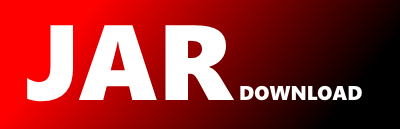
software.amazon.awssdk.services.guardduty.model.Session Maven / Gradle / Ivy
Show all versions of guardduty Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.guardduty.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about the authenticated session.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Session implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField UID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Uid")
.getter(getter(Session::uid)).setter(setter(Builder::uid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("uid").build()).build();
private static final SdkField MFA_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MfaStatus").getter(getter(Session::mfaStatusAsString)).setter(setter(Builder::mfaStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("mfaStatus").build()).build();
private static final SdkField CREATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedTime").getter(getter(Session::createdTime)).setter(setter(Builder::createdTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdTime").build()).build();
private static final SdkField ISSUER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Issuer")
.getter(getter(Session::issuer)).setter(setter(Builder::issuer))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("issuer").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UID_FIELD, MFA_STATUS_FIELD,
CREATED_TIME_FIELD, ISSUER_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String uid;
private final String mfaStatus;
private final Instant createdTime;
private final String issuer;
private Session(BuilderImpl builder) {
this.uid = builder.uid;
this.mfaStatus = builder.mfaStatus;
this.createdTime = builder.createdTime;
this.issuer = builder.issuer;
}
/**
*
* The unique identifier of the session.
*
*
* @return The unique identifier of the session.
*/
public final String uid() {
return uid;
}
/**
*
* Indicates whether or not multi-factor authencation (MFA) was used during authentication.
*
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.mfaAuthenticated
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mfaStatus} will
* return {@link MfaStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mfaStatusAsString}.
*
*
* @return Indicates whether or not multi-factor authencation (MFA) was used during authentication.
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.mfaAuthenticated
.
* @see MfaStatus
*/
public final MfaStatus mfaStatus() {
return MfaStatus.fromValue(mfaStatus);
}
/**
*
* Indicates whether or not multi-factor authencation (MFA) was used during authentication.
*
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.mfaAuthenticated
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mfaStatus} will
* return {@link MfaStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mfaStatusAsString}.
*
*
* @return Indicates whether or not multi-factor authencation (MFA) was used during authentication.
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.mfaAuthenticated
.
* @see MfaStatus
*/
public final String mfaStatusAsString() {
return mfaStatus;
}
/**
*
* The timestamp for when the session was created.
*
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.creationDate
.
*
*
* @return The timestamp for when the session was created.
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.creationDate
.
*/
public final Instant createdTime() {
return createdTime;
}
/**
*
* Identifier of the session issuer.
*
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.sessionIssuer.arn
.
*
*
* @return Identifier of the session issuer.
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.sessionIssuer.arn
.
*/
public final String issuer() {
return issuer;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(uid());
hashCode = 31 * hashCode + Objects.hashCode(mfaStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(createdTime());
hashCode = 31 * hashCode + Objects.hashCode(issuer());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Session)) {
return false;
}
Session other = (Session) obj;
return Objects.equals(uid(), other.uid()) && Objects.equals(mfaStatusAsString(), other.mfaStatusAsString())
&& Objects.equals(createdTime(), other.createdTime()) && Objects.equals(issuer(), other.issuer());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Session").add("Uid", uid()).add("MfaStatus", mfaStatusAsString())
.add("CreatedTime", createdTime()).add("Issuer", issuer()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Uid":
return Optional.ofNullable(clazz.cast(uid()));
case "MfaStatus":
return Optional.ofNullable(clazz.cast(mfaStatusAsString()));
case "CreatedTime":
return Optional.ofNullable(clazz.cast(createdTime()));
case "Issuer":
return Optional.ofNullable(clazz.cast(issuer()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("uid", UID_FIELD);
map.put("mfaStatus", MFA_STATUS_FIELD);
map.put("createdTime", CREATED_TIME_FIELD);
map.put("issuer", ISSUER_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.mfaAuthenticated
.
* @see MfaStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see MfaStatus
*/
Builder mfaStatus(String mfaStatus);
/**
*
* Indicates whether or not multi-factor authencation (MFA) was used during authentication.
*
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.mfaAuthenticated
.
*
*
* @param mfaStatus
* Indicates whether or not multi-factor authencation (MFA) was used during authentication.
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.mfaAuthenticated
.
* @see MfaStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see MfaStatus
*/
Builder mfaStatus(MfaStatus mfaStatus);
/**
*
* The timestamp for when the session was created.
*
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.creationDate
.
*
*
* @param createdTime
* The timestamp for when the session was created.
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.attributes.creationDate
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder createdTime(Instant createdTime);
/**
*
* Identifier of the session issuer.
*
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.sessionIssuer.arn
.
*
*
* @param issuer
* Identifier of the session issuer.
*
* In Amazon Web Services CloudTrail, you can find this value as
* userIdentity.sessionContext.sessionIssuer.arn
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder issuer(String issuer);
}
static final class BuilderImpl implements Builder {
private String uid;
private String mfaStatus;
private Instant createdTime;
private String issuer;
private BuilderImpl() {
}
private BuilderImpl(Session model) {
uid(model.uid);
mfaStatus(model.mfaStatus);
createdTime(model.createdTime);
issuer(model.issuer);
}
public final String getUid() {
return uid;
}
public final void setUid(String uid) {
this.uid = uid;
}
@Override
public final Builder uid(String uid) {
this.uid = uid;
return this;
}
public final String getMfaStatus() {
return mfaStatus;
}
public final void setMfaStatus(String mfaStatus) {
this.mfaStatus = mfaStatus;
}
@Override
public final Builder mfaStatus(String mfaStatus) {
this.mfaStatus = mfaStatus;
return this;
}
@Override
public final Builder mfaStatus(MfaStatus mfaStatus) {
this.mfaStatus(mfaStatus == null ? null : mfaStatus.toString());
return this;
}
public final Instant getCreatedTime() {
return createdTime;
}
public final void setCreatedTime(Instant createdTime) {
this.createdTime = createdTime;
}
@Override
public final Builder createdTime(Instant createdTime) {
this.createdTime = createdTime;
return this;
}
public final String getIssuer() {
return issuer;
}
public final void setIssuer(String issuer) {
this.issuer = issuer;
}
@Override
public final Builder issuer(String issuer) {
this.issuer = issuer;
return this;
}
@Override
public Session build() {
return new Session(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}