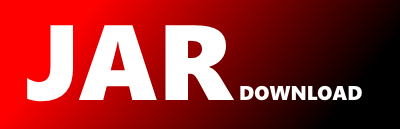
software.amazon.awssdk.services.fms.model.ViolationDetail Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Violations for a resource based on the specified Firewall Manager policy and Amazon Web Services account.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ViolationDetail implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField POLICY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyId").getter(getter(ViolationDetail::policyId)).setter(setter(Builder::policyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyId").build()).build();
private static final SdkField MEMBER_ACCOUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MemberAccount").getter(getter(ViolationDetail::memberAccount)).setter(setter(Builder::memberAccount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemberAccount").build()).build();
private static final SdkField RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceId").getter(getter(ViolationDetail::resourceId)).setter(setter(Builder::resourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceId").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceType").getter(getter(ViolationDetail::resourceType)).setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField> RESOURCE_VIOLATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourceViolations")
.getter(getter(ViolationDetail::resourceViolations))
.setter(setter(Builder::resourceViolations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceViolations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ResourceViolation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> RESOURCE_TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourceTags")
.getter(getter(ViolationDetail::resourceTags))
.setter(setter(Builder::resourceTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceTags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RESOURCE_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceDescription").getter(getter(ViolationDetail::resourceDescription))
.setter(setter(Builder::resourceDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceDescription").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(POLICY_ID_FIELD,
MEMBER_ACCOUNT_FIELD, RESOURCE_ID_FIELD, RESOURCE_TYPE_FIELD, RESOURCE_VIOLATIONS_FIELD, RESOURCE_TAGS_FIELD,
RESOURCE_DESCRIPTION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("PolicyId", POLICY_ID_FIELD);
put("MemberAccount", MEMBER_ACCOUNT_FIELD);
put("ResourceId", RESOURCE_ID_FIELD);
put("ResourceType", RESOURCE_TYPE_FIELD);
put("ResourceViolations", RESOURCE_VIOLATIONS_FIELD);
put("ResourceTags", RESOURCE_TAGS_FIELD);
put("ResourceDescription", RESOURCE_DESCRIPTION_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String policyId;
private final String memberAccount;
private final String resourceId;
private final String resourceType;
private final List resourceViolations;
private final List resourceTags;
private final String resourceDescription;
private ViolationDetail(BuilderImpl builder) {
this.policyId = builder.policyId;
this.memberAccount = builder.memberAccount;
this.resourceId = builder.resourceId;
this.resourceType = builder.resourceType;
this.resourceViolations = builder.resourceViolations;
this.resourceTags = builder.resourceTags;
this.resourceDescription = builder.resourceDescription;
}
/**
*
* The ID of the Firewall Manager policy that the violation details were requested for.
*
*
* @return The ID of the Firewall Manager policy that the violation details were requested for.
*/
public final String policyId() {
return policyId;
}
/**
*
* The Amazon Web Services account that the violation details were requested for.
*
*
* @return The Amazon Web Services account that the violation details were requested for.
*/
public final String memberAccount() {
return memberAccount;
}
/**
*
* The resource ID that the violation details were requested for.
*
*
* @return The resource ID that the violation details were requested for.
*/
public final String resourceId() {
return resourceId;
}
/**
*
* The resource type that the violation details were requested for.
*
*
* @return The resource type that the violation details were requested for.
*/
public final String resourceType() {
return resourceType;
}
/**
* For responses, this returns true if the service returned a value for the ResourceViolations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourceViolations() {
return resourceViolations != null && !(resourceViolations instanceof SdkAutoConstructList);
}
/**
*
* List of violations for the requested resource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourceViolations} method.
*
*
* @return List of violations for the requested resource.
*/
public final List resourceViolations() {
return resourceViolations;
}
/**
* For responses, this returns true if the service returned a value for the ResourceTags property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourceTags() {
return resourceTags != null && !(resourceTags instanceof SdkAutoConstructList);
}
/**
*
* The ResourceTag
objects associated with the resource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourceTags} method.
*
*
* @return The ResourceTag
objects associated with the resource.
*/
public final List resourceTags() {
return resourceTags;
}
/**
*
* Brief description for the requested resource.
*
*
* @return Brief description for the requested resource.
*/
public final String resourceDescription() {
return resourceDescription;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(policyId());
hashCode = 31 * hashCode + Objects.hashCode(memberAccount());
hashCode = 31 * hashCode + Objects.hashCode(resourceId());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(hasResourceViolations() ? resourceViolations() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasResourceTags() ? resourceTags() : null);
hashCode = 31 * hashCode + Objects.hashCode(resourceDescription());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ViolationDetail)) {
return false;
}
ViolationDetail other = (ViolationDetail) obj;
return Objects.equals(policyId(), other.policyId()) && Objects.equals(memberAccount(), other.memberAccount())
&& Objects.equals(resourceId(), other.resourceId()) && Objects.equals(resourceType(), other.resourceType())
&& hasResourceViolations() == other.hasResourceViolations()
&& Objects.equals(resourceViolations(), other.resourceViolations())
&& hasResourceTags() == other.hasResourceTags() && Objects.equals(resourceTags(), other.resourceTags())
&& Objects.equals(resourceDescription(), other.resourceDescription());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ViolationDetail").add("PolicyId", policyId()).add("MemberAccount", memberAccount())
.add("ResourceId", resourceId()).add("ResourceType", resourceType())
.add("ResourceViolations", hasResourceViolations() ? resourceViolations() : null)
.add("ResourceTags", hasResourceTags() ? resourceTags() : null).add("ResourceDescription", resourceDescription())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PolicyId":
return Optional.ofNullable(clazz.cast(policyId()));
case "MemberAccount":
return Optional.ofNullable(clazz.cast(memberAccount()));
case "ResourceId":
return Optional.ofNullable(clazz.cast(resourceId()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "ResourceViolations":
return Optional.ofNullable(clazz.cast(resourceViolations()));
case "ResourceTags":
return Optional.ofNullable(clazz.cast(resourceTags()));
case "ResourceDescription":
return Optional.ofNullable(clazz.cast(resourceDescription()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function