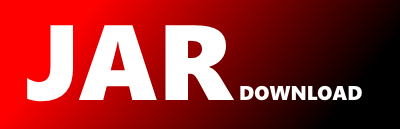
software.amazon.awssdk.services.fms.model.EntryDescription Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a single rule in a network ACL.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EntryDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENTRY_DETAIL_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("EntryDetail")
.getter(getter(EntryDescription::entryDetail)).setter(setter(Builder::entryDetail))
.constructor(NetworkAclEntry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EntryDetail").build()).build();
private static final SdkField ENTRY_RULE_NUMBER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EntryRuleNumber").getter(getter(EntryDescription::entryRuleNumber))
.setter(setter(Builder::entryRuleNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EntryRuleNumber").build()).build();
private static final SdkField ENTRY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EntryType").getter(getter(EntryDescription::entryTypeAsString)).setter(setter(Builder::entryType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EntryType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENTRY_DETAIL_FIELD,
ENTRY_RULE_NUMBER_FIELD, ENTRY_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("EntryDetail", ENTRY_DETAIL_FIELD);
put("EntryRuleNumber", ENTRY_RULE_NUMBER_FIELD);
put("EntryType", ENTRY_TYPE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final NetworkAclEntry entryDetail;
private final Integer entryRuleNumber;
private final String entryType;
private EntryDescription(BuilderImpl builder) {
this.entryDetail = builder.entryDetail;
this.entryRuleNumber = builder.entryRuleNumber;
this.entryType = builder.entryType;
}
/**
*
* Describes a rule in a network ACL.
*
*
* Each network ACL has a set of numbered ingress rules and a separate set of numbered egress rules. When
* determining whether a packet should be allowed in or out of a subnet associated with the network ACL, Amazon Web
* Services processes the entries in the network ACL according to the rule numbers, in ascending order.
*
*
* When you manage an individual network ACL, you explicitly specify the rule numbers. When you specify the network
* ACL rules in a Firewall Manager policy, you provide the rules to run first, in the order that you want them to
* run, and the rules to run last, in the order that you want them to run. Firewall Manager assigns the rule numbers
* for you when you save the network ACL policy specification.
*
*
* @return Describes a rule in a network ACL.
*
* Each network ACL has a set of numbered ingress rules and a separate set of numbered egress rules. When
* determining whether a packet should be allowed in or out of a subnet associated with the network ACL,
* Amazon Web Services processes the entries in the network ACL according to the rule numbers, in ascending
* order.
*
*
* When you manage an individual network ACL, you explicitly specify the rule numbers. When you specify the
* network ACL rules in a Firewall Manager policy, you provide the rules to run first, in the order that you
* want them to run, and the rules to run last, in the order that you want them to run. Firewall Manager
* assigns the rule numbers for you when you save the network ACL policy specification.
*/
public final NetworkAclEntry entryDetail() {
return entryDetail;
}
/**
*
* The rule number for the entry. ACL entries are processed in ascending order by rule number. In a Firewall Manager
* network ACL policy, Firewall Manager assigns rule numbers.
*
*
* @return The rule number for the entry. ACL entries are processed in ascending order by rule number. In a Firewall
* Manager network ACL policy, Firewall Manager assigns rule numbers.
*/
public final Integer entryRuleNumber() {
return entryRuleNumber;
}
/**
*
* Specifies whether the entry is managed by Firewall Manager or by a user, and, for Firewall Manager-managed
* entries, specifies whether the entry is among those that run first in the network ACL or those that run last.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #entryType} will
* return {@link EntryType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #entryTypeAsString}.
*
*
* @return Specifies whether the entry is managed by Firewall Manager or by a user, and, for Firewall
* Manager-managed entries, specifies whether the entry is among those that run first in the network ACL or
* those that run last.
* @see EntryType
*/
public final EntryType entryType() {
return EntryType.fromValue(entryType);
}
/**
*
* Specifies whether the entry is managed by Firewall Manager or by a user, and, for Firewall Manager-managed
* entries, specifies whether the entry is among those that run first in the network ACL or those that run last.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #entryType} will
* return {@link EntryType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #entryTypeAsString}.
*
*
* @return Specifies whether the entry is managed by Firewall Manager or by a user, and, for Firewall
* Manager-managed entries, specifies whether the entry is among those that run first in the network ACL or
* those that run last.
* @see EntryType
*/
public final String entryTypeAsString() {
return entryType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(entryDetail());
hashCode = 31 * hashCode + Objects.hashCode(entryRuleNumber());
hashCode = 31 * hashCode + Objects.hashCode(entryTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EntryDescription)) {
return false;
}
EntryDescription other = (EntryDescription) obj;
return Objects.equals(entryDetail(), other.entryDetail()) && Objects.equals(entryRuleNumber(), other.entryRuleNumber())
&& Objects.equals(entryTypeAsString(), other.entryTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EntryDescription").add("EntryDetail", entryDetail()).add("EntryRuleNumber", entryRuleNumber())
.add("EntryType", entryTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EntryDetail":
return Optional.ofNullable(clazz.cast(entryDetail()));
case "EntryRuleNumber":
return Optional.ofNullable(clazz.cast(entryRuleNumber()));
case "EntryType":
return Optional.ofNullable(clazz.cast(entryTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function