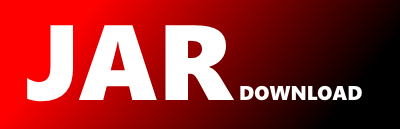
software.amazon.awssdk.services.glue.model.DataQualityResultDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glue Show documentation
Show all versions of glue Show documentation
The AWS Java SDK for AWS Glue module holds the client classes that are used for communicating
with AWS Glue Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a data quality result.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DataQualityResultDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField RESULT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResultId").getter(getter(DataQualityResultDescription::resultId)).setter(setter(Builder::resultId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResultId").build()).build();
private static final SdkField DATA_SOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("DataSource").getter(getter(DataQualityResultDescription::dataSource))
.setter(setter(Builder::dataSource)).constructor(DataSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSource").build()).build();
private static final SdkField JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobName").getter(getter(DataQualityResultDescription::jobName)).setter(setter(Builder::jobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobName").build()).build();
private static final SdkField JOB_RUN_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobRunId").getter(getter(DataQualityResultDescription::jobRunId)).setter(setter(Builder::jobRunId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobRunId").build()).build();
private static final SdkField STARTED_ON_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartedOn").getter(getter(DataQualityResultDescription::startedOn)).setter(setter(Builder::startedOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartedOn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RESULT_ID_FIELD,
DATA_SOURCE_FIELD, JOB_NAME_FIELD, JOB_RUN_ID_FIELD, STARTED_ON_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ResultId", RESULT_ID_FIELD);
put("DataSource", DATA_SOURCE_FIELD);
put("JobName", JOB_NAME_FIELD);
put("JobRunId", JOB_RUN_ID_FIELD);
put("StartedOn", STARTED_ON_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String resultId;
private final DataSource dataSource;
private final String jobName;
private final String jobRunId;
private final Instant startedOn;
private DataQualityResultDescription(BuilderImpl builder) {
this.resultId = builder.resultId;
this.dataSource = builder.dataSource;
this.jobName = builder.jobName;
this.jobRunId = builder.jobRunId;
this.startedOn = builder.startedOn;
}
/**
*
* The unique result ID for this data quality result.
*
*
* @return The unique result ID for this data quality result.
*/
public final String resultId() {
return resultId;
}
/**
*
* The table name associated with the data quality result.
*
*
* @return The table name associated with the data quality result.
*/
public final DataSource dataSource() {
return dataSource;
}
/**
*
* The job name associated with the data quality result.
*
*
* @return The job name associated with the data quality result.
*/
public final String jobName() {
return jobName;
}
/**
*
* The job run ID associated with the data quality result.
*
*
* @return The job run ID associated with the data quality result.
*/
public final String jobRunId() {
return jobRunId;
}
/**
*
* The time that the run started for this data quality result.
*
*
* @return The time that the run started for this data quality result.
*/
public final Instant startedOn() {
return startedOn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(resultId());
hashCode = 31 * hashCode + Objects.hashCode(dataSource());
hashCode = 31 * hashCode + Objects.hashCode(jobName());
hashCode = 31 * hashCode + Objects.hashCode(jobRunId());
hashCode = 31 * hashCode + Objects.hashCode(startedOn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DataQualityResultDescription)) {
return false;
}
DataQualityResultDescription other = (DataQualityResultDescription) obj;
return Objects.equals(resultId(), other.resultId()) && Objects.equals(dataSource(), other.dataSource())
&& Objects.equals(jobName(), other.jobName()) && Objects.equals(jobRunId(), other.jobRunId())
&& Objects.equals(startedOn(), other.startedOn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DataQualityResultDescription").add("ResultId", resultId()).add("DataSource", dataSource())
.add("JobName", jobName()).add("JobRunId", jobRunId()).add("StartedOn", startedOn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ResultId":
return Optional.ofNullable(clazz.cast(resultId()));
case "DataSource":
return Optional.ofNullable(clazz.cast(dataSource()));
case "JobName":
return Optional.ofNullable(clazz.cast(jobName()));
case "JobRunId":
return Optional.ofNullable(clazz.cast(jobRunId()));
case "StartedOn":
return Optional.ofNullable(clazz.cast(startedOn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy