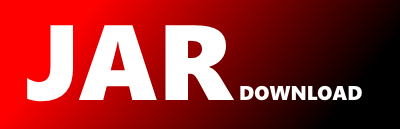
software.amazon.awssdk.services.glue.model.GetSchemaResponse Maven / Gradle / Ivy
Show all versions of glue Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetSchemaResponse extends GlueResponse implements
ToCopyableBuilder {
private static final SdkField REGISTRY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RegistryName").getter(getter(GetSchemaResponse::registryName)).setter(setter(Builder::registryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegistryName").build()).build();
private static final SdkField REGISTRY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RegistryArn").getter(getter(GetSchemaResponse::registryArn)).setter(setter(Builder::registryArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegistryArn").build()).build();
private static final SdkField SCHEMA_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaName").getter(getter(GetSchemaResponse::schemaName)).setter(setter(Builder::schemaName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaName").build()).build();
private static final SdkField SCHEMA_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaArn").getter(getter(GetSchemaResponse::schemaArn)).setter(setter(Builder::schemaArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaArn").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(GetSchemaResponse::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField DATA_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataFormat").getter(getter(GetSchemaResponse::dataFormatAsString)).setter(setter(Builder::dataFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataFormat").build()).build();
private static final SdkField COMPATIBILITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Compatibility").getter(getter(GetSchemaResponse::compatibilityAsString))
.setter(setter(Builder::compatibility))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Compatibility").build()).build();
private static final SdkField SCHEMA_CHECKPOINT_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("SchemaCheckpoint").getter(getter(GetSchemaResponse::schemaCheckpoint))
.setter(setter(Builder::schemaCheckpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaCheckpoint").build()).build();
private static final SdkField LATEST_SCHEMA_VERSION_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("LatestSchemaVersion").getter(getter(GetSchemaResponse::latestSchemaVersion))
.setter(setter(Builder::latestSchemaVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LatestSchemaVersion").build())
.build();
private static final SdkField NEXT_SCHEMA_VERSION_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("NextSchemaVersion").getter(getter(GetSchemaResponse::nextSchemaVersion))
.setter(setter(Builder::nextSchemaVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextSchemaVersion").build()).build();
private static final SdkField SCHEMA_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaStatus").getter(getter(GetSchemaResponse::schemaStatusAsString))
.setter(setter(Builder::schemaStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaStatus").build()).build();
private static final SdkField CREATED_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreatedTime").getter(getter(GetSchemaResponse::createdTime)).setter(setter(Builder::createdTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedTime").build()).build();
private static final SdkField UPDATED_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpdatedTime").getter(getter(GetSchemaResponse::updatedTime)).setter(setter(Builder::updatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdatedTime").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGISTRY_NAME_FIELD,
REGISTRY_ARN_FIELD, SCHEMA_NAME_FIELD, SCHEMA_ARN_FIELD, DESCRIPTION_FIELD, DATA_FORMAT_FIELD, COMPATIBILITY_FIELD,
SCHEMA_CHECKPOINT_FIELD, LATEST_SCHEMA_VERSION_FIELD, NEXT_SCHEMA_VERSION_FIELD, SCHEMA_STATUS_FIELD,
CREATED_TIME_FIELD, UPDATED_TIME_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String registryName;
private final String registryArn;
private final String schemaName;
private final String schemaArn;
private final String description;
private final String dataFormat;
private final String compatibility;
private final Long schemaCheckpoint;
private final Long latestSchemaVersion;
private final Long nextSchemaVersion;
private final String schemaStatus;
private final String createdTime;
private final String updatedTime;
private GetSchemaResponse(BuilderImpl builder) {
super(builder);
this.registryName = builder.registryName;
this.registryArn = builder.registryArn;
this.schemaName = builder.schemaName;
this.schemaArn = builder.schemaArn;
this.description = builder.description;
this.dataFormat = builder.dataFormat;
this.compatibility = builder.compatibility;
this.schemaCheckpoint = builder.schemaCheckpoint;
this.latestSchemaVersion = builder.latestSchemaVersion;
this.nextSchemaVersion = builder.nextSchemaVersion;
this.schemaStatus = builder.schemaStatus;
this.createdTime = builder.createdTime;
this.updatedTime = builder.updatedTime;
}
/**
*
* The name of the registry.
*
*
* @return The name of the registry.
*/
public final String registryName() {
return registryName;
}
/**
*
* The Amazon Resource Name (ARN) of the registry.
*
*
* @return The Amazon Resource Name (ARN) of the registry.
*/
public final String registryArn() {
return registryArn;
}
/**
*
* The name of the schema.
*
*
* @return The name of the schema.
*/
public final String schemaName() {
return schemaName;
}
/**
*
* The Amazon Resource Name (ARN) of the schema.
*
*
* @return The Amazon Resource Name (ARN) of the schema.
*/
public final String schemaArn() {
return schemaArn;
}
/**
*
* A description of schema if specified when created
*
*
* @return A description of schema if specified when created
*/
public final String description() {
return description;
}
/**
*
* The data format of the schema definition. Currently AVRO
, JSON
and
* PROTOBUF
are supported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataFormat} will
* return {@link DataFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataFormatAsString}.
*
*
* @return The data format of the schema definition. Currently AVRO
, JSON
and
* PROTOBUF
are supported.
* @see DataFormat
*/
public final DataFormat dataFormat() {
return DataFormat.fromValue(dataFormat);
}
/**
*
* The data format of the schema definition. Currently AVRO
, JSON
and
* PROTOBUF
are supported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataFormat} will
* return {@link DataFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataFormatAsString}.
*
*
* @return The data format of the schema definition. Currently AVRO
, JSON
and
* PROTOBUF
are supported.
* @see DataFormat
*/
public final String dataFormatAsString() {
return dataFormat;
}
/**
*
* The compatibility mode of the schema.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compatibility}
* will return {@link Compatibility#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compatibilityAsString}.
*
*
* @return The compatibility mode of the schema.
* @see Compatibility
*/
public final Compatibility compatibility() {
return Compatibility.fromValue(compatibility);
}
/**
*
* The compatibility mode of the schema.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compatibility}
* will return {@link Compatibility#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compatibilityAsString}.
*
*
* @return The compatibility mode of the schema.
* @see Compatibility
*/
public final String compatibilityAsString() {
return compatibility;
}
/**
*
* The version number of the checkpoint (the last time the compatibility mode was changed).
*
*
* @return The version number of the checkpoint (the last time the compatibility mode was changed).
*/
public final Long schemaCheckpoint() {
return schemaCheckpoint;
}
/**
*
* The latest version of the schema associated with the returned schema definition.
*
*
* @return The latest version of the schema associated with the returned schema definition.
*/
public final Long latestSchemaVersion() {
return latestSchemaVersion;
}
/**
*
* The next version of the schema associated with the returned schema definition.
*
*
* @return The next version of the schema associated with the returned schema definition.
*/
public final Long nextSchemaVersion() {
return nextSchemaVersion;
}
/**
*
* The status of the schema.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #schemaStatus} will
* return {@link SchemaStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #schemaStatusAsString}.
*
*
* @return The status of the schema.
* @see SchemaStatus
*/
public final SchemaStatus schemaStatus() {
return SchemaStatus.fromValue(schemaStatus);
}
/**
*
* The status of the schema.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #schemaStatus} will
* return {@link SchemaStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #schemaStatusAsString}.
*
*
* @return The status of the schema.
* @see SchemaStatus
*/
public final String schemaStatusAsString() {
return schemaStatus;
}
/**
*
* The date and time the schema was created.
*
*
* @return The date and time the schema was created.
*/
public final String createdTime() {
return createdTime;
}
/**
*
* The date and time the schema was updated.
*
*
* @return The date and time the schema was updated.
