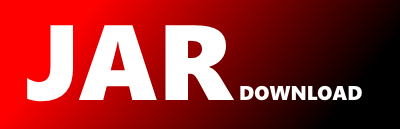
software.amazon.awssdk.services.guardduty.model.Finding Maven / Gradle / Ivy
Show all versions of guardduty Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.guardduty.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about the finding, which is generated when abnormal or suspicious activity is detected.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Finding implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountId").getter(getter(Finding::accountId)).setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("accountId").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(Finding::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField CONFIDENCE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("Confidence").getter(getter(Finding::confidence)).setter(setter(Builder::confidence))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("confidence").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreatedAt").getter(getter(Finding::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(Finding::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(Finding::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField PARTITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Partition").getter(getter(Finding::partition)).setter(setter(Builder::partition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("partition").build()).build();
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Region")
.getter(getter(Finding::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("region").build()).build();
private static final SdkField RESOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Resource").getter(getter(Finding::resource)).setter(setter(Builder::resource))
.constructor(Resource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resource").build()).build();
private static final SdkField SCHEMA_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaVersion").getter(getter(Finding::schemaVersion)).setter(setter(Builder::schemaVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("schemaVersion").build()).build();
private static final SdkField SERVICE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Service").getter(getter(Finding::service)).setter(setter(Builder::service))
.constructor(Service::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("service").build()).build();
private static final SdkField SEVERITY_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("Severity").getter(getter(Finding::severity)).setter(setter(Builder::severity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("severity").build()).build();
private static final SdkField TITLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Title")
.getter(getter(Finding::title)).setter(setter(Builder::title))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("title").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(Finding::type)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpdatedAt").getter(getter(Finding::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNT_ID_FIELD, ARN_FIELD,
CONFIDENCE_FIELD, CREATED_AT_FIELD, DESCRIPTION_FIELD, ID_FIELD, PARTITION_FIELD, REGION_FIELD, RESOURCE_FIELD,
SCHEMA_VERSION_FIELD, SERVICE_FIELD, SEVERITY_FIELD, TITLE_FIELD, TYPE_FIELD, UPDATED_AT_FIELD));
private static final long serialVersionUID = 1L;
private final String accountId;
private final String arn;
private final Double confidence;
private final String createdAt;
private final String description;
private final String id;
private final String partition;
private final String region;
private final Resource resource;
private final String schemaVersion;
private final Service service;
private final Double severity;
private final String title;
private final String type;
private final String updatedAt;
private Finding(BuilderImpl builder) {
this.accountId = builder.accountId;
this.arn = builder.arn;
this.confidence = builder.confidence;
this.createdAt = builder.createdAt;
this.description = builder.description;
this.id = builder.id;
this.partition = builder.partition;
this.region = builder.region;
this.resource = builder.resource;
this.schemaVersion = builder.schemaVersion;
this.service = builder.service;
this.severity = builder.severity;
this.title = builder.title;
this.type = builder.type;
this.updatedAt = builder.updatedAt;
}
/**
*
* The ID of the account in which the finding was generated.
*
*
* @return The ID of the account in which the finding was generated.
*/
public final String accountId() {
return accountId;
}
/**
*
* The ARN of the finding.
*
*
* @return The ARN of the finding.
*/
public final String arn() {
return arn;
}
/**
*
* The confidence score for the finding.
*
*
* @return The confidence score for the finding.
*/
public final Double confidence() {
return confidence;
}
/**
*
* The time and date when the finding was created.
*
*
* @return The time and date when the finding was created.
*/
public final String createdAt() {
return createdAt;
}
/**
*
* The description of the finding.
*
*
* @return The description of the finding.
*/
public final String description() {
return description;
}
/**
*
* The ID of the finding.
*
*
* @return The ID of the finding.
*/
public final String id() {
return id;
}
/**
*
* The partition associated with the finding.
*
*
* @return The partition associated with the finding.
*/
public final String partition() {
return partition;
}
/**
*
* The Region where the finding was generated.
*
*
* @return The Region where the finding was generated.
*/
public final String region() {
return region;
}
/**
* Returns the value of the Resource property for this object.
*
* @return The value of the Resource property for this object.
*/
public final Resource resource() {
return resource;
}
/**
*
* The version of the schema used for the finding.
*
*
* @return The version of the schema used for the finding.
*/
public final String schemaVersion() {
return schemaVersion;
}
/**
* Returns the value of the Service property for this object.
*
* @return The value of the Service property for this object.
*/
public final Service service() {
return service;
}
/**
*
* The severity of the finding.
*
*
* @return The severity of the finding.
*/
public final Double severity() {
return severity;
}
/**
*
* The title of the finding.
*
*
* @return The title of the finding.
*/
public final String title() {
return title;
}
/**
*
* The type of finding.
*
*
* @return The type of finding.
*/
public final String type() {
return type;
}
/**
*
* The time and date when the finding was last updated.
*
*
* @return The time and date when the finding was last updated.
*/
public final String updatedAt() {
return updatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(confidence());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(partition());
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(resource());
hashCode = 31 * hashCode + Objects.hashCode(schemaVersion());
hashCode = 31 * hashCode + Objects.hashCode(service());
hashCode = 31 * hashCode + Objects.hashCode(severity());
hashCode = 31 * hashCode + Objects.hashCode(title());
hashCode = 31 * hashCode + Objects.hashCode(type());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Finding)) {
return false;
}
Finding other = (Finding) obj;
return Objects.equals(accountId(), other.accountId()) && Objects.equals(arn(), other.arn())
&& Objects.equals(confidence(), other.confidence()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(description(), other.description()) && Objects.equals(id(), other.id())
&& Objects.equals(partition(), other.partition()) && Objects.equals(region(), other.region())
&& Objects.equals(resource(), other.resource()) && Objects.equals(schemaVersion(), other.schemaVersion())
&& Objects.equals(service(), other.service()) && Objects.equals(severity(), other.severity())
&& Objects.equals(title(), other.title()) && Objects.equals(type(), other.type())
&& Objects.equals(updatedAt(), other.updatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Finding").add("AccountId", accountId()).add("Arn", arn()).add("Confidence", confidence())
.add("CreatedAt", createdAt()).add("Description", description()).add("Id", id()).add("Partition", partition())
.add("Region", region()).add("Resource", resource()).add("SchemaVersion", schemaVersion())
.add("Service", service()).add("Severity", severity()).add("Title", title()).add("Type", type())
.add("UpdatedAt", updatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AccountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Confidence":
return Optional.ofNullable(clazz.cast(confidence()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Partition":
return Optional.ofNullable(clazz.cast(partition()));
case "Region":
return Optional.ofNullable(clazz.cast(region()));
case "Resource":
return Optional.ofNullable(clazz.cast(resource()));
case "SchemaVersion":
return Optional.ofNullable(clazz.cast(schemaVersion()));
case "Service":
return Optional.ofNullable(clazz.cast(service()));
case "Severity":
return Optional.ofNullable(clazz.cast(severity()));
case "Title":
return Optional.ofNullable(clazz.cast(title()));
case "Type":
return Optional.ofNullable(clazz.cast(type()));
case "UpdatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function