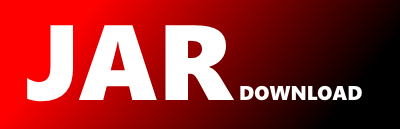
software.amazon.awssdk.services.forecast.model.DatasetSummary Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.forecast.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides a summary of the dataset properties used in the ListDatasets operation. To get the complete set of
* properties, call the DescribeDataset operation, and provide the listed DatasetArn
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DatasetSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DATASET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DatasetSummary::datasetArn)).setter(setter(Builder::datasetArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatasetArn").build()).build();
private static final SdkField DATASET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DatasetSummary::datasetName)).setter(setter(Builder::datasetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatasetName").build()).build();
private static final SdkField DATASET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DatasetSummary::datasetTypeAsString)).setter(setter(Builder::datasetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatasetType").build()).build();
private static final SdkField DOMAIN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DatasetSummary::domainAsString)).setter(setter(Builder::domain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Domain").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(DatasetSummary::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField LAST_MODIFICATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(DatasetSummary::lastModificationTime)).setter(setter(Builder::lastModificationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModificationTime").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATASET_ARN_FIELD,
DATASET_NAME_FIELD, DATASET_TYPE_FIELD, DOMAIN_FIELD, CREATION_TIME_FIELD, LAST_MODIFICATION_TIME_FIELD));
private static final long serialVersionUID = 1L;
private final String datasetArn;
private final String datasetName;
private final String datasetType;
private final String domain;
private final Instant creationTime;
private final Instant lastModificationTime;
private DatasetSummary(BuilderImpl builder) {
this.datasetArn = builder.datasetArn;
this.datasetName = builder.datasetName;
this.datasetType = builder.datasetType;
this.domain = builder.domain;
this.creationTime = builder.creationTime;
this.lastModificationTime = builder.lastModificationTime;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset.
*
*
* @return The Amazon Resource Name (ARN) of the dataset.
*/
public String datasetArn() {
return datasetArn;
}
/**
*
* The name of the dataset.
*
*
* @return The name of the dataset.
*/
public String datasetName() {
return datasetName;
}
/**
*
* The dataset type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #datasetType} will
* return {@link DatasetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #datasetTypeAsString}.
*
*
* @return The dataset type.
* @see DatasetType
*/
public DatasetType datasetType() {
return DatasetType.fromValue(datasetType);
}
/**
*
* The dataset type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #datasetType} will
* return {@link DatasetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #datasetTypeAsString}.
*
*
* @return The dataset type.
* @see DatasetType
*/
public String datasetTypeAsString() {
return datasetType;
}
/**
*
* The domain associated with the dataset.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #domain} will
* return {@link Domain#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #domainAsString}.
*
*
* @return The domain associated with the dataset.
* @see Domain
*/
public Domain domain() {
return Domain.fromValue(domain);
}
/**
*
* The domain associated with the dataset.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #domain} will
* return {@link Domain#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #domainAsString}.
*
*
* @return The domain associated with the dataset.
* @see Domain
*/
public String domainAsString() {
return domain;
}
/**
*
* When the dataset was created.
*
*
* @return When the dataset was created.
*/
public Instant creationTime() {
return creationTime;
}
/**
*
* When the dataset is created, LastModificationTime
is the same as CreationTime
. After a
* CreateDatasetImportJob operation is called, LastModificationTime
is when the import job
* finished or failed. While data is being imported to the dataset, LastModificationTime
is the current
* query time.
*
*
* @return When the dataset is created, LastModificationTime
is the same as CreationTime
.
* After a CreateDatasetImportJob operation is called, LastModificationTime
is when the
* import job finished or failed. While data is being imported to the dataset,
* LastModificationTime
is the current query time.
*/
public Instant lastModificationTime() {
return lastModificationTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(datasetArn());
hashCode = 31 * hashCode + Objects.hashCode(datasetName());
hashCode = 31 * hashCode + Objects.hashCode(datasetTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(domainAsString());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModificationTime());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DatasetSummary)) {
return false;
}
DatasetSummary other = (DatasetSummary) obj;
return Objects.equals(datasetArn(), other.datasetArn()) && Objects.equals(datasetName(), other.datasetName())
&& Objects.equals(datasetTypeAsString(), other.datasetTypeAsString())
&& Objects.equals(domainAsString(), other.domainAsString())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastModificationTime(), other.lastModificationTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DatasetSummary").add("DatasetArn", datasetArn()).add("DatasetName", datasetName())
.add("DatasetType", datasetTypeAsString()).add("Domain", domainAsString()).add("CreationTime", creationTime())
.add("LastModificationTime", lastModificationTime()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DatasetArn":
return Optional.ofNullable(clazz.cast(datasetArn()));
case "DatasetName":
return Optional.ofNullable(clazz.cast(datasetName()));
case "DatasetType":
return Optional.ofNullable(clazz.cast(datasetTypeAsString()));
case "Domain":
return Optional.ofNullable(clazz.cast(domainAsString()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "LastModificationTime":
return Optional.ofNullable(clazz.cast(lastModificationTime()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function