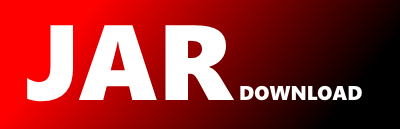
software.amazon.awssdk.services.fms.model.AdminScope Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Defines the resources that the Firewall Manager administrator can manage. For more information about administrative
* scope, see Managing Firewall
* Manager administrators in the Firewall Manager Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AdminScope implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACCOUNT_SCOPE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("AccountScope").getter(getter(AdminScope::accountScope)).setter(setter(Builder::accountScope))
.constructor(AccountScope::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountScope").build()).build();
private static final SdkField ORGANIZATIONAL_UNIT_SCOPE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OrganizationalUnitScope")
.getter(getter(AdminScope::organizationalUnitScope)).setter(setter(Builder::organizationalUnitScope))
.constructor(OrganizationalUnitScope::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationalUnitScope").build())
.build();
private static final SdkField REGION_SCOPE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("RegionScope").getter(getter(AdminScope::regionScope)).setter(setter(Builder::regionScope))
.constructor(RegionScope::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegionScope").build()).build();
private static final SdkField POLICY_TYPE_SCOPE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PolicyTypeScope")
.getter(getter(AdminScope::policyTypeScope)).setter(setter(Builder::policyTypeScope))
.constructor(PolicyTypeScope::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyTypeScope").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNT_SCOPE_FIELD,
ORGANIZATIONAL_UNIT_SCOPE_FIELD, REGION_SCOPE_FIELD, POLICY_TYPE_SCOPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AccountScope", ACCOUNT_SCOPE_FIELD);
put("OrganizationalUnitScope", ORGANIZATIONAL_UNIT_SCOPE_FIELD);
put("RegionScope", REGION_SCOPE_FIELD);
put("PolicyTypeScope", POLICY_TYPE_SCOPE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final AccountScope accountScope;
private final OrganizationalUnitScope organizationalUnitScope;
private final RegionScope regionScope;
private final PolicyTypeScope policyTypeScope;
private AdminScope(BuilderImpl builder) {
this.accountScope = builder.accountScope;
this.organizationalUnitScope = builder.organizationalUnitScope;
this.regionScope = builder.regionScope;
this.policyTypeScope = builder.policyTypeScope;
}
/**
*
* Defines the accounts that the specified Firewall Manager administrator can apply policies to.
*
*
* @return Defines the accounts that the specified Firewall Manager administrator can apply policies to.
*/
public final AccountScope accountScope() {
return accountScope;
}
/**
*
* Defines the Organizations organizational units that the specified Firewall Manager administrator can apply
* policies to. For more information about OUs in Organizations, see Managing organizational
* units (OUs) in the Organizations User Guide.
*
*
* @return Defines the Organizations organizational units that the specified Firewall Manager administrator can
* apply policies to. For more information about OUs in Organizations, see Managing
* organizational units (OUs) in the Organizations User Guide.
*/
public final OrganizationalUnitScope organizationalUnitScope() {
return organizationalUnitScope;
}
/**
*
* Defines the Amazon Web Services Regions that the specified Firewall Manager administrator can perform actions in.
*
*
* @return Defines the Amazon Web Services Regions that the specified Firewall Manager administrator can perform
* actions in.
*/
public final RegionScope regionScope() {
return regionScope;
}
/**
*
* Defines the Firewall Manager policy types that the specified Firewall Manager administrator can create and
* manage.
*
*
* @return Defines the Firewall Manager policy types that the specified Firewall Manager administrator can create
* and manage.
*/
public final PolicyTypeScope policyTypeScope() {
return policyTypeScope;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accountScope());
hashCode = 31 * hashCode + Objects.hashCode(organizationalUnitScope());
hashCode = 31 * hashCode + Objects.hashCode(regionScope());
hashCode = 31 * hashCode + Objects.hashCode(policyTypeScope());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AdminScope)) {
return false;
}
AdminScope other = (AdminScope) obj;
return Objects.equals(accountScope(), other.accountScope())
&& Objects.equals(organizationalUnitScope(), other.organizationalUnitScope())
&& Objects.equals(regionScope(), other.regionScope())
&& Objects.equals(policyTypeScope(), other.policyTypeScope());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AdminScope").add("AccountScope", accountScope())
.add("OrganizationalUnitScope", organizationalUnitScope()).add("RegionScope", regionScope())
.add("PolicyTypeScope", policyTypeScope()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AccountScope":
return Optional.ofNullable(clazz.cast(accountScope()));
case "OrganizationalUnitScope":
return Optional.ofNullable(clazz.cast(organizationalUnitScope()));
case "RegionScope":
return Optional.ofNullable(clazz.cast(regionScope()));
case "PolicyTypeScope":
return Optional.ofNullable(clazz.cast(policyTypeScope()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function