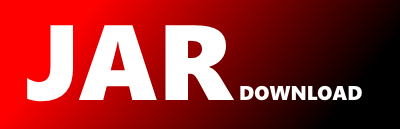
software.amazon.awssdk.services.guardduty.model.RuntimeContext Maven / Gradle / Ivy
Show all versions of guardduty Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.guardduty.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Additional information about the suspicious activity.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RuntimeContext implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField MODIFYING_PROCESS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ModifyingProcess")
.getter(getter(RuntimeContext::modifyingProcess)).setter(setter(Builder::modifyingProcess))
.constructor(ProcessDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("modifyingProcess").build()).build();
private static final SdkField MODIFIED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ModifiedAt").getter(getter(RuntimeContext::modifiedAt)).setter(setter(Builder::modifiedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("modifiedAt").build()).build();
private static final SdkField SCRIPT_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ScriptPath").getter(getter(RuntimeContext::scriptPath)).setter(setter(Builder::scriptPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scriptPath").build()).build();
private static final SdkField LIBRARY_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LibraryPath").getter(getter(RuntimeContext::libraryPath)).setter(setter(Builder::libraryPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("libraryPath").build()).build();
private static final SdkField LD_PRELOAD_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LdPreloadValue").getter(getter(RuntimeContext::ldPreloadValue)).setter(setter(Builder::ldPreloadValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ldPreloadValue").build()).build();
private static final SdkField SOCKET_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SocketPath").getter(getter(RuntimeContext::socketPath)).setter(setter(Builder::socketPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("socketPath").build()).build();
private static final SdkField RUNC_BINARY_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RuncBinaryPath").getter(getter(RuntimeContext::runcBinaryPath)).setter(setter(Builder::runcBinaryPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runcBinaryPath").build()).build();
private static final SdkField RELEASE_AGENT_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReleaseAgentPath").getter(getter(RuntimeContext::releaseAgentPath))
.setter(setter(Builder::releaseAgentPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("releaseAgentPath").build()).build();
private static final SdkField MOUNT_SOURCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MountSource").getter(getter(RuntimeContext::mountSource)).setter(setter(Builder::mountSource))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("mountSource").build()).build();
private static final SdkField MOUNT_TARGET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MountTarget").getter(getter(RuntimeContext::mountTarget)).setter(setter(Builder::mountTarget))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("mountTarget").build()).build();
private static final SdkField FILE_SYSTEM_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FileSystemType").getter(getter(RuntimeContext::fileSystemType)).setter(setter(Builder::fileSystemType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fileSystemType").build()).build();
private static final SdkField> FLAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Flags")
.getter(getter(RuntimeContext::flags))
.setter(setter(Builder::flags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("flags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField MODULE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModuleName").getter(getter(RuntimeContext::moduleName)).setter(setter(Builder::moduleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("moduleName").build()).build();
private static final SdkField MODULE_FILE_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModuleFilePath").getter(getter(RuntimeContext::moduleFilePath)).setter(setter(Builder::moduleFilePath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("moduleFilePath").build()).build();
private static final SdkField MODULE_SHA256_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModuleSha256").getter(getter(RuntimeContext::moduleSha256)).setter(setter(Builder::moduleSha256))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("moduleSha256").build()).build();
private static final SdkField SHELL_HISTORY_FILE_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ShellHistoryFilePath").getter(getter(RuntimeContext::shellHistoryFilePath))
.setter(setter(Builder::shellHistoryFilePath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("shellHistoryFilePath").build())
.build();
private static final SdkField TARGET_PROCESS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TargetProcess")
.getter(getter(RuntimeContext::targetProcess)).setter(setter(Builder::targetProcess))
.constructor(ProcessDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetProcess").build()).build();
private static final SdkField ADDRESS_FAMILY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AddressFamily").getter(getter(RuntimeContext::addressFamily)).setter(setter(Builder::addressFamily))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("addressFamily").build()).build();
private static final SdkField IANA_PROTOCOL_NUMBER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("IanaProtocolNumber").getter(getter(RuntimeContext::ianaProtocolNumber))
