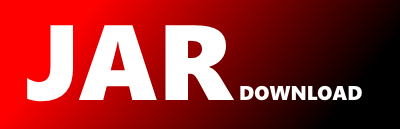
software.amazon.awssdk.services.fms.model.NetworkFirewallPolicyDescription Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The definition of the Network Firewall firewall policy.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkFirewallPolicyDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> STATELESS_RULE_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatelessRuleGroups")
.getter(getter(NetworkFirewallPolicyDescription::statelessRuleGroups))
.setter(setter(Builder::statelessRuleGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatelessRuleGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(StatelessRuleGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> STATELESS_DEFAULT_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatelessDefaultActions")
.getter(getter(NetworkFirewallPolicyDescription::statelessDefaultActions))
.setter(setter(Builder::statelessDefaultActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatelessDefaultActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> STATELESS_FRAGMENT_DEFAULT_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatelessFragmentDefaultActions")
.getter(getter(NetworkFirewallPolicyDescription::statelessFragmentDefaultActions))
.setter(setter(Builder::statelessFragmentDefaultActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatelessFragmentDefaultActions")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> STATELESS_CUSTOM_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatelessCustomActions")
.getter(getter(NetworkFirewallPolicyDescription::statelessCustomActions))
.setter(setter(Builder::statelessCustomActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatelessCustomActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> STATEFUL_RULE_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatefulRuleGroups")
.getter(getter(NetworkFirewallPolicyDescription::statefulRuleGroups))
.setter(setter(Builder::statefulRuleGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatefulRuleGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(StatefulRuleGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> STATEFUL_DEFAULT_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatefulDefaultActions")
.getter(getter(NetworkFirewallPolicyDescription::statefulDefaultActions))
.setter(setter(Builder::statefulDefaultActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatefulDefaultActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATEFUL_ENGINE_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StatefulEngineOptions")
.getter(getter(NetworkFirewallPolicyDescription::statefulEngineOptions))
.setter(setter(Builder::statefulEngineOptions)).constructor(StatefulEngineOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatefulEngineOptions").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STATELESS_RULE_GROUPS_FIELD,
STATELESS_DEFAULT_ACTIONS_FIELD, STATELESS_FRAGMENT_DEFAULT_ACTIONS_FIELD, STATELESS_CUSTOM_ACTIONS_FIELD,
STATEFUL_RULE_GROUPS_FIELD, STATEFUL_DEFAULT_ACTIONS_FIELD, STATEFUL_ENGINE_OPTIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("StatelessRuleGroups", STATELESS_RULE_GROUPS_FIELD);
put("StatelessDefaultActions", STATELESS_DEFAULT_ACTIONS_FIELD);
put("StatelessFragmentDefaultActions", STATELESS_FRAGMENT_DEFAULT_ACTIONS_FIELD);
put("StatelessCustomActions", STATELESS_CUSTOM_ACTIONS_FIELD);
put("StatefulRuleGroups", STATEFUL_RULE_GROUPS_FIELD);
put("StatefulDefaultActions", STATEFUL_DEFAULT_ACTIONS_FIELD);
put("StatefulEngineOptions", STATEFUL_ENGINE_OPTIONS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final List statelessRuleGroups;
private final List statelessDefaultActions;
private final List statelessFragmentDefaultActions;
private final List statelessCustomActions;
private final List statefulRuleGroups;
private final List statefulDefaultActions;
private final StatefulEngineOptions statefulEngineOptions;
private NetworkFirewallPolicyDescription(BuilderImpl builder) {
this.statelessRuleGroups = builder.statelessRuleGroups;
this.statelessDefaultActions = builder.statelessDefaultActions;
this.statelessFragmentDefaultActions = builder.statelessFragmentDefaultActions;
this.statelessCustomActions = builder.statelessCustomActions;
this.statefulRuleGroups = builder.statefulRuleGroups;
this.statefulDefaultActions = builder.statefulDefaultActions;
this.statefulEngineOptions = builder.statefulEngineOptions;
}
/**
* For responses, this returns true if the service returned a value for the StatelessRuleGroups property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasStatelessRuleGroups() {
return statelessRuleGroups != null && !(statelessRuleGroups instanceof SdkAutoConstructList);
}
/**
*
* The stateless rule groups that are used in the Network Firewall firewall policy.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatelessRuleGroups} method.
*
*
* @return The stateless rule groups that are used in the Network Firewall firewall policy.
*/
public final List statelessRuleGroups() {
return statelessRuleGroups;
}
/**
* For responses, this returns true if the service returned a value for the StatelessDefaultActions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasStatelessDefaultActions() {
return statelessDefaultActions != null && !(statelessDefaultActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to take on packets that don't match any of the stateless rule groups.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatelessDefaultActions} method.
*
*
* @return The actions to take on packets that don't match any of the stateless rule groups.
*/
public final List statelessDefaultActions() {
return statelessDefaultActions;
}
/**
* For responses, this returns true if the service returned a value for the StatelessFragmentDefaultActions
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasStatelessFragmentDefaultActions() {
return statelessFragmentDefaultActions != null && !(statelessFragmentDefaultActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to take on packet fragments that don't match any of the stateless rule groups.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatelessFragmentDefaultActions}
* method.
*
*
* @return The actions to take on packet fragments that don't match any of the stateless rule groups.
*/
public final List statelessFragmentDefaultActions() {
return statelessFragmentDefaultActions;
}
/**
* For responses, this returns true if the service returned a value for the StatelessCustomActions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasStatelessCustomActions() {
return statelessCustomActions != null && !(statelessCustomActions instanceof SdkAutoConstructList);
}
/**
*
* Names of custom actions that are available for use in the stateless default actions settings.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatelessCustomActions} method.
*
*
* @return Names of custom actions that are available for use in the stateless default actions settings.
*/
public final List statelessCustomActions() {
return statelessCustomActions;
}
/**
* For responses, this returns true if the service returned a value for the StatefulRuleGroups property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasStatefulRuleGroups() {
return statefulRuleGroups != null && !(statefulRuleGroups instanceof SdkAutoConstructList);
}
/**
*
* The stateful rule groups that are used in the Network Firewall firewall policy.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatefulRuleGroups} method.
*
*
* @return The stateful rule groups that are used in the Network Firewall firewall policy.
*/
public final List statefulRuleGroups() {
return statefulRuleGroups;
}
/**
* For responses, this returns true if the service returned a value for the StatefulDefaultActions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasStatefulDefaultActions() {
return statefulDefaultActions != null && !(statefulDefaultActions instanceof SdkAutoConstructList);
}
/**
*
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action is
* optional, and is only valid when using the strict rule order.
*
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatefulDefaultActions} method.
*
*
* @return The default actions to take on a packet that doesn't match any stateful rules. The stateful default
* action is optional, and is only valid when using the strict rule order.
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*/
public final List statefulDefaultActions() {
return statefulDefaultActions;
}
/**
*
* Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that you use
* in your policy must have stateful rule options settings that are compatible with these settings.
*
*
* @return Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that
* you use in your policy must have stateful rule options settings that are compatible with these settings.
*/
public final StatefulEngineOptions statefulEngineOptions() {
return statefulEngineOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasStatelessRuleGroups() ? statelessRuleGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasStatelessDefaultActions() ? statelessDefaultActions() : null);
hashCode = 31 * hashCode
+ Objects.hashCode(hasStatelessFragmentDefaultActions() ? statelessFragmentDefaultActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasStatelessCustomActions() ? statelessCustomActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasStatefulRuleGroups() ? statefulRuleGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasStatefulDefaultActions() ? statefulDefaultActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(statefulEngineOptions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkFirewallPolicyDescription)) {
return false;
}
NetworkFirewallPolicyDescription other = (NetworkFirewallPolicyDescription) obj;
return hasStatelessRuleGroups() == other.hasStatelessRuleGroups()
&& Objects.equals(statelessRuleGroups(), other.statelessRuleGroups())
&& hasStatelessDefaultActions() == other.hasStatelessDefaultActions()
&& Objects.equals(statelessDefaultActions(), other.statelessDefaultActions())
&& hasStatelessFragmentDefaultActions() == other.hasStatelessFragmentDefaultActions()
&& Objects.equals(statelessFragmentDefaultActions(), other.statelessFragmentDefaultActions())
&& hasStatelessCustomActions() == other.hasStatelessCustomActions()
&& Objects.equals(statelessCustomActions(), other.statelessCustomActions())
&& hasStatefulRuleGroups() == other.hasStatefulRuleGroups()
&& Objects.equals(statefulRuleGroups(), other.statefulRuleGroups())
&& hasStatefulDefaultActions() == other.hasStatefulDefaultActions()
&& Objects.equals(statefulDefaultActions(), other.statefulDefaultActions())
&& Objects.equals(statefulEngineOptions(), other.statefulEngineOptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("NetworkFirewallPolicyDescription")
.add("StatelessRuleGroups", hasStatelessRuleGroups() ? statelessRuleGroups() : null)
.add("StatelessDefaultActions", hasStatelessDefaultActions() ? statelessDefaultActions() : null)
.add("StatelessFragmentDefaultActions",
hasStatelessFragmentDefaultActions() ? statelessFragmentDefaultActions() : null)
.add("StatelessCustomActions", hasStatelessCustomActions() ? statelessCustomActions() : null)
.add("StatefulRuleGroups", hasStatefulRuleGroups() ? statefulRuleGroups() : null)
.add("StatefulDefaultActions", hasStatefulDefaultActions() ? statefulDefaultActions() : null)
.add("StatefulEngineOptions", statefulEngineOptions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StatelessRuleGroups":
return Optional.ofNullable(clazz.cast(statelessRuleGroups()));
case "StatelessDefaultActions":
return Optional.ofNullable(clazz.cast(statelessDefaultActions()));
case "StatelessFragmentDefaultActions":
return Optional.ofNullable(clazz.cast(statelessFragmentDefaultActions()));
case "StatelessCustomActions":
return Optional.ofNullable(clazz.cast(statelessCustomActions()));
case "StatefulRuleGroups":
return Optional.ofNullable(clazz.cast(statefulRuleGroups()));
case "StatefulDefaultActions":
return Optional.ofNullable(clazz.cast(statefulDefaultActions()));
case "StatefulEngineOptions":
return Optional.ofNullable(clazz.cast(statefulEngineOptions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function