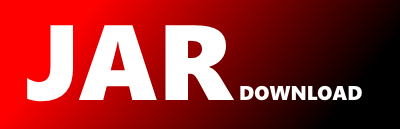
software.amazon.awssdk.services.iam.model.ServiceLastAccessed Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains details about the most recent attempt to access the service.
*
*
* This data type is used as a response element in the GetServiceLastAccessedDetails operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceLastAccessed implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceName").getter(getter(ServiceLastAccessed::serviceName)).setter(setter(Builder::serviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceName").build()).build();
private static final SdkField LAST_AUTHENTICATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastAuthenticated").getter(getter(ServiceLastAccessed::lastAuthenticated))
.setter(setter(Builder::lastAuthenticated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastAuthenticated").build()).build();
private static final SdkField SERVICE_NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceNamespace").getter(getter(ServiceLastAccessed::serviceNamespace))
.setter(setter(Builder::serviceNamespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceNamespace").build()).build();
private static final SdkField LAST_AUTHENTICATED_ENTITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LastAuthenticatedEntity").getter(getter(ServiceLastAccessed::lastAuthenticatedEntity))
.setter(setter(Builder::lastAuthenticatedEntity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastAuthenticatedEntity").build())
.build();
private static final SdkField LAST_AUTHENTICATED_REGION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LastAuthenticatedRegion").getter(getter(ServiceLastAccessed::lastAuthenticatedRegion))
.setter(setter(Builder::lastAuthenticatedRegion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastAuthenticatedRegion").build())
.build();
private static final SdkField TOTAL_AUTHENTICATED_ENTITIES_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("TotalAuthenticatedEntities")
.getter(getter(ServiceLastAccessed::totalAuthenticatedEntities))
.setter(setter(Builder::totalAuthenticatedEntities))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalAuthenticatedEntities").build())
.build();
private static final SdkField> TRACKED_ACTIONS_LAST_ACCESSED_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TrackedActionsLastAccessed")
.getter(getter(ServiceLastAccessed::trackedActionsLastAccessed))
.setter(setter(Builder::trackedActionsLastAccessed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TrackedActionsLastAccessed").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TrackedActionLastAccessed::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_NAME_FIELD,
LAST_AUTHENTICATED_FIELD, SERVICE_NAMESPACE_FIELD, LAST_AUTHENTICATED_ENTITY_FIELD, LAST_AUTHENTICATED_REGION_FIELD,
TOTAL_AUTHENTICATED_ENTITIES_FIELD, TRACKED_ACTIONS_LAST_ACCESSED_FIELD));
private static final long serialVersionUID = 1L;
private final String serviceName;
private final Instant lastAuthenticated;
private final String serviceNamespace;
private final String lastAuthenticatedEntity;
private final String lastAuthenticatedRegion;
private final Integer totalAuthenticatedEntities;
private final List trackedActionsLastAccessed;
private ServiceLastAccessed(BuilderImpl builder) {
this.serviceName = builder.serviceName;
this.lastAuthenticated = builder.lastAuthenticated;
this.serviceNamespace = builder.serviceNamespace;
this.lastAuthenticatedEntity = builder.lastAuthenticatedEntity;
this.lastAuthenticatedRegion = builder.lastAuthenticatedRegion;
this.totalAuthenticatedEntities = builder.totalAuthenticatedEntities;
this.trackedActionsLastAccessed = builder.trackedActionsLastAccessed;
}
/**
*
* The name of the service in which access was attempted.
*
*
* @return The name of the service in which access was attempted.
*/
public final String serviceName() {
return serviceName;
}
/**
*
* The date and time, in ISO 8601 date-time format, when an
* authenticated entity most recently attempted to access the service. Amazon Web Services does not report
* unauthenticated requests.
*
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*
*
* @return The date and time, in ISO 8601 date-time format, when an
* authenticated entity most recently attempted to access the service. Amazon Web Services does not report
* unauthenticated requests.
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*/
public final Instant lastAuthenticated() {
return lastAuthenticated;
}
/**
*
* The namespace of the service in which access was attempted.
*
*
* To learn the service namespace of a service, see Actions, resources, and condition keys for Amazon Web Services services in the Service Authorization
* Reference. Choose the name of the service to view details for that service. In the first paragraph, find the
* service prefix. For example, (service prefix: a4b)
. For more information about service namespaces,
* see Amazon Web Services Service Namespaces in the Amazon Web Services General Reference.
*
*
* @return The namespace of the service in which access was attempted.
