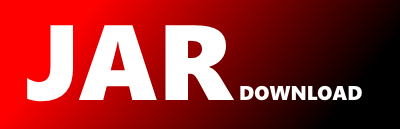
software.amazon.awssdk.services.iam.model.SimulateCustomPolicyRequest Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class SimulateCustomPolicyRequest extends IamRequest implements
ToCopyableBuilder {
private static final SdkField> POLICY_INPUT_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(SimulateCustomPolicyRequest::policyInputList))
.setter(setter(Builder::policyInputList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyInputList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PERMISSIONS_BOUNDARY_POLICY_INPUT_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(SimulateCustomPolicyRequest::permissionsBoundaryPolicyInputList))
.setter(setter(Builder::permissionsBoundaryPolicyInputList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PermissionsBoundaryPolicyInputList")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ACTION_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(SimulateCustomPolicyRequest::actionNames))
.setter(setter(Builder::actionNames))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionNames").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> RESOURCE_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(SimulateCustomPolicyRequest::resourceArns))
.setter(setter(Builder::resourceArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RESOURCE_POLICY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SimulateCustomPolicyRequest::resourcePolicy)).setter(setter(Builder::resourcePolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourcePolicy").build()).build();
private static final SdkField RESOURCE_OWNER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SimulateCustomPolicyRequest::resourceOwner)).setter(setter(Builder::resourceOwner))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceOwner").build()).build();
private static final SdkField CALLER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SimulateCustomPolicyRequest::callerArn)).setter(setter(Builder::callerArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CallerArn").build()).build();
private static final SdkField> CONTEXT_ENTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(SimulateCustomPolicyRequest::contextEntries))
.setter(setter(Builder::contextEntries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContextEntries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ContextEntry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RESOURCE_HANDLING_OPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SimulateCustomPolicyRequest::resourceHandlingOption)).setter(setter(Builder::resourceHandlingOption))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceHandlingOption").build())
.build();
private static final SdkField MAX_ITEMS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(SimulateCustomPolicyRequest::maxItems)).setter(setter(Builder::maxItems))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxItems").build()).build();
private static final SdkField MARKER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SimulateCustomPolicyRequest::marker)).setter(setter(Builder::marker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Marker").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(POLICY_INPUT_LIST_FIELD,
PERMISSIONS_BOUNDARY_POLICY_INPUT_LIST_FIELD, ACTION_NAMES_FIELD, RESOURCE_ARNS_FIELD, RESOURCE_POLICY_FIELD,
RESOURCE_OWNER_FIELD, CALLER_ARN_FIELD, CONTEXT_ENTRIES_FIELD, RESOURCE_HANDLING_OPTION_FIELD, MAX_ITEMS_FIELD,
MARKER_FIELD));
private final List policyInputList;
private final List permissionsBoundaryPolicyInputList;
private final List actionNames;
private final List resourceArns;
private final String resourcePolicy;
private final String resourceOwner;
private final String callerArn;
private final List contextEntries;
private final String resourceHandlingOption;
private final Integer maxItems;
private final String marker;
private SimulateCustomPolicyRequest(BuilderImpl builder) {
super(builder);
this.policyInputList = builder.policyInputList;
this.permissionsBoundaryPolicyInputList = builder.permissionsBoundaryPolicyInputList;
this.actionNames = builder.actionNames;
this.resourceArns = builder.resourceArns;
this.resourcePolicy = builder.resourcePolicy;
this.resourceOwner = builder.resourceOwner;
this.callerArn = builder.callerArn;
this.contextEntries = builder.contextEntries;
this.resourceHandlingOption = builder.resourceHandlingOption;
this.maxItems = builder.maxItems;
this.marker = builder.marker;
}
/**
* Returns true if the PolicyInputList property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasPolicyInputList() {
return policyInputList != null && !(policyInputList instanceof SdkAutoConstructList);
}
/**
*
* A list of policy documents to include in the simulation. Each document is specified as a string containing the
* complete, valid JSON text of an IAM policy. Do not include any resource-based policies in this parameter. Any
* resource-based policy must be submitted with the ResourcePolicy
parameter. The policies cannot be
* "scope-down" policies, such as you could include in a call to GetFederationToken or
* one of the AssumeRole API
* operations. In other words, do not use policies designed to restrict what a user can do while using the temporary
* credentials.
*
*
* The regex pattern used to validate this parameter is a string of
* characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of the ASCII
* character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (\u000D
* )
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasPolicyInputList()} to see if a value was sent in this field.
*
*
* @return A list of policy documents to include in the simulation. Each document is specified as a string
* containing the complete, valid JSON text of an IAM policy. Do not include any resource-based policies in
* this parameter. Any resource-based policy must be submitted with the ResourcePolicy
* parameter. The policies cannot be "scope-down" policies, such as you could include in a call to GetFederationToken or one of the AssumeRole API
* operations. In other words, do not use policies designed to restrict what a user can do while using the
* temporary credentials.
*
* The regex pattern used to validate this parameter is a
* string of characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of
* the ASCII character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through
* \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (
* \u000D
)
*
*
*/
public List policyInputList() {
return policyInputList;
}
/**
* Returns true if the PermissionsBoundaryPolicyInputList property was specified by the sender (it may be empty), or
* false if the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender
* is the AWS service.
*/
public boolean hasPermissionsBoundaryPolicyInputList() {
return permissionsBoundaryPolicyInputList != null
&& !(permissionsBoundaryPolicyInputList instanceof SdkAutoConstructList);
}
/**
*
* The IAM permissions boundary policy to simulate. The permissions boundary sets the maximum permissions that an
* IAM entity can have. You can input only one permissions boundary when you pass a policy to this operation. For
* more information about permissions boundaries, see Permissions Boundaries
* for IAM Entities in the IAM User Guide. The policy input is specified as a string that contains the
* complete, valid JSON text of a permissions boundary policy.
*
*
* The regex pattern used to validate this parameter is a string of
* characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of the ASCII
* character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (\u000D
* )
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasPermissionsBoundaryPolicyInputList()} to see if a value was sent in this field.
*
*
* @return The IAM permissions boundary policy to simulate. The permissions boundary sets the maximum permissions
* that an IAM entity can have. You can input only one permissions boundary when you pass a policy to this
* operation. For more information about permissions boundaries, see Permissions
* Boundaries for IAM Entities in the IAM User Guide. The policy input is specified as a string
* that contains the complete, valid JSON text of a permissions boundary policy.
*
* The regex pattern used to validate this parameter is a
* string of characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of
* the ASCII character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through
* \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (
* \u000D
)
*
*
*/
public List permissionsBoundaryPolicyInputList() {
return permissionsBoundaryPolicyInputList;
}
/**
* Returns true if the ActionNames property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasActionNames() {
return actionNames != null && !(actionNames instanceof SdkAutoConstructList);
}
/**
*
* A list of names of API operations to evaluate in the simulation. Each operation is evaluated against each
* resource. Each operation must include the service identifier, such as iam:CreateUser
. This operation
* does not support using wildcards (*) in an action name.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasActionNames()} to see if a value was sent in this field.
*
*
* @return A list of names of API operations to evaluate in the simulation. Each operation is evaluated against each
* resource. Each operation must include the service identifier, such as iam:CreateUser
. This
* operation does not support using wildcards (*) in an action name.
*/
public List actionNames() {
return actionNames;
}
/**
* Returns true if the ResourceArns property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasResourceArns() {
return resourceArns != null && !(resourceArns instanceof SdkAutoConstructList);
}
/**
*
* A list of ARNs of AWS resources to include in the simulation. If this parameter is not provided, then the value
* defaults to *
(all resources). Each API in the ActionNames
parameter is evaluated for
* each resource in this list. The simulation determines the access result (allowed or denied) of each combination
* and reports it in the response.
*
*
* The simulation does not automatically retrieve policies for the specified resources. If you want to include a
* resource policy in the simulation, then you must include the policy as a string in the
* ResourcePolicy
parameter.
*
*
* If you include a ResourcePolicy
, then it must be applicable to all of the resources included in the
* simulation or you receive an invalid input error.
