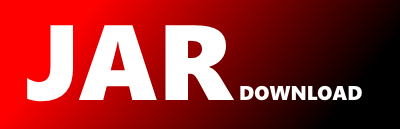
software.amazon.awssdk.services.greengrass.model.FunctionConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of greengrass Show documentation
Show all versions of greengrass Show documentation
>The AWS Java SDK for AWS Greengrass module holds the client classes that are used for communicating
with AWS Greengrass Service
The newest version!
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.greengrass.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* The configuration of the Lambda function.
*/
@Generated("software.amazon.awssdk:codegen")
public final class FunctionConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENCODING_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EncodingType").getter(getter(FunctionConfiguration::encodingTypeAsString))
.setter(setter(Builder::encodingType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EncodingType").build()).build();
private static final SdkField ENVIRONMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Environment")
.getter(getter(FunctionConfiguration::environment)).setter(setter(Builder::environment))
.constructor(FunctionConfigurationEnvironment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Environment").build()).build();
private static final SdkField EXEC_ARGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExecArgs").getter(getter(FunctionConfiguration::execArgs)).setter(setter(Builder::execArgs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecArgs").build()).build();
private static final SdkField EXECUTABLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Executable").getter(getter(FunctionConfiguration::executable)).setter(setter(Builder::executable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Executable").build()).build();
private static final SdkField MEMORY_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MemorySize").getter(getter(FunctionConfiguration::memorySize)).setter(setter(Builder::memorySize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemorySize").build()).build();
private static final SdkField PINNED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Pinned").getter(getter(FunctionConfiguration::pinned)).setter(setter(Builder::pinned))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Pinned").build()).build();
private static final SdkField TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Timeout").getter(getter(FunctionConfiguration::timeout)).setter(setter(Builder::timeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timeout").build()).build();
private static final SdkField FUNCTION_RUNTIME_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FunctionRuntimeOverride").getter(getter(FunctionConfiguration::functionRuntimeOverride))
.setter(setter(Builder::functionRuntimeOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FunctionRuntimeOverride").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENCODING_TYPE_FIELD,
ENVIRONMENT_FIELD, EXEC_ARGS_FIELD, EXECUTABLE_FIELD, MEMORY_SIZE_FIELD, PINNED_FIELD, TIMEOUT_FIELD,
FUNCTION_RUNTIME_OVERRIDE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String encodingType;
private final FunctionConfigurationEnvironment environment;
private final String execArgs;
private final String executable;
private final Integer memorySize;
private final Boolean pinned;
private final Integer timeout;
private final String functionRuntimeOverride;
private FunctionConfiguration(BuilderImpl builder) {
this.encodingType = builder.encodingType;
this.environment = builder.environment;
this.execArgs = builder.execArgs;
this.executable = builder.executable;
this.memorySize = builder.memorySize;
this.pinned = builder.pinned;
this.timeout = builder.timeout;
this.functionRuntimeOverride = builder.functionRuntimeOverride;
}
/**
* The expected encoding type of the input payload for the function. The default is ''json''.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encodingType} will
* return {@link EncodingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #encodingTypeAsString}.
*
*
* @return The expected encoding type of the input payload for the function. The default is ''json''.
* @see EncodingType
*/
public final EncodingType encodingType() {
return EncodingType.fromValue(encodingType);
}
/**
* The expected encoding type of the input payload for the function. The default is ''json''.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encodingType} will
* return {@link EncodingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #encodingTypeAsString}.
*
*
* @return The expected encoding type of the input payload for the function. The default is ''json''.
* @see EncodingType
*/
public final String encodingTypeAsString() {
return encodingType;
}
/**
* The environment configuration of the function.
*
* @return The environment configuration of the function.
*/
public final FunctionConfigurationEnvironment environment() {
return environment;
}
/**
* The execution arguments.
*
* @return The execution arguments.
*/
public final String execArgs() {
return execArgs;
}
/**
* The name of the function executable.
