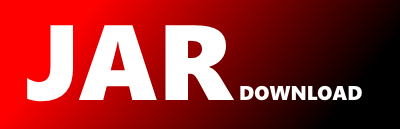
software.amazon.awssdk.services.fms.model.GetProtectionStatusResponse Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetProtectionStatusResponse extends FmsResponse implements
ToCopyableBuilder {
private static final SdkField ADMIN_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdminAccountId").getter(getter(GetProtectionStatusResponse::adminAccountId))
.setter(setter(Builder::adminAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdminAccountId").build()).build();
private static final SdkField SERVICE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceType").getter(getter(GetProtectionStatusResponse::serviceTypeAsString))
.setter(setter(Builder::serviceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceType").build()).build();
private static final SdkField DATA_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Data")
.getter(getter(GetProtectionStatusResponse::data)).setter(setter(Builder::data))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Data").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextToken").getter(getter(GetProtectionStatusResponse::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ADMIN_ACCOUNT_ID_FIELD,
SERVICE_TYPE_FIELD, DATA_FIELD, NEXT_TOKEN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AdminAccountId", ADMIN_ACCOUNT_ID_FIELD);
put("ServiceType", SERVICE_TYPE_FIELD);
put("Data", DATA_FIELD);
put("NextToken", NEXT_TOKEN_FIELD);
}
});
private final String adminAccountId;
private final String serviceType;
private final String data;
private final String nextToken;
private GetProtectionStatusResponse(BuilderImpl builder) {
super(builder);
this.adminAccountId = builder.adminAccountId;
this.serviceType = builder.serviceType;
this.data = builder.data;
this.nextToken = builder.nextToken;
}
/**
*
* The ID of the Firewall Manager administrator account for this policy.
*
*
* @return The ID of the Firewall Manager administrator account for this policy.
*/
public final String adminAccountId() {
return adminAccountId;
}
/**
*
* The service type that is protected by the policy. Currently, this is always SHIELD_ADVANCED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceType} will
* return {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceTypeAsString}.
*
*
* @return The service type that is protected by the policy. Currently, this is always SHIELD_ADVANCED
.
* @see SecurityServiceType
*/
public final SecurityServiceType serviceType() {
return SecurityServiceType.fromValue(serviceType);
}
/**
*
* The service type that is protected by the policy. Currently, this is always SHIELD_ADVANCED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceType} will
* return {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceTypeAsString}.
*
*
* @return The service type that is protected by the policy. Currently, this is always SHIELD_ADVANCED
.
* @see SecurityServiceType
*/
public final String serviceTypeAsString() {
return serviceType;
}
/**
*
* Details about the attack, including the following:
*
*
* -
*
* Attack type
*
*
* -
*
* Account ID
*
*
* -
*
* ARN of the resource attacked
*
*
* -
*
* Start time of the attack
*
*
* -
*
* End time of the attack (ongoing attacks will not have an end time)
*
*
*
*
* The details are in JSON format.
*
*
* @return Details about the attack, including the following:
*
* -
*
* Attack type
*
*
* -
*
* Account ID
*
*
* -
*
* ARN of the resource attacked
*
*
* -
*
* Start time of the attack
*
*
* -
*
* End time of the attack (ongoing attacks will not have an end time)
*
*
*
*
* The details are in JSON format.
*/
public final String data() {
return data;
}
/**
*
* If you have more objects than the number that you specified for MaxResults
in the request, the
* response includes a NextToken
value. To list more objects, submit another
* GetProtectionStatus
request, and specify the NextToken
value from the response in the
* NextToken
value in the next request.
*
*
* Amazon Web Services SDKs provide auto-pagination that identify NextToken
in a response and make
* subsequent request calls automatically on your behalf. However, this feature is not supported by
* GetProtectionStatus
. You must submit subsequent requests with NextToken
using your own
* processes.
*
*
* @return If you have more objects than the number that you specified for MaxResults
in the request,
* the response includes a NextToken
value. To list more objects, submit another
* GetProtectionStatus
request, and specify the NextToken
value from the response
* in the NextToken
value in the next request.
*
* Amazon Web Services SDKs provide auto-pagination that identify NextToken
in a response and
* make subsequent request calls automatically on your behalf. However, this feature is not supported by
* GetProtectionStatus
. You must submit subsequent requests with NextToken
using
* your own processes.
*/
public final String nextToken() {
return nextToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(adminAccountId());
hashCode = 31 * hashCode + Objects.hashCode(serviceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(data());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetProtectionStatusResponse)) {
return false;
}
GetProtectionStatusResponse other = (GetProtectionStatusResponse) obj;
return Objects.equals(adminAccountId(), other.adminAccountId())
&& Objects.equals(serviceTypeAsString(), other.serviceTypeAsString()) && Objects.equals(data(), other.data())
&& Objects.equals(nextToken(), other.nextToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetProtectionStatusResponse").add("AdminAccountId", adminAccountId())
.add("ServiceType", serviceTypeAsString()).add("Data", data()).add("NextToken", nextToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AdminAccountId":
return Optional.ofNullable(clazz.cast(adminAccountId()));
case "ServiceType":
return Optional.ofNullable(clazz.cast(serviceTypeAsString()));
case "Data":
return Optional.ofNullable(clazz.cast(data()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* -
*
* Attack type
*
*
* -
*
* Account ID
*
*
* -
*
* ARN of the resource attacked
*
*
* -
*
* Start time of the attack
*
*
* -
*
* End time of the attack (ongoing attacks will not have an end time)
*
*
*
*
* The details are in JSON format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder data(String data);
/**
*
* If you have more objects than the number that you specified for MaxResults
in the request, the
* response includes a NextToken
value. To list more objects, submit another
* GetProtectionStatus
request, and specify the NextToken
value from the response in
* the NextToken
value in the next request.
*
*
* Amazon Web Services SDKs provide auto-pagination that identify NextToken
in a response and make
* subsequent request calls automatically on your behalf. However, this feature is not supported by
* GetProtectionStatus
. You must submit subsequent requests with NextToken
using your
* own processes.
*
*
* @param nextToken
* If you have more objects than the number that you specified for MaxResults
in the
* request, the response includes a NextToken
value. To list more objects, submit another
* GetProtectionStatus
request, and specify the NextToken
value from the
* response in the NextToken
value in the next request.
*
* Amazon Web Services SDKs provide auto-pagination that identify NextToken
in a response
* and make subsequent request calls automatically on your behalf. However, this feature is not supported
* by GetProtectionStatus
. You must submit subsequent requests with NextToken
* using your own processes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nextToken(String nextToken);
}
static final class BuilderImpl extends FmsResponse.BuilderImpl implements Builder {
private String adminAccountId;
private String serviceType;
private String data;
private String nextToken;
private BuilderImpl() {
}
private BuilderImpl(GetProtectionStatusResponse model) {
super(model);
adminAccountId(model.adminAccountId);
serviceType(model.serviceType);
data(model.data);
nextToken(model.nextToken);
}
public final String getAdminAccountId() {
return adminAccountId;
}
public final void setAdminAccountId(String adminAccountId) {
this.adminAccountId = adminAccountId;
}
@Override
public final Builder adminAccountId(String adminAccountId) {
this.adminAccountId = adminAccountId;
return this;
}
public final String getServiceType() {
return serviceType;
}
public final void setServiceType(String serviceType) {
this.serviceType = serviceType;
}
@Override
public final Builder serviceType(String serviceType) {
this.serviceType = serviceType;
return this;
}
@Override
public final Builder serviceType(SecurityServiceType serviceType) {
this.serviceType(serviceType == null ? null : serviceType.toString());
return this;
}
public final String getData() {
return data;
}
public final void setData(String data) {
this.data = data;
}
@Override
public final Builder data(String data) {
this.data = data;
return this;
}
public final String getNextToken() {
return nextToken;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
@Override
public GetProtectionStatusResponse build() {
return new GetProtectionStatusResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}