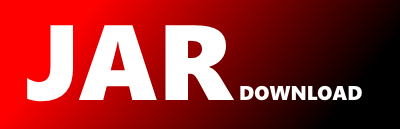
software.amazon.awssdk.services.fms.DefaultFmsAsyncClient Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.fms.internal.FmsServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.AssociateThirdPartyFirewallRequest;
import software.amazon.awssdk.services.fms.model.AssociateThirdPartyFirewallResponse;
import software.amazon.awssdk.services.fms.model.BatchAssociateResourceRequest;
import software.amazon.awssdk.services.fms.model.BatchAssociateResourceResponse;
import software.amazon.awssdk.services.fms.model.BatchDisassociateResourceRequest;
import software.amazon.awssdk.services.fms.model.BatchDisassociateResourceResponse;
import software.amazon.awssdk.services.fms.model.DeleteAppsListRequest;
import software.amazon.awssdk.services.fms.model.DeleteAppsListResponse;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.DeletePolicyRequest;
import software.amazon.awssdk.services.fms.model.DeletePolicyResponse;
import software.amazon.awssdk.services.fms.model.DeleteProtocolsListRequest;
import software.amazon.awssdk.services.fms.model.DeleteProtocolsListResponse;
import software.amazon.awssdk.services.fms.model.DeleteResourceSetRequest;
import software.amazon.awssdk.services.fms.model.DeleteResourceSetResponse;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.DisassociateThirdPartyFirewallRequest;
import software.amazon.awssdk.services.fms.model.DisassociateThirdPartyFirewallResponse;
import software.amazon.awssdk.services.fms.model.FmsException;
import software.amazon.awssdk.services.fms.model.GetAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.GetAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.GetAdminScopeRequest;
import software.amazon.awssdk.services.fms.model.GetAdminScopeResponse;
import software.amazon.awssdk.services.fms.model.GetAppsListRequest;
import software.amazon.awssdk.services.fms.model.GetAppsListResponse;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailRequest;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailResponse;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.GetPolicyRequest;
import software.amazon.awssdk.services.fms.model.GetPolicyResponse;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusRequest;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusResponse;
import software.amazon.awssdk.services.fms.model.GetProtocolsListRequest;
import software.amazon.awssdk.services.fms.model.GetProtocolsListResponse;
import software.amazon.awssdk.services.fms.model.GetResourceSetRequest;
import software.amazon.awssdk.services.fms.model.GetResourceSetResponse;
import software.amazon.awssdk.services.fms.model.GetThirdPartyFirewallAssociationStatusRequest;
import software.amazon.awssdk.services.fms.model.GetThirdPartyFirewallAssociationStatusResponse;
import software.amazon.awssdk.services.fms.model.GetViolationDetailsRequest;
import software.amazon.awssdk.services.fms.model.GetViolationDetailsResponse;
import software.amazon.awssdk.services.fms.model.InternalErrorException;
import software.amazon.awssdk.services.fms.model.InvalidInputException;
import software.amazon.awssdk.services.fms.model.InvalidOperationException;
import software.amazon.awssdk.services.fms.model.InvalidTypeException;
import software.amazon.awssdk.services.fms.model.LimitExceededException;
import software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationRequest;
import software.amazon.awssdk.services.fms.model.ListAdminAccountsForOrganizationResponse;
import software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountRequest;
import software.amazon.awssdk.services.fms.model.ListAdminsManagingAccountResponse;
import software.amazon.awssdk.services.fms.model.ListAppsListsRequest;
import software.amazon.awssdk.services.fms.model.ListAppsListsResponse;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse;
import software.amazon.awssdk.services.fms.model.ListDiscoveredResourcesRequest;
import software.amazon.awssdk.services.fms.model.ListDiscoveredResourcesResponse;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse;
import software.amazon.awssdk.services.fms.model.ListPoliciesRequest;
import software.amazon.awssdk.services.fms.model.ListPoliciesResponse;
import software.amazon.awssdk.services.fms.model.ListProtocolsListsRequest;
import software.amazon.awssdk.services.fms.model.ListProtocolsListsResponse;
import software.amazon.awssdk.services.fms.model.ListResourceSetResourcesRequest;
import software.amazon.awssdk.services.fms.model.ListResourceSetResourcesResponse;
import software.amazon.awssdk.services.fms.model.ListResourceSetsRequest;
import software.amazon.awssdk.services.fms.model.ListResourceSetsResponse;
import software.amazon.awssdk.services.fms.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.fms.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesRequest;
import software.amazon.awssdk.services.fms.model.ListThirdPartyFirewallFirewallPoliciesResponse;
import software.amazon.awssdk.services.fms.model.PutAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.PutAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.PutAppsListRequest;
import software.amazon.awssdk.services.fms.model.PutAppsListResponse;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.PutPolicyRequest;
import software.amazon.awssdk.services.fms.model.PutPolicyResponse;
import software.amazon.awssdk.services.fms.model.PutProtocolsListRequest;
import software.amazon.awssdk.services.fms.model.PutProtocolsListResponse;
import software.amazon.awssdk.services.fms.model.PutResourceSetRequest;
import software.amazon.awssdk.services.fms.model.PutResourceSetResponse;
import software.amazon.awssdk.services.fms.model.ResourceNotFoundException;
import software.amazon.awssdk.services.fms.model.TagResourceRequest;
import software.amazon.awssdk.services.fms.model.TagResourceResponse;
import software.amazon.awssdk.services.fms.model.UntagResourceRequest;
import software.amazon.awssdk.services.fms.model.UntagResourceResponse;
import software.amazon.awssdk.services.fms.transform.AssociateAdminAccountRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.AssociateThirdPartyFirewallRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.BatchAssociateResourceRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.BatchDisassociateResourceRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.DeleteAppsListRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.DeleteNotificationChannelRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.DeletePolicyRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.DeleteProtocolsListRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.DeleteResourceSetRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.DisassociateAdminAccountRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.DisassociateThirdPartyFirewallRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetAdminAccountRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetAdminScopeRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetAppsListRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetComplianceDetailRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetNotificationChannelRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetPolicyRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetProtectionStatusRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetProtocolsListRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetResourceSetRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetThirdPartyFirewallAssociationStatusRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.GetViolationDetailsRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListAdminAccountsForOrganizationRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListAdminsManagingAccountRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListAppsListsRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListComplianceStatusRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListDiscoveredResourcesRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListMemberAccountsRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListPoliciesRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListProtocolsListsRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListResourceSetResourcesRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListResourceSetsRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.ListThirdPartyFirewallFirewallPoliciesRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.PutAdminAccountRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.PutAppsListRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.PutNotificationChannelRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.PutPolicyRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.PutProtocolsListRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.PutResourceSetRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.fms.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link FmsAsyncClient}.
*
* @see FmsAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultFmsAsyncClient implements FmsAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultFmsAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultFmsAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Sets a Firewall Manager default administrator account. The Firewall Manager default administrator account can
* manage third-party firewalls and has full administrative scope that allows administration of all policy types,
* accounts, organizational units, and Regions. This account must be a member account of the organization in
* Organizations whose resources you want to protect.
*
*
* For information about working with Firewall Manager administrator accounts, see Managing Firewall
* Manager administrators in the Firewall Manager Developer Guide.
*
*
* @param associateAdminAccountRequest
* @return A Java Future containing the result of the AssociateAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.AssociateAdminAccount
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture associateAdminAccount(
AssociateAdminAccountRequest associateAdminAccountRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateAdminAccountRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateAdminAccountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateAdminAccount");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateAdminAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateAdminAccount").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateAdminAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateAdminAccountRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the Firewall Manager policy administrator as a tenant administrator of a third-party firewall service. A
* tenant is an instance of the third-party firewall service that's associated with your Amazon Web Services
* customer account.