.setter(setter(Builder::ianaProtocolNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ianaProtocolNumber").build())
.build();
private static final SdkField> MEMORY_REGIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MemoryRegions")
.getter(getter(RuntimeContext::memoryRegions))
.setter(setter(Builder::memoryRegions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memoryRegions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TOOL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ToolName").getter(getter(RuntimeContext::toolName)).setter(setter(Builder::toolName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("toolName").build()).build();
private static final SdkField TOOL_CATEGORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ToolCategory").getter(getter(RuntimeContext::toolCategory)).setter(setter(Builder::toolCategory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("toolCategory").build()).build();
private static final SdkField SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceName").getter(getter(RuntimeContext::serviceName)).setter(setter(Builder::serviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceName").build()).build();
private static final SdkField COMMAND_LINE_EXAMPLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CommandLineExample").getter(getter(RuntimeContext::commandLineExample))
.setter(setter(Builder::commandLineExample))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("commandLineExample").build())
.build();
private static final SdkField THREAT_FILE_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ThreatFilePath").getter(getter(RuntimeContext::threatFilePath)).setter(setter(Builder::threatFilePath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("threatFilePath").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MODIFYING_PROCESS_FIELD,
MODIFIED_AT_FIELD, SCRIPT_PATH_FIELD, LIBRARY_PATH_FIELD, LD_PRELOAD_VALUE_FIELD, SOCKET_PATH_FIELD,
RUNC_BINARY_PATH_FIELD, RELEASE_AGENT_PATH_FIELD, MOUNT_SOURCE_FIELD, MOUNT_TARGET_FIELD, FILE_SYSTEM_TYPE_FIELD,
FLAGS_FIELD, MODULE_NAME_FIELD, MODULE_FILE_PATH_FIELD, MODULE_SHA256_FIELD, SHELL_HISTORY_FILE_PATH_FIELD,
TARGET_PROCESS_FIELD, ADDRESS_FAMILY_FIELD, IANA_PROTOCOL_NUMBER_FIELD, MEMORY_REGIONS_FIELD, TOOL_NAME_FIELD,
TOOL_CATEGORY_FIELD, SERVICE_NAME_FIELD, COMMAND_LINE_EXAMPLE_FIELD, THREAT_FILE_PATH_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final ProcessDetails modifyingProcess;
private final Instant modifiedAt;
private final String scriptPath;
private final String libraryPath;
private final String ldPreloadValue;
private final String socketPath;
private final String runcBinaryPath;
private final String releaseAgentPath;
private final String mountSource;
private final String mountTarget;
private final String fileSystemType;
private final List flags;
private final String moduleName;
private final String moduleFilePath;
private final String moduleSha256;
private final String shellHistoryFilePath;
private final ProcessDetails targetProcess;
private final String addressFamily;
private final Integer ianaProtocolNumber;
private final List memoryRegions;
private final String toolName;
private final String toolCategory;
private final String serviceName;
private final String commandLineExample;
private final String threatFilePath;
private RuntimeContext(BuilderImpl builder) {
this.modifyingProcess = builder.modifyingProcess;
this.modifiedAt = builder.modifiedAt;
this.scriptPath = builder.scriptPath;
this.libraryPath = builder.libraryPath;
this.ldPreloadValue = builder.ldPreloadValue;
this.socketPath = builder.socketPath;
this.runcBinaryPath = builder.runcBinaryPath;
this.releaseAgentPath = builder.releaseAgentPath;
this.mountSource = builder.mountSource;
this.mountTarget = builder.mountTarget;
this.fileSystemType = builder.fileSystemType;
this.flags = builder.flags;
this.moduleName = builder.moduleName;
this.moduleFilePath = builder.moduleFilePath;
this.moduleSha256 = builder.moduleSha256;
this.shellHistoryFilePath = builder.shellHistoryFilePath;
this.targetProcess = builder.targetProcess;
this.addressFamily = builder.addressFamily;
this.ianaProtocolNumber = builder.ianaProtocolNumber;
this.memoryRegions = builder.memoryRegions;
this.toolName = builder.toolName;
this.toolCategory = builder.toolCategory;
this.serviceName = builder.serviceName;
this.commandLineExample = builder.commandLineExample;
this.threatFilePath = builder.threatFilePath;
}
/**
*
* Information about the process that modified the current process. This is available for multiple finding types.
*
*
* @return Information about the process that modified the current process. This is available for multiple finding
* types.
*/
public final ProcessDetails modifyingProcess() {
return modifyingProcess;
}
/**
*
* The timestamp at which the process modified the current process. The timestamp is in UTC date string format.
*
*
* @return The timestamp at which the process modified the current process. The timestamp is in UTC date string
* format.
*/
public final Instant modifiedAt() {
return modifiedAt;
}
/**
*
* The path to the script that was executed.
*
*
* @return The path to the script that was executed.
*/
public final String scriptPath() {
return scriptPath;
}
/**
*
* The path to the new library that was loaded.
*
*
* @return The path to the new library that was loaded.
*/
public final String libraryPath() {
return libraryPath;
}
/**
*
* The value of the LD_PRELOAD environment variable.
*
*
* @return The value of the LD_PRELOAD environment variable.