*/
public final String updatedTime() {
return updatedTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(registryName());
hashCode = 31 * hashCode + Objects.hashCode(registryArn());
hashCode = 31 * hashCode + Objects.hashCode(schemaName());
hashCode = 31 * hashCode + Objects.hashCode(schemaArn());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(dataFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(compatibilityAsString());
hashCode = 31 * hashCode + Objects.hashCode(schemaCheckpoint());
hashCode = 31 * hashCode + Objects.hashCode(latestSchemaVersion());
hashCode = 31 * hashCode + Objects.hashCode(nextSchemaVersion());
hashCode = 31 * hashCode + Objects.hashCode(schemaStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(createdTime());
hashCode = 31 * hashCode + Objects.hashCode(updatedTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetSchemaResponse)) {
return false;
}
GetSchemaResponse other = (GetSchemaResponse) obj;
return Objects.equals(registryName(), other.registryName()) && Objects.equals(registryArn(), other.registryArn())
&& Objects.equals(schemaName(), other.schemaName()) && Objects.equals(schemaArn(), other.schemaArn())
&& Objects.equals(description(), other.description())
&& Objects.equals(dataFormatAsString(), other.dataFormatAsString())
&& Objects.equals(compatibilityAsString(), other.compatibilityAsString())
&& Objects.equals(schemaCheckpoint(), other.schemaCheckpoint())
&& Objects.equals(latestSchemaVersion(), other.latestSchemaVersion())
&& Objects.equals(nextSchemaVersion(), other.nextSchemaVersion())
&& Objects.equals(schemaStatusAsString(), other.schemaStatusAsString())
&& Objects.equals(createdTime(), other.createdTime()) && Objects.equals(updatedTime(), other.updatedTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetSchemaResponse").add("RegistryName", registryName()).add("RegistryArn", registryArn())
.add("SchemaName", schemaName()).add("SchemaArn", schemaArn()).add("Description", description())
.add("DataFormat", dataFormatAsString()).add("Compatibility", compatibilityAsString())
.add("SchemaCheckpoint", schemaCheckpoint()).add("LatestSchemaVersion", latestSchemaVersion())
.add("NextSchemaVersion", nextSchemaVersion()).add("SchemaStatus", schemaStatusAsString())
.add("CreatedTime", createdTime()).add("UpdatedTime", updatedTime()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RegistryName":
return Optional.ofNullable(clazz.cast(registryName()));
case "RegistryArn":
return Optional.ofNullable(clazz.cast(registryArn()));
case "SchemaName":
return Optional.ofNullable(clazz.cast(schemaName()));
case "SchemaArn":
return Optional.ofNullable(clazz.cast(schemaArn()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "DataFormat":
return Optional.ofNullable(clazz.cast(dataFormatAsString()));
case "Compatibility":
return Optional.ofNullable(clazz.cast(compatibilityAsString()));
case "SchemaCheckpoint":
return Optional.ofNullable(clazz.cast(schemaCheckpoint()));
case "LatestSchemaVersion":
return Optional.ofNullable(clazz.cast(latestSchemaVersion()));
case "NextSchemaVersion":
return Optional.ofNullable(clazz.cast(nextSchemaVersion()));
case "SchemaStatus":
return Optional.ofNullable(clazz.cast(schemaStatusAsString()));
case "CreatedTime":
return Optional.ofNullable(clazz.cast(createdTime()));
case "UpdatedTime":
return Optional.ofNullable(clazz.cast(updatedTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("RegistryName", REGISTRY_NAME_FIELD);
map.put("RegistryArn", REGISTRY_ARN_FIELD);
map.put("SchemaName", SCHEMA_NAME_FIELD);
map.put("SchemaArn", SCHEMA_ARN_FIELD);
map.put("Description", DESCRIPTION_FIELD);
map.put("DataFormat", DATA_FORMAT_FIELD);
map.put("Compatibility", COMPATIBILITY_FIELD);
map.put("SchemaCheckpoint", SCHEMA_CHECKPOINT_FIELD);
map.put("LatestSchemaVersion", LATEST_SCHEMA_VERSION_FIELD);
map.put("NextSchemaVersion", NEXT_SCHEMA_VERSION_FIELD);
map.put("SchemaStatus", SCHEMA_STATUS_FIELD);
map.put("CreatedTime", CREATED_TIME_FIELD);
map.put("UpdatedTime", UPDATED_TIME_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function