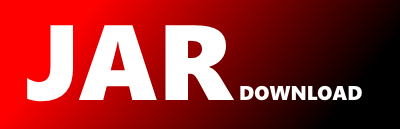
software.amazon.awssdk.services.greengrass.model.GroupVersion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of greengrass Show documentation
Show all versions of greengrass Show documentation
>The AWS Java SDK for AWS Greengrass module holds the client classes that are used for communicating
with AWS Greengrass Service
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.greengrass.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Information about a group version.
*/
@Generated("software.amazon.awssdk:codegen")
public final class GroupVersion implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CONNECTOR_DEFINITION_VERSION_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(GroupVersion::connectorDefinitionVersionArn))
.setter(setter(Builder::connectorDefinitionVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectorDefinitionVersionArn")
.build()).build();
private static final SdkField CORE_DEFINITION_VERSION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GroupVersion::coreDefinitionVersionArn)).setter(setter(Builder::coreDefinitionVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CoreDefinitionVersionArn").build())
.build();
private static final SdkField DEVICE_DEFINITION_VERSION_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(GroupVersion::deviceDefinitionVersionArn))
.setter(setter(Builder::deviceDefinitionVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeviceDefinitionVersionArn").build())
.build();
private static final SdkField FUNCTION_DEFINITION_VERSION_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(GroupVersion::functionDefinitionVersionArn))
.setter(setter(Builder::functionDefinitionVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FunctionDefinitionVersionArn")
.build()).build();
private static final SdkField LOGGER_DEFINITION_VERSION_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(GroupVersion::loggerDefinitionVersionArn))
.setter(setter(Builder::loggerDefinitionVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoggerDefinitionVersionArn").build())
.build();
private static final SdkField RESOURCE_DEFINITION_VERSION_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(GroupVersion::resourceDefinitionVersionArn))
.setter(setter(Builder::resourceDefinitionVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceDefinitionVersionArn")
.build()).build();
private static final SdkField SUBSCRIPTION_DEFINITION_VERSION_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(GroupVersion::subscriptionDefinitionVersionArn))
.setter(setter(Builder::subscriptionDefinitionVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubscriptionDefinitionVersionArn")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
CONNECTOR_DEFINITION_VERSION_ARN_FIELD, CORE_DEFINITION_VERSION_ARN_FIELD, DEVICE_DEFINITION_VERSION_ARN_FIELD,
FUNCTION_DEFINITION_VERSION_ARN_FIELD, LOGGER_DEFINITION_VERSION_ARN_FIELD, RESOURCE_DEFINITION_VERSION_ARN_FIELD,
SUBSCRIPTION_DEFINITION_VERSION_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String connectorDefinitionVersionArn;
private final String coreDefinitionVersionArn;
private final String deviceDefinitionVersionArn;
private final String functionDefinitionVersionArn;
private final String loggerDefinitionVersionArn;
private final String resourceDefinitionVersionArn;
private final String subscriptionDefinitionVersionArn;
private GroupVersion(BuilderImpl builder) {
this.connectorDefinitionVersionArn = builder.connectorDefinitionVersionArn;
this.coreDefinitionVersionArn = builder.coreDefinitionVersionArn;
this.deviceDefinitionVersionArn = builder.deviceDefinitionVersionArn;
this.functionDefinitionVersionArn = builder.functionDefinitionVersionArn;
this.loggerDefinitionVersionArn = builder.loggerDefinitionVersionArn;
this.resourceDefinitionVersionArn = builder.resourceDefinitionVersionArn;
this.subscriptionDefinitionVersionArn = builder.subscriptionDefinitionVersionArn;
}
/**
* The ARN of the connector definition version for this group.
*
* @return The ARN of the connector definition version for this group.
*/
public String connectorDefinitionVersionArn() {
return connectorDefinitionVersionArn;
}
/**
* The ARN of the core definition version for this group.
*
* @return The ARN of the core definition version for this group.
