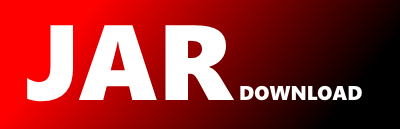
software.amazon.awssdk.services.fms.model.NetworkAclEntrySet Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The configuration of the first and last rules for the network ACL policy, and the remediation settings for each.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkAclEntrySet implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> FIRST_ENTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("FirstEntries")
.getter(getter(NetworkAclEntrySet::firstEntries))
.setter(setter(Builder::firstEntries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FirstEntries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NetworkAclEntry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FORCE_REMEDIATE_FOR_FIRST_ENTRIES_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ForceRemediateForFirstEntries")
.getter(getter(NetworkAclEntrySet::forceRemediateForFirstEntries))
.setter(setter(Builder::forceRemediateForFirstEntries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ForceRemediateForFirstEntries")
.build()).build();
private static final SdkField> LAST_ENTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LastEntries")
.getter(getter(NetworkAclEntrySet::lastEntries))
.setter(setter(Builder::lastEntries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastEntries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NetworkAclEntry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FORCE_REMEDIATE_FOR_LAST_ENTRIES_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ForceRemediateForLastEntries")
.getter(getter(NetworkAclEntrySet::forceRemediateForLastEntries))
.setter(setter(Builder::forceRemediateForLastEntries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ForceRemediateForLastEntries")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FIRST_ENTRIES_FIELD,
FORCE_REMEDIATE_FOR_FIRST_ENTRIES_FIELD, LAST_ENTRIES_FIELD, FORCE_REMEDIATE_FOR_LAST_ENTRIES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("FirstEntries", FIRST_ENTRIES_FIELD);
put("ForceRemediateForFirstEntries", FORCE_REMEDIATE_FOR_FIRST_ENTRIES_FIELD);
put("LastEntries", LAST_ENTRIES_FIELD);
put("ForceRemediateForLastEntries", FORCE_REMEDIATE_FOR_LAST_ENTRIES_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final List firstEntries;
private final Boolean forceRemediateForFirstEntries;
private final List lastEntries;
private final Boolean forceRemediateForLastEntries;
private NetworkAclEntrySet(BuilderImpl builder) {
this.firstEntries = builder.firstEntries;
this.forceRemediateForFirstEntries = builder.forceRemediateForFirstEntries;
this.lastEntries = builder.lastEntries;
this.forceRemediateForLastEntries = builder.forceRemediateForLastEntries;
}
/**
* For responses, this returns true if the service returned a value for the FirstEntries property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasFirstEntries() {
return firstEntries != null && !(firstEntries instanceof SdkAutoConstructList);
}
/**
*
* The rules that you want to run first in the Firewall Manager managed network ACLs.
*
*
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule numbers
* for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFirstEntries} method.
*
*
* @return The rules that you want to run first in the Firewall Manager managed network ACLs.
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule
* numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*/
public final List firstEntries() {
return firstEntries;
}
/**
*
* Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting when it
* finds policy violations that involve conflicts between the custom entries and the policy entries.
*
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not try to
* remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
*
*
* @return Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting
* when it finds policy violations that involve conflicts between the custom entries and the policy entries.
*
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not
* try to remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
*/
public final Boolean forceRemediateForFirstEntries() {
return forceRemediateForFirstEntries;
}
/**
* For responses, this returns true if the service returned a value for the LastEntries property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLastEntries() {
return lastEntries != null && !(lastEntries instanceof SdkAutoConstructList);
}
/**
*
* The rules that you want to run last in the Firewall Manager managed network ACLs.
*
*
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule numbers
* for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLastEntries} method.
*
*
* @return The rules that you want to run last in the Firewall Manager managed network ACLs.
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule
* numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*/
public final List lastEntries() {
return lastEntries;
}
/**
*
* Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting when it
* finds policy violations that involve conflicts between the custom entries and the policy entries.
*
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not try to
* remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
*
*
* @return Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting
* when it finds policy violations that involve conflicts between the custom entries and the policy entries.
*
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not
* try to remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
*/
public final Boolean forceRemediateForLastEntries() {
return forceRemediateForLastEntries;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasFirstEntries() ? firstEntries() : null);
hashCode = 31 * hashCode + Objects.hashCode(forceRemediateForFirstEntries());
hashCode = 31 * hashCode + Objects.hashCode(hasLastEntries() ? lastEntries() : null);
hashCode = 31 * hashCode + Objects.hashCode(forceRemediateForLastEntries());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkAclEntrySet)) {
return false;
}
NetworkAclEntrySet other = (NetworkAclEntrySet) obj;
return hasFirstEntries() == other.hasFirstEntries() && Objects.equals(firstEntries(), other.firstEntries())
&& Objects.equals(forceRemediateForFirstEntries(), other.forceRemediateForFirstEntries())
&& hasLastEntries() == other.hasLastEntries() && Objects.equals(lastEntries(), other.lastEntries())
&& Objects.equals(forceRemediateForLastEntries(), other.forceRemediateForLastEntries());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NetworkAclEntrySet").add("FirstEntries", hasFirstEntries() ? firstEntries() : null)
.add("ForceRemediateForFirstEntries", forceRemediateForFirstEntries())
.add("LastEntries", hasLastEntries() ? lastEntries() : null)
.add("ForceRemediateForLastEntries", forceRemediateForLastEntries()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "FirstEntries":
return Optional.ofNullable(clazz.cast(firstEntries()));
case "ForceRemediateForFirstEntries":
return Optional.ofNullable(clazz.cast(forceRemediateForFirstEntries()));
case "LastEntries":
return Optional.ofNullable(clazz.cast(lastEntries()));
case "ForceRemediateForLastEntries":
return Optional.ofNullable(clazz.cast(forceRemediateForLastEntries()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific
* rule numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder firstEntries(Collection firstEntries);
/**
*
* The rules that you want to run first in the Firewall Manager managed network ACLs.
*
*
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule
* numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*
*
* @param firstEntries
* The rules that you want to run first in the Firewall Manager managed network ACLs.
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific
* rule numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder firstEntries(NetworkAclEntry... firstEntries);
/**
*
* The rules that you want to run first in the Firewall Manager managed network ACLs.
*
*
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule
* numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry.Builder#build()} is called immediately and
* its result is passed to {@link #firstEntries(List)}.
*
* @param firstEntries
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #firstEntries(java.util.Collection)
*/
Builder firstEntries(Consumer... firstEntries);
/**
*
* Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting when
* it finds policy violations that involve conflicts between the custom entries and the policy entries.
*
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not try to
* remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
*
*
* @param forceRemediateForFirstEntries
* Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting
* when it finds policy violations that involve conflicts between the custom entries and the policy
* entries.
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not
* try to remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder forceRemediateForFirstEntries(Boolean forceRemediateForFirstEntries);
/**
*
* The rules that you want to run last in the Firewall Manager managed network ACLs.
*
*
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule
* numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*
*
* @param lastEntries
* The rules that you want to run last in the Firewall Manager managed network ACLs.
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific
* rule numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lastEntries(Collection lastEntries);
/**
*
* The rules that you want to run last in the Firewall Manager managed network ACLs.
*
*
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule
* numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*
*
* @param lastEntries
* The rules that you want to run last in the Firewall Manager managed network ACLs.
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific
* rule numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lastEntries(NetworkAclEntry... lastEntries);
/**
*
* The rules that you want to run last in the Firewall Manager managed network ACLs.
*
*
*
* Provide these in the order in which you want them to run. Firewall Manager will assign the specific rule
* numbers for you, in the network ACLs that it creates.
*
*
*
* You must specify at least one first entry or one last entry in any network ACL policy.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry.Builder#build()} is called immediately and
* its result is passed to {@link #lastEntries(List)}.
*
* @param lastEntries
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.fms.model.NetworkAclEntry.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #lastEntries(java.util.Collection)
*/
Builder lastEntries(Consumer... lastEntries);
/**
*
* Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting when
* it finds policy violations that involve conflicts between the custom entries and the policy entries.
*
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not try to
* remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
*
*
* @param forceRemediateForLastEntries
* Applies only when remediation is enabled for the policy as a whole. Firewall Manager uses this setting
* when it finds policy violations that involve conflicts between the custom entries and the policy
* entries.
*
* If forced remediation is disabled, Firewall Manager marks the network ACL as noncompliant and does not
* try to remediate. For more information about the remediation behavior, see Remediation for managed network ACLs in the Firewall Manager Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder forceRemediateForLastEntries(Boolean forceRemediateForLastEntries);
}
static final class BuilderImpl implements Builder {
private List firstEntries = DefaultSdkAutoConstructList.getInstance();
private Boolean forceRemediateForFirstEntries;
private List lastEntries = DefaultSdkAutoConstructList.getInstance();
private Boolean forceRemediateForLastEntries;
private BuilderImpl() {
}
private BuilderImpl(NetworkAclEntrySet model) {
firstEntries(model.firstEntries);
forceRemediateForFirstEntries(model.forceRemediateForFirstEntries);
lastEntries(model.lastEntries);
forceRemediateForLastEntries(model.forceRemediateForLastEntries);
}
public final List getFirstEntries() {
List result = NetworkAclEntriesCopier.copyToBuilder(this.firstEntries);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setFirstEntries(Collection firstEntries) {
this.firstEntries = NetworkAclEntriesCopier.copyFromBuilder(firstEntries);
}
@Override
public final Builder firstEntries(Collection firstEntries) {
this.firstEntries = NetworkAclEntriesCopier.copy(firstEntries);
return this;
}
@Override
@SafeVarargs
public final Builder firstEntries(NetworkAclEntry... firstEntries) {
firstEntries(Arrays.asList(firstEntries));
return this;
}
@Override
@SafeVarargs
public final Builder firstEntries(Consumer... firstEntries) {
firstEntries(Stream.of(firstEntries).map(c -> NetworkAclEntry.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final Boolean getForceRemediateForFirstEntries() {
return forceRemediateForFirstEntries;
}
public final void setForceRemediateForFirstEntries(Boolean forceRemediateForFirstEntries) {
this.forceRemediateForFirstEntries = forceRemediateForFirstEntries;
}
@Override
public final Builder forceRemediateForFirstEntries(Boolean forceRemediateForFirstEntries) {
this.forceRemediateForFirstEntries = forceRemediateForFirstEntries;
return this;
}
public final List getLastEntries() {
List result = NetworkAclEntriesCopier.copyToBuilder(this.lastEntries);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setLastEntries(Collection lastEntries) {
this.lastEntries = NetworkAclEntriesCopier.copyFromBuilder(lastEntries);
}
@Override
public final Builder lastEntries(Collection lastEntries) {
this.lastEntries = NetworkAclEntriesCopier.copy(lastEntries);
return this;
}
@Override
@SafeVarargs
public final Builder lastEntries(NetworkAclEntry... lastEntries) {
lastEntries(Arrays.asList(lastEntries));
return this;
}
@Override
@SafeVarargs
public final Builder lastEntries(Consumer... lastEntries) {
lastEntries(Stream.of(lastEntries).map(c -> NetworkAclEntry.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final Boolean getForceRemediateForLastEntries() {
return forceRemediateForLastEntries;
}
public final void setForceRemediateForLastEntries(Boolean forceRemediateForLastEntries) {
this.forceRemediateForLastEntries = forceRemediateForLastEntries;
}
@Override
public final Builder forceRemediateForLastEntries(Boolean forceRemediateForLastEntries) {
this.forceRemediateForLastEntries = forceRemediateForLastEntries;
return this;
}
@Override
public NetworkAclEntrySet build() {
return new NetworkAclEntrySet(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}