*/
public final String ldPreloadValue() {
return ldPreloadValue;
}
/**
*
* The path to the docket socket that was accessed.
*
*
* @return The path to the docket socket that was accessed.
*/
public final String socketPath() {
return socketPath;
}
/**
*
* The path to the leveraged runc
implementation.
*
*
* @return The path to the leveraged runc
implementation.
*/
public final String runcBinaryPath() {
return runcBinaryPath;
}
/**
*
* The path in the container that modified the release agent file.
*
*
* @return The path in the container that modified the release agent file.
*/
public final String releaseAgentPath() {
return releaseAgentPath;
}
/**
*
* The path on the host that is mounted by the container.
*
*
* @return The path on the host that is mounted by the container.
*/
public final String mountSource() {
return mountSource;
}
/**
*
* The path in the container that is mapped to the host directory.
*
*
* @return The path in the container that is mapped to the host directory.
*/
public final String mountTarget() {
return mountTarget;
}
/**
*
* Represents the type of mounted fileSystem.
*
*
* @return Represents the type of mounted fileSystem.
*/
public final String fileSystemType() {
return fileSystemType;
}
/**
* For responses, this returns true if the service returned a value for the Flags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasFlags() {
return flags != null && !(flags instanceof SdkAutoConstructList);
}
/**
*
* Represents options that control the behavior of a runtime operation or action. For example, a filesystem mount
* operation may contain a read-only flag.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFlags} method.
*
*
* @return Represents options that control the behavior of a runtime operation or action. For example, a filesystem
* mount operation may contain a read-only flag.
*/
public final List flags() {
return flags;
}
/**
*
* The name of the module loaded into the kernel.
*
*
* @return The name of the module loaded into the kernel.
*/
public final String moduleName() {
return moduleName;
}
/**
*
* The path to the module loaded into the kernel.
*
*
* @return The path to the module loaded into the kernel.
*/
public final String moduleFilePath() {
return moduleFilePath;
}
/**
*
* The SHA256
hash of the module.
*
*
* @return The SHA256
hash of the module.
*/
public final String moduleSha256() {
return moduleSha256;
}
/**
*
* The path to the modified shell history file.
*
*
* @return The path to the modified shell history file.
*/
public final String shellHistoryFilePath() {
return shellHistoryFilePath;
}
/**
*
* Information about the process that had its memory overwritten by the current process.
*
*
* @return Information about the process that had its memory overwritten by the current process.
*/
public final ProcessDetails targetProcess() {
return targetProcess;
}
/**
*
* Represents the communication protocol associated with the address. For example, the address family
* AF_INET
is used for IP version of 4 protocol.
*
*
* @return Represents the communication protocol associated with the address. For example, the address family
* AF_INET
is used for IP version of 4 protocol.
*/
public final String addressFamily() {
return addressFamily;
}
/**
*
* Specifies a particular protocol within the address family. Usually there is a single protocol in address
* families. For example, the address family AF_INET
only has the IP protocol.
*
*
* @return Specifies a particular protocol within the address family. Usually there is a single protocol in address
* families. For example, the address family AF_INET
only has the IP protocol.
*/
public final Integer ianaProtocolNumber() {
return ianaProtocolNumber;
}
/**
* For responses, this returns true if the service returned a value for the MemoryRegions property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMemoryRegions() {
return memoryRegions != null && !(memoryRegions instanceof SdkAutoConstructList);
}
/**
*
* Specifies the Region of a process's address space such as stack and heap.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMemoryRegions} method.
*
*
* @return Specifies the Region of a process's address space such as stack and heap.
*/
public final List memoryRegions() {
return memoryRegions;
}
/**
*
* Name of the potentially suspicious tool.
*
*
* @return Name of the potentially suspicious tool.
*/
public final String toolName() {
return toolName;
}
/**
*
* Category that the tool belongs to. Some of the examples are Backdoor Tool, Pentest Tool, Network Scanner, and
* Network Sniffer.
*
*
* @return Category that the tool belongs to. Some of the examples are Backdoor Tool, Pentest Tool, Network Scanner,
* and Network Sniffer.
*/
public final String toolCategory() {
return toolCategory;
}
/**
*
* Name of the security service that has been potentially disabled.
*
*
* @return Name of the security service that has been potentially disabled.
*/
public final String serviceName() {
return serviceName;
}
/**
*
* Example of the command line involved in the suspicious activity.
*
*
* @return Example of the command line involved in the suspicious activity.
*/
public final String commandLineExample() {
return commandLineExample;
}
/**
*
* The suspicious file path for which the threat intelligence details were found.
*
*
* @return The suspicious file path for which the threat intelligence details were found.
