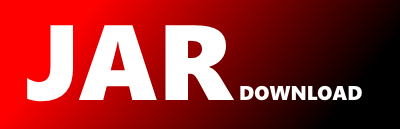
software.amazon.awssdk.services.glue.model.RemoveSchemaVersionMetadataResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glue Show documentation
Show all versions of glue Show documentation
The AWS Java SDK for AWS Glue module holds the client classes that are used for communicating
with AWS Glue Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class RemoveSchemaVersionMetadataResponse extends GlueResponse implements
ToCopyableBuilder {
private static final SdkField SCHEMA_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaArn").getter(getter(RemoveSchemaVersionMetadataResponse::schemaArn))
.setter(setter(Builder::schemaArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaArn").build()).build();
private static final SdkField SCHEMA_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaName").getter(getter(RemoveSchemaVersionMetadataResponse::schemaName))
.setter(setter(Builder::schemaName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaName").build()).build();
private static final SdkField REGISTRY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RegistryName").getter(getter(RemoveSchemaVersionMetadataResponse::registryName))
.setter(setter(Builder::registryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegistryName").build()).build();
private static final SdkField LATEST_VERSION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("LatestVersion").getter(getter(RemoveSchemaVersionMetadataResponse::latestVersion))
.setter(setter(Builder::latestVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LatestVersion").build()).build();
private static final SdkField VERSION_NUMBER_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("VersionNumber").getter(getter(RemoveSchemaVersionMetadataResponse::versionNumber))
.setter(setter(Builder::versionNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionNumber").build()).build();
private static final SdkField SCHEMA_VERSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaVersionId").getter(getter(RemoveSchemaVersionMetadataResponse::schemaVersionId))
.setter(setter(Builder::schemaVersionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaVersionId").build()).build();
private static final SdkField METADATA_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetadataKey").getter(getter(RemoveSchemaVersionMetadataResponse::metadataKey))
.setter(setter(Builder::metadataKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetadataKey").build()).build();
private static final SdkField METADATA_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetadataValue").getter(getter(RemoveSchemaVersionMetadataResponse::metadataValue))
.setter(setter(Builder::metadataValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetadataValue").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SCHEMA_ARN_FIELD,
SCHEMA_NAME_FIELD, REGISTRY_NAME_FIELD, LATEST_VERSION_FIELD, VERSION_NUMBER_FIELD, SCHEMA_VERSION_ID_FIELD,
METADATA_KEY_FIELD, METADATA_VALUE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("SchemaArn", SCHEMA_ARN_FIELD);
put("SchemaName", SCHEMA_NAME_FIELD);
put("RegistryName", REGISTRY_NAME_FIELD);
put("LatestVersion", LATEST_VERSION_FIELD);
put("VersionNumber", VERSION_NUMBER_FIELD);
put("SchemaVersionId", SCHEMA_VERSION_ID_FIELD);
put("MetadataKey", METADATA_KEY_FIELD);
put("MetadataValue", METADATA_VALUE_FIELD);
}
});
private final String schemaArn;
private final String schemaName;
private final String registryName;
private final Boolean latestVersion;
private final Long versionNumber;
private final String schemaVersionId;
private final String metadataKey;
private final String metadataValue;
private RemoveSchemaVersionMetadataResponse(BuilderImpl builder) {
super(builder);
this.schemaArn = builder.schemaArn;
this.schemaName = builder.schemaName;
this.registryName = builder.registryName;
this.latestVersion = builder.latestVersion;
this.versionNumber = builder.versionNumber;
this.schemaVersionId = builder.schemaVersionId;
this.metadataKey = builder.metadataKey;
this.metadataValue = builder.metadataValue;
}
/**
*
* The Amazon Resource Name (ARN) of the schema.
*
*
* @return The Amazon Resource Name (ARN) of the schema.
*/
public final String schemaArn() {
return schemaArn;
}
/**
*
* The name of the schema.
*
*
* @return The name of the schema.
*/
public final String schemaName() {
return schemaName;
}
/**
*
* The name of the registry.
*
*
* @return The name of the registry.
*/
public final String registryName() {
return registryName;
}
/**
*
* The latest version of the schema.
*
*
* @return The latest version of the schema.
*/
public final Boolean latestVersion() {
return latestVersion;
}
/**
*
* The version number of the schema.
*
*
* @return The version number of the schema.
*/
public final Long versionNumber() {
return versionNumber;
}
/**
*
* The version ID for the schema version.
*
*
* @return The version ID for the schema version.
*/
public final String schemaVersionId() {
return schemaVersionId;
}
/**
*
* The metadata key.
*
*
* @return The metadata key.
*/
public final String metadataKey() {
return metadataKey;
}
/**
*
* The value of the metadata key.
*
*
* @return The value of the metadata key.
*/
public final String metadataValue() {
return metadataValue;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(schemaArn());
hashCode = 31 * hashCode + Objects.hashCode(schemaName());
hashCode = 31 * hashCode + Objects.hashCode(registryName());
hashCode = 31 * hashCode + Objects.hashCode(latestVersion());
hashCode = 31 * hashCode + Objects.hashCode(versionNumber());
hashCode = 31 * hashCode + Objects.hashCode(schemaVersionId());
hashCode = 31 * hashCode + Objects.hashCode(metadataKey());
hashCode = 31 * hashCode + Objects.hashCode(metadataValue());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RemoveSchemaVersionMetadataResponse)) {
return false;
}
RemoveSchemaVersionMetadataResponse other = (RemoveSchemaVersionMetadataResponse) obj;
return Objects.equals(schemaArn(), other.schemaArn()) && Objects.equals(schemaName(), other.schemaName())
&& Objects.equals(registryName(), other.registryName()) && Objects.equals(latestVersion(), other.latestVersion())
&& Objects.equals(versionNumber(), other.versionNumber())
&& Objects.equals(schemaVersionId(), other.schemaVersionId())
&& Objects.equals(metadataKey(), other.metadataKey()) && Objects.equals(metadataValue(), other.metadataValue());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RemoveSchemaVersionMetadataResponse").add("SchemaArn", schemaArn())
.add("SchemaName", schemaName()).add("RegistryName", registryName()).add("LatestVersion", latestVersion())
.add("VersionNumber", versionNumber()).add("SchemaVersionId", schemaVersionId())
.add("MetadataKey", metadataKey()).add("MetadataValue", metadataValue()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SchemaArn":
return Optional.ofNullable(clazz.cast(schemaArn()));
case "SchemaName":
return Optional.ofNullable(clazz.cast(schemaName()));
case "RegistryName":
return Optional.ofNullable(clazz.cast(registryName()));
case "LatestVersion":
return Optional.ofNullable(clazz.cast(latestVersion()));
case "VersionNumber":
return Optional.ofNullable(clazz.cast(versionNumber()));
case "SchemaVersionId":
return Optional.ofNullable(clazz.cast(schemaVersionId()));
case "MetadataKey":
return Optional.ofNullable(clazz.cast(metadataKey()));
case "MetadataValue":
return Optional.ofNullable(clazz.cast(metadataValue()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy