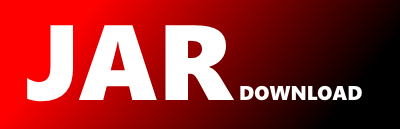
software.amazon.awssdk.services.glue.model.DataQualityResult Maven / Gradle / Ivy
Show all versions of glue Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a data quality result.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DataQualityResult implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField RESULT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResultId").getter(getter(DataQualityResult::resultId)).setter(setter(Builder::resultId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResultId").build()).build();
private static final SdkField PROFILE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProfileId").getter(getter(DataQualityResult::profileId)).setter(setter(Builder::profileId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProfileId").build()).build();
private static final SdkField SCORE_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("Score")
.getter(getter(DataQualityResult::score)).setter(setter(Builder::score))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Score").build()).build();
private static final SdkField DATA_SOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("DataSource").getter(getter(DataQualityResult::dataSource)).setter(setter(Builder::dataSource))
.constructor(DataSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSource").build()).build();
private static final SdkField RULESET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RulesetName").getter(getter(DataQualityResult::rulesetName)).setter(setter(Builder::rulesetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RulesetName").build()).build();
private static final SdkField EVALUATION_CONTEXT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EvaluationContext").getter(getter(DataQualityResult::evaluationContext))
.setter(setter(Builder::evaluationContext))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluationContext").build()).build();
private static final SdkField STARTED_ON_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartedOn").getter(getter(DataQualityResult::startedOn)).setter(setter(Builder::startedOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartedOn").build()).build();
private static final SdkField COMPLETED_ON_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CompletedOn").getter(getter(DataQualityResult::completedOn)).setter(setter(Builder::completedOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletedOn").build()).build();
private static final SdkField JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobName").getter(getter(DataQualityResult::jobName)).setter(setter(Builder::jobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobName").build()).build();
private static final SdkField JOB_RUN_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobRunId").getter(getter(DataQualityResult::jobRunId)).setter(setter(Builder::jobRunId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobRunId").build()).build();
private static final SdkField RULESET_EVALUATION_RUN_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RulesetEvaluationRunId").getter(getter(DataQualityResult::rulesetEvaluationRunId))
.setter(setter(Builder::rulesetEvaluationRunId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RulesetEvaluationRunId").build())
.build();
private static final SdkField> RULE_RESULTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RuleResults")
.getter(getter(DataQualityResult::ruleResults))
.setter(setter(Builder::ruleResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuleResults").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DataQualityRuleResult::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ANALYZER_RESULTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AnalyzerResults")
.getter(getter(DataQualityResult::analyzerResults))
.setter(setter(Builder::analyzerResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AnalyzerResults").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DataQualityAnalyzerResult::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OBSERVATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Observations")
.getter(getter(DataQualityResult::observations))
.setter(setter(Builder::observations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Observations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DataQualityObservation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RESULT_ID_FIELD,
PROFILE_ID_FIELD, SCORE_FIELD, DATA_SOURCE_FIELD, RULESET_NAME_FIELD, EVALUATION_CONTEXT_FIELD, STARTED_ON_FIELD,
COMPLETED_ON_FIELD, JOB_NAME_FIELD, JOB_RUN_ID_FIELD, RULESET_EVALUATION_RUN_ID_FIELD, RULE_RESULTS_FIELD,
ANALYZER_RESULTS_FIELD, OBSERVATIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ResultId", RESULT_ID_FIELD);
put("ProfileId", PROFILE_ID_FIELD);
put("Score", SCORE_FIELD);
put("DataSource", DATA_SOURCE_FIELD);
put("RulesetName", RULESET_NAME_FIELD);
put("EvaluationContext", EVALUATION_CONTEXT_FIELD);
put("StartedOn", STARTED_ON_FIELD);
put("CompletedOn", COMPLETED_ON_FIELD);
put("JobName", JOB_NAME_FIELD);
put("JobRunId", JOB_RUN_ID_FIELD);
put("RulesetEvaluationRunId", RULESET_EVALUATION_RUN_ID_FIELD);
put("RuleResults", RULE_RESULTS_FIELD);
put("AnalyzerResults", ANALYZER_RESULTS_FIELD);
put("Observations", OBSERVATIONS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String resultId;
private final String profileId;
private final Double score;
private final DataSource dataSource;
private final String rulesetName;
private final String evaluationContext;
private final Instant startedOn;
private final Instant completedOn;
private final String jobName;
private final String jobRunId;
private final String rulesetEvaluationRunId;
private final List ruleResults;
private final List analyzerResults;
private final List observations;
private DataQualityResult(BuilderImpl builder) {
this.resultId = builder.resultId;
this.profileId = builder.profileId;
this.score = builder.score;
this.dataSource = builder.dataSource;
this.rulesetName = builder.rulesetName;
this.evaluationContext = builder.evaluationContext;
this.startedOn = builder.startedOn;
this.completedOn = builder.completedOn;
this.jobName = builder.jobName;
this.jobRunId = builder.jobRunId;
this.rulesetEvaluationRunId = builder.rulesetEvaluationRunId;
this.ruleResults = builder.ruleResults;
this.analyzerResults = builder.analyzerResults;
this.observations = builder.observations;
}
/**
*
* A unique result ID for the data quality result.
*
*
* @return A unique result ID for the data quality result.
*/
public final String resultId() {
return resultId;
}
/**
*
* The Profile ID for the data quality result.
*
*
* @return The Profile ID for the data quality result.
*/
public final String profileId() {
return profileId;
}
/**
*
* An aggregate data quality score. Represents the ratio of rules that passed to the total number of rules.
*
*
* @return An aggregate data quality score. Represents the ratio of rules that passed to the total number of rules.
*/
public final Double score() {
return score;
}
/**
*
* The table associated with the data quality result, if any.
*
*
* @return The table associated with the data quality result, if any.
*/
public final DataSource dataSource() {
return dataSource;
}
/**
*
* The name of the ruleset associated with the data quality result.
*
*
* @return The name of the ruleset associated with the data quality result.
*/
public final String rulesetName() {
return rulesetName;
}
/**
*
* In the context of a job in Glue Studio, each node in the canvas is typically assigned some sort of name and data
* quality nodes will have names. In the case of multiple nodes, the evaluationContext
can
* differentiate the nodes.
*
*
* @return In the context of a job in Glue Studio, each node in the canvas is typically assigned some sort of name
* and data quality nodes will have names. In the case of multiple nodes, the evaluationContext
* can differentiate the nodes.
*/
public final String evaluationContext() {
return evaluationContext;
}
/**
*
* The date and time when this data quality run started.
*
*
* @return The date and time when this data quality run started.
*/
public final Instant startedOn() {
return startedOn;
}
/**
*
* The date and time when this data quality run completed.
*
*
* @return The date and time when this data quality run completed.
*/
public final Instant completedOn() {
return completedOn;
}
/**
*
* The job name associated with the data quality result, if any.
*
*
* @return The job name associated with the data quality result, if any.
*/
public final String jobName() {
return jobName;
}
/**
*
* The job run ID associated with the data quality result, if any.
*
*
* @return The job run ID associated with the data quality result, if any.
*/
public final String jobRunId() {
return jobRunId;
}
/**
*
* The unique run ID for the ruleset evaluation for this data quality result.
*
*
* @return The unique run ID for the ruleset evaluation for this data quality result.
*/
public final String rulesetEvaluationRunId() {
return rulesetEvaluationRunId;
}
/**
* For responses, this returns true if the service returned a value for the RuleResults property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRuleResults() {
return ruleResults != null && !(ruleResults instanceof SdkAutoConstructList);
}
/**
*
* A list of DataQualityRuleResult
objects representing the results for each rule.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRuleResults} method.
*
*
* @return A list of DataQualityRuleResult
objects representing the results for each rule.
*/
public final List ruleResults() {
return ruleResults;
}
/**
* For responses, this returns true if the service returned a value for the AnalyzerResults property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAnalyzerResults() {
return analyzerResults != null && !(analyzerResults instanceof SdkAutoConstructList);
}
/**
*
* A list of DataQualityAnalyzerResult
objects representing the results for each analyzer.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAnalyzerResults} method.
*
*
* @return A list of DataQualityAnalyzerResult
objects representing the results for each analyzer.
*/
public final List analyzerResults() {
return analyzerResults;
}
/**
* For responses, this returns true if the service returned a value for the Observations property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasObservations() {
return observations != null && !(observations instanceof SdkAutoConstructList);
}
/**
*
* A list of DataQualityObservation
objects representing the observations generated after evaluating
* the rules and analyzers.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasObservations} method.
*
*
* @return A list of DataQualityObservation
objects representing the observations generated after
* evaluating the rules and analyzers.
*/
public final List observations() {
return observations;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(resultId());
hashCode = 31 * hashCode + Objects.hashCode(profileId());
hashCode = 31 * hashCode + Objects.hashCode(score());
hashCode = 31 * hashCode + Objects.hashCode(dataSource());
hashCode = 31 * hashCode + Objects.hashCode(rulesetName());
hashCode = 31 * hashCode + Objects.hashCode(evaluationContext());
hashCode = 31 * hashCode + Objects.hashCode(startedOn());
hashCode = 31 * hashCode + Objects.hashCode(completedOn());
hashCode = 31 * hashCode + Objects.hashCode(jobName());
hashCode = 31 * hashCode + Objects.hashCode(jobRunId());
hashCode = 31 * hashCode + Objects.hashCode(rulesetEvaluationRunId());
hashCode = 31 * hashCode + Objects.hashCode(hasRuleResults() ? ruleResults() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAnalyzerResults() ? analyzerResults() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasObservations() ? observations() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DataQualityResult)) {
return false;
}
DataQualityResult other = (DataQualityResult) obj;
return Objects.equals(resultId(), other.resultId()) && Objects.equals(profileId(), other.profileId())
&& Objects.equals(score(), other.score()) && Objects.equals(dataSource(), other.dataSource())
&& Objects.equals(rulesetName(), other.rulesetName())
&& Objects.equals(evaluationContext(), other.evaluationContext())
&& Objects.equals(startedOn(), other.startedOn()) && Objects.equals(completedOn(), other.completedOn())
&& Objects.equals(jobName(), other.jobName()) && Objects.equals(jobRunId(), other.jobRunId())
&& Objects.equals(rulesetEvaluationRunId(), other.rulesetEvaluationRunId())
&& hasRuleResults() == other.hasRuleResults() && Objects.equals(ruleResults(), other.ruleResults())
&& hasAnalyzerResults() == other.hasAnalyzerResults()
&& Objects.equals(analyzerResults(), other.analyzerResults()) && hasObservations() == other.hasObservations()
&& Objects.equals(observations(), other.observations());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DataQualityResult").add("ResultId", resultId()).add("ProfileId", profileId())
.add("Score", score()).add("DataSource", dataSource()).add("RulesetName", rulesetName())
.add("EvaluationContext", evaluationContext()).add("StartedOn", startedOn()).add("CompletedOn", completedOn())
.add("JobName", jobName()).add("JobRunId", jobRunId()).add("RulesetEvaluationRunId", rulesetEvaluationRunId())
.add("RuleResults", hasRuleResults() ? ruleResults() : null)
.add("AnalyzerResults", hasAnalyzerResults() ? analyzerResults() : null)
.add("Observations", hasObservations() ? observations() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ResultId":
return Optional.ofNullable(clazz.cast(resultId()));
case "ProfileId":
return Optional.ofNullable(clazz.cast(profileId()));
case "Score":
return Optional.ofNullable(clazz.cast(score()));
case "DataSource":
return Optional.ofNullable(clazz.cast(dataSource()));
case "RulesetName":
return Optional.ofNullable(clazz.cast(rulesetName()));
case "EvaluationContext":
return Optional.ofNullable(clazz.cast(evaluationContext()));
case "StartedOn":
return Optional.ofNullable(clazz.cast(startedOn()));
case "CompletedOn":
return Optional.ofNullable(clazz.cast(completedOn()));
case "JobName":
return Optional.ofNullable(clazz.cast(jobName()));
case "JobRunId":
return Optional.ofNullable(clazz.cast(jobRunId()));
case "RulesetEvaluationRunId":
return Optional.ofNullable(clazz.cast(rulesetEvaluationRunId()));
case "RuleResults":
return Optional.ofNullable(clazz.cast(ruleResults()));
case "AnalyzerResults":
return Optional.ofNullable(clazz.cast(analyzerResults()));
case "Observations":
return Optional.ofNullable(clazz.cast(observations()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function