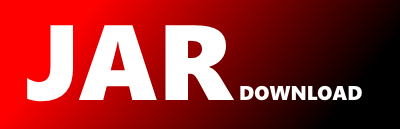
software.amazon.awssdk.services.glue.DefaultGlueAsyncClient Maven / Gradle / Ivy
Show all versions of glue Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.glue.internal.GlueServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.glue.model.AccessDeniedException;
import software.amazon.awssdk.services.glue.model.AlreadyExistsException;
import software.amazon.awssdk.services.glue.model.BatchCreatePartitionRequest;
import software.amazon.awssdk.services.glue.model.BatchCreatePartitionResponse;
import software.amazon.awssdk.services.glue.model.BatchDeleteConnectionRequest;
import software.amazon.awssdk.services.glue.model.BatchDeleteConnectionResponse;
import software.amazon.awssdk.services.glue.model.BatchDeletePartitionRequest;
import software.amazon.awssdk.services.glue.model.BatchDeletePartitionResponse;
import software.amazon.awssdk.services.glue.model.BatchDeleteTableRequest;
import software.amazon.awssdk.services.glue.model.BatchDeleteTableResponse;
import software.amazon.awssdk.services.glue.model.BatchDeleteTableVersionRequest;
import software.amazon.awssdk.services.glue.model.BatchDeleteTableVersionResponse;
import software.amazon.awssdk.services.glue.model.BatchGetBlueprintsRequest;
import software.amazon.awssdk.services.glue.model.BatchGetBlueprintsResponse;
import software.amazon.awssdk.services.glue.model.BatchGetCrawlersRequest;
import software.amazon.awssdk.services.glue.model.BatchGetCrawlersResponse;
import software.amazon.awssdk.services.glue.model.BatchGetCustomEntityTypesRequest;
import software.amazon.awssdk.services.glue.model.BatchGetCustomEntityTypesResponse;
import software.amazon.awssdk.services.glue.model.BatchGetDataQualityResultRequest;
import software.amazon.awssdk.services.glue.model.BatchGetDataQualityResultResponse;
import software.amazon.awssdk.services.glue.model.BatchGetDevEndpointsRequest;
import software.amazon.awssdk.services.glue.model.BatchGetDevEndpointsResponse;
import software.amazon.awssdk.services.glue.model.BatchGetJobsRequest;
import software.amazon.awssdk.services.glue.model.BatchGetJobsResponse;
import software.amazon.awssdk.services.glue.model.BatchGetPartitionRequest;
import software.amazon.awssdk.services.glue.model.BatchGetPartitionResponse;
import software.amazon.awssdk.services.glue.model.BatchGetTableOptimizerRequest;
import software.amazon.awssdk.services.glue.model.BatchGetTableOptimizerResponse;
import software.amazon.awssdk.services.glue.model.BatchGetTriggersRequest;
import software.amazon.awssdk.services.glue.model.BatchGetTriggersResponse;
import software.amazon.awssdk.services.glue.model.BatchGetWorkflowsRequest;
import software.amazon.awssdk.services.glue.model.BatchGetWorkflowsResponse;
import software.amazon.awssdk.services.glue.model.BatchPutDataQualityStatisticAnnotationRequest;
import software.amazon.awssdk.services.glue.model.BatchPutDataQualityStatisticAnnotationResponse;
import software.amazon.awssdk.services.glue.model.BatchStopJobRunRequest;
import software.amazon.awssdk.services.glue.model.BatchStopJobRunResponse;
import software.amazon.awssdk.services.glue.model.BatchUpdatePartitionRequest;
import software.amazon.awssdk.services.glue.model.BatchUpdatePartitionResponse;
import software.amazon.awssdk.services.glue.model.CancelDataQualityRuleRecommendationRunRequest;
import software.amazon.awssdk.services.glue.model.CancelDataQualityRuleRecommendationRunResponse;
import software.amazon.awssdk.services.glue.model.CancelDataQualityRulesetEvaluationRunRequest;
import software.amazon.awssdk.services.glue.model.CancelDataQualityRulesetEvaluationRunResponse;
import software.amazon.awssdk.services.glue.model.CancelMlTaskRunRequest;
import software.amazon.awssdk.services.glue.model.CancelMlTaskRunResponse;
import software.amazon.awssdk.services.glue.model.CancelStatementRequest;
import software.amazon.awssdk.services.glue.model.CancelStatementResponse;
import software.amazon.awssdk.services.glue.model.CheckSchemaVersionValidityRequest;
import software.amazon.awssdk.services.glue.model.CheckSchemaVersionValidityResponse;
import software.amazon.awssdk.services.glue.model.ColumnStatisticsTaskNotRunningException;
import software.amazon.awssdk.services.glue.model.ColumnStatisticsTaskRunningException;
import software.amazon.awssdk.services.glue.model.ColumnStatisticsTaskStoppingException;
import software.amazon.awssdk.services.glue.model.ConcurrentModificationException;
import software.amazon.awssdk.services.glue.model.ConcurrentRunsExceededException;
import software.amazon.awssdk.services.glue.model.ConditionCheckFailureException;
import software.amazon.awssdk.services.glue.model.ConflictException;
import software.amazon.awssdk.services.glue.model.CrawlerNotRunningException;
import software.amazon.awssdk.services.glue.model.CrawlerRunningException;
import software.amazon.awssdk.services.glue.model.CrawlerStoppingException;
import software.amazon.awssdk.services.glue.model.CreateBlueprintRequest;
import software.amazon.awssdk.services.glue.model.CreateBlueprintResponse;
import software.amazon.awssdk.services.glue.model.CreateCatalogRequest;
import software.amazon.awssdk.services.glue.model.CreateCatalogResponse;
import software.amazon.awssdk.services.glue.model.CreateClassifierRequest;
import software.amazon.awssdk.services.glue.model.CreateClassifierResponse;
import software.amazon.awssdk.services.glue.model.CreateColumnStatisticsTaskSettingsRequest;
import software.amazon.awssdk.services.glue.model.CreateColumnStatisticsTaskSettingsResponse;
import software.amazon.awssdk.services.glue.model.CreateConnectionRequest;
import software.amazon.awssdk.services.glue.model.CreateConnectionResponse;
import software.amazon.awssdk.services.glue.model.CreateCrawlerRequest;
import software.amazon.awssdk.services.glue.model.CreateCrawlerResponse;
import software.amazon.awssdk.services.glue.model.CreateCustomEntityTypeRequest;
import software.amazon.awssdk.services.glue.model.CreateCustomEntityTypeResponse;
import software.amazon.awssdk.services.glue.model.CreateDataQualityRulesetRequest;
import software.amazon.awssdk.services.glue.model.CreateDataQualityRulesetResponse;
import software.amazon.awssdk.services.glue.model.CreateDatabaseRequest;
import software.amazon.awssdk.services.glue.model.CreateDatabaseResponse;
import software.amazon.awssdk.services.glue.model.CreateDevEndpointRequest;
import software.amazon.awssdk.services.glue.model.CreateDevEndpointResponse;
import software.amazon.awssdk.services.glue.model.CreateIntegrationRequest;
import software.amazon.awssdk.services.glue.model.CreateIntegrationResourcePropertyRequest;
import software.amazon.awssdk.services.glue.model.CreateIntegrationResourcePropertyResponse;
import software.amazon.awssdk.services.glue.model.CreateIntegrationResponse;
import software.amazon.awssdk.services.glue.model.CreateIntegrationTablePropertiesRequest;
import software.amazon.awssdk.services.glue.model.CreateIntegrationTablePropertiesResponse;
import software.amazon.awssdk.services.glue.model.CreateJobRequest;
import software.amazon.awssdk.services.glue.model.CreateJobResponse;
import software.amazon.awssdk.services.glue.model.CreateMlTransformRequest;
import software.amazon.awssdk.services.glue.model.CreateMlTransformResponse;
import software.amazon.awssdk.services.glue.model.CreatePartitionIndexRequest;
import software.amazon.awssdk.services.glue.model.CreatePartitionIndexResponse;
import software.amazon.awssdk.services.glue.model.CreatePartitionRequest;
import software.amazon.awssdk.services.glue.model.CreatePartitionResponse;
import software.amazon.awssdk.services.glue.model.CreateRegistryRequest;
import software.amazon.awssdk.services.glue.model.CreateRegistryResponse;
import software.amazon.awssdk.services.glue.model.CreateSchemaRequest;
import software.amazon.awssdk.services.glue.model.CreateSchemaResponse;
import software.amazon.awssdk.services.glue.model.CreateScriptRequest;
import software.amazon.awssdk.services.glue.model.CreateScriptResponse;
import software.amazon.awssdk.services.glue.model.CreateSecurityConfigurationRequest;
import software.amazon.awssdk.services.glue.model.CreateSecurityConfigurationResponse;
import software.amazon.awssdk.services.glue.model.CreateSessionRequest;
import software.amazon.awssdk.services.glue.model.CreateSessionResponse;
import software.amazon.awssdk.services.glue.model.CreateTableOptimizerRequest;
import software.amazon.awssdk.services.glue.model.CreateTableOptimizerResponse;
import software.amazon.awssdk.services.glue.model.CreateTableRequest;
import software.amazon.awssdk.services.glue.model.CreateTableResponse;
import software.amazon.awssdk.services.glue.model.CreateTriggerRequest;
import software.amazon.awssdk.services.glue.model.CreateTriggerResponse;
import software.amazon.awssdk.services.glue.model.CreateUsageProfileRequest;
import software.amazon.awssdk.services.glue.model.CreateUsageProfileResponse;
import software.amazon.awssdk.services.glue.model.CreateUserDefinedFunctionRequest;
import software.amazon.awssdk.services.glue.model.CreateUserDefinedFunctionResponse;
import software.amazon.awssdk.services.glue.model.CreateWorkflowRequest;
import software.amazon.awssdk.services.glue.model.CreateWorkflowResponse;
import software.amazon.awssdk.services.glue.model.DeleteBlueprintRequest;
import software.amazon.awssdk.services.glue.model.DeleteBlueprintResponse;
import software.amazon.awssdk.services.glue.model.DeleteCatalogRequest;
import software.amazon.awssdk.services.glue.model.DeleteCatalogResponse;
import software.amazon.awssdk.services.glue.model.DeleteClassifierRequest;
import software.amazon.awssdk.services.glue.model.DeleteClassifierResponse;
import software.amazon.awssdk.services.glue.model.DeleteColumnStatisticsForPartitionRequest;
import software.amazon.awssdk.services.glue.model.DeleteColumnStatisticsForPartitionResponse;
import software.amazon.awssdk.services.glue.model.DeleteColumnStatisticsForTableRequest;
import software.amazon.awssdk.services.glue.model.DeleteColumnStatisticsForTableResponse;
import software.amazon.awssdk.services.glue.model.DeleteColumnStatisticsTaskSettingsRequest;
import software.amazon.awssdk.services.glue.model.DeleteColumnStatisticsTaskSettingsResponse;
import software.amazon.awssdk.services.glue.model.DeleteConnectionRequest;
import software.amazon.awssdk.services.glue.model.DeleteConnectionResponse;
import software.amazon.awssdk.services.glue.model.DeleteCrawlerRequest;
import software.amazon.awssdk.services.glue.model.DeleteCrawlerResponse;
import software.amazon.awssdk.services.glue.model.DeleteCustomEntityTypeRequest;
import software.amazon.awssdk.services.glue.model.DeleteCustomEntityTypeResponse;
import software.amazon.awssdk.services.glue.model.DeleteDataQualityRulesetRequest;
import software.amazon.awssdk.services.glue.model.DeleteDataQualityRulesetResponse;
import software.amazon.awssdk.services.glue.model.DeleteDatabaseRequest;
import software.amazon.awssdk.services.glue.model.DeleteDatabaseResponse;
import software.amazon.awssdk.services.glue.model.DeleteDevEndpointRequest;
import software.amazon.awssdk.services.glue.model.DeleteDevEndpointResponse;
import software.amazon.awssdk.services.glue.model.DeleteIntegrationRequest;
import software.amazon.awssdk.services.glue.model.DeleteIntegrationResponse;
import software.amazon.awssdk.services.glue.model.DeleteIntegrationTablePropertiesRequest;
import software.amazon.awssdk.services.glue.model.DeleteIntegrationTablePropertiesResponse;
import software.amazon.awssdk.services.glue.model.DeleteJobRequest;
import software.amazon.awssdk.services.glue.model.DeleteJobResponse;
import software.amazon.awssdk.services.glue.model.DeleteMlTransformRequest;
import software.amazon.awssdk.services.glue.model.DeleteMlTransformResponse;
import software.amazon.awssdk.services.glue.model.DeletePartitionIndexRequest;
import software.amazon.awssdk.services.glue.model.DeletePartitionIndexResponse;
import software.amazon.awssdk.services.glue.model.DeletePartitionRequest;
import software.amazon.awssdk.services.glue.model.DeletePartitionResponse;
import software.amazon.awssdk.services.glue.model.DeleteRegistryRequest;
import software.amazon.awssdk.services.glue.model.DeleteRegistryResponse;
import software.amazon.awssdk.services.glue.model.DeleteResourcePolicyRequest;
import software.amazon.awssdk.services.glue.model.DeleteResourcePolicyResponse;
import software.amazon.awssdk.services.glue.model.DeleteSchemaRequest;
import software.amazon.awssdk.services.glue.model.DeleteSchemaResponse;
import software.amazon.awssdk.services.glue.model.DeleteSchemaVersionsRequest;
import software.amazon.awssdk.services.glue.model.DeleteSchemaVersionsResponse;
import software.amazon.awssdk.services.glue.model.DeleteSecurityConfigurationRequest;
import software.amazon.awssdk.services.glue.model.DeleteSecurityConfigurationResponse;
import software.amazon.awssdk.services.glue.model.DeleteSessionRequest;
import software.amazon.awssdk.services.glue.model.DeleteSessionResponse;
import software.amazon.awssdk.services.glue.model.DeleteTableOptimizerRequest;
import software.amazon.awssdk.services.glue.model.DeleteTableOptimizerResponse;
import software.amazon.awssdk.services.glue.model.DeleteTableRequest;
import software.amazon.awssdk.services.glue.model.DeleteTableResponse;
import software.amazon.awssdk.services.glue.model.DeleteTableVersionRequest;
import software.amazon.awssdk.services.glue.model.DeleteTableVersionResponse;
import software.amazon.awssdk.services.glue.model.DeleteTriggerRequest;
import software.amazon.awssdk.services.glue.model.DeleteTriggerResponse;
import software.amazon.awssdk.services.glue.model.DeleteUsageProfileRequest;
import software.amazon.awssdk.services.glue.model.DeleteUsageProfileResponse;
import software.amazon.awssdk.services.glue.model.DeleteUserDefinedFunctionRequest;
import software.amazon.awssdk.services.glue.model.DeleteUserDefinedFunctionResponse;
import software.amazon.awssdk.services.glue.model.DeleteWorkflowRequest;
import software.amazon.awssdk.services.glue.model.DeleteWorkflowResponse;
import software.amazon.awssdk.services.glue.model.DescribeConnectionTypeRequest;
import software.amazon.awssdk.services.glue.model.DescribeConnectionTypeResponse;
import software.amazon.awssdk.services.glue.model.DescribeEntityRequest;
import software.amazon.awssdk.services.glue.model.DescribeEntityResponse;
import software.amazon.awssdk.services.glue.model.DescribeInboundIntegrationsRequest;
import software.amazon.awssdk.services.glue.model.DescribeInboundIntegrationsResponse;
import software.amazon.awssdk.services.glue.model.DescribeIntegrationsRequest;
import software.amazon.awssdk.services.glue.model.DescribeIntegrationsResponse;
import software.amazon.awssdk.services.glue.model.EntityNotFoundException;
import software.amazon.awssdk.services.glue.model.FederatedResourceAlreadyExistsException;
import software.amazon.awssdk.services.glue.model.FederationSourceException;
import software.amazon.awssdk.services.glue.model.FederationSourceRetryableException;
import software.amazon.awssdk.services.glue.model.GetBlueprintRequest;
import software.amazon.awssdk.services.glue.model.GetBlueprintResponse;
import software.amazon.awssdk.services.glue.model.GetBlueprintRunRequest;
import software.amazon.awssdk.services.glue.model.GetBlueprintRunResponse;
import software.amazon.awssdk.services.glue.model.GetBlueprintRunsRequest;
import software.amazon.awssdk.services.glue.model.GetBlueprintRunsResponse;
import software.amazon.awssdk.services.glue.model.GetCatalogImportStatusRequest;
import software.amazon.awssdk.services.glue.model.GetCatalogImportStatusResponse;
import software.amazon.awssdk.services.glue.model.GetCatalogRequest;
import software.amazon.awssdk.services.glue.model.GetCatalogResponse;
import software.amazon.awssdk.services.glue.model.GetCatalogsRequest;
import software.amazon.awssdk.services.glue.model.GetCatalogsResponse;
import software.amazon.awssdk.services.glue.model.GetClassifierRequest;
import software.amazon.awssdk.services.glue.model.GetClassifierResponse;
import software.amazon.awssdk.services.glue.model.GetClassifiersRequest;
import software.amazon.awssdk.services.glue.model.GetClassifiersResponse;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsForPartitionRequest;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsForPartitionResponse;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsForTableRequest;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsForTableResponse;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsTaskRunRequest;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsTaskRunResponse;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsTaskRunsRequest;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsTaskRunsResponse;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsTaskSettingsRequest;
import software.amazon.awssdk.services.glue.model.GetColumnStatisticsTaskSettingsResponse;
import software.amazon.awssdk.services.glue.model.GetConnectionRequest;
import software.amazon.awssdk.services.glue.model.GetConnectionResponse;
import software.amazon.awssdk.services.glue.model.GetConnectionsRequest;
import software.amazon.awssdk.services.glue.model.GetConnectionsResponse;
import software.amazon.awssdk.services.glue.model.GetCrawlerMetricsRequest;
import software.amazon.awssdk.services.glue.model.GetCrawlerMetricsResponse;
import software.amazon.awssdk.services.glue.model.GetCrawlerRequest;
import software.amazon.awssdk.services.glue.model.GetCrawlerResponse;
import software.amazon.awssdk.services.glue.model.GetCrawlersRequest;
import software.amazon.awssdk.services.glue.model.GetCrawlersResponse;
import software.amazon.awssdk.services.glue.model.GetCustomEntityTypeRequest;
import software.amazon.awssdk.services.glue.model.GetCustomEntityTypeResponse;
import software.amazon.awssdk.services.glue.model.GetDataCatalogEncryptionSettingsRequest;
import software.amazon.awssdk.services.glue.model.GetDataCatalogEncryptionSettingsResponse;
import software.amazon.awssdk.services.glue.model.GetDataQualityModelRequest;
import software.amazon.awssdk.services.glue.model.GetDataQualityModelResponse;
import software.amazon.awssdk.services.glue.model.GetDataQualityModelResultRequest;
import software.amazon.awssdk.services.glue.model.GetDataQualityModelResultResponse;
import software.amazon.awssdk.services.glue.model.GetDataQualityResultRequest;
import software.amazon.awssdk.services.glue.model.GetDataQualityResultResponse;
import software.amazon.awssdk.services.glue.model.GetDataQualityRuleRecommendationRunRequest;
import software.amazon.awssdk.services.glue.model.GetDataQualityRuleRecommendationRunResponse;
import software.amazon.awssdk.services.glue.model.GetDataQualityRulesetEvaluationRunRequest;
import software.amazon.awssdk.services.glue.model.GetDataQualityRulesetEvaluationRunResponse;
import software.amazon.awssdk.services.glue.model.GetDataQualityRulesetRequest;
import software.amazon.awssdk.services.glue.model.GetDataQualityRulesetResponse;
import software.amazon.awssdk.services.glue.model.GetDatabaseRequest;
import software.amazon.awssdk.services.glue.model.GetDatabaseResponse;
import software.amazon.awssdk.services.glue.model.GetDatabasesRequest;
import software.amazon.awssdk.services.glue.model.GetDatabasesResponse;
import software.amazon.awssdk.services.glue.model.GetDataflowGraphRequest;
import software.amazon.awssdk.services.glue.model.GetDataflowGraphResponse;
import software.amazon.awssdk.services.glue.model.GetDevEndpointRequest;
import software.amazon.awssdk.services.glue.model.GetDevEndpointResponse;
import software.amazon.awssdk.services.glue.model.GetDevEndpointsRequest;
import software.amazon.awssdk.services.glue.model.GetDevEndpointsResponse;
import software.amazon.awssdk.services.glue.model.GetEntityRecordsRequest;
import software.amazon.awssdk.services.glue.model.GetEntityRecordsResponse;
import software.amazon.awssdk.services.glue.model.GetIntegrationResourcePropertyRequest;
import software.amazon.awssdk.services.glue.model.GetIntegrationResourcePropertyResponse;
import software.amazon.awssdk.services.glue.model.GetIntegrationTablePropertiesRequest;
import software.amazon.awssdk.services.glue.model.GetIntegrationTablePropertiesResponse;
import software.amazon.awssdk.services.glue.model.GetJobBookmarkRequest;
import software.amazon.awssdk.services.glue.model.GetJobBookmarkResponse;
import software.amazon.awssdk.services.glue.model.GetJobRequest;
import software.amazon.awssdk.services.glue.model.GetJobResponse;
import software.amazon.awssdk.services.glue.model.GetJobRunRequest;
import software.amazon.awssdk.services.glue.model.GetJobRunResponse;
import software.amazon.awssdk.services.glue.model.GetJobRunsRequest;
import software.amazon.awssdk.services.glue.model.GetJobRunsResponse;
import software.amazon.awssdk.services.glue.model.GetJobsRequest;
import software.amazon.awssdk.services.glue.model.GetJobsResponse;
import software.amazon.awssdk.services.glue.model.GetMappingRequest;
import software.amazon.awssdk.services.glue.model.GetMappingResponse;
import software.amazon.awssdk.services.glue.model.GetMlTaskRunRequest;
import software.amazon.awssdk.services.glue.model.GetMlTaskRunResponse;
import software.amazon.awssdk.services.glue.model.GetMlTaskRunsRequest;
import software.amazon.awssdk.services.glue.model.GetMlTaskRunsResponse;
import software.amazon.awssdk.services.glue.model.GetMlTransformRequest;
import software.amazon.awssdk.services.glue.model.GetMlTransformResponse;
import software.amazon.awssdk.services.glue.model.GetMlTransformsRequest;
import software.amazon.awssdk.services.glue.model.GetMlTransformsResponse;
import software.amazon.awssdk.services.glue.model.GetPartitionIndexesRequest;
import software.amazon.awssdk.services.glue.model.GetPartitionIndexesResponse;
import software.amazon.awssdk.services.glue.model.GetPartitionRequest;
import software.amazon.awssdk.services.glue.model.GetPartitionResponse;
import software.amazon.awssdk.services.glue.model.GetPartitionsRequest;
import software.amazon.awssdk.services.glue.model.GetPartitionsResponse;
import software.amazon.awssdk.services.glue.model.GetPlanRequest;
import software.amazon.awssdk.services.glue.model.GetPlanResponse;
import software.amazon.awssdk.services.glue.model.GetRegistryRequest;
import software.amazon.awssdk.services.glue.model.GetRegistryResponse;
import software.amazon.awssdk.services.glue.model.GetResourcePoliciesRequest;
import software.amazon.awssdk.services.glue.model.GetResourcePoliciesResponse;
import software.amazon.awssdk.services.glue.model.GetResourcePolicyRequest;
import software.amazon.awssdk.services.glue.model.GetResourcePolicyResponse;
import software.amazon.awssdk.services.glue.model.GetSchemaByDefinitionRequest;
import software.amazon.awssdk.services.glue.model.GetSchemaByDefinitionResponse;
import software.amazon.awssdk.services.glue.model.GetSchemaRequest;
import software.amazon.awssdk.services.glue.model.GetSchemaResponse;
import software.amazon.awssdk.services.glue.model.GetSchemaVersionRequest;
import software.amazon.awssdk.services.glue.model.GetSchemaVersionResponse;
import software.amazon.awssdk.services.glue.model.GetSchemaVersionsDiffRequest;
import software.amazon.awssdk.services.glue.model.GetSchemaVersionsDiffResponse;
import software.amazon.awssdk.services.glue.model.GetSecurityConfigurationRequest;
import software.amazon.awssdk.services.glue.model.GetSecurityConfigurationResponse;
import software.amazon.awssdk.services.glue.model.GetSecurityConfigurationsRequest;
import software.amazon.awssdk.services.glue.model.GetSecurityConfigurationsResponse;
import software.amazon.awssdk.services.glue.model.GetSessionRequest;
import software.amazon.awssdk.services.glue.model.GetSessionResponse;
import software.amazon.awssdk.services.glue.model.GetStatementRequest;
import software.amazon.awssdk.services.glue.model.GetStatementResponse;
import software.amazon.awssdk.services.glue.model.GetTableOptimizerRequest;
import software.amazon.awssdk.services.glue.model.GetTableOptimizerResponse;
import software.amazon.awssdk.services.glue.model.GetTableRequest;
import software.amazon.awssdk.services.glue.model.GetTableResponse;
import software.amazon.awssdk.services.glue.model.GetTableVersionRequest;
import software.amazon.awssdk.services.glue.model.GetTableVersionResponse;
import software.amazon.awssdk.services.glue.model.GetTableVersionsRequest;
import software.amazon.awssdk.services.glue.model.GetTableVersionsResponse;
import software.amazon.awssdk.services.glue.model.GetTablesRequest;
import software.amazon.awssdk.services.glue.model.GetTablesResponse;
import software.amazon.awssdk.services.glue.model.GetTagsRequest;
import software.amazon.awssdk.services.glue.model.GetTagsResponse;
import software.amazon.awssdk.services.glue.model.GetTriggerRequest;
import software.amazon.awssdk.services.glue.model.GetTriggerResponse;
import software.amazon.awssdk.services.glue.model.GetTriggersRequest;
import software.amazon.awssdk.services.glue.model.GetTriggersResponse;
import software.amazon.awssdk.services.glue.model.GetUnfilteredPartitionMetadataRequest;
import software.amazon.awssdk.services.glue.model.GetUnfilteredPartitionMetadataResponse;
import software.amazon.awssdk.services.glue.model.GetUnfilteredPartitionsMetadataRequest;
import software.amazon.awssdk.services.glue.model.GetUnfilteredPartitionsMetadataResponse;
import software.amazon.awssdk.services.glue.model.GetUnfilteredTableMetadataRequest;
import software.amazon.awssdk.services.glue.model.GetUnfilteredTableMetadataResponse;
import software.amazon.awssdk.services.glue.model.GetUsageProfileRequest;
import software.amazon.awssdk.services.glue.model.GetUsageProfileResponse;
import software.amazon.awssdk.services.glue.model.GetUserDefinedFunctionRequest;
import software.amazon.awssdk.services.glue.model.GetUserDefinedFunctionResponse;
import software.amazon.awssdk.services.glue.model.GetUserDefinedFunctionsRequest;
import software.amazon.awssdk.services.glue.model.GetUserDefinedFunctionsResponse;
import software.amazon.awssdk.services.glue.model.GetWorkflowRequest;
import software.amazon.awssdk.services.glue.model.GetWorkflowResponse;
import software.amazon.awssdk.services.glue.model.GetWorkflowRunPropertiesRequest;
import software.amazon.awssdk.services.glue.model.GetWorkflowRunPropertiesResponse;
import software.amazon.awssdk.services.glue.model.GetWorkflowRunRequest;
import software.amazon.awssdk.services.glue.model.GetWorkflowRunResponse;
import software.amazon.awssdk.services.glue.model.GetWorkflowRunsRequest;
import software.amazon.awssdk.services.glue.model.GetWorkflowRunsResponse;
import software.amazon.awssdk.services.glue.model.GlueEncryptionException;
import software.amazon.awssdk.services.glue.model.GlueException;
import software.amazon.awssdk.services.glue.model.IdempotentParameterMismatchException;
import software.amazon.awssdk.services.glue.model.IllegalBlueprintStateException;
import software.amazon.awssdk.services.glue.model.IllegalSessionStateException;
import software.amazon.awssdk.services.glue.model.IllegalWorkflowStateException;
import software.amazon.awssdk.services.glue.model.ImportCatalogToGlueRequest;
import software.amazon.awssdk.services.glue.model.ImportCatalogToGlueResponse;
import software.amazon.awssdk.services.glue.model.IntegrationConflictOperationException;
import software.amazon.awssdk.services.glue.model.IntegrationNotFoundException;
import software.amazon.awssdk.services.glue.model.IntegrationQuotaExceededException;
import software.amazon.awssdk.services.glue.model.InternalServerException;
import software.amazon.awssdk.services.glue.model.InternalServiceException;
import software.amazon.awssdk.services.glue.model.InvalidInputException;
import software.amazon.awssdk.services.glue.model.InvalidIntegrationStateException;
import software.amazon.awssdk.services.glue.model.InvalidStateException;
import software.amazon.awssdk.services.glue.model.KmsKeyNotAccessibleException;
import software.amazon.awssdk.services.glue.model.ListBlueprintsRequest;
import software.amazon.awssdk.services.glue.model.ListBlueprintsResponse;
import software.amazon.awssdk.services.glue.model.ListColumnStatisticsTaskRunsRequest;
import software.amazon.awssdk.services.glue.model.ListColumnStatisticsTaskRunsResponse;
import software.amazon.awssdk.services.glue.model.ListConnectionTypesRequest;
import software.amazon.awssdk.services.glue.model.ListConnectionTypesResponse;
import software.amazon.awssdk.services.glue.model.ListCrawlersRequest;
import software.amazon.awssdk.services.glue.model.ListCrawlersResponse;
import software.amazon.awssdk.services.glue.model.ListCrawlsRequest;
import software.amazon.awssdk.services.glue.model.ListCrawlsResponse;
import software.amazon.awssdk.services.glue.model.ListCustomEntityTypesRequest;
import software.amazon.awssdk.services.glue.model.ListCustomEntityTypesResponse;
import software.amazon.awssdk.services.glue.model.ListDataQualityResultsRequest;
import software.amazon.awssdk.services.glue.model.ListDataQualityResultsResponse;
import software.amazon.awssdk.services.glue.model.ListDataQualityRuleRecommendationRunsRequest;
import software.amazon.awssdk.services.glue.model.ListDataQualityRuleRecommendationRunsResponse;
import software.amazon.awssdk.services.glue.model.ListDataQualityRulesetEvaluationRunsRequest;
import software.amazon.awssdk.services.glue.model.ListDataQualityRulesetEvaluationRunsResponse;
import software.amazon.awssdk.services.glue.model.ListDataQualityRulesetsRequest;
import software.amazon.awssdk.services.glue.model.ListDataQualityRulesetsResponse;
import software.amazon.awssdk.services.glue.model.ListDataQualityStatisticAnnotationsRequest;
import software.amazon.awssdk.services.glue.model.ListDataQualityStatisticAnnotationsResponse;
import software.amazon.awssdk.services.glue.model.ListDataQualityStatisticsRequest;
import software.amazon.awssdk.services.glue.model.ListDataQualityStatisticsResponse;
import software.amazon.awssdk.services.glue.model.ListDevEndpointsRequest;
import software.amazon.awssdk.services.glue.model.ListDevEndpointsResponse;
import software.amazon.awssdk.services.glue.model.ListEntitiesRequest;
import software.amazon.awssdk.services.glue.model.ListEntitiesResponse;
import software.amazon.awssdk.services.glue.model.ListJobsRequest;
import software.amazon.awssdk.services.glue.model.ListJobsResponse;
import software.amazon.awssdk.services.glue.model.ListMlTransformsRequest;
import software.amazon.awssdk.services.glue.model.ListMlTransformsResponse;
import software.amazon.awssdk.services.glue.model.ListRegistriesRequest;
import software.amazon.awssdk.services.glue.model.ListRegistriesResponse;
import software.amazon.awssdk.services.glue.model.ListSchemaVersionsRequest;
import software.amazon.awssdk.services.glue.model.ListSchemaVersionsResponse;
import software.amazon.awssdk.services.glue.model.ListSchemasRequest;
import software.amazon.awssdk.services.glue.model.ListSchemasResponse;
import software.amazon.awssdk.services.glue.model.ListSessionsRequest;
import software.amazon.awssdk.services.glue.model.ListSessionsResponse;
import software.amazon.awssdk.services.glue.model.ListStatementsRequest;
import software.amazon.awssdk.services.glue.model.ListStatementsResponse;
import software.amazon.awssdk.services.glue.model.ListTableOptimizerRunsRequest;
import software.amazon.awssdk.services.glue.model.ListTableOptimizerRunsResponse;
import software.amazon.awssdk.services.glue.model.ListTriggersRequest;
import software.amazon.awssdk.services.glue.model.ListTriggersResponse;
import software.amazon.awssdk.services.glue.model.ListUsageProfilesRequest;
import software.amazon.awssdk.services.glue.model.ListUsageProfilesResponse;
import software.amazon.awssdk.services.glue.model.ListWorkflowsRequest;
import software.amazon.awssdk.services.glue.model.ListWorkflowsResponse;
import software.amazon.awssdk.services.glue.model.MlTransformNotReadyException;
import software.amazon.awssdk.services.glue.model.ModifyIntegrationRequest;
import software.amazon.awssdk.services.glue.model.ModifyIntegrationResponse;
import software.amazon.awssdk.services.glue.model.NoScheduleException;
import software.amazon.awssdk.services.glue.model.OperationNotSupportedException;
import software.amazon.awssdk.services.glue.model.OperationTimeoutException;
import software.amazon.awssdk.services.glue.model.PermissionTypeMismatchException;
import software.amazon.awssdk.services.glue.model.PutDataCatalogEncryptionSettingsRequest;
import software.amazon.awssdk.services.glue.model.PutDataCatalogEncryptionSettingsResponse;
import software.amazon.awssdk.services.glue.model.PutDataQualityProfileAnnotationRequest;
import software.amazon.awssdk.services.glue.model.PutDataQualityProfileAnnotationResponse;
import software.amazon.awssdk.services.glue.model.PutResourcePolicyRequest;
import software.amazon.awssdk.services.glue.model.PutResourcePolicyResponse;
import software.amazon.awssdk.services.glue.model.PutSchemaVersionMetadataRequest;
import software.amazon.awssdk.services.glue.model.PutSchemaVersionMetadataResponse;
import software.amazon.awssdk.services.glue.model.PutWorkflowRunPropertiesRequest;
import software.amazon.awssdk.services.glue.model.PutWorkflowRunPropertiesResponse;
import software.amazon.awssdk.services.glue.model.QuerySchemaVersionMetadataRequest;
import software.amazon.awssdk.services.glue.model.QuerySchemaVersionMetadataResponse;
import software.amazon.awssdk.services.glue.model.RegisterSchemaVersionRequest;
import software.amazon.awssdk.services.glue.model.RegisterSchemaVersionResponse;
import software.amazon.awssdk.services.glue.model.RemoveSchemaVersionMetadataRequest;
import software.amazon.awssdk.services.glue.model.RemoveSchemaVersionMetadataResponse;
import software.amazon.awssdk.services.glue.model.ResetJobBookmarkRequest;
import software.amazon.awssdk.services.glue.model.ResetJobBookmarkResponse;
import software.amazon.awssdk.services.glue.model.ResourceNotFoundException;
import software.amazon.awssdk.services.glue.model.ResourceNotReadyException;
import software.amazon.awssdk.services.glue.model.ResourceNumberLimitExceededException;
import software.amazon.awssdk.services.glue.model.ResumeWorkflowRunRequest;
import software.amazon.awssdk.services.glue.model.ResumeWorkflowRunResponse;
import software.amazon.awssdk.services.glue.model.RunStatementRequest;
import software.amazon.awssdk.services.glue.model.RunStatementResponse;
import software.amazon.awssdk.services.glue.model.SchedulerNotRunningException;
import software.amazon.awssdk.services.glue.model.SchedulerRunningException;
import software.amazon.awssdk.services.glue.model.SchedulerTransitioningException;
import software.amazon.awssdk.services.glue.model.SearchTablesRequest;
import software.amazon.awssdk.services.glue.model.SearchTablesResponse;
import software.amazon.awssdk.services.glue.model.StartBlueprintRunRequest;
import software.amazon.awssdk.services.glue.model.StartBlueprintRunResponse;
import software.amazon.awssdk.services.glue.model.StartColumnStatisticsTaskRunRequest;
import software.amazon.awssdk.services.glue.model.StartColumnStatisticsTaskRunResponse;
import software.amazon.awssdk.services.glue.model.StartColumnStatisticsTaskRunScheduleRequest;
import software.amazon.awssdk.services.glue.model.StartColumnStatisticsTaskRunScheduleResponse;
import software.amazon.awssdk.services.glue.model.StartCrawlerRequest;
import software.amazon.awssdk.services.glue.model.StartCrawlerResponse;
import software.amazon.awssdk.services.glue.model.StartCrawlerScheduleRequest;
import software.amazon.awssdk.services.glue.model.StartCrawlerScheduleResponse;
import software.amazon.awssdk.services.glue.model.StartDataQualityRuleRecommendationRunRequest;
import software.amazon.awssdk.services.glue.model.StartDataQualityRuleRecommendationRunResponse;
import software.amazon.awssdk.services.glue.model.StartDataQualityRulesetEvaluationRunRequest;
import software.amazon.awssdk.services.glue.model.StartDataQualityRulesetEvaluationRunResponse;
import software.amazon.awssdk.services.glue.model.StartExportLabelsTaskRunRequest;
import software.amazon.awssdk.services.glue.model.StartExportLabelsTaskRunResponse;
import software.amazon.awssdk.services.glue.model.StartImportLabelsTaskRunRequest;
import software.amazon.awssdk.services.glue.model.StartImportLabelsTaskRunResponse;
import software.amazon.awssdk.services.glue.model.StartJobRunRequest;
import software.amazon.awssdk.services.glue.model.StartJobRunResponse;
import software.amazon.awssdk.services.glue.model.StartMlEvaluationTaskRunRequest;
import software.amazon.awssdk.services.glue.model.StartMlEvaluationTaskRunResponse;
import software.amazon.awssdk.services.glue.model.StartMlLabelingSetGenerationTaskRunRequest;
import software.amazon.awssdk.services.glue.model.StartMlLabelingSetGenerationTaskRunResponse;
import software.amazon.awssdk.services.glue.model.StartTriggerRequest;
import software.amazon.awssdk.services.glue.model.StartTriggerResponse;
import software.amazon.awssdk.services.glue.model.StartWorkflowRunRequest;
import software.amazon.awssdk.services.glue.model.StartWorkflowRunResponse;
import software.amazon.awssdk.services.glue.model.StopColumnStatisticsTaskRunRequest;
import software.amazon.awssdk.services.glue.model.StopColumnStatisticsTaskRunResponse;
import software.amazon.awssdk.services.glue.model.StopColumnStatisticsTaskRunScheduleRequest;
import software.amazon.awssdk.services.glue.model.StopColumnStatisticsTaskRunScheduleResponse;
import software.amazon.awssdk.services.glue.model.StopCrawlerRequest;
import software.amazon.awssdk.services.glue.model.StopCrawlerResponse;
import software.amazon.awssdk.services.glue.model.StopCrawlerScheduleRequest;
import software.amazon.awssdk.services.glue.model.StopCrawlerScheduleResponse;
import software.amazon.awssdk.services.glue.model.StopSessionRequest;
import software.amazon.awssdk.services.glue.model.StopSessionResponse;
import software.amazon.awssdk.services.glue.model.StopTriggerRequest;
import software.amazon.awssdk.services.glue.model.StopTriggerResponse;
import software.amazon.awssdk.services.glue.model.StopWorkflowRunRequest;
import software.amazon.awssdk.services.glue.model.StopWorkflowRunResponse;
import software.amazon.awssdk.services.glue.model.TagResourceRequest;
import software.amazon.awssdk.services.glue.model.TagResourceResponse;
import software.amazon.awssdk.services.glue.model.TargetResourceNotFoundException;
import software.amazon.awssdk.services.glue.model.TestConnectionRequest;
import software.amazon.awssdk.services.glue.model.TestConnectionResponse;
import software.amazon.awssdk.services.glue.model.ThrottlingException;
import software.amazon.awssdk.services.glue.model.UntagResourceRequest;
import software.amazon.awssdk.services.glue.model.UntagResourceResponse;
import software.amazon.awssdk.services.glue.model.UpdateBlueprintRequest;
import software.amazon.awssdk.services.glue.model.UpdateBlueprintResponse;
import software.amazon.awssdk.services.glue.model.UpdateCatalogRequest;
import software.amazon.awssdk.services.glue.model.UpdateCatalogResponse;
import software.amazon.awssdk.services.glue.model.UpdateClassifierRequest;
import software.amazon.awssdk.services.glue.model.UpdateClassifierResponse;
import software.amazon.awssdk.services.glue.model.UpdateColumnStatisticsForPartitionRequest;
import software.amazon.awssdk.services.glue.model.UpdateColumnStatisticsForPartitionResponse;
import software.amazon.awssdk.services.glue.model.UpdateColumnStatisticsForTableRequest;
import software.amazon.awssdk.services.glue.model.UpdateColumnStatisticsForTableResponse;
import software.amazon.awssdk.services.glue.model.UpdateColumnStatisticsTaskSettingsRequest;
import software.amazon.awssdk.services.glue.model.UpdateColumnStatisticsTaskSettingsResponse;
import software.amazon.awssdk.services.glue.model.UpdateConnectionRequest;
import software.amazon.awssdk.services.glue.model.UpdateConnectionResponse;
import software.amazon.awssdk.services.glue.model.UpdateCrawlerRequest;
import software.amazon.awssdk.services.glue.model.UpdateCrawlerResponse;
import software.amazon.awssdk.services.glue.model.UpdateCrawlerScheduleRequest;
import software.amazon.awssdk.services.glue.model.UpdateCrawlerScheduleResponse;
import software.amazon.awssdk.services.glue.model.UpdateDataQualityRulesetRequest;
import software.amazon.awssdk.services.glue.model.UpdateDataQualityRulesetResponse;
import software.amazon.awssdk.services.glue.model.UpdateDatabaseRequest;
import software.amazon.awssdk.services.glue.model.UpdateDatabaseResponse;
import software.amazon.awssdk.services.glue.model.UpdateDevEndpointRequest;
import software.amazon.awssdk.services.glue.model.UpdateDevEndpointResponse;
import software.amazon.awssdk.services.glue.model.UpdateIntegrationResourcePropertyRequest;
import software.amazon.awssdk.services.glue.model.UpdateIntegrationResourcePropertyResponse;
import software.amazon.awssdk.services.glue.model.UpdateIntegrationTablePropertiesRequest;
import software.amazon.awssdk.services.glue.model.UpdateIntegrationTablePropertiesResponse;
import software.amazon.awssdk.services.glue.model.UpdateJobFromSourceControlRequest;
import software.amazon.awssdk.services.glue.model.UpdateJobFromSourceControlResponse;
import software.amazon.awssdk.services.glue.model.UpdateJobRequest;
import software.amazon.awssdk.services.glue.model.UpdateJobResponse;
import software.amazon.awssdk.services.glue.model.UpdateMlTransformRequest;
import software.amazon.awssdk.services.glue.model.UpdateMlTransformResponse;
import software.amazon.awssdk.services.glue.model.UpdatePartitionRequest;
import software.amazon.awssdk.services.glue.model.UpdatePartitionResponse;
import software.amazon.awssdk.services.glue.model.UpdateRegistryRequest;
import software.amazon.awssdk.services.glue.model.UpdateRegistryResponse;
import software.amazon.awssdk.services.glue.model.UpdateSchemaRequest;
import software.amazon.awssdk.services.glue.model.UpdateSchemaResponse;
import software.amazon.awssdk.services.glue.model.UpdateSourceControlFromJobRequest;
import software.amazon.awssdk.services.glue.model.UpdateSourceControlFromJobResponse;
import software.amazon.awssdk.services.glue.model.UpdateTableOptimizerRequest;
import software.amazon.awssdk.services.glue.model.UpdateTableOptimizerResponse;
import software.amazon.awssdk.services.glue.model.UpdateTableRequest;
import software.amazon.awssdk.services.glue.model.UpdateTableResponse;
import software.amazon.awssdk.services.glue.model.UpdateTriggerRequest;
import software.amazon.awssdk.services.glue.model.UpdateTriggerResponse;
import software.amazon.awssdk.services.glue.model.UpdateUsageProfileRequest;
import software.amazon.awssdk.services.glue.model.UpdateUsageProfileResponse;
import software.amazon.awssdk.services.glue.model.UpdateUserDefinedFunctionRequest;
import software.amazon.awssdk.services.glue.model.UpdateUserDefinedFunctionResponse;
import software.amazon.awssdk.services.glue.model.UpdateWorkflowRequest;
import software.amazon.awssdk.services.glue.model.UpdateWorkflowResponse;
import software.amazon.awssdk.services.glue.model.ValidationException;
import software.amazon.awssdk.services.glue.model.VersionMismatchException;
import software.amazon.awssdk.services.glue.transform.BatchCreatePartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchDeleteConnectionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchDeletePartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchDeleteTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchDeleteTableVersionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetBlueprintsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetCrawlersRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetCustomEntityTypesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetDataQualityResultRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetDevEndpointsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetJobsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetPartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetTableOptimizerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetTriggersRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchGetWorkflowsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchPutDataQualityStatisticAnnotationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchStopJobRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.BatchUpdatePartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CancelDataQualityRuleRecommendationRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CancelDataQualityRulesetEvaluationRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CancelMlTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CancelStatementRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CheckSchemaVersionValidityRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateBlueprintRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateCatalogRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateClassifierRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateColumnStatisticsTaskSettingsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateConnectionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateCrawlerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateCustomEntityTypeRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateDataQualityRulesetRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateDatabaseRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateDevEndpointRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateIntegrationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateIntegrationResourcePropertyRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateIntegrationTablePropertiesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateJobRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateMlTransformRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreatePartitionIndexRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreatePartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateRegistryRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateSchemaRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateScriptRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateSessionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateTableOptimizerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateTriggerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateUsageProfileRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateUserDefinedFunctionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.CreateWorkflowRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteBlueprintRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteCatalogRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteClassifierRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteColumnStatisticsForPartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteColumnStatisticsForTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteColumnStatisticsTaskSettingsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteConnectionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteCrawlerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteCustomEntityTypeRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteDataQualityRulesetRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteDatabaseRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteDevEndpointRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteIntegrationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteIntegrationTablePropertiesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteJobRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteMlTransformRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeletePartitionIndexRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeletePartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteRegistryRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteSchemaRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteSchemaVersionsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteSessionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteTableOptimizerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteTableVersionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteTriggerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteUsageProfileRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteUserDefinedFunctionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DeleteWorkflowRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DescribeConnectionTypeRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DescribeEntityRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DescribeInboundIntegrationsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.DescribeIntegrationsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetBlueprintRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetBlueprintRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetBlueprintRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetCatalogImportStatusRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetCatalogRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetCatalogsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetClassifierRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetClassifiersRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetColumnStatisticsForPartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetColumnStatisticsForTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetColumnStatisticsTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetColumnStatisticsTaskRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetColumnStatisticsTaskSettingsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetConnectionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetConnectionsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetCrawlerMetricsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetCrawlerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetCrawlersRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetCustomEntityTypeRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataCatalogEncryptionSettingsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataQualityModelRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataQualityModelResultRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataQualityResultRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataQualityRuleRecommendationRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataQualityRulesetEvaluationRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataQualityRulesetRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDatabaseRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDatabasesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDataflowGraphRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDevEndpointRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetDevEndpointsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetEntityRecordsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetIntegrationResourcePropertyRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetIntegrationTablePropertiesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetJobBookmarkRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetJobRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetJobRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetJobRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetJobsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetMappingRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetMlTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetMlTaskRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetMlTransformRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetMlTransformsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetPartitionIndexesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetPartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetPartitionsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetPlanRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetRegistryRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetResourcePoliciesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetSchemaByDefinitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetSchemaRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetSchemaVersionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetSchemaVersionsDiffRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetSecurityConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetSessionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetStatementRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTableOptimizerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTableVersionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTableVersionsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTablesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTagsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTriggerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetTriggersRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetUnfilteredPartitionMetadataRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetUnfilteredPartitionsMetadataRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetUnfilteredTableMetadataRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetUsageProfileRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetUserDefinedFunctionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetUserDefinedFunctionsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetWorkflowRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetWorkflowRunPropertiesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetWorkflowRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.GetWorkflowRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ImportCatalogToGlueRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListBlueprintsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListColumnStatisticsTaskRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListConnectionTypesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListCrawlersRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListCrawlsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListCustomEntityTypesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListDataQualityResultsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListDataQualityRuleRecommendationRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListDataQualityRulesetEvaluationRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListDataQualityRulesetsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListDataQualityStatisticAnnotationsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListDataQualityStatisticsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListDevEndpointsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListEntitiesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListJobsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListMlTransformsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListRegistriesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListSchemaVersionsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListSchemasRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListSessionsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListStatementsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListTableOptimizerRunsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListTriggersRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListUsageProfilesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ListWorkflowsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ModifyIntegrationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.PutDataCatalogEncryptionSettingsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.PutDataQualityProfileAnnotationRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.PutResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.PutSchemaVersionMetadataRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.PutWorkflowRunPropertiesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.QuerySchemaVersionMetadataRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.RegisterSchemaVersionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.RemoveSchemaVersionMetadataRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ResetJobBookmarkRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.ResumeWorkflowRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.RunStatementRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.SearchTablesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartBlueprintRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartColumnStatisticsTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartColumnStatisticsTaskRunScheduleRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartCrawlerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartCrawlerScheduleRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartDataQualityRuleRecommendationRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartDataQualityRulesetEvaluationRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartExportLabelsTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartImportLabelsTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartJobRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartMlEvaluationTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartMlLabelingSetGenerationTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartTriggerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StartWorkflowRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StopColumnStatisticsTaskRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StopColumnStatisticsTaskRunScheduleRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StopCrawlerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StopCrawlerScheduleRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StopSessionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StopTriggerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.StopWorkflowRunRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.TestConnectionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateBlueprintRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateCatalogRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateClassifierRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateColumnStatisticsForPartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateColumnStatisticsForTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateColumnStatisticsTaskSettingsRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateConnectionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateCrawlerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateCrawlerScheduleRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateDataQualityRulesetRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateDatabaseRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateDevEndpointRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateIntegrationResourcePropertyRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateIntegrationTablePropertiesRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateJobFromSourceControlRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateJobRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateMlTransformRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdatePartitionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateRegistryRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateSchemaRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateSourceControlFromJobRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateTableOptimizerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateTableRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateTriggerRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateUsageProfileRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateUserDefinedFunctionRequestMarshaller;
import software.amazon.awssdk.services.glue.transform.UpdateWorkflowRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link GlueAsyncClient}.
*
* @see GlueAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultGlueAsyncClient implements GlueAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultGlueAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultGlueAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Creates one or more partitions in a batch operation.
*
*
* @param batchCreatePartitionRequest
* @return A Java Future containing the result of the BatchCreatePartition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - AlreadyExistsException A resource to be created or added already exists.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - InternalServiceException An internal service error occurred.
* - EntityNotFoundException A specified entity does not exist
* - OperationTimeoutException The operation timed out.
* - GlueEncryptionException An encryption operation failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchCreatePartition
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchCreatePartition(
BatchCreatePartitionRequest batchCreatePartitionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchCreatePartitionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchCreatePartitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchCreatePartition");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchCreatePartitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchCreatePartition").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchCreatePartitionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchCreatePartitionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a list of connection definitions from the Data Catalog.
*
*
* @param batchDeleteConnectionRequest
* @return A Java Future containing the result of the BatchDeleteConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchDeleteConnection
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchDeleteConnection(
BatchDeleteConnectionRequest batchDeleteConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeleteConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDeleteConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDeleteConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes one or more partitions in a batch operation.
*
*
* @param batchDeletePartitionRequest
* @return A Java Future containing the result of the BatchDeletePartition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - EntityNotFoundException A specified entity does not exist
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchDeletePartition
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchDeletePartition(
BatchDeletePartitionRequest batchDeletePartitionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeletePartitionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeletePartitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeletePartition");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeletePartitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeletePartition").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDeletePartitionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDeletePartitionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes multiple tables at once.
*
*
*
* After completing this operation, you no longer have access to the table versions and partitions that belong to
* the deleted table. Glue deletes these "orphaned" resources asynchronously in a timely manner, at the discretion
* of the service.
*
*
* To ensure the immediate deletion of all related resources, before calling BatchDeleteTable
, use
* DeleteTableVersion
or BatchDeleteTableVersion
, and DeletePartition
or
* BatchDeletePartition
, to delete any resources that belong to the table.
*
*
*
* @param batchDeleteTableRequest
* @return A Java Future containing the result of the BatchDeleteTable operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - EntityNotFoundException A specified entity does not exist
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - GlueEncryptionException An encryption operation failed.
* - ResourceNotReadyException A resource was not ready for a transaction.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchDeleteTable
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchDeleteTable(BatchDeleteTableRequest batchDeleteTableRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeleteTableRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteTableRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteTable");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteTableResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteTable").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDeleteTableRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDeleteTableRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified batch of versions of a table.
*
*
* @param batchDeleteTableVersionRequest
* @return A Java Future containing the result of the BatchDeleteTableVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityNotFoundException A specified entity does not exist
* - InvalidInputException The input provided was not valid.
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchDeleteTableVersion
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchDeleteTableVersion(
BatchDeleteTableVersionRequest batchDeleteTableVersionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeleteTableVersionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteTableVersionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteTableVersion");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteTableVersionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteTableVersion").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDeleteTableVersionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDeleteTableVersionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about a list of blueprints.
*
*
* @param batchGetBlueprintsRequest
* @return A Java Future containing the result of the BatchGetBlueprints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetBlueprints
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetBlueprints(BatchGetBlueprintsRequest batchGetBlueprintsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetBlueprintsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetBlueprintsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetBlueprints");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetBlueprintsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetBlueprints").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetBlueprintsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetBlueprintsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of resource metadata for a given list of crawler names. After calling the
* ListCrawlers
operation, you can call this operation to access the data to which you have been
* granted permissions. This operation supports all IAM permissions, including permission conditions that uses tags.
*
*
* @param batchGetCrawlersRequest
* @return A Java Future containing the result of the BatchGetCrawlers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetCrawlers
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetCrawlers(BatchGetCrawlersRequest batchGetCrawlersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetCrawlersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetCrawlersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetCrawlers");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetCrawlersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetCrawlers").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetCrawlersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetCrawlersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the details for the custom patterns specified by a list of names.
*
*
* @param batchGetCustomEntityTypesRequest
* @return A Java Future containing the result of the BatchGetCustomEntityTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetCustomEntityTypes
* @see AWS API Documentation
*/
@Override
public CompletableFuture batchGetCustomEntityTypes(
BatchGetCustomEntityTypesRequest batchGetCustomEntityTypesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetCustomEntityTypesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetCustomEntityTypesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetCustomEntityTypes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetCustomEntityTypesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetCustomEntityTypes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetCustomEntityTypesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetCustomEntityTypesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a list of data quality results for the specified result IDs.
*
*
* @param batchGetDataQualityResultRequest
* @return A Java Future containing the result of the BatchGetDataQualityResult operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetDataQualityResult
* @see AWS API Documentation
*/
@Override
public CompletableFuture batchGetDataQualityResult(
BatchGetDataQualityResultRequest batchGetDataQualityResultRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetDataQualityResultRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetDataQualityResultRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetDataQualityResult");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetDataQualityResultResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetDataQualityResult").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetDataQualityResultRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetDataQualityResultRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of resource metadata for a given list of development endpoint names. After calling the
* ListDevEndpoints
operation, you can call this operation to access the data to which you have been
* granted permissions. This operation supports all IAM permissions, including permission conditions that uses tags.