*
* @return The name of the function executable.
*/
public final String executable() {
return executable;
}
/**
* The memory size, in KB, which the function requires. This setting is not applicable and should be cleared when
* you run the Lambda function without containerization.
*
* @return The memory size, in KB, which the function requires. This setting is not applicable and should be cleared
* when you run the Lambda function without containerization.
*/
public final Integer memorySize() {
return memorySize;
}
/**
* True if the function is pinned. Pinned means the function is long-lived and starts when the core starts.
*
* @return True if the function is pinned. Pinned means the function is long-lived and starts when the core starts.
*/
public final Boolean pinned() {
return pinned;
}
/**
* The allowed function execution time, after which Lambda should terminate the function. This timeout still applies
* to pinned Lambda functions for each request.
*
* @return The allowed function execution time, after which Lambda should terminate the function. This timeout still
* applies to pinned Lambda functions for each request.
*/
public final Integer timeout() {
return timeout;
}
/**
* The Lambda runtime supported by Greengrass which is to be used instead of the one specified in the Lambda
* function.
*
* @return The Lambda runtime supported by Greengrass which is to be used instead of the one specified in the Lambda
* function.
*/
public final String functionRuntimeOverride() {
return functionRuntimeOverride;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(encodingTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(environment());
hashCode = 31 * hashCode + Objects.hashCode(execArgs());
hashCode = 31 * hashCode + Objects.hashCode(executable());
hashCode = 31 * hashCode + Objects.hashCode(memorySize());
hashCode = 31 * hashCode + Objects.hashCode(pinned());
hashCode = 31 * hashCode + Objects.hashCode(timeout());
hashCode = 31 * hashCode + Objects.hashCode(functionRuntimeOverride());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof FunctionConfiguration)) {
return false;
}
FunctionConfiguration other = (FunctionConfiguration) obj;
return Objects.equals(encodingTypeAsString(), other.encodingTypeAsString())
&& Objects.equals(environment(), other.environment()) && Objects.equals(execArgs(), other.execArgs())
&& Objects.equals(executable(), other.executable()) && Objects.equals(memorySize(), other.memorySize())
&& Objects.equals(pinned(), other.pinned()) && Objects.equals(timeout(), other.timeout())
&& Objects.equals(functionRuntimeOverride(), other.functionRuntimeOverride());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("FunctionConfiguration").add("EncodingType", encodingTypeAsString())
.add("Environment", environment()).add("ExecArgs", execArgs()).add("Executable", executable())
.add("MemorySize", memorySize()).add("Pinned", pinned()).add("Timeout", timeout())
.add("FunctionRuntimeOverride", functionRuntimeOverride()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EncodingType":
return Optional.ofNullable(clazz.cast(encodingTypeAsString()));
case "Environment":
return Optional.ofNullable(clazz.cast(environment()));
case "ExecArgs":
return Optional.ofNullable(clazz.cast(execArgs()));
case "Executable":
return Optional.ofNullable(clazz.cast(executable()));
case "MemorySize":
return Optional.ofNullable(clazz.cast(memorySize()));
case "Pinned":
return Optional.ofNullable(clazz.cast(pinned()));
case "Timeout":
return Optional.ofNullable(clazz.cast(timeout()));
case "FunctionRuntimeOverride":
return Optional.ofNullable(clazz.cast(functionRuntimeOverride()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("EncodingType", ENCODING_TYPE_FIELD);
map.put("Environment", ENVIRONMENT_FIELD);
map.put("ExecArgs", EXEC_ARGS_FIELD);
map.put("Executable", EXECUTABLE_FIELD);
map.put("MemorySize", MEMORY_SIZE_FIELD);
map.put("Pinned", PINNED_FIELD);
map.put("Timeout", TIMEOUT_FIELD);
map.put("FunctionRuntimeOverride", FUNCTION_RUNTIME_OVERRIDE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy