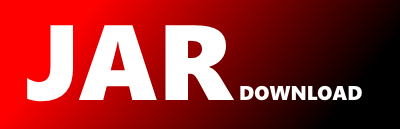
software.amazon.awssdk.services.glacier.model.DescribeJobResponse Maven / Gradle / Ivy
Show all versions of glacier Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glacier.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the description of an Amazon S3 Glacier job.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeJobResponse extends GlacierResponse implements
ToCopyableBuilder {
private static final SdkField JOB_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("JobId")
.getter(getter(DescribeJobResponse::jobId)).setter(setter(Builder::jobId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobId").build()).build();
private static final SdkField JOB_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobDescription").getter(getter(DescribeJobResponse::jobDescription))
.setter(setter(Builder::jobDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobDescription").build()).build();
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Action")
.getter(getter(DescribeJobResponse::actionAsString)).setter(setter(Builder::action))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField ARCHIVE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ArchiveId").getter(getter(DescribeJobResponse::archiveId)).setter(setter(Builder::archiveId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ArchiveId").build()).build();
private static final SdkField VAULT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VaultARN").getter(getter(DescribeJobResponse::vaultARN)).setter(setter(Builder::vaultARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VaultARN").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreationDate").getter(getter(DescribeJobResponse::creationDate)).setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationDate").build()).build();
private static final SdkField COMPLETED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Completed").getter(getter(DescribeJobResponse::completed)).setter(setter(Builder::completed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Completed").build()).build();
private static final SdkField STATUS_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusCode").getter(getter(DescribeJobResponse::statusCodeAsString)).setter(setter(Builder::statusCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusCode").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusMessage").getter(getter(DescribeJobResponse::statusMessage))
.setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage").build()).build();
private static final SdkField ARCHIVE_SIZE_IN_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ArchiveSizeInBytes").getter(getter(DescribeJobResponse::archiveSizeInBytes))
.setter(setter(Builder::archiveSizeInBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ArchiveSizeInBytes").build())
.build();
private static final SdkField INVENTORY_SIZE_IN_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("InventorySizeInBytes").getter(getter(DescribeJobResponse::inventorySizeInBytes))
.setter(setter(Builder::inventorySizeInBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InventorySizeInBytes").build())
.build();
private static final SdkField SNS_TOPIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SNSTopic").getter(getter(DescribeJobResponse::snsTopic)).setter(setter(Builder::snsTopic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SNSTopic").build()).build();
private static final SdkField COMPLETION_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompletionDate").getter(getter(DescribeJobResponse::completionDate))
.setter(setter(Builder::completionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletionDate").build()).build();
private static final SdkField SHA256_TREE_HASH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SHA256TreeHash").getter(getter(DescribeJobResponse::sha256TreeHash))
.setter(setter(Builder::sha256TreeHash))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SHA256TreeHash").build()).build();
private static final SdkField ARCHIVE_SHA256_TREE_HASH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ArchiveSHA256TreeHash").getter(getter(DescribeJobResponse::archiveSHA256TreeHash))
.setter(setter(Builder::archiveSHA256TreeHash))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ArchiveSHA256TreeHash").build())
.build();
private static final SdkField RETRIEVAL_BYTE_RANGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RetrievalByteRange").getter(getter(DescribeJobResponse::retrievalByteRange))
.setter(setter(Builder::retrievalByteRange))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetrievalByteRange").build())
.build();
private static final SdkField TIER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Tier")
.getter(getter(DescribeJobResponse::tier)).setter(setter(Builder::tier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tier").build()).build();
private static final SdkField INVENTORY_RETRIEVAL_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("InventoryRetrievalParameters")
.getter(getter(DescribeJobResponse::inventoryRetrievalParameters))
.setter(setter(Builder::inventoryRetrievalParameters))
.constructor(InventoryRetrievalJobDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InventoryRetrievalParameters")
.build()).build();
private static final SdkField JOB_OUTPUT_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobOutputPath").getter(getter(DescribeJobResponse::jobOutputPath))
.setter(setter(Builder::jobOutputPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobOutputPath").build()).build();
private static final SdkField SELECT_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SelectParameters")
.getter(getter(DescribeJobResponse::selectParameters)).setter(setter(Builder::selectParameters))
.constructor(SelectParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SelectParameters").build()).build();
private static final SdkField OUTPUT_LOCATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OutputLocation")
.getter(getter(DescribeJobResponse::outputLocation)).setter(setter(Builder::outputLocation))
.constructor(OutputLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputLocation").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(JOB_ID_FIELD,
JOB_DESCRIPTION_FIELD, ACTION_FIELD, ARCHIVE_ID_FIELD, VAULT_ARN_FIELD, CREATION_DATE_FIELD, COMPLETED_FIELD,
STATUS_CODE_FIELD, STATUS_MESSAGE_FIELD, ARCHIVE_SIZE_IN_BYTES_FIELD, INVENTORY_SIZE_IN_BYTES_FIELD, SNS_TOPIC_FIELD,
COMPLETION_DATE_FIELD, SHA256_TREE_HASH_FIELD, ARCHIVE_SHA256_TREE_HASH_FIELD, RETRIEVAL_BYTE_RANGE_FIELD,
TIER_FIELD, INVENTORY_RETRIEVAL_PARAMETERS_FIELD, JOB_OUTPUT_PATH_FIELD, SELECT_PARAMETERS_FIELD,
OUTPUT_LOCATION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String jobId;
private final String jobDescription;
private final String action;
private final String archiveId;
private final String vaultARN;
private final String creationDate;
private final Boolean completed;
private final String statusCode;
private final String statusMessage;
private final Long archiveSizeInBytes;
private final Long inventorySizeInBytes;
private final String snsTopic;
private final String completionDate;
private final String sha256TreeHash;
private final String archiveSHA256TreeHash;
private final String retrievalByteRange;
private final String tier;
private final InventoryRetrievalJobDescription inventoryRetrievalParameters;
private final String jobOutputPath;
private final SelectParameters selectParameters;
private final OutputLocation outputLocation;
private DescribeJobResponse(BuilderImpl builder) {
super(builder);
this.jobId = builder.jobId;
this.jobDescription = builder.jobDescription;
this.action = builder.action;
this.archiveId = builder.archiveId;
this.vaultARN = builder.vaultARN;
this.creationDate = builder.creationDate;
this.completed = builder.completed;
this.statusCode = builder.statusCode;
this.statusMessage = builder.statusMessage;
this.archiveSizeInBytes = builder.archiveSizeInBytes;
this.inventorySizeInBytes = builder.inventorySizeInBytes;
this.snsTopic = builder.snsTopic;
this.completionDate = builder.completionDate;
this.sha256TreeHash = builder.sha256TreeHash;
this.archiveSHA256TreeHash = builder.archiveSHA256TreeHash;
this.retrievalByteRange = builder.retrievalByteRange;
this.tier = builder.tier;
this.inventoryRetrievalParameters = builder.inventoryRetrievalParameters;
this.jobOutputPath = builder.jobOutputPath;
this.selectParameters = builder.selectParameters;
this.outputLocation = builder.outputLocation;
}
/**
*
* An opaque string that identifies an Amazon S3 Glacier job.
*
*
* @return An opaque string that identifies an Amazon S3 Glacier job.
*/
public final String jobId() {
return jobId;
}
/**
*
* The job description provided when initiating the job.
*
*
* @return The job description provided when initiating the job.
*/
public final String jobDescription() {
return jobDescription;
}
/**
*
* The job type. This value is either ArchiveRetrieval
, InventoryRetrieval
, or
* Select
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link ActionCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #actionAsString}.
*
*
* @return The job type. This value is either ArchiveRetrieval
, InventoryRetrieval
, or
* Select
.
* @see ActionCode
*/
public final ActionCode action() {
return ActionCode.fromValue(action);
}
/**
*
* The job type. This value is either ArchiveRetrieval
, InventoryRetrieval
, or
* Select
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link ActionCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #actionAsString}.
*
*
* @return The job type. This value is either ArchiveRetrieval
, InventoryRetrieval
, or
* Select
.
* @see ActionCode
*/
public final String actionAsString() {
return action;
}
/**
*
* The archive ID requested for a select job or archive retrieval. Otherwise, this field is null.
*
*
* @return The archive ID requested for a select job or archive retrieval. Otherwise, this field is null.
*/
public final String archiveId() {
return archiveId;
}
/**
*
* The Amazon Resource Name (ARN) of the vault from which an archive retrieval was requested.
*
*
* @return The Amazon Resource Name (ARN) of the vault from which an archive retrieval was requested.
*/
public final String vaultARN() {
return vaultARN;
}
/**
*
* The UTC date when the job was created. This value is a string representation of ISO 8601 date format, for example
* "2012-03-20T17:03:43.221Z"
.
*
*
* @return The UTC date when the job was created. This value is a string representation of ISO 8601 date format, for
* example "2012-03-20T17:03:43.221Z"
.
*/
public final String creationDate() {
return creationDate;
}
/**
*
* The job status. When a job is completed, you get the job's output using Get Job Output (GET output).
*
*
* @return The job status. When a job is completed, you get the job's output using Get Job Output (GET output).
*/
public final Boolean completed() {
return completed;
}
/**
*
* The status code can be InProgress
, Succeeded
, or Failed
, and indicates the
* status of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statusCode} will
* return {@link StatusCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusCodeAsString}.
*
*
* @return The status code can be InProgress
, Succeeded
, or Failed
, and
* indicates the status of the job.
* @see StatusCode
*/
public final StatusCode statusCode() {
return StatusCode.fromValue(statusCode);
}
/**
*
* The status code can be InProgress
, Succeeded
, or Failed
, and indicates the
* status of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statusCode} will
* return {@link StatusCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusCodeAsString}.
*
*
* @return The status code can be InProgress
, Succeeded
, or Failed
, and
* indicates the status of the job.
* @see StatusCode
*/
public final String statusCodeAsString() {
return statusCode;
}
/**
*
* A friendly message that describes the job status.
*
*
* @return A friendly message that describes the job status.
*/
public final String statusMessage() {
return statusMessage;
}
/**
*
* For an archive retrieval job, this value is the size in bytes of the archive being requested for download. For an
* inventory retrieval or select job, this value is null.
*
*
* @return For an archive retrieval job, this value is the size in bytes of the archive being requested for
* download. For an inventory retrieval or select job, this value is null.
*/
public final Long archiveSizeInBytes() {
return archiveSizeInBytes;
}
/**
*
* For an inventory retrieval job, this value is the size in bytes of the inventory requested for download. For an
* archive retrieval or select job, this value is null.
*
*
* @return For an inventory retrieval job, this value is the size in bytes of the inventory requested for download.
* For an archive retrieval or select job, this value is null.
*/
public final Long inventorySizeInBytes() {
return inventorySizeInBytes;
}
/**
*
* An Amazon SNS topic that receives notification.
*
*
* @return An Amazon SNS topic that receives notification.
*/
public final String snsTopic() {
return snsTopic;
}
/**
*
* The UTC time that the job request completed. While the job is in progress, the value is null.
*
*
* @return The UTC time that the job request completed. While the job is in progress, the value is null.
*/
public final String completionDate() {
return completionDate;
}
/**
*
* For an archive retrieval job, this value is the checksum of the archive. Otherwise, this value is null.
*
*
* The SHA256 tree hash value for the requested range of an archive. If the InitiateJob request for an
* archive specified a tree-hash aligned range, then this field returns a value.
*
*
* If the whole archive is retrieved, this value is the same as the ArchiveSHA256TreeHash value.
*
*
* This field is null for the following:
*
*
* -
*
* Archive retrieval jobs that specify a range that is not tree-hash aligned
*
*
*
*
* -
*
* Archival jobs that specify a range that is equal to the whole archive, when the job status is
* InProgress
*
*
*
*
* -
*
* Inventory jobs
*
*
* -
*
* Select jobs
*
*
*
*
* @return For an archive retrieval job, this value is the checksum of the archive. Otherwise, this value is
* null.
*
* The SHA256 tree hash value for the requested range of an archive. If the InitiateJob request for
* an archive specified a tree-hash aligned range, then this field returns a value.
*
*
* If the whole archive is retrieved, this value is the same as the ArchiveSHA256TreeHash value.
*
*
* This field is null for the following:
*
*
* -
*
* Archive retrieval jobs that specify a range that is not tree-hash aligned
*
*
*
*
* -
*
* Archival jobs that specify a range that is equal to the whole archive, when the job status is
* InProgress
*
*
*
*
* -
*
* Inventory jobs
*
*
* -
*
* Select jobs
*
*
*/
public final String sha256TreeHash() {
return sha256TreeHash;
}
/**
*
* The SHA256 tree hash of the entire archive for an archive retrieval. For inventory retrieval or select jobs, this
* field is null.
*
*
* @return The SHA256 tree hash of the entire archive for an archive retrieval. For inventory retrieval or select
* jobs, this field is null.
