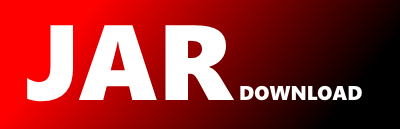
software.amazon.awssdk.services.globalaccelerator.GlobalAcceleratorClient Maven / Gradle / Ivy
Show all versions of globalaccelerator Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.globalaccelerator;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.globalaccelerator.model.AcceleratorNotDisabledException;
import software.amazon.awssdk.services.globalaccelerator.model.AcceleratorNotFoundException;
import software.amazon.awssdk.services.globalaccelerator.model.AccessDeniedException;
import software.amazon.awssdk.services.globalaccelerator.model.AdvertiseByoipCidrRequest;
import software.amazon.awssdk.services.globalaccelerator.model.AdvertiseByoipCidrResponse;
import software.amazon.awssdk.services.globalaccelerator.model.AssociatedEndpointGroupFoundException;
import software.amazon.awssdk.services.globalaccelerator.model.AssociatedListenerFoundException;
import software.amazon.awssdk.services.globalaccelerator.model.ByoipCidrNotFoundException;
import software.amazon.awssdk.services.globalaccelerator.model.CreateAcceleratorRequest;
import software.amazon.awssdk.services.globalaccelerator.model.CreateAcceleratorResponse;
import software.amazon.awssdk.services.globalaccelerator.model.CreateEndpointGroupRequest;
import software.amazon.awssdk.services.globalaccelerator.model.CreateEndpointGroupResponse;
import software.amazon.awssdk.services.globalaccelerator.model.CreateListenerRequest;
import software.amazon.awssdk.services.globalaccelerator.model.CreateListenerResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DeleteAcceleratorRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DeleteAcceleratorResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DeleteEndpointGroupRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DeleteEndpointGroupResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DeleteListenerRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DeleteListenerResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DeprovisionByoipCidrRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DeprovisionByoipCidrResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeAcceleratorAttributesRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeAcceleratorAttributesResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeAcceleratorRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeAcceleratorResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeEndpointGroupRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeEndpointGroupResponse;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeListenerRequest;
import software.amazon.awssdk.services.globalaccelerator.model.DescribeListenerResponse;
import software.amazon.awssdk.services.globalaccelerator.model.EndpointGroupAlreadyExistsException;
import software.amazon.awssdk.services.globalaccelerator.model.EndpointGroupNotFoundException;
import software.amazon.awssdk.services.globalaccelerator.model.GlobalAcceleratorException;
import software.amazon.awssdk.services.globalaccelerator.model.IncorrectCidrStateException;
import software.amazon.awssdk.services.globalaccelerator.model.InternalServiceErrorException;
import software.amazon.awssdk.services.globalaccelerator.model.InvalidArgumentException;
import software.amazon.awssdk.services.globalaccelerator.model.InvalidNextTokenException;
import software.amazon.awssdk.services.globalaccelerator.model.InvalidPortRangeException;
import software.amazon.awssdk.services.globalaccelerator.model.LimitExceededException;
import software.amazon.awssdk.services.globalaccelerator.model.ListAcceleratorsRequest;
import software.amazon.awssdk.services.globalaccelerator.model.ListAcceleratorsResponse;
import software.amazon.awssdk.services.globalaccelerator.model.ListByoipCidrsRequest;
import software.amazon.awssdk.services.globalaccelerator.model.ListByoipCidrsResponse;
import software.amazon.awssdk.services.globalaccelerator.model.ListEndpointGroupsRequest;
import software.amazon.awssdk.services.globalaccelerator.model.ListEndpointGroupsResponse;
import software.amazon.awssdk.services.globalaccelerator.model.ListListenersRequest;
import software.amazon.awssdk.services.globalaccelerator.model.ListListenersResponse;
import software.amazon.awssdk.services.globalaccelerator.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.globalaccelerator.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.globalaccelerator.model.ListenerNotFoundException;
import software.amazon.awssdk.services.globalaccelerator.model.ProvisionByoipCidrRequest;
import software.amazon.awssdk.services.globalaccelerator.model.ProvisionByoipCidrResponse;
import software.amazon.awssdk.services.globalaccelerator.model.TagResourceRequest;
import software.amazon.awssdk.services.globalaccelerator.model.TagResourceResponse;
import software.amazon.awssdk.services.globalaccelerator.model.UntagResourceRequest;
import software.amazon.awssdk.services.globalaccelerator.model.UntagResourceResponse;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateAcceleratorAttributesRequest;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateAcceleratorAttributesResponse;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateAcceleratorRequest;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateAcceleratorResponse;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateEndpointGroupRequest;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateEndpointGroupResponse;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateListenerRequest;
import software.amazon.awssdk.services.globalaccelerator.model.UpdateListenerResponse;
import software.amazon.awssdk.services.globalaccelerator.model.WithdrawByoipCidrRequest;
import software.amazon.awssdk.services.globalaccelerator.model.WithdrawByoipCidrResponse;
/**
* Service client for accessing AWS Global Accelerator. This can be created using the static {@link #builder()} method.
*
* AWS Global Accelerator
*
* This is the AWS Global Accelerator API Reference. This guide is for developers who need detailed information
* about AWS Global Accelerator API actions, data types, and errors. For more information about Global Accelerator
* features, see the AWS Global
* Accelerator Developer Guide.
*
*
* AWS Global Accelerator is a service in which you create accelerators to improve availability and performance of your
* applications for local and global users.
*
*
*
* You must specify the US West (Oregon) Region to create or update accelerators.
*
*
*
* By default, Global Accelerator provides you with static IP addresses that you associate with your accelerator.
* (Instead of using the IP addresses that Global Accelerator provides, you can configure these entry points to be IPv4
* addresses from your own IP address ranges that you bring to Global Accelerator.) The static IP addresses are anycast
* from the AWS edge network and distribute incoming application traffic across multiple endpoint resources in multiple
* AWS Regions, which increases the availability of your applications. Endpoints can be Network Load Balancers,
* Application Load Balancers, EC2 instances, or Elastic IP addresses that are located in one AWS Region or multiple
* Regions.
*
*
* Global Accelerator uses the AWS global network to route traffic to the optimal regional endpoint based on health,
* client location, and policies that you configure. The service reacts instantly to changes in health or configuration
* to ensure that internet traffic from clients is directed to only healthy endpoints.
*
*
* Global Accelerator includes components that work together to help you improve performance and availability for your
* applications:
*
*
* - Static IP address
* -
*
* By default, AWS Global Accelerator provides you with a set of static IP addresses that are anycast from the AWS edge
* network and serve as the single fixed entry points for your clients. Or you can configure these entry points to be
* IPv4 addresses from your own IP address ranges that you bring to Global Accelerator (BYOIP). For more information,
* see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide. If you already have load balancers, EC2 instances,
* or Elastic IP addresses set up for your applications, you can easily add those to Global Accelerator to allow the
* resources to be accessed by the static IP addresses.
*
*
*
* The static IP addresses remain assigned to your accelerator for as long as it exists, even if you disable the
* accelerator and it no longer accepts or routes traffic. However, when you delete an accelerator, you lose the
* static IP addresses that are assigned to it, so you can no longer route traffic by using them. You can use IAM
* policies with Global Accelerator to limit the users who have permissions to delete an accelerator. For more
* information, see Authentication and
* Access Control in the AWS Global Accelerator Developer Guide.
*
*
* - Accelerator
* -
*
* An accelerator directs traffic to optimal endpoints over the AWS global network to improve availability and
* performance for your internet applications that have a global audience. Each accelerator includes one or more
* listeners.
*
*
* - DNS name
* -
*
* Global Accelerator assigns each accelerator a default Domain Name System (DNS) name, similar to
* a1234567890abcdef.awsglobalaccelerator.com
, that points to your Global Accelerator static IP addresses.
* Depending on the use case, you can use your accelerator's static IP addresses or DNS name to route traffic to your
* accelerator, or set up DNS records to route traffic using your own custom domain name.
*
*
* - Network zone
* -
*
* A network zone services the static IP addresses for your accelerator from a unique IP subnet. Similar to an AWS
* Availability Zone, a network zone is an isolated unit with its own set of physical infrastructure. When you configure
* an accelerator, by default, Global Accelerator allocates two IPv4 addresses for it. If one IP address from a network
* zone becomes unavailable due to IP address blocking by certain client networks, or network disruptions, then client
* applications can retry on the healthy static IP address from the other isolated network zone.
*
*
* - Listener
* -
*
* A listener processes inbound connections from clients to Global Accelerator, based on the protocol and port that you
* configure. Each listener has one or more endpoint groups associated with it, and traffic is forwarded to endpoints in
* one of the groups. You associate endpoint groups with listeners by specifying the Regions that you want to distribute
* traffic to. Traffic is distributed to optimal endpoints within the endpoint groups associated with a listener.
*
*
* - Endpoint group
* -
*
* Each endpoint group is associated with a specific AWS Region. Endpoint groups include one or more endpoints in the
* Region. You can increase or reduce the percentage of traffic that would be otherwise directed to an endpoint group by
* adjusting a setting called a traffic dial. The traffic dial lets you easily do performance testing or
* blue/green deployment testing for new releases across different AWS Regions, for example.
*
*
* - Endpoint
* -
*
* An endpoint is a Network Load Balancer, Application Load Balancer, EC2 instance, or Elastic IP address. Traffic is
* routed to endpoints based on several factors, including the geo-proximity to the user, the health of the endpoint,
* and the configuration options that you choose, such as endpoint weights. For each endpoint, you can configure
* weights, which are numbers that you can use to specify the proportion of traffic to route to each one. This can be
* useful, for example, to do performance testing within a Region.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface GlobalAcceleratorClient extends SdkClient {
String SERVICE_NAME = "globalaccelerator";
/**
* Create a {@link GlobalAcceleratorClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static GlobalAcceleratorClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link GlobalAcceleratorClient}.
*/
static GlobalAcceleratorClientBuilder builder() {
return new DefaultGlobalAcceleratorClientBuilder();
}
/**
*
* Advertises an IPv4 address range that is provisioned for use with your AWS resources through bring your own IP
* addresses (BYOIP). It can take a few minutes before traffic to the specified addresses starts routing to AWS
* because of propagation delays. To see an AWS CLI example of advertising an address range, scroll down to
* Example.
*
*
* To stop advertising the BYOIP address range, use WithdrawByoipCidr.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
* @param advertiseByoipCidrRequest
* @return Result of the AdvertiseByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws ByoipCidrNotFoundException
* The CIDR that you specified was not found or is incorrect.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.AdvertiseByoipCidr
* @see AWS API Documentation
*/
default AdvertiseByoipCidrResponse advertiseByoipCidr(AdvertiseByoipCidrRequest advertiseByoipCidrRequest)
throws InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, ByoipCidrNotFoundException,
IncorrectCidrStateException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Advertises an IPv4 address range that is provisioned for use with your AWS resources through bring your own IP
* addresses (BYOIP). It can take a few minutes before traffic to the specified addresses starts routing to AWS
* because of propagation delays. To see an AWS CLI example of advertising an address range, scroll down to
* Example.
*
*
* To stop advertising the BYOIP address range, use WithdrawByoipCidr.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AdvertiseByoipCidrRequest.Builder} avoiding the
* need to create one manually via {@link AdvertiseByoipCidrRequest#builder()}
*
*
* @param advertiseByoipCidrRequest
* A {@link Consumer} that will call methods on {@link AdvertiseByoipCidrRequest.Builder} to create a
* request.
* @return Result of the AdvertiseByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws ByoipCidrNotFoundException
* The CIDR that you specified was not found or is incorrect.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.AdvertiseByoipCidr
* @see AWS API Documentation
*/
default AdvertiseByoipCidrResponse advertiseByoipCidr(Consumer advertiseByoipCidrRequest)
throws InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, ByoipCidrNotFoundException,
IncorrectCidrStateException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
return advertiseByoipCidr(AdvertiseByoipCidrRequest.builder().applyMutation(advertiseByoipCidrRequest).build());
}
/**
*
* Create an accelerator. An accelerator includes one or more listeners that process inbound connections and direct
* traffic to one or more endpoint groups, each of which includes endpoints, such as Network Load Balancers. To see
* an AWS CLI example of creating an accelerator, scroll down to Example.
*
*
* If you bring your own IP address ranges to AWS Global Accelerator (BYOIP), you can assign IP addresses from your
* own pool to your accelerator as the static IP address entry points. Only one IP address from each of your IP
* address ranges can be used for each accelerator.
*
*
*
* You must specify the US West (Oregon) Region to create or update accelerators.
*
*
*
* @param createAcceleratorRequest
* @return Result of the CreateAccelerator operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.CreateAccelerator
* @see AWS API Documentation
*/
default CreateAcceleratorResponse createAccelerator(CreateAcceleratorRequest createAcceleratorRequest)
throws InternalServiceErrorException, InvalidArgumentException, LimitExceededException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Create an accelerator. An accelerator includes one or more listeners that process inbound connections and direct
* traffic to one or more endpoint groups, each of which includes endpoints, such as Network Load Balancers. To see
* an AWS CLI example of creating an accelerator, scroll down to Example.
*
*
* If you bring your own IP address ranges to AWS Global Accelerator (BYOIP), you can assign IP addresses from your
* own pool to your accelerator as the static IP address entry points. Only one IP address from each of your IP
* address ranges can be used for each accelerator.
*
*
*
* You must specify the US West (Oregon) Region to create or update accelerators.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAcceleratorRequest.Builder} avoiding the need
* to create one manually via {@link CreateAcceleratorRequest#builder()}
*
*
* @param createAcceleratorRequest
* A {@link Consumer} that will call methods on {@link CreateAcceleratorRequest.Builder} to create a request.
* @return Result of the CreateAccelerator operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.CreateAccelerator
* @see AWS API Documentation
*/
default CreateAcceleratorResponse createAccelerator(Consumer createAcceleratorRequest)
throws InternalServiceErrorException, InvalidArgumentException, LimitExceededException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
return createAccelerator(CreateAcceleratorRequest.builder().applyMutation(createAcceleratorRequest).build());
}
/**
*
* Create an endpoint group for the specified listener. An endpoint group is a collection of endpoints in one AWS
* Region. To see an AWS CLI example of creating an endpoint group, scroll down to Example.
*
*
* @param createEndpointGroupRequest
* @return Result of the CreateEndpointGroup operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws EndpointGroupAlreadyExistsException
* The endpoint group that you specified already exists.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws AccessDeniedException
* You don't have access permission.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.CreateEndpointGroup
* @see AWS API Documentation
*/
default CreateEndpointGroupResponse createEndpointGroup(CreateEndpointGroupRequest createEndpointGroupRequest)
throws AcceleratorNotFoundException, EndpointGroupAlreadyExistsException, ListenerNotFoundException,
InternalServiceErrorException, InvalidArgumentException, LimitExceededException, AccessDeniedException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Create an endpoint group for the specified listener. An endpoint group is a collection of endpoints in one AWS
* Region. To see an AWS CLI example of creating an endpoint group, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link CreateEndpointGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateEndpointGroupRequest#builder()}
*
*
* @param createEndpointGroupRequest
* A {@link Consumer} that will call methods on {@link CreateEndpointGroupRequest.Builder} to create a
* request.
* @return Result of the CreateEndpointGroup operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws EndpointGroupAlreadyExistsException
* The endpoint group that you specified already exists.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws AccessDeniedException
* You don't have access permission.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.CreateEndpointGroup
* @see AWS API Documentation
*/
default CreateEndpointGroupResponse createEndpointGroup(
Consumer createEndpointGroupRequest) throws AcceleratorNotFoundException,
EndpointGroupAlreadyExistsException, ListenerNotFoundException, InternalServiceErrorException,
InvalidArgumentException, LimitExceededException, AccessDeniedException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
return createEndpointGroup(CreateEndpointGroupRequest.builder().applyMutation(createEndpointGroupRequest).build());
}
/**
*
* Create a listener to process inbound connections from clients to an accelerator. Connections arrive to assigned
* static IP addresses on a port, port range, or list of port ranges that you specify. To see an AWS CLI example of
* creating a listener, scroll down to Example.
*
*
* @param createListenerRequest
* @return Result of the CreateListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InvalidPortRangeException
* The port numbers that you specified are not valid numbers or are not unique for this accelerator.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.CreateListener
* @see AWS API Documentation
*/
default CreateListenerResponse createListener(CreateListenerRequest createListenerRequest) throws InvalidArgumentException,
AcceleratorNotFoundException, InvalidPortRangeException, InternalServiceErrorException, LimitExceededException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Create a listener to process inbound connections from clients to an accelerator. Connections arrive to assigned
* static IP addresses on a port, port range, or list of port ranges that you specify. To see an AWS CLI example of
* creating a listener, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link CreateListenerRequest.Builder} avoiding the need to
* create one manually via {@link CreateListenerRequest#builder()}
*
*
* @param createListenerRequest
* A {@link Consumer} that will call methods on {@link CreateListenerRequest.Builder} to create a request.
* @return Result of the CreateListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InvalidPortRangeException
* The port numbers that you specified are not valid numbers or are not unique for this accelerator.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.CreateListener
* @see AWS API Documentation
*/
default CreateListenerResponse createListener(Consumer createListenerRequest)
throws InvalidArgumentException, AcceleratorNotFoundException, InvalidPortRangeException,
InternalServiceErrorException, LimitExceededException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
return createListener(CreateListenerRequest.builder().applyMutation(createListenerRequest).build());
}
/**
*
* Delete an accelerator. Before you can delete an accelerator, you must disable it and remove all dependent
* resources (listeners and endpoint groups). To disable the accelerator, update the accelerator to set
* Enabled
to false.
*
*
*
* When you create an accelerator, by default, Global Accelerator provides you with a set of two static IP
* addresses. Alternatively, you can bring your own IP address ranges to Global Accelerator and assign IP addresses
* from those ranges.
*
*
* The IP addresses are assigned to your accelerator for as long as it exists, even if you disable the accelerator
* and it no longer accepts or routes traffic. However, when you delete an accelerator, you lose the static
* IP addresses that are assigned to the accelerator, so you can no longer route traffic by using them. As a best
* practice, ensure that you have permissions in place to avoid inadvertently deleting accelerators. You can use IAM
* policies with Global Accelerator to limit the users who have permissions to delete an accelerator. For more
* information, see Authentication and
* Access Control in the AWS Global Accelerator Developer Guide.
*
*
*
* @param deleteAcceleratorRequest
* @return Result of the DeleteAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws AcceleratorNotDisabledException
* The accelerator that you specified could not be disabled.
* @throws AssociatedListenerFoundException
* The accelerator that you specified has a listener associated with it. You must remove all dependent
* resources from an accelerator before you can delete it.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeleteAccelerator
* @see AWS API Documentation
*/
default DeleteAcceleratorResponse deleteAccelerator(DeleteAcceleratorRequest deleteAcceleratorRequest)
throws AcceleratorNotFoundException, AcceleratorNotDisabledException, AssociatedListenerFoundException,
InternalServiceErrorException, InvalidArgumentException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Delete an accelerator. Before you can delete an accelerator, you must disable it and remove all dependent
* resources (listeners and endpoint groups). To disable the accelerator, update the accelerator to set
* Enabled
to false.
*
*
*
* When you create an accelerator, by default, Global Accelerator provides you with a set of two static IP
* addresses. Alternatively, you can bring your own IP address ranges to Global Accelerator and assign IP addresses
* from those ranges.
*
*
* The IP addresses are assigned to your accelerator for as long as it exists, even if you disable the accelerator
* and it no longer accepts or routes traffic. However, when you delete an accelerator, you lose the static
* IP addresses that are assigned to the accelerator, so you can no longer route traffic by using them. As a best
* practice, ensure that you have permissions in place to avoid inadvertently deleting accelerators. You can use IAM
* policies with Global Accelerator to limit the users who have permissions to delete an accelerator. For more
* information, see Authentication and
* Access Control in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAcceleratorRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAcceleratorRequest#builder()}
*
*
* @param deleteAcceleratorRequest
* A {@link Consumer} that will call methods on {@link DeleteAcceleratorRequest.Builder} to create a request.
* @return Result of the DeleteAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws AcceleratorNotDisabledException
* The accelerator that you specified could not be disabled.
* @throws AssociatedListenerFoundException
* The accelerator that you specified has a listener associated with it. You must remove all dependent
* resources from an accelerator before you can delete it.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeleteAccelerator
* @see AWS API Documentation
*/
default DeleteAcceleratorResponse deleteAccelerator(Consumer deleteAcceleratorRequest)
throws AcceleratorNotFoundException, AcceleratorNotDisabledException, AssociatedListenerFoundException,
InternalServiceErrorException, InvalidArgumentException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
return deleteAccelerator(DeleteAcceleratorRequest.builder().applyMutation(deleteAcceleratorRequest).build());
}
/**
*
* Delete an endpoint group from a listener.
*
*
* @param deleteEndpointGroupRequest
* @return Result of the DeleteEndpointGroup operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeleteEndpointGroup
* @see AWS API Documentation
*/
default DeleteEndpointGroupResponse deleteEndpointGroup(DeleteEndpointGroupRequest deleteEndpointGroupRequest)
throws InvalidArgumentException, EndpointGroupNotFoundException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Delete an endpoint group from a listener.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEndpointGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteEndpointGroupRequest#builder()}
*
*
* @param deleteEndpointGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteEndpointGroupRequest.Builder} to create a
* request.
* @return Result of the DeleteEndpointGroup operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeleteEndpointGroup
* @see AWS API Documentation
*/
default DeleteEndpointGroupResponse deleteEndpointGroup(
Consumer deleteEndpointGroupRequest) throws InvalidArgumentException,
EndpointGroupNotFoundException, InternalServiceErrorException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
return deleteEndpointGroup(DeleteEndpointGroupRequest.builder().applyMutation(deleteEndpointGroupRequest).build());
}
/**
*
* Delete a listener from an accelerator.
*
*
* @param deleteListenerRequest
* @return Result of the DeleteListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws AssociatedEndpointGroupFoundException
* The listener that you specified has an endpoint group associated with it. You must remove all dependent
* resources from a listener before you can delete it.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeleteListener
* @see AWS API Documentation
*/
default DeleteListenerResponse deleteListener(DeleteListenerRequest deleteListenerRequest) throws InvalidArgumentException,
ListenerNotFoundException, AssociatedEndpointGroupFoundException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Delete a listener from an accelerator.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteListenerRequest.Builder} avoiding the need to
* create one manually via {@link DeleteListenerRequest#builder()}
*
*
* @param deleteListenerRequest
* A {@link Consumer} that will call methods on {@link DeleteListenerRequest.Builder} to create a request.
* @return Result of the DeleteListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws AssociatedEndpointGroupFoundException
* The listener that you specified has an endpoint group associated with it. You must remove all dependent
* resources from a listener before you can delete it.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeleteListener
* @see AWS API Documentation
*/
default DeleteListenerResponse deleteListener(Consumer deleteListenerRequest)
throws InvalidArgumentException, ListenerNotFoundException, AssociatedEndpointGroupFoundException,
InternalServiceErrorException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
return deleteListener(DeleteListenerRequest.builder().applyMutation(deleteListenerRequest).build());
}
/**
*
* Releases the specified address range that you provisioned to use with your AWS resources through bring your own
* IP addresses (BYOIP) and deletes the corresponding address pool. To see an AWS CLI example of deprovisioning an
* address range, scroll down to Example.
