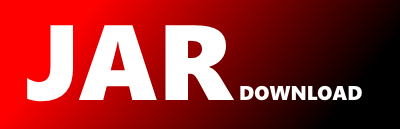
software.amazon.awssdk.services.glue.model.AuthenticationConfiguration Maven / Gradle / Ivy
Show all versions of glue Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A structure containing the authentication configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AuthenticationConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AUTHENTICATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthenticationType").getter(getter(AuthenticationConfiguration::authenticationTypeAsString))
.setter(setter(Builder::authenticationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthenticationType").build())
.build();
private static final SdkField SECRET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecretArn").getter(getter(AuthenticationConfiguration::secretArn)).setter(setter(Builder::secretArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretArn").build()).build();
private static final SdkField O_AUTH2_PROPERTIES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OAuth2Properties")
.getter(getter(AuthenticationConfiguration::oAuth2Properties)).setter(setter(Builder::oAuth2Properties))
.constructor(OAuth2Properties::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OAuth2Properties").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTHENTICATION_TYPE_FIELD,
SECRET_ARN_FIELD, O_AUTH2_PROPERTIES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AuthenticationType", AUTHENTICATION_TYPE_FIELD);
put("SecretArn", SECRET_ARN_FIELD);
put("OAuth2Properties", O_AUTH2_PROPERTIES_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String authenticationType;
private final String secretArn;
private final OAuth2Properties oAuth2Properties;
private AuthenticationConfiguration(BuilderImpl builder) {
this.authenticationType = builder.authenticationType;
this.secretArn = builder.secretArn;
this.oAuth2Properties = builder.oAuth2Properties;
}
/**
*
* A structure containing the authentication configuration.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authenticationType} will return {@link AuthenticationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #authenticationTypeAsString}.
*
*
* @return A structure containing the authentication configuration.
* @see AuthenticationType
*/
public final AuthenticationType authenticationType() {
return AuthenticationType.fromValue(authenticationType);
}
/**
*
* A structure containing the authentication configuration.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authenticationType} will return {@link AuthenticationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #authenticationTypeAsString}.
*
*
* @return A structure containing the authentication configuration.
* @see AuthenticationType
*/
public final String authenticationTypeAsString() {
return authenticationType;
}
/**
*
* The secret manager ARN to store credentials.
*
*
* @return The secret manager ARN to store credentials.
*/
public final String secretArn() {
return secretArn;
}
/**
*
* The properties for OAuth2 authentication.
*
*
* @return The properties for OAuth2 authentication.
*/
public final OAuth2Properties oAuth2Properties() {
return oAuth2Properties;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(authenticationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(secretArn());
hashCode = 31 * hashCode + Objects.hashCode(oAuth2Properties());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AuthenticationConfiguration)) {
return false;
}
AuthenticationConfiguration other = (AuthenticationConfiguration) obj;
return Objects.equals(authenticationTypeAsString(), other.authenticationTypeAsString())
&& Objects.equals(secretArn(), other.secretArn()) && Objects.equals(oAuth2Properties(), other.oAuth2Properties());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AuthenticationConfiguration").add("AuthenticationType", authenticationTypeAsString())
.add("SecretArn", secretArn()).add("OAuth2Properties", oAuth2Properties()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AuthenticationType":
return Optional.ofNullable(clazz.cast(authenticationTypeAsString()));
case "SecretArn":
return Optional.ofNullable(clazz.cast(secretArn()));
case "OAuth2Properties":
return Optional.ofNullable(clazz.cast(oAuth2Properties()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function