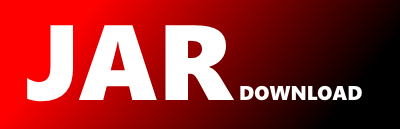
software.amazon.awssdk.services.glue.model.S3CsvSource Maven / Gradle / Ivy
Show all versions of glue Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.glue.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies a command-separated value (CSV) data store stored in Amazon S3.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class S3CsvSource implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(S3CsvSource::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField> PATHS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Paths")
.getter(getter(S3CsvSource::paths))
.setter(setter(Builder::paths))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Paths").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField COMPRESSION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompressionType").getter(getter(S3CsvSource::compressionTypeAsString))
.setter(setter(Builder::compressionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompressionType").build()).build();
private static final SdkField> EXCLUSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Exclusions")
.getter(getter(S3CsvSource::exclusions))
.setter(setter(Builder::exclusions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Exclusions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField GROUP_SIZE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GroupSize").getter(getter(S3CsvSource::groupSize)).setter(setter(Builder::groupSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GroupSize").build()).build();
private static final SdkField GROUP_FILES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GroupFiles").getter(getter(S3CsvSource::groupFiles)).setter(setter(Builder::groupFiles))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GroupFiles").build()).build();
private static final SdkField RECURSE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Recurse").getter(getter(S3CsvSource::recurse)).setter(setter(Builder::recurse))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Recurse").build()).build();
private static final SdkField MAX_BAND_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxBand").getter(getter(S3CsvSource::maxBand)).setter(setter(Builder::maxBand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxBand").build()).build();
private static final SdkField MAX_FILES_IN_BAND_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxFilesInBand").getter(getter(S3CsvSource::maxFilesInBand)).setter(setter(Builder::maxFilesInBand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxFilesInBand").build()).build();
private static final SdkField ADDITIONAL_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AdditionalOptions")
.getter(getter(S3CsvSource::additionalOptions)).setter(setter(Builder::additionalOptions))
.constructor(S3DirectSourceAdditionalOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdditionalOptions").build()).build();
private static final SdkField SEPARATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Separator").getter(getter(S3CsvSource::separatorAsString)).setter(setter(Builder::separator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Separator").build()).build();
private static final SdkField ESCAPER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Escaper")
.getter(getter(S3CsvSource::escaper)).setter(setter(Builder::escaper))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Escaper").build()).build();
private static final SdkField QUOTE_CHAR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("QuoteChar").getter(getter(S3CsvSource::quoteCharAsString)).setter(setter(Builder::quoteChar))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QuoteChar").build()).build();
private static final SdkField MULTILINE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Multiline").getter(getter(S3CsvSource::multiline)).setter(setter(Builder::multiline))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Multiline").build()).build();
private static final SdkField WITH_HEADER_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("WithHeader").getter(getter(S3CsvSource::withHeader)).setter(setter(Builder::withHeader))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WithHeader").build()).build();
private static final SdkField WRITE_HEADER_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("WriteHeader").getter(getter(S3CsvSource::writeHeader)).setter(setter(Builder::writeHeader))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WriteHeader").build()).build();
private static final SdkField SKIP_FIRST_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("SkipFirst").getter(getter(S3CsvSource::skipFirst)).setter(setter(Builder::skipFirst))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SkipFirst").build()).build();
private static final SdkField OPTIMIZE_PERFORMANCE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("OptimizePerformance").getter(getter(S3CsvSource::optimizePerformance))
.setter(setter(Builder::optimizePerformance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptimizePerformance").build())
.build();
private static final SdkField> OUTPUT_SCHEMAS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OutputSchemas")
.getter(getter(S3CsvSource::outputSchemas))
.setter(setter(Builder::outputSchemas))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputSchemas").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(GlueSchema::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, PATHS_FIELD,
COMPRESSION_TYPE_FIELD, EXCLUSIONS_FIELD, GROUP_SIZE_FIELD, GROUP_FILES_FIELD, RECURSE_FIELD, MAX_BAND_FIELD,
MAX_FILES_IN_BAND_FIELD, ADDITIONAL_OPTIONS_FIELD, SEPARATOR_FIELD, ESCAPER_FIELD, QUOTE_CHAR_FIELD, MULTILINE_FIELD,
WITH_HEADER_FIELD, WRITE_HEADER_FIELD, SKIP_FIRST_FIELD, OPTIMIZE_PERFORMANCE_FIELD, OUTPUT_SCHEMAS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String name;
private final List paths;
private final String compressionType;
private final List exclusions;
private final String groupSize;
private final String groupFiles;
private final Boolean recurse;
private final Integer maxBand;
private final Integer maxFilesInBand;
private final S3DirectSourceAdditionalOptions additionalOptions;
private final String separator;
private final String escaper;
private final String quoteChar;
private final Boolean multiline;
private final Boolean withHeader;
private final Boolean writeHeader;
private final Boolean skipFirst;
private final Boolean optimizePerformance;
private final List outputSchemas;
private S3CsvSource(BuilderImpl builder) {
this.name = builder.name;
this.paths = builder.paths;
this.compressionType = builder.compressionType;
this.exclusions = builder.exclusions;
this.groupSize = builder.groupSize;
this.groupFiles = builder.groupFiles;
this.recurse = builder.recurse;
this.maxBand = builder.maxBand;
this.maxFilesInBand = builder.maxFilesInBand;
this.additionalOptions = builder.additionalOptions;
this.separator = builder.separator;
this.escaper = builder.escaper;
this.quoteChar = builder.quoteChar;
this.multiline = builder.multiline;
this.withHeader = builder.withHeader;
this.writeHeader = builder.writeHeader;
this.skipFirst = builder.skipFirst;
this.optimizePerformance = builder.optimizePerformance;
this.outputSchemas = builder.outputSchemas;
}
/**
*
* The name of the data store.
*
*
* @return The name of the data store.
*/
public final String name() {
return name;
}
/**
* For responses, this returns true if the service returned a value for the Paths property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasPaths() {
return paths != null && !(paths instanceof SdkAutoConstructList);
}
/**
*
* A list of the Amazon S3 paths to read from.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPaths} method.
*
*
* @return A list of the Amazon S3 paths to read from.
*/
public final List paths() {
return paths;
}
/**
*
* Specifies how the data is compressed. This is generally not necessary if the data has a standard file extension.
* Possible values are "gzip"
and "bzip"
).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compressionType}
* will return {@link CompressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #compressionTypeAsString}.
*
*
* @return Specifies how the data is compressed. This is generally not necessary if the data has a standard file
* extension. Possible values are "gzip"
and "bzip"
).
* @see CompressionType
*/
public final CompressionType compressionType() {
return CompressionType.fromValue(compressionType);
}
/**
*
* Specifies how the data is compressed. This is generally not necessary if the data has a standard file extension.
* Possible values are "gzip"
and "bzip"
).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compressionType}
* will return {@link CompressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #compressionTypeAsString}.
*
*
* @return Specifies how the data is compressed. This is generally not necessary if the data has a standard file
* extension. Possible values are "gzip"
and "bzip"
).
* @see CompressionType
*/
public final String compressionTypeAsString() {
return compressionType;
}
/**
* For responses, this returns true if the service returned a value for the Exclusions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasExclusions() {
return exclusions != null && !(exclusions instanceof SdkAutoConstructList);
}
/**
*
* A string containing a JSON list of Unix-style glob patterns to exclude. For example, "[\"**.pdf\"]" excludes all
* PDF files.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExclusions} method.
*
*
* @return A string containing a JSON list of Unix-style glob patterns to exclude. For example, "[\"**.pdf\"]"
* excludes all PDF files.
*/
public final List exclusions() {
return exclusions;
}
/**
*
* The target group size in bytes. The default is computed based on the input data size and the size of your
* cluster. When there are fewer than 50,000 input files, "groupFiles"
must be set to
* "inPartition"
for this to take effect.
*
*
* @return The target group size in bytes. The default is computed based on the input data size and the size of your
* cluster. When there are fewer than 50,000 input files, "groupFiles"
must be set to
* "inPartition"
for this to take effect.
*/
public final String groupSize() {
return groupSize;
}
/**
*
* Grouping files is turned on by default when the input contains more than 50,000 files. To turn on grouping with
* fewer than 50,000 files, set this parameter to "inPartition". To disable grouping when there are more than 50,000
* files, set this parameter to "none"
.