*/
public String coreDefinitionVersionArn() {
return coreDefinitionVersionArn;
}
/**
* The ARN of the device definition version for this group.
*
* @return The ARN of the device definition version for this group.
*/
public String deviceDefinitionVersionArn() {
return deviceDefinitionVersionArn;
}
/**
* The ARN of the function definition version for this group.
*
* @return The ARN of the function definition version for this group.
*/
public String functionDefinitionVersionArn() {
return functionDefinitionVersionArn;
}
/**
* The ARN of the logger definition version for this group.
*
* @return The ARN of the logger definition version for this group.
*/
public String loggerDefinitionVersionArn() {
return loggerDefinitionVersionArn;
}
/**
* The ARN of the resource definition version for this group.
*
* @return The ARN of the resource definition version for this group.
*/
public String resourceDefinitionVersionArn() {
return resourceDefinitionVersionArn;
}
/**
* The ARN of the subscription definition version for this group.
*
* @return The ARN of the subscription definition version for this group.
*/
public String subscriptionDefinitionVersionArn() {
return subscriptionDefinitionVersionArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(connectorDefinitionVersionArn());
hashCode = 31 * hashCode + Objects.hashCode(coreDefinitionVersionArn());
hashCode = 31 * hashCode + Objects.hashCode(deviceDefinitionVersionArn());
hashCode = 31 * hashCode + Objects.hashCode(functionDefinitionVersionArn());
hashCode = 31 * hashCode + Objects.hashCode(loggerDefinitionVersionArn());
hashCode = 31 * hashCode + Objects.hashCode(resourceDefinitionVersionArn());
hashCode = 31 * hashCode + Objects.hashCode(subscriptionDefinitionVersionArn());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GroupVersion)) {
return false;
}
GroupVersion other = (GroupVersion) obj;
return Objects.equals(connectorDefinitionVersionArn(), other.connectorDefinitionVersionArn())
&& Objects.equals(coreDefinitionVersionArn(), other.coreDefinitionVersionArn())
&& Objects.equals(deviceDefinitionVersionArn(), other.deviceDefinitionVersionArn())
&& Objects.equals(functionDefinitionVersionArn(), other.functionDefinitionVersionArn())
&& Objects.equals(loggerDefinitionVersionArn(), other.loggerDefinitionVersionArn())
&& Objects.equals(resourceDefinitionVersionArn(), other.resourceDefinitionVersionArn())
&& Objects.equals(subscriptionDefinitionVersionArn(), other.subscriptionDefinitionVersionArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("GroupVersion").add("ConnectorDefinitionVersionArn", connectorDefinitionVersionArn())
.add("CoreDefinitionVersionArn", coreDefinitionVersionArn())
.add("DeviceDefinitionVersionArn", deviceDefinitionVersionArn())
.add("FunctionDefinitionVersionArn", functionDefinitionVersionArn())
.add("LoggerDefinitionVersionArn", loggerDefinitionVersionArn())
.add("ResourceDefinitionVersionArn", resourceDefinitionVersionArn())
.add("SubscriptionDefinitionVersionArn", subscriptionDefinitionVersionArn()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ConnectorDefinitionVersionArn":
return Optional.ofNullable(clazz.cast(connectorDefinitionVersionArn()));
case "CoreDefinitionVersionArn":
return Optional.ofNullable(clazz.cast(coreDefinitionVersionArn()));
case "DeviceDefinitionVersionArn":
return Optional.ofNullable(clazz.cast(deviceDefinitionVersionArn()));
case "FunctionDefinitionVersionArn":
return Optional.ofNullable(clazz.cast(functionDefinitionVersionArn()));
case "LoggerDefinitionVersionArn":
return Optional.ofNullable(clazz.cast(loggerDefinitionVersionArn()));
case "ResourceDefinitionVersionArn":
return Optional.ofNullable(clazz.cast(resourceDefinitionVersionArn()));
case "SubscriptionDefinitionVersionArn":
return Optional.ofNullable(clazz.cast(subscriptionDefinitionVersionArn()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy