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Firewall Manager policy.
*
*
* A Firewall Manager policy is specific to the individual policy type. If you want to enforce multiple policy types
* across accounts, you can create multiple policies. You can create more than one policy for each type.
*
*
* If you add a new account to an organization that you created with Organizations, Firewall Manager automatically
* applies the policy to the resources in that account that are within scope of the policy.
*
*
* Firewall Manager provides the following types of policies:
*
*
* -
*
* WAF policy - This policy applies WAF web ACL protections to specified accounts and resources.
*
*
* -
*
* Shield Advanced policy - This policy applies Shield Advanced protection to specified accounts and
* resources.
*
*
* -
*
* Security Groups policy - This type of policy gives you control over security groups that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of rules across your
* organization.
*
*
* -
*
* Network ACL policy - This type of policy gives you control over the network ACLs that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of first and last network ACL
* rules across your organization.
*
*
* -
*
* Network Firewall policy - This policy applies Network Firewall protection to your organization's VPCs.
*
*
* -
*
* DNS Firewall policy - This policy applies Amazon Route 53 Resolver DNS Firewall protections to your
* organization's VPCs.
*
*
* -
*
* Third-party firewall policy - This policy applies third-party firewall protections. Third-party firewalls
* are available by subscription through the Amazon Web Services Marketplace console at Amazon Web Services Marketplace.
*
*
* -
*
* Palo Alto Networks Cloud NGFW policy - This policy applies Palo Alto Networks Cloud Next Generation
* Firewall (NGFW) protections and Palo Alto Networks Cloud NGFW rulestacks to your organization's VPCs.
*
*
* -
*
* Fortigate CNF policy - This policy applies Fortigate Cloud Native Firewall (CNF) protections. Fortigate
* CNF is a cloud-centered solution that blocks Zero-Day threats and secures cloud infrastructures with
* industry-leading advanced threat prevention, smart web application firewalls (WAF), and API protection.
*
*
*
*
*
*
*
* This is a convenience which creates an instance of the {@link PutPolicyRequest.Builder} avoiding the need to
* create one manually via {@link PutPolicyRequest#builder()}
*
*
* @param putPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.PutPolicyRequest.Builder} to create a request.
* @return Result of the PutPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutPolicy
* @see AWS API
* Documentation
*/
default PutPolicyResponse putPolicy(Consumer putPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InvalidInputException, LimitExceededException, InternalErrorException,
InvalidTypeException, AwsServiceException, SdkClientException, FmsException {
return putPolicy(PutPolicyRequest.builder().applyMutation(putPolicyRequest).build());
}
/**
*
* Creates an Firewall Manager protocols list.
*
*
* @param putProtocolsListRequest
* @return Result of the PutProtocolsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutProtocolsList
* @see AWS API
* Documentation
*/
default PutProtocolsListResponse putProtocolsList(PutProtocolsListRequest putProtocolsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InvalidInputException, LimitExceededException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Firewall Manager protocols list.
*
*
*
* This is a convenience which creates an instance of the {@link PutProtocolsListRequest.Builder} avoiding the need
* to create one manually via {@link PutProtocolsListRequest#builder()}
*
*
* @param putProtocolsListRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.PutProtocolsListRequest.Builder} to create a request.
* @return Result of the PutProtocolsList operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutProtocolsList
* @see AWS API
* Documentation
*/
default PutProtocolsListResponse putProtocolsList(Consumer putProtocolsListRequest)
throws ResourceNotFoundException, InvalidOperationException, InvalidInputException, LimitExceededException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return putProtocolsList(PutProtocolsListRequest.builder().applyMutation(putProtocolsListRequest).build());
}
/**
*
* Creates the resource set.
*
*
* An Firewall Manager resource set defines the resources to import into an Firewall Manager policy from another
* Amazon Web Services service.
*
*
* @param putResourceSetRequest
* @return Result of the PutResourceSet operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutResourceSet
* @see AWS API
* Documentation
*/
default PutResourceSetResponse putResourceSet(PutResourceSetRequest putResourceSetRequest) throws InvalidOperationException,
InvalidInputException, LimitExceededException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates the resource set.
*
*
* An Firewall Manager resource set defines the resources to import into an Firewall Manager policy from another
* Amazon Web Services service.
*
*
*
* This is a convenience which creates an instance of the {@link PutResourceSetRequest.Builder} avoiding the need to
* create one manually via {@link PutResourceSetRequest#builder()}
*
*
* @param putResourceSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.PutResourceSetRequest.Builder} to create a request.
* @return Result of the PutResourceSet operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutResourceSet
* @see AWS API
* Documentation
*/
default PutResourceSetResponse putResourceSet(Consumer putResourceSetRequest)
throws InvalidOperationException, InvalidInputException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return putResourceSet(PutResourceSetRequest.builder().applyMutation(putResourceSetRequest).build());
}
/**
*
* Adds one or more tags to an Amazon Web Services resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidInputException, LimitExceededException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an Amazon Web Services resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an Amazon Web Services account. For more information, see Firewall Manager Limits
* in the WAF Developer Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, InvalidInputException,
LimitExceededException, AwsServiceException, SdkClientException, FmsException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes one or more tags from an Amazon Web Services resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidInputException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Removes one or more tags from an Amazon Web Services resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fms.model.UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do or the operation wasn't possible. For example, you
* might have submitted an AssociateAdminAccount
request for an account ID that was already set
* as the Firewall Manager administrator. Or you might have tried to access a Region that's disabled by
* default, and that you need to enable for the Firewall Manager administrator account and for Organizations
* before you can access it.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, InvalidInputException,
AwsServiceException, SdkClientException, FmsException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
* Create a {@link FmsClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static FmsClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link FmsClient}.
*/
static FmsClientBuilder builder() {
return new DefaultFmsClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default FmsServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}