*
*
* @param associateThirdPartyFirewallRequest
* @return A Java Future containing the result of the AssociateThirdPartyFirewall operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.AssociateThirdPartyFirewall
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateThirdPartyFirewall(
AssociateThirdPartyFirewallRequest associateThirdPartyFirewallRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateThirdPartyFirewallRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateThirdPartyFirewallRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateThirdPartyFirewall");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateThirdPartyFirewallResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateThirdPartyFirewall").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateThirdPartyFirewallRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateThirdPartyFirewallRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Associate resources to a Firewall Manager resource set.
*
*
* @param batchAssociateResourceRequest
* @return A Java Future containing the result of the BatchAssociateResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.BatchAssociateResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchAssociateResource(
BatchAssociateResourceRequest batchAssociateResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchAssociateResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchAssociateResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchAssociateResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchAssociateResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchAssociateResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchAssociateResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchAssociateResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disassociates resources from a Firewall Manager resource set.
*
*
* @param batchDisassociateResourceRequest
* @return A Java Future containing the result of the BatchDisassociateResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.BatchDisassociateResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchDisassociateResource(
BatchDisassociateResourceRequest batchDisassociateResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDisassociateResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDisassociateResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDisassociateResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDisassociateResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDisassociateResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDisassociateResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDisassociateResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Permanently deletes an Firewall Manager applications list.
*
*
* @param deleteAppsListRequest
* @return A Java Future containing the result of the DeleteAppsList operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeleteAppsList
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAppsList(DeleteAppsListRequest deleteAppsListRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAppsListRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppsListRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAppsList");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAppsListResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAppsList").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAppsListRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAppsListRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS) topic
* that is used to record Firewall Manager SNS logs.
*
*
* @param deleteNotificationChannelRequest
* @return A Java Future containing the result of the DeleteNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteNotificationChannel(
DeleteNotificationChannelRequest deleteNotificationChannelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteNotificationChannelRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteNotificationChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteNotificationChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteNotificationChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteNotificationChannel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteNotificationChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteNotificationChannelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Permanently deletes an Firewall Manager policy.
*
*
* @param deletePolicyRequest
* @return A Java Future containing the result of the DeletePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeletePolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deletePolicy(DeletePolicyRequest deletePolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePolicyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeletePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeletePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deletePolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Permanently deletes an Firewall Manager protocols list.
*
*
* @param deleteProtocolsListRequest
* @return A Java Future containing the result of the DeleteProtocolsList operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeleteProtocolsList
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteProtocolsList(
DeleteProtocolsListRequest deleteProtocolsListRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteProtocolsListRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProtocolsListRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProtocolsList");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteProtocolsListResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProtocolsList").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteProtocolsListRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteProtocolsListRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified ResourceSet.
*
*
* @param deleteResourceSetRequest
* @return A Java Future containing the result of the DeleteResourceSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeleteResourceSet
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteResourceSet(DeleteResourceSetRequest deleteResourceSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteResourceSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteResourceSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteResourceSet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteResourceSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteResourceSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteResourceSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteResourceSetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disassociates an Firewall Manager administrator account. To set a different account as an Firewall Manager
* administrator, submit a PutAdminAccount request. To set an account as a default administrator account, you
* must submit an AssociateAdminAccount request.
*
*
* Disassociation of the default administrator account follows the first in, last out principle. If you are the
* default administrator, all Firewall Manager administrators within the organization must first disassociate their
* accounts before you can disassociate your account.
*
*
* @param disassociateAdminAccountRequest
* @return A Java Future containing the result of the DisassociateAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture disassociateAdminAccount(
DisassociateAdminAccountRequest disassociateAdminAccountRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateAdminAccountRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateAdminAccountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateAdminAccount");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateAdminAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateAdminAccount").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateAdminAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateAdminAccountRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disassociates a Firewall Manager policy administrator from a third-party firewall tenant. When you call
* DisassociateThirdPartyFirewall
, the third-party firewall vendor deletes all of the firewalls that
* are associated with the account.
