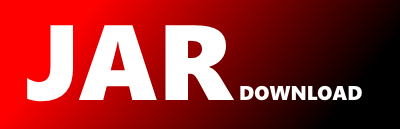
software.amazon.awssdk.services.glue.model.WorkflowRunStatistics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glue Show documentation
Show all versions of glue Show documentation
The AWS Java SDK for AWS Glue module holds the client classes that are used for communicating
with AWS Glue Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Workflow run statistics provides statistics about the workflow run.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class WorkflowRunStatistics implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TOTAL_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TotalActions").getter(getter(WorkflowRunStatistics::totalActions)).setter(setter(Builder::totalActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalActions").build()).build();
private static final SdkField TIMEOUT_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TimeoutActions").getter(getter(WorkflowRunStatistics::timeoutActions))
.setter(setter(Builder::timeoutActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeoutActions").build()).build();
private static final SdkField FAILED_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("FailedActions").getter(getter(WorkflowRunStatistics::failedActions))
.setter(setter(Builder::failedActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailedActions").build()).build();
private static final SdkField STOPPED_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StoppedActions").getter(getter(WorkflowRunStatistics::stoppedActions))
.setter(setter(Builder::stoppedActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StoppedActions").build()).build();
private static final SdkField SUCCEEDED_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SucceededActions").getter(getter(WorkflowRunStatistics::succeededActions))
.setter(setter(Builder::succeededActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SucceededActions").build()).build();
private static final SdkField RUNNING_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RunningActions").getter(getter(WorkflowRunStatistics::runningActions))
.setter(setter(Builder::runningActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RunningActions").build()).build();
private static final SdkField ERRORED_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ErroredActions").getter(getter(WorkflowRunStatistics::erroredActions))
.setter(setter(Builder::erroredActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ErroredActions").build()).build();
private static final SdkField WAITING_ACTIONS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("WaitingActions").getter(getter(WorkflowRunStatistics::waitingActions))
.setter(setter(Builder::waitingActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WaitingActions").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TOTAL_ACTIONS_FIELD,
TIMEOUT_ACTIONS_FIELD, FAILED_ACTIONS_FIELD, STOPPED_ACTIONS_FIELD, SUCCEEDED_ACTIONS_FIELD, RUNNING_ACTIONS_FIELD,
ERRORED_ACTIONS_FIELD, WAITING_ACTIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("TotalActions", TOTAL_ACTIONS_FIELD);
put("TimeoutActions", TIMEOUT_ACTIONS_FIELD);
put("FailedActions", FAILED_ACTIONS_FIELD);
put("StoppedActions", STOPPED_ACTIONS_FIELD);
put("SucceededActions", SUCCEEDED_ACTIONS_FIELD);
put("RunningActions", RUNNING_ACTIONS_FIELD);
put("ErroredActions", ERRORED_ACTIONS_FIELD);
put("WaitingActions", WAITING_ACTIONS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final Integer totalActions;
private final Integer timeoutActions;
private final Integer failedActions;
private final Integer stoppedActions;
private final Integer succeededActions;
private final Integer runningActions;
private final Integer erroredActions;
private final Integer waitingActions;
private WorkflowRunStatistics(BuilderImpl builder) {
this.totalActions = builder.totalActions;
this.timeoutActions = builder.timeoutActions;
this.failedActions = builder.failedActions;
this.stoppedActions = builder.stoppedActions;
this.succeededActions = builder.succeededActions;
this.runningActions = builder.runningActions;
this.erroredActions = builder.erroredActions;
this.waitingActions = builder.waitingActions;
}
/**
*
* Total number of Actions in the workflow run.
*
*
* @return Total number of Actions in the workflow run.
*/
public final Integer totalActions() {
return totalActions;
}
/**
*
* Total number of Actions that timed out.
*
*
* @return Total number of Actions that timed out.
*/
public final Integer timeoutActions() {
return timeoutActions;
}
/**
*
* Total number of Actions that have failed.
*
*
* @return Total number of Actions that have failed.
*/
public final Integer failedActions() {
return failedActions;
}
/**
*
* Total number of Actions that have stopped.
*
*
* @return Total number of Actions that have stopped.
*/
public final Integer stoppedActions() {
return stoppedActions;
}
/**
*
* Total number of Actions that have succeeded.
*
*
* @return Total number of Actions that have succeeded.
*/
public final Integer succeededActions() {
return succeededActions;
}
/**
*
* Total number Actions in running state.
*
*
* @return Total number Actions in running state.
*/
public final Integer runningActions() {
return runningActions;
}
/**
*
* Indicates the count of job runs in the ERROR state in the workflow run.
*
*
* @return Indicates the count of job runs in the ERROR state in the workflow run.
*/
public final Integer erroredActions() {
return erroredActions;
}
/**
*
* Indicates the count of job runs in WAITING state in the workflow run.
*
*
* @return Indicates the count of job runs in WAITING state in the workflow run.
*/
public final Integer waitingActions() {
return waitingActions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(totalActions());
hashCode = 31 * hashCode + Objects.hashCode(timeoutActions());
hashCode = 31 * hashCode + Objects.hashCode(failedActions());
hashCode = 31 * hashCode + Objects.hashCode(stoppedActions());
hashCode = 31 * hashCode + Objects.hashCode(succeededActions());
hashCode = 31 * hashCode + Objects.hashCode(runningActions());
hashCode = 31 * hashCode + Objects.hashCode(erroredActions());
hashCode = 31 * hashCode + Objects.hashCode(waitingActions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof WorkflowRunStatistics)) {
return false;
}
WorkflowRunStatistics other = (WorkflowRunStatistics) obj;
return Objects.equals(totalActions(), other.totalActions()) && Objects.equals(timeoutActions(), other.timeoutActions())
&& Objects.equals(failedActions(), other.failedActions())
&& Objects.equals(stoppedActions(), other.stoppedActions())
&& Objects.equals(succeededActions(), other.succeededActions())
&& Objects.equals(runningActions(), other.runningActions())
&& Objects.equals(erroredActions(), other.erroredActions())
&& Objects.equals(waitingActions(), other.waitingActions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("WorkflowRunStatistics").add("TotalActions", totalActions())
.add("TimeoutActions", timeoutActions()).add("FailedActions", failedActions())
.add("StoppedActions", stoppedActions()).add("SucceededActions", succeededActions())
.add("RunningActions", runningActions()).add("ErroredActions", erroredActions())
.add("WaitingActions", waitingActions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TotalActions":
return Optional.ofNullable(clazz.cast(totalActions()));
case "TimeoutActions":
return Optional.ofNullable(clazz.cast(timeoutActions()));
case "FailedActions":
return Optional.ofNullable(clazz.cast(failedActions()));
case "StoppedActions":
return Optional.ofNullable(clazz.cast(stoppedActions()));
case "SucceededActions":
return Optional.ofNullable(clazz.cast(succeededActions()));
case "RunningActions":
return Optional.ofNullable(clazz.cast(runningActions()));
case "ErroredActions":
return Optional.ofNullable(clazz.cast(erroredActions()));
case "WaitingActions":
return Optional.ofNullable(clazz.cast(waitingActions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy