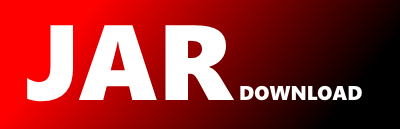
software.amazon.awssdk.services.fms.FmsClient Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.DeletePolicyRequest;
import software.amazon.awssdk.services.fms.model.DeletePolicyResponse;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.FmsException;
import software.amazon.awssdk.services.fms.model.GetAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.GetAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailRequest;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailResponse;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.GetPolicyRequest;
import software.amazon.awssdk.services.fms.model.GetPolicyResponse;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusRequest;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusResponse;
import software.amazon.awssdk.services.fms.model.InternalErrorException;
import software.amazon.awssdk.services.fms.model.InvalidInputException;
import software.amazon.awssdk.services.fms.model.InvalidOperationException;
import software.amazon.awssdk.services.fms.model.InvalidTypeException;
import software.amazon.awssdk.services.fms.model.LimitExceededException;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse;
import software.amazon.awssdk.services.fms.model.ListPoliciesRequest;
import software.amazon.awssdk.services.fms.model.ListPoliciesResponse;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.PutPolicyRequest;
import software.amazon.awssdk.services.fms.model.PutPolicyResponse;
import software.amazon.awssdk.services.fms.model.ResourceNotFoundException;
import software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable;
import software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable;
import software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable;
/**
* Service client for accessing FMS. This can be created using the static {@link #builder()} method.
*
* AWS Firewall Manager
*
* This is the AWS Firewall Manager API Reference. This guide is for developers who need detailed information
* about the AWS Firewall Manager API actions, data types, and errors. For detailed information about AWS Firewall
* Manager features, see the AWS
* Firewall Manager Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface FmsClient extends SdkClient {
String SERVICE_NAME = "fms";
/**
* Create a {@link FmsClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static FmsClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link FmsClient}.
*/
static FmsClientBuilder builder() {
return new DefaultFmsClientBuilder();
}
/**
*
* Sets the AWS Firewall Manager administrator account. AWS Firewall Manager must be associated with the master
* account your AWS organization or associated with a member account that has the appropriate permissions. If the
* account ID that you submit is not an AWS Organizations master account, AWS Firewall Manager will set the
* appropriate permissions for the given member account.
*
*
* The account that you associate with AWS Firewall Manager is called the AWS Firewall Manager administrator
* account.
*
*
* @param associateAdminAccountRequest
* @return Result of the AssociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.AssociateAdminAccount
* @see AWS API
* Documentation
*/
default AssociateAdminAccountResponse associateAdminAccount(AssociateAdminAccountRequest associateAdminAccountRequest)
throws InvalidOperationException, InvalidInputException, ResourceNotFoundException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the AWS Firewall Manager administrator account. AWS Firewall Manager must be associated with the master
* account your AWS organization or associated with a member account that has the appropriate permissions. If the
* account ID that you submit is not an AWS Organizations master account, AWS Firewall Manager will set the
* appropriate permissions for the given member account.
*
*
* The account that you associate with AWS Firewall Manager is called the AWS Firewall Manager administrator
* account.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateAdminAccountRequest.Builder} avoiding the
* need to create one manually via {@link AssociateAdminAccountRequest#builder()}
*
*
* @param associateAdminAccountRequest
* A {@link Consumer} that will call methods on {@link AssociateAdminAccountRequest.Builder} to create a
* request.
* @return Result of the AssociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.AssociateAdminAccount
* @see AWS API
* Documentation
*/
default AssociateAdminAccountResponse associateAdminAccount(
Consumer associateAdminAccountRequest) throws InvalidOperationException,
InvalidInputException, ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
return associateAdminAccount(AssociateAdminAccountRequest.builder().applyMutation(associateAdminAccountRequest).build());
}
/**
*
* Deletes an AWS Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS)
* topic that is used to record AWS Firewall Manager SNS logs.
*
*
* @param deleteNotificationChannelRequest
* @return Result of the DeleteNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
default DeleteNotificationChannelResponse deleteNotificationChannel(
DeleteNotificationChannelRequest deleteNotificationChannelRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an AWS Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS)
* topic that is used to record AWS Firewall Manager SNS logs.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNotificationChannelRequest.Builder} avoiding
* the need to create one manually via {@link DeleteNotificationChannelRequest#builder()}
*
*
* @param deleteNotificationChannelRequest
* A {@link Consumer} that will call methods on {@link DeleteNotificationChannelRequest.Builder} to create a
* request.
* @return Result of the DeleteNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
default DeleteNotificationChannelResponse deleteNotificationChannel(
Consumer deleteNotificationChannelRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return deleteNotificationChannel(DeleteNotificationChannelRequest.builder()
.applyMutation(deleteNotificationChannelRequest).build());
}
/**
*
* Permanently deletes an AWS Firewall Manager policy.
*
*
* @param deletePolicyRequest
* @return Result of the DeletePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeletePolicy
* @see AWS API
* Documentation
*/
default DeletePolicyResponse deletePolicy(DeletePolicyRequest deletePolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes an AWS Firewall Manager policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePolicyRequest.Builder} avoiding the need to
* create one manually via {@link DeletePolicyRequest#builder()}
*
*
* @param deletePolicyRequest
* A {@link Consumer} that will call methods on {@link DeletePolicyRequest.Builder} to create a request.
* @return Result of the DeletePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DeletePolicy
* @see AWS API
* Documentation
*/
default DeletePolicyResponse deletePolicy(Consumer deletePolicyRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return deletePolicy(DeletePolicyRequest.builder().applyMutation(deletePolicyRequest).build());
}
/**
*
* Disassociates the account that has been set as the AWS Firewall Manager administrator account. To set a different
* account as the administrator account, you must submit an AssociateAdminAccount
request .
*
*
* @param disassociateAdminAccountRequest
* @return Result of the DisassociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
default DisassociateAdminAccountResponse disassociateAdminAccount(
DisassociateAdminAccountRequest disassociateAdminAccountRequest) throws InvalidOperationException,
ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates the account that has been set as the AWS Firewall Manager administrator account. To set a different
* account as the administrator account, you must submit an AssociateAdminAccount
request .
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateAdminAccountRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateAdminAccountRequest#builder()}
*
*
* @param disassociateAdminAccountRequest
* A {@link Consumer} that will call methods on {@link DisassociateAdminAccountRequest.Builder} to create a
* request.
* @return Result of the DisassociateAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
default DisassociateAdminAccountResponse disassociateAdminAccount(
Consumer disassociateAdminAccountRequest) throws InvalidOperationException,
ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return disassociateAdminAccount(DisassociateAdminAccountRequest.builder().applyMutation(disassociateAdminAccountRequest)
.build());
}
/**
*
* Returns the AWS Organizations master account that is associated with AWS Firewall Manager as the AWS Firewall
* Manager administrator.
*
*
* @param getAdminAccountRequest
* @return Result of the GetAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAdminAccount
* @see AWS API
* Documentation
*/
default GetAdminAccountResponse getAdminAccount(GetAdminAccountRequest getAdminAccountRequest)
throws InvalidOperationException, ResourceNotFoundException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the AWS Organizations master account that is associated with AWS Firewall Manager as the AWS Firewall
* Manager administrator.
*
*
*
* This is a convenience which creates an instance of the {@link GetAdminAccountRequest.Builder} avoiding the need
* to create one manually via {@link GetAdminAccountRequest#builder()}
*
*
* @param getAdminAccountRequest
* A {@link Consumer} that will call methods on {@link GetAdminAccountRequest.Builder} to create a request.
* @return Result of the GetAdminAccount operation returned by the service.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetAdminAccount
* @see AWS API
* Documentation
*/
default GetAdminAccountResponse getAdminAccount(Consumer getAdminAccountRequest)
throws InvalidOperationException, ResourceNotFoundException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
return getAdminAccount(GetAdminAccountRequest.builder().applyMutation(getAdminAccountRequest).build());
}
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy. Resources are considered non-compliant if the specified policy
* has not been applied to them.
*
*
* @param getComplianceDetailRequest
* @return Result of the GetComplianceDetail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetComplianceDetail
* @see AWS API
* Documentation
*/
default GetComplianceDetailResponse getComplianceDetail(GetComplianceDetailRequest getComplianceDetailRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy. Resources are considered non-compliant if the specified policy
* has not been applied to them.
*
*
*
* This is a convenience which creates an instance of the {@link GetComplianceDetailRequest.Builder} avoiding the
* need to create one manually via {@link GetComplianceDetailRequest#builder()}
*
*
* @param getComplianceDetailRequest
* A {@link Consumer} that will call methods on {@link GetComplianceDetailRequest.Builder} to create a
* request.
* @return Result of the GetComplianceDetail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetComplianceDetail
* @see AWS API
* Documentation
*/
default GetComplianceDetailResponse getComplianceDetail(
Consumer getComplianceDetailRequest) throws ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return getComplianceDetail(GetComplianceDetailRequest.builder().applyMutation(getComplianceDetailRequest).build());
}
/**
*
* Returns information about the Amazon Simple Notification Service (SNS) topic that is used to record AWS Firewall
* Manager SNS logs.
*
*
* @param getNotificationChannelRequest
* @return Result of the GetNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetNotificationChannel
* @see AWS API
* Documentation
*/
default GetNotificationChannelResponse getNotificationChannel(GetNotificationChannelRequest getNotificationChannelRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the Amazon Simple Notification Service (SNS) topic that is used to record AWS Firewall
* Manager SNS logs.
*
*
*
* This is a convenience which creates an instance of the {@link GetNotificationChannelRequest.Builder} avoiding the
* need to create one manually via {@link GetNotificationChannelRequest#builder()}
*
*
* @param getNotificationChannelRequest
* A {@link Consumer} that will call methods on {@link GetNotificationChannelRequest.Builder} to create a
* request.
* @return Result of the GetNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetNotificationChannel
* @see AWS API
* Documentation
*/
default GetNotificationChannelResponse getNotificationChannel(
Consumer getNotificationChannelRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return getNotificationChannel(GetNotificationChannelRequest.builder().applyMutation(getNotificationChannelRequest)
.build());
}
/**
*
* Returns information about the specified AWS Firewall Manager policy.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetPolicy
* @see AWS API
* Documentation
*/
default GetPolicyResponse getPolicy(GetPolicyRequest getPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidTypeException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified AWS Firewall Manager policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetPolicyRequest#builder()}
*
*
* @param getPolicyRequest
* A {@link Consumer} that will call methods on {@link GetPolicyRequest.Builder} to create a request.
* @return Result of the GetPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetPolicy
* @see AWS API
* Documentation
*/
default GetPolicyResponse getPolicy(Consumer getPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, InvalidTypeException, AwsServiceException, SdkClientException,
FmsException {
return getPolicy(GetPolicyRequest.builder().applyMutation(getPolicyRequest).build());
}
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack.
*
*
* @param getProtectionStatusRequest
* @return Result of the GetProtectionStatus operation returned by the service.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetProtectionStatus
* @see AWS API
* Documentation
*/
default GetProtectionStatusResponse getProtectionStatus(GetProtectionStatusRequest getProtectionStatusRequest)
throws InvalidInputException, ResourceNotFoundException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack.
*
*
*
* This is a convenience which creates an instance of the {@link GetProtectionStatusRequest.Builder} avoiding the
* need to create one manually via {@link GetProtectionStatusRequest#builder()}
*
*
* @param getProtectionStatusRequest
* A {@link Consumer} that will call methods on {@link GetProtectionStatusRequest.Builder} to create a
* request.
* @return Result of the GetProtectionStatus operation returned by the service.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.GetProtectionStatus
* @see AWS API
* Documentation
*/
default GetProtectionStatusResponse getProtectionStatus(
Consumer getProtectionStatusRequest) throws InvalidInputException,
ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return getProtectionStatus(GetProtectionStatusRequest.builder().applyMutation(getProtectionStatusRequest).build());
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
* @param listComplianceStatusRequest
* @return Result of the ListComplianceStatus operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusResponse listComplianceStatus(ListComplianceStatusRequest listComplianceStatusRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
*
* This is a convenience which creates an instance of the {@link ListComplianceStatusRequest.Builder} avoiding the
* need to create one manually via {@link ListComplianceStatusRequest#builder()}
*
*
* @param listComplianceStatusRequest
* A {@link Consumer} that will call methods on {@link ListComplianceStatusRequest.Builder} to create a
* request.
* @return Result of the ListComplianceStatus operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusResponse listComplianceStatus(
Consumer listComplianceStatusRequest) throws ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listComplianceStatus(ListComplianceStatusRequest.builder().applyMutation(listComplianceStatusRequest).build());
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
*
* This is a variant of
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client
* .listComplianceStatusPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)}
* operation.
*
*
* @param listComplianceStatusRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusIterable listComplianceStatusPaginator(ListComplianceStatusRequest listComplianceStatusRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
*
* This is a variant of
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client
* .listComplianceStatusPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusIterable responses = client.listComplianceStatusPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListComplianceStatusRequest.Builder} avoiding the
* need to create one manually via {@link ListComplianceStatusRequest#builder()}
*
*
* @param listComplianceStatusRequest
* A {@link Consumer} that will call methods on {@link ListComplianceStatusRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusIterable listComplianceStatusPaginator(
Consumer listComplianceStatusRequest) throws ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listComplianceStatusPaginator(ListComplianceStatusRequest.builder().applyMutation(listComplianceStatusRequest)
.build());
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
* @param listMemberAccountsRequest
* @return Result of the ListMemberAccounts operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsResponse listMemberAccounts(ListMemberAccountsRequest listMemberAccountsRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
*
* This is a convenience which creates an instance of the {@link ListMemberAccountsRequest.Builder} avoiding the
* need to create one manually via {@link ListMemberAccountsRequest#builder()}
*
*
* @param listMemberAccountsRequest
* A {@link Consumer} that will call methods on {@link ListMemberAccountsRequest.Builder} to create a
* request.
* @return Result of the ListMemberAccounts operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsResponse listMemberAccounts(Consumer listMemberAccountsRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listMemberAccounts(ListMemberAccountsRequest.builder().applyMutation(listMemberAccountsRequest).build());
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
*
* This is a variant of
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client
* .listMemberAccountsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation.
*
*
* @param listMemberAccountsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsIterable listMemberAccountsPaginator(ListMemberAccountsRequest listMemberAccountsRequest)
throws ResourceNotFoundException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
*
* This is a variant of
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client
* .listMemberAccountsPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsIterable responses = client.listMemberAccountsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListMemberAccountsRequest.Builder} avoiding the
* need to create one manually via {@link ListMemberAccountsRequest#builder()}
*
*
* @param listMemberAccountsRequest
* A {@link Consumer} that will call methods on {@link ListMemberAccountsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsIterable listMemberAccountsPaginator(
Consumer listMemberAccountsRequest) throws ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return listMemberAccountsPaginator(ListMemberAccountsRequest.builder().applyMutation(listMemberAccountsRequest).build());
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
* @param listPoliciesRequest
* @return Result of the ListPolicies operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesResponse listPolicies(ListPoliciesRequest listPoliciesRequest) throws ResourceNotFoundException,
InvalidOperationException, LimitExceededException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListPoliciesRequest.Builder} to create a request.
* @return Result of the ListPolicies operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesResponse listPolicies(Consumer listPoliciesRequest)
throws ResourceNotFoundException, InvalidOperationException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listPolicies(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)} operation.
*
*
* @param listPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesIterable listPoliciesPaginator(ListPoliciesRequest listPoliciesRequest) throws ResourceNotFoundException,
InvalidOperationException, LimitExceededException, InternalErrorException, AwsServiceException, SdkClientException,
FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* for (software.amazon.awssdk.services.fms.model.ListPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListPoliciesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesIterable listPoliciesPaginator(Consumer listPoliciesRequest)
throws ResourceNotFoundException, InvalidOperationException, LimitExceededException, InternalErrorException,
AwsServiceException, SdkClientException, FmsException {
return listPoliciesPaginator(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that AWS Firewall Manager uses to
* record SNS logs.
*
*
* @param putNotificationChannelRequest
* @return Result of the PutNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutNotificationChannel
* @see AWS API
* Documentation
*/
default PutNotificationChannelResponse putNotificationChannel(PutNotificationChannelRequest putNotificationChannelRequest)
throws ResourceNotFoundException, InvalidOperationException, InternalErrorException, AwsServiceException,
SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that AWS Firewall Manager uses to
* record SNS logs.
*
*
*
* This is a convenience which creates an instance of the {@link PutNotificationChannelRequest.Builder} avoiding the
* need to create one manually via {@link PutNotificationChannelRequest#builder()}
*
*
* @param putNotificationChannelRequest
* A {@link Consumer} that will call methods on {@link PutNotificationChannelRequest.Builder} to create a
* request.
* @return Result of the PutNotificationChannel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutNotificationChannel
* @see AWS API
* Documentation
*/
default PutNotificationChannelResponse putNotificationChannel(
Consumer putNotificationChannelRequest) throws ResourceNotFoundException,
InvalidOperationException, InternalErrorException, AwsServiceException, SdkClientException, FmsException {
return putNotificationChannel(PutNotificationChannelRequest.builder().applyMutation(putNotificationChannelRequest)
.build());
}
/**
*
* Creates an AWS Firewall Manager policy.
*
*
* Firewall Manager provides two types of policies: A Shield Advanced policy, which applies Shield Advanced
* protection to specified accounts and resources, or a WAF policy, which contains a rule group and defines which
* resources are to be protected by that rule group. A policy is specific to either WAF or Shield Advanced. If you
* want to enforce both WAF rules and Shield Advanced protection across accounts, you can create multiple policies.
* You can create one or more policies for WAF rules, and one or more policies for Shield Advanced.
*
*
* You must be subscribed to Shield Advanced to create a Shield Advanced policy. For more information on subscribing
* to Shield Advanced, see CreateSubscription.
*
*
* @param putPolicyRequest
* @return Result of the PutPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutPolicy
* @see AWS API
* Documentation
*/
default PutPolicyResponse putPolicy(PutPolicyRequest putPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InvalidInputException, LimitExceededException, InternalErrorException,
InvalidTypeException, AwsServiceException, SdkClientException, FmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an AWS Firewall Manager policy.
*
*
* Firewall Manager provides two types of policies: A Shield Advanced policy, which applies Shield Advanced
* protection to specified accounts and resources, or a WAF policy, which contains a rule group and defines which
* resources are to be protected by that rule group. A policy is specific to either WAF or Shield Advanced. If you
* want to enforce both WAF rules and Shield Advanced protection across accounts, you can create multiple policies.
* You can create one or more policies for WAF rules, and one or more policies for Shield Advanced.
*
*
* You must be subscribed to Shield Advanced to create a Shield Advanced policy. For more information on subscribing
* to Shield Advanced, see CreateSubscription.
*
*
*
* This is a convenience which creates an instance of the {@link PutPolicyRequest.Builder} avoiding the need to
* create one manually via {@link PutPolicyRequest#builder()}
*
*
* @param putPolicyRequest
* A {@link Consumer} that will call methods on {@link PutPolicyRequest.Builder} to create a request.
* @return Result of the PutPolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidOperationException
* The operation failed because there was nothing to do. For example, you might have submitted an
* AssociateAdminAccount
request, but the account ID that you submitted was already set as the
* AWS Firewall Manager administrator.
* @throws InvalidInputException
* The parameters of the request were invalid.
* @throws LimitExceededException
* The operation exceeds a resource limit, for example, the maximum number of policy
objects
* that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* @throws InternalErrorException
* The operation failed because of a system problem, even though the request was valid. Retry your request.
* @throws InvalidTypeException
* The value of the Type
parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws FmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample FmsClient.PutPolicy
* @see AWS API
* Documentation
*/
default PutPolicyResponse putPolicy(Consumer putPolicyRequest) throws ResourceNotFoundException,
InvalidOperationException, InvalidInputException, LimitExceededException, InternalErrorException,
InvalidTypeException, AwsServiceException, SdkClientException, FmsException {
return putPolicy(PutPolicyRequest.builder().applyMutation(putPolicyRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("fms");
}
}