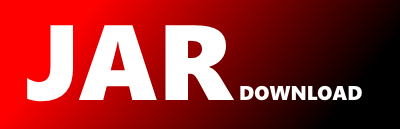
software.amazon.awssdk.services.glue.model.CrawlerTargets Maven / Gradle / Ivy
Show all versions of glue Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies data stores to crawl.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CrawlerTargets implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField> S3_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("S3Targets")
.getter(getter(CrawlerTargets::s3Targets))
.setter(setter(Builder::s3Targets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3Targets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(S3Target::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> JDBC_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("JdbcTargets")
.getter(getter(CrawlerTargets::jdbcTargets))
.setter(setter(Builder::jdbcTargets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JdbcTargets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(JdbcTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> MONGO_DB_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MongoDBTargets")
.getter(getter(CrawlerTargets::mongoDBTargets))
.setter(setter(Builder::mongoDBTargets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MongoDBTargets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MongoDBTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DYNAMO_DB_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DynamoDBTargets")
.getter(getter(CrawlerTargets::dynamoDBTargets))
.setter(setter(Builder::dynamoDBTargets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DynamoDBTargets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DynamoDBTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CATALOG_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CatalogTargets")
.getter(getter(CrawlerTargets::catalogTargets))
.setter(setter(Builder::catalogTargets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CatalogTargets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CatalogTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DELTA_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DeltaTargets")
.getter(getter(CrawlerTargets::deltaTargets))
.setter(setter(Builder::deltaTargets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeltaTargets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DeltaTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ICEBERG_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("IcebergTargets")
.getter(getter(CrawlerTargets::icebergTargets))
.setter(setter(Builder::icebergTargets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IcebergTargets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(IcebergTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> HUDI_TARGETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("HudiTargets")
.getter(getter(CrawlerTargets::hudiTargets))
.setter(setter(Builder::hudiTargets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HudiTargets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(HudiTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(S3_TARGETS_FIELD,
JDBC_TARGETS_FIELD, MONGO_DB_TARGETS_FIELD, DYNAMO_DB_TARGETS_FIELD, CATALOG_TARGETS_FIELD, DELTA_TARGETS_FIELD,
ICEBERG_TARGETS_FIELD, HUDI_TARGETS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final List s3Targets;
private final List jdbcTargets;
private final List mongoDBTargets;
private final List dynamoDBTargets;
private final List catalogTargets;
private final List deltaTargets;
private final List icebergTargets;
private final List hudiTargets;
private CrawlerTargets(BuilderImpl builder) {
this.s3Targets = builder.s3Targets;
this.jdbcTargets = builder.jdbcTargets;
this.mongoDBTargets = builder.mongoDBTargets;
this.dynamoDBTargets = builder.dynamoDBTargets;
this.catalogTargets = builder.catalogTargets;
this.deltaTargets = builder.deltaTargets;
this.icebergTargets = builder.icebergTargets;
this.hudiTargets = builder.hudiTargets;
}
/**
* For responses, this returns true if the service returned a value for the S3Targets property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasS3Targets() {
return s3Targets != null && !(s3Targets instanceof SdkAutoConstructList);
}
/**
*
* Specifies Amazon Simple Storage Service (Amazon S3) targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasS3Targets} method.
*
*
* @return Specifies Amazon Simple Storage Service (Amazon S3) targets.
*/
public final List s3Targets() {
return s3Targets;
}
/**
* For responses, this returns true if the service returned a value for the JdbcTargets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasJdbcTargets() {
return jdbcTargets != null && !(jdbcTargets instanceof SdkAutoConstructList);
}
/**
*
* Specifies JDBC targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasJdbcTargets} method.
*
*
* @return Specifies JDBC targets.
*/
public final List jdbcTargets() {
return jdbcTargets;
}
/**
* For responses, this returns true if the service returned a value for the MongoDBTargets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMongoDBTargets() {
return mongoDBTargets != null && !(mongoDBTargets instanceof SdkAutoConstructList);
}
/**
*
* Specifies Amazon DocumentDB or MongoDB targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMongoDBTargets} method.
*
*
* @return Specifies Amazon DocumentDB or MongoDB targets.
*/
public final List mongoDBTargets() {
return mongoDBTargets;
}
/**
* For responses, this returns true if the service returned a value for the DynamoDBTargets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDynamoDBTargets() {
return dynamoDBTargets != null && !(dynamoDBTargets instanceof SdkAutoConstructList);
}
/**
*
* Specifies Amazon DynamoDB targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDynamoDBTargets} method.
*
*
* @return Specifies Amazon DynamoDB targets.
*/
public final List dynamoDBTargets() {
return dynamoDBTargets;
}
/**
* For responses, this returns true if the service returned a value for the CatalogTargets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCatalogTargets() {
return catalogTargets != null && !(catalogTargets instanceof SdkAutoConstructList);
}
/**
*
* Specifies Glue Data Catalog targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCatalogTargets} method.
