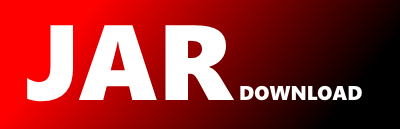
software.amazon.awssdk.services.fms.model.PolicySummary Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details of the Firewall Manager policy.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PolicySummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField POLICY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyArn").getter(getter(PolicySummary::policyArn)).setter(setter(Builder::policyArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyArn").build()).build();
private static final SdkField POLICY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyId").getter(getter(PolicySummary::policyId)).setter(setter(Builder::policyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyId").build()).build();
private static final SdkField POLICY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyName").getter(getter(PolicySummary::policyName)).setter(setter(Builder::policyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyName").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceType").getter(getter(PolicySummary::resourceType)).setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField SECURITY_SERVICE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecurityServiceType").getter(getter(PolicySummary::securityServiceTypeAsString))
.setter(setter(Builder::securityServiceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityServiceType").build())
.build();
private static final SdkField REMEDIATION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RemediationEnabled").getter(getter(PolicySummary::remediationEnabled))
.setter(setter(Builder::remediationEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RemediationEnabled").build())
.build();
private static final SdkField DELETE_UNUSED_FM_MANAGED_RESOURCES_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DeleteUnusedFMManagedResources")
.getter(getter(PolicySummary::deleteUnusedFMManagedResources))
.setter(setter(Builder::deleteUnusedFMManagedResources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeleteUnusedFMManagedResources")
.build()).build();
private static final SdkField POLICY_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PolicyStatus").getter(getter(PolicySummary::policyStatusAsString)).setter(setter(Builder::policyStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyStatus").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(POLICY_ARN_FIELD,
POLICY_ID_FIELD, POLICY_NAME_FIELD, RESOURCE_TYPE_FIELD, SECURITY_SERVICE_TYPE_FIELD, REMEDIATION_ENABLED_FIELD,
DELETE_UNUSED_FM_MANAGED_RESOURCES_FIELD, POLICY_STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("PolicyArn", POLICY_ARN_FIELD);
put("PolicyId", POLICY_ID_FIELD);
put("PolicyName", POLICY_NAME_FIELD);
put("ResourceType", RESOURCE_TYPE_FIELD);
put("SecurityServiceType", SECURITY_SERVICE_TYPE_FIELD);
put("RemediationEnabled", REMEDIATION_ENABLED_FIELD);
put("DeleteUnusedFMManagedResources", DELETE_UNUSED_FM_MANAGED_RESOURCES_FIELD);
put("PolicyStatus", POLICY_STATUS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String policyArn;
private final String policyId;
private final String policyName;
private final String resourceType;
private final String securityServiceType;
private final Boolean remediationEnabled;
private final Boolean deleteUnusedFMManagedResources;
private final String policyStatus;
private PolicySummary(BuilderImpl builder) {
this.policyArn = builder.policyArn;
this.policyId = builder.policyId;
this.policyName = builder.policyName;
this.resourceType = builder.resourceType;
this.securityServiceType = builder.securityServiceType;
this.remediationEnabled = builder.remediationEnabled;
this.deleteUnusedFMManagedResources = builder.deleteUnusedFMManagedResources;
this.policyStatus = builder.policyStatus;
}
/**
*
* The Amazon Resource Name (ARN) of the specified policy.
*
*
* @return The Amazon Resource Name (ARN) of the specified policy.
*/
public final String policyArn() {
return policyArn;
}
/**
*
* The ID of the specified policy.
*
*
* @return The ID of the specified policy.
*/
public final String policyId() {
return policyId;
}
/**
*
* The name of the specified policy.
*
*
* @return The name of the specified policy.
*/
public final String policyName() {
return policyName;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference.
*
*
* @return The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon Web Services Resource Types Reference.
*/
public final String resourceType() {
return resourceType;
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #securityServiceType} will return {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #securityServiceTypeAsString}.
*
*
* @return The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an WAF policy, a Shield Advanced policy, or a security group policy.
* @see SecurityServiceType
*/
public final SecurityServiceType securityServiceType() {
return SecurityServiceType.fromValue(securityServiceType);
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #securityServiceType} will return {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #securityServiceTypeAsString}.
*
*
* @return The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an WAF policy, a Shield Advanced policy, or a security group policy.
* @see SecurityServiceType
*/
public final String securityServiceTypeAsString() {
return securityServiceType;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @return Indicates if the policy should be automatically applied to new resources.
*/
public final Boolean remediationEnabled() {
return remediationEnabled;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @return Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*/
public final Boolean deleteUnusedFMManagedResources() {
return deleteUnusedFMManagedResources;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #policyStatus} will
* return {@link CustomerPolicyStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #policyStatusAsString}.
*
*
* @return Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete
* the policy. Existing policy protections stay in place. Any new resources that come into scope of the
* policy won't be protected.
*
*
* @see CustomerPolicyStatus
*/
public final CustomerPolicyStatus policyStatus() {
return CustomerPolicyStatus.fromValue(policyStatus);
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #policyStatus} will
* return {@link CustomerPolicyStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #policyStatusAsString}.
*
*
* @return Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete
* the policy. Existing policy protections stay in place. Any new resources that come into scope of the
* policy won't be protected.
*
*
* @see CustomerPolicyStatus
*/
public final String policyStatusAsString() {
return policyStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(policyArn());
hashCode = 31 * hashCode + Objects.hashCode(policyId());
hashCode = 31 * hashCode + Objects.hashCode(policyName());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(securityServiceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(remediationEnabled());
hashCode = 31 * hashCode + Objects.hashCode(deleteUnusedFMManagedResources());
hashCode = 31 * hashCode + Objects.hashCode(policyStatusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PolicySummary)) {
return false;
}
PolicySummary other = (PolicySummary) obj;
return Objects.equals(policyArn(), other.policyArn()) && Objects.equals(policyId(), other.policyId())
&& Objects.equals(policyName(), other.policyName()) && Objects.equals(resourceType(), other.resourceType())
&& Objects.equals(securityServiceTypeAsString(), other.securityServiceTypeAsString())
&& Objects.equals(remediationEnabled(), other.remediationEnabled())
&& Objects.equals(deleteUnusedFMManagedResources(), other.deleteUnusedFMManagedResources())
&& Objects.equals(policyStatusAsString(), other.policyStatusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PolicySummary").add("PolicyArn", policyArn()).add("PolicyId", policyId())
.add("PolicyName", policyName()).add("ResourceType", resourceType())
.add("SecurityServiceType", securityServiceTypeAsString()).add("RemediationEnabled", remediationEnabled())
.add("DeleteUnusedFMManagedResources", deleteUnusedFMManagedResources())
.add("PolicyStatus", policyStatusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PolicyArn":
return Optional.ofNullable(clazz.cast(policyArn()));
case "PolicyId":
return Optional.ofNullable(clazz.cast(policyId()));
case "PolicyName":
return Optional.ofNullable(clazz.cast(policyName()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "SecurityServiceType":
return Optional.ofNullable(clazz.cast(securityServiceTypeAsString()));
case "RemediationEnabled":
return Optional.ofNullable(clazz.cast(remediationEnabled()));
case "DeleteUnusedFMManagedResources":
return Optional.ofNullable(clazz.cast(deleteUnusedFMManagedResources()));
case "PolicyStatus":
return Optional.ofNullable(clazz.cast(policyStatusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function