*/
public final String threatFilePath() {
return threatFilePath;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(modifyingProcess());
hashCode = 31 * hashCode + Objects.hashCode(modifiedAt());
hashCode = 31 * hashCode + Objects.hashCode(scriptPath());
hashCode = 31 * hashCode + Objects.hashCode(libraryPath());
hashCode = 31 * hashCode + Objects.hashCode(ldPreloadValue());
hashCode = 31 * hashCode + Objects.hashCode(socketPath());
hashCode = 31 * hashCode + Objects.hashCode(runcBinaryPath());
hashCode = 31 * hashCode + Objects.hashCode(releaseAgentPath());
hashCode = 31 * hashCode + Objects.hashCode(mountSource());
hashCode = 31 * hashCode + Objects.hashCode(mountTarget());
hashCode = 31 * hashCode + Objects.hashCode(fileSystemType());
hashCode = 31 * hashCode + Objects.hashCode(hasFlags() ? flags() : null);
hashCode = 31 * hashCode + Objects.hashCode(moduleName());
hashCode = 31 * hashCode + Objects.hashCode(moduleFilePath());
hashCode = 31 * hashCode + Objects.hashCode(moduleSha256());
hashCode = 31 * hashCode + Objects.hashCode(shellHistoryFilePath());
hashCode = 31 * hashCode + Objects.hashCode(targetProcess());
hashCode = 31 * hashCode + Objects.hashCode(addressFamily());
hashCode = 31 * hashCode + Objects.hashCode(ianaProtocolNumber());
hashCode = 31 * hashCode + Objects.hashCode(hasMemoryRegions() ? memoryRegions() : null);
hashCode = 31 * hashCode + Objects.hashCode(toolName());
hashCode = 31 * hashCode + Objects.hashCode(toolCategory());
hashCode = 31 * hashCode + Objects.hashCode(serviceName());
hashCode = 31 * hashCode + Objects.hashCode(commandLineExample());
hashCode = 31 * hashCode + Objects.hashCode(threatFilePath());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RuntimeContext)) {
return false;
}
RuntimeContext other = (RuntimeContext) obj;
return Objects.equals(modifyingProcess(), other.modifyingProcess()) && Objects.equals(modifiedAt(), other.modifiedAt())
&& Objects.equals(scriptPath(), other.scriptPath()) && Objects.equals(libraryPath(), other.libraryPath())
&& Objects.equals(ldPreloadValue(), other.ldPreloadValue()) && Objects.equals(socketPath(), other.socketPath())
&& Objects.equals(runcBinaryPath(), other.runcBinaryPath())
&& Objects.equals(releaseAgentPath(), other.releaseAgentPath())
&& Objects.equals(mountSource(), other.mountSource()) && Objects.equals(mountTarget(), other.mountTarget())
&& Objects.equals(fileSystemType(), other.fileSystemType()) && hasFlags() == other.hasFlags()
&& Objects.equals(flags(), other.flags()) && Objects.equals(moduleName(), other.moduleName())
&& Objects.equals(moduleFilePath(), other.moduleFilePath())
&& Objects.equals(moduleSha256(), other.moduleSha256())
&& Objects.equals(shellHistoryFilePath(), other.shellHistoryFilePath())
&& Objects.equals(targetProcess(), other.targetProcess())
&& Objects.equals(addressFamily(), other.addressFamily())
&& Objects.equals(ianaProtocolNumber(), other.ianaProtocolNumber())
&& hasMemoryRegions() == other.hasMemoryRegions() && Objects.equals(memoryRegions(), other.memoryRegions())
&& Objects.equals(toolName(), other.toolName()) && Objects.equals(toolCategory(), other.toolCategory())
&& Objects.equals(serviceName(), other.serviceName())
&& Objects.equals(commandLineExample(), other.commandLineExample())
&& Objects.equals(threatFilePath(), other.threatFilePath());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RuntimeContext").add("ModifyingProcess", modifyingProcess()).add("ModifiedAt", modifiedAt())
.add("ScriptPath", scriptPath()).add("LibraryPath", libraryPath()).add("LdPreloadValue", ldPreloadValue())
.add("SocketPath", socketPath()).add("RuncBinaryPath", runcBinaryPath())
.add("ReleaseAgentPath", releaseAgentPath()).add("MountSource", mountSource()).add("MountTarget", mountTarget())
.add("FileSystemType", fileSystemType()).add("Flags", hasFlags() ? flags() : null)
.add("ModuleName", moduleName()).add("ModuleFilePath", moduleFilePath()).add("ModuleSha256", moduleSha256())
.add("ShellHistoryFilePath", shellHistoryFilePath()).add("TargetProcess", targetProcess())
.add("AddressFamily", addressFamily()).add("IanaProtocolNumber", ianaProtocolNumber())
.add("MemoryRegions", hasMemoryRegions() ? memoryRegions() : null).add("ToolName", toolName())
.add("ToolCategory", toolCategory()).add("ServiceName", serviceName())
.add("CommandLineExample", commandLineExample()).add("ThreatFilePath", threatFilePath()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ModifyingProcess":
return Optional.ofNullable(clazz.cast(modifyingProcess()));
case "ModifiedAt":
return Optional.ofNullable(clazz.cast(modifiedAt()));
case "ScriptPath":
return Optional.ofNullable(clazz.cast(scriptPath()));
case "LibraryPath":
return Optional.ofNullable(clazz.cast(libraryPath()));
case "LdPreloadValue":
return Optional.ofNullable(clazz.cast(ldPreloadValue()));
case "SocketPath":
return Optional.ofNullable(clazz.cast(socketPath()));
case "RuncBinaryPath":
return Optional.ofNullable(clazz.cast(runcBinaryPath()));
case "ReleaseAgentPath":
return Optional.ofNullable(clazz.cast(releaseAgentPath()));
case "MountSource":
return Optional.ofNullable(clazz.cast(mountSource()));
case "MountTarget":
return Optional.ofNullable(clazz.cast(mountTarget()));
case "FileSystemType":
return Optional.ofNullable(clazz.cast(fileSystemType()));
case "Flags":
return Optional.ofNullable(clazz.cast(flags()));
case "ModuleName":
return Optional.ofNullable(clazz.cast(moduleName()));
case "ModuleFilePath":
return Optional.ofNullable(clazz.cast(moduleFilePath()));
case "ModuleSha256":
return Optional.ofNullable(clazz.cast(moduleSha256()));
case "ShellHistoryFilePath":
return Optional.ofNullable(clazz.cast(shellHistoryFilePath()));
case "TargetProcess":
return Optional.ofNullable(clazz.cast(targetProcess()));
case "AddressFamily":
return Optional.ofNullable(clazz.cast(addressFamily()));
case "IanaProtocolNumber":
return Optional.ofNullable(clazz.cast(ianaProtocolNumber()));
case "MemoryRegions":
return Optional.ofNullable(clazz.cast(memoryRegions()));
case "ToolName":
return Optional.ofNullable(clazz.cast(toolName()));
case "ToolCategory":
return Optional.ofNullable(clazz.cast(toolCategory()));
case "ServiceName":
return Optional.ofNullable(clazz.cast(serviceName()));
case "CommandLineExample":
return Optional.ofNullable(clazz.cast(commandLineExample()));
case "ThreatFilePath":
return Optional.ofNullable(clazz.cast(threatFilePath()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("modifyingProcess", MODIFYING_PROCESS_FIELD);
map.put("modifiedAt", MODIFIED_AT_FIELD);
map.put("scriptPath", SCRIPT_PATH_FIELD);
map.put("libraryPath", LIBRARY_PATH_FIELD);
map.put("ldPreloadValue", LD_PRELOAD_VALUE_FIELD);
map.put("socketPath", SOCKET_PATH_FIELD);
map.put("runcBinaryPath", RUNC_BINARY_PATH_FIELD);
map.put("releaseAgentPath", RELEASE_AGENT_PATH_FIELD);
map.put("mountSource", MOUNT_SOURCE_FIELD);
map.put("mountTarget", MOUNT_TARGET_FIELD);
map.put("fileSystemType", FILE_SYSTEM_TYPE_FIELD);
map.put("flags", FLAGS_FIELD);
map.put("moduleName", MODULE_NAME_FIELD);
map.put("moduleFilePath", MODULE_FILE_PATH_FIELD);
map.put("moduleSha256", MODULE_SHA256_FIELD);
map.put("shellHistoryFilePath", SHELL_HISTORY_FILE_PATH_FIELD);
map.put("targetProcess", TARGET_PROCESS_FIELD);
map.put("addressFamily", ADDRESS_FAMILY_FIELD);
map.put("ianaProtocolNumber", IANA_PROTOCOL_NUMBER_FIELD);
map.put("memoryRegions", MEMORY_REGIONS_FIELD);
map.put("toolName", TOOL_NAME_FIELD);
map.put("toolCategory", TOOL_CATEGORY_FIELD);
map.put("serviceName", SERVICE_NAME_FIELD);
map.put("commandLineExample", COMMAND_LINE_EXAMPLE_FIELD);
map.put("threatFilePath", THREAT_FILE_PATH_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function