*/
public final String archiveSHA256TreeHash() {
return archiveSHA256TreeHash;
}
/**
*
* The retrieved byte range for archive retrieval jobs in the form StartByteValue-EndByteValue. If no
* range was specified in the archive retrieval, then the whole archive is retrieved. In this case,
* StartByteValue equals 0 and EndByteValue equals the size of the archive minus 1. For inventory
* retrieval or select jobs, this field is null.
*
*
* @return The retrieved byte range for archive retrieval jobs in the form
* StartByteValue-EndByteValue. If no range was specified in the archive retrieval, then the
* whole archive is retrieved. In this case, StartByteValue equals 0 and EndByteValue equals
* the size of the archive minus 1. For inventory retrieval or select jobs, this field is null.
*/
public final String retrievalByteRange() {
return retrievalByteRange;
}
/**
*
* The tier to use for a select or an archive retrieval. Valid values are Expedited
,
* Standard
, or Bulk
. Standard
is the default.
*
*
* @return The tier to use for a select or an archive retrieval. Valid values are Expedited
,
* Standard
, or Bulk
. Standard
is the default.
*/
public final String tier() {
return tier;
}
/**
*
* Parameters used for range inventory retrieval.
*
*
* @return Parameters used for range inventory retrieval.
*/
public final InventoryRetrievalJobDescription inventoryRetrievalParameters() {
return inventoryRetrievalParameters;
}
/**
*
* Contains the job output location.
*
*
* @return Contains the job output location.
*/
public final String jobOutputPath() {
return jobOutputPath;
}
/**
*
* Contains the parameters used for a select.
*
*
* @return Contains the parameters used for a select.
*/
public final SelectParameters selectParameters() {
return selectParameters;
}
/**
*
* Contains the location where the data from the select job is stored.
*
*
* @return Contains the location where the data from the select job is stored.
*/
public final OutputLocation outputLocation() {
return outputLocation;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(jobId());
hashCode = 31 * hashCode + Objects.hashCode(jobDescription());
hashCode = 31 * hashCode + Objects.hashCode(actionAsString());
hashCode = 31 * hashCode + Objects.hashCode(archiveId());
hashCode = 31 * hashCode + Objects.hashCode(vaultARN());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(completed());
hashCode = 31 * hashCode + Objects.hashCode(statusCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(archiveSizeInBytes());
hashCode = 31 * hashCode + Objects.hashCode(inventorySizeInBytes());
hashCode = 31 * hashCode + Objects.hashCode(snsTopic());
hashCode = 31 * hashCode + Objects.hashCode(completionDate());
hashCode = 31 * hashCode + Objects.hashCode(sha256TreeHash());
hashCode = 31 * hashCode + Objects.hashCode(archiveSHA256TreeHash());
hashCode = 31 * hashCode + Objects.hashCode(retrievalByteRange());
hashCode = 31 * hashCode + Objects.hashCode(tier());
hashCode = 31 * hashCode + Objects.hashCode(inventoryRetrievalParameters());
hashCode = 31 * hashCode + Objects.hashCode(jobOutputPath());
hashCode = 31 * hashCode + Objects.hashCode(selectParameters());
hashCode = 31 * hashCode + Objects.hashCode(outputLocation());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeJobResponse)) {
return false;
}
DescribeJobResponse other = (DescribeJobResponse) obj;
return Objects.equals(jobId(), other.jobId()) && Objects.equals(jobDescription(), other.jobDescription())
&& Objects.equals(actionAsString(), other.actionAsString()) && Objects.equals(archiveId(), other.archiveId())
&& Objects.equals(vaultARN(), other.vaultARN()) && Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(completed(), other.completed())
&& Objects.equals(statusCodeAsString(), other.statusCodeAsString())
&& Objects.equals(statusMessage(), other.statusMessage())
&& Objects.equals(archiveSizeInBytes(), other.archiveSizeInBytes())
&& Objects.equals(inventorySizeInBytes(), other.inventorySizeInBytes())
&& Objects.equals(snsTopic(), other.snsTopic()) && Objects.equals(completionDate(), other.completionDate())
&& Objects.equals(sha256TreeHash(), other.sha256TreeHash())
&& Objects.equals(archiveSHA256TreeHash(), other.archiveSHA256TreeHash())
&& Objects.equals(retrievalByteRange(), other.retrievalByteRange()) && Objects.equals(tier(), other.tier())
&& Objects.equals(inventoryRetrievalParameters(), other.inventoryRetrievalParameters())
&& Objects.equals(jobOutputPath(), other.jobOutputPath())
&& Objects.equals(selectParameters(), other.selectParameters())
&& Objects.equals(outputLocation(), other.outputLocation());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeJobResponse").add("JobId", jobId()).add("JobDescription", jobDescription())
.add("Action", actionAsString()).add("ArchiveId", archiveId()).add("VaultARN", vaultARN())
.add("CreationDate", creationDate()).add("Completed", completed()).add("StatusCode", statusCodeAsString())
.add("StatusMessage", statusMessage()).add("ArchiveSizeInBytes", archiveSizeInBytes())
.add("InventorySizeInBytes", inventorySizeInBytes()).add("SNSTopic", snsTopic())
.add("CompletionDate", completionDate()).add("SHA256TreeHash", sha256TreeHash())
.add("ArchiveSHA256TreeHash", archiveSHA256TreeHash()).add("RetrievalByteRange", retrievalByteRange())
.add("Tier", tier()).add("InventoryRetrievalParameters", inventoryRetrievalParameters())
.add("JobOutputPath", jobOutputPath()).add("SelectParameters", selectParameters())
.add("OutputLocation", outputLocation()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "JobId":
return Optional.ofNullable(clazz.cast(jobId()));
case "JobDescription":
return Optional.ofNullable(clazz.cast(jobDescription()));
case "Action":
return Optional.ofNullable(clazz.cast(actionAsString()));
case "ArchiveId":
return Optional.ofNullable(clazz.cast(archiveId()));
case "VaultARN":
return Optional.ofNullable(clazz.cast(vaultARN()));
case "CreationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "Completed":
return Optional.ofNullable(clazz.cast(completed()));
case "StatusCode":
return Optional.ofNullable(clazz.cast(statusCodeAsString()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "ArchiveSizeInBytes":
return Optional.ofNullable(clazz.cast(archiveSizeInBytes()));
case "InventorySizeInBytes":
return Optional.ofNullable(clazz.cast(inventorySizeInBytes()));
case "SNSTopic":
return Optional.ofNullable(clazz.cast(snsTopic()));
case "CompletionDate":
return Optional.ofNullable(clazz.cast(completionDate()));
case "SHA256TreeHash":
return Optional.ofNullable(clazz.cast(sha256TreeHash()));
case "ArchiveSHA256TreeHash":
return Optional.ofNullable(clazz.cast(archiveSHA256TreeHash()));
case "RetrievalByteRange":
return Optional.ofNullable(clazz.cast(retrievalByteRange()));
case "Tier":
return Optional.ofNullable(clazz.cast(tier()));
case "InventoryRetrievalParameters":
return Optional.ofNullable(clazz.cast(inventoryRetrievalParameters()));
case "JobOutputPath":
return Optional.ofNullable(clazz.cast(jobOutputPath()));
case "SelectParameters":
return Optional.ofNullable(clazz.cast(selectParameters()));
case "OutputLocation":
return Optional.ofNullable(clazz.cast(outputLocation()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("JobId", JOB_ID_FIELD);
map.put("JobDescription", JOB_DESCRIPTION_FIELD);
map.put("Action", ACTION_FIELD);
map.put("ArchiveId", ARCHIVE_ID_FIELD);
map.put("VaultARN", VAULT_ARN_FIELD);
map.put("CreationDate", CREATION_DATE_FIELD);
map.put("Completed", COMPLETED_FIELD);
map.put("StatusCode", STATUS_CODE_FIELD);
map.put("StatusMessage", STATUS_MESSAGE_FIELD);
map.put("ArchiveSizeInBytes", ARCHIVE_SIZE_IN_BYTES_FIELD);
map.put("InventorySizeInBytes", INVENTORY_SIZE_IN_BYTES_FIELD);
map.put("SNSTopic", SNS_TOPIC_FIELD);
map.put("CompletionDate", COMPLETION_DATE_FIELD);
map.put("SHA256TreeHash", SHA256_TREE_HASH_FIELD);
map.put("ArchiveSHA256TreeHash", ARCHIVE_SHA256_TREE_HASH_FIELD);
map.put("RetrievalByteRange", RETRIEVAL_BYTE_RANGE_FIELD);
map.put("Tier", TIER_FIELD);
map.put("InventoryRetrievalParameters", INVENTORY_RETRIEVAL_PARAMETERS_FIELD);
map.put("JobOutputPath", JOB_OUTPUT_PATH_FIELD);
map.put("SelectParameters", SELECT_PARAMETERS_FIELD);
map.put("OutputLocation", OUTPUT_LOCATION_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function