*
*
* Before you can release an address range, you must stop advertising it by using WithdrawByoipCidr and
* you must not have any accelerators that are using static IP addresses allocated from its address range.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
* @param deprovisionByoipCidrRequest
* @return Result of the DeprovisionByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws ByoipCidrNotFoundException
* The CIDR that you specified was not found or is incorrect.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeprovisionByoipCidr
* @see AWS API Documentation
*/
default DeprovisionByoipCidrResponse deprovisionByoipCidr(DeprovisionByoipCidrRequest deprovisionByoipCidrRequest)
throws InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, ByoipCidrNotFoundException,
IncorrectCidrStateException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Releases the specified address range that you provisioned to use with your AWS resources through bring your own
* IP addresses (BYOIP) and deletes the corresponding address pool. To see an AWS CLI example of deprovisioning an
* address range, scroll down to Example.
*
*
* Before you can release an address range, you must stop advertising it by using WithdrawByoipCidr and
* you must not have any accelerators that are using static IP addresses allocated from its address range.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeprovisionByoipCidrRequest.Builder} avoiding the
* need to create one manually via {@link DeprovisionByoipCidrRequest#builder()}
*
*
* @param deprovisionByoipCidrRequest
* A {@link Consumer} that will call methods on {@link DeprovisionByoipCidrRequest.Builder} to create a
* request.
* @return Result of the DeprovisionByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws ByoipCidrNotFoundException
* The CIDR that you specified was not found or is incorrect.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DeprovisionByoipCidr
* @see AWS API Documentation
*/
default DeprovisionByoipCidrResponse deprovisionByoipCidr(
Consumer deprovisionByoipCidrRequest) throws InternalServiceErrorException,
InvalidArgumentException, AccessDeniedException, ByoipCidrNotFoundException, IncorrectCidrStateException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
return deprovisionByoipCidr(DeprovisionByoipCidrRequest.builder().applyMutation(deprovisionByoipCidrRequest).build());
}
/**
*
* Describe an accelerator. To see an AWS CLI example of describing an accelerator, scroll down to Example.
*
*
* @param describeAcceleratorRequest
* @return Result of the DescribeAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeAccelerator
* @see AWS API Documentation
*/
default DescribeAcceleratorResponse describeAccelerator(DescribeAcceleratorRequest describeAcceleratorRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Describe an accelerator. To see an AWS CLI example of describing an accelerator, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAcceleratorRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAcceleratorRequest#builder()}
*
*
* @param describeAcceleratorRequest
* A {@link Consumer} that will call methods on {@link DescribeAcceleratorRequest.Builder} to create a
* request.
* @return Result of the DescribeAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeAccelerator
* @see AWS API Documentation
*/
default DescribeAcceleratorResponse describeAccelerator(
Consumer describeAcceleratorRequest) throws AcceleratorNotFoundException,
InternalServiceErrorException, InvalidArgumentException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
return describeAccelerator(DescribeAcceleratorRequest.builder().applyMutation(describeAcceleratorRequest).build());
}
/**
*
* Describe the attributes of an accelerator. To see an AWS CLI example of describing the attributes of an
* accelerator, scroll down to Example.
*
*
* @param describeAcceleratorAttributesRequest
* @return Result of the DescribeAcceleratorAttributes operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeAcceleratorAttributes
* @see AWS API Documentation
*/
default DescribeAcceleratorAttributesResponse describeAcceleratorAttributes(
DescribeAcceleratorAttributesRequest describeAcceleratorAttributesRequest) throws AcceleratorNotFoundException,
InternalServiceErrorException, InvalidArgumentException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Describe the attributes of an accelerator. To see an AWS CLI example of describing the attributes of an
* accelerator, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAcceleratorAttributesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeAcceleratorAttributesRequest#builder()}
*
*
* @param describeAcceleratorAttributesRequest
* A {@link Consumer} that will call methods on {@link DescribeAcceleratorAttributesRequest.Builder} to
* create a request.
* @return Result of the DescribeAcceleratorAttributes operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeAcceleratorAttributes
* @see AWS API Documentation
*/
default DescribeAcceleratorAttributesResponse describeAcceleratorAttributes(
Consumer describeAcceleratorAttributesRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
return describeAcceleratorAttributes(DescribeAcceleratorAttributesRequest.builder()
.applyMutation(describeAcceleratorAttributesRequest).build());
}
/**
*
* Describe an endpoint group. To see an AWS CLI example of describing an endpoint group, scroll down to
* Example.
*
*
* @param describeEndpointGroupRequest
* @return Result of the DescribeEndpointGroup operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeEndpointGroup
* @see AWS API Documentation
*/
default DescribeEndpointGroupResponse describeEndpointGroup(DescribeEndpointGroupRequest describeEndpointGroupRequest)
throws InvalidArgumentException, EndpointGroupNotFoundException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Describe an endpoint group. To see an AWS CLI example of describing an endpoint group, scroll down to
* Example.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEndpointGroupRequest.Builder} avoiding the
* need to create one manually via {@link DescribeEndpointGroupRequest#builder()}
*
*
* @param describeEndpointGroupRequest
* A {@link Consumer} that will call methods on {@link DescribeEndpointGroupRequest.Builder} to create a
* request.
* @return Result of the DescribeEndpointGroup operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeEndpointGroup
* @see AWS API Documentation
*/
default DescribeEndpointGroupResponse describeEndpointGroup(
Consumer describeEndpointGroupRequest) throws InvalidArgumentException,
EndpointGroupNotFoundException, InternalServiceErrorException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
return describeEndpointGroup(DescribeEndpointGroupRequest.builder().applyMutation(describeEndpointGroupRequest).build());
}
/**
*
* Describe a listener. To see an AWS CLI example of describing a listener, scroll down to Example.
*
*
* @param describeListenerRequest
* @return Result of the DescribeListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeListener
* @see AWS API Documentation
*/
default DescribeListenerResponse describeListener(DescribeListenerRequest describeListenerRequest)
throws InvalidArgumentException, ListenerNotFoundException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Describe a listener. To see an AWS CLI example of describing a listener, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeListenerRequest.Builder} avoiding the need
* to create one manually via {@link DescribeListenerRequest#builder()}
*
*
* @param describeListenerRequest
* A {@link Consumer} that will call methods on {@link DescribeListenerRequest.Builder} to create a request.
* @return Result of the DescribeListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.DescribeListener
* @see AWS API Documentation
*/
default DescribeListenerResponse describeListener(Consumer describeListenerRequest)
throws InvalidArgumentException, ListenerNotFoundException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
return describeListener(DescribeListenerRequest.builder().applyMutation(describeListenerRequest).build());
}
/**
*
* List the accelerators for an AWS account. To see an AWS CLI example of listing the accelerators for an AWS
* account, scroll down to Example.
*
*
* @return Result of the ListAccelerators operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListAccelerators
* @see #listAccelerators(ListAcceleratorsRequest)
* @see AWS API Documentation
*/
default ListAcceleratorsResponse listAccelerators() throws InvalidArgumentException, InvalidNextTokenException,
InternalServiceErrorException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
return listAccelerators(ListAcceleratorsRequest.builder().build());
}
/**
*
* List the accelerators for an AWS account. To see an AWS CLI example of listing the accelerators for an AWS
* account, scroll down to Example.
*
*
* @param listAcceleratorsRequest
* @return Result of the ListAccelerators operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListAccelerators
* @see AWS API Documentation
*/
default ListAcceleratorsResponse listAccelerators(ListAcceleratorsRequest listAcceleratorsRequest)
throws InvalidArgumentException, InvalidNextTokenException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* List the accelerators for an AWS account. To see an AWS CLI example of listing the accelerators for an AWS
* account, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link ListAcceleratorsRequest.Builder} avoiding the need
* to create one manually via {@link ListAcceleratorsRequest#builder()}
*
*
* @param listAcceleratorsRequest
* A {@link Consumer} that will call methods on {@link ListAcceleratorsRequest.Builder} to create a request.
* @return Result of the ListAccelerators operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListAccelerators
* @see AWS API Documentation
*/
default ListAcceleratorsResponse listAccelerators(Consumer listAcceleratorsRequest)
throws InvalidArgumentException, InvalidNextTokenException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
return listAccelerators(ListAcceleratorsRequest.builder().applyMutation(listAcceleratorsRequest).build());
}
/**
*
* Lists the IP address ranges that were specified in calls to ProvisionByoipCidr,
* including the current state and a history of state changes.
*
*
* To see an AWS CLI example of listing BYOIP CIDR addresses, scroll down to Example.
*
*
* @param listByoipCidrsRequest
* @return Result of the ListByoipCidrs operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListByoipCidrs
* @see AWS API Documentation
*/
default ListByoipCidrsResponse listByoipCidrs(ListByoipCidrsRequest listByoipCidrsRequest)
throws InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, InvalidNextTokenException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IP address ranges that were specified in calls to ProvisionByoipCidr,
* including the current state and a history of state changes.
*
*
* To see an AWS CLI example of listing BYOIP CIDR addresses, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link ListByoipCidrsRequest.Builder} avoiding the need to
* create one manually via {@link ListByoipCidrsRequest#builder()}
*
*
* @param listByoipCidrsRequest
* A {@link Consumer} that will call methods on {@link ListByoipCidrsRequest.Builder} to create a request.
* @return Result of the ListByoipCidrs operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListByoipCidrs
* @see AWS API Documentation
*/
default ListByoipCidrsResponse listByoipCidrs(Consumer listByoipCidrsRequest)
throws InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, InvalidNextTokenException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
return listByoipCidrs(ListByoipCidrsRequest.builder().applyMutation(listByoipCidrsRequest).build());
}
/**
*
* List the endpoint groups that are associated with a listener. To see an AWS CLI example of listing the endpoint
* groups for listener, scroll down to Example.
*
*
* @param listEndpointGroupsRequest
* @return Result of the ListEndpointGroups operation returned by the service.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListEndpointGroups
* @see AWS API Documentation
*/
default ListEndpointGroupsResponse listEndpointGroups(ListEndpointGroupsRequest listEndpointGroupsRequest)
throws ListenerNotFoundException, InvalidNextTokenException, InvalidArgumentException, InternalServiceErrorException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* List the endpoint groups that are associated with a listener. To see an AWS CLI example of listing the endpoint
* groups for listener, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link ListEndpointGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListEndpointGroupsRequest#builder()}
*
*
* @param listEndpointGroupsRequest
* A {@link Consumer} that will call methods on {@link ListEndpointGroupsRequest.Builder} to create a
* request.
* @return Result of the ListEndpointGroups operation returned by the service.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListEndpointGroups
* @see AWS API Documentation
*/
default ListEndpointGroupsResponse listEndpointGroups(Consumer listEndpointGroupsRequest)
throws ListenerNotFoundException, InvalidNextTokenException, InvalidArgumentException, InternalServiceErrorException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
return listEndpointGroups(ListEndpointGroupsRequest.builder().applyMutation(listEndpointGroupsRequest).build());
}
/**
*
* List the listeners for an accelerator. To see an AWS CLI example of listing the listeners for an accelerator,
* scroll down to Example.
*
*
* @param listListenersRequest
* @return Result of the ListListeners operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListListeners
* @see AWS API Documentation
*/
default ListListenersResponse listListeners(ListListenersRequest listListenersRequest) throws InvalidArgumentException,
AcceleratorNotFoundException, InvalidNextTokenException, InternalServiceErrorException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* List the listeners for an accelerator. To see an AWS CLI example of listing the listeners for an accelerator,
* scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link ListListenersRequest.Builder} avoiding the need to
* create one manually via {@link ListListenersRequest#builder()}
*
*
* @param listListenersRequest
* A {@link Consumer} that will call methods on {@link ListListenersRequest.Builder} to create a request.
* @return Result of the ListListeners operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListListeners
* @see AWS API Documentation
*/
default ListListenersResponse listListeners(Consumer listListenersRequest)
throws InvalidArgumentException, AcceleratorNotFoundException, InvalidNextTokenException,
InternalServiceErrorException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
return listListeners(ListListenersRequest.builder().applyMutation(listListenersRequest).build());
}
/**
*
* List all tags for an accelerator. To see an AWS CLI example of listing tags for an accelerator, scroll down to
* Example.
*
*
* For more information, see Tagging in AWS
* Global Accelerator in the AWS Global Accelerator Developer Guide.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* List all tags for an accelerator. To see an AWS CLI example of listing tags for an accelerator, scroll down to
* Example.
*
*
* For more information, see Tagging in AWS
* Global Accelerator in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws AcceleratorNotFoundException,
InternalServiceErrorException, InvalidArgumentException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Provisions an IP address range to use with your AWS resources through bring your own IP addresses (BYOIP) and
* creates a corresponding address pool. After the address range is provisioned, it is ready to be advertised using
*
* AdvertiseByoipCidr.
*
*
* To see an AWS CLI example of provisioning an address range for BYOIP, scroll down to Example.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
* @param provisionByoipCidrRequest
* @return Result of the ProvisionByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws AccessDeniedException
* You don't have access permission.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ProvisionByoipCidr
* @see AWS API Documentation
*/
default ProvisionByoipCidrResponse provisionByoipCidr(ProvisionByoipCidrRequest provisionByoipCidrRequest)
throws InternalServiceErrorException, InvalidArgumentException, LimitExceededException, AccessDeniedException,
IncorrectCidrStateException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Provisions an IP address range to use with your AWS resources through bring your own IP addresses (BYOIP) and
* creates a corresponding address pool. After the address range is provisioned, it is ready to be advertised using
*
* AdvertiseByoipCidr.
*
*
* To see an AWS CLI example of provisioning an address range for BYOIP, scroll down to Example.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ProvisionByoipCidrRequest.Builder} avoiding the
* need to create one manually via {@link ProvisionByoipCidrRequest#builder()}
*
*
* @param provisionByoipCidrRequest
* A {@link Consumer} that will call methods on {@link ProvisionByoipCidrRequest.Builder} to create a
* request.
* @return Result of the ProvisionByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws AccessDeniedException
* You don't have access permission.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.ProvisionByoipCidr
* @see AWS API Documentation
*/
default ProvisionByoipCidrResponse provisionByoipCidr(Consumer provisionByoipCidrRequest)
throws InternalServiceErrorException, InvalidArgumentException, LimitExceededException, AccessDeniedException,
IncorrectCidrStateException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
return provisionByoipCidr(ProvisionByoipCidrRequest.builder().applyMutation(provisionByoipCidrRequest).build());
}
/**
*
* Add tags to an accelerator resource. To see an AWS CLI example of adding tags to an accelerator, scroll down to
* Example.
*
*
* For more information, see Tagging in AWS
* Global Accelerator in the AWS Global Accelerator Developer Guide.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws AcceleratorNotFoundException,
InternalServiceErrorException, InvalidArgumentException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Add tags to an accelerator resource. To see an AWS CLI example of adding tags to an accelerator, scroll down to
* Example.
*
*
* For more information, see Tagging in AWS
* Global Accelerator in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Remove tags from a Global Accelerator resource. When you specify a tag key, the action removes both that key and
* its associated value. To see an AWS CLI example of removing tags from an accelerator, scroll down to
* Example. The operation succeeds even if you attempt to remove tags from an accelerator that was already
* removed.
*
*
* For more information, see Tagging in AWS
* Global Accelerator in the AWS Global Accelerator Developer Guide.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UntagResource
* @see AWS API Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws AcceleratorNotFoundException,
InternalServiceErrorException, InvalidArgumentException, AwsServiceException, SdkClientException,
GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Remove tags from a Global Accelerator resource. When you specify a tag key, the action removes both that key and
* its associated value. To see an AWS CLI example of removing tags from an accelerator, scroll down to
* Example. The operation succeeds even if you attempt to remove tags from an accelerator that was already
* removed.
*
*
* For more information, see Tagging in AWS
* Global Accelerator in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UntagResource
* @see AWS API Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Update an accelerator. To see an AWS CLI example of updating an accelerator, scroll down to Example.
*
*
*
* You must specify the US West (Oregon) Region to create or update accelerators.
*
*
*
* @param updateAcceleratorRequest
* @return Result of the UpdateAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateAccelerator
* @see AWS API Documentation
*/
default UpdateAcceleratorResponse updateAccelerator(UpdateAcceleratorRequest updateAcceleratorRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Update an accelerator. To see an AWS CLI example of updating an accelerator, scroll down to Example.
*
*
*
* You must specify the US West (Oregon) Region to create or update accelerators.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAcceleratorRequest.Builder} avoiding the need
* to create one manually via {@link UpdateAcceleratorRequest#builder()}
*
*
* @param updateAcceleratorRequest
* A {@link Consumer} that will call methods on {@link UpdateAcceleratorRequest.Builder} to create a request.
* @return Result of the UpdateAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateAccelerator
* @see AWS API Documentation
*/
default UpdateAcceleratorResponse updateAccelerator(Consumer updateAcceleratorRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
return updateAccelerator(UpdateAcceleratorRequest.builder().applyMutation(updateAcceleratorRequest).build());
}
/**
*
* Update the attributes for an accelerator. To see an AWS CLI example of updating an accelerator to enable flow
* logs, scroll down to Example.
*
*
* @param updateAcceleratorAttributesRequest
* @return Result of the UpdateAcceleratorAttributes operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateAcceleratorAttributes
* @see AWS API Documentation
*/
default UpdateAcceleratorAttributesResponse updateAcceleratorAttributes(
UpdateAcceleratorAttributesRequest updateAcceleratorAttributesRequest) throws AcceleratorNotFoundException,
InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, AwsServiceException,
SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Update the attributes for an accelerator. To see an AWS CLI example of updating an accelerator to enable flow
* logs, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAcceleratorAttributesRequest.Builder}
* avoiding the need to create one manually via {@link UpdateAcceleratorAttributesRequest#builder()}
*
*
* @param updateAcceleratorAttributesRequest
* A {@link Consumer} that will call methods on {@link UpdateAcceleratorAttributesRequest.Builder} to create
* a request.
* @return Result of the UpdateAcceleratorAttributes operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateAcceleratorAttributes
* @see AWS API Documentation
*/
default UpdateAcceleratorAttributesResponse updateAcceleratorAttributes(
Consumer updateAcceleratorAttributesRequest)
throws AcceleratorNotFoundException, InternalServiceErrorException, InvalidArgumentException, AccessDeniedException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
return updateAcceleratorAttributes(UpdateAcceleratorAttributesRequest.builder()
.applyMutation(updateAcceleratorAttributesRequest).build());
}
/**
*
* Update an endpoint group. To see an AWS CLI example of updating an endpoint group, scroll down to Example.
*
*
* @param updateEndpointGroupRequest
* @return Result of the UpdateEndpointGroup operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws AccessDeniedException
* You don't have access permission.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateEndpointGroup
* @see AWS API Documentation
*/
default UpdateEndpointGroupResponse updateEndpointGroup(UpdateEndpointGroupRequest updateEndpointGroupRequest)
throws InvalidArgumentException, EndpointGroupNotFoundException, InternalServiceErrorException,
LimitExceededException, AccessDeniedException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Update an endpoint group. To see an AWS CLI example of updating an endpoint group, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEndpointGroupRequest.Builder} avoiding the
* need to create one manually via {@link UpdateEndpointGroupRequest#builder()}
*
*
* @param updateEndpointGroupRequest
* A {@link Consumer} that will call methods on {@link UpdateEndpointGroupRequest.Builder} to create a
* request.
* @return Result of the UpdateEndpointGroup operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws AccessDeniedException
* You don't have access permission.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateEndpointGroup
* @see AWS API Documentation
*/
default UpdateEndpointGroupResponse updateEndpointGroup(
Consumer updateEndpointGroupRequest) throws InvalidArgumentException,
EndpointGroupNotFoundException, InternalServiceErrorException, LimitExceededException, AccessDeniedException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
return updateEndpointGroup(UpdateEndpointGroupRequest.builder().applyMutation(updateEndpointGroupRequest).build());
}
/**
*
* Update a listener. To see an AWS CLI example of updating listener, scroll down to Example.
*
*
* @param updateListenerRequest
* @return Result of the UpdateListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InvalidPortRangeException
* The port numbers that you specified are not valid numbers or are not unique for this accelerator.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateListener
* @see AWS API Documentation
*/
default UpdateListenerResponse updateListener(UpdateListenerRequest updateListenerRequest) throws InvalidArgumentException,
InvalidPortRangeException, ListenerNotFoundException, InternalServiceErrorException, LimitExceededException,
AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Update a listener. To see an AWS CLI example of updating listener, scroll down to Example.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateListenerRequest.Builder} avoiding the need to
* create one manually via {@link UpdateListenerRequest#builder()}
*
*
* @param updateListenerRequest
* A {@link Consumer} that will call methods on {@link UpdateListenerRequest.Builder} to create a request.
* @return Result of the UpdateListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InvalidPortRangeException
* The port numbers that you specified are not valid numbers or are not unique for this accelerator.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.UpdateListener
* @see AWS API Documentation
*/
default UpdateListenerResponse updateListener(Consumer updateListenerRequest)
throws InvalidArgumentException, InvalidPortRangeException, ListenerNotFoundException, InternalServiceErrorException,
LimitExceededException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
return updateListener(UpdateListenerRequest.builder().applyMutation(updateListenerRequest).build());
}
/**
*
* Stops advertising an address range that is provisioned as an address pool. You can perform this operation at most
* once every 10 seconds, even if you specify different address ranges each time. To see an AWS CLI example of
* withdrawing an address range for BYOIP so it will no longer be advertised by AWS, scroll down to Example.
*
*
* It can take a few minutes before traffic to the specified addresses stops routing to AWS because of propagation
* delays.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
* @param withdrawByoipCidrRequest
* @return Result of the WithdrawByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws ByoipCidrNotFoundException
* The CIDR that you specified was not found or is incorrect.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.WithdrawByoipCidr
* @see AWS API Documentation
*/
default WithdrawByoipCidrResponse withdrawByoipCidr(WithdrawByoipCidrRequest withdrawByoipCidrRequest)
throws InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, ByoipCidrNotFoundException,
IncorrectCidrStateException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
throw new UnsupportedOperationException();
}
/**
*
* Stops advertising an address range that is provisioned as an address pool. You can perform this operation at most
* once every 10 seconds, even if you specify different address ranges each time. To see an AWS CLI example of
* withdrawing an address range for BYOIP so it will no longer be advertised by AWS, scroll down to Example.
*
*
* It can take a few minutes before traffic to the specified addresses stops routing to AWS because of propagation
* delays.
*
*
* For more information, see Bring Your Own IP Addresses
* (BYOIP) in the AWS Global Accelerator Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link WithdrawByoipCidrRequest.Builder} avoiding the need
* to create one manually via {@link WithdrawByoipCidrRequest#builder()}
*
*
* @param withdrawByoipCidrRequest
* A {@link Consumer} that will call methods on {@link WithdrawByoipCidrRequest.Builder} to create a request.
* @return Result of the WithdrawByoipCidr operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AccessDeniedException
* You don't have access permission.
* @throws ByoipCidrNotFoundException
* The CIDR that you specified was not found or is incorrect.
* @throws IncorrectCidrStateException
* The CIDR that you specified is not valid for this action. For example, the state of the CIDR might be
* incorrect for this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws GlobalAcceleratorException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample GlobalAcceleratorClient.WithdrawByoipCidr
* @see AWS API Documentation
*/
default WithdrawByoipCidrResponse withdrawByoipCidr(Consumer withdrawByoipCidrRequest)
throws InternalServiceErrorException, InvalidArgumentException, AccessDeniedException, ByoipCidrNotFoundException,
IncorrectCidrStateException, AwsServiceException, SdkClientException, GlobalAcceleratorException {
return withdrawByoipCidr(WithdrawByoipCidrRequest.builder().applyMutation(withdrawByoipCidrRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("globalaccelerator");
}
}