*
*
* For more information about ARNs, see Amazon Resource Names (ARNs)
* and AWS Service Namespaces in the AWS General Reference.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasResourceArns()} to see if a value was sent in this field.
*
*
* @return A list of ARNs of AWS resources to include in the simulation. If this parameter is not provided, then the
* value defaults to *
(all resources). Each API in the ActionNames
parameter is
* evaluated for each resource in this list. The simulation determines the access result (allowed or denied)
* of each combination and reports it in the response.
*
* The simulation does not automatically retrieve policies for the specified resources. If you want to
* include a resource policy in the simulation, then you must include the policy as a string in the
* ResourcePolicy
parameter.
*
*
* If you include a ResourcePolicy
, then it must be applicable to all of the resources included
* in the simulation or you receive an invalid input error.
*
*
* For more information about ARNs, see Amazon Resource Names
* (ARNs) and AWS Service Namespaces in the AWS General Reference.
*/
public List resourceArns() {
return resourceArns;
}
/**
*
* A resource-based policy to include in the simulation provided as a string. Each resource in the simulation is
* treated as if it had this policy attached. You can include only one resource-based policy in a simulation.
*
*
* The regex pattern used to validate this parameter is a string of
* characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of the ASCII
* character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (\u000D
* )
*
*
*
*
* @return A resource-based policy to include in the simulation provided as a string. Each resource in the
* simulation is treated as if it had this policy attached. You can include only one resource-based policy
* in a simulation.
*
* The regex pattern used to validate this parameter is a
* string of characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of
* the ASCII character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through
* \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (
* \u000D
)
*
*
*/
public String resourcePolicy() {
return resourcePolicy;
}
/**
*
* An ARN representing the AWS account ID that specifies the owner of any simulated resource that does not identify
* its owner in the resource ARN. Examples of resource ARNs include an S3 bucket or object. If
* ResourceOwner
is specified, it is also used as the account owner of any ResourcePolicy
* included in the simulation. If the ResourceOwner
parameter is not specified, then the owner of the
* resources and the resource policy defaults to the account of the identity provided in CallerArn
.
* This parameter is required only if you specify a resource-based policy and account that owns the resource is
* different from the account that owns the simulated calling user CallerArn
.
*
*
* The ARN for an account uses the following syntax: arn:aws:iam::AWS-account-ID:root
. For
* example, to represent the account with the 112233445566 ID, use the following ARN:
* arn:aws:iam::112233445566-ID:root
.
*
*
* @return An ARN representing the AWS account ID that specifies the owner of any simulated resource that does not
* identify its owner in the resource ARN. Examples of resource ARNs include an S3 bucket or object. If
* ResourceOwner
is specified, it is also used as the account owner of any
* ResourcePolicy
included in the simulation. If the ResourceOwner
parameter is
* not specified, then the owner of the resources and the resource policy defaults to the account of the
* identity provided in CallerArn
. This parameter is required only if you specify a
* resource-based policy and account that owns the resource is different from the account that owns the
* simulated calling user CallerArn
.
*
* The ARN for an account uses the following syntax: arn:aws:iam::AWS-account-ID:root
.
* For example, to represent the account with the 112233445566 ID, use the following ARN:
* arn:aws:iam::112233445566-ID:root
.
*/
public String resourceOwner() {
return resourceOwner;
}
/**
*
* The ARN of the IAM user that you want to use as the simulated caller of the API operations.
* CallerArn
is required if you include a ResourcePolicy
so that the policy's
* Principal
element has a value to use in evaluating the policy.
*
*
* You can specify only the ARN of an IAM user. You cannot specify the ARN of an assumed role, federated user, or a
* service principal.
*
*
* @return The ARN of the IAM user that you want to use as the simulated caller of the API operations.
* CallerArn
is required if you include a ResourcePolicy
so that the policy's
* Principal
element has a value to use in evaluating the policy.
*
* You can specify only the ARN of an IAM user. You cannot specify the ARN of an assumed role, federated
* user, or a service principal.
*/
public String callerArn() {
return callerArn;
}
/**
* Returns true if the ContextEntries property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasContextEntries() {
return contextEntries != null && !(contextEntries instanceof SdkAutoConstructList);
}
/**
*
* A list of context keys and corresponding values for the simulation to use. Whenever a context key is evaluated in
* one of the simulated IAM permissions policies, the corresponding value is supplied.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasContextEntries()} to see if a value was sent in this field.
*
*
* @return A list of context keys and corresponding values for the simulation to use. Whenever a context key is
* evaluated in one of the simulated IAM permissions policies, the corresponding value is supplied.
*/
public List contextEntries() {
return contextEntries;
}
/**
*
* Specifies the type of simulation to run. Different API operations that support resource-based policies require
* different combinations of resources. By specifying the type of simulation to run, you enable the policy simulator
* to enforce the presence of the required resources to ensure reliable simulation results. If your simulation does
* not match one of the following scenarios, then you can omit this parameter. The following list shows each of the
* supported scenario values and the resources that you must define to run the simulation.
*
*
* Each of the EC2 scenarios requires that you specify instance, image, and security-group resources. If your
* scenario includes an EBS volume, then you must specify that volume as a resource. If the EC2 scenario includes
* VPC, then you must supply the network-interface resource. If it includes an IP subnet, then you must specify the
* subnet resource. For more information on the EC2 scenario options, see Supported Platforms
* in the Amazon EC2 User Guide.
*
*
* -
*
* EC2-Classic-InstanceStore
*
*
* instance, image, security-group
*
*
* -
*
* EC2-Classic-EBS
*
*
* instance, image, security-group, volume
*
*
* -
*
* EC2-VPC-InstanceStore
*
*
* instance, image, security-group, network-interface
*
*
* -
*
* EC2-VPC-InstanceStore-Subnet
*
*
* instance, image, security-group, network-interface, subnet
*
*
* -
*
* EC2-VPC-EBS
*
*
* instance, image, security-group, network-interface, volume
*
*
* -
*
* EC2-VPC-EBS-Subnet
*
*
* instance, image, security-group, network-interface, subnet, volume
*
*
*
*
* @return Specifies the type of simulation to run. Different API operations that support resource-based policies
* require different combinations of resources. By specifying the type of simulation to run, you enable the
* policy simulator to enforce the presence of the required resources to ensure reliable simulation results.
* If your simulation does not match one of the following scenarios, then you can omit this parameter. The
* following list shows each of the supported scenario values and the resources that you must define to run
* the simulation.
*
* Each of the EC2 scenarios requires that you specify instance, image, and security-group resources. If
* your scenario includes an EBS volume, then you must specify that volume as a resource. If the EC2
* scenario includes VPC, then you must supply the network-interface resource. If it includes an IP subnet,
* then you must specify the subnet resource. For more information on the EC2 scenario options, see Supported
* Platforms in the Amazon EC2 User Guide.
*
*
* -
*
* EC2-Classic-InstanceStore
*
*
* instance, image, security-group
*
*
* -
*
* EC2-Classic-EBS
*
*
* instance, image, security-group, volume
*
*
* -
*
* EC2-VPC-InstanceStore
*
*
* instance, image, security-group, network-interface
*
*
* -
*
* EC2-VPC-InstanceStore-Subnet
*
*
* instance, image, security-group, network-interface, subnet
*
*
* -
*
* EC2-VPC-EBS
*
*
* instance, image, security-group, network-interface, volume
*
*
* -
*
* EC2-VPC-EBS-Subnet
*
*
* instance, image, security-group, network-interface, subnet, volume
*
*
*/
public String resourceHandlingOption() {
return resourceHandlingOption;
}
/**
*
* Use this only when paginating results to indicate the maximum number of items you want in the response. If
* additional items exist beyond the maximum you specify, the IsTruncated
response element is
* true
.
*
*
* If you do not include this parameter, the number of items defaults to 100. Note that IAM might return fewer
* results, even when there are more results available. In that case, the IsTruncated
response element
* returns true
, and Marker
contains a value to include in the subsequent call that tells
* the service where to continue from.
*
*
* @return Use this only when paginating results to indicate the maximum number of items you want in the response.
* If additional items exist beyond the maximum you specify, the IsTruncated
response element
* is true
.
*
* If you do not include this parameter, the number of items defaults to 100. Note that IAM might return
* fewer results, even when there are more results available. In that case, the IsTruncated
* response element returns true
, and Marker
contains a value to include in the
* subsequent call that tells the service where to continue from.
*/
public Integer maxItems() {
return maxItems;
}
/**
*
* Use this parameter only when paginating results and only after you receive a response indicating that the results
* are truncated. Set it to the value of the Marker
element in the response that you received to
* indicate where the next call should start.
*
*
* @return Use this parameter only when paginating results and only after you receive a response indicating that the
* results are truncated. Set it to the value of the Marker
element in the response that you
* received to indicate where the next call should start.
*/
public String marker() {
return marker;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(policyInputList());
hashCode = 31 * hashCode + Objects.hashCode(permissionsBoundaryPolicyInputList());
hashCode = 31 * hashCode + Objects.hashCode(actionNames());
hashCode = 31 * hashCode + Objects.hashCode(resourceArns());
hashCode = 31 * hashCode + Objects.hashCode(resourcePolicy());
hashCode = 31 * hashCode + Objects.hashCode(resourceOwner());
hashCode = 31 * hashCode + Objects.hashCode(callerArn());
hashCode = 31 * hashCode + Objects.hashCode(contextEntries());
hashCode = 31 * hashCode + Objects.hashCode(resourceHandlingOption());
hashCode = 31 * hashCode + Objects.hashCode(maxItems());
hashCode = 31 * hashCode + Objects.hashCode(marker());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SimulateCustomPolicyRequest)) {
return false;
}
SimulateCustomPolicyRequest other = (SimulateCustomPolicyRequest) obj;
return Objects.equals(policyInputList(), other.policyInputList())
&& Objects.equals(permissionsBoundaryPolicyInputList(), other.permissionsBoundaryPolicyInputList())
&& Objects.equals(actionNames(), other.actionNames()) && Objects.equals(resourceArns(), other.resourceArns())
&& Objects.equals(resourcePolicy(), other.resourcePolicy())
&& Objects.equals(resourceOwner(), other.resourceOwner()) && Objects.equals(callerArn(), other.callerArn())
&& Objects.equals(contextEntries(), other.contextEntries())
&& Objects.equals(resourceHandlingOption(), other.resourceHandlingOption())
&& Objects.equals(maxItems(), other.maxItems()) && Objects.equals(marker(), other.marker());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("SimulateCustomPolicyRequest").add("PolicyInputList", policyInputList())
.add("PermissionsBoundaryPolicyInputList", permissionsBoundaryPolicyInputList())
.add("ActionNames", actionNames()).add("ResourceArns", resourceArns()).add("ResourcePolicy", resourcePolicy())
.add("ResourceOwner", resourceOwner()).add("CallerArn", callerArn()).add("ContextEntries", contextEntries())
.add("ResourceHandlingOption", resourceHandlingOption()).add("MaxItems", maxItems()).add("Marker", marker())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PolicyInputList":
return Optional.ofNullable(clazz.cast(policyInputList()));
case "PermissionsBoundaryPolicyInputList":
return Optional.ofNullable(clazz.cast(permissionsBoundaryPolicyInputList()));
case "ActionNames":
return Optional.ofNullable(clazz.cast(actionNames()));
case "ResourceArns":
return Optional.ofNullable(clazz.cast(resourceArns()));
case "ResourcePolicy":
return Optional.ofNullable(clazz.cast(resourcePolicy()));
case "ResourceOwner":
return Optional.ofNullable(clazz.cast(resourceOwner()));
case "CallerArn":
return Optional.ofNullable(clazz.cast(callerArn()));
case "ContextEntries":
return Optional.ofNullable(clazz.cast(contextEntries()));
case "ResourceHandlingOption":
return Optional.ofNullable(clazz.cast(resourceHandlingOption()));
case "MaxItems":
return Optional.ofNullable(clazz.cast(maxItems()));
case "Marker":
return Optional.ofNullable(clazz.cast(marker()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function