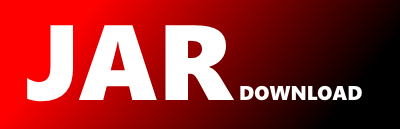
software.amazon.awssdk.services.glue.model.DecimalColumnStatisticsData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glue Show documentation
Show all versions of glue Show documentation
The AWS Java SDK for AWS Glue module holds the client classes that are used for communicating
with AWS Glue Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Defines column statistics supported for fixed-point number data columns.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DecimalColumnStatisticsData implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MINIMUM_VALUE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("MinimumValue").getter(getter(DecimalColumnStatisticsData::minimumValue))
.setter(setter(Builder::minimumValue)).constructor(DecimalNumber::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinimumValue").build()).build();
private static final SdkField MAXIMUM_VALUE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("MaximumValue").getter(getter(DecimalColumnStatisticsData::maximumValue))
.setter(setter(Builder::maximumValue)).constructor(DecimalNumber::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumValue").build()).build();
private static final SdkField NUMBER_OF_NULLS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("NumberOfNulls").getter(getter(DecimalColumnStatisticsData::numberOfNulls))
.setter(setter(Builder::numberOfNulls))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfNulls").build()).build();
private static final SdkField NUMBER_OF_DISTINCT_VALUES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("NumberOfDistinctValues").getter(getter(DecimalColumnStatisticsData::numberOfDistinctValues))
.setter(setter(Builder::numberOfDistinctValues))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfDistinctValues").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MINIMUM_VALUE_FIELD,
MAXIMUM_VALUE_FIELD, NUMBER_OF_NULLS_FIELD, NUMBER_OF_DISTINCT_VALUES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final DecimalNumber minimumValue;
private final DecimalNumber maximumValue;
private final Long numberOfNulls;
private final Long numberOfDistinctValues;
private DecimalColumnStatisticsData(BuilderImpl builder) {
this.minimumValue = builder.minimumValue;
this.maximumValue = builder.maximumValue;
this.numberOfNulls = builder.numberOfNulls;
this.numberOfDistinctValues = builder.numberOfDistinctValues;
}
/**
*
* The lowest value in the column.
*
*
* @return The lowest value in the column.
*/
public final DecimalNumber minimumValue() {
return minimumValue;
}
/**
*
* The highest value in the column.
*
*
* @return The highest value in the column.
*/
public final DecimalNumber maximumValue() {
return maximumValue;
}
/**
*
* The number of null values in the column.
*
*
* @return The number of null values in the column.
*/
public final Long numberOfNulls() {
return numberOfNulls;
}
/**
*
* The number of distinct values in a column.
*
*
* @return The number of distinct values in a column.
*/
public final Long numberOfDistinctValues() {
return numberOfDistinctValues;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(minimumValue());
hashCode = 31 * hashCode + Objects.hashCode(maximumValue());
hashCode = 31 * hashCode + Objects.hashCode(numberOfNulls());
hashCode = 31 * hashCode + Objects.hashCode(numberOfDistinctValues());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DecimalColumnStatisticsData)) {
return false;
}
DecimalColumnStatisticsData other = (DecimalColumnStatisticsData) obj;
return Objects.equals(minimumValue(), other.minimumValue()) && Objects.equals(maximumValue(), other.maximumValue())
&& Objects.equals(numberOfNulls(), other.numberOfNulls())
&& Objects.equals(numberOfDistinctValues(), other.numberOfDistinctValues());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DecimalColumnStatisticsData").add("MinimumValue", minimumValue())
.add("MaximumValue", maximumValue()).add("NumberOfNulls", numberOfNulls())
.add("NumberOfDistinctValues", numberOfDistinctValues()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MinimumValue":
return Optional.ofNullable(clazz.cast(minimumValue()));
case "MaximumValue":
return Optional.ofNullable(clazz.cast(maximumValue()));
case "NumberOfNulls":
return Optional.ofNullable(clazz.cast(numberOfNulls()));
case "NumberOfDistinctValues":
return Optional.ofNullable(clazz.cast(numberOfDistinctValues()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("MinimumValue", MINIMUM_VALUE_FIELD);
map.put("MaximumValue", MAXIMUM_VALUE_FIELD);
map.put("NumberOfNulls", NUMBER_OF_NULLS_FIELD);
map.put("NumberOfDistinctValues", NUMBER_OF_DISTINCT_VALUES_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy