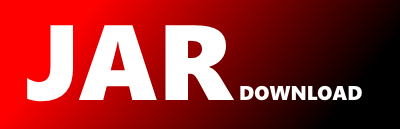
software.amazon.awssdk.services.globalaccelerator.model.CustomRoutingEndpointGroup Maven / Gradle / Ivy
Show all versions of globalaccelerator Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.globalaccelerator.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A complex type for the endpoint group for a custom routing accelerator. An Amazon Web Services Region can have only
* one endpoint group for a specific listener.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CustomRoutingEndpointGroup implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENDPOINT_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointGroupArn").getter(getter(CustomRoutingEndpointGroup::endpointGroupArn))
.setter(setter(Builder::endpointGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointGroupArn").build()).build();
private static final SdkField ENDPOINT_GROUP_REGION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointGroupRegion").getter(getter(CustomRoutingEndpointGroup::endpointGroupRegion))
.setter(setter(Builder::endpointGroupRegion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointGroupRegion").build())
.build();
private static final SdkField> DESTINATION_DESCRIPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DestinationDescriptions")
.getter(getter(CustomRoutingEndpointGroup::destinationDescriptions))
.setter(setter(Builder::destinationDescriptions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DestinationDescriptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CustomRoutingDestinationDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ENDPOINT_DESCRIPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EndpointDescriptions")
.getter(getter(CustomRoutingEndpointGroup::endpointDescriptions))
.setter(setter(Builder::endpointDescriptions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointDescriptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CustomRoutingEndpointDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENDPOINT_GROUP_ARN_FIELD,
ENDPOINT_GROUP_REGION_FIELD, DESTINATION_DESCRIPTIONS_FIELD, ENDPOINT_DESCRIPTIONS_FIELD));
private static final long serialVersionUID = 1L;
private final String endpointGroupArn;
private final String endpointGroupRegion;
private final List destinationDescriptions;
private final List endpointDescriptions;
private CustomRoutingEndpointGroup(BuilderImpl builder) {
this.endpointGroupArn = builder.endpointGroupArn;
this.endpointGroupRegion = builder.endpointGroupRegion;
this.destinationDescriptions = builder.destinationDescriptions;
this.endpointDescriptions = builder.endpointDescriptions;
}
/**
*
* The Amazon Resource Name (ARN) of the endpoint group.
*
*
* @return The Amazon Resource Name (ARN) of the endpoint group.
*/
public final String endpointGroupArn() {
return endpointGroupArn;
}
/**
*
* The Amazon Web Services Region where the endpoint group is located.
*
*
* @return The Amazon Web Services Region where the endpoint group is located.
*/
public final String endpointGroupRegion() {
return endpointGroupRegion;
}
/**
* For responses, this returns true if the service returned a value for the DestinationDescriptions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasDestinationDescriptions() {
return destinationDescriptions != null && !(destinationDescriptions instanceof SdkAutoConstructList);
}
/**
*
* For a custom routing accelerator, describes the port range and protocol for all endpoints (virtual private cloud
* subnets) in an endpoint group to accept client traffic on.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDestinationDescriptions} method.
*
*
* @return For a custom routing accelerator, describes the port range and protocol for all endpoints (virtual
* private cloud subnets) in an endpoint group to accept client traffic on.
*/
public final List destinationDescriptions() {
return destinationDescriptions;
}
/**
* For responses, this returns true if the service returned a value for the EndpointDescriptions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEndpointDescriptions() {
return endpointDescriptions != null && !(endpointDescriptions instanceof SdkAutoConstructList);
}
/**
*
* For a custom routing accelerator, describes the endpoints (virtual private cloud subnets) in an endpoint group to
* accept client traffic on.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEndpointDescriptions} method.
*
*
* @return For a custom routing accelerator, describes the endpoints (virtual private cloud subnets) in an endpoint
* group to accept client traffic on.
*/
public final List endpointDescriptions() {
return endpointDescriptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(endpointGroupArn());
hashCode = 31 * hashCode + Objects.hashCode(endpointGroupRegion());
hashCode = 31 * hashCode + Objects.hashCode(hasDestinationDescriptions() ? destinationDescriptions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasEndpointDescriptions() ? endpointDescriptions() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CustomRoutingEndpointGroup)) {
return false;
}
CustomRoutingEndpointGroup other = (CustomRoutingEndpointGroup) obj;
return Objects.equals(endpointGroupArn(), other.endpointGroupArn())
&& Objects.equals(endpointGroupRegion(), other.endpointGroupRegion())
&& hasDestinationDescriptions() == other.hasDestinationDescriptions()
&& Objects.equals(destinationDescriptions(), other.destinationDescriptions())
&& hasEndpointDescriptions() == other.hasEndpointDescriptions()
&& Objects.equals(endpointDescriptions(), other.endpointDescriptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CustomRoutingEndpointGroup").add("EndpointGroupArn", endpointGroupArn())
.add("EndpointGroupRegion", endpointGroupRegion())
.add("DestinationDescriptions", hasDestinationDescriptions() ? destinationDescriptions() : null)
.add("EndpointDescriptions", hasEndpointDescriptions() ? endpointDescriptions() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EndpointGroupArn":
return Optional.ofNullable(clazz.cast(endpointGroupArn()));
case "EndpointGroupRegion":
return Optional.ofNullable(clazz.cast(endpointGroupRegion()));
case "DestinationDescriptions":
return Optional.ofNullable(clazz.cast(destinationDescriptions()));
case "EndpointDescriptions":
return Optional.ofNullable(clazz.cast(endpointDescriptions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function