*
*
* @return Specifies Glue Data Catalog targets.
*/
public final List catalogTargets() {
return catalogTargets;
}
/**
* For responses, this returns true if the service returned a value for the DeltaTargets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDeltaTargets() {
return deltaTargets != null && !(deltaTargets instanceof SdkAutoConstructList);
}
/**
*
* Specifies Delta data store targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDeltaTargets} method.
*
*
* @return Specifies Delta data store targets.
*/
public final List deltaTargets() {
return deltaTargets;
}
/**
* For responses, this returns true if the service returned a value for the IcebergTargets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasIcebergTargets() {
return icebergTargets != null && !(icebergTargets instanceof SdkAutoConstructList);
}
/**
*
* Specifies Apache Iceberg data store targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIcebergTargets} method.
*
*
* @return Specifies Apache Iceberg data store targets.
*/
public final List icebergTargets() {
return icebergTargets;
}
/**
* For responses, this returns true if the service returned a value for the HudiTargets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasHudiTargets() {
return hudiTargets != null && !(hudiTargets instanceof SdkAutoConstructList);
}
/**
*
* Specifies Apache Hudi data store targets.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasHudiTargets} method.
*
*
* @return Specifies Apache Hudi data store targets.
*/
public final List hudiTargets() {
return hudiTargets;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasS3Targets() ? s3Targets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasJdbcTargets() ? jdbcTargets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasMongoDBTargets() ? mongoDBTargets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDynamoDBTargets() ? dynamoDBTargets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCatalogTargets() ? catalogTargets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDeltaTargets() ? deltaTargets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasIcebergTargets() ? icebergTargets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasHudiTargets() ? hudiTargets() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CrawlerTargets)) {
return false;
}
CrawlerTargets other = (CrawlerTargets) obj;
return hasS3Targets() == other.hasS3Targets() && Objects.equals(s3Targets(), other.s3Targets())
&& hasJdbcTargets() == other.hasJdbcTargets() && Objects.equals(jdbcTargets(), other.jdbcTargets())
&& hasMongoDBTargets() == other.hasMongoDBTargets() && Objects.equals(mongoDBTargets(), other.mongoDBTargets())
&& hasDynamoDBTargets() == other.hasDynamoDBTargets()
&& Objects.equals(dynamoDBTargets(), other.dynamoDBTargets()) && hasCatalogTargets() == other.hasCatalogTargets()
&& Objects.equals(catalogTargets(), other.catalogTargets()) && hasDeltaTargets() == other.hasDeltaTargets()
&& Objects.equals(deltaTargets(), other.deltaTargets()) && hasIcebergTargets() == other.hasIcebergTargets()
&& Objects.equals(icebergTargets(), other.icebergTargets()) && hasHudiTargets() == other.hasHudiTargets()
&& Objects.equals(hudiTargets(), other.hudiTargets());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CrawlerTargets").add("S3Targets", hasS3Targets() ? s3Targets() : null)
.add("JdbcTargets", hasJdbcTargets() ? jdbcTargets() : null)
.add("MongoDBTargets", hasMongoDBTargets() ? mongoDBTargets() : null)
.add("DynamoDBTargets", hasDynamoDBTargets() ? dynamoDBTargets() : null)
.add("CatalogTargets", hasCatalogTargets() ? catalogTargets() : null)
.add("DeltaTargets", hasDeltaTargets() ? deltaTargets() : null)
.add("IcebergTargets", hasIcebergTargets() ? icebergTargets() : null)
.add("HudiTargets", hasHudiTargets() ? hudiTargets() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "S3Targets":
return Optional.ofNullable(clazz.cast(s3Targets()));
case "JdbcTargets":
return Optional.ofNullable(clazz.cast(jdbcTargets()));
case "MongoDBTargets":
return Optional.ofNullable(clazz.cast(mongoDBTargets()));
case "DynamoDBTargets":
return Optional.ofNullable(clazz.cast(dynamoDBTargets()));
case "CatalogTargets":
return Optional.ofNullable(clazz.cast(catalogTargets()));
case "DeltaTargets":
return Optional.ofNullable(clazz.cast(deltaTargets()));
case "IcebergTargets":
return Optional.ofNullable(clazz.cast(icebergTargets()));
case "HudiTargets":
return Optional.ofNullable(clazz.cast(hudiTargets()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("S3Targets", S3_TARGETS_FIELD);
map.put("JdbcTargets", JDBC_TARGETS_FIELD);
map.put("MongoDBTargets", MONGO_DB_TARGETS_FIELD);
map.put("DynamoDBTargets", DYNAMO_DB_TARGETS_FIELD);
map.put("CatalogTargets", CATALOG_TARGETS_FIELD);
map.put("DeltaTargets", DELTA_TARGETS_FIELD);
map.put("IcebergTargets", ICEBERG_TARGETS_FIELD);
map.put("HudiTargets", HUDI_TARGETS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function