*
* To learn the service namespace of a service, see Actions, resources, and condition keys for Amazon Web Services services in the Service
* Authorization Reference. Choose the name of the service to view details for that service. In the
* first paragraph, find the service prefix. For example, (service prefix: a4b)
. For more
* information about service namespaces, see Amazon Web Services Service Namespaces in the Amazon Web Services General Reference.
*/
public final String serviceNamespace() {
return serviceNamespace;
}
/**
*
* The ARN of the authenticated entity (user or role) that last attempted to access the service. Amazon Web Services
* does not report unauthenticated requests.
*
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*
*
* @return The ARN of the authenticated entity (user or role) that last attempted to access the service. Amazon Web
* Services does not report unauthenticated requests.
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*/
public final String lastAuthenticatedEntity() {
return lastAuthenticatedEntity;
}
/**
*
* The Region from which the authenticated entity (user or role) last attempted to access the service. Amazon Web
* Services does not report unauthenticated requests.
*
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*
*
* @return The Region from which the authenticated entity (user or role) last attempted to access the service.
* Amazon Web Services does not report unauthenticated requests.
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*/
public final String lastAuthenticatedRegion() {
return lastAuthenticatedRegion;
}
/**
*
* The total number of authenticated principals (root user, IAM users, or IAM roles) that have attempted to access
* the service.
*
*
* This field is null if no principals attempted to access the service within the reporting period.
*
*
* @return The total number of authenticated principals (root user, IAM users, or IAM roles) that have attempted to
* access the service.
*
* This field is null if no principals attempted to access the service within the reporting period.
*/
public final Integer totalAuthenticatedEntities() {
return totalAuthenticatedEntities;
}
/**
* For responses, this returns true if the service returned a value for the TrackedActionsLastAccessed property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasTrackedActionsLastAccessed() {
return trackedActionsLastAccessed != null && !(trackedActionsLastAccessed instanceof SdkAutoConstructList);
}
/**
*
* An object that contains details about the most recent attempt to access a tracked action within the service.
*
*
* This field is null if there no tracked actions or if the principal did not use the tracked actions within the reporting period. This field is also null if the report was generated at the service level and not the
* action level. For more information, see the Granularity
field in
* GenerateServiceLastAccessedDetails.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTrackedActionsLastAccessed} method.
*
*
* @return An object that contains details about the most recent attempt to access a tracked action within the
* service.
*
* This field is null if there no tracked actions or if the principal did not use the tracked actions within
* the reporting period. This field is also null if the report was generated at the service level and not
* the action level. For more information, see the Granularity
field in
* GenerateServiceLastAccessedDetails.
*/
public final List trackedActionsLastAccessed() {
return trackedActionsLastAccessed;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serviceName());
hashCode = 31 * hashCode + Objects.hashCode(lastAuthenticated());
hashCode = 31 * hashCode + Objects.hashCode(serviceNamespace());
hashCode = 31 * hashCode + Objects.hashCode(lastAuthenticatedEntity());
hashCode = 31 * hashCode + Objects.hashCode(lastAuthenticatedRegion());
hashCode = 31 * hashCode + Objects.hashCode(totalAuthenticatedEntities());
hashCode = 31 * hashCode + Objects.hashCode(hasTrackedActionsLastAccessed() ? trackedActionsLastAccessed() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceLastAccessed)) {
return false;
}
ServiceLastAccessed other = (ServiceLastAccessed) obj;
return Objects.equals(serviceName(), other.serviceName())
&& Objects.equals(lastAuthenticated(), other.lastAuthenticated())
&& Objects.equals(serviceNamespace(), other.serviceNamespace())
&& Objects.equals(lastAuthenticatedEntity(), other.lastAuthenticatedEntity())
&& Objects.equals(lastAuthenticatedRegion(), other.lastAuthenticatedRegion())
&& Objects.equals(totalAuthenticatedEntities(), other.totalAuthenticatedEntities())
&& hasTrackedActionsLastAccessed() == other.hasTrackedActionsLastAccessed()
&& Objects.equals(trackedActionsLastAccessed(), other.trackedActionsLastAccessed());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceLastAccessed").add("ServiceName", serviceName())
.add("LastAuthenticated", lastAuthenticated()).add("ServiceNamespace", serviceNamespace())
.add("LastAuthenticatedEntity", lastAuthenticatedEntity())
.add("LastAuthenticatedRegion", lastAuthenticatedRegion())
.add("TotalAuthenticatedEntities", totalAuthenticatedEntities())
.add("TrackedActionsLastAccessed", hasTrackedActionsLastAccessed() ? trackedActionsLastAccessed() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ServiceName":
return Optional.ofNullable(clazz.cast(serviceName()));
case "LastAuthenticated":
return Optional.ofNullable(clazz.cast(lastAuthenticated()));
case "ServiceNamespace":
return Optional.ofNullable(clazz.cast(serviceNamespace()));
case "LastAuthenticatedEntity":
return Optional.ofNullable(clazz.cast(lastAuthenticatedEntity()));
case "LastAuthenticatedRegion":
return Optional.ofNullable(clazz.cast(lastAuthenticatedRegion()));
case "TotalAuthenticatedEntities":
return Optional.ofNullable(clazz.cast(totalAuthenticatedEntities()));
case "TrackedActionsLastAccessed":
return Optional.ofNullable(clazz.cast(trackedActionsLastAccessed()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lastAuthenticated(Instant lastAuthenticated);
/**
*
* The namespace of the service in which access was attempted.
*
*
* To learn the service namespace of a service, see Actions, resources, and condition keys for Amazon Web Services services in the Service Authorization
* Reference. Choose the name of the service to view details for that service. In the first paragraph, find
* the service prefix. For example, (service prefix: a4b)
. For more information about service
* namespaces, see Amazon Web Services Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the service in which access was attempted.
*
* To learn the service namespace of a service, see Actions, resources, and condition keys for Amazon Web Services services in the Service
* Authorization Reference. Choose the name of the service to view details for that service. In the
* first paragraph, find the service prefix. For example, (service prefix: a4b)
. For more
* information about service namespaces, see Amazon Web Services Service Namespaces in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder serviceNamespace(String serviceNamespace);
/**
*
* The ARN of the authenticated entity (user or role) that last attempted to access the service. Amazon Web
* Services does not report unauthenticated requests.
*
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*
*
* @param lastAuthenticatedEntity
* The ARN of the authenticated entity (user or role) that last attempted to access the service. Amazon
* Web Services does not report unauthenticated requests.
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lastAuthenticatedEntity(String lastAuthenticatedEntity);
/**
*
* The Region from which the authenticated entity (user or role) last attempted to access the service. Amazon
* Web Services does not report unauthenticated requests.
*
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
*
*
* @param lastAuthenticatedRegion
* The Region from which the authenticated entity (user or role) last attempted to access the service.
* Amazon Web Services does not report unauthenticated requests.
*
* This field is null if no IAM entities attempted to access the service within the reporting period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lastAuthenticatedRegion(String lastAuthenticatedRegion);
/**
*
* The total number of authenticated principals (root user, IAM users, or IAM roles) that have attempted to
* access the service.
*
*
* This field is null if no principals attempted to access the service within the reporting period.
*
*
* @param totalAuthenticatedEntities
* The total number of authenticated principals (root user, IAM users, or IAM roles) that have attempted
* to access the service.
*
* This field is null if no principals attempted to access the service within the reporting period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder totalAuthenticatedEntities(Integer totalAuthenticatedEntities);
/**
*
* An object that contains details about the most recent attempt to access a tracked action within the service.
*
*
* This field is null if there no tracked actions or if the principal did not use the tracked actions within the
* reporting period. This field is also null if the report was generated at the service level and not the
* action level. For more information, see the Granularity
field in
* GenerateServiceLastAccessedDetails.
*
*
* @param trackedActionsLastAccessed
* An object that contains details about the most recent attempt to access a tracked action within the
* service.
*
* This field is null if there no tracked actions or if the principal did not use the tracked actions
* within the reporting period. This field is also null if the report was generated at the service level and
* not the action level. For more information, see the Granularity
field in
* GenerateServiceLastAccessedDetails.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder trackedActionsLastAccessed(Collection trackedActionsLastAccessed);
/**
*
* An object that contains details about the most recent attempt to access a tracked action within the service.
*
*
* This field is null if there no tracked actions or if the principal did not use the tracked actions within the
* reporting period. This field is also null if the report was generated at the service level and not the
* action level. For more information, see the Granularity
field in
* GenerateServiceLastAccessedDetails.
*
*
* @param trackedActionsLastAccessed
* An object that contains details about the most recent attempt to access a tracked action within the
* service.
*
* This field is null if there no tracked actions or if the principal did not use the tracked actions
* within the reporting period. This field is also null if the report was generated at the service level and
* not the action level. For more information, see the Granularity
field in
* GenerateServiceLastAccessedDetails.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder trackedActionsLastAccessed(TrackedActionLastAccessed... trackedActionsLastAccessed);
/**
*
* An object that contains details about the most recent attempt to access a tracked action within the service.
*
*
* This field is null if there no tracked actions or if the principal did not use the tracked actions within the
* reporting period. This field is also null if the report was generated at the service level and not the
* action level. For more information, see the Granularity
field in
* GenerateServiceLastAccessedDetails.
*
* This is a convenience method that creates an instance of the {@link List.Builder}
* avoiding the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called
* immediately and its result is passed to {@link #trackedActionsLastAccessed(List)}.
*
* @param trackedActionsLastAccessed
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #trackedActionsLastAccessed(List)
*/
Builder trackedActionsLastAccessed(Consumer... trackedActionsLastAccessed);
}
static final class BuilderImpl implements Builder {
private String serviceName;
private Instant lastAuthenticated;
private String serviceNamespace;
private String lastAuthenticatedEntity;
private String lastAuthenticatedRegion;
private Integer totalAuthenticatedEntities;
private List trackedActionsLastAccessed = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(ServiceLastAccessed model) {
serviceName(model.serviceName);
lastAuthenticated(model.lastAuthenticated);
serviceNamespace(model.serviceNamespace);
lastAuthenticatedEntity(model.lastAuthenticatedEntity);
lastAuthenticatedRegion(model.lastAuthenticatedRegion);
totalAuthenticatedEntities(model.totalAuthenticatedEntities);
trackedActionsLastAccessed(model.trackedActionsLastAccessed);
}
public final String getServiceName() {
return serviceName;
}
public final void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
@Override
public final Builder serviceName(String serviceName) {
this.serviceName = serviceName;
return this;
}
public final Instant getLastAuthenticated() {
return lastAuthenticated;
}
public final void setLastAuthenticated(Instant lastAuthenticated) {
this.lastAuthenticated = lastAuthenticated;
}
@Override
public final Builder lastAuthenticated(Instant lastAuthenticated) {
this.lastAuthenticated = lastAuthenticated;
return this;
}
public final String getServiceNamespace() {
return serviceNamespace;
}
public final void setServiceNamespace(String serviceNamespace) {
this.serviceNamespace = serviceNamespace;
}
@Override
public final Builder serviceNamespace(String serviceNamespace) {
this.serviceNamespace = serviceNamespace;
return this;
}
public final String getLastAuthenticatedEntity() {
return lastAuthenticatedEntity;
}
public final void setLastAuthenticatedEntity(String lastAuthenticatedEntity) {
this.lastAuthenticatedEntity = lastAuthenticatedEntity;
}
@Override
public final Builder lastAuthenticatedEntity(String lastAuthenticatedEntity) {
this.lastAuthenticatedEntity = lastAuthenticatedEntity;
return this;
}
public final String getLastAuthenticatedRegion() {
return lastAuthenticatedRegion;
}
public final void setLastAuthenticatedRegion(String lastAuthenticatedRegion) {
this.lastAuthenticatedRegion = lastAuthenticatedRegion;
}
@Override
public final Builder lastAuthenticatedRegion(String lastAuthenticatedRegion) {
this.lastAuthenticatedRegion = lastAuthenticatedRegion;
return this;
}
public final Integer getTotalAuthenticatedEntities() {
return totalAuthenticatedEntities;
}
public final void setTotalAuthenticatedEntities(Integer totalAuthenticatedEntities) {
this.totalAuthenticatedEntities = totalAuthenticatedEntities;
}
@Override
public final Builder totalAuthenticatedEntities(Integer totalAuthenticatedEntities) {
this.totalAuthenticatedEntities = totalAuthenticatedEntities;
return this;
}
public final List getTrackedActionsLastAccessed() {
List result = TrackedActionsLastAccessedCopier
.copyToBuilder(this.trackedActionsLastAccessed);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTrackedActionsLastAccessed(
Collection trackedActionsLastAccessed) {
this.trackedActionsLastAccessed = TrackedActionsLastAccessedCopier.copyFromBuilder(trackedActionsLastAccessed);
}
@Override
public final Builder trackedActionsLastAccessed(Collection trackedActionsLastAccessed) {
this.trackedActionsLastAccessed = TrackedActionsLastAccessedCopier.copy(trackedActionsLastAccessed);
return this;
}
@Override
@SafeVarargs
public final Builder trackedActionsLastAccessed(TrackedActionLastAccessed... trackedActionsLastAccessed) {
trackedActionsLastAccessed(Arrays.asList(trackedActionsLastAccessed));
return this;
}
@Override
@SafeVarargs
public final Builder trackedActionsLastAccessed(Consumer... trackedActionsLastAccessed) {
trackedActionsLastAccessed(Stream.of(trackedActionsLastAccessed)
.map(c -> TrackedActionLastAccessed.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
@Override
public ServiceLastAccessed build() {
return new ServiceLastAccessed(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}