*
*
* @param batchGetDevEndpointsRequest
* @return A Java Future containing the result of the BatchGetDevEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException Access to a resource was denied.
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetDevEndpoints
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetDevEndpoints(
BatchGetDevEndpointsRequest batchGetDevEndpointsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetDevEndpointsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetDevEndpointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetDevEndpoints");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetDevEndpointsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetDevEndpoints").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetDevEndpointsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetDevEndpointsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of resource metadata for a given list of job names. After calling the ListJobs
* operation, you can call this operation to access the data to which you have been granted permissions. This
* operation supports all IAM permissions, including permission conditions that uses tags.
*
*
* @param batchGetJobsRequest
* @return A Java Future containing the result of the BatchGetJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetJobs
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetJobs(BatchGetJobsRequest batchGetJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetJobsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
BatchGetJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves partitions in a batch request.
*
*
* @param batchGetPartitionRequest
* @return A Java Future containing the result of the BatchGetPartition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - EntityNotFoundException A specified entity does not exist
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - GlueEncryptionException An encryption operation failed.
* - InvalidStateException An error that indicates your data is in an invalid state.
* - FederationSourceException A federation source failed.
* - FederationSourceRetryableException A federation source failed, but the operation may be retried.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetPartition
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetPartition(BatchGetPartitionRequest batchGetPartitionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetPartitionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetPartitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetPartition");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetPartitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetPartition").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetPartitionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetPartitionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the configuration for the specified table optimizers.
*
*
* @param batchGetTableOptimizerRequest
* @return A Java Future containing the result of the BatchGetTableOptimizer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityNotFoundException A specified entity does not exist
* - InvalidInputException The input provided was not valid.
* - AccessDeniedException Access to a resource was denied.
* - InternalServiceException An internal service error occurred.
* - ThrottlingException The throttling threshhold was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetTableOptimizer
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchGetTableOptimizer(
BatchGetTableOptimizerRequest batchGetTableOptimizerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetTableOptimizerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetTableOptimizerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetTableOptimizer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetTableOptimizerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetTableOptimizer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetTableOptimizerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetTableOptimizerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of resource metadata for a given list of trigger names. After calling the
* ListTriggers
operation, you can call this operation to access the data to which you have been
* granted permissions. This operation supports all IAM permissions, including permission conditions that uses tags.
*
*
* @param batchGetTriggersRequest
* @return A Java Future containing the result of the BatchGetTriggers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetTriggers
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetTriggers(BatchGetTriggersRequest batchGetTriggersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetTriggersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetTriggersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetTriggers");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetTriggersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetTriggers").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetTriggersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetTriggersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of resource metadata for a given list of workflow names. After calling the
* ListWorkflows
operation, you can call this operation to access the data to which you have been
* granted permissions. This operation supports all IAM permissions, including permission conditions that uses tags.
*
*
* @param batchGetWorkflowsRequest
* @return A Java Future containing the result of the BatchGetWorkflows operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchGetWorkflows
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetWorkflows(BatchGetWorkflowsRequest batchGetWorkflowsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetWorkflowsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetWorkflowsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetWorkflows");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetWorkflowsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetWorkflows").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchGetWorkflowsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchGetWorkflowsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Annotate datapoints over time for a specific data quality statistic.
*
*
* @param batchPutDataQualityStatisticAnnotationRequest
* @return A Java Future containing the result of the BatchPutDataQualityStatisticAnnotation operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityNotFoundException A specified entity does not exist
* - InvalidInputException The input provided was not valid.
* - InternalServiceException An internal service error occurred.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchPutDataQualityStatisticAnnotation
* @see AWS API Documentation
*/
@Override
public CompletableFuture batchPutDataQualityStatisticAnnotation(
BatchPutDataQualityStatisticAnnotationRequest batchPutDataQualityStatisticAnnotationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchPutDataQualityStatisticAnnotationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
batchPutDataQualityStatisticAnnotationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchPutDataQualityStatisticAnnotation");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, BatchPutDataQualityStatisticAnnotationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchPutDataQualityStatisticAnnotation").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchPutDataQualityStatisticAnnotationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchPutDataQualityStatisticAnnotationRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Stops one or more job runs for a specified job definition.
*
*
* @param batchStopJobRunRequest
* @return A Java Future containing the result of the BatchStopJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchStopJobRun
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchStopJobRun(BatchStopJobRunRequest batchStopJobRunRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchStopJobRunRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchStopJobRunRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchStopJobRun");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchStopJobRunResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchStopJobRun").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchStopJobRunRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchStopJobRunRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates one or more partitions in a batch operation.
*
*
* @param batchUpdatePartitionRequest
* @return A Java Future containing the result of the BatchUpdatePartition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - EntityNotFoundException A specified entity does not exist
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - GlueEncryptionException An encryption operation failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.BatchUpdatePartition
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchUpdatePartition(
BatchUpdatePartitionRequest batchUpdatePartitionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchUpdatePartitionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchUpdatePartitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchUpdatePartition");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchUpdatePartitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchUpdatePartition").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchUpdatePartitionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchUpdatePartitionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels the specified recommendation run that was being used to generate rules.
*
*
* @param cancelDataQualityRuleRecommendationRunRequest
* @return A Java Future containing the result of the CancelDataQualityRuleRecommendationRun operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityNotFoundException A specified entity does not exist
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CancelDataQualityRuleRecommendationRun
* @see AWS API Documentation
*/
@Override
public CompletableFuture cancelDataQualityRuleRecommendationRun(
CancelDataQualityRuleRecommendationRunRequest cancelDataQualityRuleRecommendationRunRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelDataQualityRuleRecommendationRunRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
cancelDataQualityRuleRecommendationRunRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelDataQualityRuleRecommendationRun");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, CancelDataQualityRuleRecommendationRunResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelDataQualityRuleRecommendationRun").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelDataQualityRuleRecommendationRunRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelDataQualityRuleRecommendationRunRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a run where a ruleset is being evaluated against a data source.
*
*
* @param cancelDataQualityRulesetEvaluationRunRequest
* @return A Java Future containing the result of the CancelDataQualityRulesetEvaluationRun operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityNotFoundException A specified entity does not exist
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CancelDataQualityRulesetEvaluationRun
* @see AWS API Documentation
*/
@Override
public CompletableFuture cancelDataQualityRulesetEvaluationRun(
CancelDataQualityRulesetEvaluationRunRequest cancelDataQualityRulesetEvaluationRunRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelDataQualityRulesetEvaluationRunRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
cancelDataQualityRulesetEvaluationRunRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelDataQualityRulesetEvaluationRun");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, CancelDataQualityRulesetEvaluationRunResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelDataQualityRulesetEvaluationRun").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelDataQualityRulesetEvaluationRunRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelDataQualityRulesetEvaluationRunRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels (stops) a task run. Machine learning task runs are asynchronous tasks that Glue runs on your behalf as
* part of various machine learning workflows. You can cancel a machine learning task run at any time by calling
* CancelMLTaskRun
with a task run's parent transform's TransformID
and the task run's
* TaskRunId
.
*
*
* @param cancelMlTaskRunRequest
* @return A Java Future containing the result of the CancelMLTaskRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityNotFoundException A specified entity does not exist
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CancelMLTaskRun
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cancelMLTaskRun(CancelMlTaskRunRequest cancelMlTaskRunRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelMlTaskRunRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelMlTaskRunRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelMLTaskRun");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelMlTaskRunResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelMLTaskRun").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelMlTaskRunRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelMlTaskRunRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels the statement.
*
*
* @param cancelStatementRequest
* @return A Java Future containing the result of the CancelStatement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException Access to a resource was denied.
* - EntityNotFoundException A specified entity does not exist
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - InvalidInputException The input provided was not valid.
* - IllegalSessionStateException The session is in an invalid state to perform a requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CancelStatement
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cancelStatement(CancelStatementRequest cancelStatementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelStatementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelStatementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelStatement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelStatementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelStatement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelStatementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelStatementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Validates the supplied schema. This call has no side effects, it simply validates using the supplied schema using
* DataFormat
as the format. Since it does not take a schema set name, no compatibility checks are
* performed.
*
*
* @param checkSchemaVersionValidityRequest
* @return A Java Future containing the result of the CheckSchemaVersionValidity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - AccessDeniedException Access to a resource was denied.
* - InternalServiceException An internal service error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CheckSchemaVersionValidity
* @see AWS API Documentation
*/
@Override
public CompletableFuture checkSchemaVersionValidity(
CheckSchemaVersionValidityRequest checkSchemaVersionValidityRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(checkSchemaVersionValidityRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, checkSchemaVersionValidityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CheckSchemaVersionValidity");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CheckSchemaVersionValidityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CheckSchemaVersionValidity").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CheckSchemaVersionValidityRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(checkSchemaVersionValidityRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Registers a blueprint with Glue.
*
*
* @param createBlueprintRequest
* @return A Java Future containing the result of the CreateBlueprint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException A resource to be created or added already exists.
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateBlueprint
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createBlueprint(CreateBlueprintRequest createBlueprintRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createBlueprintRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createBlueprintRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateBlueprint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateBlueprintResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateBlueprint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateBlueprintRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createBlueprintRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new catalog in the Glue Data Catalog.
*
*
* @param createCatalogRequest
* @return A Java Future containing the result of the CreateCatalog operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - AlreadyExistsException A resource to be created or added already exists.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - GlueEncryptionException An encryption operation failed.
* - ConcurrentModificationException Two processes are trying to modify a resource simultaneously.
* - AccessDeniedException Access to a resource was denied.
* - EntityNotFoundException A specified entity does not exist
* - FederatedResourceAlreadyExistsException A federated resource already exists.
* - FederationSourceException A federation source failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateCatalog
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createCatalog(CreateCatalogRequest createCatalogRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCatalogRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCatalog");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCatalog").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateCatalogRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createCatalogRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a classifier in the user's account. This can be a GrokClassifier
, an
* XMLClassifier
, a JsonClassifier
, or a CsvClassifier
, depending on which
* field of the request is present.
*
*
* @param createClassifierRequest
* @return A Java Future containing the result of the CreateClassifier operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException A resource to be created or added already exists.
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateClassifier
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createClassifier(CreateClassifierRequest createClassifierRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createClassifierRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClassifierRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateClassifier");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateClassifierResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateClassifier").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateClassifierRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createClassifierRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates settings for a column statistics task.
*
*
* @param createColumnStatisticsTaskSettingsRequest
* @return A Java Future containing the result of the CreateColumnStatisticsTaskSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException A resource to be created or added already exists.
* - AccessDeniedException Access to a resource was denied.
* - EntityNotFoundException A specified entity does not exist
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - ColumnStatisticsTaskRunningException An exception thrown when you try to start another job while
* running a column stats generation job.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateColumnStatisticsTaskSettings
* @see AWS API Documentation
*/
@Override
public CompletableFuture createColumnStatisticsTaskSettings(
CreateColumnStatisticsTaskSettingsRequest createColumnStatisticsTaskSettingsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createColumnStatisticsTaskSettingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createColumnStatisticsTaskSettingsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateColumnStatisticsTaskSettings");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, CreateColumnStatisticsTaskSettingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateColumnStatisticsTaskSettings").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateColumnStatisticsTaskSettingsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createColumnStatisticsTaskSettingsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a connection definition in the Data Catalog.
*
*
* Connections used for creating federated resources require the IAM glue:PassConnection
permission.
*
*
* @param createConnectionRequest
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException A resource to be created or added already exists.
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - GlueEncryptionException An encryption operation failed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateConnection
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createConnection(CreateConnectionRequest createConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new crawler with specified targets, role, configuration, and optional schedule. At least one crawl
* target must be specified, in the s3Targets
field, the jdbcTargets
field, or the
* DynamoDBTargets
field.
*
*
* @param createCrawlerRequest
* @return A Java Future containing the result of the CreateCrawler operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - AlreadyExistsException A resource to be created or added already exists.
* - OperationTimeoutException The operation timed out.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateCrawler
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createCrawler(CreateCrawlerRequest createCrawlerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCrawlerRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCrawlerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCrawler");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateCrawlerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCrawler").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateCrawlerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createCrawlerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a custom pattern that is used to detect sensitive data across the columns and rows of your structured
* data.
*
*
* Each custom pattern you create specifies a regular expression and an optional list of context words. If no
* context words are passed only a regular expression is checked.
*
*
* @param createCustomEntityTypeRequest
* @return A Java Future containing the result of the CreateCustomEntityType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException Access to a resource was denied.
* - AlreadyExistsException A resource to be created or added already exists.
* - IdempotentParameterMismatchException The same unique identifier was associated with two different
* records.
* - InternalServiceException An internal service error occurred.
* - InvalidInputException The input provided was not valid.
* - OperationTimeoutException The operation timed out.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateCustomEntityType
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createCustomEntityType(
CreateCustomEntityTypeRequest createCustomEntityTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCustomEntityTypeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCustomEntityTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCustomEntityType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateCustomEntityTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCustomEntityType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateCustomEntityTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createCustomEntityTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a data quality ruleset with DQDL rules applied to a specified Glue table.
*
*
* You create the ruleset using the Data Quality Definition Language (DQDL). For more information, see the Glue
* developer guide.
*
*
* @param createDataQualityRulesetRequest
* @return A Java Future containing the result of the CreateDataQualityRuleset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - AlreadyExistsException A resource to be created or added already exists.
* - OperationTimeoutException The operation timed out.
* - InternalServiceException An internal service error occurred.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateDataQualityRuleset
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createDataQualityRuleset(
CreateDataQualityRulesetRequest createDataQualityRulesetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDataQualityRulesetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDataQualityRulesetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDataQualityRuleset");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDataQualityRulesetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDataQualityRuleset").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDataQualityRulesetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDataQualityRulesetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new database in a Data Catalog.
*
*
* @param createDatabaseRequest
* @return A Java Future containing the result of the CreateDatabase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The input provided was not valid.
* - AlreadyExistsException A resource to be created or added already exists.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - GlueEncryptionException An encryption operation failed.
* - ConcurrentModificationException Two processes are trying to modify a resource simultaneously.
* - FederatedResourceAlreadyExistsException A federated resource already exists.
* - FederationSourceException A federation source failed.
* - FederationSourceRetryableException A federation source failed, but the operation may be retried.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateDatabase
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createDatabase(CreateDatabaseRequest createDatabaseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDatabaseRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDatabaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDatabase");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDatabaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDatabase").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDatabaseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDatabaseRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new development endpoint.
*
*
* @param createDevEndpointRequest
* @return A Java Future containing the result of the CreateDevEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException Access to a resource was denied.
* - AlreadyExistsException A resource to be created or added already exists.
* - IdempotentParameterMismatchException The same unique identifier was associated with two different
* records.
* - InternalServiceException An internal service error occurred.
* - OperationTimeoutException The operation timed out.
* - InvalidInputException The input provided was not valid.
* - ValidationException A value could not be validated.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateDevEndpoint
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createDevEndpoint(CreateDevEndpointRequest createDevEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDevEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDevEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDevEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDevEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDevEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDevEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDevEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a Zero-ETL integration in the caller's account between two resources with Amazon Resource Names (ARNs):
* the SourceArn
and TargetArn
.
*
*
* @param createIntegrationRequest
* @return A Java Future containing the result of the CreateIntegration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A value could not be validated.
* - AccessDeniedException Access to a resource was denied.
* - ResourceNotFoundException The resource could not be found.
* - InternalServerException An internal server error occurred.
* - IntegrationConflictOperationException The requested operation conflicts with another operation.
* - IntegrationQuotaExceededException The data processed through your integration exceeded your quota.
* - KmsKeyNotAccessibleException The KMS key specified is not accessible.
* - EntityNotFoundException A specified entity does not exist
* - InternalServiceException An internal service error occurred.
* - ConflictException The
CreatePartitions
API was called on a table that has indexes
* enabled.
* - ResourceNumberLimitExceededException A resource numerical limit was exceeded.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateIntegration
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createIntegration(CreateIntegrationRequest createIntegrationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createIntegrationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createIntegrationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIntegration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateIntegrationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateIntegration").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateIntegrationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createIntegrationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API can be used for setting up the ResourceProperty
of the Glue connection (for the source) or
* Glue database ARN (for the target). These properties can include the role to access the connection or database.
* To set both source and target properties the same API needs to be invoked with the Glue connection ARN as
* ResourceArn
with SourceProcessingProperties
and the Glue database ARN as
* ResourceArn
with TargetProcessingProperties
respectively.
*
*
* @param createIntegrationResourcePropertyRequest
* @return A Java Future containing the result of the CreateIntegrationResourceProperty operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A value could not be validated.
* - AccessDeniedException Access to a resource was denied.
* - ConflictException The
CreatePartitions
API was called on a table that has indexes
* enabled.
* - InternalServerException An internal server error occurred.
* - ResourceNotFoundException The resource could not be found.
* - EntityNotFoundException A specified entity does not exist
* - InternalServiceException An internal service error occurred.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateIntegrationResourceProperty
* @see AWS API Documentation
*/
@Override
public CompletableFuture createIntegrationResourceProperty(
CreateIntegrationResourcePropertyRequest createIntegrationResourcePropertyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createIntegrationResourcePropertyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createIntegrationResourcePropertyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIntegrationResourceProperty");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, CreateIntegrationResourcePropertyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateIntegrationResourceProperty").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateIntegrationResourcePropertyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createIntegrationResourcePropertyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is used to provide optional override properties for the the tables that need to be replicated. These
* properties can include properties for filtering and partitioning for the source and target tables. To set both
* source and target properties the same API need to be invoked with the Glue connection ARN as
* ResourceArn
with SourceTableConfig
, and the Glue database ARN as
* ResourceArn
with TargetTableConfig
respectively.
*
*
* @param createIntegrationTablePropertiesRequest
* @return A Java Future containing the result of the CreateIntegrationTableProperties operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A value could not be validated.
* - AccessDeniedException Access to a resource was denied.
* - ResourceNotFoundException The resource could not be found.
* - InternalServerException An internal server error occurred.
* - EntityNotFoundException A specified entity does not exist
* - InternalServiceException An internal service error occurred.
* - InvalidInputException The input provided was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - GlueException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample GlueAsyncClient.CreateIntegrationTableProperties
* @see AWS API Documentation
*/
@Override
public CompletableFuture createIntegrationTableProperties(
CreateIntegrationTablePropertiesRequest createIntegrationTablePropertiesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createIntegrationTablePropertiesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createIntegrationTablePropertiesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Glue");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIntegrationTableProperties");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, CreateIntegrationTablePropertiesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams