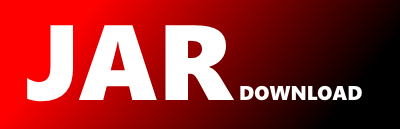
software.amazon.awssdk.services.glue.model.GetDataQualityRuleRecommendationRunResponse Maven / Gradle / Ivy
Show all versions of glue Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetDataQualityRuleRecommendationRunResponse extends GlueResponse implements
ToCopyableBuilder {
private static final SdkField RUN_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("RunId")
.getter(getter(GetDataQualityRuleRecommendationRunResponse::runId)).setter(setter(Builder::runId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RunId").build()).build();
private static final SdkField DATA_SOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("DataSource").getter(getter(GetDataQualityRuleRecommendationRunResponse::dataSource))
.setter(setter(Builder::dataSource)).constructor(DataSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSource").build()).build();
private static final SdkField ROLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Role")
.getter(getter(GetDataQualityRuleRecommendationRunResponse::role)).setter(setter(Builder::role))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Role").build()).build();
private static final SdkField NUMBER_OF_WORKERS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumberOfWorkers").getter(getter(GetDataQualityRuleRecommendationRunResponse::numberOfWorkers))
.setter(setter(Builder::numberOfWorkers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfWorkers").build()).build();
private static final SdkField TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Timeout").getter(getter(GetDataQualityRuleRecommendationRunResponse::timeout))
.setter(setter(Builder::timeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timeout").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(GetDataQualityRuleRecommendationRunResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField ERROR_STRING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ErrorString").getter(getter(GetDataQualityRuleRecommendationRunResponse::errorString))
.setter(setter(Builder::errorString))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ErrorString").build()).build();
private static final SdkField STARTED_ON_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartedOn").getter(getter(GetDataQualityRuleRecommendationRunResponse::startedOn))
.setter(setter(Builder::startedOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartedOn").build()).build();
private static final SdkField LAST_MODIFIED_ON_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedOn").getter(getter(GetDataQualityRuleRecommendationRunResponse::lastModifiedOn))
.setter(setter(Builder::lastModifiedOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedOn").build()).build();
private static final SdkField COMPLETED_ON_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CompletedOn").getter(getter(GetDataQualityRuleRecommendationRunResponse::completedOn))
.setter(setter(Builder::completedOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletedOn").build()).build();
private static final SdkField EXECUTION_TIME_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ExecutionTime").getter(getter(GetDataQualityRuleRecommendationRunResponse::executionTime))
.setter(setter(Builder::executionTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionTime").build()).build();
private static final SdkField RECOMMENDED_RULESET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RecommendedRuleset").getter(getter(GetDataQualityRuleRecommendationRunResponse::recommendedRuleset))
.setter(setter(Builder::recommendedRuleset))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecommendedRuleset").build())
.build();
private static final SdkField CREATED_RULESET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreatedRulesetName").getter(getter(GetDataQualityRuleRecommendationRunResponse::createdRulesetName))
.setter(setter(Builder::createdRulesetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedRulesetName").build())
.build();
private static final SdkField DATA_QUALITY_SECURITY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DataQualitySecurityConfiguration")
.getter(getter(GetDataQualityRuleRecommendationRunResponse::dataQualitySecurityConfiguration))
.setter(setter(Builder::dataQualitySecurityConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataQualitySecurityConfiguration")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RUN_ID_FIELD,
DATA_SOURCE_FIELD, ROLE_FIELD, NUMBER_OF_WORKERS_FIELD, TIMEOUT_FIELD, STATUS_FIELD, ERROR_STRING_FIELD,
STARTED_ON_FIELD, LAST_MODIFIED_ON_FIELD, COMPLETED_ON_FIELD, EXECUTION_TIME_FIELD, RECOMMENDED_RULESET_FIELD,
CREATED_RULESET_NAME_FIELD, DATA_QUALITY_SECURITY_CONFIGURATION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("RunId", RUN_ID_FIELD);
put("DataSource", DATA_SOURCE_FIELD);
put("Role", ROLE_FIELD);
put("NumberOfWorkers", NUMBER_OF_WORKERS_FIELD);
put("Timeout", TIMEOUT_FIELD);
put("Status", STATUS_FIELD);
put("ErrorString", ERROR_STRING_FIELD);
put("StartedOn", STARTED_ON_FIELD);
put("LastModifiedOn", LAST_MODIFIED_ON_FIELD);
put("CompletedOn", COMPLETED_ON_FIELD);
put("ExecutionTime", EXECUTION_TIME_FIELD);
put("RecommendedRuleset", RECOMMENDED_RULESET_FIELD);
put("CreatedRulesetName", CREATED_RULESET_NAME_FIELD);
put("DataQualitySecurityConfiguration", DATA_QUALITY_SECURITY_CONFIGURATION_FIELD);
}
});
private final String runId;
private final DataSource dataSource;
private final String role;
private final Integer numberOfWorkers;
private final Integer timeout;
private final String status;
private final String errorString;
private final Instant startedOn;
private final Instant lastModifiedOn;
private final Instant completedOn;
private final Integer executionTime;
private final String recommendedRuleset;
private final String createdRulesetName;
private final String dataQualitySecurityConfiguration;
private GetDataQualityRuleRecommendationRunResponse(BuilderImpl builder) {
super(builder);
this.runId = builder.runId;
this.dataSource = builder.dataSource;
this.role = builder.role;
this.numberOfWorkers = builder.numberOfWorkers;
this.timeout = builder.timeout;
this.status = builder.status;
this.errorString = builder.errorString;
this.startedOn = builder.startedOn;
this.lastModifiedOn = builder.lastModifiedOn;
this.completedOn = builder.completedOn;
this.executionTime = builder.executionTime;
this.recommendedRuleset = builder.recommendedRuleset;
this.createdRulesetName = builder.createdRulesetName;
this.dataQualitySecurityConfiguration = builder.dataQualitySecurityConfiguration;
}
/**
*
* The unique run identifier associated with this run.
*
*
* @return The unique run identifier associated with this run.
*/
public final String runId() {
return runId;
}
/**
*
* The data source (an Glue table) associated with this run.
*
*
* @return The data source (an Glue table) associated with this run.
*/
public final DataSource dataSource() {
return dataSource;
}
/**
*
* An IAM role supplied to encrypt the results of the run.
*
*
* @return An IAM role supplied to encrypt the results of the run.
*/
public final String role() {
return role;
}
/**
*
* The number of G.1X
workers to be used in the run. The default is 5.
*
*
* @return The number of G.1X
workers to be used in the run. The default is 5.
*/
public final Integer numberOfWorkers() {
return numberOfWorkers;
}
/**
*
* The timeout for a run in minutes. This is the maximum time that a run can consume resources before it is
* terminated and enters TIMEOUT
status. The default is 2,880 minutes (48 hours).
*
*
* @return The timeout for a run in minutes. This is the maximum time that a run can consume resources before it is
* terminated and enters TIMEOUT
status. The default is 2,880 minutes (48 hours).
*/
public final Integer timeout() {
return timeout;
}
/**
*
* The status for this run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TaskStatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status for this run.
* @see TaskStatusType
*/
public final TaskStatusType status() {
return TaskStatusType.fromValue(status);
}
/**
*
* The status for this run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TaskStatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status for this run.
* @see TaskStatusType
*/
public final String statusAsString() {
return status;
}
/**
*
* The error strings that are associated with the run.
*
*
* @return The error strings that are associated with the run.
*/
public final String errorString() {
return errorString;
}
/**
*
* The date and time when this run started.
*
*
* @return The date and time when this run started.
*/
public final Instant startedOn() {
return startedOn;
}
/**
*
* A timestamp. The last point in time when this data quality rule recommendation run was modified.
*
*
* @return A timestamp. The last point in time when this data quality rule recommendation run was modified.
*/
public final Instant lastModifiedOn() {
return lastModifiedOn;
}
/**
*
* The date and time when this run was completed.
*
*
* @return The date and time when this run was completed.
*/
public final Instant completedOn() {
return completedOn;
}
/**
*
* The amount of time (in seconds) that the run consumed resources.
*
*
* @return The amount of time (in seconds) that the run consumed resources.
*/
public final Integer executionTime() {
return executionTime;
}
/**
*
* When a start rule recommendation run completes, it creates a recommended ruleset (a set of rules). This member
* has those rules in Data Quality Definition Language (DQDL) format.
*
*
* @return When a start rule recommendation run completes, it creates a recommended ruleset (a set of rules). This
* member has those rules in Data Quality Definition Language (DQDL) format.
*/
public final String recommendedRuleset() {
return recommendedRuleset;
}
/**
*
* The name of the ruleset that was created by the run.
*
*
* @return The name of the ruleset that was created by the run.
*/
public final String createdRulesetName() {
return createdRulesetName;
}
/**
*
* The name of the security configuration created with the data quality encryption option.
*
*
* @return The name of the security configuration created with the data quality encryption option.
*/
public final String dataQualitySecurityConfiguration() {
return dataQualitySecurityConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(runId());
hashCode = 31 * hashCode + Objects.hashCode(dataSource());
hashCode = 31 * hashCode + Objects.hashCode(role());
hashCode = 31 * hashCode + Objects.hashCode(numberOfWorkers());
hashCode = 31 * hashCode + Objects.hashCode(timeout());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(errorString());
hashCode = 31 * hashCode + Objects.hashCode(startedOn());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedOn());
hashCode = 31 * hashCode + Objects.hashCode(completedOn());
hashCode = 31 * hashCode + Objects.hashCode(executionTime());
hashCode = 31 * hashCode + Objects.hashCode(recommendedRuleset());
hashCode = 31 * hashCode + Objects.hashCode(createdRulesetName());
hashCode = 31 * hashCode + Objects.hashCode(dataQualitySecurityConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetDataQualityRuleRecommendationRunResponse)) {
return false;
}
GetDataQualityRuleRecommendationRunResponse other = (GetDataQualityRuleRecommendationRunResponse) obj;
return Objects.equals(runId(), other.runId()) && Objects.equals(dataSource(), other.dataSource())
&& Objects.equals(role(), other.role()) && Objects.equals(numberOfWorkers(), other.numberOfWorkers())
&& Objects.equals(timeout(), other.timeout()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(errorString(), other.errorString()) && Objects.equals(startedOn(), other.startedOn())
&& Objects.equals(lastModifiedOn(), other.lastModifiedOn()) && Objects.equals(completedOn(), other.completedOn())
&& Objects.equals(executionTime(), other.executionTime())
&& Objects.equals(recommendedRuleset(), other.recommendedRuleset())
&& Objects.equals(createdRulesetName(), other.createdRulesetName())
&& Objects.equals(dataQualitySecurityConfiguration(), other.dataQualitySecurityConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetDataQualityRuleRecommendationRunResponse").add("RunId", runId())
.add("DataSource", dataSource()).add("Role", role()).add("NumberOfWorkers", numberOfWorkers())
.add("Timeout", timeout()).add("Status", statusAsString()).add("ErrorString", errorString())
.add("StartedOn", startedOn()).add("LastModifiedOn", lastModifiedOn()).add("CompletedOn", completedOn())
.add("ExecutionTime", executionTime()).add("RecommendedRuleset", recommendedRuleset())
.add("CreatedRulesetName", createdRulesetName())
.add("DataQualitySecurityConfiguration", dataQualitySecurityConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RunId":
return Optional.ofNullable(clazz.cast(runId()));
case "DataSource":
return Optional.ofNullable(clazz.cast(dataSource()));
case "Role":
return Optional.ofNullable(clazz.cast(role()));
case "NumberOfWorkers":
return Optional.ofNullable(clazz.cast(numberOfWorkers()));
case "Timeout":
return Optional.ofNullable(clazz.cast(timeout()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "ErrorString":
return Optional.ofNullable(clazz.cast(errorString()));
case "StartedOn":
return Optional.ofNullable(clazz.cast(startedOn()));
case "LastModifiedOn":
return Optional.ofNullable(clazz.cast(lastModifiedOn()));
case "CompletedOn":
return Optional.ofNullable(clazz.cast(completedOn()));
case "ExecutionTime":
return Optional.ofNullable(clazz.cast(executionTime()));
case "RecommendedRuleset":
return Optional.ofNullable(clazz.cast(recommendedRuleset()));
case "CreatedRulesetName":
return Optional.ofNullable(clazz.cast(createdRulesetName()));
case "DataQualitySecurityConfiguration":
return Optional.ofNullable(clazz.cast(dataQualitySecurityConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function