*
*
* @return Grouping files is turned on by default when the input contains more than 50,000 files. To turn on
* grouping with fewer than 50,000 files, set this parameter to "inPartition". To disable grouping when
* there are more than 50,000 files, set this parameter to "none"
.
*/
public final String groupFiles() {
return groupFiles;
}
/**
*
* If set to true, recursively reads files in all subdirectories under the specified paths.
*
*
* @return If set to true, recursively reads files in all subdirectories under the specified paths.
*/
public final Boolean recurse() {
return recurse;
}
/**
*
* This option controls the duration in milliseconds after which the s3 listing is likely to be consistent. Files
* with modification timestamps falling within the last maxBand milliseconds are tracked specially when using
* JobBookmarks to account for Amazon S3 eventual consistency. Most users don't need to set this option. The default
* is 900000 milliseconds, or 15 minutes.
*
*
* @return This option controls the duration in milliseconds after which the s3 listing is likely to be consistent.
* Files with modification timestamps falling within the last maxBand milliseconds are tracked specially
* when using JobBookmarks to account for Amazon S3 eventual consistency. Most users don't need to set this
* option. The default is 900000 milliseconds, or 15 minutes.
*/
public final Integer maxBand() {
return maxBand;
}
/**
*
* This option specifies the maximum number of files to save from the last maxBand seconds. If this number is
* exceeded, extra files are skipped and only processed in the next job run.
*
*
* @return This option specifies the maximum number of files to save from the last maxBand seconds. If this number
* is exceeded, extra files are skipped and only processed in the next job run.
*/
public final Integer maxFilesInBand() {
return maxFilesInBand;
}
/**
*
* Specifies additional connection options.
*
*
* @return Specifies additional connection options.
*/
public final S3DirectSourceAdditionalOptions additionalOptions() {
return additionalOptions;
}
/**
*
* Specifies the delimiter character. The default is a comma: ",", but any other character can be specified.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #separator} will
* return {@link Separator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #separatorAsString}.
*
*
* @return Specifies the delimiter character. The default is a comma: ",", but any other character can be specified.
* @see Separator
*/
public final Separator separator() {
return Separator.fromValue(separator);
}
/**
*
* Specifies the delimiter character. The default is a comma: ",", but any other character can be specified.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #separator} will
* return {@link Separator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #separatorAsString}.
*
*
* @return Specifies the delimiter character. The default is a comma: ",", but any other character can be specified.
* @see Separator
*/
public final String separatorAsString() {
return separator;
}
/**
*
* Specifies a character to use for escaping. This option is used only when reading CSV files. The default value is
* none
. If enabled, the character which immediately follows is used as-is, except for a small set of
* well-known escapes (\n
, \r
, \t
, and \0
).
*
*
* @return Specifies a character to use for escaping. This option is used only when reading CSV files. The default
* value is none
. If enabled, the character which immediately follows is used as-is, except for
* a small set of well-known escapes (\n
, \r
, \t
, and \0
* ).
*/
public final String escaper() {
return escaper;
}
/**
*
* Specifies the character to use for quoting. The default is a double quote: '"'
. Set this to
* -1
to turn off quoting entirely.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #quoteChar} will
* return {@link QuoteChar#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #quoteCharAsString}.
*
*
* @return Specifies the character to use for quoting. The default is a double quote: '"'
. Set this to
* -1
to turn off quoting entirely.
* @see QuoteChar
*/
public final QuoteChar quoteChar() {
return QuoteChar.fromValue(quoteChar);
}
/**
*
* Specifies the character to use for quoting. The default is a double quote: '"'
. Set this to
* -1
to turn off quoting entirely.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #quoteChar} will
* return {@link QuoteChar#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #quoteCharAsString}.
*
*
* @return Specifies the character to use for quoting. The default is a double quote: '"'
. Set this to
* -1
to turn off quoting entirely.
* @see QuoteChar
*/
public final String quoteCharAsString() {
return quoteChar;
}
/**
*
* A Boolean value that specifies whether a single record can span multiple lines. This can occur when a field
* contains a quoted new-line character. You must set this option to True if any record spans multiple lines. The
* default value is False
, which allows for more aggressive file-splitting during parsing.
*
*
* @return A Boolean value that specifies whether a single record can span multiple lines. This can occur when a
* field contains a quoted new-line character. You must set this option to True if any record spans multiple
* lines. The default value is False
, which allows for more aggressive file-splitting during
* parsing.
*/
public final Boolean multiline() {
return multiline;
}
/**
*
* A Boolean value that specifies whether to treat the first line as a header. The default value is
* False
.
*
*
* @return A Boolean value that specifies whether to treat the first line as a header. The default value is
* False
.
*/
public final Boolean withHeader() {
return withHeader;
}
/**
*
* A Boolean value that specifies whether to write the header to output. The default value is True
.
*
*
* @return A Boolean value that specifies whether to write the header to output. The default value is
* True
.
*/
public final Boolean writeHeader() {
return writeHeader;
}
/**
*
* A Boolean value that specifies whether to skip the first data line. The default value is False
.
*
*
* @return A Boolean value that specifies whether to skip the first data line. The default value is
* False
.
*/
public final Boolean skipFirst() {
return skipFirst;
}
/**
*
* A Boolean value that specifies whether to use the advanced SIMD CSV reader along with Apache Arrow based columnar
* memory formats. Only available in Glue version 3.0.
*
*
* @return A Boolean value that specifies whether to use the advanced SIMD CSV reader along with Apache Arrow based
* columnar memory formats. Only available in Glue version 3.0.
*/
public final Boolean optimizePerformance() {
return optimizePerformance;
}
/**
* For responses, this returns true if the service returned a value for the OutputSchemas property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOutputSchemas() {
return outputSchemas != null && !(outputSchemas instanceof SdkAutoConstructList);
}
/**
*
* Specifies the data schema for the S3 CSV source.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOutputSchemas} method.
*
*
* @return Specifies the data schema for the S3 CSV source.
*/
public final List outputSchemas() {
return outputSchemas;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(hasPaths() ? paths() : null);
hashCode = 31 * hashCode + Objects.hashCode(compressionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasExclusions() ? exclusions() : null);
hashCode = 31 * hashCode + Objects.hashCode(groupSize());
hashCode = 31 * hashCode + Objects.hashCode(groupFiles());
hashCode = 31 * hashCode + Objects.hashCode(recurse());
hashCode = 31 * hashCode + Objects.hashCode(maxBand());
hashCode = 31 * hashCode + Objects.hashCode(maxFilesInBand());
hashCode = 31 * hashCode + Objects.hashCode(additionalOptions());
hashCode = 31 * hashCode + Objects.hashCode(separatorAsString());
hashCode = 31 * hashCode + Objects.hashCode(escaper());
hashCode = 31 * hashCode + Objects.hashCode(quoteCharAsString());
hashCode = 31 * hashCode + Objects.hashCode(multiline());
hashCode = 31 * hashCode + Objects.hashCode(withHeader());
hashCode = 31 * hashCode + Objects.hashCode(writeHeader());
hashCode = 31 * hashCode + Objects.hashCode(skipFirst());
hashCode = 31 * hashCode + Objects.hashCode(optimizePerformance());
hashCode = 31 * hashCode + Objects.hashCode(hasOutputSchemas() ? outputSchemas() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof S3CsvSource)) {
return false;
}
S3CsvSource other = (S3CsvSource) obj;
return Objects.equals(name(), other.name()) && hasPaths() == other.hasPaths() && Objects.equals(paths(), other.paths())
&& Objects.equals(compressionTypeAsString(), other.compressionTypeAsString())
&& hasExclusions() == other.hasExclusions() && Objects.equals(exclusions(), other.exclusions())
&& Objects.equals(groupSize(), other.groupSize()) && Objects.equals(groupFiles(), other.groupFiles())
&& Objects.equals(recurse(), other.recurse()) && Objects.equals(maxBand(), other.maxBand())
&& Objects.equals(maxFilesInBand(), other.maxFilesInBand())
&& Objects.equals(additionalOptions(), other.additionalOptions())
&& Objects.equals(separatorAsString(), other.separatorAsString()) && Objects.equals(escaper(), other.escaper())
&& Objects.equals(quoteCharAsString(), other.quoteCharAsString())
&& Objects.equals(multiline(), other.multiline()) && Objects.equals(withHeader(), other.withHeader())
&& Objects.equals(writeHeader(), other.writeHeader()) && Objects.equals(skipFirst(), other.skipFirst())
&& Objects.equals(optimizePerformance(), other.optimizePerformance())
&& hasOutputSchemas() == other.hasOutputSchemas() && Objects.equals(outputSchemas(), other.outputSchemas());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("S3CsvSource").add("Name", name()).add("Paths", hasPaths() ? paths() : null)
.add("CompressionType", compressionTypeAsString()).add("Exclusions", hasExclusions() ? exclusions() : null)
.add("GroupSize", groupSize()).add("GroupFiles", groupFiles()).add("Recurse", recurse())
.add("MaxBand", maxBand()).add("MaxFilesInBand", maxFilesInBand()).add("AdditionalOptions", additionalOptions())
.add("Separator", separatorAsString()).add("Escaper", escaper()).add("QuoteChar", quoteCharAsString())
.add("Multiline", multiline()).add("WithHeader", withHeader()).add("WriteHeader", writeHeader())
.add("SkipFirst", skipFirst()).add("OptimizePerformance", optimizePerformance())
.add("OutputSchemas", hasOutputSchemas() ? outputSchemas() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Paths":
return Optional.ofNullable(clazz.cast(paths()));
case "CompressionType":
return Optional.ofNullable(clazz.cast(compressionTypeAsString()));
case "Exclusions":
return Optional.ofNullable(clazz.cast(exclusions()));
case "GroupSize":
return Optional.ofNullable(clazz.cast(groupSize()));
case "GroupFiles":
return Optional.ofNullable(clazz.cast(groupFiles()));
case "Recurse":
return Optional.ofNullable(clazz.cast(recurse()));
case "MaxBand":
return Optional.ofNullable(clazz.cast(maxBand()));
case "MaxFilesInBand":
return Optional.ofNullable(clazz.cast(maxFilesInBand()));
case "AdditionalOptions":
return Optional.ofNullable(clazz.cast(additionalOptions()));
case "Separator":
return Optional.ofNullable(clazz.cast(separatorAsString()));
case "Escaper":
return Optional.ofNullable(clazz.cast(escaper()));
case "QuoteChar":
return Optional.ofNullable(clazz.cast(quoteCharAsString()));
case "Multiline":
return Optional.ofNullable(clazz.cast(multiline()));
case "WithHeader":
return Optional.ofNullable(clazz.cast(withHeader()));
case "WriteHeader":
return Optional.ofNullable(clazz.cast(writeHeader()));
case "SkipFirst":
return Optional.ofNullable(clazz.cast(skipFirst()));
case "OptimizePerformance":
return Optional.ofNullable(clazz.cast(optimizePerformance()));
case "OutputSchemas":
return Optional.ofNullable(clazz.cast(outputSchemas()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Name", NAME_FIELD);
map.put("Paths", PATHS_FIELD);
map.put("CompressionType", COMPRESSION_TYPE_FIELD);
map.put("Exclusions", EXCLUSIONS_FIELD);
map.put("GroupSize", GROUP_SIZE_FIELD);
map.put("GroupFiles", GROUP_FILES_FIELD);
map.put("Recurse", RECURSE_FIELD);
map.put("MaxBand", MAX_BAND_FIELD);
map.put("MaxFilesInBand", MAX_FILES_IN_BAND_FIELD);
map.put("AdditionalOptions", ADDITIONAL_OPTIONS_FIELD);
map.put("Separator", SEPARATOR_FIELD);
map.put("Escaper", ESCAPER_FIELD);
map.put("QuoteChar", QUOTE_CHAR_FIELD);
map.put("Multiline", MULTILINE_FIELD);
map.put("WithHeader", WITH_HEADER_FIELD);
map.put("WriteHeader", WRITE_HEADER_FIELD);
map.put("SkipFirst", SKIP_FIRST_FIELD);
map.put("OptimizePerformance", OPTIMIZE_PERFORMANCE_FIELD);
map.put("OutputSchemas", OUTPUT_SCHEMAS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function