*
*
* @param disassociateThirdPartyFirewallRequest
* @return A Java Future containing the result of the DisassociateThirdPartyFirewall operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DisassociateThirdPartyFirewall
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateThirdPartyFirewall(
DisassociateThirdPartyFirewallRequest disassociateThirdPartyFirewallRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateThirdPartyFirewallRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateThirdPartyFirewallRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateThirdPartyFirewall");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateThirdPartyFirewallResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateThirdPartyFirewall").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateThirdPartyFirewallRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateThirdPartyFirewallRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the Organizations account that is associated with Firewall Manager as the Firewall Manager default
* administrator.
*
*
* @param getAdminAccountRequest
* @return A Java Future containing the result of the GetAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetAdminAccount
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getAdminAccount(GetAdminAccountRequest getAdminAccountRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAdminAccountRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAdminAccountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAdminAccount");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAdminAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAdminAccount").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAdminAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAdminAccountRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about the specified account's administrative scope. The administrative scope defines the
* resources that an Firewall Manager administrator can manage.
*
*
* @param getAdminScopeRequest
* @return A Java Future containing the result of the GetAdminScope operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetAdminScope
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getAdminScope(GetAdminScopeRequest getAdminScopeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAdminScopeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAdminScopeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAdminScope");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAdminScopeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAdminScope").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAdminScopeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAdminScopeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about the specified Firewall Manager applications list.
*
*
* @param getAppsListRequest
* @return A Java Future containing the result of the GetAppsList operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetAppsList
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getAppsList(GetAppsListRequest getAppsListRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAppsListRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAppsListRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAppsList");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAppsListResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAppsList").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAppsListRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAppsListRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy.
*
*
* The reasons for resources being considered compliant depend on the Firewall Manager policy type.
*
*
* @param getComplianceDetailRequest
* @return A Java Future containing the result of the GetComplianceDetail operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetComplianceDetail
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getComplianceDetail(
GetComplianceDetailRequest getComplianceDetailRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getComplianceDetailRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getComplianceDetailRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetComplianceDetail");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetComplianceDetailResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetComplianceDetail").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetComplianceDetailRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getComplianceDetailRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Information about the Amazon Simple Notification Service (SNS) topic that is used to record Firewall Manager SNS
* logs.
*
*
* @param getNotificationChannelRequest
* @return A Java Future containing the result of the GetNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetNotificationChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getNotificationChannel(
GetNotificationChannelRequest getNotificationChannelRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getNotificationChannelRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getNotificationChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetNotificationChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetNotificationChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetNotificationChannel").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetNotificationChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getNotificationChannelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about the specified Firewall Manager policy.
*
*
* @param getPolicyRequest
* @return A Java Future containing the result of the GetPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidTypeException The value of the
Type
parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getPolicy(GetPolicyRequest getPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getPolicyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetPolicy")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetPolicyRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(getPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack. Other policy types are currently unsupported.
*
*
* @param getProtectionStatusRequest
* @return A Java Future containing the result of the GetProtectionStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetProtectionStatus
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getProtectionStatus(
GetProtectionStatusRequest getProtectionStatusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getProtectionStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getProtectionStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetProtectionStatus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetProtectionStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetProtectionStatus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetProtectionStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getProtectionStatusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about the specified Firewall Manager protocols list.
*
*
* @param getProtocolsListRequest
* @return A Java Future containing the result of the GetProtocolsList operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetProtocolsList
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getProtocolsList(GetProtocolsListRequest getProtocolsListRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getProtocolsListRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getProtocolsListRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetProtocolsList");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetProtocolsListResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetProtocolsList").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetProtocolsListRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getProtocolsListRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a specific resource set.
*
*
* @param getResourceSetRequest
* @return A Java Future containing the result of the GetResourceSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetResourceSet
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getResourceSet(GetResourceSetRequest getResourceSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getResourceSetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getResourceSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetResourceSet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetResourceSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetResourceSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetResourceSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getResourceSetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The onboarding status of a Firewall Manager admin account to third-party firewall vendor tenant.
*
*
* @param getThirdPartyFirewallAssociationStatusRequest
* @return A Java Future containing the result of the GetThirdPartyFirewallAssociationStatus operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetThirdPartyFirewallAssociationStatus
* @see AWS API Documentation
*/
@Override
public CompletableFuture getThirdPartyFirewallAssociationStatus(
GetThirdPartyFirewallAssociationStatusRequest getThirdPartyFirewallAssociationStatusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getThirdPartyFirewallAssociationStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getThirdPartyFirewallAssociationStatusRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetThirdPartyFirewallAssociationStatus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetThirdPartyFirewallAssociationStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetThirdPartyFirewallAssociationStatus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetThirdPartyFirewallAssociationStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getThirdPartyFirewallAssociationStatusRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves violations for a resource based on the specified Firewall Manager policy and Amazon Web Services
* account.
*
*
* @param getViolationDetailsRequest
* @return A Java Future containing the result of the GetViolationDetails operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidInputException The parameters of the request were invalid.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetViolationDetails
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getViolationDetails(
GetViolationDetailsRequest getViolationDetailsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getViolationDetailsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getViolationDetailsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetViolationDetails");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetViolationDetailsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetViolationDetails").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetViolationDetailsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getViolationDetailsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a AdminAccounts
object that lists the Firewall Manager administrators within the
* organization that are onboarded to Firewall Manager by AssociateAdminAccount.
*
*
* This operation can be called only from the organization's management account.
*
*
* @param listAdminAccountsForOrganizationRequest
* @return A Java Future containing the result of the ListAdminAccountsForOrganization operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListAdminAccountsForOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture listAdminAccountsForOrganization(
ListAdminAccountsForOrganizationRequest listAdminAccountsForOrganizationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAdminAccountsForOrganizationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listAdminAccountsForOrganizationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAdminAccountsForOrganization");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListAdminAccountsForOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAdminAccountsForOrganization").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAdminAccountsForOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAdminAccountsForOrganizationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the accounts that are managing the specified Organizations member account. This is useful for any member
* account so that they can view the accounts who are managing their account. This operation only returns the
* managing administrators that have the requested account within their AdminScope.
*
*
* @param listAdminsManagingAccountRequest
* @return A Java Future containing the result of the ListAdminsManagingAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidInputException The parameters of the request were invalid.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListAdminsManagingAccount
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listAdminsManagingAccount(
ListAdminsManagingAccountRequest listAdminsManagingAccountRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAdminsManagingAccountRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAdminsManagingAccountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAdminsManagingAccount");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAdminsManagingAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAdminsManagingAccount").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAdminsManagingAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAdminsManagingAccountRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of AppsListDataSummary
objects.
*
*
* @param listAppsListsRequest
* @return A Java Future containing the result of the ListAppsLists operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListAppsLists
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAppsLists(ListAppsListsRequest listAppsListsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAppsListsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAppsListsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAppsLists");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAppsListsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAppsLists").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAppsListsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAppsListsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of PolicyComplianceStatus
objects. Use PolicyComplianceStatus
to get a
* summary of which member accounts are protected by the specified policy.
*
*
* @param listComplianceStatusRequest
* @return A Java Future containing the result of the ListComplianceStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listComplianceStatus(
ListComplianceStatusRequest listComplianceStatusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listComplianceStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listComplianceStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListComplianceStatus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListComplianceStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListComplianceStatus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListComplianceStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listComplianceStatusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of resources in the organization's accounts that are available to be associated with a resource
* set.
*
*
* @param listDiscoveredResourcesRequest
* @return A Java Future containing the result of the ListDiscoveredResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListDiscoveredResources
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listDiscoveredResources(
ListDiscoveredResourcesRequest listDiscoveredResourcesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDiscoveredResourcesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDiscoveredResourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDiscoveredResources");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDiscoveredResourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDiscoveredResources").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDiscoveredResourcesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDiscoveredResourcesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's Amazon Web
* Services organization.
*
*
* Either an Firewall Manager administrator or the organization's management account can make this request.
*
*
* @param listMemberAccountsRequest
* @return A Java Future containing the result of the ListMemberAccounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listMemberAccounts(ListMemberAccountsRequest listMemberAccountsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMemberAccountsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMemberAccountsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMemberAccounts");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMemberAccountsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListMemberAccounts").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListMemberAccountsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listMemberAccountsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of PolicySummary
objects.
*
*
* @param listPoliciesRequest
* @return A Java Future containing the result of the ListPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListPolicies
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listPolicies(ListPoliciesRequest listPoliciesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listPoliciesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPoliciesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPolicies");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPolicies").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListPoliciesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listPoliciesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of ProtocolsListDataSummary
objects.
*
*
* @param listProtocolsListsRequest
* @return A Java Future containing the result of the ListProtocolsLists operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListProtocolsLists
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listProtocolsLists(ListProtocolsListsRequest listProtocolsListsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listProtocolsListsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProtocolsListsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProtocolsLists");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListProtocolsListsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProtocolsLists").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListProtocolsListsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listProtocolsListsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of resources that are currently associated to a resource set.
*
*
* @param listResourceSetResourcesRequest
* @return A Java Future containing the result of the ListResourceSetResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListResourceSetResources
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listResourceSetResources(
ListResourceSetResourcesRequest listResourceSetResourcesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listResourceSetResourcesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listResourceSetResourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListResourceSetResources");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListResourceSetResourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListResourceSetResources").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListResourceSetResourcesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listResourceSetResourcesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of ResourceSetSummary
objects.
*
*
* @param listResourceSetsRequest
* @return A Java Future containing the result of the ListResourceSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListResourceSets
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listResourceSets(ListResourceSetsRequest listResourceSetsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listResourceSetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listResourceSetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListResourceSets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListResourceSetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListResourceSets").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListResourceSetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listResourceSetsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the list of tags for the specified Amazon Web Services resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a list of all of the third-party firewall policies that are associated with the third-party firewall
* administrator's account.
*
*
* @param listThirdPartyFirewallFirewallPoliciesRequest
* @return A Java Future containing the result of the ListThirdPartyFirewallFirewallPolicies operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListThirdPartyFirewallFirewallPolicies
* @see AWS API Documentation
*/
@Override
public CompletableFuture listThirdPartyFirewallFirewallPolicies(
ListThirdPartyFirewallFirewallPoliciesRequest listThirdPartyFirewallFirewallPoliciesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listThirdPartyFirewallFirewallPoliciesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listThirdPartyFirewallFirewallPoliciesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListThirdPartyFirewallFirewallPolicies");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListThirdPartyFirewallFirewallPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListThirdPartyFirewallFirewallPolicies").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListThirdPartyFirewallFirewallPoliciesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listThirdPartyFirewallFirewallPoliciesRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates or updates an Firewall Manager administrator account. The account must be a member of the organization
* that was onboarded to Firewall Manager by AssociateAdminAccount. Only the organization's management
* account can create an Firewall Manager administrator account. When you create an Firewall Manager administrator
* account, the service checks to see if the account is already a delegated administrator within Organizations. If
* the account isn't a delegated administrator, Firewall Manager calls Organizations to delegate the account within
* Organizations. For more information about administrator accounts within Organizations, see Managing the Amazon
* Web Services Accounts in Your Organization.
*
*
* @param putAdminAccountRequest
* @return A Java Future containing the result of the PutAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.PutAdminAccount
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putAdminAccount(PutAdminAccountRequest putAdminAccountRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAdminAccountRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAdminAccountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAdminAccount");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAdminAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAdminAccount").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutAdminAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putAdminAccountRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Firewall Manager applications list.
*
*
* @param putAppsListRequest
* @return A Java Future containing the result of the PutAppsList operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do or the operation
* wasn't possible. For example, you might have submitted an
AssociateAdminAccount
request for
* an account ID that was already set as the Firewall Manager administrator. Or you might have tried to
* access a Region that's disabled by default, and that you need to enable for the Firewall Manager
* administrator account and for Organizations before you can access it.
* - InvalidInputException The parameters of the request were invalid.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an Amazon Web Services account. For more information,
* see Firewall Manager
* Limits in the WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.PutAppsList
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putAppsList(PutAppsListRequest putAppsListRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAppsListRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAppsListRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "FMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAppsList");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutAppsListResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams