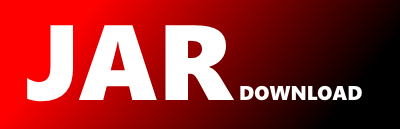
software.amazon.awssdk.services.iam.IamClient Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.iam.model.AddClientIdToOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.AddClientIdToOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.AddRoleToInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.AddRoleToInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.AddUserToGroupRequest;
import software.amazon.awssdk.services.iam.model.AddUserToGroupResponse;
import software.amazon.awssdk.services.iam.model.AttachGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.AttachGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.AttachRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.AttachRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.AttachUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.AttachUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.ChangePasswordRequest;
import software.amazon.awssdk.services.iam.model.ChangePasswordResponse;
import software.amazon.awssdk.services.iam.model.ConcurrentModificationException;
import software.amazon.awssdk.services.iam.model.CreateAccessKeyRequest;
import software.amazon.awssdk.services.iam.model.CreateAccessKeyResponse;
import software.amazon.awssdk.services.iam.model.CreateAccountAliasRequest;
import software.amazon.awssdk.services.iam.model.CreateAccountAliasResponse;
import software.amazon.awssdk.services.iam.model.CreateGroupRequest;
import software.amazon.awssdk.services.iam.model.CreateGroupResponse;
import software.amazon.awssdk.services.iam.model.CreateInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.CreateInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.CreateLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.CreateLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.CreateOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.CreateOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.CreatePolicyRequest;
import software.amazon.awssdk.services.iam.model.CreatePolicyResponse;
import software.amazon.awssdk.services.iam.model.CreatePolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.CreatePolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.CreateRoleRequest;
import software.amazon.awssdk.services.iam.model.CreateRoleResponse;
import software.amazon.awssdk.services.iam.model.CreateSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.CreateSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.CreateServiceLinkedRoleRequest;
import software.amazon.awssdk.services.iam.model.CreateServiceLinkedRoleResponse;
import software.amazon.awssdk.services.iam.model.CreateServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.CreateServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.CreateUserRequest;
import software.amazon.awssdk.services.iam.model.CreateUserResponse;
import software.amazon.awssdk.services.iam.model.CreateVirtualMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.CreateVirtualMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.CredentialReportExpiredException;
import software.amazon.awssdk.services.iam.model.CredentialReportNotPresentException;
import software.amazon.awssdk.services.iam.model.CredentialReportNotReadyException;
import software.amazon.awssdk.services.iam.model.DeactivateMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.DeactivateMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.DeleteAccessKeyRequest;
import software.amazon.awssdk.services.iam.model.DeleteAccessKeyResponse;
import software.amazon.awssdk.services.iam.model.DeleteAccountAliasRequest;
import software.amazon.awssdk.services.iam.model.DeleteAccountAliasResponse;
import software.amazon.awssdk.services.iam.model.DeleteAccountPasswordPolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteAccountPasswordPolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteConflictException;
import software.amazon.awssdk.services.iam.model.DeleteGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteGroupRequest;
import software.amazon.awssdk.services.iam.model.DeleteGroupResponse;
import software.amazon.awssdk.services.iam.model.DeleteInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.DeleteInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.DeleteLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.DeleteLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.DeleteOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.DeleteOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.DeletePolicyRequest;
import software.amazon.awssdk.services.iam.model.DeletePolicyResponse;
import software.amazon.awssdk.services.iam.model.DeletePolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.DeletePolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.DeleteRolePermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.DeleteRolePermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.DeleteRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteRoleRequest;
import software.amazon.awssdk.services.iam.model.DeleteRoleResponse;
import software.amazon.awssdk.services.iam.model.DeleteSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.DeleteSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.DeleteServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.DeleteServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.DeleteServiceLinkedRoleRequest;
import software.amazon.awssdk.services.iam.model.DeleteServiceLinkedRoleResponse;
import software.amazon.awssdk.services.iam.model.DeleteServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.DeleteServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.DeleteSigningCertificateRequest;
import software.amazon.awssdk.services.iam.model.DeleteSigningCertificateResponse;
import software.amazon.awssdk.services.iam.model.DeleteSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.DeleteSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.model.DeleteUserPermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.DeleteUserPermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.DeleteUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteUserRequest;
import software.amazon.awssdk.services.iam.model.DeleteUserResponse;
import software.amazon.awssdk.services.iam.model.DeleteVirtualMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.DeleteVirtualMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.DetachGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.DetachGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.DetachRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.DetachRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.DetachUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.DetachUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.DuplicateCertificateException;
import software.amazon.awssdk.services.iam.model.DuplicateSshPublicKeyException;
import software.amazon.awssdk.services.iam.model.EnableMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.EnableMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.EntityAlreadyExistsException;
import software.amazon.awssdk.services.iam.model.EntityTemporarilyUnmodifiableException;
import software.amazon.awssdk.services.iam.model.GenerateCredentialReportRequest;
import software.amazon.awssdk.services.iam.model.GenerateCredentialReportResponse;
import software.amazon.awssdk.services.iam.model.GenerateOrganizationsAccessReportRequest;
import software.amazon.awssdk.services.iam.model.GenerateOrganizationsAccessReportResponse;
import software.amazon.awssdk.services.iam.model.GenerateServiceLastAccessedDetailsRequest;
import software.amazon.awssdk.services.iam.model.GenerateServiceLastAccessedDetailsResponse;
import software.amazon.awssdk.services.iam.model.GetAccessKeyLastUsedRequest;
import software.amazon.awssdk.services.iam.model.GetAccessKeyLastUsedResponse;
import software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest;
import software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsResponse;
import software.amazon.awssdk.services.iam.model.GetAccountPasswordPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetAccountPasswordPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetAccountSummaryRequest;
import software.amazon.awssdk.services.iam.model.GetAccountSummaryResponse;
import software.amazon.awssdk.services.iam.model.GetContextKeysForCustomPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetContextKeysForCustomPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetContextKeysForPrincipalPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetContextKeysForPrincipalPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetCredentialReportRequest;
import software.amazon.awssdk.services.iam.model.GetCredentialReportResponse;
import software.amazon.awssdk.services.iam.model.GetGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetGroupRequest;
import software.amazon.awssdk.services.iam.model.GetGroupResponse;
import software.amazon.awssdk.services.iam.model.GetInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.GetInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.GetLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.GetLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.GetOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.GetOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.GetOrganizationsAccessReportRequest;
import software.amazon.awssdk.services.iam.model.GetOrganizationsAccessReportResponse;
import software.amazon.awssdk.services.iam.model.GetPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetPolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.GetPolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.GetRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.GetRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.GetRoleRequest;
import software.amazon.awssdk.services.iam.model.GetRoleResponse;
import software.amazon.awssdk.services.iam.model.GetSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.GetSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.GetServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.GetServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsRequest;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsResponse;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsWithEntitiesRequest;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsWithEntitiesResponse;
import software.amazon.awssdk.services.iam.model.GetServiceLinkedRoleDeletionStatusRequest;
import software.amazon.awssdk.services.iam.model.GetServiceLinkedRoleDeletionStatusResponse;
import software.amazon.awssdk.services.iam.model.GetSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.GetSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.model.GetUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetUserRequest;
import software.amazon.awssdk.services.iam.model.GetUserResponse;
import software.amazon.awssdk.services.iam.model.IamException;
import software.amazon.awssdk.services.iam.model.InvalidAuthenticationCodeException;
import software.amazon.awssdk.services.iam.model.InvalidCertificateException;
import software.amazon.awssdk.services.iam.model.InvalidInputException;
import software.amazon.awssdk.services.iam.model.InvalidPublicKeyException;
import software.amazon.awssdk.services.iam.model.InvalidUserTypeException;
import software.amazon.awssdk.services.iam.model.KeyPairMismatchException;
import software.amazon.awssdk.services.iam.model.LimitExceededException;
import software.amazon.awssdk.services.iam.model.ListAccessKeysRequest;
import software.amazon.awssdk.services.iam.model.ListAccessKeysResponse;
import software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest;
import software.amazon.awssdk.services.iam.model.ListAccountAliasesResponse;
import software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyRequest;
import software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyResponse;
import software.amazon.awssdk.services.iam.model.ListGroupPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListGroupPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListGroupsForUserRequest;
import software.amazon.awssdk.services.iam.model.ListGroupsForUserResponse;
import software.amazon.awssdk.services.iam.model.ListGroupsRequest;
import software.amazon.awssdk.services.iam.model.ListGroupsResponse;
import software.amazon.awssdk.services.iam.model.ListInstanceProfileTagsRequest;
import software.amazon.awssdk.services.iam.model.ListInstanceProfileTagsResponse;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleRequest;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleResponse;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesResponse;
import software.amazon.awssdk.services.iam.model.ListMfaDeviceTagsRequest;
import software.amazon.awssdk.services.iam.model.ListMfaDeviceTagsResponse;
import software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest;
import software.amazon.awssdk.services.iam.model.ListMfaDevicesResponse;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProviderTagsRequest;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProviderTagsResponse;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProvidersRequest;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProvidersResponse;
import software.amazon.awssdk.services.iam.model.ListPoliciesGrantingServiceAccessRequest;
import software.amazon.awssdk.services.iam.model.ListPoliciesGrantingServiceAccessResponse;
import software.amazon.awssdk.services.iam.model.ListPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListPolicyTagsRequest;
import software.amazon.awssdk.services.iam.model.ListPolicyTagsResponse;
import software.amazon.awssdk.services.iam.model.ListPolicyVersionsRequest;
import software.amazon.awssdk.services.iam.model.ListPolicyVersionsResponse;
import software.amazon.awssdk.services.iam.model.ListRolePoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListRolePoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListRoleTagsRequest;
import software.amazon.awssdk.services.iam.model.ListRoleTagsResponse;
import software.amazon.awssdk.services.iam.model.ListRolesRequest;
import software.amazon.awssdk.services.iam.model.ListRolesResponse;
import software.amazon.awssdk.services.iam.model.ListSamlProviderTagsRequest;
import software.amazon.awssdk.services.iam.model.ListSamlProviderTagsResponse;
import software.amazon.awssdk.services.iam.model.ListSamlProvidersRequest;
import software.amazon.awssdk.services.iam.model.ListSamlProvidersResponse;
import software.amazon.awssdk.services.iam.model.ListServerCertificateTagsRequest;
import software.amazon.awssdk.services.iam.model.ListServerCertificateTagsResponse;
import software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest;
import software.amazon.awssdk.services.iam.model.ListServerCertificatesResponse;
import software.amazon.awssdk.services.iam.model.ListServiceSpecificCredentialsRequest;
import software.amazon.awssdk.services.iam.model.ListServiceSpecificCredentialsResponse;
import software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest;
import software.amazon.awssdk.services.iam.model.ListSigningCertificatesResponse;
import software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest;
import software.amazon.awssdk.services.iam.model.ListSshPublicKeysResponse;
import software.amazon.awssdk.services.iam.model.ListUserPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListUserPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListUserTagsRequest;
import software.amazon.awssdk.services.iam.model.ListUserTagsResponse;
import software.amazon.awssdk.services.iam.model.ListUsersRequest;
import software.amazon.awssdk.services.iam.model.ListUsersResponse;
import software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest;
import software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesResponse;
import software.amazon.awssdk.services.iam.model.MalformedCertificateException;
import software.amazon.awssdk.services.iam.model.MalformedPolicyDocumentException;
import software.amazon.awssdk.services.iam.model.NoSuchEntityException;
import software.amazon.awssdk.services.iam.model.PasswordPolicyViolationException;
import software.amazon.awssdk.services.iam.model.PolicyEvaluationException;
import software.amazon.awssdk.services.iam.model.PolicyNotAttachableException;
import software.amazon.awssdk.services.iam.model.PutGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.PutGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.PutRolePermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.PutRolePermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.PutRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.PutRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.PutUserPermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.PutUserPermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.PutUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.PutUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.RemoveClientIdFromOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.RemoveClientIdFromOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.RemoveRoleFromInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.RemoveRoleFromInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.RemoveUserFromGroupRequest;
import software.amazon.awssdk.services.iam.model.RemoveUserFromGroupResponse;
import software.amazon.awssdk.services.iam.model.ReportGenerationLimitExceededException;
import software.amazon.awssdk.services.iam.model.ResetServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.ResetServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.ResyncMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.ResyncMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.ServiceFailureException;
import software.amazon.awssdk.services.iam.model.ServiceNotSupportedException;
import software.amazon.awssdk.services.iam.model.SetDefaultPolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.SetDefaultPolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.SetSecurityTokenServicePreferencesRequest;
import software.amazon.awssdk.services.iam.model.SetSecurityTokenServicePreferencesResponse;
import software.amazon.awssdk.services.iam.model.SimulateCustomPolicyRequest;
import software.amazon.awssdk.services.iam.model.SimulateCustomPolicyResponse;
import software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyRequest;
import software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyResponse;
import software.amazon.awssdk.services.iam.model.TagInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.TagInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.TagMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.TagMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.TagOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.TagOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.TagPolicyRequest;
import software.amazon.awssdk.services.iam.model.TagPolicyResponse;
import software.amazon.awssdk.services.iam.model.TagRoleRequest;
import software.amazon.awssdk.services.iam.model.TagRoleResponse;
import software.amazon.awssdk.services.iam.model.TagSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.TagSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.TagServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.TagServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.TagUserRequest;
import software.amazon.awssdk.services.iam.model.TagUserResponse;
import software.amazon.awssdk.services.iam.model.UnmodifiableEntityException;
import software.amazon.awssdk.services.iam.model.UnrecognizedPublicKeyEncodingException;
import software.amazon.awssdk.services.iam.model.UntagInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.UntagInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.UntagMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.UntagMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.UntagOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.UntagOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.UntagPolicyRequest;
import software.amazon.awssdk.services.iam.model.UntagPolicyResponse;
import software.amazon.awssdk.services.iam.model.UntagRoleRequest;
import software.amazon.awssdk.services.iam.model.UntagRoleResponse;
import software.amazon.awssdk.services.iam.model.UntagSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.UntagSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.UntagServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.UntagServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.UntagUserRequest;
import software.amazon.awssdk.services.iam.model.UntagUserResponse;
import software.amazon.awssdk.services.iam.model.UpdateAccessKeyRequest;
import software.amazon.awssdk.services.iam.model.UpdateAccessKeyResponse;
import software.amazon.awssdk.services.iam.model.UpdateAccountPasswordPolicyRequest;
import software.amazon.awssdk.services.iam.model.UpdateAccountPasswordPolicyResponse;
import software.amazon.awssdk.services.iam.model.UpdateAssumeRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.UpdateAssumeRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.UpdateGroupRequest;
import software.amazon.awssdk.services.iam.model.UpdateGroupResponse;
import software.amazon.awssdk.services.iam.model.UpdateLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.UpdateLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.UpdateOpenIdConnectProviderThumbprintRequest;
import software.amazon.awssdk.services.iam.model.UpdateOpenIdConnectProviderThumbprintResponse;
import software.amazon.awssdk.services.iam.model.UpdateRoleDescriptionRequest;
import software.amazon.awssdk.services.iam.model.UpdateRoleDescriptionResponse;
import software.amazon.awssdk.services.iam.model.UpdateRoleRequest;
import software.amazon.awssdk.services.iam.model.UpdateRoleResponse;
import software.amazon.awssdk.services.iam.model.UpdateSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.UpdateSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.UpdateServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.UpdateServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.UpdateServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.UpdateServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.UpdateSigningCertificateRequest;
import software.amazon.awssdk.services.iam.model.UpdateSigningCertificateResponse;
import software.amazon.awssdk.services.iam.model.UpdateSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.UpdateSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.model.UpdateUserRequest;
import software.amazon.awssdk.services.iam.model.UpdateUserResponse;
import software.amazon.awssdk.services.iam.model.UploadServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.UploadServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.UploadSigningCertificateRequest;
import software.amazon.awssdk.services.iam.model.UploadSigningCertificateResponse;
import software.amazon.awssdk.services.iam.model.UploadSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.UploadSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable;
import software.amazon.awssdk.services.iam.paginators.GetGroupIterable;
import software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable;
import software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable;
import software.amazon.awssdk.services.iam.paginators.ListAttachedGroupPoliciesIterable;
import software.amazon.awssdk.services.iam.paginators.ListAttachedRolePoliciesIterable;
import software.amazon.awssdk.services.iam.paginators.ListAttachedUserPoliciesIterable;
import software.amazon.awssdk.services.iam.paginators.ListEntitiesForPolicyIterable;
import software.amazon.awssdk.services.iam.paginators.ListGroupPoliciesIterable;
import software.amazon.awssdk.services.iam.paginators.ListGroupsForUserIterable;
import software.amazon.awssdk.services.iam.paginators.ListGroupsIterable;
import software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesForRoleIterable;
import software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable;
import software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable;
import software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable;
import software.amazon.awssdk.services.iam.paginators.ListPolicyVersionsIterable;
import software.amazon.awssdk.services.iam.paginators.ListRolePoliciesIterable;
import software.amazon.awssdk.services.iam.paginators.ListRolesIterable;
import software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable;
import software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable;
import software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable;
import software.amazon.awssdk.services.iam.paginators.ListUserPoliciesIterable;
import software.amazon.awssdk.services.iam.paginators.ListUserTagsIterable;
import software.amazon.awssdk.services.iam.paginators.ListUsersIterable;
import software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable;
import software.amazon.awssdk.services.iam.paginators.SimulateCustomPolicyIterable;
import software.amazon.awssdk.services.iam.paginators.SimulatePrincipalPolicyIterable;
import software.amazon.awssdk.services.iam.waiters.IamWaiter;
/**
* Service client for accessing IAM. This can be created using the static {@link #builder()} method.
*
* Identity and Access Management
*
* Identity and Access Management (IAM) is a web service for securely controlling access to Amazon Web Services
* services. With IAM, you can centrally manage users, security credentials such as access keys, and permissions that
* control which Amazon Web Services resources users and applications can access. For more information about IAM, see Identity and Access Management (IAM) and the Identity and Access Management User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface IamClient extends SdkClient {
String SERVICE_NAME = "iam";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "iam";
/**
*
* Adds a new client ID (also known as audience) to the list of client IDs already registered for the specified IAM
* OpenID Connect (OIDC) provider resource.
*
*
* This operation is idempotent; it does not fail or return an error if you add an existing client ID to the
* provider.
*
*
* @param addClientIdToOpenIdConnectProviderRequest
* @return Result of the AddClientIDToOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AddClientIDToOpenIDConnectProvider
* @see AWS API Documentation
*/
default AddClientIdToOpenIdConnectProviderResponse addClientIDToOpenIDConnectProvider(
AddClientIdToOpenIdConnectProviderRequest addClientIdToOpenIdConnectProviderRequest) throws InvalidInputException,
NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds a new client ID (also known as audience) to the list of client IDs already registered for the specified IAM
* OpenID Connect (OIDC) provider resource.
*
*
* This operation is idempotent; it does not fail or return an error if you add an existing client ID to the
* provider.
*
*
*
* This is a convenience which creates an instance of the {@link AddClientIdToOpenIdConnectProviderRequest.Builder}
* avoiding the need to create one manually via {@link AddClientIdToOpenIdConnectProviderRequest#builder()}
*
*
* @param addClientIdToOpenIdConnectProviderRequest
* A {@link Consumer} that will call methods on {@link AddClientIDToOpenIDConnectProviderRequest.Builder} to
* create a request.
* @return Result of the AddClientIDToOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AddClientIDToOpenIDConnectProvider
* @see AWS API Documentation
*/
default AddClientIdToOpenIdConnectProviderResponse addClientIDToOpenIDConnectProvider(
Consumer addClientIdToOpenIdConnectProviderRequest)
throws InvalidInputException, NoSuchEntityException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return addClientIDToOpenIDConnectProvider(AddClientIdToOpenIdConnectProviderRequest.builder()
.applyMutation(addClientIdToOpenIdConnectProviderRequest).build());
}
/**
*
* Adds the specified IAM role to the specified instance profile. An instance profile can contain only one role, and
* this quota cannot be increased. You can remove the existing role and then add a different role to an instance
* profile. You must then wait for the change to appear across all of Amazon Web Services because of eventual consistency. To force the change, you must
*
* disassociate the instance profile and then associate the
* instance profile, or you can stop your instance and then restart it.
*
*
*
* The caller of this operation must be granted the PassRole
permission on the IAM role by a
* permissions policy.
*
*
*
* For more information about roles, see Working with roles. For more
* information about instance profiles, see About instance profiles.
*
*
* @param addRoleToInstanceProfileRequest
* @return Result of the AddRoleToInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AddRoleToInstanceProfile
* @see AWS
* API Documentation
*/
default AddRoleToInstanceProfileResponse addRoleToInstanceProfile(
AddRoleToInstanceProfileRequest addRoleToInstanceProfileRequest) throws NoSuchEntityException,
EntityAlreadyExistsException, LimitExceededException, UnmodifiableEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified IAM role to the specified instance profile. An instance profile can contain only one role, and
* this quota cannot be increased. You can remove the existing role and then add a different role to an instance
* profile. You must then wait for the change to appear across all of Amazon Web Services because of eventual consistency. To force the change, you must
*
* disassociate the instance profile and then associate the
* instance profile, or you can stop your instance and then restart it.
*
*
*
* The caller of this operation must be granted the PassRole
permission on the IAM role by a
* permissions policy.
*
*
*
* For more information about roles, see Working with roles. For more
* information about instance profiles, see About instance profiles.
*
*
*
* This is a convenience which creates an instance of the {@link AddRoleToInstanceProfileRequest.Builder} avoiding
* the need to create one manually via {@link AddRoleToInstanceProfileRequest#builder()}
*
*
* @param addRoleToInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link AddRoleToInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the AddRoleToInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AddRoleToInstanceProfile
* @see AWS
* API Documentation
*/
default AddRoleToInstanceProfileResponse addRoleToInstanceProfile(
Consumer addRoleToInstanceProfileRequest) throws NoSuchEntityException,
EntityAlreadyExistsException, LimitExceededException, UnmodifiableEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return addRoleToInstanceProfile(AddRoleToInstanceProfileRequest.builder().applyMutation(addRoleToInstanceProfileRequest)
.build());
}
/**
*
* Adds the specified user to the specified group.
*
*
* @param addUserToGroupRequest
* @return Result of the AddUserToGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AddUserToGroup
* @see AWS API
* Documentation
*/
default AddUserToGroupResponse addUserToGroup(AddUserToGroupRequest addUserToGroupRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified user to the specified group.
*
*
*
* This is a convenience which creates an instance of the {@link AddUserToGroupRequest.Builder} avoiding the need to
* create one manually via {@link AddUserToGroupRequest#builder()}
*
*
* @param addUserToGroupRequest
* A {@link Consumer} that will call methods on {@link AddUserToGroupRequest.Builder} to create a request.
* @return Result of the AddUserToGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AddUserToGroup
* @see AWS API
* Documentation
*/
default AddUserToGroupResponse addUserToGroup(Consumer addUserToGroupRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return addUserToGroup(AddUserToGroupRequest.builder().applyMutation(addUserToGroupRequest).build());
}
/**
*
* Attaches the specified managed policy to the specified IAM group.
*
*
* You use this operation to attach a managed policy to a group. To embed an inline policy in a group, use
* PutGroupPolicy.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param attachGroupPolicyRequest
* @return Result of the AttachGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AttachGroupPolicy
* @see AWS API
* Documentation
*/
default AttachGroupPolicyResponse attachGroupPolicy(AttachGroupPolicyRequest attachGroupPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, PolicyNotAttachableException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches the specified managed policy to the specified IAM group.
*
*
* You use this operation to attach a managed policy to a group. To embed an inline policy in a group, use
* PutGroupPolicy.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AttachGroupPolicyRequest.Builder} avoiding the need
* to create one manually via {@link AttachGroupPolicyRequest#builder()}
*
*
* @param attachGroupPolicyRequest
* A {@link Consumer} that will call methods on {@link AttachGroupPolicyRequest.Builder} to create a request.
* @return Result of the AttachGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AttachGroupPolicy
* @see AWS API
* Documentation
*/
default AttachGroupPolicyResponse attachGroupPolicy(Consumer attachGroupPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, PolicyNotAttachableException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return attachGroupPolicy(AttachGroupPolicyRequest.builder().applyMutation(attachGroupPolicyRequest).build());
}
/**
*
* Attaches the specified managed policy to the specified IAM role. When you attach a managed policy to a role, the
* managed policy becomes part of the role's permission (access) policy.
*
*
*
* You cannot use a managed policy as the role's trust policy. The role's trust policy is created at the same time
* as the role, using CreateRole. You can update a role's trust policy using UpdateAssumeRolePolicy.
*
*
*
* Use this operation to attach a managed policy to a role. To embed an inline policy in a role, use
* PutRolePolicy. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* @param attachRolePolicyRequest
* @return Result of the AttachRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AttachRolePolicy
* @see AWS API
* Documentation
*/
default AttachRolePolicyResponse attachRolePolicy(AttachRolePolicyRequest attachRolePolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, UnmodifiableEntityException,
PolicyNotAttachableException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches the specified managed policy to the specified IAM role. When you attach a managed policy to a role, the
* managed policy becomes part of the role's permission (access) policy.
*
*
*
* You cannot use a managed policy as the role's trust policy. The role's trust policy is created at the same time
* as the role, using CreateRole. You can update a role's trust policy using UpdateAssumeRolePolicy.
*
*
*
* Use this operation to attach a managed policy to a role. To embed an inline policy in a role, use
* PutRolePolicy. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AttachRolePolicyRequest.Builder} avoiding the need
* to create one manually via {@link AttachRolePolicyRequest#builder()}
*
*
* @param attachRolePolicyRequest
* A {@link Consumer} that will call methods on {@link AttachRolePolicyRequest.Builder} to create a request.
* @return Result of the AttachRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AttachRolePolicy
* @see AWS API
* Documentation
*/
default AttachRolePolicyResponse attachRolePolicy(Consumer attachRolePolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, UnmodifiableEntityException,
PolicyNotAttachableException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return attachRolePolicy(AttachRolePolicyRequest.builder().applyMutation(attachRolePolicyRequest).build());
}
/**
*
* Attaches the specified managed policy to the specified user.
*
*
* You use this operation to attach a managed policy to a user. To embed an inline policy in a user, use
* PutUserPolicy.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param attachUserPolicyRequest
* @return Result of the AttachUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AttachUserPolicy
* @see AWS API
* Documentation
*/
default AttachUserPolicyResponse attachUserPolicy(AttachUserPolicyRequest attachUserPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, PolicyNotAttachableException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches the specified managed policy to the specified user.
*
*
* You use this operation to attach a managed policy to a user. To embed an inline policy in a user, use
* PutUserPolicy.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AttachUserPolicyRequest.Builder} avoiding the need
* to create one manually via {@link AttachUserPolicyRequest#builder()}
*
*
* @param attachUserPolicyRequest
* A {@link Consumer} that will call methods on {@link AttachUserPolicyRequest.Builder} to create a request.
* @return Result of the AttachUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.AttachUserPolicy
* @see AWS API
* Documentation
*/
default AttachUserPolicyResponse attachUserPolicy(Consumer attachUserPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, PolicyNotAttachableException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return attachUserPolicy(AttachUserPolicyRequest.builder().applyMutation(attachUserPolicyRequest).build());
}
/**
*
* Changes the password of the IAM user who is calling this operation. This operation can be performed using the
* CLI, the Amazon Web Services API, or the My Security Credentials page in the Amazon Web Services
* Management Console. The Amazon Web Services account root user password is not affected by this operation.
*
*
* Use UpdateLoginProfile to use the CLI, the Amazon Web Services API, or the Users page in the IAM
* console to change the password for any IAM user. For more information about modifying passwords, see Managing passwords in the
* IAM User Guide.
*
*
* @param changePasswordRequest
* @return Result of the ChangePassword operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidUserTypeException
* The request was rejected because the type of user for the transaction was incorrect.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ChangePassword
* @see AWS API
* Documentation
*/
default ChangePasswordResponse changePassword(ChangePasswordRequest changePasswordRequest) throws NoSuchEntityException,
InvalidUserTypeException, LimitExceededException, EntityTemporarilyUnmodifiableException,
PasswordPolicyViolationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Changes the password of the IAM user who is calling this operation. This operation can be performed using the
* CLI, the Amazon Web Services API, or the My Security Credentials page in the Amazon Web Services
* Management Console. The Amazon Web Services account root user password is not affected by this operation.
*
*
* Use UpdateLoginProfile to use the CLI, the Amazon Web Services API, or the Users page in the IAM
* console to change the password for any IAM user. For more information about modifying passwords, see Managing passwords in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ChangePasswordRequest.Builder} avoiding the need to
* create one manually via {@link ChangePasswordRequest#builder()}
*
*
* @param changePasswordRequest
* A {@link Consumer} that will call methods on {@link ChangePasswordRequest.Builder} to create a request.
* @return Result of the ChangePassword operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidUserTypeException
* The request was rejected because the type of user for the transaction was incorrect.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ChangePassword
* @see AWS API
* Documentation
*/
default ChangePasswordResponse changePassword(Consumer changePasswordRequest)
throws NoSuchEntityException, InvalidUserTypeException, LimitExceededException,
EntityTemporarilyUnmodifiableException, PasswordPolicyViolationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return changePassword(ChangePasswordRequest.builder().applyMutation(changePasswordRequest).build());
}
/**
*
* Creates a new Amazon Web Services secret access key and corresponding Amazon Web Services access key ID for the
* specified user. The default status for new keys is Active
.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials. This is
* true even if the Amazon Web Services account has no associated users.
*
*
* For information about quotas on the number of keys you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation. You must save the key (for example, in a text file) if you want to be able to access it again.
* If a secret key is lost, you can delete the access keys for the associated user and then create new keys.
*
*
*
* @return Result of the CreateAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateAccessKey
* @see #createAccessKey(CreateAccessKeyRequest)
* @see AWS API
* Documentation
*/
default CreateAccessKeyResponse createAccessKey() throws NoSuchEntityException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return createAccessKey(CreateAccessKeyRequest.builder().build());
}
/**
*
* Creates a new Amazon Web Services secret access key and corresponding Amazon Web Services access key ID for the
* specified user. The default status for new keys is Active
.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials. This is
* true even if the Amazon Web Services account has no associated users.
*
*
* For information about quotas on the number of keys you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation. You must save the key (for example, in a text file) if you want to be able to access it again.
* If a secret key is lost, you can delete the access keys for the associated user and then create new keys.
*
*
*
* @param createAccessKeyRequest
* @return Result of the CreateAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateAccessKey
* @see AWS API
* Documentation
*/
default CreateAccessKeyResponse createAccessKey(CreateAccessKeyRequest createAccessKeyRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Amazon Web Services secret access key and corresponding Amazon Web Services access key ID for the
* specified user. The default status for new keys is Active
.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials. This is
* true even if the Amazon Web Services account has no associated users.
*
*
* For information about quotas on the number of keys you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation. You must save the key (for example, in a text file) if you want to be able to access it again.
* If a secret key is lost, you can delete the access keys for the associated user and then create new keys.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAccessKeyRequest.Builder} avoiding the need
* to create one manually via {@link CreateAccessKeyRequest#builder()}
*
*
* @param createAccessKeyRequest
* A {@link Consumer} that will call methods on {@link CreateAccessKeyRequest.Builder} to create a request.
* @return Result of the CreateAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateAccessKey
* @see AWS API
* Documentation
*/
default CreateAccessKeyResponse createAccessKey(Consumer createAccessKeyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return createAccessKey(CreateAccessKeyRequest.builder().applyMutation(createAccessKeyRequest).build());
}
/**
*
* Creates an alias for your Amazon Web Services account. For information about using an Amazon Web Services account
* alias, see Using an alias for your
* Amazon Web Services account ID in the IAM User Guide.
*
*
* @param createAccountAliasRequest
* @return Result of the CreateAccountAlias operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateAccountAlias
* @see AWS API
* Documentation
*/
default CreateAccountAliasResponse createAccountAlias(CreateAccountAliasRequest createAccountAliasRequest)
throws EntityAlreadyExistsException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an alias for your Amazon Web Services account. For information about using an Amazon Web Services account
* alias, see Using an alias for your
* Amazon Web Services account ID in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAccountAliasRequest.Builder} avoiding the
* need to create one manually via {@link CreateAccountAliasRequest#builder()}
*
*
* @param createAccountAliasRequest
* A {@link Consumer} that will call methods on {@link CreateAccountAliasRequest.Builder} to create a
* request.
* @return Result of the CreateAccountAlias operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateAccountAlias
* @see AWS API
* Documentation
*/
default CreateAccountAliasResponse createAccountAlias(Consumer createAccountAliasRequest)
throws EntityAlreadyExistsException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return createAccountAlias(CreateAccountAliasRequest.builder().applyMutation(createAccountAliasRequest).build());
}
/**
*
* Creates a new group.
*
*
* For information about the number of groups you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @param createGroupRequest
* @return Result of the CreateGroup operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateGroup
* @see AWS API
* Documentation
*/
default CreateGroupResponse createGroup(CreateGroupRequest createGroupRequest) throws LimitExceededException,
EntityAlreadyExistsException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new group.
*
*
* For information about the number of groups you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateGroupRequest.Builder} avoiding the need to
* create one manually via {@link CreateGroupRequest#builder()}
*
*
* @param createGroupRequest
* A {@link Consumer} that will call methods on {@link CreateGroupRequest.Builder} to create a request.
* @return Result of the CreateGroup operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateGroup
* @see AWS API
* Documentation
*/
default CreateGroupResponse createGroup(Consumer createGroupRequest)
throws LimitExceededException, EntityAlreadyExistsException, NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return createGroup(CreateGroupRequest.builder().applyMutation(createGroupRequest).build());
}
/**
*
* Creates a new instance profile. For information about instance profiles, see Using roles for
* applications on Amazon EC2 in the IAM User Guide, and Instance profiles in the Amazon EC2 User Guide.
*
*
* For information about the number of instance profiles you can create, see IAM object quotas in the
* IAM User Guide.
*
*
* @param createInstanceProfileRequest
* @return Result of the CreateInstanceProfile operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateInstanceProfile
* @see AWS API
* Documentation
*/
default CreateInstanceProfileResponse createInstanceProfile(CreateInstanceProfileRequest createInstanceProfileRequest)
throws EntityAlreadyExistsException, InvalidInputException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new instance profile. For information about instance profiles, see Using roles for
* applications on Amazon EC2 in the IAM User Guide, and Instance profiles in the Amazon EC2 User Guide.
*
*
* For information about the number of instance profiles you can create, see IAM object quotas in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateInstanceProfileRequest#builder()}
*
*
* @param createInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link CreateInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the CreateInstanceProfile operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateInstanceProfile
* @see AWS API
* Documentation
*/
default CreateInstanceProfileResponse createInstanceProfile(
Consumer createInstanceProfileRequest) throws EntityAlreadyExistsException,
InvalidInputException, LimitExceededException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return createInstanceProfile(CreateInstanceProfileRequest.builder().applyMutation(createInstanceProfileRequest).build());
}
/**
*
* Creates a password for the specified IAM user. A password allows an IAM user to access Amazon Web Services
* services through the Amazon Web Services Management Console.
*
*
* You can use the CLI, the Amazon Web Services API, or the Users page in the IAM console to create a
* password for any IAM user. Use ChangePassword to update your own existing password in the My Security
* Credentials page in the Amazon Web Services Management Console.
*
*
* For more information about managing passwords, see Managing passwords in the
* IAM User Guide.
*
*
* @param createLoginProfileRequest
* @return Result of the CreateLoginProfile operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateLoginProfile
* @see AWS API
* Documentation
*/
default CreateLoginProfileResponse createLoginProfile(CreateLoginProfileRequest createLoginProfileRequest)
throws EntityAlreadyExistsException, NoSuchEntityException, PasswordPolicyViolationException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a password for the specified IAM user. A password allows an IAM user to access Amazon Web Services
* services through the Amazon Web Services Management Console.
*
*
* You can use the CLI, the Amazon Web Services API, or the Users page in the IAM console to create a
* password for any IAM user. Use ChangePassword to update your own existing password in the My Security
* Credentials page in the Amazon Web Services Management Console.
*
*
* For more information about managing passwords, see Managing passwords in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLoginProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateLoginProfileRequest#builder()}
*
*
* @param createLoginProfileRequest
* A {@link Consumer} that will call methods on {@link CreateLoginProfileRequest.Builder} to create a
* request.
* @return Result of the CreateLoginProfile operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateLoginProfile
* @see AWS API
* Documentation
*/
default CreateLoginProfileResponse createLoginProfile(Consumer createLoginProfileRequest)
throws EntityAlreadyExistsException, NoSuchEntityException, PasswordPolicyViolationException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return createLoginProfile(CreateLoginProfileRequest.builder().applyMutation(createLoginProfileRequest).build());
}
/**
*
* Creates an IAM entity to describe an identity provider (IdP) that supports OpenID Connect (OIDC).
*
*
* The OIDC provider that you create with this operation can be used as a principal in a role's trust policy. Such a
* policy establishes a trust relationship between Amazon Web Services and the OIDC provider.
*
*
* If you are using an OIDC identity provider from Google, Facebook, or Amazon Cognito, you don't need to create a
* separate IAM identity provider. These OIDC identity providers are already built-in to Amazon Web Services and are
* available for your use. Instead, you can move directly to creating new roles using your identity provider. To
* learn more, see Creating a role for web
* identity or OpenID connect federation in the IAM User Guide.
*
*
* When you create the IAM OIDC provider, you specify the following:
*
*
* -
*
* The URL of the OIDC identity provider (IdP) to trust
*
*
* -
*
* A list of client IDs (also known as audiences) that identify the application or applications allowed to
* authenticate using the OIDC provider
*
*
* -
*
* A list of thumbprints of one or more server certificates that the IdP uses
*
*
*
*
* You get all of this information from the OIDC IdP you want to use to access Amazon Web Services.
*
*
*
* Amazon Web Services secures communication with some OIDC identity providers (IdPs) through our library of trusted
* certificate authorities (CAs) instead of using a certificate thumbprint to verify your IdP server certificate.
* These OIDC IdPs include Google, Auth0, and those that use an Amazon S3 bucket to host a JSON Web Key Set (JWKS)
* endpoint. In these cases, your legacy thumbprint remains in your configuration, but is no longer used for
* validation.
*
*
*
* The trust for the OIDC provider is derived from the IAM provider that this operation creates. Therefore, it is
* best to limit access to the CreateOpenIDConnectProvider operation to highly privileged users.
*
*
*
* @param createOpenIdConnectProviderRequest
* @return Result of the CreateOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateOpenIDConnectProvider
* @see AWS API Documentation
*/
default CreateOpenIdConnectProviderResponse createOpenIDConnectProvider(
CreateOpenIdConnectProviderRequest createOpenIdConnectProviderRequest) throws InvalidInputException,
EntityAlreadyExistsException, LimitExceededException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an IAM entity to describe an identity provider (IdP) that supports OpenID Connect (OIDC).
*
*
* The OIDC provider that you create with this operation can be used as a principal in a role's trust policy. Such a
* policy establishes a trust relationship between Amazon Web Services and the OIDC provider.
*
*
* If you are using an OIDC identity provider from Google, Facebook, or Amazon Cognito, you don't need to create a
* separate IAM identity provider. These OIDC identity providers are already built-in to Amazon Web Services and are
* available for your use. Instead, you can move directly to creating new roles using your identity provider. To
* learn more, see Creating a role for web
* identity or OpenID connect federation in the IAM User Guide.
*
*
* When you create the IAM OIDC provider, you specify the following:
*
*
* -
*
* The URL of the OIDC identity provider (IdP) to trust
*
*
* -
*
* A list of client IDs (also known as audiences) that identify the application or applications allowed to
* authenticate using the OIDC provider
*
*
* -
*
* A list of thumbprints of one or more server certificates that the IdP uses
*
*
*
*
* You get all of this information from the OIDC IdP you want to use to access Amazon Web Services.
*
*
*
* Amazon Web Services secures communication with some OIDC identity providers (IdPs) through our library of trusted
* certificate authorities (CAs) instead of using a certificate thumbprint to verify your IdP server certificate.
* These OIDC IdPs include Google, Auth0, and those that use an Amazon S3 bucket to host a JSON Web Key Set (JWKS)
* endpoint. In these cases, your legacy thumbprint remains in your configuration, but is no longer used for
* validation.
*
*
*
* The trust for the OIDC provider is derived from the IAM provider that this operation creates. Therefore, it is
* best to limit access to the CreateOpenIDConnectProvider operation to highly privileged users.
*
*
*
* This is a convenience which creates an instance of the {@link CreateOpenIdConnectProviderRequest.Builder}
* avoiding the need to create one manually via {@link CreateOpenIdConnectProviderRequest#builder()}
*
*
* @param createOpenIdConnectProviderRequest
* A {@link Consumer} that will call methods on {@link CreateOpenIDConnectProviderRequest.Builder} to create
* a request.
* @return Result of the CreateOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateOpenIDConnectProvider
* @see AWS API Documentation
*/
default CreateOpenIdConnectProviderResponse createOpenIDConnectProvider(
Consumer createOpenIdConnectProviderRequest)
throws InvalidInputException, EntityAlreadyExistsException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return createOpenIDConnectProvider(CreateOpenIdConnectProviderRequest.builder()
.applyMutation(createOpenIdConnectProviderRequest).build());
}
/**
*
* Creates a new managed policy for your Amazon Web Services account.
*
*
* This operation creates a policy version with a version identifier of v1
and sets v1 as the policy's
* default version. For more information about policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about managed policies in general, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param createPolicyRequest
* @return Result of the CreatePolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreatePolicy
* @see AWS API
* Documentation
*/
default CreatePolicyResponse createPolicy(CreatePolicyRequest createPolicyRequest) throws InvalidInputException,
LimitExceededException, EntityAlreadyExistsException, MalformedPolicyDocumentException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new managed policy for your Amazon Web Services account.
*
*
* This operation creates a policy version with a version identifier of v1
and sets v1 as the policy's
* default version. For more information about policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about managed policies in general, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePolicyRequest.Builder} avoiding the need to
* create one manually via {@link CreatePolicyRequest#builder()}
*
*
* @param createPolicyRequest
* A {@link Consumer} that will call methods on {@link CreatePolicyRequest.Builder} to create a request.
* @return Result of the CreatePolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreatePolicy
* @see AWS API
* Documentation
*/
default CreatePolicyResponse createPolicy(Consumer createPolicyRequest)
throws InvalidInputException, LimitExceededException, EntityAlreadyExistsException, MalformedPolicyDocumentException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return createPolicy(CreatePolicyRequest.builder().applyMutation(createPolicyRequest).build());
}
/**
*
* Creates a new version of the specified managed policy. To update a managed policy, you create a new policy
* version. A managed policy can have up to five versions. If the policy has five versions, you must delete an
* existing version using DeletePolicyVersion before you create a new version.
*
*
* Optionally, you can set the new version as the policy's default version. The default version is the version that
* is in effect for the IAM users, groups, and roles to which the policy is attached.
*
*
* For more information about managed policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
* @param createPolicyVersionRequest
* @return Result of the CreatePolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreatePolicyVersion
* @see AWS API
* Documentation
*/
default CreatePolicyVersionResponse createPolicyVersion(CreatePolicyVersionRequest createPolicyVersionRequest)
throws NoSuchEntityException, MalformedPolicyDocumentException, InvalidInputException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new version of the specified managed policy. To update a managed policy, you create a new policy
* version. A managed policy can have up to five versions. If the policy has five versions, you must delete an
* existing version using DeletePolicyVersion before you create a new version.
*
*
* Optionally, you can set the new version as the policy's default version. The default version is the version that
* is in effect for the IAM users, groups, and roles to which the policy is attached.
*
*
* For more information about managed policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePolicyVersionRequest.Builder} avoiding the
* need to create one manually via {@link CreatePolicyVersionRequest#builder()}
*
*
* @param createPolicyVersionRequest
* A {@link Consumer} that will call methods on {@link CreatePolicyVersionRequest.Builder} to create a
* request.
* @return Result of the CreatePolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreatePolicyVersion
* @see AWS API
* Documentation
*/
default CreatePolicyVersionResponse createPolicyVersion(
Consumer createPolicyVersionRequest) throws NoSuchEntityException,
MalformedPolicyDocumentException, InvalidInputException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return createPolicyVersion(CreatePolicyVersionRequest.builder().applyMutation(createPolicyVersionRequest).build());
}
/**
*
* Creates a new role for your Amazon Web Services account. For more information about roles, see IAM roles. For information
* about quotas for role names and the number of roles you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @param createRoleRequest
* @return Result of the CreateRole operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateRole
* @see AWS API
* Documentation
*/
default CreateRoleResponse createRole(CreateRoleRequest createRoleRequest) throws LimitExceededException,
InvalidInputException, EntityAlreadyExistsException, MalformedPolicyDocumentException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new role for your Amazon Web Services account. For more information about roles, see IAM roles. For information
* about quotas for role names and the number of roles you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRoleRequest.Builder} avoiding the need to
* create one manually via {@link CreateRoleRequest#builder()}
*
*
* @param createRoleRequest
* A {@link Consumer} that will call methods on {@link CreateRoleRequest.Builder} to create a request.
* @return Result of the CreateRole operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateRole
* @see AWS API
* Documentation
*/
default CreateRoleResponse createRole(Consumer createRoleRequest) throws LimitExceededException,
InvalidInputException, EntityAlreadyExistsException, MalformedPolicyDocumentException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return createRole(CreateRoleRequest.builder().applyMutation(createRoleRequest).build());
}
/**
*
* Creates an IAM resource that describes an identity provider (IdP) that supports SAML 2.0.
*
*
* The SAML provider resource that you create with this operation can be used as a principal in an IAM role's trust
* policy. Such a policy can enable federated users who sign in using the SAML IdP to assume the role. You can
* create an IAM role that supports Web-based single sign-on (SSO) to the Amazon Web Services Management Console or
* one that supports API access to Amazon Web Services.
*
*
* When you create the SAML provider resource, you upload a SAML metadata document that you get from your IdP. That
* document includes the issuer's name, expiration information, and keys that can be used to validate the SAML
* authentication response (assertions) that the IdP sends. You must generate the metadata document using the
* identity management software that is used as your organization's IdP.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* For more information, see Enabling SAML
* 2.0 federated users to access the Amazon Web Services Management Console and About SAML 2.0-based
* federation in the IAM User Guide.
*
*
* @param createSamlProviderRequest
* @return Result of the CreateSAMLProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateSAMLProvider
* @see AWS API
* Documentation
*/
default CreateSamlProviderResponse createSAMLProvider(CreateSamlProviderRequest createSamlProviderRequest)
throws InvalidInputException, EntityAlreadyExistsException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an IAM resource that describes an identity provider (IdP) that supports SAML 2.0.
*
*
* The SAML provider resource that you create with this operation can be used as a principal in an IAM role's trust
* policy. Such a policy can enable federated users who sign in using the SAML IdP to assume the role. You can
* create an IAM role that supports Web-based single sign-on (SSO) to the Amazon Web Services Management Console or
* one that supports API access to Amazon Web Services.
*
*
* When you create the SAML provider resource, you upload a SAML metadata document that you get from your IdP. That
* document includes the issuer's name, expiration information, and keys that can be used to validate the SAML
* authentication response (assertions) that the IdP sends. You must generate the metadata document using the
* identity management software that is used as your organization's IdP.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* For more information, see Enabling SAML
* 2.0 federated users to access the Amazon Web Services Management Console and About SAML 2.0-based
* federation in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSamlProviderRequest.Builder} avoiding the
* need to create one manually via {@link CreateSamlProviderRequest#builder()}
*
*
* @param createSamlProviderRequest
* A {@link Consumer} that will call methods on {@link CreateSAMLProviderRequest.Builder} to create a
* request.
* @return Result of the CreateSAMLProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateSAMLProvider
* @see AWS API
* Documentation
*/
default CreateSamlProviderResponse createSAMLProvider(Consumer createSamlProviderRequest)
throws InvalidInputException, EntityAlreadyExistsException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return createSAMLProvider(CreateSamlProviderRequest.builder().applyMutation(createSamlProviderRequest).build());
}
/**
*
* Creates an IAM role that is linked to a specific Amazon Web Services service. The service controls the attached
* policies and when the role can be deleted. This helps ensure that the service is not broken by an unexpectedly
* changed or deleted role, which could put your Amazon Web Services resources into an unknown state. Allowing the
* service to control the role helps improve service stability and proper cleanup when a service and its role are no
* longer needed. For more information, see Using service-linked
* roles in the IAM User Guide.
*
*
* To attach a policy to this service-linked role, you must make the request using the Amazon Web Services service
* that depends on this role.
*
*
* @param createServiceLinkedRoleRequest
* @return Result of the CreateServiceLinkedRole operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateServiceLinkedRole
* @see AWS
* API Documentation
*/
default CreateServiceLinkedRoleResponse createServiceLinkedRole(CreateServiceLinkedRoleRequest createServiceLinkedRoleRequest)
throws InvalidInputException, LimitExceededException, NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an IAM role that is linked to a specific Amazon Web Services service. The service controls the attached
* policies and when the role can be deleted. This helps ensure that the service is not broken by an unexpectedly
* changed or deleted role, which could put your Amazon Web Services resources into an unknown state. Allowing the
* service to control the role helps improve service stability and proper cleanup when a service and its role are no
* longer needed. For more information, see Using service-linked
* roles in the IAM User Guide.
*
*
* To attach a policy to this service-linked role, you must make the request using the Amazon Web Services service
* that depends on this role.
*
*
*
* This is a convenience which creates an instance of the {@link CreateServiceLinkedRoleRequest.Builder} avoiding
* the need to create one manually via {@link CreateServiceLinkedRoleRequest#builder()}
*
*
* @param createServiceLinkedRoleRequest
* A {@link Consumer} that will call methods on {@link CreateServiceLinkedRoleRequest.Builder} to create a
* request.
* @return Result of the CreateServiceLinkedRole operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateServiceLinkedRole
* @see AWS
* API Documentation
*/
default CreateServiceLinkedRoleResponse createServiceLinkedRole(
Consumer createServiceLinkedRoleRequest) throws InvalidInputException,
LimitExceededException, NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return createServiceLinkedRole(CreateServiceLinkedRoleRequest.builder().applyMutation(createServiceLinkedRoleRequest)
.build());
}
/**
*
* Generates a set of credentials consisting of a user name and password that can be used to access the service
* specified in the request. These credentials are generated by IAM, and can be used only for the specified service.
*
*
* You can have a maximum of two sets of service-specific credentials for each supported service per user.
*
*
* You can create service-specific credentials for CodeCommit and Amazon Keyspaces (for Apache Cassandra).
*
*
* You can reset the password to a new service-generated value by calling ResetServiceSpecificCredential.
*
*
* For more information about service-specific credentials, see Using IAM with CodeCommit:
* Git credentials, SSH keys, and Amazon Web Services access keys in the IAM User Guide.
*
*
* @param createServiceSpecificCredentialRequest
* @return Result of the CreateServiceSpecificCredential operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceNotSupportedException
* The specified service does not support service-specific credentials.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateServiceSpecificCredential
* @see AWS API Documentation
*/
default CreateServiceSpecificCredentialResponse createServiceSpecificCredential(
CreateServiceSpecificCredentialRequest createServiceSpecificCredentialRequest) throws LimitExceededException,
NoSuchEntityException, ServiceNotSupportedException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Generates a set of credentials consisting of a user name and password that can be used to access the service
* specified in the request. These credentials are generated by IAM, and can be used only for the specified service.
*
*
* You can have a maximum of two sets of service-specific credentials for each supported service per user.
*
*
* You can create service-specific credentials for CodeCommit and Amazon Keyspaces (for Apache Cassandra).
*
*
* You can reset the password to a new service-generated value by calling ResetServiceSpecificCredential.
*
*
* For more information about service-specific credentials, see Using IAM with CodeCommit:
* Git credentials, SSH keys, and Amazon Web Services access keys in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateServiceSpecificCredentialRequest.Builder}
* avoiding the need to create one manually via {@link CreateServiceSpecificCredentialRequest#builder()}
*
*
* @param createServiceSpecificCredentialRequest
* A {@link Consumer} that will call methods on {@link CreateServiceSpecificCredentialRequest.Builder} to
* create a request.
* @return Result of the CreateServiceSpecificCredential operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceNotSupportedException
* The specified service does not support service-specific credentials.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateServiceSpecificCredential
* @see AWS API Documentation
*/
default CreateServiceSpecificCredentialResponse createServiceSpecificCredential(
Consumer createServiceSpecificCredentialRequest)
throws LimitExceededException, NoSuchEntityException, ServiceNotSupportedException, AwsServiceException,
SdkClientException, IamException {
return createServiceSpecificCredential(CreateServiceSpecificCredentialRequest.builder()
.applyMutation(createServiceSpecificCredentialRequest).build());
}
/**
*
* Creates a new IAM user for your Amazon Web Services account.
*
*
* For information about quotas for the number of IAM users you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateUser
* @see AWS API
* Documentation
*/
default CreateUserResponse createUser(CreateUserRequest createUserRequest) throws LimitExceededException,
EntityAlreadyExistsException, NoSuchEntityException, InvalidInputException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new IAM user for your Amazon Web Services account.
*
*
* For information about quotas for the number of IAM users you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return Result of the CreateUser operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateUser
* @see AWS API
* Documentation
*/
default CreateUserResponse createUser(Consumer createUserRequest) throws LimitExceededException,
EntityAlreadyExistsException, NoSuchEntityException, InvalidInputException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Creates a new virtual MFA device for the Amazon Web Services account. After creating the virtual MFA, use
* EnableMFADevice to attach the MFA device to an IAM user. For more information about creating and working
* with virtual MFA devices, see Using a virtual MFA device in
* the IAM User Guide.
*
*
* For information about the maximum number of MFA devices you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* The seed information contained in the QR code and the Base32 string should be treated like any other secret
* access information. In other words, protect the seed information as you would your Amazon Web Services access
* keys or your passwords. After you provision your virtual device, you should ensure that the information is
* destroyed following secure procedures.
*
*
*
* @param createVirtualMfaDeviceRequest
* @return Result of the CreateVirtualMFADevice operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateVirtualMFADevice
* @see AWS
* API Documentation
*/
default CreateVirtualMfaDeviceResponse createVirtualMFADevice(CreateVirtualMfaDeviceRequest createVirtualMfaDeviceRequest)
throws LimitExceededException, InvalidInputException, EntityAlreadyExistsException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new virtual MFA device for the Amazon Web Services account. After creating the virtual MFA, use
* EnableMFADevice to attach the MFA device to an IAM user. For more information about creating and working
* with virtual MFA devices, see Using a virtual MFA device in
* the IAM User Guide.
*
*
* For information about the maximum number of MFA devices you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* The seed information contained in the QR code and the Base32 string should be treated like any other secret
* access information. In other words, protect the seed information as you would your Amazon Web Services access
* keys or your passwords. After you provision your virtual device, you should ensure that the information is
* destroyed following secure procedures.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVirtualMfaDeviceRequest.Builder} avoiding the
* need to create one manually via {@link CreateVirtualMfaDeviceRequest#builder()}
*
*
* @param createVirtualMfaDeviceRequest
* A {@link Consumer} that will call methods on {@link CreateVirtualMFADeviceRequest.Builder} to create a
* request.
* @return Result of the CreateVirtualMFADevice operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.CreateVirtualMFADevice
* @see AWS
* API Documentation
*/
default CreateVirtualMfaDeviceResponse createVirtualMFADevice(
Consumer createVirtualMfaDeviceRequest) throws LimitExceededException,
InvalidInputException, EntityAlreadyExistsException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return createVirtualMFADevice(CreateVirtualMfaDeviceRequest.builder().applyMutation(createVirtualMfaDeviceRequest)
.build());
}
/**
*
* Deactivates the specified MFA device and removes it from association with the user name for which it was
* originally enabled.
*
*
* For more information about creating and working with virtual MFA devices, see Enabling a virtual multi-factor
* authentication (MFA) device in the IAM User Guide.
*
*
* @param deactivateMfaDeviceRequest
* @return Result of the DeactivateMFADevice operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeactivateMFADevice
* @see AWS API
* Documentation
*/
default DeactivateMfaDeviceResponse deactivateMFADevice(DeactivateMfaDeviceRequest deactivateMfaDeviceRequest)
throws EntityTemporarilyUnmodifiableException, NoSuchEntityException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deactivates the specified MFA device and removes it from association with the user name for which it was
* originally enabled.
*
*
* For more information about creating and working with virtual MFA devices, see Enabling a virtual multi-factor
* authentication (MFA) device in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeactivateMfaDeviceRequest.Builder} avoiding the
* need to create one manually via {@link DeactivateMfaDeviceRequest#builder()}
*
*
* @param deactivateMfaDeviceRequest
* A {@link Consumer} that will call methods on {@link DeactivateMFADeviceRequest.Builder} to create a
* request.
* @return Result of the DeactivateMFADevice operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeactivateMFADevice
* @see AWS API
* Documentation
*/
default DeactivateMfaDeviceResponse deactivateMFADevice(
Consumer deactivateMfaDeviceRequest)
throws EntityTemporarilyUnmodifiableException, NoSuchEntityException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deactivateMFADevice(DeactivateMfaDeviceRequest.builder().applyMutation(deactivateMfaDeviceRequest).build());
}
/**
*
* Deletes the access key pair associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials even if the
* Amazon Web Services account has no associated users.
*
*
* @param deleteAccessKeyRequest
* @return Result of the DeleteAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteAccessKey
* @see AWS API
* Documentation
*/
default DeleteAccessKeyResponse deleteAccessKey(DeleteAccessKeyRequest deleteAccessKeyRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the access key pair associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials even if the
* Amazon Web Services account has no associated users.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccessKeyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAccessKeyRequest#builder()}
*
*
* @param deleteAccessKeyRequest
* A {@link Consumer} that will call methods on {@link DeleteAccessKeyRequest.Builder} to create a request.
* @return Result of the DeleteAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteAccessKey
* @see AWS API
* Documentation
*/
default DeleteAccessKeyResponse deleteAccessKey(Consumer deleteAccessKeyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deleteAccessKey(DeleteAccessKeyRequest.builder().applyMutation(deleteAccessKeyRequest).build());
}
/**
*
* Deletes the specified Amazon Web Services account alias. For information about using an Amazon Web Services
* account alias, see Using an alias
* for your Amazon Web Services account ID in the IAM User Guide.
*
*
* @param deleteAccountAliasRequest
* @return Result of the DeleteAccountAlias operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteAccountAlias
* @see AWS API
* Documentation
*/
default DeleteAccountAliasResponse deleteAccountAlias(DeleteAccountAliasRequest deleteAccountAliasRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified Amazon Web Services account alias. For information about using an Amazon Web Services
* account alias, see Using an alias
* for your Amazon Web Services account ID in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccountAliasRequest.Builder} avoiding the
* need to create one manually via {@link DeleteAccountAliasRequest#builder()}
*
*
* @param deleteAccountAliasRequest
* A {@link Consumer} that will call methods on {@link DeleteAccountAliasRequest.Builder} to create a
* request.
* @return Result of the DeleteAccountAlias operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteAccountAlias
* @see AWS API
* Documentation
*/
default DeleteAccountAliasResponse deleteAccountAlias(Consumer deleteAccountAliasRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deleteAccountAlias(DeleteAccountAliasRequest.builder().applyMutation(deleteAccountAliasRequest).build());
}
/**
*
* Deletes the password policy for the Amazon Web Services account. There are no parameters.
*
*
* @return Result of the DeleteAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteAccountPasswordPolicy
* @see #deleteAccountPasswordPolicy(DeleteAccountPasswordPolicyRequest)
* @see AWS API Documentation
*/
default DeleteAccountPasswordPolicyResponse deleteAccountPasswordPolicy() throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deleteAccountPasswordPolicy(DeleteAccountPasswordPolicyRequest.builder().build());
}
/**
*
* Deletes the password policy for the Amazon Web Services account. There are no parameters.
*
*
* @param deleteAccountPasswordPolicyRequest
* @return Result of the DeleteAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteAccountPasswordPolicy
* @see AWS API Documentation
*/
default DeleteAccountPasswordPolicyResponse deleteAccountPasswordPolicy(
DeleteAccountPasswordPolicyRequest deleteAccountPasswordPolicyRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the password policy for the Amazon Web Services account. There are no parameters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccountPasswordPolicyRequest.Builder}
* avoiding the need to create one manually via {@link DeleteAccountPasswordPolicyRequest#builder()}
*
*
* @param deleteAccountPasswordPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteAccountPasswordPolicyRequest.Builder} to create
* a request.
* @return Result of the DeleteAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteAccountPasswordPolicy
* @see AWS API Documentation
*/
default DeleteAccountPasswordPolicyResponse deleteAccountPasswordPolicy(
Consumer deleteAccountPasswordPolicyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deleteAccountPasswordPolicy(DeleteAccountPasswordPolicyRequest.builder()
.applyMutation(deleteAccountPasswordPolicyRequest).build());
}
/**
*
* Deletes the specified IAM group. The group must not contain any users or have any attached policies.
*
*
* @param deleteGroupRequest
* @return Result of the DeleteGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteGroup
* @see AWS API
* Documentation
*/
default DeleteGroupResponse deleteGroup(DeleteGroupRequest deleteGroupRequest) throws NoSuchEntityException,
DeleteConflictException, LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified IAM group. The group must not contain any users or have any attached policies.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGroupRequest.Builder} avoiding the need to
* create one manually via {@link DeleteGroupRequest#builder()}
*
*
* @param deleteGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteGroupRequest.Builder} to create a request.
* @return Result of the DeleteGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteGroup
* @see AWS API
* Documentation
*/
default DeleteGroupResponse deleteGroup(Consumer deleteGroupRequest)
throws NoSuchEntityException, DeleteConflictException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return deleteGroup(DeleteGroupRequest.builder().applyMutation(deleteGroupRequest).build());
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM group.
*
*
* A group can also have managed policies attached to it. To detach a managed policy from a group, use
* DetachGroupPolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param deleteGroupPolicyRequest
* @return Result of the DeleteGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteGroupPolicy
* @see AWS API
* Documentation
*/
default DeleteGroupPolicyResponse deleteGroupPolicy(DeleteGroupPolicyRequest deleteGroupPolicyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM group.
*
*
* A group can also have managed policies attached to it. To detach a managed policy from a group, use
* DetachGroupPolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGroupPolicyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteGroupPolicyRequest#builder()}
*
*
* @param deleteGroupPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteGroupPolicyRequest.Builder} to create a request.
* @return Result of the DeleteGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteGroupPolicy
* @see AWS API
* Documentation
*/
default DeleteGroupPolicyResponse deleteGroupPolicy(Consumer deleteGroupPolicyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deleteGroupPolicy(DeleteGroupPolicyRequest.builder().applyMutation(deleteGroupPolicyRequest).build());
}
/**
*
* Deletes the specified instance profile. The instance profile must not have an associated role.
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the instance profile you are about to
* delete. Deleting a role or instance profile that is associated with a running instance will break any
* applications running on the instance.
*
*
*
* For more information about instance profiles, see About instance profiles.
*
*
* @param deleteInstanceProfileRequest
* @return Result of the DeleteInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteInstanceProfile
* @see AWS API
* Documentation
*/
default DeleteInstanceProfileResponse deleteInstanceProfile(DeleteInstanceProfileRequest deleteInstanceProfileRequest)
throws NoSuchEntityException, DeleteConflictException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified instance profile. The instance profile must not have an associated role.
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the instance profile you are about to
* delete. Deleting a role or instance profile that is associated with a running instance will break any
* applications running on the instance.
*
*
*
* For more information about instance profiles, see About instance profiles.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link DeleteInstanceProfileRequest#builder()}
*
*
* @param deleteInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the DeleteInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteInstanceProfile
* @see AWS API
* Documentation
*/
default DeleteInstanceProfileResponse deleteInstanceProfile(
Consumer deleteInstanceProfileRequest) throws NoSuchEntityException,
DeleteConflictException, LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return deleteInstanceProfile(DeleteInstanceProfileRequest.builder().applyMutation(deleteInstanceProfileRequest).build());
}
/**
*
* Deletes the password for the specified IAM user, For more information, see Managing
* passwords for IAM users.
*
*
* You can use the CLI, the Amazon Web Services API, or the Users page in the IAM console to delete a
* password for any IAM user. You can use ChangePassword to update, but not delete, your own password in the
* My Security Credentials page in the Amazon Web Services Management Console.
*
*
*
* Deleting a user's password does not prevent a user from accessing Amazon Web Services through the command line
* interface or the API. To prevent all user access, you must also either make any access keys inactive or delete
* them. For more information about making keys inactive or deleting them, see UpdateAccessKey and
* DeleteAccessKey.
*
*
*
* @param deleteLoginProfileRequest
* @return Result of the DeleteLoginProfile operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteLoginProfile
* @see AWS API
* Documentation
*/
default DeleteLoginProfileResponse deleteLoginProfile(DeleteLoginProfileRequest deleteLoginProfileRequest)
throws EntityTemporarilyUnmodifiableException, NoSuchEntityException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the password for the specified IAM user, For more information, see Managing
* passwords for IAM users.
*
*
* You can use the CLI, the Amazon Web Services API, or the Users page in the IAM console to delete a
* password for any IAM user. You can use ChangePassword to update, but not delete, your own password in the
* My Security Credentials page in the Amazon Web Services Management Console.
*
*
*
* Deleting a user's password does not prevent a user from accessing Amazon Web Services through the command line
* interface or the API. To prevent all user access, you must also either make any access keys inactive or delete
* them. For more information about making keys inactive or deleting them, see UpdateAccessKey and
* DeleteAccessKey.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLoginProfileRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLoginProfileRequest#builder()}
*
*
* @param deleteLoginProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteLoginProfileRequest.Builder} to create a
* request.
* @return Result of the DeleteLoginProfile operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteLoginProfile
* @see AWS API
* Documentation
*/
default DeleteLoginProfileResponse deleteLoginProfile(Consumer deleteLoginProfileRequest)
throws EntityTemporarilyUnmodifiableException, NoSuchEntityException, LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deleteLoginProfile(DeleteLoginProfileRequest.builder().applyMutation(deleteLoginProfileRequest).build());
}
/**
*
* Deletes an OpenID Connect identity provider (IdP) resource object in IAM.
*
*
* Deleting an IAM OIDC provider resource does not update any roles that reference the provider as a principal in
* their trust policies. Any attempt to assume a role that references a deleted provider fails.
*
*
* This operation is idempotent; it does not fail or return an error if you call the operation for a provider that
* does not exist.
*
*
* @param deleteOpenIdConnectProviderRequest
* @return Result of the DeleteOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteOpenIDConnectProvider
* @see AWS API Documentation
*/
default DeleteOpenIdConnectProviderResponse deleteOpenIDConnectProvider(
DeleteOpenIdConnectProviderRequest deleteOpenIdConnectProviderRequest) throws InvalidInputException,
NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an OpenID Connect identity provider (IdP) resource object in IAM.
*
*
* Deleting an IAM OIDC provider resource does not update any roles that reference the provider as a principal in
* their trust policies. Any attempt to assume a role that references a deleted provider fails.
*
*
* This operation is idempotent; it does not fail or return an error if you call the operation for a provider that
* does not exist.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteOpenIdConnectProviderRequest.Builder}
* avoiding the need to create one manually via {@link DeleteOpenIdConnectProviderRequest#builder()}
*
*
* @param deleteOpenIdConnectProviderRequest
* A {@link Consumer} that will call methods on {@link DeleteOpenIDConnectProviderRequest.Builder} to create
* a request.
* @return Result of the DeleteOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteOpenIDConnectProvider
* @see AWS API Documentation
*/
default DeleteOpenIdConnectProviderResponse deleteOpenIDConnectProvider(
Consumer deleteOpenIdConnectProviderRequest)
throws InvalidInputException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deleteOpenIDConnectProvider(DeleteOpenIdConnectProviderRequest.builder()
.applyMutation(deleteOpenIdConnectProviderRequest).build());
}
/**
*
* Deletes the specified managed policy.
*
*
* Before you can delete a managed policy, you must first detach the policy from all users, groups, and roles that
* it is attached to. In addition, you must delete all the policy's versions. The following steps describe the
* process for deleting a managed policy:
*
*
* -
*
* Detach the policy from all users, groups, and roles that the policy is attached to, using
* DetachUserPolicy, DetachGroupPolicy, or DetachRolePolicy. To list all the users, groups, and
* roles that a policy is attached to, use ListEntitiesForPolicy.
*
*
* -
*
* Delete all versions of the policy using DeletePolicyVersion. To list the policy's versions, use
* ListPolicyVersions. You cannot use DeletePolicyVersion to delete the version that is marked as the
* default version. You delete the policy's default version in the next step of the process.
*
*
* -
*
* Delete the policy (this automatically deletes the policy's default version) using this operation.
*
*
*
*
* For information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param deletePolicyRequest
* @return Result of the DeletePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeletePolicy
* @see AWS API
* Documentation
*/
default DeletePolicyResponse deletePolicy(DeletePolicyRequest deletePolicyRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, DeleteConflictException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified managed policy.
*
*
* Before you can delete a managed policy, you must first detach the policy from all users, groups, and roles that
* it is attached to. In addition, you must delete all the policy's versions. The following steps describe the
* process for deleting a managed policy:
*
*
* -
*
* Detach the policy from all users, groups, and roles that the policy is attached to, using
* DetachUserPolicy, DetachGroupPolicy, or DetachRolePolicy. To list all the users, groups, and
* roles that a policy is attached to, use ListEntitiesForPolicy.
*
*
* -
*
* Delete all versions of the policy using DeletePolicyVersion. To list the policy's versions, use
* ListPolicyVersions. You cannot use DeletePolicyVersion to delete the version that is marked as the
* default version. You delete the policy's default version in the next step of the process.
*
*
* -
*
* Delete the policy (this automatically deletes the policy's default version) using this operation.
*
*
*
*
* For information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePolicyRequest.Builder} avoiding the need to
* create one manually via {@link DeletePolicyRequest#builder()}
*
*
* @param deletePolicyRequest
* A {@link Consumer} that will call methods on {@link DeletePolicyRequest.Builder} to create a request.
* @return Result of the DeletePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeletePolicy
* @see AWS API
* Documentation
*/
default DeletePolicyResponse deletePolicy(Consumer deletePolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, DeleteConflictException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deletePolicy(DeletePolicyRequest.builder().applyMutation(deletePolicyRequest).build());
}
/**
*
* Deletes the specified version from the specified managed policy.
*
*
* You cannot delete the default version from a policy using this operation. To delete the default version from a
* policy, use DeletePolicy. To find out which version of a policy is marked as the default version, use
* ListPolicyVersions.
*
*
* For information about versions for managed policies, see Versioning for managed
* policies in the IAM User Guide.
*
*
* @param deletePolicyVersionRequest
* @return Result of the DeletePolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeletePolicyVersion
* @see AWS API
* Documentation
*/
default DeletePolicyVersionResponse deletePolicyVersion(DeletePolicyVersionRequest deletePolicyVersionRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, DeleteConflictException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified version from the specified managed policy.
*
*
* You cannot delete the default version from a policy using this operation. To delete the default version from a
* policy, use DeletePolicy. To find out which version of a policy is marked as the default version, use
* ListPolicyVersions.
*
*
* For information about versions for managed policies, see Versioning for managed
* policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePolicyVersionRequest.Builder} avoiding the
* need to create one manually via {@link DeletePolicyVersionRequest#builder()}
*
*
* @param deletePolicyVersionRequest
* A {@link Consumer} that will call methods on {@link DeletePolicyVersionRequest.Builder} to create a
* request.
* @return Result of the DeletePolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeletePolicyVersion
* @see AWS API
* Documentation
*/
default DeletePolicyVersionResponse deletePolicyVersion(
Consumer deletePolicyVersionRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, DeleteConflictException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deletePolicyVersion(DeletePolicyVersionRequest.builder().applyMutation(deletePolicyVersionRequest).build());
}
/**
*
* Deletes the specified role. Unlike the Amazon Web Services Management Console, when you delete a role
* programmatically, you must delete the items attached to the role manually, or the deletion fails. For more
* information, see Deleting an IAM role. Before attempting to delete a role, remove the following attached items:
*
*
* -
*
* Inline policies (DeleteRolePolicy)
*
*
* -
*
* Attached managed policies (DetachRolePolicy)
*
*
* -
*
* Instance profile (RemoveRoleFromInstanceProfile)
*
*
* -
*
* Optional – Delete instance profile after detaching from role for resource clean up (DeleteInstanceProfile)
*
*
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the role you are about to delete. Deleting a
* role or instance profile that is associated with a running instance will break any applications running on the
* instance.
*
*
*
* @param deleteRoleRequest
* @return Result of the DeleteRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteRole
* @see AWS API
* Documentation
*/
default DeleteRoleResponse deleteRole(DeleteRoleRequest deleteRoleRequest) throws NoSuchEntityException,
DeleteConflictException, LimitExceededException, UnmodifiableEntityException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified role. Unlike the Amazon Web Services Management Console, when you delete a role
* programmatically, you must delete the items attached to the role manually, or the deletion fails. For more
* information, see Deleting an IAM role. Before attempting to delete a role, remove the following attached items:
*
*
* -
*
* Inline policies (DeleteRolePolicy)
*
*
* -
*
* Attached managed policies (DetachRolePolicy)
*
*
* -
*
* Instance profile (RemoveRoleFromInstanceProfile)
*
*
* -
*
* Optional – Delete instance profile after detaching from role for resource clean up (DeleteInstanceProfile)
*
*
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the role you are about to delete. Deleting a
* role or instance profile that is associated with a running instance will break any applications running on the
* instance.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRoleRequest.Builder} avoiding the need to
* create one manually via {@link DeleteRoleRequest#builder()}
*
*
* @param deleteRoleRequest
* A {@link Consumer} that will call methods on {@link DeleteRoleRequest.Builder} to create a request.
* @return Result of the DeleteRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteRole
* @see AWS API
* Documentation
*/
default DeleteRoleResponse deleteRole(Consumer deleteRoleRequest) throws NoSuchEntityException,
DeleteConflictException, LimitExceededException, UnmodifiableEntityException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deleteRole(DeleteRoleRequest.builder().applyMutation(deleteRoleRequest).build());
}
/**
*
* Deletes the permissions boundary for the specified IAM role.
*
*
*
* Deleting the permissions boundary for a role might increase its permissions. For example, it might allow anyone
* who assumes the role to perform all the actions granted in its permissions policies.
*
*
*
* @param deleteRolePermissionsBoundaryRequest
* @return Result of the DeleteRolePermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteRolePermissionsBoundary
* @see AWS API Documentation
*/
default DeleteRolePermissionsBoundaryResponse deleteRolePermissionsBoundary(
DeleteRolePermissionsBoundaryRequest deleteRolePermissionsBoundaryRequest) throws NoSuchEntityException,
UnmodifiableEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the permissions boundary for the specified IAM role.
*
*
*
* Deleting the permissions boundary for a role might increase its permissions. For example, it might allow anyone
* who assumes the role to perform all the actions granted in its permissions policies.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRolePermissionsBoundaryRequest.Builder}
* avoiding the need to create one manually via {@link DeleteRolePermissionsBoundaryRequest#builder()}
*
*
* @param deleteRolePermissionsBoundaryRequest
* A {@link Consumer} that will call methods on {@link DeleteRolePermissionsBoundaryRequest.Builder} to
* create a request.
* @return Result of the DeleteRolePermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteRolePermissionsBoundary
* @see AWS API Documentation
*/
default DeleteRolePermissionsBoundaryResponse deleteRolePermissionsBoundary(
Consumer deleteRolePermissionsBoundaryRequest)
throws NoSuchEntityException, UnmodifiableEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deleteRolePermissionsBoundary(DeleteRolePermissionsBoundaryRequest.builder()
.applyMutation(deleteRolePermissionsBoundaryRequest).build());
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM role.
*
*
* A role can also have managed policies attached to it. To detach a managed policy from a role, use
* DetachRolePolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param deleteRolePolicyRequest
* @return Result of the DeleteRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteRolePolicy
* @see AWS API
* Documentation
*/
default DeleteRolePolicyResponse deleteRolePolicy(DeleteRolePolicyRequest deleteRolePolicyRequest)
throws NoSuchEntityException, LimitExceededException, UnmodifiableEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM role.
*
*
* A role can also have managed policies attached to it. To detach a managed policy from a role, use
* DetachRolePolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRolePolicyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteRolePolicyRequest#builder()}
*
*
* @param deleteRolePolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteRolePolicyRequest.Builder} to create a request.
* @return Result of the DeleteRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteRolePolicy
* @see AWS API
* Documentation
*/
default DeleteRolePolicyResponse deleteRolePolicy(Consumer deleteRolePolicyRequest)
throws NoSuchEntityException, LimitExceededException, UnmodifiableEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return deleteRolePolicy(DeleteRolePolicyRequest.builder().applyMutation(deleteRolePolicyRequest).build());
}
/**
*
* Deletes a SAML provider resource in IAM.
*
*
* Deleting the provider resource from IAM does not update any roles that reference the SAML provider resource's ARN
* as a principal in their trust policies. Any attempt to assume a role that references a non-existent provider
* resource ARN fails.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param deleteSamlProviderRequest
* @return Result of the DeleteSAMLProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteSAMLProvider
* @see AWS API
* Documentation
*/
default DeleteSamlProviderResponse deleteSAMLProvider(DeleteSamlProviderRequest deleteSamlProviderRequest)
throws InvalidInputException, LimitExceededException, NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a SAML provider resource in IAM.
*
*
* Deleting the provider resource from IAM does not update any roles that reference the SAML provider resource's ARN
* as a principal in their trust policies. Any attempt to assume a role that references a non-existent provider
* resource ARN fails.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSamlProviderRequest.Builder} avoiding the
* need to create one manually via {@link DeleteSamlProviderRequest#builder()}
*
*
* @param deleteSamlProviderRequest
* A {@link Consumer} that will call methods on {@link DeleteSAMLProviderRequest.Builder} to create a
* request.
* @return Result of the DeleteSAMLProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteSAMLProvider
* @see AWS API
* Documentation
*/
default DeleteSamlProviderResponse deleteSAMLProvider(Consumer deleteSamlProviderRequest)
throws InvalidInputException, LimitExceededException, NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return deleteSAMLProvider(DeleteSamlProviderRequest.builder().applyMutation(deleteSamlProviderRequest).build());
}
/**
*
* Deletes the specified SSH public key.
*
*
* The SSH public key deleted by this operation is used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
* @param deleteSshPublicKeyRequest
* @return Result of the DeleteSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteSSHPublicKey
* @see AWS API
* Documentation
*/
default DeleteSshPublicKeyResponse deleteSSHPublicKey(DeleteSshPublicKeyRequest deleteSshPublicKeyRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified SSH public key.
*
*
* The SSH public key deleted by this operation is used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSshPublicKeyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteSshPublicKeyRequest#builder()}
*
*
* @param deleteSshPublicKeyRequest
* A {@link Consumer} that will call methods on {@link DeleteSSHPublicKeyRequest.Builder} to create a
* request.
* @return Result of the DeleteSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteSSHPublicKey
* @see AWS API
* Documentation
*/
default DeleteSshPublicKeyResponse deleteSSHPublicKey(Consumer deleteSshPublicKeyRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
return deleteSSHPublicKey(DeleteSshPublicKeyRequest.builder().applyMutation(deleteSshPublicKeyRequest).build());
}
/**
*
* Deletes the specified server certificate.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* If you are using a server certificate with Elastic Load Balancing, deleting the certificate could have
* implications for your application. If Elastic Load Balancing doesn't detect the deletion of bound certificates,
* it may continue to use the certificates. This could cause Elastic Load Balancing to stop accepting traffic. We
* recommend that you remove the reference to the certificate from Elastic Load Balancing before using this command
* to delete the certificate. For more information, see DeleteLoadBalancerListeners in the Elastic Load Balancing API Reference.
*
*
*
* @param deleteServerCertificateRequest
* @return Result of the DeleteServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteServerCertificate
* @see AWS
* API Documentation
*/
default DeleteServerCertificateResponse deleteServerCertificate(DeleteServerCertificateRequest deleteServerCertificateRequest)
throws NoSuchEntityException, DeleteConflictException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified server certificate.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* If you are using a server certificate with Elastic Load Balancing, deleting the certificate could have
* implications for your application. If Elastic Load Balancing doesn't detect the deletion of bound certificates,
* it may continue to use the certificates. This could cause Elastic Load Balancing to stop accepting traffic. We
* recommend that you remove the reference to the certificate from Elastic Load Balancing before using this command
* to delete the certificate. For more information, see DeleteLoadBalancerListeners in the Elastic Load Balancing API Reference.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServerCertificateRequest.Builder} avoiding
* the need to create one manually via {@link DeleteServerCertificateRequest#builder()}
*
*
* @param deleteServerCertificateRequest
* A {@link Consumer} that will call methods on {@link DeleteServerCertificateRequest.Builder} to create a
* request.
* @return Result of the DeleteServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteServerCertificate
* @see AWS
* API Documentation
*/
default DeleteServerCertificateResponse deleteServerCertificate(
Consumer deleteServerCertificateRequest) throws NoSuchEntityException,
DeleteConflictException, LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return deleteServerCertificate(DeleteServerCertificateRequest.builder().applyMutation(deleteServerCertificateRequest)
.build());
}
/**
*
* Submits a service-linked role deletion request and returns a DeletionTaskId
, which you can use to
* check the status of the deletion. Before you call this operation, confirm that the role has no active sessions
* and that any resources used by the role in the linked service are deleted. If you call this operation more than
* once for the same service-linked role and an earlier deletion task is not complete, then the
* DeletionTaskId
of the earlier request is returned.
*
*
* If you submit a deletion request for a service-linked role whose linked service is still accessing a resource,
* then the deletion task fails. If it fails, the GetServiceLinkedRoleDeletionStatus operation returns the
* reason for the failure, usually including the resources that must be deleted. To delete the service-linked role,
* you must first remove those resources from the linked service and then submit the deletion request again.
* Resources are specific to the service that is linked to the role. For more information about removing resources
* from a service, see the Amazon Web Services documentation for your
* service.
*
*
* For more information about service-linked roles, see Roles terms and concepts: Amazon Web Services service-linked role in the IAM User Guide.
*
*
* @param deleteServiceLinkedRoleRequest
* @return Result of the DeleteServiceLinkedRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteServiceLinkedRole
* @see AWS
* API Documentation
*/
default DeleteServiceLinkedRoleResponse deleteServiceLinkedRole(DeleteServiceLinkedRoleRequest deleteServiceLinkedRoleRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Submits a service-linked role deletion request and returns a DeletionTaskId
, which you can use to
* check the status of the deletion. Before you call this operation, confirm that the role has no active sessions
* and that any resources used by the role in the linked service are deleted. If you call this operation more than
* once for the same service-linked role and an earlier deletion task is not complete, then the
* DeletionTaskId
of the earlier request is returned.
*
*
* If you submit a deletion request for a service-linked role whose linked service is still accessing a resource,
* then the deletion task fails. If it fails, the GetServiceLinkedRoleDeletionStatus operation returns the
* reason for the failure, usually including the resources that must be deleted. To delete the service-linked role,
* you must first remove those resources from the linked service and then submit the deletion request again.
* Resources are specific to the service that is linked to the role. For more information about removing resources
* from a service, see the Amazon Web Services documentation for your
* service.
*
*
* For more information about service-linked roles, see Roles terms and concepts: Amazon Web Services service-linked role in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServiceLinkedRoleRequest.Builder} avoiding
* the need to create one manually via {@link DeleteServiceLinkedRoleRequest#builder()}
*
*
* @param deleteServiceLinkedRoleRequest
* A {@link Consumer} that will call methods on {@link DeleteServiceLinkedRoleRequest.Builder} to create a
* request.
* @return Result of the DeleteServiceLinkedRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteServiceLinkedRole
* @see AWS
* API Documentation
*/
default DeleteServiceLinkedRoleResponse deleteServiceLinkedRole(
Consumer deleteServiceLinkedRoleRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deleteServiceLinkedRole(DeleteServiceLinkedRoleRequest.builder().applyMutation(deleteServiceLinkedRoleRequest)
.build());
}
/**
*
* Deletes the specified service-specific credential.
*
*
* @param deleteServiceSpecificCredentialRequest
* @return Result of the DeleteServiceSpecificCredential operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteServiceSpecificCredential
* @see AWS API Documentation
*/
default DeleteServiceSpecificCredentialResponse deleteServiceSpecificCredential(
DeleteServiceSpecificCredentialRequest deleteServiceSpecificCredentialRequest) throws NoSuchEntityException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified service-specific credential.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServiceSpecificCredentialRequest.Builder}
* avoiding the need to create one manually via {@link DeleteServiceSpecificCredentialRequest#builder()}
*
*
* @param deleteServiceSpecificCredentialRequest
* A {@link Consumer} that will call methods on {@link DeleteServiceSpecificCredentialRequest.Builder} to
* create a request.
* @return Result of the DeleteServiceSpecificCredential operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteServiceSpecificCredential
* @see AWS API Documentation
*/
default DeleteServiceSpecificCredentialResponse deleteServiceSpecificCredential(
Consumer deleteServiceSpecificCredentialRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
return deleteServiceSpecificCredential(DeleteServiceSpecificCredentialRequest.builder()
.applyMutation(deleteServiceSpecificCredentialRequest).build());
}
/**
*
* Deletes a signing certificate associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials even if the
* Amazon Web Services account has no associated IAM users.
*
*
* @param deleteSigningCertificateRequest
* @return Result of the DeleteSigningCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteSigningCertificate
* @see AWS
* API Documentation
*/
default DeleteSigningCertificateResponse deleteSigningCertificate(
DeleteSigningCertificateRequest deleteSigningCertificateRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a signing certificate associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials even if the
* Amazon Web Services account has no associated IAM users.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSigningCertificateRequest.Builder} avoiding
* the need to create one manually via {@link DeleteSigningCertificateRequest#builder()}
*
*
* @param deleteSigningCertificateRequest
* A {@link Consumer} that will call methods on {@link DeleteSigningCertificateRequest.Builder} to create a
* request.
* @return Result of the DeleteSigningCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteSigningCertificate
* @see AWS
* API Documentation
*/
default DeleteSigningCertificateResponse deleteSigningCertificate(
Consumer deleteSigningCertificateRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deleteSigningCertificate(DeleteSigningCertificateRequest.builder().applyMutation(deleteSigningCertificateRequest)
.build());
}
/**
*
* Deletes the specified IAM user. Unlike the Amazon Web Services Management Console, when you delete a user
* programmatically, you must delete the items attached to the user manually, or the deletion fails. For more
* information, see Deleting an
* IAM user. Before attempting to delete a user, remove the following items:
*
*
* -
*
* Password (DeleteLoginProfile)
*
*
* -
*
* Access keys (DeleteAccessKey)
*
*
* -
*
* Signing certificate (DeleteSigningCertificate)
*
*
* -
*
* SSH public key (DeleteSSHPublicKey)
*
*
* -
*
* Git credentials (DeleteServiceSpecificCredential)
*
*
* -
*
* Multi-factor authentication (MFA) device (DeactivateMFADevice, DeleteVirtualMFADevice)
*
*
* -
*
* Inline policies (DeleteUserPolicy)
*
*
* -
*
* Attached managed policies (DetachUserPolicy)
*
*
* -
*
* Group memberships (RemoveUserFromGroup)
*
*
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteUser
* @see AWS API
* Documentation
*/
default DeleteUserResponse deleteUser(DeleteUserRequest deleteUserRequest) throws LimitExceededException,
NoSuchEntityException, DeleteConflictException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified IAM user. Unlike the Amazon Web Services Management Console, when you delete a user
* programmatically, you must delete the items attached to the user manually, or the deletion fails. For more
* information, see Deleting an
* IAM user. Before attempting to delete a user, remove the following items:
*
*
* -
*
* Password (DeleteLoginProfile)
*
*
* -
*
* Access keys (DeleteAccessKey)
*
*
* -
*
* Signing certificate (DeleteSigningCertificate)
*
*
* -
*
* SSH public key (DeleteSSHPublicKey)
*
*
* -
*
* Git credentials (DeleteServiceSpecificCredential)
*
*
* -
*
* Multi-factor authentication (MFA) device (DeactivateMFADevice, DeleteVirtualMFADevice)
*
*
* -
*
* Inline policies (DeleteUserPolicy)
*
*
* -
*
* Attached managed policies (DetachUserPolicy)
*
*
* -
*
* Group memberships (RemoveUserFromGroup)
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on {@link DeleteUserRequest.Builder} to create a request.
* @return Result of the DeleteUser operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteUser
* @see AWS API
* Documentation
*/
default DeleteUserResponse deleteUser(Consumer deleteUserRequest) throws LimitExceededException,
NoSuchEntityException, DeleteConflictException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Deletes the permissions boundary for the specified IAM user.
*
*
*
* Deleting the permissions boundary for a user might increase its permissions by allowing the user to perform all
* the actions granted in its permissions policies.
*
*
*
* @param deleteUserPermissionsBoundaryRequest
* @return Result of the DeleteUserPermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteUserPermissionsBoundary
* @see AWS API Documentation
*/
default DeleteUserPermissionsBoundaryResponse deleteUserPermissionsBoundary(
DeleteUserPermissionsBoundaryRequest deleteUserPermissionsBoundaryRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the permissions boundary for the specified IAM user.
*
*
*
* Deleting the permissions boundary for a user might increase its permissions by allowing the user to perform all
* the actions granted in its permissions policies.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserPermissionsBoundaryRequest.Builder}
* avoiding the need to create one manually via {@link DeleteUserPermissionsBoundaryRequest#builder()}
*
*
* @param deleteUserPermissionsBoundaryRequest
* A {@link Consumer} that will call methods on {@link DeleteUserPermissionsBoundaryRequest.Builder} to
* create a request.
* @return Result of the DeleteUserPermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteUserPermissionsBoundary
* @see AWS API Documentation
*/
default DeleteUserPermissionsBoundaryResponse deleteUserPermissionsBoundary(
Consumer deleteUserPermissionsBoundaryRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return deleteUserPermissionsBoundary(DeleteUserPermissionsBoundaryRequest.builder()
.applyMutation(deleteUserPermissionsBoundaryRequest).build());
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM user.
*
*
* A user can also have managed policies attached to it. To detach a managed policy from a user, use
* DetachUserPolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param deleteUserPolicyRequest
* @return Result of the DeleteUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteUserPolicy
* @see AWS API
* Documentation
*/
default DeleteUserPolicyResponse deleteUserPolicy(DeleteUserPolicyRequest deleteUserPolicyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM user.
*
*
* A user can also have managed policies attached to it. To detach a managed policy from a user, use
* DetachUserPolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserPolicyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteUserPolicyRequest#builder()}
*
*
* @param deleteUserPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteUserPolicyRequest.Builder} to create a request.
* @return Result of the DeleteUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteUserPolicy
* @see AWS API
* Documentation
*/
default DeleteUserPolicyResponse deleteUserPolicy(Consumer deleteUserPolicyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return deleteUserPolicy(DeleteUserPolicyRequest.builder().applyMutation(deleteUserPolicyRequest).build());
}
/**
*
* Deletes a virtual MFA device.
*
*
*
* You must deactivate a user's virtual MFA device before you can delete it. For information about deactivating MFA
* devices, see DeactivateMFADevice.
*
*
*
* @param deleteVirtualMfaDeviceRequest
* @return Result of the DeleteVirtualMFADevice operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteVirtualMFADevice
* @see AWS
* API Documentation
*/
default DeleteVirtualMfaDeviceResponse deleteVirtualMFADevice(DeleteVirtualMfaDeviceRequest deleteVirtualMfaDeviceRequest)
throws NoSuchEntityException, DeleteConflictException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a virtual MFA device.
*
*
*
* You must deactivate a user's virtual MFA device before you can delete it. For information about deactivating MFA
* devices, see DeactivateMFADevice.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVirtualMfaDeviceRequest.Builder} avoiding the
* need to create one manually via {@link DeleteVirtualMfaDeviceRequest#builder()}
*
*
* @param deleteVirtualMfaDeviceRequest
* A {@link Consumer} that will call methods on {@link DeleteVirtualMFADeviceRequest.Builder} to create a
* request.
* @return Result of the DeleteVirtualMFADevice operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DeleteVirtualMFADevice
* @see AWS
* API Documentation
*/
default DeleteVirtualMfaDeviceResponse deleteVirtualMFADevice(
Consumer deleteVirtualMfaDeviceRequest) throws NoSuchEntityException,
DeleteConflictException, LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return deleteVirtualMFADevice(DeleteVirtualMfaDeviceRequest.builder().applyMutation(deleteVirtualMfaDeviceRequest)
.build());
}
/**
*
* Removes the specified managed policy from the specified IAM group.
*
*
* A group can also have inline policies embedded with it. To delete an inline policy, use DeleteGroupPolicy.
* For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param detachGroupPolicyRequest
* @return Result of the DetachGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DetachGroupPolicy
* @see AWS API
* Documentation
*/
default DetachGroupPolicyResponse detachGroupPolicy(DetachGroupPolicyRequest detachGroupPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified managed policy from the specified IAM group.
*
*
* A group can also have inline policies embedded with it. To delete an inline policy, use DeleteGroupPolicy.
* For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DetachGroupPolicyRequest.Builder} avoiding the need
* to create one manually via {@link DetachGroupPolicyRequest#builder()}
*
*
* @param detachGroupPolicyRequest
* A {@link Consumer} that will call methods on {@link DetachGroupPolicyRequest.Builder} to create a request.
* @return Result of the DetachGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DetachGroupPolicy
* @see AWS API
* Documentation
*/
default DetachGroupPolicyResponse detachGroupPolicy(Consumer detachGroupPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return detachGroupPolicy(DetachGroupPolicyRequest.builder().applyMutation(detachGroupPolicyRequest).build());
}
/**
*
* Removes the specified managed policy from the specified role.
*
*
* A role can also have inline policies embedded with it. To delete an inline policy, use DeleteRolePolicy.
* For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param detachRolePolicyRequest
* @return Result of the DetachRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DetachRolePolicy
* @see AWS API
* Documentation
*/
default DetachRolePolicyResponse detachRolePolicy(DetachRolePolicyRequest detachRolePolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, UnmodifiableEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified managed policy from the specified role.
*
*
* A role can also have inline policies embedded with it. To delete an inline policy, use DeleteRolePolicy.
* For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DetachRolePolicyRequest.Builder} avoiding the need
* to create one manually via {@link DetachRolePolicyRequest#builder()}
*
*
* @param detachRolePolicyRequest
* A {@link Consumer} that will call methods on {@link DetachRolePolicyRequest.Builder} to create a request.
* @return Result of the DetachRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DetachRolePolicy
* @see AWS API
* Documentation
*/
default DetachRolePolicyResponse detachRolePolicy(Consumer detachRolePolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, UnmodifiableEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return detachRolePolicy(DetachRolePolicyRequest.builder().applyMutation(detachRolePolicyRequest).build());
}
/**
*
* Removes the specified managed policy from the specified user.
*
*
* A user can also have inline policies embedded with it. To delete an inline policy, use DeleteUserPolicy.
* For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param detachUserPolicyRequest
* @return Result of the DetachUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DetachUserPolicy
* @see AWS API
* Documentation
*/
default DetachUserPolicyResponse detachUserPolicy(DetachUserPolicyRequest detachUserPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified managed policy from the specified user.
*
*
* A user can also have inline policies embedded with it. To delete an inline policy, use DeleteUserPolicy.
* For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DetachUserPolicyRequest.Builder} avoiding the need
* to create one manually via {@link DetachUserPolicyRequest#builder()}
*
*
* @param detachUserPolicyRequest
* A {@link Consumer} that will call methods on {@link DetachUserPolicyRequest.Builder} to create a request.
* @return Result of the DetachUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.DetachUserPolicy
* @see AWS API
* Documentation
*/
default DetachUserPolicyResponse detachUserPolicy(Consumer detachUserPolicyRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return detachUserPolicy(DetachUserPolicyRequest.builder().applyMutation(detachUserPolicyRequest).build());
}
/**
*
* Enables the specified MFA device and associates it with the specified IAM user. When enabled, the MFA device is
* required for every subsequent login by the IAM user associated with the device.
*
*
* @param enableMfaDeviceRequest
* @return Result of the EnableMFADevice operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws InvalidAuthenticationCodeException
* The request was rejected because the authentication code was not recognized. The error message describes
* the specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.EnableMFADevice
* @see AWS API
* Documentation
*/
default EnableMfaDeviceResponse enableMFADevice(EnableMfaDeviceRequest enableMfaDeviceRequest)
throws EntityAlreadyExistsException, EntityTemporarilyUnmodifiableException, InvalidAuthenticationCodeException,
LimitExceededException, NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
throw new UnsupportedOperationException();
}
/**
*
* Enables the specified MFA device and associates it with the specified IAM user. When enabled, the MFA device is
* required for every subsequent login by the IAM user associated with the device.
*
*
*
* This is a convenience which creates an instance of the {@link EnableMfaDeviceRequest.Builder} avoiding the need
* to create one manually via {@link EnableMfaDeviceRequest#builder()}
*
*
* @param enableMfaDeviceRequest
* A {@link Consumer} that will call methods on {@link EnableMFADeviceRequest.Builder} to create a request.
* @return Result of the EnableMFADevice operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws InvalidAuthenticationCodeException
* The request was rejected because the authentication code was not recognized. The error message describes
* the specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.EnableMFADevice
* @see AWS API
* Documentation
*/
default EnableMfaDeviceResponse enableMFADevice(Consumer enableMfaDeviceRequest)
throws EntityAlreadyExistsException, EntityTemporarilyUnmodifiableException, InvalidAuthenticationCodeException,
LimitExceededException, NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return enableMFADevice(EnableMfaDeviceRequest.builder().applyMutation(enableMfaDeviceRequest).build());
}
/**
*
* Generates a credential report for the Amazon Web Services account. For more information about the credential
* report, see Getting credential
* reports in the IAM User Guide.
*
*
* @return Result of the GenerateCredentialReport operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GenerateCredentialReport
* @see #generateCredentialReport(GenerateCredentialReportRequest)
* @see AWS
* API Documentation
*/
default GenerateCredentialReportResponse generateCredentialReport() throws LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return generateCredentialReport(GenerateCredentialReportRequest.builder().build());
}
/**
*
* Generates a credential report for the Amazon Web Services account. For more information about the credential
* report, see Getting credential
* reports in the IAM User Guide.
*
*
* @param generateCredentialReportRequest
* @return Result of the GenerateCredentialReport operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GenerateCredentialReport
* @see AWS
* API Documentation
*/
default GenerateCredentialReportResponse generateCredentialReport(
GenerateCredentialReportRequest generateCredentialReportRequest) throws LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Generates a credential report for the Amazon Web Services account. For more information about the credential
* report, see Getting credential
* reports in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GenerateCredentialReportRequest.Builder} avoiding
* the need to create one manually via {@link GenerateCredentialReportRequest#builder()}
*
*
* @param generateCredentialReportRequest
* A {@link Consumer} that will call methods on {@link GenerateCredentialReportRequest.Builder} to create a
* request.
* @return Result of the GenerateCredentialReport operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GenerateCredentialReport
* @see AWS
* API Documentation
*/
default GenerateCredentialReportResponse generateCredentialReport(
Consumer generateCredentialReportRequest) throws LimitExceededException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return generateCredentialReport(GenerateCredentialReportRequest.builder().applyMutation(generateCredentialReportRequest)
.build());
}
/**
*
* Generates a report for service last accessed data for Organizations. You can generate a report for any entities
* (organization root, organizational unit, or account) or policies in your organization.
*
*
* To call this operation, you must be signed in using your Organizations management account credentials. You can
* use your long-term IAM user or root user credentials, or temporary credentials from assuming an IAM role. SCPs
* must be enabled for your organization root. You must have the required IAM and Organizations permissions. For
* more information, see Refining permissions
* using service last accessed data in the IAM User Guide.
*
*
* You can generate a service last accessed data report for entities by specifying only the entity's path. This data
* includes a list of services that are allowed by any service control policies (SCPs) that apply to the entity.
*
*
* You can generate a service last accessed data report for a policy by specifying an entity's path and an optional
* Organizations policy ID. This data includes a list of services that are allowed by the specified SCP.
*
*
* For each service in both report types, the data includes the most recent account activity that the policy allows
* to account principals in the entity or the entity's children. For important information about the data, reporting
* period, permissions required, troubleshooting, and supported Regions see Reducing permissions
* using service last accessed data in the IAM User Guide.
*
*
*
* The data includes all attempts to access Amazon Web Services, not just the successful ones. This includes all
* attempts that were made using the Amazon Web Services Management Console, the Amazon Web Services API through any
* of the SDKs, or any of the command line tools. An unexpected entry in the service last accessed data does not
* mean that an account has been compromised, because the request might have been denied. Refer to your CloudTrail
* logs as the authoritative source for information about all API calls and whether they were successful or denied
* access. For more information, see Logging IAM events with
* CloudTrail in the IAM User Guide.
*
*
*
* This operation returns a JobId
. Use this parameter in the
* GetOrganizationsAccessReport
operation to check the status of the report generation. To
* check the status of this request, use the JobId
parameter in the
* GetOrganizationsAccessReport
operation and test the JobStatus
response
* parameter. When the job is complete, you can retrieve the report.
*
*
* To generate a service last accessed data report for entities, specify an entity path without specifying the
* optional Organizations policy ID. The type of entity that you specify determines the data returned in the report.
*
*
* -
*
* Root – When you specify the organizations root as the entity, the resulting report lists all of the
* services allowed by SCPs that are attached to your root. For each service, the report includes data for all
* accounts in your organization except the management account, because the management account is not limited by
* SCPs.
*
*
* -
*
* OU – When you specify an organizational unit (OU) as the entity, the resulting report lists all of the
* services allowed by SCPs that are attached to the OU and its parents. For each service, the report includes data
* for all accounts in the OU or its children. This data excludes the management account, because the management
* account is not limited by SCPs.
*
*
* -
*
* management account – When you specify the management account, the resulting report lists all Amazon Web
* Services services, because the management account is not limited by SCPs. For each service, the report includes
* data for only the management account.
*
*
* -
*
* Account – When you specify another account as the entity, the resulting report lists all of the services
* allowed by SCPs that are attached to the account and its parents. For each service, the report includes data for
* only the specified account.
*
*
*
*
* To generate a service last accessed data report for policies, specify an entity path and the optional
* Organizations policy ID. The type of entity that you specify determines the data returned for each service.
*
*
* -
*
* Root – When you specify the root entity and a policy ID, the resulting report lists all of the services
* that are allowed by the specified SCP. For each service, the report includes data for all accounts in your
* organization to which the SCP applies. This data excludes the management account, because the management account
* is not limited by SCPs. If the SCP is not attached to any entities in the organization, then the report will
* return a list of services with no data.
*
*
* -
*
* OU – When you specify an OU entity and a policy ID, the resulting report lists all of the services that
* are allowed by the specified SCP. For each service, the report includes data for all accounts in the OU or its
* children to which the SCP applies. This means that other accounts outside the OU that are affected by the SCP
* might not be included in the data. This data excludes the management account, because the management account is
* not limited by SCPs. If the SCP is not attached to the OU or one of its children, the report will return a list
* of services with no data.
*
*
* -
*
* management account – When you specify the management account, the resulting report lists all Amazon Web
* Services services, because the management account is not limited by SCPs. If you specify a policy ID in the CLI
* or API, the policy is ignored. For each service, the report includes data for only the management account.
*
*
* -
*
* Account – When you specify another account entity and a policy ID, the resulting report lists all of the
* services that are allowed by the specified SCP. For each service, the report includes data for only the specified
* account. This means that other accounts in the organization that are affected by the SCP might not be included in
* the data. If the SCP is not attached to the account, the report will return a list of services with no data.
*
*
*
*
*
* Service last accessed data does not use other policy types when determining whether a principal could access a
* service. These other policy types include identity-based policies, resource-based policies, access control lists,
* IAM permissions boundaries, and STS assume role policies. It only applies SCP logic. For more about the
* evaluation of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* For more information about service last accessed data, see Reducing policy scope
* by viewing user activity in the IAM User Guide.
*
*
* @param generateOrganizationsAccessReportRequest
* @return Result of the GenerateOrganizationsAccessReport operation returned by the service.
* @throws ReportGenerationLimitExceededException
* The request failed because the maximum number of concurrent requests for this account are already
* running.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GenerateOrganizationsAccessReport
* @see AWS API Documentation
*/
default GenerateOrganizationsAccessReportResponse generateOrganizationsAccessReport(
GenerateOrganizationsAccessReportRequest generateOrganizationsAccessReportRequest)
throws ReportGenerationLimitExceededException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Generates a report for service last accessed data for Organizations. You can generate a report for any entities
* (organization root, organizational unit, or account) or policies in your organization.
*
*
* To call this operation, you must be signed in using your Organizations management account credentials. You can
* use your long-term IAM user or root user credentials, or temporary credentials from assuming an IAM role. SCPs
* must be enabled for your organization root. You must have the required IAM and Organizations permissions. For
* more information, see Refining permissions
* using service last accessed data in the IAM User Guide.
*
*
* You can generate a service last accessed data report for entities by specifying only the entity's path. This data
* includes a list of services that are allowed by any service control policies (SCPs) that apply to the entity.
*
*
* You can generate a service last accessed data report for a policy by specifying an entity's path and an optional
* Organizations policy ID. This data includes a list of services that are allowed by the specified SCP.
*
*
* For each service in both report types, the data includes the most recent account activity that the policy allows
* to account principals in the entity or the entity's children. For important information about the data, reporting
* period, permissions required, troubleshooting, and supported Regions see Reducing permissions
* using service last accessed data in the IAM User Guide.
*
*
*
* The data includes all attempts to access Amazon Web Services, not just the successful ones. This includes all
* attempts that were made using the Amazon Web Services Management Console, the Amazon Web Services API through any
* of the SDKs, or any of the command line tools. An unexpected entry in the service last accessed data does not
* mean that an account has been compromised, because the request might have been denied. Refer to your CloudTrail
* logs as the authoritative source for information about all API calls and whether they were successful or denied
* access. For more information, see Logging IAM events with
* CloudTrail in the IAM User Guide.
*
*
*
* This operation returns a JobId
. Use this parameter in the
* GetOrganizationsAccessReport
operation to check the status of the report generation. To
* check the status of this request, use the JobId
parameter in the
* GetOrganizationsAccessReport
operation and test the JobStatus
response
* parameter. When the job is complete, you can retrieve the report.
*
*
* To generate a service last accessed data report for entities, specify an entity path without specifying the
* optional Organizations policy ID. The type of entity that you specify determines the data returned in the report.
*
*
* -
*
* Root – When you specify the organizations root as the entity, the resulting report lists all of the
* services allowed by SCPs that are attached to your root. For each service, the report includes data for all
* accounts in your organization except the management account, because the management account is not limited by
* SCPs.
*
*
* -
*
* OU – When you specify an organizational unit (OU) as the entity, the resulting report lists all of the
* services allowed by SCPs that are attached to the OU and its parents. For each service, the report includes data
* for all accounts in the OU or its children. This data excludes the management account, because the management
* account is not limited by SCPs.
*
*
* -
*
* management account – When you specify the management account, the resulting report lists all Amazon Web
* Services services, because the management account is not limited by SCPs. For each service, the report includes
* data for only the management account.
*
*
* -
*
* Account – When you specify another account as the entity, the resulting report lists all of the services
* allowed by SCPs that are attached to the account and its parents. For each service, the report includes data for
* only the specified account.
*
*
*
*
* To generate a service last accessed data report for policies, specify an entity path and the optional
* Organizations policy ID. The type of entity that you specify determines the data returned for each service.
*
*
* -
*
* Root – When you specify the root entity and a policy ID, the resulting report lists all of the services
* that are allowed by the specified SCP. For each service, the report includes data for all accounts in your
* organization to which the SCP applies. This data excludes the management account, because the management account
* is not limited by SCPs. If the SCP is not attached to any entities in the organization, then the report will
* return a list of services with no data.
*
*
* -
*
* OU – When you specify an OU entity and a policy ID, the resulting report lists all of the services that
* are allowed by the specified SCP. For each service, the report includes data for all accounts in the OU or its
* children to which the SCP applies. This means that other accounts outside the OU that are affected by the SCP
* might not be included in the data. This data excludes the management account, because the management account is
* not limited by SCPs. If the SCP is not attached to the OU or one of its children, the report will return a list
* of services with no data.
*
*
* -
*
* management account – When you specify the management account, the resulting report lists all Amazon Web
* Services services, because the management account is not limited by SCPs. If you specify a policy ID in the CLI
* or API, the policy is ignored. For each service, the report includes data for only the management account.
*
*
* -
*
* Account – When you specify another account entity and a policy ID, the resulting report lists all of the
* services that are allowed by the specified SCP. For each service, the report includes data for only the specified
* account. This means that other accounts in the organization that are affected by the SCP might not be included in
* the data. If the SCP is not attached to the account, the report will return a list of services with no data.
*
*
*
*
*
* Service last accessed data does not use other policy types when determining whether a principal could access a
* service. These other policy types include identity-based policies, resource-based policies, access control lists,
* IAM permissions boundaries, and STS assume role policies. It only applies SCP logic. For more about the
* evaluation of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* For more information about service last accessed data, see Reducing policy scope
* by viewing user activity in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GenerateOrganizationsAccessReportRequest.Builder}
* avoiding the need to create one manually via {@link GenerateOrganizationsAccessReportRequest#builder()}
*
*
* @param generateOrganizationsAccessReportRequest
* A {@link Consumer} that will call methods on {@link GenerateOrganizationsAccessReportRequest.Builder} to
* create a request.
* @return Result of the GenerateOrganizationsAccessReport operation returned by the service.
* @throws ReportGenerationLimitExceededException
* The request failed because the maximum number of concurrent requests for this account are already
* running.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GenerateOrganizationsAccessReport
* @see AWS API Documentation
*/
default GenerateOrganizationsAccessReportResponse generateOrganizationsAccessReport(
Consumer generateOrganizationsAccessReportRequest)
throws ReportGenerationLimitExceededException, AwsServiceException, SdkClientException, IamException {
return generateOrganizationsAccessReport(GenerateOrganizationsAccessReportRequest.builder()
.applyMutation(generateOrganizationsAccessReportRequest).build());
}
/**
*
* Generates a report that includes details about when an IAM resource (user, group, role, or policy) was last used
* in an attempt to access Amazon Web Services services. Recent activity usually appears within four hours. IAM
* reports activity for at least the last 400 days, or less if your Region began supporting this feature within the
* last year. For more information, see Regions where data is tracked.
*
*
*
* The service last accessed data includes all attempts to access an Amazon Web Services API, not just the
* successful ones. This includes all attempts that were made using the Amazon Web Services Management Console, the
* Amazon Web Services API through any of the SDKs, or any of the command line tools. An unexpected entry in the
* service last accessed data does not mean that your account has been compromised, because the request might have
* been denied. Refer to your CloudTrail logs as the authoritative source for information about all API calls and
* whether they were successful or denied access. For more information, see Logging IAM events with
* CloudTrail in the IAM User Guide.
*
*
*
* The GenerateServiceLastAccessedDetails
operation returns a JobId
. Use this parameter in
* the following operations to retrieve the following details from your report:
*
*
* -
*
* GetServiceLastAccessedDetails – Use this operation for users, groups, roles, or policies to list every
* Amazon Web Services service that the resource could access using permissions policies. For each service, the
* response includes information about the most recent access attempt.
*
*
* The JobId
returned by GenerateServiceLastAccessedDetail
must be used by the same role
* within a session, or by the same user when used to call GetServiceLastAccessedDetail
.
*
*
* -
*
* GetServiceLastAccessedDetailsWithEntities – Use this operation for groups and policies to list information
* about the associated entities (users or roles) that attempted to access a specific Amazon Web Services service.
*
*
*
*
* To check the status of the GenerateServiceLastAccessedDetails
request, use the JobId
* parameter in the same operations and test the JobStatus
response parameter.
*
*
* For additional information about the permissions policies that allow an identity (user, group, or role) to access
* specific services, use the ListPoliciesGrantingServiceAccess operation.
*
*
*
* Service last accessed data does not use other policy types when determining whether a resource could access a
* service. These other policy types include resource-based policies, access control lists, Organizations policies,
* IAM permissions boundaries, and STS assume role policies. It only applies permissions policy logic. For more
* about the evaluation of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* For more information about service and action last accessed data, see Reducing permissions
* using service last accessed data in the IAM User Guide.
*
*
* @param generateServiceLastAccessedDetailsRequest
* @return Result of the GenerateServiceLastAccessedDetails operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GenerateServiceLastAccessedDetails
* @see AWS API Documentation
*/
default GenerateServiceLastAccessedDetailsResponse generateServiceLastAccessedDetails(
GenerateServiceLastAccessedDetailsRequest generateServiceLastAccessedDetailsRequest) throws NoSuchEntityException,
InvalidInputException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Generates a report that includes details about when an IAM resource (user, group, role, or policy) was last used
* in an attempt to access Amazon Web Services services. Recent activity usually appears within four hours. IAM
* reports activity for at least the last 400 days, or less if your Region began supporting this feature within the
* last year. For more information, see Regions where data is tracked.
*
*
*
* The service last accessed data includes all attempts to access an Amazon Web Services API, not just the
* successful ones. This includes all attempts that were made using the Amazon Web Services Management Console, the
* Amazon Web Services API through any of the SDKs, or any of the command line tools. An unexpected entry in the
* service last accessed data does not mean that your account has been compromised, because the request might have
* been denied. Refer to your CloudTrail logs as the authoritative source for information about all API calls and
* whether they were successful or denied access. For more information, see Logging IAM events with
* CloudTrail in the IAM User Guide.
*
*
*
* The GenerateServiceLastAccessedDetails
operation returns a JobId
. Use this parameter in
* the following operations to retrieve the following details from your report:
*
*
* -
*
* GetServiceLastAccessedDetails – Use this operation for users, groups, roles, or policies to list every
* Amazon Web Services service that the resource could access using permissions policies. For each service, the
* response includes information about the most recent access attempt.
*
*
* The JobId
returned by GenerateServiceLastAccessedDetail
must be used by the same role
* within a session, or by the same user when used to call GetServiceLastAccessedDetail
.
*
*
* -
*
* GetServiceLastAccessedDetailsWithEntities – Use this operation for groups and policies to list information
* about the associated entities (users or roles) that attempted to access a specific Amazon Web Services service.
*
*
*
*
* To check the status of the GenerateServiceLastAccessedDetails
request, use the JobId
* parameter in the same operations and test the JobStatus
response parameter.
*
*
* For additional information about the permissions policies that allow an identity (user, group, or role) to access
* specific services, use the ListPoliciesGrantingServiceAccess operation.
*
*
*
* Service last accessed data does not use other policy types when determining whether a resource could access a
* service. These other policy types include resource-based policies, access control lists, Organizations policies,
* IAM permissions boundaries, and STS assume role policies. It only applies permissions policy logic. For more
* about the evaluation of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* For more information about service and action last accessed data, see Reducing permissions
* using service last accessed data in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GenerateServiceLastAccessedDetailsRequest.Builder}
* avoiding the need to create one manually via {@link GenerateServiceLastAccessedDetailsRequest#builder()}
*
*
* @param generateServiceLastAccessedDetailsRequest
* A {@link Consumer} that will call methods on {@link GenerateServiceLastAccessedDetailsRequest.Builder} to
* create a request.
* @return Result of the GenerateServiceLastAccessedDetails operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GenerateServiceLastAccessedDetails
* @see AWS API Documentation
*/
default GenerateServiceLastAccessedDetailsResponse generateServiceLastAccessedDetails(
Consumer generateServiceLastAccessedDetailsRequest)
throws NoSuchEntityException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
return generateServiceLastAccessedDetails(GenerateServiceLastAccessedDetailsRequest.builder()
.applyMutation(generateServiceLastAccessedDetailsRequest).build());
}
/**
*
* Retrieves information about when the specified access key was last used. The information includes the date and
* time of last use, along with the Amazon Web Services service and Region that were specified in the last request
* made with that key.
*
*
* @param getAccessKeyLastUsedRequest
* @return Result of the GetAccessKeyLastUsed operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccessKeyLastUsed
* @see AWS API
* Documentation
*/
default GetAccessKeyLastUsedResponse getAccessKeyLastUsed(GetAccessKeyLastUsedRequest getAccessKeyLastUsedRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about when the specified access key was last used. The information includes the date and
* time of last use, along with the Amazon Web Services service and Region that were specified in the last request
* made with that key.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccessKeyLastUsedRequest.Builder} avoiding the
* need to create one manually via {@link GetAccessKeyLastUsedRequest#builder()}
*
*
* @param getAccessKeyLastUsedRequest
* A {@link Consumer} that will call methods on {@link GetAccessKeyLastUsedRequest.Builder} to create a
* request.
* @return Result of the GetAccessKeyLastUsed operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccessKeyLastUsed
* @see AWS API
* Documentation
*/
default GetAccessKeyLastUsedResponse getAccessKeyLastUsed(
Consumer getAccessKeyLastUsedRequest) throws NoSuchEntityException,
AwsServiceException, SdkClientException, IamException {
return getAccessKeyLastUsed(GetAccessKeyLastUsedRequest.builder().applyMutation(getAccessKeyLastUsedRequest).build());
}
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in your Amazon Web Services account,
* including their relationships to one another. Use this operation to obtain a snapshot of the configuration of IAM
* permissions (users, groups, roles, and policies) in your account.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* You can optionally filter the results using the Filter
parameter. You can paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* @return Result of the GetAccountAuthorizationDetails operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountAuthorizationDetails
* @see #getAccountAuthorizationDetails(GetAccountAuthorizationDetailsRequest)
* @see AWS API Documentation
*/
default GetAccountAuthorizationDetailsResponse getAccountAuthorizationDetails() throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return getAccountAuthorizationDetails(GetAccountAuthorizationDetailsRequest.builder().build());
}
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in your Amazon Web Services account,
* including their relationships to one another. Use this operation to obtain a snapshot of the configuration of IAM
* permissions (users, groups, roles, and policies) in your account.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* You can optionally filter the results using the Filter
parameter. You can paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* @param getAccountAuthorizationDetailsRequest
* @return Result of the GetAccountAuthorizationDetails operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountAuthorizationDetails
* @see AWS API Documentation
*/
default GetAccountAuthorizationDetailsResponse getAccountAuthorizationDetails(
GetAccountAuthorizationDetailsRequest getAccountAuthorizationDetailsRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in your Amazon Web Services account,
* including their relationships to one another. Use this operation to obtain a snapshot of the configuration of IAM
* permissions (users, groups, roles, and policies) in your account.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* You can optionally filter the results using the Filter
parameter. You can paginate the results using
* the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccountAuthorizationDetailsRequest.Builder}
* avoiding the need to create one manually via {@link GetAccountAuthorizationDetailsRequest#builder()}
*
*
* @param getAccountAuthorizationDetailsRequest
* A {@link Consumer} that will call methods on {@link GetAccountAuthorizationDetailsRequest.Builder} to
* create a request.
* @return Result of the GetAccountAuthorizationDetails operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountAuthorizationDetails
* @see AWS API Documentation
*/
default GetAccountAuthorizationDetailsResponse getAccountAuthorizationDetails(
Consumer getAccountAuthorizationDetailsRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getAccountAuthorizationDetails(GetAccountAuthorizationDetailsRequest.builder()
.applyMutation(getAccountAuthorizationDetailsRequest).build());
}
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in your Amazon Web Services account,
* including their relationships to one another. Use this operation to obtain a snapshot of the configuration of IAM
* permissions (users, groups, roles, and policies) in your account.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* You can optionally filter the results using the Filter
parameter. You can paginate the results using
* the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #getAccountAuthorizationDetails(software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client.getAccountAuthorizationDetailsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client
* .getAccountAuthorizationDetailsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client.getAccountAuthorizationDetailsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getAccountAuthorizationDetails(software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountAuthorizationDetails
* @see #getAccountAuthorizationDetailsPaginator(GetAccountAuthorizationDetailsRequest)
* @see AWS API Documentation
*/
default GetAccountAuthorizationDetailsIterable getAccountAuthorizationDetailsPaginator() throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return getAccountAuthorizationDetailsPaginator(GetAccountAuthorizationDetailsRequest.builder().build());
}
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in your Amazon Web Services account,
* including their relationships to one another. Use this operation to obtain a snapshot of the configuration of IAM
* permissions (users, groups, roles, and policies) in your account.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* You can optionally filter the results using the Filter
parameter. You can paginate the results using
* the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #getAccountAuthorizationDetails(software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client.getAccountAuthorizationDetailsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client
* .getAccountAuthorizationDetailsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client.getAccountAuthorizationDetailsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getAccountAuthorizationDetails(software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest)}
* operation.
*
*
* @param getAccountAuthorizationDetailsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountAuthorizationDetails
* @see AWS API Documentation
*/
default GetAccountAuthorizationDetailsIterable getAccountAuthorizationDetailsPaginator(
GetAccountAuthorizationDetailsRequest getAccountAuthorizationDetailsRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in your Amazon Web Services account,
* including their relationships to one another. Use this operation to obtain a snapshot of the configuration of IAM
* permissions (users, groups, roles, and policies) in your account.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* You can optionally filter the results using the Filter
parameter. You can paginate the results using
* the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #getAccountAuthorizationDetails(software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client.getAccountAuthorizationDetailsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client
* .getAccountAuthorizationDetailsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetAccountAuthorizationDetailsIterable responses = client.getAccountAuthorizationDetailsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getAccountAuthorizationDetails(software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetAccountAuthorizationDetailsRequest.Builder}
* avoiding the need to create one manually via {@link GetAccountAuthorizationDetailsRequest#builder()}
*
*
* @param getAccountAuthorizationDetailsRequest
* A {@link Consumer} that will call methods on {@link GetAccountAuthorizationDetailsRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountAuthorizationDetails
* @see AWS API Documentation
*/
default GetAccountAuthorizationDetailsIterable getAccountAuthorizationDetailsPaginator(
Consumer getAccountAuthorizationDetailsRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getAccountAuthorizationDetailsPaginator(GetAccountAuthorizationDetailsRequest.builder()
.applyMutation(getAccountAuthorizationDetailsRequest).build());
}
/**
*
* Retrieves the password policy for the Amazon Web Services account. This tells you the complexity requirements and
* mandatory rotation periods for the IAM user passwords in your account. For more information about using a
* password policy, see Managing an IAM
* password policy.
*
*
* @return Result of the GetAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountPasswordPolicy
* @see #getAccountPasswordPolicy(GetAccountPasswordPolicyRequest)
* @see AWS
* API Documentation
*/
default GetAccountPasswordPolicyResponse getAccountPasswordPolicy() throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return getAccountPasswordPolicy(GetAccountPasswordPolicyRequest.builder().build());
}
/**
*
* Retrieves the password policy for the Amazon Web Services account. This tells you the complexity requirements and
* mandatory rotation periods for the IAM user passwords in your account. For more information about using a
* password policy, see Managing an IAM
* password policy.
*
*
* @param getAccountPasswordPolicyRequest
* @return Result of the GetAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountPasswordPolicy
* @see AWS
* API Documentation
*/
default GetAccountPasswordPolicyResponse getAccountPasswordPolicy(
GetAccountPasswordPolicyRequest getAccountPasswordPolicyRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the password policy for the Amazon Web Services account. This tells you the complexity requirements and
* mandatory rotation periods for the IAM user passwords in your account. For more information about using a
* password policy, see Managing an IAM
* password policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccountPasswordPolicyRequest.Builder} avoiding
* the need to create one manually via {@link GetAccountPasswordPolicyRequest#builder()}
*
*
* @param getAccountPasswordPolicyRequest
* A {@link Consumer} that will call methods on {@link GetAccountPasswordPolicyRequest.Builder} to create a
* request.
* @return Result of the GetAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountPasswordPolicy
* @see AWS
* API Documentation
*/
default GetAccountPasswordPolicyResponse getAccountPasswordPolicy(
Consumer getAccountPasswordPolicyRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getAccountPasswordPolicy(GetAccountPasswordPolicyRequest.builder().applyMutation(getAccountPasswordPolicyRequest)
.build());
}
/**
*
* Retrieves information about IAM entity usage and IAM quotas in the Amazon Web Services account.
*
*
* For information about IAM quotas, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @return Result of the GetAccountSummary operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountSummary
* @see #getAccountSummary(GetAccountSummaryRequest)
* @see AWS API
* Documentation
*/
default GetAccountSummaryResponse getAccountSummary() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return getAccountSummary(GetAccountSummaryRequest.builder().build());
}
/**
*
* Retrieves information about IAM entity usage and IAM quotas in the Amazon Web Services account.
*
*
* For information about IAM quotas, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @param getAccountSummaryRequest
* @return Result of the GetAccountSummary operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountSummary
* @see AWS API
* Documentation
*/
default GetAccountSummaryResponse getAccountSummary(GetAccountSummaryRequest getAccountSummaryRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about IAM entity usage and IAM quotas in the Amazon Web Services account.
*
*
* For information about IAM quotas, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccountSummaryRequest.Builder} avoiding the need
* to create one manually via {@link GetAccountSummaryRequest#builder()}
*
*
* @param getAccountSummaryRequest
* A {@link Consumer} that will call methods on {@link GetAccountSummaryRequest.Builder} to create a request.
* @return Result of the GetAccountSummary operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetAccountSummary
* @see AWS API
* Documentation
*/
default GetAccountSummaryResponse getAccountSummary(Consumer getAccountSummaryRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getAccountSummary(GetAccountSummaryRequest.builder().applyMutation(getAccountSummaryRequest).build());
}
/**
*
* Gets a list of all of the context keys referenced in the input policies. The policies are supplied as a list of
* one or more strings. To get the context keys from policies associated with an IAM user, group, or role, use
* GetContextKeysForPrincipalPolicy.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. Context keys can be evaluated by testing against a value specified in an IAM
* policy. Use GetContextKeysForCustomPolicy
to understand what key names and values you must supply
* when you call SimulateCustomPolicy. Note that all parameters are shown in unencoded form here for clarity
* but must be URL encoded to be included as a part of a real HTML request.
*
*
* @param getContextKeysForCustomPolicyRequest
* @return Result of the GetContextKeysForCustomPolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetContextKeysForCustomPolicy
* @see AWS API Documentation
*/
default GetContextKeysForCustomPolicyResponse getContextKeysForCustomPolicy(
GetContextKeysForCustomPolicyRequest getContextKeysForCustomPolicyRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of all of the context keys referenced in the input policies. The policies are supplied as a list of
* one or more strings. To get the context keys from policies associated with an IAM user, group, or role, use
* GetContextKeysForPrincipalPolicy.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. Context keys can be evaluated by testing against a value specified in an IAM
* policy. Use GetContextKeysForCustomPolicy
to understand what key names and values you must supply
* when you call SimulateCustomPolicy. Note that all parameters are shown in unencoded form here for clarity
* but must be URL encoded to be included as a part of a real HTML request.
*
*
*
* This is a convenience which creates an instance of the {@link GetContextKeysForCustomPolicyRequest.Builder}
* avoiding the need to create one manually via {@link GetContextKeysForCustomPolicyRequest#builder()}
*
*
* @param getContextKeysForCustomPolicyRequest
* A {@link Consumer} that will call methods on {@link GetContextKeysForCustomPolicyRequest.Builder} to
* create a request.
* @return Result of the GetContextKeysForCustomPolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetContextKeysForCustomPolicy
* @see AWS API Documentation
*/
default GetContextKeysForCustomPolicyResponse getContextKeysForCustomPolicy(
Consumer getContextKeysForCustomPolicyRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, IamException {
return getContextKeysForCustomPolicy(GetContextKeysForCustomPolicyRequest.builder()
.applyMutation(getContextKeysForCustomPolicyRequest).build());
}
/**
*
* Gets a list of all of the context keys referenced in all the IAM policies that are attached to the specified IAM
* entity. The entity can be an IAM user, group, or role. If you specify a user, then the request also includes all
* of the policies attached to groups that the user is a member of.
*
*
* You can optionally include a list of one or more additional policies, specified as strings. If you want to
* include only a list of policies by string, use GetContextKeysForCustomPolicy instead.
*
*
* Note: This operation discloses information about the permissions granted to other users. If you do not
* want users to see other user's permissions, then consider allowing them to use
* GetContextKeysForCustomPolicy instead.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. Context keys can be evaluated by testing against a value in an IAM policy. Use
* GetContextKeysForPrincipalPolicy to understand what key names and values you must supply when you call
* SimulatePrincipalPolicy.
*
*
* @param getContextKeysForPrincipalPolicyRequest
* @return Result of the GetContextKeysForPrincipalPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetContextKeysForPrincipalPolicy
* @see AWS API Documentation
*/
default GetContextKeysForPrincipalPolicyResponse getContextKeysForPrincipalPolicy(
GetContextKeysForPrincipalPolicyRequest getContextKeysForPrincipalPolicyRequest) throws NoSuchEntityException,
InvalidInputException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of all of the context keys referenced in all the IAM policies that are attached to the specified IAM
* entity. The entity can be an IAM user, group, or role. If you specify a user, then the request also includes all
* of the policies attached to groups that the user is a member of.
*
*
* You can optionally include a list of one or more additional policies, specified as strings. If you want to
* include only a list of policies by string, use GetContextKeysForCustomPolicy instead.
*
*
* Note: This operation discloses information about the permissions granted to other users. If you do not
* want users to see other user's permissions, then consider allowing them to use
* GetContextKeysForCustomPolicy instead.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. Context keys can be evaluated by testing against a value in an IAM policy. Use
* GetContextKeysForPrincipalPolicy to understand what key names and values you must supply when you call
* SimulatePrincipalPolicy.
*
*
*
* This is a convenience which creates an instance of the {@link GetContextKeysForPrincipalPolicyRequest.Builder}
* avoiding the need to create one manually via {@link GetContextKeysForPrincipalPolicyRequest#builder()}
*
*
* @param getContextKeysForPrincipalPolicyRequest
* A {@link Consumer} that will call methods on {@link GetContextKeysForPrincipalPolicyRequest.Builder} to
* create a request.
* @return Result of the GetContextKeysForPrincipalPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetContextKeysForPrincipalPolicy
* @see AWS API Documentation
*/
default GetContextKeysForPrincipalPolicyResponse getContextKeysForPrincipalPolicy(
Consumer getContextKeysForPrincipalPolicyRequest)
throws NoSuchEntityException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
return getContextKeysForPrincipalPolicy(GetContextKeysForPrincipalPolicyRequest.builder()
.applyMutation(getContextKeysForPrincipalPolicyRequest).build());
}
/**
*
* Retrieves a credential report for the Amazon Web Services account. For more information about the credential
* report, see Getting credential
* reports in the IAM User Guide.
*
*
* @return Result of the GetCredentialReport operation returned by the service.
* @throws CredentialReportNotPresentException
* The request was rejected because the credential report does not exist. To generate a credential report,
* use GenerateCredentialReport.
* @throws CredentialReportExpiredException
* The request was rejected because the most recent credential report has expired. To generate a new
* credential report, use GenerateCredentialReport. For more information about credential report
* expiration, see Getting credential
* reports in the IAM User Guide.
* @throws CredentialReportNotReadyException
* The request was rejected because the credential report is still being generated.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetCredentialReport
* @see #getCredentialReport(GetCredentialReportRequest)
* @see AWS API
* Documentation
*/
default GetCredentialReportResponse getCredentialReport() throws CredentialReportNotPresentException,
CredentialReportExpiredException, CredentialReportNotReadyException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return getCredentialReport(GetCredentialReportRequest.builder().build());
}
/**
*
* Retrieves a credential report for the Amazon Web Services account. For more information about the credential
* report, see Getting credential
* reports in the IAM User Guide.
*
*
* @param getCredentialReportRequest
* @return Result of the GetCredentialReport operation returned by the service.
* @throws CredentialReportNotPresentException
* The request was rejected because the credential report does not exist. To generate a credential report,
* use GenerateCredentialReport.
* @throws CredentialReportExpiredException
* The request was rejected because the most recent credential report has expired. To generate a new
* credential report, use GenerateCredentialReport. For more information about credential report
* expiration, see Getting credential
* reports in the IAM User Guide.
* @throws CredentialReportNotReadyException
* The request was rejected because the credential report is still being generated.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetCredentialReport
* @see AWS API
* Documentation
*/
default GetCredentialReportResponse getCredentialReport(GetCredentialReportRequest getCredentialReportRequest)
throws CredentialReportNotPresentException, CredentialReportExpiredException, CredentialReportNotReadyException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a credential report for the Amazon Web Services account. For more information about the credential
* report, see Getting credential
* reports in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetCredentialReportRequest.Builder} avoiding the
* need to create one manually via {@link GetCredentialReportRequest#builder()}
*
*
* @param getCredentialReportRequest
* A {@link Consumer} that will call methods on {@link GetCredentialReportRequest.Builder} to create a
* request.
* @return Result of the GetCredentialReport operation returned by the service.
* @throws CredentialReportNotPresentException
* The request was rejected because the credential report does not exist. To generate a credential report,
* use GenerateCredentialReport.
* @throws CredentialReportExpiredException
* The request was rejected because the most recent credential report has expired. To generate a new
* credential report, use GenerateCredentialReport. For more information about credential report
* expiration, see Getting credential
* reports in the IAM User Guide.
* @throws CredentialReportNotReadyException
* The request was rejected because the credential report is still being generated.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetCredentialReport
* @see AWS API
* Documentation
*/
default GetCredentialReportResponse getCredentialReport(
Consumer getCredentialReportRequest) throws CredentialReportNotPresentException,
CredentialReportExpiredException, CredentialReportNotReadyException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return getCredentialReport(GetCredentialReportRequest.builder().applyMutation(getCredentialReportRequest).build());
}
/**
*
* Returns a list of IAM users that are in the specified IAM group. You can paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* @param getGroupRequest
* @return Result of the GetGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetGroup
* @see AWS API
* Documentation
*/
default GetGroupResponse getGroup(GetGroupRequest getGroupRequest) throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of IAM users that are in the specified IAM group. You can paginate the results using the
* MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link GetGroupRequest.Builder} avoiding the need to
* create one manually via {@link GetGroupRequest#builder()}
*
*
* @param getGroupRequest
* A {@link Consumer} that will call methods on {@link GetGroupRequest.Builder} to create a request.
* @return Result of the GetGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetGroup
* @see AWS API
* Documentation
*/
default GetGroupResponse getGroup(Consumer getGroupRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getGroup(GetGroupRequest.builder().applyMutation(getGroupRequest).build());
}
/**
*
* Returns a list of IAM users that are in the specified IAM group. You can paginate the results using the
* MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #getGroup(software.amazon.awssdk.services.iam.model.GetGroupRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetGroupIterable responses = client.getGroupPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.GetGroupIterable responses = client.getGroupPaginator(request);
* for (software.amazon.awssdk.services.iam.model.GetGroupResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetGroupIterable responses = client.getGroupPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getGroup(software.amazon.awssdk.services.iam.model.GetGroupRequest)} operation.
*
*
* @param getGroupRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetGroup
* @see AWS API
* Documentation
*/
default GetGroupIterable getGroupPaginator(GetGroupRequest getGroupRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of IAM users that are in the specified IAM group. You can paginate the results using the
* MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #getGroup(software.amazon.awssdk.services.iam.model.GetGroupRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetGroupIterable responses = client.getGroupPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.GetGroupIterable responses = client.getGroupPaginator(request);
* for (software.amazon.awssdk.services.iam.model.GetGroupResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.GetGroupIterable responses = client.getGroupPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getGroup(software.amazon.awssdk.services.iam.model.GetGroupRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetGroupRequest.Builder} avoiding the need to
* create one manually via {@link GetGroupRequest#builder()}
*
*
* @param getGroupRequest
* A {@link Consumer} that will call methods on {@link GetGroupRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetGroup
* @see AWS API
* Documentation
*/
default GetGroupIterable getGroupPaginator(Consumer getGroupRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getGroupPaginator(GetGroupRequest.builder().applyMutation(getGroupRequest).build());
}
/**
*
* Retrieves the specified inline policy document that is embedded in the specified IAM group.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* An IAM group can also have managed policies attached to it. To retrieve a managed policy document that is
* attached to a group, use GetPolicy to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param getGroupPolicyRequest
* @return Result of the GetGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetGroupPolicy
* @see AWS API
* Documentation
*/
default GetGroupPolicyResponse getGroupPolicy(GetGroupPolicyRequest getGroupPolicyRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the specified inline policy document that is embedded in the specified IAM group.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* An IAM group can also have managed policies attached to it. To retrieve a managed policy document that is
* attached to a group, use GetPolicy to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetGroupPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetGroupPolicyRequest#builder()}
*
*
* @param getGroupPolicyRequest
* A {@link Consumer} that will call methods on {@link GetGroupPolicyRequest.Builder} to create a request.
* @return Result of the GetGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetGroupPolicy
* @see AWS API
* Documentation
*/
default GetGroupPolicyResponse getGroupPolicy(Consumer getGroupPolicyRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getGroupPolicy(GetGroupPolicyRequest.builder().applyMutation(getGroupPolicyRequest).build());
}
/**
*
* Retrieves information about the specified instance profile, including the instance profile's path, GUID, ARN, and
* role. For more information about instance profiles, see About instance profiles in
* the IAM User Guide.
*
*
* @param getInstanceProfileRequest
* @return Result of the GetInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetInstanceProfile
* @see AWS API
* Documentation
*/
default GetInstanceProfileResponse getInstanceProfile(GetInstanceProfileRequest getInstanceProfileRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the specified instance profile, including the instance profile's path, GUID, ARN, and
* role. For more information about instance profiles, see About instance profiles in
* the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link GetInstanceProfileRequest#builder()}
*
*
* @param getInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link GetInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the GetInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetInstanceProfile
* @see AWS API
* Documentation
*/
default GetInstanceProfileResponse getInstanceProfile(Consumer getInstanceProfileRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getInstanceProfile(GetInstanceProfileRequest.builder().applyMutation(getInstanceProfileRequest).build());
}
/**
*
* Retrieves the user name for the specified IAM user. A login profile is created when you create a password for the
* user to access the Amazon Web Services Management Console. If the user does not exist or does not have a
* password, the operation returns a 404 (NoSuchEntity
) error.
*
*
* If you create an IAM user with access to the console, the CreateDate
reflects the date you created
* the initial password for the user.
*
*
* If you create an IAM user with programmatic access, and then later add a password for the user to access the
* Amazon Web Services Management Console, the CreateDate
reflects the initial password creation date.
* A user with programmatic access does not have a login profile unless you create a password for the user to access
* the Amazon Web Services Management Console.
*
*
* @param getLoginProfileRequest
* @return Result of the GetLoginProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetLoginProfile
* @see AWS API
* Documentation
*/
default GetLoginProfileResponse getLoginProfile(GetLoginProfileRequest getLoginProfileRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the user name for the specified IAM user. A login profile is created when you create a password for the
* user to access the Amazon Web Services Management Console. If the user does not exist or does not have a
* password, the operation returns a 404 (NoSuchEntity
) error.
*
*
* If you create an IAM user with access to the console, the CreateDate
reflects the date you created
* the initial password for the user.
*
*
* If you create an IAM user with programmatic access, and then later add a password for the user to access the
* Amazon Web Services Management Console, the CreateDate
reflects the initial password creation date.
* A user with programmatic access does not have a login profile unless you create a password for the user to access
* the Amazon Web Services Management Console.
*
*
*
* This is a convenience which creates an instance of the {@link GetLoginProfileRequest.Builder} avoiding the need
* to create one manually via {@link GetLoginProfileRequest#builder()}
*
*
* @param getLoginProfileRequest
* A {@link Consumer} that will call methods on {@link GetLoginProfileRequest.Builder} to create a request.
* @return Result of the GetLoginProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetLoginProfile
* @see AWS API
* Documentation
*/
default GetLoginProfileResponse getLoginProfile(Consumer getLoginProfileRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getLoginProfile(GetLoginProfileRequest.builder().applyMutation(getLoginProfileRequest).build());
}
/**
*
* Returns information about the specified OpenID Connect (OIDC) provider resource object in IAM.
*
*
* @param getOpenIdConnectProviderRequest
* @return Result of the GetOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetOpenIDConnectProvider
* @see AWS
* API Documentation
*/
default GetOpenIdConnectProviderResponse getOpenIDConnectProvider(
GetOpenIdConnectProviderRequest getOpenIdConnectProviderRequest) throws InvalidInputException, NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified OpenID Connect (OIDC) provider resource object in IAM.
*
*
*
* This is a convenience which creates an instance of the {@link GetOpenIdConnectProviderRequest.Builder} avoiding
* the need to create one manually via {@link GetOpenIdConnectProviderRequest#builder()}
*
*
* @param getOpenIdConnectProviderRequest
* A {@link Consumer} that will call methods on {@link GetOpenIDConnectProviderRequest.Builder} to create a
* request.
* @return Result of the GetOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetOpenIDConnectProvider
* @see AWS
* API Documentation
*/
default GetOpenIdConnectProviderResponse getOpenIDConnectProvider(
Consumer getOpenIdConnectProviderRequest) throws InvalidInputException,
NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getOpenIDConnectProvider(GetOpenIdConnectProviderRequest.builder().applyMutation(getOpenIdConnectProviderRequest)
.build());
}
/**
*
* Retrieves the service last accessed data report for Organizations that was previously generated using the
* GenerateOrganizationsAccessReport
operation. This operation retrieves the status of your
* report job and the report contents.
*
*
* Depending on the parameters that you passed when you generated the report, the data returned could include
* different information. For details, see GenerateOrganizationsAccessReport.
*
*
* To call this operation, you must be signed in to the management account in your organization. SCPs must be
* enabled for your organization root. You must have permissions to perform this operation. For more information,
* see Refining
* permissions using service last accessed data in the IAM User Guide.
*
*
* For each service that principals in an account (root users, IAM users, or IAM roles) could access using SCPs, the
* operation returns details about the most recent access attempt. If there was no attempt, the service is listed
* without details about the most recent attempt to access the service. If the operation fails, it returns the
* reason that it failed.
*
*
* By default, the list is sorted by service namespace.
*
*
* @param getOrganizationsAccessReportRequest
* @return Result of the GetOrganizationsAccessReport operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetOrganizationsAccessReport
* @see AWS API Documentation
*/
default GetOrganizationsAccessReportResponse getOrganizationsAccessReport(
GetOrganizationsAccessReportRequest getOrganizationsAccessReportRequest) throws NoSuchEntityException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the service last accessed data report for Organizations that was previously generated using the
* GenerateOrganizationsAccessReport
operation. This operation retrieves the status of your
* report job and the report contents.
*
*
* Depending on the parameters that you passed when you generated the report, the data returned could include
* different information. For details, see GenerateOrganizationsAccessReport.
*
*
* To call this operation, you must be signed in to the management account in your organization. SCPs must be
* enabled for your organization root. You must have permissions to perform this operation. For more information,
* see Refining
* permissions using service last accessed data in the IAM User Guide.
*
*
* For each service that principals in an account (root users, IAM users, or IAM roles) could access using SCPs, the
* operation returns details about the most recent access attempt. If there was no attempt, the service is listed
* without details about the most recent attempt to access the service. If the operation fails, it returns the
* reason that it failed.
*
*
* By default, the list is sorted by service namespace.
*
*
*
* This is a convenience which creates an instance of the {@link GetOrganizationsAccessReportRequest.Builder}
* avoiding the need to create one manually via {@link GetOrganizationsAccessReportRequest#builder()}
*
*
* @param getOrganizationsAccessReportRequest
* A {@link Consumer} that will call methods on {@link GetOrganizationsAccessReportRequest.Builder} to create
* a request.
* @return Result of the GetOrganizationsAccessReport operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetOrganizationsAccessReport
* @see AWS API Documentation
*/
default GetOrganizationsAccessReportResponse getOrganizationsAccessReport(
Consumer getOrganizationsAccessReportRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
return getOrganizationsAccessReport(GetOrganizationsAccessReportRequest.builder()
.applyMutation(getOrganizationsAccessReportRequest).build());
}
/**
*
* Retrieves information about the specified managed policy, including the policy's default version and the total
* number of IAM users, groups, and roles to which the policy is attached. To retrieve the list of the specific
* users, groups, and roles that the policy is attached to, use ListEntitiesForPolicy. This operation returns
* metadata about the policy. To retrieve the actual policy document for a specific version of the policy, use
* GetPolicyVersion.
*
*
* This operation retrieves information about managed policies. To retrieve information about an inline policy that
* is embedded with an IAM user, group, or role, use GetUserPolicy, GetGroupPolicy, or
* GetRolePolicy.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetPolicy
* @see AWS API
* Documentation
*/
default GetPolicyResponse getPolicy(GetPolicyRequest getPolicyRequest) throws NoSuchEntityException, InvalidInputException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the specified managed policy, including the policy's default version and the total
* number of IAM users, groups, and roles to which the policy is attached. To retrieve the list of the specific
* users, groups, and roles that the policy is attached to, use ListEntitiesForPolicy. This operation returns
* metadata about the policy. To retrieve the actual policy document for a specific version of the policy, use
* GetPolicyVersion.
*
*
* This operation retrieves information about managed policies. To retrieve information about an inline policy that
* is embedded with an IAM user, group, or role, use GetUserPolicy, GetGroupPolicy, or
* GetRolePolicy.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetPolicyRequest#builder()}
*
*
* @param getPolicyRequest
* A {@link Consumer} that will call methods on {@link GetPolicyRequest.Builder} to create a request.
* @return Result of the GetPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetPolicy
* @see AWS API
* Documentation
*/
default GetPolicyResponse getPolicy(Consumer getPolicyRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getPolicy(GetPolicyRequest.builder().applyMutation(getPolicyRequest).build());
}
/**
*
* Retrieves information about the specified version of the specified managed policy, including the policy document.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* To list the available versions for a policy, use ListPolicyVersions.
*
*
* This operation retrieves information about managed policies. To retrieve information about an inline policy that
* is embedded in a user, group, or role, use GetUserPolicy, GetGroupPolicy, or GetRolePolicy.
*
*
* For more information about the types of policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* For more information about managed policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
* @param getPolicyVersionRequest
* @return Result of the GetPolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetPolicyVersion
* @see AWS API
* Documentation
*/
default GetPolicyVersionResponse getPolicyVersion(GetPolicyVersionRequest getPolicyVersionRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the specified version of the specified managed policy, including the policy document.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* To list the available versions for a policy, use ListPolicyVersions.
*
*
* This operation retrieves information about managed policies. To retrieve information about an inline policy that
* is embedded in a user, group, or role, use GetUserPolicy, GetGroupPolicy, or GetRolePolicy.
*
*
* For more information about the types of policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* For more information about managed policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetPolicyVersionRequest.Builder} avoiding the need
* to create one manually via {@link GetPolicyVersionRequest#builder()}
*
*
* @param getPolicyVersionRequest
* A {@link Consumer} that will call methods on {@link GetPolicyVersionRequest.Builder} to create a request.
* @return Result of the GetPolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetPolicyVersion
* @see AWS API
* Documentation
*/
default GetPolicyVersionResponse getPolicyVersion(Consumer getPolicyVersionRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return getPolicyVersion(GetPolicyVersionRequest.builder().applyMutation(getPolicyVersionRequest).build());
}
/**
*
* Retrieves information about the specified role, including the role's path, GUID, ARN, and the role's trust policy
* that grants permission to assume the role. For more information about roles, see Working with roles.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* @param getRoleRequest
* @return Result of the GetRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetRole
* @see AWS API
* Documentation
*/
default GetRoleResponse getRole(GetRoleRequest getRoleRequest) throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the specified role, including the role's path, GUID, ARN, and the role's trust policy
* that grants permission to assume the role. For more information about roles, see Working with roles.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* This is a convenience which creates an instance of the {@link GetRoleRequest.Builder} avoiding the need to create
* one manually via {@link GetRoleRequest#builder()}
*
*
* @param getRoleRequest
* A {@link Consumer} that will call methods on {@link GetRoleRequest.Builder} to create a request.
* @return Result of the GetRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetRole
* @see AWS API
* Documentation
*/
default GetRoleResponse getRole(Consumer getRoleRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getRole(GetRoleRequest.builder().applyMutation(getRoleRequest).build());
}
/**
*
* Retrieves the specified inline policy document that is embedded with the specified IAM role.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* An IAM role can also have managed policies attached to it. To retrieve a managed policy document that is attached
* to a role, use GetPolicy to determine the policy's default version, then use GetPolicyVersion to
* retrieve the policy document.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* For more information about roles, see Using roles to delegate permissions
* and federate identities.
*
*
* @param getRolePolicyRequest
* @return Result of the GetRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetRolePolicy
* @see AWS API
* Documentation
*/
default GetRolePolicyResponse getRolePolicy(GetRolePolicyRequest getRolePolicyRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the specified inline policy document that is embedded with the specified IAM role.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* An IAM role can also have managed policies attached to it. To retrieve a managed policy document that is attached
* to a role, use GetPolicy to determine the policy's default version, then use GetPolicyVersion to
* retrieve the policy document.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* For more information about roles, see Using roles to delegate permissions
* and federate identities.
*
*
*
* This is a convenience which creates an instance of the {@link GetRolePolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetRolePolicyRequest#builder()}
*
*
* @param getRolePolicyRequest
* A {@link Consumer} that will call methods on {@link GetRolePolicyRequest.Builder} to create a request.
* @return Result of the GetRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetRolePolicy
* @see AWS API
* Documentation
*/
default GetRolePolicyResponse getRolePolicy(Consumer getRolePolicyRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getRolePolicy(GetRolePolicyRequest.builder().applyMutation(getRolePolicyRequest).build());
}
/**
*
* Returns the SAML provider metadocument that was uploaded when the IAM SAML provider resource object was created
* or updated.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param getSamlProviderRequest
* @return Result of the GetSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetSAMLProvider
* @see AWS API
* Documentation
*/
default GetSamlProviderResponse getSAMLProvider(GetSamlProviderRequest getSamlProviderRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the SAML provider metadocument that was uploaded when the IAM SAML provider resource object was created
* or updated.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* This is a convenience which creates an instance of the {@link GetSamlProviderRequest.Builder} avoiding the need
* to create one manually via {@link GetSamlProviderRequest#builder()}
*
*
* @param getSamlProviderRequest
* A {@link Consumer} that will call methods on {@link GetSAMLProviderRequest.Builder} to create a request.
* @return Result of the GetSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetSAMLProvider
* @see AWS API
* Documentation
*/
default GetSamlProviderResponse getSAMLProvider(Consumer getSamlProviderRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return getSAMLProvider(GetSamlProviderRequest.builder().applyMutation(getSamlProviderRequest).build());
}
/**
*
* Retrieves the specified SSH public key, including metadata about the key.
*
*
* The SSH public key retrieved by this operation is used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
* @param getSshPublicKeyRequest
* @return Result of the GetSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnrecognizedPublicKeyEncodingException
* The request was rejected because the public key encoding format is unsupported or unrecognized.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetSSHPublicKey
* @see AWS API
* Documentation
*/
default GetSshPublicKeyResponse getSSHPublicKey(GetSshPublicKeyRequest getSshPublicKeyRequest) throws NoSuchEntityException,
UnrecognizedPublicKeyEncodingException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the specified SSH public key, including metadata about the key.
*
*
* The SSH public key retrieved by this operation is used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetSshPublicKeyRequest.Builder} avoiding the need
* to create one manually via {@link GetSshPublicKeyRequest#builder()}
*
*
* @param getSshPublicKeyRequest
* A {@link Consumer} that will call methods on {@link GetSSHPublicKeyRequest.Builder} to create a request.
* @return Result of the GetSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnrecognizedPublicKeyEncodingException
* The request was rejected because the public key encoding format is unsupported or unrecognized.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetSSHPublicKey
* @see AWS API
* Documentation
*/
default GetSshPublicKeyResponse getSSHPublicKey(Consumer getSshPublicKeyRequest)
throws NoSuchEntityException, UnrecognizedPublicKeyEncodingException, AwsServiceException, SdkClientException,
IamException {
return getSSHPublicKey(GetSshPublicKeyRequest.builder().applyMutation(getSshPublicKeyRequest).build());
}
/**
*
* Retrieves information about the specified server certificate stored in IAM.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic includes a list of Amazon Web Services services that
* can use the server certificates that you manage with IAM.
*
*
* @param getServerCertificateRequest
* @return Result of the GetServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServerCertificate
* @see AWS API
* Documentation
*/
default GetServerCertificateResponse getServerCertificate(GetServerCertificateRequest getServerCertificateRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the specified server certificate stored in IAM.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic includes a list of Amazon Web Services services that
* can use the server certificates that you manage with IAM.
*
*
*
* This is a convenience which creates an instance of the {@link GetServerCertificateRequest.Builder} avoiding the
* need to create one manually via {@link GetServerCertificateRequest#builder()}
*
*
* @param getServerCertificateRequest
* A {@link Consumer} that will call methods on {@link GetServerCertificateRequest.Builder} to create a
* request.
* @return Result of the GetServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServerCertificate
* @see AWS API
* Documentation
*/
default GetServerCertificateResponse getServerCertificate(
Consumer getServerCertificateRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getServerCertificate(GetServerCertificateRequest.builder().applyMutation(getServerCertificateRequest).build());
}
/**
*
* Retrieves a service last accessed report that was created using the
* GenerateServiceLastAccessedDetails
operation. You can use the JobId
parameter in
* GetServiceLastAccessedDetails
to retrieve the status of your report job. When the report is
* complete, you can retrieve the generated report. The report includes a list of Amazon Web Services services that
* the resource (user, group, role, or managed policy) can access.
*
*
*
* Service last accessed data does not use other policy types when determining whether a resource could access a
* service. These other policy types include resource-based policies, access control lists, Organizations policies,
* IAM permissions boundaries, and STS assume role policies. It only applies permissions policy logic. For more
* about the evaluation of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* For each service that the resource could access using permissions policies, the operation returns details about
* the most recent access attempt. If there was no attempt, the service is listed without details about the most
* recent attempt to access the service. If the operation fails, the GetServiceLastAccessedDetails
* operation returns the reason that it failed.
*
*
* The GetServiceLastAccessedDetails
operation returns a list of services. This list includes the
* number of entities that have attempted to access the service and the date and time of the last attempt. It also
* returns the ARN of the following entity, depending on the resource ARN that you used to generate the report:
*
*
* -
*
* User – Returns the user ARN that you used to generate the report
*
*
* -
*
* Group – Returns the ARN of the group member (user) that last attempted to access the service
*
*
* -
*
* Role – Returns the role ARN that you used to generate the report
*
*
* -
*
* Policy – Returns the ARN of the user or role that last used the policy to attempt to access the service
*
*
*
*
* By default, the list is sorted by service namespace.
*
*
* If you specified ACTION_LEVEL
granularity when you generated the report, this operation returns
* service and action last accessed data. This includes the most recent access attempt for each tracked action
* within a service. Otherwise, this operation returns only service data.
*
*
* For more information about service and action last accessed data, see Reducing permissions
* using service last accessed data in the IAM User Guide.
*
*
* @param getServiceLastAccessedDetailsRequest
* @return Result of the GetServiceLastAccessedDetails operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServiceLastAccessedDetails
* @see AWS API Documentation
*/
default GetServiceLastAccessedDetailsResponse getServiceLastAccessedDetails(
GetServiceLastAccessedDetailsRequest getServiceLastAccessedDetailsRequest) throws NoSuchEntityException,
InvalidInputException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a service last accessed report that was created using the
* GenerateServiceLastAccessedDetails
operation. You can use the JobId
parameter in
* GetServiceLastAccessedDetails
to retrieve the status of your report job. When the report is
* complete, you can retrieve the generated report. The report includes a list of Amazon Web Services services that
* the resource (user, group, role, or managed policy) can access.
*
*
*
* Service last accessed data does not use other policy types when determining whether a resource could access a
* service. These other policy types include resource-based policies, access control lists, Organizations policies,
* IAM permissions boundaries, and STS assume role policies. It only applies permissions policy logic. For more
* about the evaluation of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* For each service that the resource could access using permissions policies, the operation returns details about
* the most recent access attempt. If there was no attempt, the service is listed without details about the most
* recent attempt to access the service. If the operation fails, the GetServiceLastAccessedDetails
* operation returns the reason that it failed.
*
*
* The GetServiceLastAccessedDetails
operation returns a list of services. This list includes the
* number of entities that have attempted to access the service and the date and time of the last attempt. It also
* returns the ARN of the following entity, depending on the resource ARN that you used to generate the report:
*
*
* -
*
* User – Returns the user ARN that you used to generate the report
*
*
* -
*
* Group – Returns the ARN of the group member (user) that last attempted to access the service
*
*
* -
*
* Role – Returns the role ARN that you used to generate the report
*
*
* -
*
* Policy – Returns the ARN of the user or role that last used the policy to attempt to access the service
*
*
*
*
* By default, the list is sorted by service namespace.
*
*
* If you specified ACTION_LEVEL
granularity when you generated the report, this operation returns
* service and action last accessed data. This includes the most recent access attempt for each tracked action
* within a service. Otherwise, this operation returns only service data.
*
*
* For more information about service and action last accessed data, see Reducing permissions
* using service last accessed data in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetServiceLastAccessedDetailsRequest.Builder}
* avoiding the need to create one manually via {@link GetServiceLastAccessedDetailsRequest#builder()}
*
*
* @param getServiceLastAccessedDetailsRequest
* A {@link Consumer} that will call methods on {@link GetServiceLastAccessedDetailsRequest.Builder} to
* create a request.
* @return Result of the GetServiceLastAccessedDetails operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServiceLastAccessedDetails
* @see AWS API Documentation
*/
default GetServiceLastAccessedDetailsResponse getServiceLastAccessedDetails(
Consumer getServiceLastAccessedDetailsRequest)
throws NoSuchEntityException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
return getServiceLastAccessedDetails(GetServiceLastAccessedDetailsRequest.builder()
.applyMutation(getServiceLastAccessedDetailsRequest).build());
}
/**
*
* After you generate a group or policy report using the GenerateServiceLastAccessedDetails
operation,
* you can use the JobId
parameter in GetServiceLastAccessedDetailsWithEntities
. This
* operation retrieves the status of your report job and a list of entities that could have used group or policy
* permissions to access the specified service.
*
*
* -
*
* Group – For a group report, this operation returns a list of users in the group that could have used the
* group’s policies in an attempt to access the service.
*
*
* -
*
* Policy – For a policy report, this operation returns a list of entities (users or roles) that could have
* used the policy in an attempt to access the service.
*
*
*
*
* You can also use this operation for user or role reports to retrieve details about those entities.
*
*
* If the operation fails, the GetServiceLastAccessedDetailsWithEntities
operation returns the reason
* that it failed.
*
*
* By default, the list of associated entities is sorted by date, with the most recent access listed first.
*
*
* @param getServiceLastAccessedDetailsWithEntitiesRequest
* @return Result of the GetServiceLastAccessedDetailsWithEntities operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServiceLastAccessedDetailsWithEntities
* @see AWS API Documentation
*/
default GetServiceLastAccessedDetailsWithEntitiesResponse getServiceLastAccessedDetailsWithEntities(
GetServiceLastAccessedDetailsWithEntitiesRequest getServiceLastAccessedDetailsWithEntitiesRequest)
throws NoSuchEntityException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* After you generate a group or policy report using the GenerateServiceLastAccessedDetails
operation,
* you can use the JobId
parameter in GetServiceLastAccessedDetailsWithEntities
. This
* operation retrieves the status of your report job and a list of entities that could have used group or policy
* permissions to access the specified service.
*
*
* -
*
* Group – For a group report, this operation returns a list of users in the group that could have used the
* group’s policies in an attempt to access the service.
*
*
* -
*
* Policy – For a policy report, this operation returns a list of entities (users or roles) that could have
* used the policy in an attempt to access the service.
*
*
*
*
* You can also use this operation for user or role reports to retrieve details about those entities.
*
*
* If the operation fails, the GetServiceLastAccessedDetailsWithEntities
operation returns the reason
* that it failed.
*
*
* By default, the list of associated entities is sorted by date, with the most recent access listed first.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetServiceLastAccessedDetailsWithEntitiesRequest.Builder} avoiding the need to create one manually via
* {@link GetServiceLastAccessedDetailsWithEntitiesRequest#builder()}
*
*
* @param getServiceLastAccessedDetailsWithEntitiesRequest
* A {@link Consumer} that will call methods on
* {@link GetServiceLastAccessedDetailsWithEntitiesRequest.Builder} to create a request.
* @return Result of the GetServiceLastAccessedDetailsWithEntities operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServiceLastAccessedDetailsWithEntities
* @see AWS API Documentation
*/
default GetServiceLastAccessedDetailsWithEntitiesResponse getServiceLastAccessedDetailsWithEntities(
Consumer getServiceLastAccessedDetailsWithEntitiesRequest)
throws NoSuchEntityException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
return getServiceLastAccessedDetailsWithEntities(GetServiceLastAccessedDetailsWithEntitiesRequest.builder()
.applyMutation(getServiceLastAccessedDetailsWithEntitiesRequest).build());
}
/**
*
* Retrieves the status of your service-linked role deletion. After you use DeleteServiceLinkedRole to submit
* a service-linked role for deletion, you can use the DeletionTaskId
parameter in
* GetServiceLinkedRoleDeletionStatus
to check the status of the deletion. If the deletion fails, this
* operation returns the reason that it failed, if that information is returned by the service.
*
*
* @param getServiceLinkedRoleDeletionStatusRequest
* @return Result of the GetServiceLinkedRoleDeletionStatus operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServiceLinkedRoleDeletionStatus
* @see AWS API Documentation
*/
default GetServiceLinkedRoleDeletionStatusResponse getServiceLinkedRoleDeletionStatus(
GetServiceLinkedRoleDeletionStatusRequest getServiceLinkedRoleDeletionStatusRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the status of your service-linked role deletion. After you use DeleteServiceLinkedRole to submit
* a service-linked role for deletion, you can use the DeletionTaskId
parameter in
* GetServiceLinkedRoleDeletionStatus
to check the status of the deletion. If the deletion fails, this
* operation returns the reason that it failed, if that information is returned by the service.
*
*
*
* This is a convenience which creates an instance of the {@link GetServiceLinkedRoleDeletionStatusRequest.Builder}
* avoiding the need to create one manually via {@link GetServiceLinkedRoleDeletionStatusRequest#builder()}
*
*
* @param getServiceLinkedRoleDeletionStatusRequest
* A {@link Consumer} that will call methods on {@link GetServiceLinkedRoleDeletionStatusRequest.Builder} to
* create a request.
* @return Result of the GetServiceLinkedRoleDeletionStatus operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetServiceLinkedRoleDeletionStatus
* @see AWS API Documentation
*/
default GetServiceLinkedRoleDeletionStatusResponse getServiceLinkedRoleDeletionStatus(
Consumer getServiceLinkedRoleDeletionStatusRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return getServiceLinkedRoleDeletionStatus(GetServiceLinkedRoleDeletionStatusRequest.builder()
.applyMutation(getServiceLinkedRoleDeletionStatusRequest).build());
}
/**
*
* Retrieves information about the specified IAM user, including the user's creation date, path, unique ID, and ARN.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID used to sign the request to this operation.
*
*
* @return Result of the GetUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetUser
* @see #getUser(GetUserRequest)
* @see AWS API
* Documentation
*/
default GetUserResponse getUser() throws NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return getUser(GetUserRequest.builder().build());
}
/**
*
* Retrieves information about the specified IAM user, including the user's creation date, path, unique ID, and ARN.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID used to sign the request to this operation.
*
*
* @param getUserRequest
* @return Result of the GetUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetUser
* @see AWS API
* Documentation
*/
default GetUserResponse getUser(GetUserRequest getUserRequest) throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the specified IAM user, including the user's creation date, path, unique ID, and ARN.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID used to sign the request to this operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetUserRequest.Builder} avoiding the need to create
* one manually via {@link GetUserRequest#builder()}
*
*
* @param getUserRequest
* A {@link Consumer} that will call methods on {@link GetUserRequest.Builder} to create a request.
* @return Result of the GetUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetUser
* @see AWS API
* Documentation
*/
default GetUserResponse getUser(Consumer getUserRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getUser(GetUserRequest.builder().applyMutation(getUserRequest).build());
}
/**
*
* Retrieves the specified inline policy document that is embedded in the specified IAM user.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* An IAM user can also have managed policies attached to it. To retrieve a managed policy document that is attached
* to a user, use GetPolicy to determine the policy's default version. Then use GetPolicyVersion to
* retrieve the policy document.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param getUserPolicyRequest
* @return Result of the GetUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetUserPolicy
* @see AWS API
* Documentation
*/
default GetUserPolicyResponse getUserPolicy(GetUserPolicyRequest getUserPolicyRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the specified inline policy document that is embedded in the specified IAM user.
*
*
*
* Policies returned by this operation are URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
*
* An IAM user can also have managed policies attached to it. To retrieve a managed policy document that is attached
* to a user, use GetPolicy to determine the policy's default version. Then use GetPolicyVersion to
* retrieve the policy document.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetUserPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetUserPolicyRequest#builder()}
*
*
* @param getUserPolicyRequest
* A {@link Consumer} that will call methods on {@link GetUserPolicyRequest.Builder} to create a request.
* @return Result of the GetUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.GetUserPolicy
* @see AWS API
* Documentation
*/
default GetUserPolicyResponse getUserPolicy(Consumer getUserPolicyRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return getUserPolicy(GetUserPolicyRequest.builder().applyMutation(getUserPolicyRequest).build());
}
/**
*
* Returns information about the access key IDs associated with the specified IAM user. If there is none, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation.
*
*
*
* @return Result of the ListAccessKeys operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccessKeys
* @see #listAccessKeys(ListAccessKeysRequest)
* @see AWS API
* Documentation
*/
default ListAccessKeysResponse listAccessKeys() throws NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listAccessKeys(ListAccessKeysRequest.builder().build());
}
/**
*
* Returns information about the access key IDs associated with the specified IAM user. If there is none, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation.
*
*
*
* @param listAccessKeysRequest
* @return Result of the ListAccessKeys operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccessKeys
* @see AWS API
* Documentation
*/
default ListAccessKeysResponse listAccessKeys(ListAccessKeysRequest listAccessKeysRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the access key IDs associated with the specified IAM user. If there is none, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessKeysRequest.Builder} avoiding the need to
* create one manually via {@link ListAccessKeysRequest#builder()}
*
*
* @param listAccessKeysRequest
* A {@link Consumer} that will call methods on {@link ListAccessKeysRequest.Builder} to create a request.
* @return Result of the ListAccessKeys operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccessKeys
* @see AWS API
* Documentation
*/
default ListAccessKeysResponse listAccessKeys(Consumer listAccessKeysRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAccessKeys(ListAccessKeysRequest.builder().applyMutation(listAccessKeysRequest).build());
}
/**
*
* Returns information about the access key IDs associated with the specified IAM user. If there is none, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation.
*
*
*
* This is a variant of {@link #listAccessKeys(software.amazon.awssdk.services.iam.model.ListAccessKeysRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAccessKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessKeys(software.amazon.awssdk.services.iam.model.ListAccessKeysRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccessKeys
* @see #listAccessKeysPaginator(ListAccessKeysRequest)
* @see AWS API
* Documentation
*/
default ListAccessKeysIterable listAccessKeysPaginator() throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listAccessKeysPaginator(ListAccessKeysRequest.builder().build());
}
/**
*
* Returns information about the access key IDs associated with the specified IAM user. If there is none, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation.
*
*
*
* This is a variant of {@link #listAccessKeys(software.amazon.awssdk.services.iam.model.ListAccessKeysRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAccessKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessKeys(software.amazon.awssdk.services.iam.model.ListAccessKeysRequest)} operation.
*
*
* @param listAccessKeysRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccessKeys
* @see AWS API
* Documentation
*/
default ListAccessKeysIterable listAccessKeysPaginator(ListAccessKeysRequest listAccessKeysRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the access key IDs associated with the specified IAM user. If there is none, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation.
*
*
*
* This is a variant of {@link #listAccessKeys(software.amazon.awssdk.services.iam.model.ListAccessKeysRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAccessKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccessKeysIterable responses = client.listAccessKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessKeys(software.amazon.awssdk.services.iam.model.ListAccessKeysRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAccessKeysRequest.Builder} avoiding the need to
* create one manually via {@link ListAccessKeysRequest#builder()}
*
*
* @param listAccessKeysRequest
* A {@link Consumer} that will call methods on {@link ListAccessKeysRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccessKeys
* @see AWS API
* Documentation
*/
default ListAccessKeysIterable listAccessKeysPaginator(Consumer listAccessKeysRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAccessKeysPaginator(ListAccessKeysRequest.builder().applyMutation(listAccessKeysRequest).build());
}
/**
*
* Lists the account alias associated with the Amazon Web Services account (Note: you can have only one). For
* information about using an Amazon Web Services account alias, see Using an alias for your Amazon Web
* Services account ID in the IAM User Guide.
*
*
* @return Result of the ListAccountAliases operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccountAliases
* @see #listAccountAliases(ListAccountAliasesRequest)
* @see AWS API
* Documentation
*/
default ListAccountAliasesResponse listAccountAliases() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listAccountAliases(ListAccountAliasesRequest.builder().build());
}
/**
*
* Lists the account alias associated with the Amazon Web Services account (Note: you can have only one). For
* information about using an Amazon Web Services account alias, see Using an alias for your Amazon Web
* Services account ID in the IAM User Guide.
*
*
* @param listAccountAliasesRequest
* @return Result of the ListAccountAliases operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccountAliases
* @see AWS API
* Documentation
*/
default ListAccountAliasesResponse listAccountAliases(ListAccountAliasesRequest listAccountAliasesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the account alias associated with the Amazon Web Services account (Note: you can have only one). For
* information about using an Amazon Web Services account alias, see Using an alias for your Amazon Web
* Services account ID in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccountAliasesRequest.Builder} avoiding the
* need to create one manually via {@link ListAccountAliasesRequest#builder()}
*
*
* @param listAccountAliasesRequest
* A {@link Consumer} that will call methods on {@link ListAccountAliasesRequest.Builder} to create a
* request.
* @return Result of the ListAccountAliases operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccountAliases
* @see AWS API
* Documentation
*/
default ListAccountAliasesResponse listAccountAliases(Consumer listAccountAliasesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAccountAliases(ListAccountAliasesRequest.builder().applyMutation(listAccountAliasesRequest).build());
}
/**
*
* Lists the account alias associated with the Amazon Web Services account (Note: you can have only one). For
* information about using an Amazon Web Services account alias, see Using an alias for your Amazon Web
* Services account ID in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #listAccountAliases(software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client.listAccountAliasesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client
* .listAccountAliasesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAccountAliasesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client.listAccountAliasesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountAliases(software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccountAliases
* @see #listAccountAliasesPaginator(ListAccountAliasesRequest)
* @see AWS API
* Documentation
*/
default ListAccountAliasesIterable listAccountAliasesPaginator() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listAccountAliasesPaginator(ListAccountAliasesRequest.builder().build());
}
/**
*
* Lists the account alias associated with the Amazon Web Services account (Note: you can have only one). For
* information about using an Amazon Web Services account alias, see Using an alias for your Amazon Web
* Services account ID in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #listAccountAliases(software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client.listAccountAliasesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client
* .listAccountAliasesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAccountAliasesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client.listAccountAliasesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountAliases(software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest)} operation.
*
*
* @param listAccountAliasesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccountAliases
* @see AWS API
* Documentation
*/
default ListAccountAliasesIterable listAccountAliasesPaginator(ListAccountAliasesRequest listAccountAliasesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the account alias associated with the Amazon Web Services account (Note: you can have only one). For
* information about using an Amazon Web Services account alias, see Using an alias for your Amazon Web
* Services account ID in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #listAccountAliases(software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client.listAccountAliasesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client
* .listAccountAliasesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAccountAliasesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAccountAliasesIterable responses = client.listAccountAliasesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountAliases(software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAccountAliasesRequest.Builder} avoiding the
* need to create one manually via {@link ListAccountAliasesRequest#builder()}
*
*
* @param listAccountAliasesRequest
* A {@link Consumer} that will call methods on {@link ListAccountAliasesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAccountAliases
* @see AWS API
* Documentation
*/
default ListAccountAliasesIterable listAccountAliasesPaginator(
Consumer listAccountAliasesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listAccountAliasesPaginator(ListAccountAliasesRequest.builder().applyMutation(listAccountAliasesRequest).build());
}
/**
*
* Lists all managed policies that are attached to the specified IAM group.
*
*
* An IAM group can also have inline policies embedded with it. To list the inline policies for a group, use
* ListGroupPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
* @param listAttachedGroupPoliciesRequest
* @return Result of the ListAttachedGroupPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedGroupPolicies
* @see AWS
* API Documentation
*/
default ListAttachedGroupPoliciesResponse listAttachedGroupPolicies(
ListAttachedGroupPoliciesRequest listAttachedGroupPoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all managed policies that are attached to the specified IAM group.
*
*
* An IAM group can also have inline policies embedded with it. To list the inline policies for a group, use
* ListGroupPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a convenience which creates an instance of the {@link ListAttachedGroupPoliciesRequest.Builder} avoiding
* the need to create one manually via {@link ListAttachedGroupPoliciesRequest#builder()}
*
*
* @param listAttachedGroupPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedGroupPoliciesRequest.Builder} to create a
* request.
* @return Result of the ListAttachedGroupPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedGroupPolicies
* @see AWS
* API Documentation
*/
default ListAttachedGroupPoliciesResponse listAttachedGroupPolicies(
Consumer listAttachedGroupPoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAttachedGroupPolicies(ListAttachedGroupPoliciesRequest.builder()
.applyMutation(listAttachedGroupPoliciesRequest).build());
}
/**
*
* Lists all managed policies that are attached to the specified IAM group.
*
*
* An IAM group can also have inline policies embedded with it. To list the inline policies for a group, use
* ListGroupPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listAttachedGroupPolicies(software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedGroupPoliciesIterable responses = client.listAttachedGroupPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAttachedGroupPoliciesIterable responses = client
* .listAttachedGroupPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedGroupPoliciesIterable responses = client.listAttachedGroupPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedGroupPolicies(software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesRequest)}
* operation.
*
*
* @param listAttachedGroupPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedGroupPolicies
* @see AWS
* API Documentation
*/
default ListAttachedGroupPoliciesIterable listAttachedGroupPoliciesPaginator(
ListAttachedGroupPoliciesRequest listAttachedGroupPoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all managed policies that are attached to the specified IAM group.
*
*
* An IAM group can also have inline policies embedded with it. To list the inline policies for a group, use
* ListGroupPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listAttachedGroupPolicies(software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedGroupPoliciesIterable responses = client.listAttachedGroupPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAttachedGroupPoliciesIterable responses = client
* .listAttachedGroupPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedGroupPoliciesIterable responses = client.listAttachedGroupPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedGroupPolicies(software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListAttachedGroupPoliciesRequest.Builder} avoiding
* the need to create one manually via {@link ListAttachedGroupPoliciesRequest#builder()}
*
*
* @param listAttachedGroupPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedGroupPoliciesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedGroupPolicies
* @see AWS
* API Documentation
*/
default ListAttachedGroupPoliciesIterable listAttachedGroupPoliciesPaginator(
Consumer listAttachedGroupPoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAttachedGroupPoliciesPaginator(ListAttachedGroupPoliciesRequest.builder()
.applyMutation(listAttachedGroupPoliciesRequest).build());
}
/**
*
* Lists all managed policies that are attached to the specified IAM role.
*
*
* An IAM role can also have inline policies embedded with it. To list the inline policies for a role, use
* ListRolePolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified role (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
* @param listAttachedRolePoliciesRequest
* @return Result of the ListAttachedRolePolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedRolePolicies
* @see AWS
* API Documentation
*/
default ListAttachedRolePoliciesResponse listAttachedRolePolicies(
ListAttachedRolePoliciesRequest listAttachedRolePoliciesRequest) throws NoSuchEntityException, InvalidInputException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all managed policies that are attached to the specified IAM role.
*
*
* An IAM role can also have inline policies embedded with it. To list the inline policies for a role, use
* ListRolePolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified role (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a convenience which creates an instance of the {@link ListAttachedRolePoliciesRequest.Builder} avoiding
* the need to create one manually via {@link ListAttachedRolePoliciesRequest#builder()}
*
*
* @param listAttachedRolePoliciesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedRolePoliciesRequest.Builder} to create a
* request.
* @return Result of the ListAttachedRolePolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedRolePolicies
* @see AWS
* API Documentation
*/
default ListAttachedRolePoliciesResponse listAttachedRolePolicies(
Consumer listAttachedRolePoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAttachedRolePolicies(ListAttachedRolePoliciesRequest.builder().applyMutation(listAttachedRolePoliciesRequest)
.build());
}
/**
*
* Lists all managed policies that are attached to the specified IAM role.
*
*
* An IAM role can also have inline policies embedded with it. To list the inline policies for a role, use
* ListRolePolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified role (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listAttachedRolePolicies(software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedRolePoliciesIterable responses = client.listAttachedRolePoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAttachedRolePoliciesIterable responses = client
* .listAttachedRolePoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedRolePoliciesIterable responses = client.listAttachedRolePoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedRolePolicies(software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesRequest)}
* operation.
*
*
* @param listAttachedRolePoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedRolePolicies
* @see AWS
* API Documentation
*/
default ListAttachedRolePoliciesIterable listAttachedRolePoliciesPaginator(
ListAttachedRolePoliciesRequest listAttachedRolePoliciesRequest) throws NoSuchEntityException, InvalidInputException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all managed policies that are attached to the specified IAM role.
*
*
* An IAM role can also have inline policies embedded with it. To list the inline policies for a role, use
* ListRolePolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified role (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listAttachedRolePolicies(software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedRolePoliciesIterable responses = client.listAttachedRolePoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAttachedRolePoliciesIterable responses = client
* .listAttachedRolePoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedRolePoliciesIterable responses = client.listAttachedRolePoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedRolePolicies(software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListAttachedRolePoliciesRequest.Builder} avoiding
* the need to create one manually via {@link ListAttachedRolePoliciesRequest#builder()}
*
*
* @param listAttachedRolePoliciesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedRolePoliciesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedRolePolicies
* @see AWS
* API Documentation
*/
default ListAttachedRolePoliciesIterable listAttachedRolePoliciesPaginator(
Consumer listAttachedRolePoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAttachedRolePoliciesPaginator(ListAttachedRolePoliciesRequest.builder()
.applyMutation(listAttachedRolePoliciesRequest).build());
}
/**
*
* Lists all managed policies that are attached to the specified IAM user.
*
*
* An IAM user can also have inline policies embedded with it. To list the inline policies for a user, use
* ListUserPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
* @param listAttachedUserPoliciesRequest
* @return Result of the ListAttachedUserPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedUserPolicies
* @see AWS
* API Documentation
*/
default ListAttachedUserPoliciesResponse listAttachedUserPolicies(
ListAttachedUserPoliciesRequest listAttachedUserPoliciesRequest) throws NoSuchEntityException, InvalidInputException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all managed policies that are attached to the specified IAM user.
*
*
* An IAM user can also have inline policies embedded with it. To list the inline policies for a user, use
* ListUserPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a convenience which creates an instance of the {@link ListAttachedUserPoliciesRequest.Builder} avoiding
* the need to create one manually via {@link ListAttachedUserPoliciesRequest#builder()}
*
*
* @param listAttachedUserPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedUserPoliciesRequest.Builder} to create a
* request.
* @return Result of the ListAttachedUserPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedUserPolicies
* @see AWS
* API Documentation
*/
default ListAttachedUserPoliciesResponse listAttachedUserPolicies(
Consumer listAttachedUserPoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAttachedUserPolicies(ListAttachedUserPoliciesRequest.builder().applyMutation(listAttachedUserPoliciesRequest)
.build());
}
/**
*
* Lists all managed policies that are attached to the specified IAM user.
*
*
* An IAM user can also have inline policies embedded with it. To list the inline policies for a user, use
* ListUserPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listAttachedUserPolicies(software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedUserPoliciesIterable responses = client.listAttachedUserPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAttachedUserPoliciesIterable responses = client
* .listAttachedUserPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedUserPoliciesIterable responses = client.listAttachedUserPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedUserPolicies(software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesRequest)}
* operation.
*
*
* @param listAttachedUserPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedUserPolicies
* @see AWS
* API Documentation
*/
default ListAttachedUserPoliciesIterable listAttachedUserPoliciesPaginator(
ListAttachedUserPoliciesRequest listAttachedUserPoliciesRequest) throws NoSuchEntityException, InvalidInputException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all managed policies that are attached to the specified IAM user.
*
*
* An IAM user can also have inline policies embedded with it. To list the inline policies for a user, use
* ListUserPolicies. For information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. You can use the
* PathPrefix
parameter to limit the list of policies to only those matching the specified path prefix.
* If there are no policies attached to the specified group (or none that match the specified path prefix), the
* operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listAttachedUserPolicies(software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedUserPoliciesIterable responses = client.listAttachedUserPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListAttachedUserPoliciesIterable responses = client
* .listAttachedUserPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListAttachedUserPoliciesIterable responses = client.listAttachedUserPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedUserPolicies(software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListAttachedUserPoliciesRequest.Builder} avoiding
* the need to create one manually via {@link ListAttachedUserPoliciesRequest#builder()}
*
*
* @param listAttachedUserPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedUserPoliciesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListAttachedUserPolicies
* @see AWS
* API Documentation
*/
default ListAttachedUserPoliciesIterable listAttachedUserPoliciesPaginator(
Consumer listAttachedUserPoliciesRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listAttachedUserPoliciesPaginator(ListAttachedUserPoliciesRequest.builder()
.applyMutation(listAttachedUserPoliciesRequest).build());
}
/**
*
* Lists all IAM users, groups, and roles that the specified managed policy is attached to.
*
*
* You can use the optional EntityFilter
parameter to limit the results to a particular type of entity
* (users, groups, or roles). For example, to list only the roles that are attached to the specified policy, set
* EntityFilter
to Role
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listEntitiesForPolicyRequest
* @return Result of the ListEntitiesForPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListEntitiesForPolicy
* @see AWS API
* Documentation
*/
default ListEntitiesForPolicyResponse listEntitiesForPolicy(ListEntitiesForPolicyRequest listEntitiesForPolicyRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all IAM users, groups, and roles that the specified managed policy is attached to.
*
*
* You can use the optional EntityFilter
parameter to limit the results to a particular type of entity
* (users, groups, or roles). For example, to list only the roles that are attached to the specified policy, set
* EntityFilter
to Role
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListEntitiesForPolicyRequest.Builder} avoiding the
* need to create one manually via {@link ListEntitiesForPolicyRequest#builder()}
*
*
* @param listEntitiesForPolicyRequest
* A {@link Consumer} that will call methods on {@link ListEntitiesForPolicyRequest.Builder} to create a
* request.
* @return Result of the ListEntitiesForPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListEntitiesForPolicy
* @see AWS API
* Documentation
*/
default ListEntitiesForPolicyResponse listEntitiesForPolicy(
Consumer listEntitiesForPolicyRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listEntitiesForPolicy(ListEntitiesForPolicyRequest.builder().applyMutation(listEntitiesForPolicyRequest).build());
}
/**
*
* Lists all IAM users, groups, and roles that the specified managed policy is attached to.
*
*
* You can use the optional EntityFilter
parameter to limit the results to a particular type of entity
* (users, groups, or roles). For example, to list only the roles that are attached to the specified policy, set
* EntityFilter
to Role
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listEntitiesForPolicy(software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListEntitiesForPolicyIterable responses = client.listEntitiesForPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListEntitiesForPolicyIterable responses = client
* .listEntitiesForPolicyPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListEntitiesForPolicyIterable responses = client.listEntitiesForPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEntitiesForPolicy(software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyRequest)}
* operation.
*
*
* @param listEntitiesForPolicyRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListEntitiesForPolicy
* @see AWS API
* Documentation
*/
default ListEntitiesForPolicyIterable listEntitiesForPolicyPaginator(ListEntitiesForPolicyRequest listEntitiesForPolicyRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all IAM users, groups, and roles that the specified managed policy is attached to.
*
*
* You can use the optional EntityFilter
parameter to limit the results to a particular type of entity
* (users, groups, or roles). For example, to list only the roles that are attached to the specified policy, set
* EntityFilter
to Role
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listEntitiesForPolicy(software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListEntitiesForPolicyIterable responses = client.listEntitiesForPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListEntitiesForPolicyIterable responses = client
* .listEntitiesForPolicyPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListEntitiesForPolicyIterable responses = client.listEntitiesForPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEntitiesForPolicy(software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListEntitiesForPolicyRequest.Builder} avoiding the
* need to create one manually via {@link ListEntitiesForPolicyRequest#builder()}
*
*
* @param listEntitiesForPolicyRequest
* A {@link Consumer} that will call methods on {@link ListEntitiesForPolicyRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListEntitiesForPolicy
* @see AWS API
* Documentation
*/
default ListEntitiesForPolicyIterable listEntitiesForPolicyPaginator(
Consumer listEntitiesForPolicyRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listEntitiesForPolicyPaginator(ListEntitiesForPolicyRequest.builder().applyMutation(listEntitiesForPolicyRequest)
.build());
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM group.
*
*
* An IAM group can also have managed policies attached to it. To list the managed policies that are attached to a
* group, use ListAttachedGroupPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified group, the operation returns an empty list.
*
*
* @param listGroupPoliciesRequest
* @return Result of the ListGroupPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupPolicies
* @see AWS API
* Documentation
*/
default ListGroupPoliciesResponse listGroupPolicies(ListGroupPoliciesRequest listGroupPoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM group.
*
*
* An IAM group can also have managed policies attached to it. To list the managed policies that are attached to a
* group, use ListAttachedGroupPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified group, the operation returns an empty list.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupPoliciesRequest.Builder} avoiding the need
* to create one manually via {@link ListGroupPoliciesRequest#builder()}
*
*
* @param listGroupPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListGroupPoliciesRequest.Builder} to create a request.
* @return Result of the ListGroupPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupPolicies
* @see AWS API
* Documentation
*/
default ListGroupPoliciesResponse listGroupPolicies(Consumer listGroupPoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listGroupPolicies(ListGroupPoliciesRequest.builder().applyMutation(listGroupPoliciesRequest).build());
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM group.
*
*
* An IAM group can also have managed policies attached to it. To list the managed policies that are attached to a
* group, use ListAttachedGroupPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified group, the operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listGroupPolicies(software.amazon.awssdk.services.iam.model.ListGroupPoliciesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupPoliciesIterable responses = client.listGroupPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListGroupPoliciesIterable responses = client
* .listGroupPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListGroupPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupPoliciesIterable responses = client.listGroupPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupPolicies(software.amazon.awssdk.services.iam.model.ListGroupPoliciesRequest)} operation.
*
*
* @param listGroupPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupPolicies
* @see AWS API
* Documentation
*/
default ListGroupPoliciesIterable listGroupPoliciesPaginator(ListGroupPoliciesRequest listGroupPoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM group.
*
*
* An IAM group can also have managed policies attached to it. To list the managed policies that are attached to a
* group, use ListAttachedGroupPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified group, the operation returns an empty list.
*
*
*
* This is a variant of
* {@link #listGroupPolicies(software.amazon.awssdk.services.iam.model.ListGroupPoliciesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupPoliciesIterable responses = client.listGroupPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListGroupPoliciesIterable responses = client
* .listGroupPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListGroupPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupPoliciesIterable responses = client.listGroupPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupPolicies(software.amazon.awssdk.services.iam.model.ListGroupPoliciesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListGroupPoliciesRequest.Builder} avoiding the need
* to create one manually via {@link ListGroupPoliciesRequest#builder()}
*
*
* @param listGroupPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListGroupPoliciesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupPolicies
* @see AWS API
* Documentation
*/
default ListGroupPoliciesIterable listGroupPoliciesPaginator(
Consumer listGroupPoliciesRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listGroupPoliciesPaginator(ListGroupPoliciesRequest.builder().applyMutation(listGroupPoliciesRequest).build());
}
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @return Result of the ListGroups operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroups
* @see #listGroups(ListGroupsRequest)
* @see AWS API
* Documentation
*/
default ListGroupsResponse listGroups() throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listGroups(ListGroupsRequest.builder().build());
}
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listGroupsRequest
* @return Result of the ListGroups operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsResponse listGroups(ListGroupsRequest listGroupsRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on {@link ListGroupsRequest.Builder} to create a request.
* @return Result of the ListGroups operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsResponse listGroups(Consumer listGroupsRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listGroups(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.iam.model.ListGroupsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.iam.model.ListGroupsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroups
* @see #listGroupsPaginator(ListGroupsRequest)
* @see AWS API
* Documentation
*/
default ListGroupsIterable listGroupsPaginator() throws ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return listGroupsPaginator(ListGroupsRequest.builder().build());
}
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.iam.model.ListGroupsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.iam.model.ListGroupsRequest)} operation.
*
*
* @param listGroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsIterable listGroupsPaginator(ListGroupsRequest listGroupsRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listGroups(software.amazon.awssdk.services.iam.model.ListGroupsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.iam.model.ListGroupsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on {@link ListGroupsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroups
* @see AWS API
* Documentation
*/
default ListGroupsIterable listGroupsPaginator(Consumer listGroupsRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listGroupsPaginator(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* Lists the IAM groups that the specified IAM user belongs to.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listGroupsForUserRequest
* @return Result of the ListGroupsForUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupsForUser
* @see AWS API
* Documentation
*/
default ListGroupsForUserResponse listGroupsForUser(ListGroupsForUserRequest listGroupsForUserRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM groups that the specified IAM user belongs to.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsForUserRequest.Builder} avoiding the need
* to create one manually via {@link ListGroupsForUserRequest#builder()}
*
*
* @param listGroupsForUserRequest
* A {@link Consumer} that will call methods on {@link ListGroupsForUserRequest.Builder} to create a request.
* @return Result of the ListGroupsForUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupsForUser
* @see AWS API
* Documentation
*/
default ListGroupsForUserResponse listGroupsForUser(Consumer listGroupsForUserRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listGroupsForUser(ListGroupsForUserRequest.builder().applyMutation(listGroupsForUserRequest).build());
}
/**
*
* Lists the IAM groups that the specified IAM user belongs to.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listGroupsForUser(software.amazon.awssdk.services.iam.model.ListGroupsForUserRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsForUserIterable responses = client.listGroupsForUserPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListGroupsForUserIterable responses = client
* .listGroupsForUserPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListGroupsForUserResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsForUserIterable responses = client.listGroupsForUserPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupsForUser(software.amazon.awssdk.services.iam.model.ListGroupsForUserRequest)} operation.
*
*
* @param listGroupsForUserRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupsForUser
* @see AWS API
* Documentation
*/
default ListGroupsForUserIterable listGroupsForUserPaginator(ListGroupsForUserRequest listGroupsForUserRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM groups that the specified IAM user belongs to.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listGroupsForUser(software.amazon.awssdk.services.iam.model.ListGroupsForUserRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsForUserIterable responses = client.listGroupsForUserPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListGroupsForUserIterable responses = client
* .listGroupsForUserPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListGroupsForUserResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListGroupsForUserIterable responses = client.listGroupsForUserPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupsForUser(software.amazon.awssdk.services.iam.model.ListGroupsForUserRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListGroupsForUserRequest.Builder} avoiding the need
* to create one manually via {@link ListGroupsForUserRequest#builder()}
*
*
* @param listGroupsForUserRequest
* A {@link Consumer} that will call methods on {@link ListGroupsForUserRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListGroupsForUser
* @see AWS API
* Documentation
*/
default ListGroupsForUserIterable listGroupsForUserPaginator(
Consumer listGroupsForUserRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listGroupsForUserPaginator(ListGroupsForUserRequest.builder().applyMutation(listGroupsForUserRequest).build());
}
/**
*
* Lists the tags that are attached to the specified IAM instance profile. The returned list of tags is sorted by
* tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param listInstanceProfileTagsRequest
* @return Result of the ListInstanceProfileTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfileTags
* @see AWS
* API Documentation
*/
default ListInstanceProfileTagsResponse listInstanceProfileTags(ListInstanceProfileTagsRequest listInstanceProfileTagsRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified IAM instance profile. The returned list of tags is sorted by
* tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListInstanceProfileTagsRequest.Builder} avoiding
* the need to create one manually via {@link ListInstanceProfileTagsRequest#builder()}
*
*
* @param listInstanceProfileTagsRequest
* A {@link Consumer} that will call methods on {@link ListInstanceProfileTagsRequest.Builder} to create a
* request.
* @return Result of the ListInstanceProfileTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfileTags
* @see AWS
* API Documentation
*/
default ListInstanceProfileTagsResponse listInstanceProfileTags(
Consumer listInstanceProfileTagsRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listInstanceProfileTags(ListInstanceProfileTagsRequest.builder().applyMutation(listInstanceProfileTagsRequest)
.build());
}
/**
*
* Lists the instance profiles that have the specified path prefix. If there are none, the operation returns an
* empty list. For more information about instance profiles, see About instance profiles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an instance profile, see GetInstanceProfile.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @return Result of the ListInstanceProfiles operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfiles
* @see #listInstanceProfiles(ListInstanceProfilesRequest)
* @see AWS API
* Documentation
*/
default ListInstanceProfilesResponse listInstanceProfiles() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listInstanceProfiles(ListInstanceProfilesRequest.builder().build());
}
/**
*
* Lists the instance profiles that have the specified path prefix. If there are none, the operation returns an
* empty list. For more information about instance profiles, see About instance profiles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an instance profile, see GetInstanceProfile.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listInstanceProfilesRequest
* @return Result of the ListInstanceProfiles operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfiles
* @see AWS API
* Documentation
*/
default ListInstanceProfilesResponse listInstanceProfiles(ListInstanceProfilesRequest listInstanceProfilesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the instance profiles that have the specified path prefix. If there are none, the operation returns an
* empty list. For more information about instance profiles, see About instance profiles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an instance profile, see GetInstanceProfile.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListInstanceProfilesRequest.Builder} avoiding the
* need to create one manually via {@link ListInstanceProfilesRequest#builder()}
*
*
* @param listInstanceProfilesRequest
* A {@link Consumer} that will call methods on {@link ListInstanceProfilesRequest.Builder} to create a
* request.
* @return Result of the ListInstanceProfiles operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfiles
* @see AWS API
* Documentation
*/
default ListInstanceProfilesResponse listInstanceProfiles(
Consumer listInstanceProfilesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listInstanceProfiles(ListInstanceProfilesRequest.builder().applyMutation(listInstanceProfilesRequest).build());
}
/**
*
* Lists the instance profiles that have the specified path prefix. If there are none, the operation returns an
* empty list. For more information about instance profiles, see About instance profiles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an instance profile, see GetInstanceProfile.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listInstanceProfiles(software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client.listInstanceProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client
* .listInstanceProfilesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListInstanceProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client.listInstanceProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceProfiles(software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfiles
* @see #listInstanceProfilesPaginator(ListInstanceProfilesRequest)
* @see AWS API
* Documentation
*/
default ListInstanceProfilesIterable listInstanceProfilesPaginator() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listInstanceProfilesPaginator(ListInstanceProfilesRequest.builder().build());
}
/**
*
* Lists the instance profiles that have the specified path prefix. If there are none, the operation returns an
* empty list. For more information about instance profiles, see About instance profiles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an instance profile, see GetInstanceProfile.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listInstanceProfiles(software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client.listInstanceProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client
* .listInstanceProfilesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListInstanceProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client.listInstanceProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceProfiles(software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest)}
* operation.
*
*
* @param listInstanceProfilesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfiles
* @see AWS API
* Documentation
*/
default ListInstanceProfilesIterable listInstanceProfilesPaginator(ListInstanceProfilesRequest listInstanceProfilesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the instance profiles that have the specified path prefix. If there are none, the operation returns an
* empty list. For more information about instance profiles, see About instance profiles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an instance profile, see GetInstanceProfile.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listInstanceProfiles(software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client.listInstanceProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client
* .listInstanceProfilesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListInstanceProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesIterable responses = client.listInstanceProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceProfiles(software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListInstanceProfilesRequest.Builder} avoiding the
* need to create one manually via {@link ListInstanceProfilesRequest#builder()}
*
*
* @param listInstanceProfilesRequest
* A {@link Consumer} that will call methods on {@link ListInstanceProfilesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfiles
* @see AWS API
* Documentation
*/
default ListInstanceProfilesIterable listInstanceProfilesPaginator(
Consumer listInstanceProfilesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listInstanceProfilesPaginator(ListInstanceProfilesRequest.builder().applyMutation(listInstanceProfilesRequest)
.build());
}
/**
*
* Lists the instance profiles that have the specified associated IAM role. If there are none, the operation returns
* an empty list. For more information about instance profiles, go to About instance profiles.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listInstanceProfilesForRoleRequest
* @return Result of the ListInstanceProfilesForRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfilesForRole
* @see AWS API Documentation
*/
default ListInstanceProfilesForRoleResponse listInstanceProfilesForRole(
ListInstanceProfilesForRoleRequest listInstanceProfilesForRoleRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the instance profiles that have the specified associated IAM role. If there are none, the operation returns
* an empty list. For more information about instance profiles, go to About instance profiles.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListInstanceProfilesForRoleRequest.Builder}
* avoiding the need to create one manually via {@link ListInstanceProfilesForRoleRequest#builder()}
*
*
* @param listInstanceProfilesForRoleRequest
* A {@link Consumer} that will call methods on {@link ListInstanceProfilesForRoleRequest.Builder} to create
* a request.
* @return Result of the ListInstanceProfilesForRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfilesForRole
* @see AWS API Documentation
*/
default ListInstanceProfilesForRoleResponse listInstanceProfilesForRole(
Consumer listInstanceProfilesForRoleRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listInstanceProfilesForRole(ListInstanceProfilesForRoleRequest.builder()
.applyMutation(listInstanceProfilesForRoleRequest).build());
}
/**
*
* Lists the instance profiles that have the specified associated IAM role. If there are none, the operation returns
* an empty list. For more information about instance profiles, go to About instance profiles.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listInstanceProfilesForRole(software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesForRoleIterable responses = client.listInstanceProfilesForRolePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesForRoleIterable responses = client
* .listInstanceProfilesForRolePaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesForRoleIterable responses = client.listInstanceProfilesForRolePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceProfilesForRole(software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleRequest)}
* operation.
*
*
* @param listInstanceProfilesForRoleRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfilesForRole
* @see AWS API Documentation
*/
default ListInstanceProfilesForRoleIterable listInstanceProfilesForRolePaginator(
ListInstanceProfilesForRoleRequest listInstanceProfilesForRoleRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the instance profiles that have the specified associated IAM role. If there are none, the operation returns
* an empty list. For more information about instance profiles, go to About instance profiles.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listInstanceProfilesForRole(software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesForRoleIterable responses = client.listInstanceProfilesForRolePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesForRoleIterable responses = client
* .listInstanceProfilesForRolePaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListInstanceProfilesForRoleIterable responses = client.listInstanceProfilesForRolePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceProfilesForRole(software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListInstanceProfilesForRoleRequest.Builder}
* avoiding the need to create one manually via {@link ListInstanceProfilesForRoleRequest#builder()}
*
*
* @param listInstanceProfilesForRoleRequest
* A {@link Consumer} that will call methods on {@link ListInstanceProfilesForRoleRequest.Builder} to create
* a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListInstanceProfilesForRole
* @see AWS API Documentation
*/
default ListInstanceProfilesForRoleIterable listInstanceProfilesForRolePaginator(
Consumer listInstanceProfilesForRoleRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listInstanceProfilesForRolePaginator(ListInstanceProfilesForRoleRequest.builder()
.applyMutation(listInstanceProfilesForRoleRequest).build());
}
/**
*
* Lists the tags that are attached to the specified IAM virtual multi-factor authentication (MFA) device. The
* returned list of tags is sorted by tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param listMfaDeviceTagsRequest
* @return Result of the ListMFADeviceTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADeviceTags
* @see AWS API
* Documentation
*/
default ListMfaDeviceTagsResponse listMFADeviceTags(ListMfaDeviceTagsRequest listMfaDeviceTagsRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified IAM virtual multi-factor authentication (MFA) device. The
* returned list of tags is sorted by tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListMfaDeviceTagsRequest.Builder} avoiding the need
* to create one manually via {@link ListMfaDeviceTagsRequest#builder()}
*
*
* @param listMfaDeviceTagsRequest
* A {@link Consumer} that will call methods on {@link ListMFADeviceTagsRequest.Builder} to create a request.
* @return Result of the ListMFADeviceTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADeviceTags
* @see AWS API
* Documentation
*/
default ListMfaDeviceTagsResponse listMFADeviceTags(Consumer listMfaDeviceTagsRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listMFADeviceTags(ListMfaDeviceTagsRequest.builder().applyMutation(listMfaDeviceTagsRequest).build());
}
/**
*
* Lists the MFA devices for an IAM user. If the request includes a IAM user name, then this operation lists all the
* MFA devices associated with the specified user. If you do not specify a user name, IAM determines the user name
* implicitly based on the Amazon Web Services access key ID signing the request for this operation.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @return Result of the ListMFADevices operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADevices
* @see #listMFADevices(ListMfaDevicesRequest)
* @see AWS API
* Documentation
*/
default ListMfaDevicesResponse listMFADevices() throws NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listMFADevices(ListMfaDevicesRequest.builder().build());
}
/**
*
* Lists the MFA devices for an IAM user. If the request includes a IAM user name, then this operation lists all the
* MFA devices associated with the specified user. If you do not specify a user name, IAM determines the user name
* implicitly based on the Amazon Web Services access key ID signing the request for this operation.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listMfaDevicesRequest
* @return Result of the ListMFADevices operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADevices
* @see AWS API
* Documentation
*/
default ListMfaDevicesResponse listMFADevices(ListMfaDevicesRequest listMfaDevicesRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the MFA devices for an IAM user. If the request includes a IAM user name, then this operation lists all the
* MFA devices associated with the specified user. If you do not specify a user name, IAM determines the user name
* implicitly based on the Amazon Web Services access key ID signing the request for this operation.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListMfaDevicesRequest.Builder} avoiding the need to
* create one manually via {@link ListMfaDevicesRequest#builder()}
*
*
* @param listMfaDevicesRequest
* A {@link Consumer} that will call methods on {@link ListMFADevicesRequest.Builder} to create a request.
* @return Result of the ListMFADevices operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADevices
* @see AWS API
* Documentation
*/
default ListMfaDevicesResponse listMFADevices(Consumer listMfaDevicesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listMFADevices(ListMfaDevicesRequest.builder().applyMutation(listMfaDevicesRequest).build());
}
/**
*
* Lists the MFA devices for an IAM user. If the request includes a IAM user name, then this operation lists all the
* MFA devices associated with the specified user. If you do not specify a user name, IAM determines the user name
* implicitly based on the Amazon Web Services access key ID signing the request for this operation.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listMFADevices(software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListMfaDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMFADevices(software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADevices
* @see #listMFADevicesPaginator(ListMfaDevicesRequest)
* @see AWS API
* Documentation
*/
default ListMFADevicesIterable listMFADevicesPaginator() throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listMFADevicesPaginator(ListMfaDevicesRequest.builder().build());
}
/**
*
* Lists the MFA devices for an IAM user. If the request includes a IAM user name, then this operation lists all the
* MFA devices associated with the specified user. If you do not specify a user name, IAM determines the user name
* implicitly based on the Amazon Web Services access key ID signing the request for this operation.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listMFADevices(software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListMfaDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMFADevices(software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest)} operation.
*
*
* @param listMfaDevicesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADevices
* @see AWS API
* Documentation
*/
default ListMFADevicesIterable listMFADevicesPaginator(ListMfaDevicesRequest listMfaDevicesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the MFA devices for an IAM user. If the request includes a IAM user name, then this operation lists all the
* MFA devices associated with the specified user. If you do not specify a user name, IAM determines the user name
* implicitly based on the Amazon Web Services access key ID signing the request for this operation.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listMFADevices(software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListMfaDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListMFADevicesIterable responses = client.listMFADevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMFADevices(software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListMfaDevicesRequest.Builder} avoiding the need to
* create one manually via {@link ListMfaDevicesRequest#builder()}
*
*
* @param listMfaDevicesRequest
* A {@link Consumer} that will call methods on {@link ListMFADevicesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListMFADevices
* @see AWS API
* Documentation
*/
default ListMFADevicesIterable listMFADevicesPaginator(Consumer listMfaDevicesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listMFADevicesPaginator(ListMfaDevicesRequest.builder().applyMutation(listMfaDevicesRequest).build());
}
/**
*
* Lists the tags that are attached to the specified OpenID Connect (OIDC)-compatible identity provider. The
* returned list of tags is sorted by tag key. For more information, see About web identity
* federation.
*
*
* For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param listOpenIdConnectProviderTagsRequest
* @return Result of the ListOpenIDConnectProviderTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListOpenIDConnectProviderTags
* @see AWS API Documentation
*/
default ListOpenIdConnectProviderTagsResponse listOpenIDConnectProviderTags(
ListOpenIdConnectProviderTagsRequest listOpenIdConnectProviderTagsRequest) throws NoSuchEntityException,
ServiceFailureException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified OpenID Connect (OIDC)-compatible identity provider. The
* returned list of tags is sorted by tag key. For more information, see About web identity
* federation.
*
*
* For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListOpenIdConnectProviderTagsRequest.Builder}
* avoiding the need to create one manually via {@link ListOpenIdConnectProviderTagsRequest#builder()}
*
*
* @param listOpenIdConnectProviderTagsRequest
* A {@link Consumer} that will call methods on {@link ListOpenIDConnectProviderTagsRequest.Builder} to
* create a request.
* @return Result of the ListOpenIDConnectProviderTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListOpenIDConnectProviderTags
* @see AWS API Documentation
*/
default ListOpenIdConnectProviderTagsResponse listOpenIDConnectProviderTags(
Consumer listOpenIdConnectProviderTagsRequest)
throws NoSuchEntityException, ServiceFailureException, InvalidInputException, AwsServiceException,
SdkClientException, IamException {
return listOpenIDConnectProviderTags(ListOpenIdConnectProviderTagsRequest.builder()
.applyMutation(listOpenIdConnectProviderTagsRequest).build());
}
/**
*
* Lists information about the IAM OpenID Connect (OIDC) provider resource objects defined in the Amazon Web
* Services account.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an OIDC provider, see GetOpenIDConnectProvider.
*
*
*
* @return Result of the ListOpenIDConnectProviders operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListOpenIDConnectProviders
* @see #listOpenIDConnectProviders(ListOpenIdConnectProvidersRequest)
* @see AWS API Documentation
*/
default ListOpenIdConnectProvidersResponse listOpenIDConnectProviders() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listOpenIDConnectProviders(ListOpenIdConnectProvidersRequest.builder().build());
}
/**
*
* Lists information about the IAM OpenID Connect (OIDC) provider resource objects defined in the Amazon Web
* Services account.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an OIDC provider, see GetOpenIDConnectProvider.
*
*
*
* @param listOpenIdConnectProvidersRequest
* @return Result of the ListOpenIDConnectProviders operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListOpenIDConnectProviders
* @see AWS API Documentation
*/
default ListOpenIdConnectProvidersResponse listOpenIDConnectProviders(
ListOpenIdConnectProvidersRequest listOpenIdConnectProvidersRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists information about the IAM OpenID Connect (OIDC) provider resource objects defined in the Amazon Web
* Services account.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for an OIDC provider, see GetOpenIDConnectProvider.
*
*
*
* This is a convenience which creates an instance of the {@link ListOpenIdConnectProvidersRequest.Builder} avoiding
* the need to create one manually via {@link ListOpenIdConnectProvidersRequest#builder()}
*
*
* @param listOpenIdConnectProvidersRequest
* A {@link Consumer} that will call methods on {@link ListOpenIDConnectProvidersRequest.Builder} to create a
* request.
* @return Result of the ListOpenIDConnectProviders operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListOpenIDConnectProviders
* @see AWS API Documentation
*/
default ListOpenIdConnectProvidersResponse listOpenIDConnectProviders(
Consumer listOpenIdConnectProvidersRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listOpenIDConnectProviders(ListOpenIdConnectProvidersRequest.builder()
.applyMutation(listOpenIdConnectProvidersRequest).build());
}
/**
*
* Lists all the managed policies that are available in your Amazon Web Services account, including your own
* customer-defined managed policies and all Amazon Web Services managed policies.
*
*
* You can filter the list of policies that is returned using the optional OnlyAttached
,
* Scope
, and PathPrefix
parameters. For example, to list only the customer managed
* policies in your Amazon Web Services account, set Scope
to Local
. To list only Amazon
* Web Services managed policies, set Scope
to AWS
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a customer manged policy, see GetPolicy.
*
*
*
* @return Result of the ListPolicies operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicies
* @see #listPolicies(ListPoliciesRequest)
* @see AWS API
* Documentation
*/
default ListPoliciesResponse listPolicies() throws ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return listPolicies(ListPoliciesRequest.builder().build());
}
/**
*
* Lists all the managed policies that are available in your Amazon Web Services account, including your own
* customer-defined managed policies and all Amazon Web Services managed policies.
*
*
* You can filter the list of policies that is returned using the optional OnlyAttached
,
* Scope
, and PathPrefix
parameters. For example, to list only the customer managed
* policies in your Amazon Web Services account, set Scope
to Local
. To list only Amazon
* Web Services managed policies, set Scope
to AWS
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a customer manged policy, see GetPolicy.
*
*
*
* @param listPoliciesRequest
* @return Result of the ListPolicies operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesResponse listPolicies(ListPoliciesRequest listPoliciesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the managed policies that are available in your Amazon Web Services account, including your own
* customer-defined managed policies and all Amazon Web Services managed policies.
*
*
* You can filter the list of policies that is returned using the optional OnlyAttached
,
* Scope
, and PathPrefix
parameters. For example, to list only the customer managed
* policies in your Amazon Web Services account, set Scope
to Local
. To list only Amazon
* Web Services managed policies, set Scope
to AWS
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a customer manged policy, see GetPolicy.
*
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListPoliciesRequest.Builder} to create a request.
* @return Result of the ListPolicies operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesResponse listPolicies(Consumer listPoliciesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listPolicies(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* Lists all the managed policies that are available in your Amazon Web Services account, including your own
* customer-defined managed policies and all Amazon Web Services managed policies.
*
*
* You can filter the list of policies that is returned using the optional OnlyAttached
,
* Scope
, and PathPrefix
parameters. For example, to list only the customer managed
* policies in your Amazon Web Services account, set Scope
to Local
. To list only Amazon
* Web Services managed policies, set Scope
to AWS
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a customer manged policy, see GetPolicy.
*
*
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.iam.model.ListPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.iam.model.ListPoliciesRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicies
* @see #listPoliciesPaginator(ListPoliciesRequest)
* @see AWS API
* Documentation
*/
default ListPoliciesIterable listPoliciesPaginator() throws ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return listPoliciesPaginator(ListPoliciesRequest.builder().build());
}
/**
*
* Lists all the managed policies that are available in your Amazon Web Services account, including your own
* customer-defined managed policies and all Amazon Web Services managed policies.
*
*
* You can filter the list of policies that is returned using the optional OnlyAttached
,
* Scope
, and PathPrefix
parameters. For example, to list only the customer managed
* policies in your Amazon Web Services account, set Scope
to Local
. To list only Amazon
* Web Services managed policies, set Scope
to AWS
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a customer manged policy, see GetPolicy.
*
*
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.iam.model.ListPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.iam.model.ListPoliciesRequest)} operation.
*
*
* @param listPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesIterable listPoliciesPaginator(ListPoliciesRequest listPoliciesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the managed policies that are available in your Amazon Web Services account, including your own
* customer-defined managed policies and all Amazon Web Services managed policies.
*
*
* You can filter the list of policies that is returned using the optional OnlyAttached
,
* Scope
, and PathPrefix
parameters. For example, to list only the customer managed
* policies in your Amazon Web Services account, set Scope
to Local
. To list only Amazon
* Web Services managed policies, set Scope
to AWS
.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a customer manged policy, see GetPolicy.
*
*
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.iam.model.ListPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPoliciesIterable responses = client.listPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.iam.model.ListPoliciesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListPoliciesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesIterable listPoliciesPaginator(Consumer listPoliciesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listPoliciesPaginator(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* Retrieves a list of policies that the IAM identity (user, group, or role) can use to access each specified
* service.
*
*
*
* This operation does not use other policy types when determining whether a resource could access a service. These
* other policy types include resource-based policies, access control lists, Organizations policies, IAM permissions
* boundaries, and STS assume role policies. It only applies permissions policy logic. For more about the evaluation
* of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* The list of policies returned by the operation depends on the ARN of the identity that you provide.
*
*
* -
*
* User – The list of policies includes the managed and inline policies that are attached to the user
* directly. The list also includes any additional managed and inline policies that are attached to the group to
* which the user belongs.
*
*
* -
*
* Group – The list of policies includes only the managed and inline policies that are attached to the group
* directly. Policies that are attached to the group’s user are not included.
*
*
* -
*
* Role – The list of policies includes only the managed and inline policies that are attached to the role.
*
*
*
*
* For each managed policy, this operation returns the ARN and policy name. For each inline policy, it returns the
* policy name and the entity to which it is attached. Inline policies do not have an ARN. For more information
* about these policy types, see Managed policies
* and inline policies in the IAM User Guide.
*
*
* Policies that are attached to users and roles as permissions boundaries are not returned. To view which managed
* policy is currently used to set the permissions boundary for a user or role, use the GetUser or
* GetRole operations.
*
*
* @param listPoliciesGrantingServiceAccessRequest
* @return Result of the ListPoliciesGrantingServiceAccess operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPoliciesGrantingServiceAccess
* @see AWS API Documentation
*/
default ListPoliciesGrantingServiceAccessResponse listPoliciesGrantingServiceAccess(
ListPoliciesGrantingServiceAccessRequest listPoliciesGrantingServiceAccessRequest) throws NoSuchEntityException,
InvalidInputException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of policies that the IAM identity (user, group, or role) can use to access each specified
* service.
*
*
*
* This operation does not use other policy types when determining whether a resource could access a service. These
* other policy types include resource-based policies, access control lists, Organizations policies, IAM permissions
* boundaries, and STS assume role policies. It only applies permissions policy logic. For more about the evaluation
* of policy types, see Evaluating policies in the IAM User Guide.
*
*
*
* The list of policies returned by the operation depends on the ARN of the identity that you provide.
*
*
* -
*
* User – The list of policies includes the managed and inline policies that are attached to the user
* directly. The list also includes any additional managed and inline policies that are attached to the group to
* which the user belongs.
*
*
* -
*
* Group – The list of policies includes only the managed and inline policies that are attached to the group
* directly. Policies that are attached to the group’s user are not included.
*
*
* -
*
* Role – The list of policies includes only the managed and inline policies that are attached to the role.
*
*
*
*
* For each managed policy, this operation returns the ARN and policy name. For each inline policy, it returns the
* policy name and the entity to which it is attached. Inline policies do not have an ARN. For more information
* about these policy types, see Managed policies
* and inline policies in the IAM User Guide.
*
*
* Policies that are attached to users and roles as permissions boundaries are not returned. To view which managed
* policy is currently used to set the permissions boundary for a user or role, use the GetUser or
* GetRole operations.
*
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesGrantingServiceAccessRequest.Builder}
* avoiding the need to create one manually via {@link ListPoliciesGrantingServiceAccessRequest#builder()}
*
*
* @param listPoliciesGrantingServiceAccessRequest
* A {@link Consumer} that will call methods on {@link ListPoliciesGrantingServiceAccessRequest.Builder} to
* create a request.
* @return Result of the ListPoliciesGrantingServiceAccess operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPoliciesGrantingServiceAccess
* @see AWS API Documentation
*/
default ListPoliciesGrantingServiceAccessResponse listPoliciesGrantingServiceAccess(
Consumer listPoliciesGrantingServiceAccessRequest)
throws NoSuchEntityException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
return listPoliciesGrantingServiceAccess(ListPoliciesGrantingServiceAccessRequest.builder()
.applyMutation(listPoliciesGrantingServiceAccessRequest).build());
}
/**
*
* Lists the tags that are attached to the specified IAM customer managed policy. The returned list of tags is
* sorted by tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param listPolicyTagsRequest
* @return Result of the ListPolicyTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicyTags
* @see AWS API
* Documentation
*/
default ListPolicyTagsResponse listPolicyTags(ListPolicyTagsRequest listPolicyTagsRequest) throws NoSuchEntityException,
ServiceFailureException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified IAM customer managed policy. The returned list of tags is
* sorted by tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListPolicyTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListPolicyTagsRequest#builder()}
*
*
* @param listPolicyTagsRequest
* A {@link Consumer} that will call methods on {@link ListPolicyTagsRequest.Builder} to create a request.
* @return Result of the ListPolicyTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicyTags
* @see AWS API
* Documentation
*/
default ListPolicyTagsResponse listPolicyTags(Consumer listPolicyTagsRequest)
throws NoSuchEntityException, ServiceFailureException, InvalidInputException, AwsServiceException,
SdkClientException, IamException {
return listPolicyTags(ListPolicyTagsRequest.builder().applyMutation(listPolicyTagsRequest).build());
}
/**
*
* Lists information about the versions of the specified managed policy, including the version that is currently set
* as the policy's default version.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param listPolicyVersionsRequest
* @return Result of the ListPolicyVersions operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicyVersions
* @see AWS API
* Documentation
*/
default ListPolicyVersionsResponse listPolicyVersions(ListPolicyVersionsRequest listPolicyVersionsRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists information about the versions of the specified managed policy, including the version that is currently set
* as the policy's default version.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListPolicyVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPolicyVersionsRequest#builder()}
*
*
* @param listPolicyVersionsRequest
* A {@link Consumer} that will call methods on {@link ListPolicyVersionsRequest.Builder} to create a
* request.
* @return Result of the ListPolicyVersions operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicyVersions
* @see AWS API
* Documentation
*/
default ListPolicyVersionsResponse listPolicyVersions(Consumer listPolicyVersionsRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listPolicyVersions(ListPolicyVersionsRequest.builder().applyMutation(listPolicyVersionsRequest).build());
}
/**
*
* Lists information about the versions of the specified managed policy, including the version that is currently set
* as the policy's default version.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #listPolicyVersions(software.amazon.awssdk.services.iam.model.ListPolicyVersionsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPolicyVersionsIterable responses = client.listPolicyVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListPolicyVersionsIterable responses = client
* .listPolicyVersionsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListPolicyVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPolicyVersionsIterable responses = client.listPolicyVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicyVersions(software.amazon.awssdk.services.iam.model.ListPolicyVersionsRequest)} operation.
*
*
* @param listPolicyVersionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicyVersions
* @see AWS API
* Documentation
*/
default ListPolicyVersionsIterable listPolicyVersionsPaginator(ListPolicyVersionsRequest listPolicyVersionsRequest)
throws NoSuchEntityException, InvalidInputException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists information about the versions of the specified managed policy, including the version that is currently set
* as the policy's default version.
*
*
* For more information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #listPolicyVersions(software.amazon.awssdk.services.iam.model.ListPolicyVersionsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPolicyVersionsIterable responses = client.listPolicyVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListPolicyVersionsIterable responses = client
* .listPolicyVersionsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListPolicyVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListPolicyVersionsIterable responses = client.listPolicyVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicyVersions(software.amazon.awssdk.services.iam.model.ListPolicyVersionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListPolicyVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPolicyVersionsRequest#builder()}
*
*
* @param listPolicyVersionsRequest
* A {@link Consumer} that will call methods on {@link ListPolicyVersionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListPolicyVersions
* @see AWS API
* Documentation
*/
default ListPolicyVersionsIterable listPolicyVersionsPaginator(
Consumer listPolicyVersionsRequest) throws NoSuchEntityException,
InvalidInputException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listPolicyVersionsPaginator(ListPolicyVersionsRequest.builder().applyMutation(listPolicyVersionsRequest).build());
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM role.
*
*
* An IAM role can also have managed policies attached to it. To list the managed policies that are attached to a
* role, use ListAttachedRolePolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified role, the operation returns an empty list.
*
*
* @param listRolePoliciesRequest
* @return Result of the ListRolePolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRolePolicies
* @see AWS API
* Documentation
*/
default ListRolePoliciesResponse listRolePolicies(ListRolePoliciesRequest listRolePoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM role.
*
*
* An IAM role can also have managed policies attached to it. To list the managed policies that are attached to a
* role, use ListAttachedRolePolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified role, the operation returns an empty list.
*
*
*
* This is a convenience which creates an instance of the {@link ListRolePoliciesRequest.Builder} avoiding the need
* to create one manually via {@link ListRolePoliciesRequest#builder()}
*
*
* @param listRolePoliciesRequest
* A {@link Consumer} that will call methods on {@link ListRolePoliciesRequest.Builder} to create a request.
* @return Result of the ListRolePolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRolePolicies
* @see AWS API
* Documentation
*/
default ListRolePoliciesResponse listRolePolicies(Consumer listRolePoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listRolePolicies(ListRolePoliciesRequest.builder().applyMutation(listRolePoliciesRequest).build());
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM role.
*
*
* An IAM role can also have managed policies attached to it. To list the managed policies that are attached to a
* role, use ListAttachedRolePolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified role, the operation returns an empty list.
*
*
*
* This is a variant of {@link #listRolePolicies(software.amazon.awssdk.services.iam.model.ListRolePoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolePoliciesIterable responses = client.listRolePoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListRolePoliciesIterable responses = client.listRolePoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListRolePoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolePoliciesIterable responses = client.listRolePoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRolePolicies(software.amazon.awssdk.services.iam.model.ListRolePoliciesRequest)} operation.
*
*
* @param listRolePoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRolePolicies
* @see AWS API
* Documentation
*/
default ListRolePoliciesIterable listRolePoliciesPaginator(ListRolePoliciesRequest listRolePoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the names of the inline policies that are embedded in the specified IAM role.
*
*
* An IAM role can also have managed policies attached to it. To list the managed policies that are attached to a
* role, use ListAttachedRolePolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified role, the operation returns an empty list.
*
*
*
* This is a variant of {@link #listRolePolicies(software.amazon.awssdk.services.iam.model.ListRolePoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolePoliciesIterable responses = client.listRolePoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListRolePoliciesIterable responses = client.listRolePoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListRolePoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolePoliciesIterable responses = client.listRolePoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRolePolicies(software.amazon.awssdk.services.iam.model.ListRolePoliciesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListRolePoliciesRequest.Builder} avoiding the need
* to create one manually via {@link ListRolePoliciesRequest#builder()}
*
*
* @param listRolePoliciesRequest
* A {@link Consumer} that will call methods on {@link ListRolePoliciesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRolePolicies
* @see AWS API
* Documentation
*/
default ListRolePoliciesIterable listRolePoliciesPaginator(Consumer listRolePoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listRolePoliciesPaginator(ListRolePoliciesRequest.builder().applyMutation(listRolePoliciesRequest).build());
}
/**
*
* Lists the tags that are attached to the specified role. The returned list of tags is sorted by tag key. For more
* information about tagging, see Tagging
* IAM resources in the IAM User Guide.
*
*
* @param listRoleTagsRequest
* @return Result of the ListRoleTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoleTags
* @see AWS API
* Documentation
*/
default ListRoleTagsResponse listRoleTags(ListRoleTagsRequest listRoleTagsRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified role. The returned list of tags is sorted by tag key. For more
* information about tagging, see Tagging
* IAM resources in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListRoleTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListRoleTagsRequest#builder()}
*
*
* @param listRoleTagsRequest
* A {@link Consumer} that will call methods on {@link ListRoleTagsRequest.Builder} to create a request.
* @return Result of the ListRoleTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoleTags
* @see AWS API
* Documentation
*/
default ListRoleTagsResponse listRoleTags(Consumer listRoleTagsRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listRoleTags(ListRoleTagsRequest.builder().applyMutation(listRoleTagsRequest).build());
}
/**
*
* Lists the IAM roles that have the specified path prefix. If there are none, the operation returns an empty list.
* For more information about roles, see Working with roles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a role, see GetRole.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @return Result of the ListRoles operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoles
* @see #listRoles(ListRolesRequest)
* @see AWS API
* Documentation
*/
default ListRolesResponse listRoles() throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listRoles(ListRolesRequest.builder().build());
}
/**
*
* Lists the IAM roles that have the specified path prefix. If there are none, the operation returns an empty list.
* For more information about roles, see Working with roles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a role, see GetRole.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listRolesRequest
* @return Result of the ListRoles operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoles
* @see AWS API
* Documentation
*/
default ListRolesResponse listRoles(ListRolesRequest listRolesRequest) throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM roles that have the specified path prefix. If there are none, the operation returns an empty list.
* For more information about roles, see Working with roles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a role, see GetRole.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListRolesRequest.Builder} avoiding the need to
* create one manually via {@link ListRolesRequest#builder()}
*
*
* @param listRolesRequest
* A {@link Consumer} that will call methods on {@link ListRolesRequest.Builder} to create a request.
* @return Result of the ListRoles operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoles
* @see AWS API
* Documentation
*/
default ListRolesResponse listRoles(Consumer listRolesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listRoles(ListRolesRequest.builder().applyMutation(listRolesRequest).build());
}
/**
*
* Lists the IAM roles that have the specified path prefix. If there are none, the operation returns an empty list.
* For more information about roles, see Working with roles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a role, see GetRole.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listRoles(software.amazon.awssdk.services.iam.model.ListRolesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListRolesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRoles(software.amazon.awssdk.services.iam.model.ListRolesRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoles
* @see #listRolesPaginator(ListRolesRequest)
* @see AWS API
* Documentation
*/
default ListRolesIterable listRolesPaginator() throws ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return listRolesPaginator(ListRolesRequest.builder().build());
}
/**
*
* Lists the IAM roles that have the specified path prefix. If there are none, the operation returns an empty list.
* For more information about roles, see Working with roles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a role, see GetRole.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listRoles(software.amazon.awssdk.services.iam.model.ListRolesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListRolesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRoles(software.amazon.awssdk.services.iam.model.ListRolesRequest)} operation.
*
*
* @param listRolesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoles
* @see AWS API
* Documentation
*/
default ListRolesIterable listRolesPaginator(ListRolesRequest listRolesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM roles that have the specified path prefix. If there are none, the operation returns an empty list.
* For more information about roles, see Working with roles.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a role, see GetRole.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listRoles(software.amazon.awssdk.services.iam.model.ListRolesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListRolesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListRolesIterable responses = client.listRolesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRoles(software.amazon.awssdk.services.iam.model.ListRolesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListRolesRequest.Builder} avoiding the need to
* create one manually via {@link ListRolesRequest#builder()}
*
*
* @param listRolesRequest
* A {@link Consumer} that will call methods on {@link ListRolesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListRoles
* @see AWS API
* Documentation
*/
default ListRolesIterable listRolesPaginator(Consumer listRolesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listRolesPaginator(ListRolesRequest.builder().applyMutation(listRolesRequest).build());
}
/**
*
* Lists the tags that are attached to the specified Security Assertion Markup Language (SAML) identity provider.
* The returned list of tags is sorted by tag key. For more information, see About SAML 2.0-based
* federation.
*
*
* For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param listSamlProviderTagsRequest
* @return Result of the ListSAMLProviderTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSAMLProviderTags
* @see AWS API
* Documentation
*/
default ListSamlProviderTagsResponse listSAMLProviderTags(ListSamlProviderTagsRequest listSamlProviderTagsRequest)
throws NoSuchEntityException, ServiceFailureException, InvalidInputException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified Security Assertion Markup Language (SAML) identity provider.
* The returned list of tags is sorted by tag key. For more information, see About SAML 2.0-based
* federation.
*
*
* For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListSamlProviderTagsRequest.Builder} avoiding the
* need to create one manually via {@link ListSamlProviderTagsRequest#builder()}
*
*
* @param listSamlProviderTagsRequest
* A {@link Consumer} that will call methods on {@link ListSAMLProviderTagsRequest.Builder} to create a
* request.
* @return Result of the ListSAMLProviderTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSAMLProviderTags
* @see AWS API
* Documentation
*/
default ListSamlProviderTagsResponse listSAMLProviderTags(
Consumer listSamlProviderTagsRequest) throws NoSuchEntityException,
ServiceFailureException, InvalidInputException, AwsServiceException, SdkClientException, IamException {
return listSAMLProviderTags(ListSamlProviderTagsRequest.builder().applyMutation(listSamlProviderTagsRequest).build());
}
/**
*
* Lists the SAML provider resource objects defined in IAM in the account. IAM resource-listing operations return a
* subset of the available attributes for the resource. For example, this operation does not return tags, even
* though they are an attribute of the returned object. To view all of the information for a SAML provider, see
* GetSAMLProvider.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @return Result of the ListSAMLProviders operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSAMLProviders
* @see #listSAMLProviders(ListSamlProvidersRequest)
* @see AWS API
* Documentation
*/
default ListSamlProvidersResponse listSAMLProviders() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listSAMLProviders(ListSamlProvidersRequest.builder().build());
}
/**
*
* Lists the SAML provider resource objects defined in IAM in the account. IAM resource-listing operations return a
* subset of the available attributes for the resource. For example, this operation does not return tags, even
* though they are an attribute of the returned object. To view all of the information for a SAML provider, see
* GetSAMLProvider.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param listSamlProvidersRequest
* @return Result of the ListSAMLProviders operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSAMLProviders
* @see AWS API
* Documentation
*/
default ListSamlProvidersResponse listSAMLProviders(ListSamlProvidersRequest listSamlProvidersRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the SAML provider resource objects defined in IAM in the account. IAM resource-listing operations return a
* subset of the available attributes for the resource. For example, this operation does not return tags, even
* though they are an attribute of the returned object. To view all of the information for a SAML provider, see
* GetSAMLProvider.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* This is a convenience which creates an instance of the {@link ListSamlProvidersRequest.Builder} avoiding the need
* to create one manually via {@link ListSamlProvidersRequest#builder()}
*
*
* @param listSamlProvidersRequest
* A {@link Consumer} that will call methods on {@link ListSAMLProvidersRequest.Builder} to create a request.
* @return Result of the ListSAMLProviders operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSAMLProviders
* @see AWS API
* Documentation
*/
default ListSamlProvidersResponse listSAMLProviders(Consumer listSamlProvidersRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listSAMLProviders(ListSamlProvidersRequest.builder().applyMutation(listSamlProvidersRequest).build());
}
/**
*
* Returns information about the SSH public keys associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* The SSH public keys returned by this operation are used only for authenticating the IAM user to an CodeCommit
* repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see Set up CodeCommit
* for SSH connections in the CodeCommit User Guide.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* @return Result of the ListSSHPublicKeys operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSSHPublicKeys
* @see #listSSHPublicKeys(ListSshPublicKeysRequest)
* @see AWS API
* Documentation
*/
default ListSshPublicKeysResponse listSSHPublicKeys() throws NoSuchEntityException, AwsServiceException, SdkClientException,
IamException {
return listSSHPublicKeys(ListSshPublicKeysRequest.builder().build());
}
/**
*
* Returns information about the SSH public keys associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* The SSH public keys returned by this operation are used only for authenticating the IAM user to an CodeCommit
* repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see Set up CodeCommit
* for SSH connections in the CodeCommit User Guide.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* @param listSshPublicKeysRequest
* @return Result of the ListSSHPublicKeys operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSSHPublicKeys
* @see AWS API
* Documentation
*/
default ListSshPublicKeysResponse listSSHPublicKeys(ListSshPublicKeysRequest listSshPublicKeysRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the SSH public keys associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* The SSH public keys returned by this operation are used only for authenticating the IAM user to an CodeCommit
* repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see Set up CodeCommit
* for SSH connections in the CodeCommit User Guide.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListSshPublicKeysRequest.Builder} avoiding the need
* to create one manually via {@link ListSshPublicKeysRequest#builder()}
*
*
* @param listSshPublicKeysRequest
* A {@link Consumer} that will call methods on {@link ListSSHPublicKeysRequest.Builder} to create a request.
* @return Result of the ListSSHPublicKeys operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSSHPublicKeys
* @see AWS API
* Documentation
*/
default ListSshPublicKeysResponse listSSHPublicKeys(Consumer listSshPublicKeysRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
return listSSHPublicKeys(ListSshPublicKeysRequest.builder().applyMutation(listSshPublicKeysRequest).build());
}
/**
*
* Returns information about the SSH public keys associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* The SSH public keys returned by this operation are used only for authenticating the IAM user to an CodeCommit
* repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see Set up CodeCommit
* for SSH connections in the CodeCommit User Guide.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listSSHPublicKeys(software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client.listSSHPublicKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client
* .listSSHPublicKeysPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListSshPublicKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client.listSSHPublicKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSSHPublicKeys(software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSSHPublicKeys
* @see #listSSHPublicKeysPaginator(ListSshPublicKeysRequest)
* @see AWS API
* Documentation
*/
default ListSSHPublicKeysIterable listSSHPublicKeysPaginator() throws NoSuchEntityException, AwsServiceException,
SdkClientException, IamException {
return listSSHPublicKeysPaginator(ListSshPublicKeysRequest.builder().build());
}
/**
*
* Returns information about the SSH public keys associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* The SSH public keys returned by this operation are used only for authenticating the IAM user to an CodeCommit
* repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see Set up CodeCommit
* for SSH connections in the CodeCommit User Guide.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listSSHPublicKeys(software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client.listSSHPublicKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client
* .listSSHPublicKeysPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListSshPublicKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client.listSSHPublicKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSSHPublicKeys(software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest)} operation.
*
*
* @param listSshPublicKeysRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSSHPublicKeys
* @see AWS API
* Documentation
*/
default ListSSHPublicKeysIterable listSSHPublicKeysPaginator(ListSshPublicKeysRequest listSshPublicKeysRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the SSH public keys associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* The SSH public keys returned by this operation are used only for authenticating the IAM user to an CodeCommit
* repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see Set up CodeCommit
* for SSH connections in the CodeCommit User Guide.
*
*
* Although each user is limited to a small number of keys, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listSSHPublicKeys(software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client.listSSHPublicKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client
* .listSSHPublicKeysPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListSshPublicKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSSHPublicKeysIterable responses = client.listSSHPublicKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSSHPublicKeys(software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSshPublicKeysRequest.Builder} avoiding the need
* to create one manually via {@link ListSshPublicKeysRequest#builder()}
*
*
* @param listSshPublicKeysRequest
* A {@link Consumer} that will call methods on {@link ListSSHPublicKeysRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSSHPublicKeys
* @see AWS API
* Documentation
*/
default ListSSHPublicKeysIterable listSSHPublicKeysPaginator(
Consumer listSshPublicKeysRequest) throws NoSuchEntityException,
AwsServiceException, SdkClientException, IamException {
return listSSHPublicKeysPaginator(ListSshPublicKeysRequest.builder().applyMutation(listSshPublicKeysRequest).build());
}
/**
*
* Lists the tags that are attached to the specified IAM server certificate. The returned list of tags is sorted by
* tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* For certificates in a Region supported by Certificate Manager (ACM), we recommend that you don't use IAM server
* certificates. Instead, use ACM to provision, manage, and deploy your server certificates. For more information
* about IAM server certificates, Working with server
* certificates in the IAM User Guide.
*
*
*
* @param listServerCertificateTagsRequest
* @return Result of the ListServerCertificateTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificateTags
* @see AWS
* API Documentation
*/
default ListServerCertificateTagsResponse listServerCertificateTags(
ListServerCertificateTagsRequest listServerCertificateTagsRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified IAM server certificate. The returned list of tags is sorted by
* tag key. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* For certificates in a Region supported by Certificate Manager (ACM), we recommend that you don't use IAM server
* certificates. Instead, use ACM to provision, manage, and deploy your server certificates. For more information
* about IAM server certificates, Working with server
* certificates in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListServerCertificateTagsRequest.Builder} avoiding
* the need to create one manually via {@link ListServerCertificateTagsRequest#builder()}
*
*
* @param listServerCertificateTagsRequest
* A {@link Consumer} that will call methods on {@link ListServerCertificateTagsRequest.Builder} to create a
* request.
* @return Result of the ListServerCertificateTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificateTags
* @see AWS
* API Documentation
*/
default ListServerCertificateTagsResponse listServerCertificateTags(
Consumer listServerCertificateTagsRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listServerCertificateTags(ListServerCertificateTagsRequest.builder()
.applyMutation(listServerCertificateTagsRequest).build());
}
/**
*
* Lists the server certificates stored in IAM that have the specified path prefix. If none exist, the operation
* returns an empty list.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a servercertificate, see GetServerCertificate.
*
*
*
* @return Result of the ListServerCertificates operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificates
* @see #listServerCertificates(ListServerCertificatesRequest)
* @see AWS
* API Documentation
*/
default ListServerCertificatesResponse listServerCertificates() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listServerCertificates(ListServerCertificatesRequest.builder().build());
}
/**
*
* Lists the server certificates stored in IAM that have the specified path prefix. If none exist, the operation
* returns an empty list.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a servercertificate, see GetServerCertificate.
*
*
*
* @param listServerCertificatesRequest
* @return Result of the ListServerCertificates operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificates
* @see AWS
* API Documentation
*/
default ListServerCertificatesResponse listServerCertificates(ListServerCertificatesRequest listServerCertificatesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the server certificates stored in IAM that have the specified path prefix. If none exist, the operation
* returns an empty list.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a servercertificate, see GetServerCertificate.
*
*
*
* This is a convenience which creates an instance of the {@link ListServerCertificatesRequest.Builder} avoiding the
* need to create one manually via {@link ListServerCertificatesRequest#builder()}
*
*
* @param listServerCertificatesRequest
* A {@link Consumer} that will call methods on {@link ListServerCertificatesRequest.Builder} to create a
* request.
* @return Result of the ListServerCertificates operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificates
* @see AWS
* API Documentation
*/
default ListServerCertificatesResponse listServerCertificates(
Consumer listServerCertificatesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listServerCertificates(ListServerCertificatesRequest.builder().applyMutation(listServerCertificatesRequest)
.build());
}
/**
*
* Lists the server certificates stored in IAM that have the specified path prefix. If none exist, the operation
* returns an empty list.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a servercertificate, see GetServerCertificate.
*
*
*
* This is a variant of
* {@link #listServerCertificates(software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client.listServerCertificatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client
* .listServerCertificatesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListServerCertificatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client.listServerCertificatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServerCertificates(software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificates
* @see #listServerCertificatesPaginator(ListServerCertificatesRequest)
* @see AWS
* API Documentation
*/
default ListServerCertificatesIterable listServerCertificatesPaginator() throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return listServerCertificatesPaginator(ListServerCertificatesRequest.builder().build());
}
/**
*
* Lists the server certificates stored in IAM that have the specified path prefix. If none exist, the operation
* returns an empty list.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a servercertificate, see GetServerCertificate.
*
*
*
* This is a variant of
* {@link #listServerCertificates(software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client.listServerCertificatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client
* .listServerCertificatesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListServerCertificatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client.listServerCertificatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServerCertificates(software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest)}
* operation.
*
*
* @param listServerCertificatesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificates
* @see AWS
* API Documentation
*/
default ListServerCertificatesIterable listServerCertificatesPaginator(
ListServerCertificatesRequest listServerCertificatesRequest) throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the server certificates stored in IAM that have the specified path prefix. If none exist, the operation
* returns an empty list.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a servercertificate, see GetServerCertificate.
*
*
*
* This is a variant of
* {@link #listServerCertificates(software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client.listServerCertificatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client
* .listServerCertificatesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListServerCertificatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListServerCertificatesIterable responses = client.listServerCertificatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServerCertificates(software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListServerCertificatesRequest.Builder} avoiding the
* need to create one manually via {@link ListServerCertificatesRequest#builder()}
*
*
* @param listServerCertificatesRequest
* A {@link Consumer} that will call methods on {@link ListServerCertificatesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServerCertificates
* @see AWS
* API Documentation
*/
default ListServerCertificatesIterable listServerCertificatesPaginator(
Consumer listServerCertificatesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listServerCertificatesPaginator(ListServerCertificatesRequest.builder()
.applyMutation(listServerCertificatesRequest).build());
}
/**
*
* Returns information about the service-specific credentials associated with the specified IAM user. If none
* exists, the operation returns an empty list. The service-specific credentials returned by this operation are used
* only for authenticating the IAM user to a specific service. For more information about using service-specific
* credentials to authenticate to an Amazon Web Services service, see Set up service-specific
* credentials in the CodeCommit User Guide.
*
*
* @return Result of the ListServiceSpecificCredentials operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceNotSupportedException
* The specified service does not support service-specific credentials.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServiceSpecificCredentials
* @see #listServiceSpecificCredentials(ListServiceSpecificCredentialsRequest)
* @see AWS API Documentation
*/
default ListServiceSpecificCredentialsResponse listServiceSpecificCredentials() throws NoSuchEntityException,
ServiceNotSupportedException, AwsServiceException, SdkClientException, IamException {
return listServiceSpecificCredentials(ListServiceSpecificCredentialsRequest.builder().build());
}
/**
*
* Returns information about the service-specific credentials associated with the specified IAM user. If none
* exists, the operation returns an empty list. The service-specific credentials returned by this operation are used
* only for authenticating the IAM user to a specific service. For more information about using service-specific
* credentials to authenticate to an Amazon Web Services service, see Set up service-specific
* credentials in the CodeCommit User Guide.
*
*
* @param listServiceSpecificCredentialsRequest
* @return Result of the ListServiceSpecificCredentials operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceNotSupportedException
* The specified service does not support service-specific credentials.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServiceSpecificCredentials
* @see AWS API Documentation
*/
default ListServiceSpecificCredentialsResponse listServiceSpecificCredentials(
ListServiceSpecificCredentialsRequest listServiceSpecificCredentialsRequest) throws NoSuchEntityException,
ServiceNotSupportedException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the service-specific credentials associated with the specified IAM user. If none
* exists, the operation returns an empty list. The service-specific credentials returned by this operation are used
* only for authenticating the IAM user to a specific service. For more information about using service-specific
* credentials to authenticate to an Amazon Web Services service, see Set up service-specific
* credentials in the CodeCommit User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListServiceSpecificCredentialsRequest.Builder}
* avoiding the need to create one manually via {@link ListServiceSpecificCredentialsRequest#builder()}
*
*
* @param listServiceSpecificCredentialsRequest
* A {@link Consumer} that will call methods on {@link ListServiceSpecificCredentialsRequest.Builder} to
* create a request.
* @return Result of the ListServiceSpecificCredentials operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceNotSupportedException
* The specified service does not support service-specific credentials.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListServiceSpecificCredentials
* @see AWS API Documentation
*/
default ListServiceSpecificCredentialsResponse listServiceSpecificCredentials(
Consumer listServiceSpecificCredentialsRequest)
throws NoSuchEntityException, ServiceNotSupportedException, AwsServiceException, SdkClientException, IamException {
return listServiceSpecificCredentials(ListServiceSpecificCredentialsRequest.builder()
.applyMutation(listServiceSpecificCredentialsRequest).build());
}
/**
*
* Returns information about the signing certificates associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of signing certificates, you can still paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request for this operation. This operation works for access keys
* under the Amazon Web Services account. Consequently, you can use this operation to manage Amazon Web Services
* account root user credentials even if the Amazon Web Services account has no associated users.
*
*
* @return Result of the ListSigningCertificates operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSigningCertificates
* @see #listSigningCertificates(ListSigningCertificatesRequest)
* @see AWS
* API Documentation
*/
default ListSigningCertificatesResponse listSigningCertificates() throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listSigningCertificates(ListSigningCertificatesRequest.builder().build());
}
/**
*
* Returns information about the signing certificates associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of signing certificates, you can still paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request for this operation. This operation works for access keys
* under the Amazon Web Services account. Consequently, you can use this operation to manage Amazon Web Services
* account root user credentials even if the Amazon Web Services account has no associated users.
*
*
* @param listSigningCertificatesRequest
* @return Result of the ListSigningCertificates operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSigningCertificates
* @see AWS
* API Documentation
*/
default ListSigningCertificatesResponse listSigningCertificates(ListSigningCertificatesRequest listSigningCertificatesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the signing certificates associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of signing certificates, you can still paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request for this operation. This operation works for access keys
* under the Amazon Web Services account. Consequently, you can use this operation to manage Amazon Web Services
* account root user credentials even if the Amazon Web Services account has no associated users.
*
*
*
* This is a convenience which creates an instance of the {@link ListSigningCertificatesRequest.Builder} avoiding
* the need to create one manually via {@link ListSigningCertificatesRequest#builder()}
*
*
* @param listSigningCertificatesRequest
* A {@link Consumer} that will call methods on {@link ListSigningCertificatesRequest.Builder} to create a
* request.
* @return Result of the ListSigningCertificates operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSigningCertificates
* @see AWS
* API Documentation
*/
default ListSigningCertificatesResponse listSigningCertificates(
Consumer listSigningCertificatesRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listSigningCertificates(ListSigningCertificatesRequest.builder().applyMutation(listSigningCertificatesRequest)
.build());
}
/**
*
* Returns information about the signing certificates associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of signing certificates, you can still paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request for this operation. This operation works for access keys
* under the Amazon Web Services account. Consequently, you can use this operation to manage Amazon Web Services
* account root user credentials even if the Amazon Web Services account has no associated users.
*
*
*
* This is a variant of
* {@link #listSigningCertificates(software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client.listSigningCertificatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client
* .listSigningCertificatesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListSigningCertificatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client.listSigningCertificatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSigningCertificates(software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSigningCertificates
* @see #listSigningCertificatesPaginator(ListSigningCertificatesRequest)
* @see AWS
* API Documentation
*/
default ListSigningCertificatesIterable listSigningCertificatesPaginator() throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listSigningCertificatesPaginator(ListSigningCertificatesRequest.builder().build());
}
/**
*
* Returns information about the signing certificates associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of signing certificates, you can still paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request for this operation. This operation works for access keys
* under the Amazon Web Services account. Consequently, you can use this operation to manage Amazon Web Services
* account root user credentials even if the Amazon Web Services account has no associated users.
*
*
*
* This is a variant of
* {@link #listSigningCertificates(software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client.listSigningCertificatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client
* .listSigningCertificatesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListSigningCertificatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client.listSigningCertificatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSigningCertificates(software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest)}
* operation.
*
*
* @param listSigningCertificatesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSigningCertificates
* @see AWS
* API Documentation
*/
default ListSigningCertificatesIterable listSigningCertificatesPaginator(
ListSigningCertificatesRequest listSigningCertificatesRequest) throws NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the signing certificates associated with the specified IAM user. If none exists, the
* operation returns an empty list.
*
*
* Although each user is limited to a small number of signing certificates, you can still paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request for this operation. This operation works for access keys
* under the Amazon Web Services account. Consequently, you can use this operation to manage Amazon Web Services
* account root user credentials even if the Amazon Web Services account has no associated users.
*
*
*
* This is a variant of
* {@link #listSigningCertificates(software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client.listSigningCertificatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client
* .listSigningCertificatesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListSigningCertificatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListSigningCertificatesIterable responses = client.listSigningCertificatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSigningCertificates(software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListSigningCertificatesRequest.Builder} avoiding
* the need to create one manually via {@link ListSigningCertificatesRequest#builder()}
*
*
* @param listSigningCertificatesRequest
* A {@link Consumer} that will call methods on {@link ListSigningCertificatesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListSigningCertificates
* @see AWS
* API Documentation
*/
default ListSigningCertificatesIterable listSigningCertificatesPaginator(
Consumer listSigningCertificatesRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listSigningCertificatesPaginator(ListSigningCertificatesRequest.builder()
.applyMutation(listSigningCertificatesRequest).build());
}
/**
*
* Lists the names of the inline policies embedded in the specified IAM user.
*
*
* An IAM user can also have managed policies attached to it. To list the managed policies that are attached to a
* user, use ListAttachedUserPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified user, the operation returns an empty list.
*
*
* @param listUserPoliciesRequest
* @return Result of the ListUserPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserPolicies
* @see AWS API
* Documentation
*/
default ListUserPoliciesResponse listUserPolicies(ListUserPoliciesRequest listUserPoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the names of the inline policies embedded in the specified IAM user.
*
*
* An IAM user can also have managed policies attached to it. To list the managed policies that are attached to a
* user, use ListAttachedUserPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified user, the operation returns an empty list.
*
*
*
* This is a convenience which creates an instance of the {@link ListUserPoliciesRequest.Builder} avoiding the need
* to create one manually via {@link ListUserPoliciesRequest#builder()}
*
*
* @param listUserPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListUserPoliciesRequest.Builder} to create a request.
* @return Result of the ListUserPolicies operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserPolicies
* @see AWS API
* Documentation
*/
default ListUserPoliciesResponse listUserPolicies(Consumer listUserPoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listUserPolicies(ListUserPoliciesRequest.builder().applyMutation(listUserPoliciesRequest).build());
}
/**
*
* Lists the names of the inline policies embedded in the specified IAM user.
*
*
* An IAM user can also have managed policies attached to it. To list the managed policies that are attached to a
* user, use ListAttachedUserPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified user, the operation returns an empty list.
*
*
*
* This is a variant of {@link #listUserPolicies(software.amazon.awssdk.services.iam.model.ListUserPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserPoliciesIterable responses = client.listUserPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListUserPoliciesIterable responses = client.listUserPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListUserPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserPoliciesIterable responses = client.listUserPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserPolicies(software.amazon.awssdk.services.iam.model.ListUserPoliciesRequest)} operation.
*
*
* @param listUserPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserPolicies
* @see AWS API
* Documentation
*/
default ListUserPoliciesIterable listUserPoliciesPaginator(ListUserPoliciesRequest listUserPoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the names of the inline policies embedded in the specified IAM user.
*
*
* An IAM user can also have managed policies attached to it. To list the managed policies that are attached to a
* user, use ListAttachedUserPolicies. For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and Marker
parameters. If there are no
* inline policies embedded with the specified user, the operation returns an empty list.
*
*
*
* This is a variant of {@link #listUserPolicies(software.amazon.awssdk.services.iam.model.ListUserPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserPoliciesIterable responses = client.listUserPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListUserPoliciesIterable responses = client.listUserPoliciesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListUserPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserPoliciesIterable responses = client.listUserPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserPolicies(software.amazon.awssdk.services.iam.model.ListUserPoliciesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListUserPoliciesRequest.Builder} avoiding the need
* to create one manually via {@link ListUserPoliciesRequest#builder()}
*
*
* @param listUserPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListUserPoliciesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserPolicies
* @see AWS API
* Documentation
*/
default ListUserPoliciesIterable listUserPoliciesPaginator(Consumer listUserPoliciesRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listUserPoliciesPaginator(ListUserPoliciesRequest.builder().applyMutation(listUserPoliciesRequest).build());
}
/**
*
* Lists the tags that are attached to the specified IAM user. The returned list of tags is sorted by tag key. For
* more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param listUserTagsRequest
* @return Result of the ListUserTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserTags
* @see AWS API
* Documentation
*/
default ListUserTagsResponse listUserTags(ListUserTagsRequest listUserTagsRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified IAM user. The returned list of tags is sorted by tag key. For
* more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListUserTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListUserTagsRequest#builder()}
*
*
* @param listUserTagsRequest
* A {@link Consumer} that will call methods on {@link ListUserTagsRequest.Builder} to create a request.
* @return Result of the ListUserTags operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserTags
* @see AWS API
* Documentation
*/
default ListUserTagsResponse listUserTags(Consumer listUserTagsRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listUserTags(ListUserTagsRequest.builder().applyMutation(listUserTagsRequest).build());
}
/**
*
* Lists the tags that are attached to the specified IAM user. The returned list of tags is sorted by tag key. For
* more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a variant of {@link #listUserTags(software.amazon.awssdk.services.iam.model.ListUserTagsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserTagsIterable responses = client.listUserTagsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListUserTagsIterable responses = client.listUserTagsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListUserTagsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserTagsIterable responses = client.listUserTagsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserTags(software.amazon.awssdk.services.iam.model.ListUserTagsRequest)} operation.
*
*
* @param listUserTagsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserTags
* @see AWS API
* Documentation
*/
default ListUserTagsIterable listUserTagsPaginator(ListUserTagsRequest listUserTagsRequest) throws NoSuchEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags that are attached to the specified IAM user. The returned list of tags is sorted by tag key. For
* more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a variant of {@link #listUserTags(software.amazon.awssdk.services.iam.model.ListUserTagsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserTagsIterable responses = client.listUserTagsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListUserTagsIterable responses = client.listUserTagsPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListUserTagsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUserTagsIterable responses = client.listUserTagsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserTags(software.amazon.awssdk.services.iam.model.ListUserTagsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListUserTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListUserTagsRequest#builder()}
*
*
* @param listUserTagsRequest
* A {@link Consumer} that will call methods on {@link ListUserTagsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUserTags
* @see AWS API
* Documentation
*/
default ListUserTagsIterable listUserTagsPaginator(Consumer listUserTagsRequest)
throws NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listUserTagsPaginator(ListUserTagsRequest.builder().applyMutation(listUserTagsRequest).build());
}
/**
*
* Lists the IAM users that have the specified path prefix. If no path prefix is specified, the operation returns
* all users in the Amazon Web Services account. If there are none, the operation returns an empty list.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a user, see GetUser.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @return Result of the ListUsers operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUsers
* @see #listUsers(ListUsersRequest)
* @see AWS API
* Documentation
*/
default ListUsersResponse listUsers() throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listUsers(ListUsersRequest.builder().build());
}
/**
*
* Lists the IAM users that have the specified path prefix. If no path prefix is specified, the operation returns
* all users in the Amazon Web Services account. If there are none, the operation returns an empty list.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a user, see GetUser.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listUsersRequest
* @return Result of the ListUsers operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersResponse listUsers(ListUsersRequest listUsersRequest) throws ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM users that have the specified path prefix. If no path prefix is specified, the operation returns
* all users in the Amazon Web Services account. If there are none, the operation returns an empty list.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a user, see GetUser.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on {@link ListUsersRequest.Builder} to create a request.
* @return Result of the ListUsers operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersResponse listUsers(Consumer listUsersRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return listUsers(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Lists the IAM users that have the specified path prefix. If no path prefix is specified, the operation returns
* all users in the Amazon Web Services account. If there are none, the operation returns an empty list.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a user, see GetUser.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.iam.model.ListUsersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.iam.model.ListUsersRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUsers
* @see #listUsersPaginator(ListUsersRequest)
* @see AWS API
* Documentation
*/
default ListUsersIterable listUsersPaginator() throws ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return listUsersPaginator(ListUsersRequest.builder().build());
}
/**
*
* Lists the IAM users that have the specified path prefix. If no path prefix is specified, the operation returns
* all users in the Amazon Web Services account. If there are none, the operation returns an empty list.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a user, see GetUser.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.iam.model.ListUsersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.iam.model.ListUsersRequest)} operation.
*
*
* @param listUsersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersIterable listUsersPaginator(ListUsersRequest listUsersRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the IAM users that have the specified path prefix. If no path prefix is specified, the operation returns
* all users in the Amazon Web Services account. If there are none, the operation returns an empty list.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view all of the
* information for a user, see GetUser.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.iam.model.ListUsersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.iam.model.ListUsersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on {@link ListUsersRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersIterable listUsersPaginator(Consumer listUsersRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return listUsersPaginator(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Lists the virtual MFA devices defined in the Amazon Web Services account by assignment status. If you do not
* specify an assignment status, the operation returns a list of all virtual MFA devices. Assignment status can be
* Assigned
, Unassigned
, or Any
.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view tag information
* for a virtual MFA device, see ListMFADeviceTags.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @return Result of the ListVirtualMFADevices operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListVirtualMFADevices
* @see #listVirtualMFADevices(ListVirtualMfaDevicesRequest)
* @see AWS API
* Documentation
*/
default ListVirtualMfaDevicesResponse listVirtualMFADevices() throws AwsServiceException, SdkClientException, IamException {
return listVirtualMFADevices(ListVirtualMfaDevicesRequest.builder().build());
}
/**
*
* Lists the virtual MFA devices defined in the Amazon Web Services account by assignment status. If you do not
* specify an assignment status, the operation returns a list of all virtual MFA devices. Assignment status can be
* Assigned
, Unassigned
, or Any
.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view tag information
* for a virtual MFA device, see ListMFADeviceTags.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
* @param listVirtualMfaDevicesRequest
* @return Result of the ListVirtualMFADevices operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListVirtualMFADevices
* @see AWS API
* Documentation
*/
default ListVirtualMfaDevicesResponse listVirtualMFADevices(ListVirtualMfaDevicesRequest listVirtualMfaDevicesRequest)
throws AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the virtual MFA devices defined in the Amazon Web Services account by assignment status. If you do not
* specify an assignment status, the operation returns a list of all virtual MFA devices. Assignment status can be
* Assigned
, Unassigned
, or Any
.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view tag information
* for a virtual MFA device, see ListMFADeviceTags.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListVirtualMfaDevicesRequest.Builder} avoiding the
* need to create one manually via {@link ListVirtualMfaDevicesRequest#builder()}
*
*
* @param listVirtualMfaDevicesRequest
* A {@link Consumer} that will call methods on {@link ListVirtualMFADevicesRequest.Builder} to create a
* request.
* @return Result of the ListVirtualMFADevices operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListVirtualMFADevices
* @see AWS API
* Documentation
*/
default ListVirtualMfaDevicesResponse listVirtualMFADevices(
Consumer listVirtualMfaDevicesRequest) throws AwsServiceException,
SdkClientException, IamException {
return listVirtualMFADevices(ListVirtualMfaDevicesRequest.builder().applyMutation(listVirtualMfaDevicesRequest).build());
}
/**
*
* Lists the virtual MFA devices defined in the Amazon Web Services account by assignment status. If you do not
* specify an assignment status, the operation returns a list of all virtual MFA devices. Assignment status can be
* Assigned
, Unassigned
, or Any
.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view tag information
* for a virtual MFA device, see ListMFADeviceTags.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listVirtualMFADevices(software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client.listVirtualMFADevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client
* .listVirtualMFADevicesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client.listVirtualMFADevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVirtualMFADevices(software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListVirtualMFADevices
* @see #listVirtualMFADevicesPaginator(ListVirtualMfaDevicesRequest)
* @see AWS API
* Documentation
*/
default ListVirtualMFADevicesIterable listVirtualMFADevicesPaginator() throws AwsServiceException, SdkClientException,
IamException {
return listVirtualMFADevicesPaginator(ListVirtualMfaDevicesRequest.builder().build());
}
/**
*
* Lists the virtual MFA devices defined in the Amazon Web Services account by assignment status. If you do not
* specify an assignment status, the operation returns a list of all virtual MFA devices. Assignment status can be
* Assigned
, Unassigned
, or Any
.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view tag information
* for a virtual MFA device, see ListMFADeviceTags.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listVirtualMFADevices(software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client.listVirtualMFADevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client
* .listVirtualMFADevicesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client.listVirtualMFADevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVirtualMFADevices(software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest)}
* operation.
*
*
* @param listVirtualMfaDevicesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListVirtualMFADevices
* @see AWS API
* Documentation
*/
default ListVirtualMFADevicesIterable listVirtualMFADevicesPaginator(ListVirtualMfaDevicesRequest listVirtualMfaDevicesRequest)
throws AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the virtual MFA devices defined in the Amazon Web Services account by assignment status. If you do not
* specify an assignment status, the operation returns a list of all virtual MFA devices. Assignment status can be
* Assigned
, Unassigned
, or Any
.
*
*
*
* IAM resource-listing operations return a subset of the available attributes for the resource. For example, this
* operation does not return tags, even though they are an attribute of the returned object. To view tag information
* for a virtual MFA device, see ListMFADeviceTags.
*
*
*
* You can paginate the results using the MaxItems
and Marker
parameters.
*
*
*
* This is a variant of
* {@link #listVirtualMFADevices(software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client.listVirtualMFADevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client
* .listVirtualMFADevicesPaginator(request);
* for (software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.ListVirtualMFADevicesIterable responses = client.listVirtualMFADevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVirtualMFADevices(software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListVirtualMfaDevicesRequest.Builder} avoiding the
* need to create one manually via {@link ListVirtualMfaDevicesRequest#builder()}
*
*
* @param listVirtualMfaDevicesRequest
* A {@link Consumer} that will call methods on {@link ListVirtualMFADevicesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ListVirtualMFADevices
* @see AWS API
* Documentation
*/
default ListVirtualMFADevicesIterable listVirtualMFADevicesPaginator(
Consumer listVirtualMfaDevicesRequest) throws AwsServiceException,
SdkClientException, IamException {
return listVirtualMFADevicesPaginator(ListVirtualMfaDevicesRequest.builder().applyMutation(listVirtualMfaDevicesRequest)
.build());
}
/**
*
* Adds or updates an inline policy document that is embedded in the specified IAM group.
*
*
* A user can also have managed policies attached to it. To attach a managed policy to a group, use
* AttachGroupPolicy. To create a new managed policy, use CreatePolicy. For information about
* policies, see Managed
* policies and inline policies in the IAM User Guide.
*
*
* For information about the maximum number of inline policies that you can embed in a group, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because policy documents can be large, you should use POST rather than GET when calling
* PutGroupPolicy
. For general information about using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* @param putGroupPolicyRequest
* @return Result of the PutGroupPolicy operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutGroupPolicy
* @see AWS API
* Documentation
*/
default PutGroupPolicyResponse putGroupPolicy(PutGroupPolicyRequest putGroupPolicyRequest) throws LimitExceededException,
MalformedPolicyDocumentException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates an inline policy document that is embedded in the specified IAM group.
*
*
* A user can also have managed policies attached to it. To attach a managed policy to a group, use
* AttachGroupPolicy. To create a new managed policy, use CreatePolicy. For information about
* policies, see Managed
* policies and inline policies in the IAM User Guide.
*
*
* For information about the maximum number of inline policies that you can embed in a group, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because policy documents can be large, you should use POST rather than GET when calling
* PutGroupPolicy
. For general information about using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutGroupPolicyRequest.Builder} avoiding the need to
* create one manually via {@link PutGroupPolicyRequest#builder()}
*
*
* @param putGroupPolicyRequest
* A {@link Consumer} that will call methods on {@link PutGroupPolicyRequest.Builder} to create a request.
* @return Result of the PutGroupPolicy operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutGroupPolicy
* @see AWS API
* Documentation
*/
default PutGroupPolicyResponse putGroupPolicy(Consumer putGroupPolicyRequest)
throws LimitExceededException, MalformedPolicyDocumentException, NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return putGroupPolicy(PutGroupPolicyRequest.builder().applyMutation(putGroupPolicyRequest).build());
}
/**
*
* Adds or updates the policy that is specified as the IAM role's permissions boundary. You can use an Amazon Web
* Services managed policy or a customer managed policy to set the boundary for a role. Use the boundary to control
* the maximum permissions that the role can have. Setting a permissions boundary is an advanced feature that can
* affect the permissions for the role.
*
*
* You cannot set the boundary for a service-linked role.
*
*
*
* Policies used as permissions boundaries do not provide permissions. You must also attach a permissions policy to
* the role. To learn how the effective permissions for a role are evaluated, see IAM JSON policy
* evaluation logic in the IAM User Guide.
*
*
*
* @param putRolePermissionsBoundaryRequest
* @return Result of the PutRolePermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutRolePermissionsBoundary
* @see AWS API Documentation
*/
default PutRolePermissionsBoundaryResponse putRolePermissionsBoundary(
PutRolePermissionsBoundaryRequest putRolePermissionsBoundaryRequest) throws NoSuchEntityException,
InvalidInputException, UnmodifiableEntityException, PolicyNotAttachableException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates the policy that is specified as the IAM role's permissions boundary. You can use an Amazon Web
* Services managed policy or a customer managed policy to set the boundary for a role. Use the boundary to control
* the maximum permissions that the role can have. Setting a permissions boundary is an advanced feature that can
* affect the permissions for the role.
*
*
* You cannot set the boundary for a service-linked role.
*
*
*
* Policies used as permissions boundaries do not provide permissions. You must also attach a permissions policy to
* the role. To learn how the effective permissions for a role are evaluated, see IAM JSON policy
* evaluation logic in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutRolePermissionsBoundaryRequest.Builder} avoiding
* the need to create one manually via {@link PutRolePermissionsBoundaryRequest#builder()}
*
*
* @param putRolePermissionsBoundaryRequest
* A {@link Consumer} that will call methods on {@link PutRolePermissionsBoundaryRequest.Builder} to create a
* request.
* @return Result of the PutRolePermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutRolePermissionsBoundary
* @see AWS API Documentation
*/
default PutRolePermissionsBoundaryResponse putRolePermissionsBoundary(
Consumer putRolePermissionsBoundaryRequest) throws NoSuchEntityException,
InvalidInputException, UnmodifiableEntityException, PolicyNotAttachableException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return putRolePermissionsBoundary(PutRolePermissionsBoundaryRequest.builder()
.applyMutation(putRolePermissionsBoundaryRequest).build());
}
/**
*
* Adds or updates an inline policy document that is embedded in the specified IAM role.
*
*
* When you embed an inline policy in a role, the inline policy is used as part of the role's access (permissions)
* policy. The role's trust policy is created at the same time as the role, using CreateRole. You can update
* a role's trust policy using UpdateAssumeRolePolicy. For more information about IAM roles, see Using roles to delegate permissions
* and federate identities.
*
*
* A role can also have a managed policy attached to it. To attach a managed policy to a role, use
* AttachRolePolicy. To create a new managed policy, use CreatePolicy. For information about policies,
* see Managed policies
* and inline policies in the IAM User Guide.
*
*
* For information about the maximum number of inline policies that you can embed with a role, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because policy documents can be large, you should use POST rather than GET when calling
* PutRolePolicy
. For general information about using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* @param putRolePolicyRequest
* @return Result of the PutRolePolicy operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutRolePolicy
* @see AWS API
* Documentation
*/
default PutRolePolicyResponse putRolePolicy(PutRolePolicyRequest putRolePolicyRequest) throws LimitExceededException,
MalformedPolicyDocumentException, NoSuchEntityException, UnmodifiableEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates an inline policy document that is embedded in the specified IAM role.
*
*
* When you embed an inline policy in a role, the inline policy is used as part of the role's access (permissions)
* policy. The role's trust policy is created at the same time as the role, using CreateRole. You can update
* a role's trust policy using UpdateAssumeRolePolicy. For more information about IAM roles, see Using roles to delegate permissions
* and federate identities.
*
*
* A role can also have a managed policy attached to it. To attach a managed policy to a role, use
* AttachRolePolicy. To create a new managed policy, use CreatePolicy. For information about policies,
* see Managed policies
* and inline policies in the IAM User Guide.
*
*
* For information about the maximum number of inline policies that you can embed with a role, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because policy documents can be large, you should use POST rather than GET when calling
* PutRolePolicy
. For general information about using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutRolePolicyRequest.Builder} avoiding the need to
* create one manually via {@link PutRolePolicyRequest#builder()}
*
*
* @param putRolePolicyRequest
* A {@link Consumer} that will call methods on {@link PutRolePolicyRequest.Builder} to create a request.
* @return Result of the PutRolePolicy operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutRolePolicy
* @see AWS API
* Documentation
*/
default PutRolePolicyResponse putRolePolicy(Consumer putRolePolicyRequest)
throws LimitExceededException, MalformedPolicyDocumentException, NoSuchEntityException, UnmodifiableEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return putRolePolicy(PutRolePolicyRequest.builder().applyMutation(putRolePolicyRequest).build());
}
/**
*
* Adds or updates the policy that is specified as the IAM user's permissions boundary. You can use an Amazon Web
* Services managed policy or a customer managed policy to set the boundary for a user. Use the boundary to control
* the maximum permissions that the user can have. Setting a permissions boundary is an advanced feature that can
* affect the permissions for the user.
*
*
*
* Policies that are used as permissions boundaries do not provide permissions. You must also attach a permissions
* policy to the user. To learn how the effective permissions for a user are evaluated, see IAM JSON policy
* evaluation logic in the IAM User Guide.
*
*
*
* @param putUserPermissionsBoundaryRequest
* @return Result of the PutUserPermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutUserPermissionsBoundary
* @see AWS API Documentation
*/
default PutUserPermissionsBoundaryResponse putUserPermissionsBoundary(
PutUserPermissionsBoundaryRequest putUserPermissionsBoundaryRequest) throws NoSuchEntityException,
InvalidInputException, PolicyNotAttachableException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates the policy that is specified as the IAM user's permissions boundary. You can use an Amazon Web
* Services managed policy or a customer managed policy to set the boundary for a user. Use the boundary to control
* the maximum permissions that the user can have. Setting a permissions boundary is an advanced feature that can
* affect the permissions for the user.
*
*
*
* Policies that are used as permissions boundaries do not provide permissions. You must also attach a permissions
* policy to the user. To learn how the effective permissions for a user are evaluated, see IAM JSON policy
* evaluation logic in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutUserPermissionsBoundaryRequest.Builder} avoiding
* the need to create one manually via {@link PutUserPermissionsBoundaryRequest#builder()}
*
*
* @param putUserPermissionsBoundaryRequest
* A {@link Consumer} that will call methods on {@link PutUserPermissionsBoundaryRequest.Builder} to create a
* request.
* @return Result of the PutUserPermissionsBoundary operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyNotAttachableException
* The request failed because Amazon Web Services service role policies can only be attached to the
* service-linked role for that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutUserPermissionsBoundary
* @see AWS API Documentation
*/
default PutUserPermissionsBoundaryResponse putUserPermissionsBoundary(
Consumer putUserPermissionsBoundaryRequest) throws NoSuchEntityException,
InvalidInputException, PolicyNotAttachableException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return putUserPermissionsBoundary(PutUserPermissionsBoundaryRequest.builder()
.applyMutation(putUserPermissionsBoundaryRequest).build());
}
/**
*
* Adds or updates an inline policy document that is embedded in the specified IAM user.
*
*
* An IAM user can also have a managed policy attached to it. To attach a managed policy to a user, use
* AttachUserPolicy. To create a new managed policy, use CreatePolicy. For information about policies,
* see Managed policies
* and inline policies in the IAM User Guide.
*
*
* For information about the maximum number of inline policies that you can embed in a user, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because policy documents can be large, you should use POST rather than GET when calling
* PutUserPolicy
. For general information about using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* @param putUserPolicyRequest
* @return Result of the PutUserPolicy operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutUserPolicy
* @see AWS API
* Documentation
*/
default PutUserPolicyResponse putUserPolicy(PutUserPolicyRequest putUserPolicyRequest) throws LimitExceededException,
MalformedPolicyDocumentException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates an inline policy document that is embedded in the specified IAM user.
*
*
* An IAM user can also have a managed policy attached to it. To attach a managed policy to a user, use
* AttachUserPolicy. To create a new managed policy, use CreatePolicy. For information about policies,
* see Managed policies
* and inline policies in the IAM User Guide.
*
*
* For information about the maximum number of inline policies that you can embed in a user, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because policy documents can be large, you should use POST rather than GET when calling
* PutUserPolicy
. For general information about using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutUserPolicyRequest.Builder} avoiding the need to
* create one manually via {@link PutUserPolicyRequest#builder()}
*
*
* @param putUserPolicyRequest
* A {@link Consumer} that will call methods on {@link PutUserPolicyRequest.Builder} to create a request.
* @return Result of the PutUserPolicy operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.PutUserPolicy
* @see AWS API
* Documentation
*/
default PutUserPolicyResponse putUserPolicy(Consumer putUserPolicyRequest)
throws LimitExceededException, MalformedPolicyDocumentException, NoSuchEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return putUserPolicy(PutUserPolicyRequest.builder().applyMutation(putUserPolicyRequest).build());
}
/**
*
* Removes the specified client ID (also known as audience) from the list of client IDs registered for the specified
* IAM OpenID Connect (OIDC) provider resource object.
*
*
* This operation is idempotent; it does not fail or return an error if you try to remove a client ID that does not
* exist.
*
*
* @param removeClientIdFromOpenIdConnectProviderRequest
* @return Result of the RemoveClientIDFromOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.RemoveClientIDFromOpenIDConnectProvider
* @see AWS API Documentation
*/
default RemoveClientIdFromOpenIdConnectProviderResponse removeClientIDFromOpenIDConnectProvider(
RemoveClientIdFromOpenIdConnectProviderRequest removeClientIdFromOpenIdConnectProviderRequest)
throws InvalidInputException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified client ID (also known as audience) from the list of client IDs registered for the specified
* IAM OpenID Connect (OIDC) provider resource object.
*
*
* This operation is idempotent; it does not fail or return an error if you try to remove a client ID that does not
* exist.
*
*
*
* This is a convenience which creates an instance of the
* {@link RemoveClientIdFromOpenIdConnectProviderRequest.Builder} avoiding the need to create one manually via
* {@link RemoveClientIdFromOpenIdConnectProviderRequest#builder()}
*
*
* @param removeClientIdFromOpenIdConnectProviderRequest
* A {@link Consumer} that will call methods on
* {@link RemoveClientIDFromOpenIDConnectProviderRequest.Builder} to create a request.
* @return Result of the RemoveClientIDFromOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.RemoveClientIDFromOpenIDConnectProvider
* @see AWS API Documentation
*/
default RemoveClientIdFromOpenIdConnectProviderResponse removeClientIDFromOpenIDConnectProvider(
Consumer removeClientIdFromOpenIdConnectProviderRequest)
throws InvalidInputException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return removeClientIDFromOpenIDConnectProvider(RemoveClientIdFromOpenIdConnectProviderRequest.builder()
.applyMutation(removeClientIdFromOpenIdConnectProviderRequest).build());
}
/**
*
* Removes the specified IAM role from the specified EC2 instance profile.
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the role you are about to remove from the
* instance profile. Removing a role from an instance profile that is associated with a running instance might break
* any applications running on the instance.
*
*
*
* For more information about IAM roles, see Working with roles. For more
* information about instance profiles, see About instance profiles.
*
*
* @param removeRoleFromInstanceProfileRequest
* @return Result of the RemoveRoleFromInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.RemoveRoleFromInstanceProfile
* @see AWS API Documentation
*/
default RemoveRoleFromInstanceProfileResponse removeRoleFromInstanceProfile(
RemoveRoleFromInstanceProfileRequest removeRoleFromInstanceProfileRequest) throws NoSuchEntityException,
LimitExceededException, UnmodifiableEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified IAM role from the specified EC2 instance profile.
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the role you are about to remove from the
* instance profile. Removing a role from an instance profile that is associated with a running instance might break
* any applications running on the instance.
*
*
*
* For more information about IAM roles, see Working with roles. For more
* information about instance profiles, see About instance profiles.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveRoleFromInstanceProfileRequest.Builder}
* avoiding the need to create one manually via {@link RemoveRoleFromInstanceProfileRequest#builder()}
*
*
* @param removeRoleFromInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link RemoveRoleFromInstanceProfileRequest.Builder} to
* create a request.
* @return Result of the RemoveRoleFromInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.RemoveRoleFromInstanceProfile
* @see AWS API Documentation
*/
default RemoveRoleFromInstanceProfileResponse removeRoleFromInstanceProfile(
Consumer removeRoleFromInstanceProfileRequest)
throws NoSuchEntityException, LimitExceededException, UnmodifiableEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return removeRoleFromInstanceProfile(RemoveRoleFromInstanceProfileRequest.builder()
.applyMutation(removeRoleFromInstanceProfileRequest).build());
}
/**
*
* Removes the specified user from the specified group.
*
*
* @param removeUserFromGroupRequest
* @return Result of the RemoveUserFromGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.RemoveUserFromGroup
* @see AWS API
* Documentation
*/
default RemoveUserFromGroupResponse removeUserFromGroup(RemoveUserFromGroupRequest removeUserFromGroupRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified user from the specified group.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveUserFromGroupRequest.Builder} avoiding the
* need to create one manually via {@link RemoveUserFromGroupRequest#builder()}
*
*
* @param removeUserFromGroupRequest
* A {@link Consumer} that will call methods on {@link RemoveUserFromGroupRequest.Builder} to create a
* request.
* @return Result of the RemoveUserFromGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.RemoveUserFromGroup
* @see AWS API
* Documentation
*/
default RemoveUserFromGroupResponse removeUserFromGroup(
Consumer removeUserFromGroupRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return removeUserFromGroup(RemoveUserFromGroupRequest.builder().applyMutation(removeUserFromGroupRequest).build());
}
/**
*
* Resets the password for a service-specific credential. The new password is Amazon Web Services generated and
* cryptographically strong. It cannot be configured by the user. Resetting the password immediately invalidates the
* previous password associated with this user.
*
*
* @param resetServiceSpecificCredentialRequest
* @return Result of the ResetServiceSpecificCredential operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ResetServiceSpecificCredential
* @see AWS API Documentation
*/
default ResetServiceSpecificCredentialResponse resetServiceSpecificCredential(
ResetServiceSpecificCredentialRequest resetServiceSpecificCredentialRequest) throws NoSuchEntityException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Resets the password for a service-specific credential. The new password is Amazon Web Services generated and
* cryptographically strong. It cannot be configured by the user. Resetting the password immediately invalidates the
* previous password associated with this user.
*
*
*
* This is a convenience which creates an instance of the {@link ResetServiceSpecificCredentialRequest.Builder}
* avoiding the need to create one manually via {@link ResetServiceSpecificCredentialRequest#builder()}
*
*
* @param resetServiceSpecificCredentialRequest
* A {@link Consumer} that will call methods on {@link ResetServiceSpecificCredentialRequest.Builder} to
* create a request.
* @return Result of the ResetServiceSpecificCredential operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ResetServiceSpecificCredential
* @see AWS API Documentation
*/
default ResetServiceSpecificCredentialResponse resetServiceSpecificCredential(
Consumer resetServiceSpecificCredentialRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
return resetServiceSpecificCredential(ResetServiceSpecificCredentialRequest.builder()
.applyMutation(resetServiceSpecificCredentialRequest).build());
}
/**
*
* Synchronizes the specified MFA device with its IAM resource object on the Amazon Web Services servers.
*
*
* For more information about creating and working with virtual MFA devices, see Using a virtual MFA device in
* the IAM User Guide.
*
*
* @param resyncMfaDeviceRequest
* @return Result of the ResyncMFADevice operation returned by the service.
* @throws InvalidAuthenticationCodeException
* The request was rejected because the authentication code was not recognized. The error message describes
* the specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ResyncMFADevice
* @see AWS API
* Documentation
*/
default ResyncMfaDeviceResponse resyncMFADevice(ResyncMfaDeviceRequest resyncMfaDeviceRequest)
throws InvalidAuthenticationCodeException, NoSuchEntityException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Synchronizes the specified MFA device with its IAM resource object on the Amazon Web Services servers.
*
*
* For more information about creating and working with virtual MFA devices, see Using a virtual MFA device in
* the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ResyncMfaDeviceRequest.Builder} avoiding the need
* to create one manually via {@link ResyncMfaDeviceRequest#builder()}
*
*
* @param resyncMfaDeviceRequest
* A {@link Consumer} that will call methods on {@link ResyncMFADeviceRequest.Builder} to create a request.
* @return Result of the ResyncMFADevice operation returned by the service.
* @throws InvalidAuthenticationCodeException
* The request was rejected because the authentication code was not recognized. The error message describes
* the specific error.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.ResyncMFADevice
* @see AWS API
* Documentation
*/
default ResyncMfaDeviceResponse resyncMFADevice(Consumer resyncMfaDeviceRequest)
throws InvalidAuthenticationCodeException, NoSuchEntityException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return resyncMFADevice(ResyncMfaDeviceRequest.builder().applyMutation(resyncMfaDeviceRequest).build());
}
/**
*
* Sets the specified version of the specified policy as the policy's default (operative) version.
*
*
* This operation affects all users, groups, and roles that the policy is attached to. To list the users, groups,
* and roles that the policy is attached to, use ListEntitiesForPolicy.
*
*
* For information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param setDefaultPolicyVersionRequest
* @return Result of the SetDefaultPolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SetDefaultPolicyVersion
* @see AWS
* API Documentation
*/
default SetDefaultPolicyVersionResponse setDefaultPolicyVersion(SetDefaultPolicyVersionRequest setDefaultPolicyVersionRequest)
throws NoSuchEntityException, InvalidInputException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the specified version of the specified policy as the policy's default (operative) version.
*
*
* This operation affects all users, groups, and roles that the policy is attached to. To list the users, groups,
* and roles that the policy is attached to, use ListEntitiesForPolicy.
*
*
* For information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link SetDefaultPolicyVersionRequest.Builder} avoiding
* the need to create one manually via {@link SetDefaultPolicyVersionRequest#builder()}
*
*
* @param setDefaultPolicyVersionRequest
* A {@link Consumer} that will call methods on {@link SetDefaultPolicyVersionRequest.Builder} to create a
* request.
* @return Result of the SetDefaultPolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SetDefaultPolicyVersion
* @see AWS
* API Documentation
*/
default SetDefaultPolicyVersionResponse setDefaultPolicyVersion(
Consumer setDefaultPolicyVersionRequest) throws NoSuchEntityException,
InvalidInputException, LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException,
IamException {
return setDefaultPolicyVersion(SetDefaultPolicyVersionRequest.builder().applyMutation(setDefaultPolicyVersionRequest)
.build());
}
/**
*
* Sets the specified version of the global endpoint token as the token version used for the Amazon Web Services
* account.
*
*
* By default, Security Token Service (STS) is available as a global service, and all STS requests go to a single
* endpoint at https://sts.amazonaws.com
. Amazon Web Services recommends using Regional STS endpoints
* to reduce latency, build in redundancy, and increase session token availability. For information about Regional
* endpoints for STS, see Security Token Service
* endpoints and quotas in the Amazon Web Services General Reference.
*
*
* If you make an STS call to the global endpoint, the resulting session tokens might be valid in some Regions but
* not others. It depends on the version that is set in this operation. Version 1 tokens are valid only in Amazon
* Web Services Regions that are available by default. These tokens do not work in manually enabled Regions, such as
* Asia Pacific (Hong Kong). Version 2 tokens are valid in all Regions. However, version 2 tokens are longer and
* might affect systems where you temporarily store tokens. For information, see Activating and
* deactivating STS in an Amazon Web Services Region in the IAM User Guide.
*
*
* To view the current session token version, see the GlobalEndpointTokenVersion
entry in the response
* of the GetAccountSummary operation.
*
*
* @param setSecurityTokenServicePreferencesRequest
* @return Result of the SetSecurityTokenServicePreferences operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SetSecurityTokenServicePreferences
* @see AWS API Documentation
*/
default SetSecurityTokenServicePreferencesResponse setSecurityTokenServicePreferences(
SetSecurityTokenServicePreferencesRequest setSecurityTokenServicePreferencesRequest) throws ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the specified version of the global endpoint token as the token version used for the Amazon Web Services
* account.
*
*
* By default, Security Token Service (STS) is available as a global service, and all STS requests go to a single
* endpoint at https://sts.amazonaws.com
. Amazon Web Services recommends using Regional STS endpoints
* to reduce latency, build in redundancy, and increase session token availability. For information about Regional
* endpoints for STS, see Security Token Service
* endpoints and quotas in the Amazon Web Services General Reference.
*
*
* If you make an STS call to the global endpoint, the resulting session tokens might be valid in some Regions but
* not others. It depends on the version that is set in this operation. Version 1 tokens are valid only in Amazon
* Web Services Regions that are available by default. These tokens do not work in manually enabled Regions, such as
* Asia Pacific (Hong Kong). Version 2 tokens are valid in all Regions. However, version 2 tokens are longer and
* might affect systems where you temporarily store tokens. For information, see Activating and
* deactivating STS in an Amazon Web Services Region in the IAM User Guide.
*
*
* To view the current session token version, see the GlobalEndpointTokenVersion
entry in the response
* of the GetAccountSummary operation.
*
*
*
* This is a convenience which creates an instance of the {@link SetSecurityTokenServicePreferencesRequest.Builder}
* avoiding the need to create one manually via {@link SetSecurityTokenServicePreferencesRequest#builder()}
*
*
* @param setSecurityTokenServicePreferencesRequest
* A {@link Consumer} that will call methods on {@link SetSecurityTokenServicePreferencesRequest.Builder} to
* create a request.
* @return Result of the SetSecurityTokenServicePreferences operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SetSecurityTokenServicePreferences
* @see AWS API Documentation
*/
default SetSecurityTokenServicePreferencesResponse setSecurityTokenServicePreferences(
Consumer setSecurityTokenServicePreferencesRequest)
throws ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return setSecurityTokenServicePreferences(SetSecurityTokenServicePreferencesRequest.builder()
.applyMutation(setSecurityTokenServicePreferencesRequest).build());
}
/**
*
* Simulate how a set of IAM policies and optionally a resource-based policy works with a list of API operations and
* Amazon Web Services resources to determine the policies' effective permissions. The policies are provided as
* strings.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations. You can simulate resources that don't exist in your account.
*
*
* If you want to simulate existing policies that are attached to an IAM user, group, or role, use
* SimulatePrincipalPolicy instead.
*
*
* Context keys are variables that are maintained by Amazon Web Services and its services and which provide details
* about the context of an API query request. You can use the Condition
element of an IAM policy to
* evaluate context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForCustomPolicy.
*
*
* If the output is long, you can use MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* @param simulateCustomPolicyRequest
* @return Result of the SimulateCustomPolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulateCustomPolicy
* @see AWS API
* Documentation
*/
default SimulateCustomPolicyResponse simulateCustomPolicy(SimulateCustomPolicyRequest simulateCustomPolicyRequest)
throws InvalidInputException, PolicyEvaluationException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Simulate how a set of IAM policies and optionally a resource-based policy works with a list of API operations and
* Amazon Web Services resources to determine the policies' effective permissions. The policies are provided as
* strings.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations. You can simulate resources that don't exist in your account.
*
*
* If you want to simulate existing policies that are attached to an IAM user, group, or role, use
* SimulatePrincipalPolicy instead.
*
*
* Context keys are variables that are maintained by Amazon Web Services and its services and which provide details
* about the context of an API query request. You can use the Condition
element of an IAM policy to
* evaluate context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForCustomPolicy.
*
*
* If the output is long, you can use MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link SimulateCustomPolicyRequest.Builder} avoiding the
* need to create one manually via {@link SimulateCustomPolicyRequest#builder()}
*
*
* @param simulateCustomPolicyRequest
* A {@link Consumer} that will call methods on {@link SimulateCustomPolicyRequest.Builder} to create a
* request.
* @return Result of the SimulateCustomPolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulateCustomPolicy
* @see AWS API
* Documentation
*/
default SimulateCustomPolicyResponse simulateCustomPolicy(
Consumer simulateCustomPolicyRequest) throws InvalidInputException,
PolicyEvaluationException, AwsServiceException, SdkClientException, IamException {
return simulateCustomPolicy(SimulateCustomPolicyRequest.builder().applyMutation(simulateCustomPolicyRequest).build());
}
/**
*
* Simulate how a set of IAM policies and optionally a resource-based policy works with a list of API operations and
* Amazon Web Services resources to determine the policies' effective permissions. The policies are provided as
* strings.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations. You can simulate resources that don't exist in your account.
*
*
* If you want to simulate existing policies that are attached to an IAM user, group, or role, use
* SimulatePrincipalPolicy instead.
*
*
* Context keys are variables that are maintained by Amazon Web Services and its services and which provide details
* about the context of an API query request. You can use the Condition
element of an IAM policy to
* evaluate context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForCustomPolicy.
*
*
* If the output is long, you can use MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #simulateCustomPolicy(software.amazon.awssdk.services.iam.model.SimulateCustomPolicyRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulateCustomPolicyIterable responses = client.simulateCustomPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.SimulateCustomPolicyIterable responses = client
* .simulateCustomPolicyPaginator(request);
* for (software.amazon.awssdk.services.iam.model.SimulateCustomPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulateCustomPolicyIterable responses = client.simulateCustomPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #simulateCustomPolicy(software.amazon.awssdk.services.iam.model.SimulateCustomPolicyRequest)}
* operation.
*
*
* @param simulateCustomPolicyRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulateCustomPolicy
* @see AWS API
* Documentation
*/
default SimulateCustomPolicyIterable simulateCustomPolicyPaginator(SimulateCustomPolicyRequest simulateCustomPolicyRequest)
throws InvalidInputException, PolicyEvaluationException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Simulate how a set of IAM policies and optionally a resource-based policy works with a list of API operations and
* Amazon Web Services resources to determine the policies' effective permissions. The policies are provided as
* strings.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations. You can simulate resources that don't exist in your account.
*
*
* If you want to simulate existing policies that are attached to an IAM user, group, or role, use
* SimulatePrincipalPolicy instead.
*
*
* Context keys are variables that are maintained by Amazon Web Services and its services and which provide details
* about the context of an API query request. You can use the Condition
element of an IAM policy to
* evaluate context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForCustomPolicy.
*
*
* If the output is long, you can use MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #simulateCustomPolicy(software.amazon.awssdk.services.iam.model.SimulateCustomPolicyRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulateCustomPolicyIterable responses = client.simulateCustomPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.SimulateCustomPolicyIterable responses = client
* .simulateCustomPolicyPaginator(request);
* for (software.amazon.awssdk.services.iam.model.SimulateCustomPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulateCustomPolicyIterable responses = client.simulateCustomPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #simulateCustomPolicy(software.amazon.awssdk.services.iam.model.SimulateCustomPolicyRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SimulateCustomPolicyRequest.Builder} avoiding the
* need to create one manually via {@link SimulateCustomPolicyRequest#builder()}
*
*
* @param simulateCustomPolicyRequest
* A {@link Consumer} that will call methods on {@link SimulateCustomPolicyRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulateCustomPolicy
* @see AWS API
* Documentation
*/
default SimulateCustomPolicyIterable simulateCustomPolicyPaginator(
Consumer simulateCustomPolicyRequest) throws InvalidInputException,
PolicyEvaluationException, AwsServiceException, SdkClientException, IamException {
return simulateCustomPolicyPaginator(SimulateCustomPolicyRequest.builder().applyMutation(simulateCustomPolicyRequest)
.build());
}
/**
*
* Simulate how a set of IAM policies attached to an IAM entity works with a list of API operations and Amazon Web
* Services resources to determine the policies' effective permissions. The entity can be an IAM user, group, or
* role. If you specify a user, then the simulation also includes all of the policies that are attached to groups
* that the user belongs to. You can simulate resources that don't exist in your account.
*
*
* You can optionally include a list of one or more additional policies specified as strings to include in the
* simulation. If you want to simulate only policies specified as strings, use SimulateCustomPolicy instead.
*
*
* You can also optionally include one resource-based policy to be evaluated with each of the resources included in
* the simulation for IAM users only.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations.
*
*
* Note: This operation discloses information about the permissions granted to other users. If you do not
* want users to see other user's permissions, then consider allowing them to use SimulateCustomPolicy
* instead.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. You can use the Condition
element of an IAM policy to evaluate
* context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForPrincipalPolicy.
*
*
* If the output is long, you can use the MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* @param simulatePrincipalPolicyRequest
* @return Result of the SimulatePrincipalPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulatePrincipalPolicy
* @see AWS
* API Documentation
*/
default SimulatePrincipalPolicyResponse simulatePrincipalPolicy(SimulatePrincipalPolicyRequest simulatePrincipalPolicyRequest)
throws NoSuchEntityException, InvalidInputException, PolicyEvaluationException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Simulate how a set of IAM policies attached to an IAM entity works with a list of API operations and Amazon Web
* Services resources to determine the policies' effective permissions. The entity can be an IAM user, group, or
* role. If you specify a user, then the simulation also includes all of the policies that are attached to groups
* that the user belongs to. You can simulate resources that don't exist in your account.
*
*
* You can optionally include a list of one or more additional policies specified as strings to include in the
* simulation. If you want to simulate only policies specified as strings, use SimulateCustomPolicy instead.
*
*
* You can also optionally include one resource-based policy to be evaluated with each of the resources included in
* the simulation for IAM users only.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations.
*
*
* Note: This operation discloses information about the permissions granted to other users. If you do not
* want users to see other user's permissions, then consider allowing them to use SimulateCustomPolicy
* instead.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. You can use the Condition
element of an IAM policy to evaluate
* context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForPrincipalPolicy.
*
*
* If the output is long, you can use the MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link SimulatePrincipalPolicyRequest.Builder} avoiding
* the need to create one manually via {@link SimulatePrincipalPolicyRequest#builder()}
*
*
* @param simulatePrincipalPolicyRequest
* A {@link Consumer} that will call methods on {@link SimulatePrincipalPolicyRequest.Builder} to create a
* request.
* @return Result of the SimulatePrincipalPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulatePrincipalPolicy
* @see AWS
* API Documentation
*/
default SimulatePrincipalPolicyResponse simulatePrincipalPolicy(
Consumer simulatePrincipalPolicyRequest) throws NoSuchEntityException,
InvalidInputException, PolicyEvaluationException, AwsServiceException, SdkClientException, IamException {
return simulatePrincipalPolicy(SimulatePrincipalPolicyRequest.builder().applyMutation(simulatePrincipalPolicyRequest)
.build());
}
/**
*
* Simulate how a set of IAM policies attached to an IAM entity works with a list of API operations and Amazon Web
* Services resources to determine the policies' effective permissions. The entity can be an IAM user, group, or
* role. If you specify a user, then the simulation also includes all of the policies that are attached to groups
* that the user belongs to. You can simulate resources that don't exist in your account.
*
*
* You can optionally include a list of one or more additional policies specified as strings to include in the
* simulation. If you want to simulate only policies specified as strings, use SimulateCustomPolicy instead.
*
*
* You can also optionally include one resource-based policy to be evaluated with each of the resources included in
* the simulation for IAM users only.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations.
*
*
* Note: This operation discloses information about the permissions granted to other users. If you do not
* want users to see other user's permissions, then consider allowing them to use SimulateCustomPolicy
* instead.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. You can use the Condition
element of an IAM policy to evaluate
* context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForPrincipalPolicy.
*
*
* If the output is long, you can use the MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #simulatePrincipalPolicy(software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulatePrincipalPolicyIterable responses = client.simulatePrincipalPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.SimulatePrincipalPolicyIterable responses = client
* .simulatePrincipalPolicyPaginator(request);
* for (software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulatePrincipalPolicyIterable responses = client.simulatePrincipalPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #simulatePrincipalPolicy(software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyRequest)}
* operation.
*
*
* @param simulatePrincipalPolicyRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulatePrincipalPolicy
* @see AWS
* API Documentation
*/
default SimulatePrincipalPolicyIterable simulatePrincipalPolicyPaginator(
SimulatePrincipalPolicyRequest simulatePrincipalPolicyRequest) throws NoSuchEntityException, InvalidInputException,
PolicyEvaluationException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Simulate how a set of IAM policies attached to an IAM entity works with a list of API operations and Amazon Web
* Services resources to determine the policies' effective permissions. The entity can be an IAM user, group, or
* role. If you specify a user, then the simulation also includes all of the policies that are attached to groups
* that the user belongs to. You can simulate resources that don't exist in your account.
*
*
* You can optionally include a list of one or more additional policies specified as strings to include in the
* simulation. If you want to simulate only policies specified as strings, use SimulateCustomPolicy instead.
*
*
* You can also optionally include one resource-based policy to be evaluated with each of the resources included in
* the simulation for IAM users only.
*
*
* The simulation does not perform the API operations; it only checks the authorization to determine if the
* simulated policies allow or deny the operations.
*
*
* Note: This operation discloses information about the permissions granted to other users. If you do not
* want users to see other user's permissions, then consider allowing them to use SimulateCustomPolicy
* instead.
*
*
* Context keys are variables maintained by Amazon Web Services and its services that provide details about the
* context of an API query request. You can use the Condition
element of an IAM policy to evaluate
* context keys. To get the list of context keys that the policies require for correct simulation, use
* GetContextKeysForPrincipalPolicy.
*
*
* If the output is long, you can use the MaxItems
and Marker
parameters to paginate the
* results.
*
*
*
* The IAM policy simulator evaluates statements in the identity-based policy and the inputs that you provide during
* simulation. The policy simulator results can differ from your live Amazon Web Services environment. We recommend
* that you check your policies against your live Amazon Web Services environment after testing using the policy
* simulator to confirm that you have the desired results. For more information about using the policy simulator,
* see Testing IAM
* policies with the IAM policy simulator in the IAM User Guide.
*
*
*
* This is a variant of
* {@link #simulatePrincipalPolicy(software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulatePrincipalPolicyIterable responses = client.simulatePrincipalPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.iam.paginators.SimulatePrincipalPolicyIterable responses = client
* .simulatePrincipalPolicyPaginator(request);
* for (software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.iam.paginators.SimulatePrincipalPolicyIterable responses = client.simulatePrincipalPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #simulatePrincipalPolicy(software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link SimulatePrincipalPolicyRequest.Builder} avoiding
* the need to create one manually via {@link SimulatePrincipalPolicyRequest#builder()}
*
*
* @param simulatePrincipalPolicyRequest
* A {@link Consumer} that will call methods on {@link SimulatePrincipalPolicyRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws PolicyEvaluationException
* The request failed because a provided policy could not be successfully evaluated. An additional detailed
* message indicates the source of the failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.SimulatePrincipalPolicy
* @see AWS
* API Documentation
*/
default SimulatePrincipalPolicyIterable simulatePrincipalPolicyPaginator(
Consumer simulatePrincipalPolicyRequest) throws NoSuchEntityException,
InvalidInputException, PolicyEvaluationException, AwsServiceException, SdkClientException, IamException {
return simulatePrincipalPolicyPaginator(SimulatePrincipalPolicyRequest.builder()
.applyMutation(simulatePrincipalPolicyRequest).build());
}
/**
*
* Adds one or more tags to an IAM instance profile. If a tag with the same key name already exists, then that tag
* is overwritten with the new value.
*
*
* Each tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM instance profile that has a specified tag attached. For examples of policies that show how
* to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* @param tagInstanceProfileRequest
* @return Result of the TagInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagInstanceProfile
* @see AWS API
* Documentation
*/
default TagInstanceProfileResponse tagInstanceProfile(TagInstanceProfileRequest tagInstanceProfileRequest)
throws NoSuchEntityException, InvalidInputException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an IAM instance profile. If a tag with the same key name already exists, then that tag
* is overwritten with the new value.
*
*
* Each tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM instance profile that has a specified tag attached. For examples of policies that show how
* to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TagInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link TagInstanceProfileRequest#builder()}
*
*
* @param tagInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link TagInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the TagInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagInstanceProfile
* @see AWS API
* Documentation
*/
default TagInstanceProfileResponse tagInstanceProfile(Consumer tagInstanceProfileRequest)
throws NoSuchEntityException, InvalidInputException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return tagInstanceProfile(TagInstanceProfileRequest.builder().applyMutation(tagInstanceProfileRequest).build());
}
/**
*
* Adds one or more tags to an IAM virtual multi-factor authentication (MFA) device. If a tag with the same key name
* already exists, then that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM virtual MFA device that has a specified tag attached. For examples of policies that show
* how to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* @param tagMfaDeviceRequest
* @return Result of the TagMFADevice operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagMFADevice
* @see AWS API
* Documentation
*/
default TagMfaDeviceResponse tagMFADevice(TagMfaDeviceRequest tagMfaDeviceRequest) throws InvalidInputException,
NoSuchEntityException, LimitExceededException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an IAM virtual multi-factor authentication (MFA) device. If a tag with the same key name
* already exists, then that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM virtual MFA device that has a specified tag attached. For examples of policies that show
* how to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TagMfaDeviceRequest.Builder} avoiding the need to
* create one manually via {@link TagMfaDeviceRequest#builder()}
*
*
* @param tagMfaDeviceRequest
* A {@link Consumer} that will call methods on {@link TagMFADeviceRequest.Builder} to create a request.
* @return Result of the TagMFADevice operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagMFADevice
* @see AWS API
* Documentation
*/
default TagMfaDeviceResponse tagMFADevice(Consumer tagMfaDeviceRequest)
throws InvalidInputException, NoSuchEntityException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return tagMFADevice(TagMfaDeviceRequest.builder().applyMutation(tagMfaDeviceRequest).build());
}
/**
*
* Adds one or more tags to an OpenID Connect (OIDC)-compatible identity provider. For more information about these
* providers, see About web
* identity federation. If a tag with the same key name already exists, then that tag is overwritten with the
* new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an OIDC provider that has a specified tag attached. For examples of policies that show how to use
* tags to control access, see Control
* access using IAM tags in the IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* @param tagOpenIdConnectProviderRequest
* @return Result of the TagOpenIDConnectProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagOpenIDConnectProvider
* @see AWS
* API Documentation
*/
default TagOpenIdConnectProviderResponse tagOpenIDConnectProvider(
TagOpenIdConnectProviderRequest tagOpenIdConnectProviderRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an OpenID Connect (OIDC)-compatible identity provider. For more information about these
* providers, see About web
* identity federation. If a tag with the same key name already exists, then that tag is overwritten with the
* new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an OIDC provider that has a specified tag attached. For examples of policies that show how to use
* tags to control access, see Control
* access using IAM tags in the IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TagOpenIdConnectProviderRequest.Builder} avoiding
* the need to create one manually via {@link TagOpenIdConnectProviderRequest#builder()}
*
*
* @param tagOpenIdConnectProviderRequest
* A {@link Consumer} that will call methods on {@link TagOpenIDConnectProviderRequest.Builder} to create a
* request.
* @return Result of the TagOpenIDConnectProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagOpenIDConnectProvider
* @see AWS
* API Documentation
*/
default TagOpenIdConnectProviderResponse tagOpenIDConnectProvider(
Consumer tagOpenIdConnectProviderRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return tagOpenIDConnectProvider(TagOpenIdConnectProviderRequest.builder().applyMutation(tagOpenIdConnectProviderRequest)
.build());
}
/**
*
* Adds one or more tags to an IAM customer managed policy. If a tag with the same key name already exists, then
* that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM customer managed policy that has a specified tag attached. For examples of policies that
* show how to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* @param tagPolicyRequest
* @return Result of the TagPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagPolicy
* @see AWS API
* Documentation
*/
default TagPolicyResponse tagPolicy(TagPolicyRequest tagPolicyRequest) throws NoSuchEntityException, LimitExceededException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an IAM customer managed policy. If a tag with the same key name already exists, then
* that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM customer managed policy that has a specified tag attached. For examples of policies that
* show how to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TagPolicyRequest.Builder} avoiding the need to
* create one manually via {@link TagPolicyRequest#builder()}
*
*
* @param tagPolicyRequest
* A {@link Consumer} that will call methods on {@link TagPolicyRequest.Builder} to create a request.
* @return Result of the TagPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagPolicy
* @see AWS API
* Documentation
*/
default TagPolicyResponse tagPolicy(Consumer tagPolicyRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return tagPolicy(TagPolicyRequest.builder().applyMutation(tagPolicyRequest).build());
}
/**
*
* Adds one or more tags to an IAM role. The role can be a regular role or a service-linked role. If a tag with the
* same key name already exists, then that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM role that has a specified tag attached. You can also restrict access to only those
* resources that have a certain tag attached. For examples of policies that show how to use tags to control access,
* see Control access using IAM tags
* in the IAM User Guide.
*
*
* -
*
* Cost allocation - Use tags to help track which individuals and teams are using which Amazon Web Services
* resources.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* For more information about tagging, see Tagging IAM identities in the IAM
* User Guide.
*
*
* @param tagRoleRequest
* @return Result of the TagRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagRole
* @see AWS API
* Documentation
*/
default TagRoleResponse tagRole(TagRoleRequest tagRoleRequest) throws NoSuchEntityException, LimitExceededException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an IAM role. The role can be a regular role or a service-linked role. If a tag with the
* same key name already exists, then that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM role that has a specified tag attached. You can also restrict access to only those
* resources that have a certain tag attached. For examples of policies that show how to use tags to control access,
* see Control access using IAM tags
* in the IAM User Guide.
*
*
* -
*
* Cost allocation - Use tags to help track which individuals and teams are using which Amazon Web Services
* resources.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* For more information about tagging, see Tagging IAM identities in the IAM
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link TagRoleRequest.Builder} avoiding the need to create
* one manually via {@link TagRoleRequest#builder()}
*
*
* @param tagRoleRequest
* A {@link Consumer} that will call methods on {@link TagRoleRequest.Builder} to create a request.
* @return Result of the TagRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagRole
* @see AWS API
* Documentation
*/
default TagRoleResponse tagRole(Consumer tagRoleRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return tagRole(TagRoleRequest.builder().applyMutation(tagRoleRequest).build());
}
/**
*
* Adds one or more tags to a Security Assertion Markup Language (SAML) identity provider. For more information
* about these providers, see About SAML 2.0-based
* federation . If a tag with the same key name already exists, then that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only a SAML identity provider that has a specified tag attached. For examples of policies that show how
* to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* @param tagSamlProviderRequest
* @return Result of the TagSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagSAMLProvider
* @see AWS API
* Documentation
*/
default TagSamlProviderResponse tagSAMLProvider(TagSamlProviderRequest tagSamlProviderRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to a Security Assertion Markup Language (SAML) identity provider. For more information
* about these providers, see About SAML 2.0-based
* federation . If a tag with the same key name already exists, then that tag is overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only a SAML identity provider that has a specified tag attached. For examples of policies that show how
* to use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TagSamlProviderRequest.Builder} avoiding the need
* to create one manually via {@link TagSamlProviderRequest#builder()}
*
*
* @param tagSamlProviderRequest
* A {@link Consumer} that will call methods on {@link TagSAMLProviderRequest.Builder} to create a request.
* @return Result of the TagSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagSAMLProvider
* @see AWS API
* Documentation
*/
default TagSamlProviderResponse tagSAMLProvider(Consumer tagSamlProviderRequest)
throws NoSuchEntityException, LimitExceededException, InvalidInputException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return tagSAMLProvider(TagSamlProviderRequest.builder().applyMutation(tagSamlProviderRequest).build());
}
/**
*
* Adds one or more tags to an IAM server certificate. If a tag with the same key name already exists, then that tag
* is overwritten with the new value.
*
*
*
* For certificates in a Region supported by Certificate Manager (ACM), we recommend that you don't use IAM server
* certificates. Instead, use ACM to provision, manage, and deploy your server certificates. For more information
* about IAM server certificates, Working with server
* certificates in the IAM User Guide.
*
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only a server certificate that has a specified tag attached. For examples of policies that show how to
* use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
* -
*
* Cost allocation - Use tags to help track which individuals and teams are using which Amazon Web Services
* resources.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* @param tagServerCertificateRequest
* @return Result of the TagServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagServerCertificate
* @see AWS API
* Documentation
*/
default TagServerCertificateResponse tagServerCertificate(TagServerCertificateRequest tagServerCertificateRequest)
throws NoSuchEntityException, InvalidInputException, LimitExceededException, ConcurrentModificationException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an IAM server certificate. If a tag with the same key name already exists, then that tag
* is overwritten with the new value.
*
*
*
* For certificates in a Region supported by Certificate Manager (ACM), we recommend that you don't use IAM server
* certificates. Instead, use ACM to provision, manage, and deploy your server certificates. For more information
* about IAM server certificates, Working with server
* certificates in the IAM User Guide.
*
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only a server certificate that has a specified tag attached. For examples of policies that show how to
* use tags to control access, see Control access using IAM tags in the
* IAM User Guide.
*
*
* -
*
* Cost allocation - Use tags to help track which individuals and teams are using which Amazon Web Services
* resources.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link TagServerCertificateRequest.Builder} avoiding the
* need to create one manually via {@link TagServerCertificateRequest#builder()}
*
*
* @param tagServerCertificateRequest
* A {@link Consumer} that will call methods on {@link TagServerCertificateRequest.Builder} to create a
* request.
* @return Result of the TagServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagServerCertificate
* @see AWS API
* Documentation
*/
default TagServerCertificateResponse tagServerCertificate(
Consumer tagServerCertificateRequest) throws NoSuchEntityException,
InvalidInputException, LimitExceededException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return tagServerCertificate(TagServerCertificateRequest.builder().applyMutation(tagServerCertificateRequest).build());
}
/**
*
* Adds one or more tags to an IAM user. If a tag with the same key name already exists, then that tag is
* overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM requesting user that has a specified tag attached. You can also restrict access to only
* those resources that have a certain tag attached. For examples of policies that show how to use tags to control
* access, see Control access using IAM
* tags in the IAM User Guide.
*
*
* -
*
* Cost allocation - Use tags to help track which individuals and teams are using which Amazon Web Services
* resources.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* For more information about tagging, see Tagging IAM identities in the IAM
* User Guide.
*
*
* @param tagUserRequest
* @return Result of the TagUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagUser
* @see AWS API
* Documentation
*/
default TagUserResponse tagUser(TagUserRequest tagUserRequest) throws NoSuchEntityException, LimitExceededException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an IAM user. If a tag with the same key name already exists, then that tag is
* overwritten with the new value.
*
*
* A tag consists of a key name and an associated value. By assigning tags to your resources, you can do the
* following:
*
*
* -
*
* Administrative grouping and discovery - Attach tags to resources to aid in organization and search. For
* example, you could search for all resources with the key name Project and the value
* MyImportantProject. Or search for all resources with the key name Cost Center and the value
* 41200.
*
*
* -
*
* Access control - Include tags in IAM user-based and resource-based policies. You can use tags to restrict
* access to only an IAM requesting user that has a specified tag attached. You can also restrict access to only
* those resources that have a certain tag attached. For examples of policies that show how to use tags to control
* access, see Control access using IAM
* tags in the IAM User Guide.
*
*
* -
*
* Cost allocation - Use tags to help track which individuals and teams are using which Amazon Web Services
* resources.
*
*
*
*
*
* -
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* -
*
* Amazon Web Services always interprets the tag Value
as a single string. If you need to store an
* array, you can store comma-separated values in the string. However, you must interpret the value in your code.
*
*
*
*
*
* For more information about tagging, see Tagging IAM identities in the IAM
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link TagUserRequest.Builder} avoiding the need to create
* one manually via {@link TagUserRequest#builder()}
*
*
* @param tagUserRequest
* A {@link Consumer} that will call methods on {@link TagUserRequest.Builder} to create a request.
* @return Result of the TagUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.TagUser
* @see AWS API
* Documentation
*/
default TagUserResponse tagUser(Consumer tagUserRequest) throws NoSuchEntityException,
LimitExceededException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return tagUser(TagUserRequest.builder().applyMutation(tagUserRequest).build());
}
/**
*
* Removes the specified tags from the IAM instance profile. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param untagInstanceProfileRequest
* @return Result of the UntagInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagInstanceProfile
* @see AWS API
* Documentation
*/
default UntagInstanceProfileResponse untagInstanceProfile(UntagInstanceProfileRequest untagInstanceProfileRequest)
throws NoSuchEntityException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the IAM instance profile. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link UntagInstanceProfileRequest#builder()}
*
*
* @param untagInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link UntagInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the UntagInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagInstanceProfile
* @see AWS API
* Documentation
*/
default UntagInstanceProfileResponse untagInstanceProfile(
Consumer untagInstanceProfileRequest) throws NoSuchEntityException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return untagInstanceProfile(UntagInstanceProfileRequest.builder().applyMutation(untagInstanceProfileRequest).build());
}
/**
*
* Removes the specified tags from the IAM virtual multi-factor authentication (MFA) device. For more information
* about tagging, see Tagging IAM
* resources in the IAM User Guide.
*
*
* @param untagMfaDeviceRequest
* @return Result of the UntagMFADevice operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagMFADevice
* @see AWS API
* Documentation
*/
default UntagMfaDeviceResponse untagMFADevice(UntagMfaDeviceRequest untagMfaDeviceRequest) throws NoSuchEntityException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the IAM virtual multi-factor authentication (MFA) device. For more information
* about tagging, see Tagging IAM
* resources in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagMfaDeviceRequest.Builder} avoiding the need to
* create one manually via {@link UntagMfaDeviceRequest#builder()}
*
*
* @param untagMfaDeviceRequest
* A {@link Consumer} that will call methods on {@link UntagMFADeviceRequest.Builder} to create a request.
* @return Result of the UntagMFADevice operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagMFADevice
* @see AWS API
* Documentation
*/
default UntagMfaDeviceResponse untagMFADevice(Consumer untagMfaDeviceRequest)
throws NoSuchEntityException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return untagMFADevice(UntagMfaDeviceRequest.builder().applyMutation(untagMfaDeviceRequest).build());
}
/**
*
* Removes the specified tags from the specified OpenID Connect (OIDC)-compatible identity provider in IAM. For more
* information about OIDC providers, see About web identity
* federation. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param untagOpenIdConnectProviderRequest
* @return Result of the UntagOpenIDConnectProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagOpenIDConnectProvider
* @see AWS API Documentation
*/
default UntagOpenIdConnectProviderResponse untagOpenIDConnectProvider(
UntagOpenIdConnectProviderRequest untagOpenIdConnectProviderRequest) throws NoSuchEntityException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the specified OpenID Connect (OIDC)-compatible identity provider in IAM. For more
* information about OIDC providers, see About web identity
* federation. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagOpenIdConnectProviderRequest.Builder} avoiding
* the need to create one manually via {@link UntagOpenIdConnectProviderRequest#builder()}
*
*
* @param untagOpenIdConnectProviderRequest
* A {@link Consumer} that will call methods on {@link UntagOpenIDConnectProviderRequest.Builder} to create a
* request.
* @return Result of the UntagOpenIDConnectProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagOpenIDConnectProvider
* @see AWS API Documentation
*/
default UntagOpenIdConnectProviderResponse untagOpenIDConnectProvider(
Consumer untagOpenIdConnectProviderRequest) throws NoSuchEntityException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return untagOpenIDConnectProvider(UntagOpenIdConnectProviderRequest.builder()
.applyMutation(untagOpenIdConnectProviderRequest).build());
}
/**
*
* Removes the specified tags from the customer managed policy. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param untagPolicyRequest
* @return Result of the UntagPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagPolicy
* @see AWS API
* Documentation
*/
default UntagPolicyResponse untagPolicy(UntagPolicyRequest untagPolicyRequest) throws NoSuchEntityException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the customer managed policy. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagPolicyRequest.Builder} avoiding the need to
* create one manually via {@link UntagPolicyRequest#builder()}
*
*
* @param untagPolicyRequest
* A {@link Consumer} that will call methods on {@link UntagPolicyRequest.Builder} to create a request.
* @return Result of the UntagPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagPolicy
* @see AWS API
* Documentation
*/
default UntagPolicyResponse untagPolicy(Consumer untagPolicyRequest)
throws NoSuchEntityException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return untagPolicy(UntagPolicyRequest.builder().applyMutation(untagPolicyRequest).build());
}
/**
*
* Removes the specified tags from the role. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param untagRoleRequest
* @return Result of the UntagRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagRole
* @see AWS API
* Documentation
*/
default UntagRoleResponse untagRole(UntagRoleRequest untagRoleRequest) throws NoSuchEntityException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the role. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagRoleRequest.Builder} avoiding the need to
* create one manually via {@link UntagRoleRequest#builder()}
*
*
* @param untagRoleRequest
* A {@link Consumer} that will call methods on {@link UntagRoleRequest.Builder} to create a request.
* @return Result of the UntagRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagRole
* @see AWS API
* Documentation
*/
default UntagRoleResponse untagRole(Consumer untagRoleRequest) throws NoSuchEntityException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return untagRole(UntagRoleRequest.builder().applyMutation(untagRoleRequest).build());
}
/**
*
* Removes the specified tags from the specified Security Assertion Markup Language (SAML) identity provider in IAM.
* For more information about these providers, see About web identity
* federation. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param untagSamlProviderRequest
* @return Result of the UntagSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagSAMLProvider
* @see AWS API
* Documentation
*/
default UntagSamlProviderResponse untagSAMLProvider(UntagSamlProviderRequest untagSamlProviderRequest)
throws NoSuchEntityException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the specified Security Assertion Markup Language (SAML) identity provider in IAM.
* For more information about these providers, see About web identity
* federation. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagSamlProviderRequest.Builder} avoiding the need
* to create one manually via {@link UntagSamlProviderRequest#builder()}
*
*
* @param untagSamlProviderRequest
* A {@link Consumer} that will call methods on {@link UntagSAMLProviderRequest.Builder} to create a request.
* @return Result of the UntagSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagSAMLProvider
* @see AWS API
* Documentation
*/
default UntagSamlProviderResponse untagSAMLProvider(Consumer untagSamlProviderRequest)
throws NoSuchEntityException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return untagSAMLProvider(UntagSamlProviderRequest.builder().applyMutation(untagSamlProviderRequest).build());
}
/**
*
* Removes the specified tags from the IAM server certificate. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* For certificates in a Region supported by Certificate Manager (ACM), we recommend that you don't use IAM server
* certificates. Instead, use ACM to provision, manage, and deploy your server certificates. For more information
* about IAM server certificates, Working with server
* certificates in the IAM User Guide.
*
*
*
* @param untagServerCertificateRequest
* @return Result of the UntagServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagServerCertificate
* @see AWS
* API Documentation
*/
default UntagServerCertificateResponse untagServerCertificate(UntagServerCertificateRequest untagServerCertificateRequest)
throws NoSuchEntityException, InvalidInputException, ConcurrentModificationException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the IAM server certificate. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* For certificates in a Region supported by Certificate Manager (ACM), we recommend that you don't use IAM server
* certificates. Instead, use ACM to provision, manage, and deploy your server certificates. For more information
* about IAM server certificates, Working with server
* certificates in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagServerCertificateRequest.Builder} avoiding the
* need to create one manually via {@link UntagServerCertificateRequest#builder()}
*
*
* @param untagServerCertificateRequest
* A {@link Consumer} that will call methods on {@link UntagServerCertificateRequest.Builder} to create a
* request.
* @return Result of the UntagServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagServerCertificate
* @see AWS
* API Documentation
*/
default UntagServerCertificateResponse untagServerCertificate(
Consumer untagServerCertificateRequest) throws NoSuchEntityException,
InvalidInputException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return untagServerCertificate(UntagServerCertificateRequest.builder().applyMutation(untagServerCertificateRequest)
.build());
}
/**
*
* Removes the specified tags from the user. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* @param untagUserRequest
* @return Result of the UntagUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagUser
* @see AWS API
* Documentation
*/
default UntagUserResponse untagUser(UntagUserRequest untagUserRequest) throws NoSuchEntityException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the user. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagUserRequest.Builder} avoiding the need to
* create one manually via {@link UntagUserRequest#builder()}
*
*
* @param untagUserRequest
* A {@link Consumer} that will call methods on {@link UntagUserRequest.Builder} to create a request.
* @return Result of the UntagUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UntagUser
* @see AWS API
* Documentation
*/
default UntagUserResponse untagUser(Consumer untagUserRequest) throws NoSuchEntityException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return untagUser(UntagUserRequest.builder().applyMutation(untagUserRequest).build());
}
/**
*
* Changes the status of the specified access key from Active to Inactive, or vice versa. This operation can be used
* to disable a user's key as part of a key rotation workflow.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
* For information about rotating keys, see Managing keys and
* certificates in the IAM User Guide.
*
*
* @param updateAccessKeyRequest
* @return Result of the UpdateAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateAccessKey
* @see AWS API
* Documentation
*/
default UpdateAccessKeyResponse updateAccessKey(UpdateAccessKeyRequest updateAccessKeyRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Changes the status of the specified access key from Active to Inactive, or vice versa. This operation can be used
* to disable a user's key as part of a key rotation workflow.
*
*
* If the UserName
is not specified, the user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. If a temporary access key is used, then UserName
is
* required. If a long-term key is assigned to the user, then UserName
is not required. This operation
* works for access keys under the Amazon Web Services account. Consequently, you can use this operation to manage
* Amazon Web Services account root user credentials even if the Amazon Web Services account has no associated
* users.
*
*
* For information about rotating keys, see Managing keys and
* certificates in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAccessKeyRequest.Builder} avoiding the need
* to create one manually via {@link UpdateAccessKeyRequest#builder()}
*
*
* @param updateAccessKeyRequest
* A {@link Consumer} that will call methods on {@link UpdateAccessKeyRequest.Builder} to create a request.
* @return Result of the UpdateAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateAccessKey
* @see AWS API
* Documentation
*/
default UpdateAccessKeyResponse updateAccessKey(Consumer updateAccessKeyRequest)
throws NoSuchEntityException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return updateAccessKey(UpdateAccessKeyRequest.builder().applyMutation(updateAccessKeyRequest).build());
}
/**
*
* Updates the password policy settings for the Amazon Web Services account.
*
*
*
* This operation does not support partial updates. No parameters are required, but if you do not specify a
* parameter, that parameter's value reverts to its default value. See the Request Parameters section for
* each parameter's default value. Also note that some parameters do not allow the default parameter to be
* explicitly set. Instead, to invoke the default value, do not include that parameter when you invoke the
* operation.
*
*
*
* For more information about using a password policy, see Managing an IAM
* password policy in the IAM User Guide.
*
*
* @return Result of the UpdateAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateAccountPasswordPolicy
* @see #updateAccountPasswordPolicy(UpdateAccountPasswordPolicyRequest)
* @see AWS API Documentation
*/
default UpdateAccountPasswordPolicyResponse updateAccountPasswordPolicy() throws NoSuchEntityException,
MalformedPolicyDocumentException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return updateAccountPasswordPolicy(UpdateAccountPasswordPolicyRequest.builder().build());
}
/**
*
* Updates the password policy settings for the Amazon Web Services account.
*
*
*
* This operation does not support partial updates. No parameters are required, but if you do not specify a
* parameter, that parameter's value reverts to its default value. See the Request Parameters section for
* each parameter's default value. Also note that some parameters do not allow the default parameter to be
* explicitly set. Instead, to invoke the default value, do not include that parameter when you invoke the
* operation.
*
*
*
* For more information about using a password policy, see Managing an IAM
* password policy in the IAM User Guide.
*
*
* @param updateAccountPasswordPolicyRequest
* @return Result of the UpdateAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateAccountPasswordPolicy
* @see AWS API Documentation
*/
default UpdateAccountPasswordPolicyResponse updateAccountPasswordPolicy(
UpdateAccountPasswordPolicyRequest updateAccountPasswordPolicyRequest) throws NoSuchEntityException,
MalformedPolicyDocumentException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the password policy settings for the Amazon Web Services account.
*
*
*
* This operation does not support partial updates. No parameters are required, but if you do not specify a
* parameter, that parameter's value reverts to its default value. See the Request Parameters section for
* each parameter's default value. Also note that some parameters do not allow the default parameter to be
* explicitly set. Instead, to invoke the default value, do not include that parameter when you invoke the
* operation.
*
*
*
* For more information about using a password policy, see Managing an IAM
* password policy in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAccountPasswordPolicyRequest.Builder}
* avoiding the need to create one manually via {@link UpdateAccountPasswordPolicyRequest#builder()}
*
*
* @param updateAccountPasswordPolicyRequest
* A {@link Consumer} that will call methods on {@link UpdateAccountPasswordPolicyRequest.Builder} to create
* a request.
* @return Result of the UpdateAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateAccountPasswordPolicy
* @see AWS API Documentation
*/
default UpdateAccountPasswordPolicyResponse updateAccountPasswordPolicy(
Consumer updateAccountPasswordPolicyRequest)
throws NoSuchEntityException, MalformedPolicyDocumentException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return updateAccountPasswordPolicy(UpdateAccountPasswordPolicyRequest.builder()
.applyMutation(updateAccountPasswordPolicyRequest).build());
}
/**
*
* Updates the policy that grants an IAM entity permission to assume a role. This is typically referred to as the
* "role trust policy". For more information about roles, see Using roles to delegate permissions
* and federate identities.
*
*
* @param updateAssumeRolePolicyRequest
* @return Result of the UpdateAssumeRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateAssumeRolePolicy
* @see AWS
* API Documentation
*/
default UpdateAssumeRolePolicyResponse updateAssumeRolePolicy(UpdateAssumeRolePolicyRequest updateAssumeRolePolicyRequest)
throws NoSuchEntityException, MalformedPolicyDocumentException, LimitExceededException, UnmodifiableEntityException,
ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the policy that grants an IAM entity permission to assume a role. This is typically referred to as the
* "role trust policy". For more information about roles, see Using roles to delegate permissions
* and federate identities.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAssumeRolePolicyRequest.Builder} avoiding the
* need to create one manually via {@link UpdateAssumeRolePolicyRequest#builder()}
*
*
* @param updateAssumeRolePolicyRequest
* A {@link Consumer} that will call methods on {@link UpdateAssumeRolePolicyRequest.Builder} to create a
* request.
* @return Result of the UpdateAssumeRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateAssumeRolePolicy
* @see AWS
* API Documentation
*/
default UpdateAssumeRolePolicyResponse updateAssumeRolePolicy(
Consumer updateAssumeRolePolicyRequest) throws NoSuchEntityException,
MalformedPolicyDocumentException, LimitExceededException, UnmodifiableEntityException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return updateAssumeRolePolicy(UpdateAssumeRolePolicyRequest.builder().applyMutation(updateAssumeRolePolicyRequest)
.build());
}
/**
*
* Updates the name and/or the path of the specified IAM group.
*
*
*
* You should understand the implications of changing a group's path or name. For more information, see Renaming users and
* groups in the IAM User Guide.
*
*
*
* The person making the request (the principal), must have permission to change the role group with the old name
* and the new name. For example, to change the group named Managers
to MGRs
, the
* principal must have a policy that allows them to update both groups. If the principal has permission to update
* the Managers
group, but not the MGRs
group, then the update fails. For more information
* about permissions, see Access
* management.
*
*
*
* @param updateGroupRequest
* @return Result of the UpdateGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateGroup
* @see AWS API
* Documentation
*/
default UpdateGroupResponse updateGroup(UpdateGroupRequest updateGroupRequest) throws NoSuchEntityException,
EntityAlreadyExistsException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the name and/or the path of the specified IAM group.
*
*
*
* You should understand the implications of changing a group's path or name. For more information, see Renaming users and
* groups in the IAM User Guide.
*
*
*
* The person making the request (the principal), must have permission to change the role group with the old name
* and the new name. For example, to change the group named Managers
to MGRs
, the
* principal must have a policy that allows them to update both groups. If the principal has permission to update
* the Managers
group, but not the MGRs
group, then the update fails. For more information
* about permissions, see Access
* management.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGroupRequest.Builder} avoiding the need to
* create one manually via {@link UpdateGroupRequest#builder()}
*
*
* @param updateGroupRequest
* A {@link Consumer} that will call methods on {@link UpdateGroupRequest.Builder} to create a request.
* @return Result of the UpdateGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateGroup
* @see AWS API
* Documentation
*/
default UpdateGroupResponse updateGroup(Consumer updateGroupRequest)
throws NoSuchEntityException, EntityAlreadyExistsException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return updateGroup(UpdateGroupRequest.builder().applyMutation(updateGroupRequest).build());
}
/**
*
* Changes the password for the specified IAM user. You can use the CLI, the Amazon Web Services API, or the
* Users page in the IAM console to change the password for any IAM user. Use ChangePassword to change
* your own password in the My Security Credentials page in the Amazon Web Services Management Console.
*
*
* For more information about modifying passwords, see Managing passwords in the
* IAM User Guide.
*
*
* @param updateLoginProfileRequest
* @return Result of the UpdateLoginProfile operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateLoginProfile
* @see AWS API
* Documentation
*/
default UpdateLoginProfileResponse updateLoginProfile(UpdateLoginProfileRequest updateLoginProfileRequest)
throws EntityTemporarilyUnmodifiableException, NoSuchEntityException, PasswordPolicyViolationException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Changes the password for the specified IAM user. You can use the CLI, the Amazon Web Services API, or the
* Users page in the IAM console to change the password for any IAM user. Use ChangePassword to change
* your own password in the My Security Credentials page in the Amazon Web Services Management Console.
*
*
* For more information about modifying passwords, see Managing passwords in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateLoginProfileRequest.Builder} avoiding the
* need to create one manually via {@link UpdateLoginProfileRequest#builder()}
*
*
* @param updateLoginProfileRequest
* A {@link Consumer} that will call methods on {@link UpdateLoginProfileRequest.Builder} to create a
* request.
* @return Result of the UpdateLoginProfile operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateLoginProfile
* @see AWS API
* Documentation
*/
default UpdateLoginProfileResponse updateLoginProfile(Consumer updateLoginProfileRequest)
throws EntityTemporarilyUnmodifiableException, NoSuchEntityException, PasswordPolicyViolationException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return updateLoginProfile(UpdateLoginProfileRequest.builder().applyMutation(updateLoginProfileRequest).build());
}
/**
*
* Replaces the existing list of server certificate thumbprints associated with an OpenID Connect (OIDC) provider
* resource object with a new list of thumbprints.
*
*
* The list that you pass with this operation completely replaces the existing list of thumbprints. (The lists are
* not merged.)
*
*
* Typically, you need to update a thumbprint only when the identity provider certificate changes, which occurs
* rarely. However, if the provider's certificate does change, any attempt to assume an IAM role that
* specifies the OIDC provider as a principal fails until the certificate thumbprint is updated.
*
*
*
* Amazon Web Services secures communication with some OIDC identity providers (IdPs) through our library of trusted
* certificate authorities (CAs) instead of using a certificate thumbprint to verify your IdP server certificate.
* These OIDC IdPs include Google, Auth0, and those that use an Amazon S3 bucket to host a JSON Web Key Set (JWKS)
* endpoint. In these cases, your legacy thumbprint remains in your configuration, but is no longer used for
* validation.
*
*
*
* Trust for the OIDC provider is derived from the provider certificate and is validated by the thumbprint.
* Therefore, it is best to limit access to the UpdateOpenIDConnectProviderThumbprint
operation to
* highly privileged users.
*
*
*
* @param updateOpenIdConnectProviderThumbprintRequest
* @return Result of the UpdateOpenIDConnectProviderThumbprint operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateOpenIDConnectProviderThumbprint
* @see AWS API Documentation
*/
default UpdateOpenIdConnectProviderThumbprintResponse updateOpenIDConnectProviderThumbprint(
UpdateOpenIdConnectProviderThumbprintRequest updateOpenIdConnectProviderThumbprintRequest)
throws InvalidInputException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Replaces the existing list of server certificate thumbprints associated with an OpenID Connect (OIDC) provider
* resource object with a new list of thumbprints.
*
*
* The list that you pass with this operation completely replaces the existing list of thumbprints. (The lists are
* not merged.)
*
*
* Typically, you need to update a thumbprint only when the identity provider certificate changes, which occurs
* rarely. However, if the provider's certificate does change, any attempt to assume an IAM role that
* specifies the OIDC provider as a principal fails until the certificate thumbprint is updated.
*
*
*
* Amazon Web Services secures communication with some OIDC identity providers (IdPs) through our library of trusted
* certificate authorities (CAs) instead of using a certificate thumbprint to verify your IdP server certificate.
* These OIDC IdPs include Google, Auth0, and those that use an Amazon S3 bucket to host a JSON Web Key Set (JWKS)
* endpoint. In these cases, your legacy thumbprint remains in your configuration, but is no longer used for
* validation.
*
*
*
* Trust for the OIDC provider is derived from the provider certificate and is validated by the thumbprint.
* Therefore, it is best to limit access to the UpdateOpenIDConnectProviderThumbprint
operation to
* highly privileged users.
*
*
*
* This is a convenience which creates an instance of the
* {@link UpdateOpenIdConnectProviderThumbprintRequest.Builder} avoiding the need to create one manually via
* {@link UpdateOpenIdConnectProviderThumbprintRequest#builder()}
*
*
* @param updateOpenIdConnectProviderThumbprintRequest
* A {@link Consumer} that will call methods on {@link UpdateOpenIDConnectProviderThumbprintRequest.Builder}
* to create a request.
* @return Result of the UpdateOpenIDConnectProviderThumbprint operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateOpenIDConnectProviderThumbprint
* @see AWS API Documentation
*/
default UpdateOpenIdConnectProviderThumbprintResponse updateOpenIDConnectProviderThumbprint(
Consumer updateOpenIdConnectProviderThumbprintRequest)
throws InvalidInputException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return updateOpenIDConnectProviderThumbprint(UpdateOpenIdConnectProviderThumbprintRequest.builder()
.applyMutation(updateOpenIdConnectProviderThumbprintRequest).build());
}
/**
*
* Updates the description or maximum session duration setting of a role.
*
*
* @param updateRoleRequest
* @return Result of the UpdateRole operation returned by the service.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateRole
* @see AWS API
* Documentation
*/
default UpdateRoleResponse updateRole(UpdateRoleRequest updateRoleRequest) throws UnmodifiableEntityException,
NoSuchEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the description or maximum session duration setting of a role.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateRoleRequest.Builder} avoiding the need to
* create one manually via {@link UpdateRoleRequest#builder()}
*
*
* @param updateRoleRequest
* A {@link Consumer} that will call methods on {@link UpdateRoleRequest.Builder} to create a request.
* @return Result of the UpdateRole operation returned by the service.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateRole
* @see AWS API
* Documentation
*/
default UpdateRoleResponse updateRole(Consumer updateRoleRequest)
throws UnmodifiableEntityException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return updateRole(UpdateRoleRequest.builder().applyMutation(updateRoleRequest).build());
}
/**
*
* Use UpdateRole instead.
*
*
* Modifies only the description of a role. This operation performs the same function as the
* Description
parameter in the UpdateRole
operation.
*
*
* @param updateRoleDescriptionRequest
* @return Result of the UpdateRoleDescription operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateRoleDescription
* @see AWS API
* Documentation
*/
default UpdateRoleDescriptionResponse updateRoleDescription(UpdateRoleDescriptionRequest updateRoleDescriptionRequest)
throws NoSuchEntityException, UnmodifiableEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Use UpdateRole instead.
*
*
* Modifies only the description of a role. This operation performs the same function as the
* Description
parameter in the UpdateRole
operation.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateRoleDescriptionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateRoleDescriptionRequest#builder()}
*
*
* @param updateRoleDescriptionRequest
* A {@link Consumer} that will call methods on {@link UpdateRoleDescriptionRequest.Builder} to create a
* request.
* @return Result of the UpdateRoleDescription operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws UnmodifiableEntityException
* The request was rejected because only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateRoleDescription
* @see AWS API
* Documentation
*/
default UpdateRoleDescriptionResponse updateRoleDescription(
Consumer updateRoleDescriptionRequest) throws NoSuchEntityException,
UnmodifiableEntityException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return updateRoleDescription(UpdateRoleDescriptionRequest.builder().applyMutation(updateRoleDescriptionRequest).build());
}
/**
*
* Updates the metadata document for an existing SAML provider resource object.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param updateSamlProviderRequest
* @return Result of the UpdateSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateSAMLProvider
* @see AWS API
* Documentation
*/
default UpdateSamlProviderResponse updateSAMLProvider(UpdateSamlProviderRequest updateSamlProviderRequest)
throws NoSuchEntityException, InvalidInputException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the metadata document for an existing SAML provider resource object.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSamlProviderRequest.Builder} avoiding the
* need to create one manually via {@link UpdateSamlProviderRequest#builder()}
*
*
* @param updateSamlProviderRequest
* A {@link Consumer} that will call methods on {@link UpdateSAMLProviderRequest.Builder} to create a
* request.
* @return Result of the UpdateSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateSAMLProvider
* @see AWS API
* Documentation
*/
default UpdateSamlProviderResponse updateSAMLProvider(Consumer updateSamlProviderRequest)
throws NoSuchEntityException, InvalidInputException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
return updateSAMLProvider(UpdateSamlProviderRequest.builder().applyMutation(updateSamlProviderRequest).build());
}
/**
*
* Sets the status of an IAM user's SSH public key to active or inactive. SSH public keys that are inactive cannot
* be used for authentication. This operation can be used to disable a user's SSH public key as part of a key
* rotation work flow.
*
*
* The SSH public key affected by this operation is used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
* @param updateSshPublicKeyRequest
* @return Result of the UpdateSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateSSHPublicKey
* @see AWS API
* Documentation
*/
default UpdateSshPublicKeyResponse updateSSHPublicKey(UpdateSshPublicKeyRequest updateSshPublicKeyRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the status of an IAM user's SSH public key to active or inactive. SSH public keys that are inactive cannot
* be used for authentication. This operation can be used to disable a user's SSH public key as part of a key
* rotation work flow.
*
*
* The SSH public key affected by this operation is used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSshPublicKeyRequest.Builder} avoiding the
* need to create one manually via {@link UpdateSshPublicKeyRequest#builder()}
*
*
* @param updateSshPublicKeyRequest
* A {@link Consumer} that will call methods on {@link UpdateSSHPublicKeyRequest.Builder} to create a
* request.
* @return Result of the UpdateSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateSSHPublicKey
* @see AWS API
* Documentation
*/
default UpdateSshPublicKeyResponse updateSSHPublicKey(Consumer updateSshPublicKeyRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
return updateSSHPublicKey(UpdateSshPublicKeyRequest.builder().applyMutation(updateSshPublicKeyRequest).build());
}
/**
*
* Updates the name and/or the path of the specified server certificate stored in IAM.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* You should understand the implications of changing a server certificate's path or name. For more information, see
* Renaming a server certificate in the IAM User Guide.
*
*
*
* The person making the request (the principal), must have permission to change the server certificate with the old
* name and the new name. For example, to change the certificate named ProductionCert
to
* ProdCert
, the principal must have a policy that allows them to update both certificates. If the
* principal has permission to update the ProductionCert
group, but not the ProdCert
* certificate, then the update fails. For more information about permissions, see Access management in the IAM User
* Guide.
*
*
*
* @param updateServerCertificateRequest
* @return Result of the UpdateServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateServerCertificate
* @see AWS
* API Documentation
*/
default UpdateServerCertificateResponse updateServerCertificate(UpdateServerCertificateRequest updateServerCertificateRequest)
throws NoSuchEntityException, EntityAlreadyExistsException, LimitExceededException, ServiceFailureException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the name and/or the path of the specified server certificate stored in IAM.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic also includes a list of Amazon Web Services services
* that can use the server certificates that you manage with IAM.
*
*
*
* You should understand the implications of changing a server certificate's path or name. For more information, see
* Renaming a server certificate in the IAM User Guide.
*
*
*
* The person making the request (the principal), must have permission to change the server certificate with the old
* name and the new name. For example, to change the certificate named ProductionCert
to
* ProdCert
, the principal must have a policy that allows them to update both certificates. If the
* principal has permission to update the ProductionCert
group, but not the ProdCert
* certificate, then the update fails. For more information about permissions, see Access management in the IAM User
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateServerCertificateRequest.Builder} avoiding
* the need to create one manually via {@link UpdateServerCertificateRequest#builder()}
*
*
* @param updateServerCertificateRequest
* A {@link Consumer} that will call methods on {@link UpdateServerCertificateRequest.Builder} to create a
* request.
* @return Result of the UpdateServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateServerCertificate
* @see AWS
* API Documentation
*/
default UpdateServerCertificateResponse updateServerCertificate(
Consumer updateServerCertificateRequest) throws NoSuchEntityException,
EntityAlreadyExistsException, LimitExceededException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return updateServerCertificate(UpdateServerCertificateRequest.builder().applyMutation(updateServerCertificateRequest)
.build());
}
/**
*
* Sets the status of a service-specific credential to Active
or Inactive
.
* Service-specific credentials that are inactive cannot be used for authentication to the service. This operation
* can be used to disable a user's service-specific credential as part of a credential rotation work flow.
*
*
* @param updateServiceSpecificCredentialRequest
* @return Result of the UpdateServiceSpecificCredential operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateServiceSpecificCredential
* @see AWS API Documentation
*/
default UpdateServiceSpecificCredentialResponse updateServiceSpecificCredential(
UpdateServiceSpecificCredentialRequest updateServiceSpecificCredentialRequest) throws NoSuchEntityException,
AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Sets the status of a service-specific credential to Active
or Inactive
.
* Service-specific credentials that are inactive cannot be used for authentication to the service. This operation
* can be used to disable a user's service-specific credential as part of a credential rotation work flow.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateServiceSpecificCredentialRequest.Builder}
* avoiding the need to create one manually via {@link UpdateServiceSpecificCredentialRequest#builder()}
*
*
* @param updateServiceSpecificCredentialRequest
* A {@link Consumer} that will call methods on {@link UpdateServiceSpecificCredentialRequest.Builder} to
* create a request.
* @return Result of the UpdateServiceSpecificCredential operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateServiceSpecificCredential
* @see AWS API Documentation
*/
default UpdateServiceSpecificCredentialResponse updateServiceSpecificCredential(
Consumer updateServiceSpecificCredentialRequest)
throws NoSuchEntityException, AwsServiceException, SdkClientException, IamException {
return updateServiceSpecificCredential(UpdateServiceSpecificCredentialRequest.builder()
.applyMutation(updateServiceSpecificCredentialRequest).build());
}
/**
*
* Changes the status of the specified user signing certificate from active to disabled, or vice versa. This
* operation can be used to disable an IAM user's signing certificate as part of a certificate rotation work flow.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request. This operation works for access keys under the Amazon Web
* Services account. Consequently, you can use this operation to manage Amazon Web Services account root user
* credentials even if the Amazon Web Services account has no associated users.
*
*
* @param updateSigningCertificateRequest
* @return Result of the UpdateSigningCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateSigningCertificate
* @see AWS
* API Documentation
*/
default UpdateSigningCertificateResponse updateSigningCertificate(
UpdateSigningCertificateRequest updateSigningCertificateRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Changes the status of the specified user signing certificate from active to disabled, or vice versa. This
* operation can be used to disable an IAM user's signing certificate as part of a certificate rotation work flow.
*
*
* If the UserName
field is not specified, the user name is determined implicitly based on the Amazon
* Web Services access key ID used to sign the request. This operation works for access keys under the Amazon Web
* Services account. Consequently, you can use this operation to manage Amazon Web Services account root user
* credentials even if the Amazon Web Services account has no associated users.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSigningCertificateRequest.Builder} avoiding
* the need to create one manually via {@link UpdateSigningCertificateRequest#builder()}
*
*
* @param updateSigningCertificateRequest
* A {@link Consumer} that will call methods on {@link UpdateSigningCertificateRequest.Builder} to create a
* request.
* @return Result of the UpdateSigningCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateSigningCertificate
* @see AWS
* API Documentation
*/
default UpdateSigningCertificateResponse updateSigningCertificate(
Consumer updateSigningCertificateRequest) throws NoSuchEntityException,
LimitExceededException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return updateSigningCertificate(UpdateSigningCertificateRequest.builder().applyMutation(updateSigningCertificateRequest)
.build());
}
/**
*
* Updates the name and/or the path of the specified IAM user.
*
*
*
* You should understand the implications of changing an IAM user's path or name. For more information, see Renaming an IAM
* user and Renaming an
* IAM group in the IAM User Guide.
*
*
*
* To change a user name, the requester must have appropriate permissions on both the source object and the target
* object. For example, to change Bob to Robert, the entity making the request must have permission on Bob and
* Robert, or must have permission on all (*). For more information about permissions, see Permissions and policies.
*
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateUser
* @see AWS API
* Documentation
*/
default UpdateUserResponse updateUser(UpdateUserRequest updateUserRequest) throws NoSuchEntityException,
LimitExceededException, EntityAlreadyExistsException, EntityTemporarilyUnmodifiableException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the name and/or the path of the specified IAM user.
*
*
*
* You should understand the implications of changing an IAM user's path or name. For more information, see Renaming an IAM
* user and Renaming an
* IAM group in the IAM User Guide.
*
*
*
* To change a user name, the requester must have appropriate permissions on both the source object and the target
* object. For example, to change Bob to Robert, the entity making the request must have permission on Bob and
* Robert, or must have permission on all (*). For more information about permissions, see Permissions and policies.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUserRequest#builder()}
*
*
* @param updateUserRequest
* A {@link Consumer} that will call methods on {@link UpdateUserRequest.Builder} to create a request.
* @return Result of the UpdateUser operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UpdateUser
* @see AWS API
* Documentation
*/
default UpdateUserResponse updateUser(Consumer updateUserRequest) throws NoSuchEntityException,
LimitExceededException, EntityAlreadyExistsException, EntityTemporarilyUnmodifiableException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return updateUser(UpdateUserRequest.builder().applyMutation(updateUserRequest).build());
}
/**
*
* Uploads an SSH public key and associates it with the specified IAM user.
*
*
* The SSH public key uploaded by this operation can be used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
* @param uploadSshPublicKeyRequest
* @return Result of the UploadSSHPublicKey operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidPublicKeyException
* The request was rejected because the public key is malformed or otherwise invalid.
* @throws DuplicateSshPublicKeyException
* The request was rejected because the SSH public key is already associated with the specified IAM user.
* @throws UnrecognizedPublicKeyEncodingException
* The request was rejected because the public key encoding format is unsupported or unrecognized.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UploadSSHPublicKey
* @see AWS API
* Documentation
*/
default UploadSshPublicKeyResponse uploadSSHPublicKey(UploadSshPublicKeyRequest uploadSshPublicKeyRequest)
throws LimitExceededException, NoSuchEntityException, InvalidPublicKeyException, DuplicateSshPublicKeyException,
UnrecognizedPublicKeyEncodingException, AwsServiceException, SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an SSH public key and associates it with the specified IAM user.
*
*
* The SSH public key uploaded by this operation can be used only for authenticating the associated IAM user to an
* CodeCommit repository. For more information about using SSH keys to authenticate to an CodeCommit repository, see
* Set up
* CodeCommit for SSH connections in the CodeCommit User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UploadSshPublicKeyRequest.Builder} avoiding the
* need to create one manually via {@link UploadSshPublicKeyRequest#builder()}
*
*
* @param uploadSshPublicKeyRequest
* A {@link Consumer} that will call methods on {@link UploadSSHPublicKeyRequest.Builder} to create a
* request.
* @return Result of the UploadSSHPublicKey operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws InvalidPublicKeyException
* The request was rejected because the public key is malformed or otherwise invalid.
* @throws DuplicateSshPublicKeyException
* The request was rejected because the SSH public key is already associated with the specified IAM user.
* @throws UnrecognizedPublicKeyEncodingException
* The request was rejected because the public key encoding format is unsupported or unrecognized.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UploadSSHPublicKey
* @see AWS API
* Documentation
*/
default UploadSshPublicKeyResponse uploadSSHPublicKey(Consumer uploadSshPublicKeyRequest)
throws LimitExceededException, NoSuchEntityException, InvalidPublicKeyException, DuplicateSshPublicKeyException,
UnrecognizedPublicKeyEncodingException, AwsServiceException, SdkClientException, IamException {
return uploadSSHPublicKey(UploadSshPublicKeyRequest.builder().applyMutation(uploadSshPublicKeyRequest).build());
}
/**
*
* Uploads a server certificate entity for the Amazon Web Services account. The server certificate entity includes a
* public key certificate, a private key, and an optional certificate chain, which should all be PEM-encoded.
*
*
* We recommend that you use Certificate Manager to provision,
* manage, and deploy your server certificates. With ACM you can request a certificate, deploy it to Amazon Web
* Services resources, and let ACM handle certificate renewals for you. Certificates provided by ACM are free. For
* more information about using ACM, see the Certificate
* Manager User Guide.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic includes a list of Amazon Web Services services that
* can use the server certificates that you manage with IAM.
*
*
* For information about the number of server certificates you can upload, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because the body of the public key certificate, private key, and the certificate chain can be large, you should
* use POST rather than GET when calling UploadServerCertificate
. For information about setting up
* signatures and authorization through the API, see Signing Amazon Web Services
* API requests in the Amazon Web Services General Reference. For general information about using the
* Query API with IAM, see Calling the
* API by making HTTP query requests in the IAM User Guide.
*
*
*
* @param uploadServerCertificateRequest
* @return Result of the UploadServerCertificate operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedCertificateException
* The request was rejected because the certificate was malformed or expired. The error message describes
* the specific error.
* @throws KeyPairMismatchException
* The request was rejected because the public key certificate and the private key do not match.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UploadServerCertificate
* @see AWS
* API Documentation
*/
default UploadServerCertificateResponse uploadServerCertificate(UploadServerCertificateRequest uploadServerCertificateRequest)
throws LimitExceededException, InvalidInputException, EntityAlreadyExistsException, MalformedCertificateException,
KeyPairMismatchException, ConcurrentModificationException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads a server certificate entity for the Amazon Web Services account. The server certificate entity includes a
* public key certificate, a private key, and an optional certificate chain, which should all be PEM-encoded.
*
*
* We recommend that you use Certificate Manager to provision,
* manage, and deploy your server certificates. With ACM you can request a certificate, deploy it to Amazon Web
* Services resources, and let ACM handle certificate renewals for you. Certificates provided by ACM are free. For
* more information about using ACM, see the Certificate
* Manager User Guide.
*
*
* For more information about working with server certificates, see Working with server
* certificates in the IAM User Guide. This topic includes a list of Amazon Web Services services that
* can use the server certificates that you manage with IAM.
*
*
* For information about the number of server certificates you can upload, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* Because the body of the public key certificate, private key, and the certificate chain can be large, you should
* use POST rather than GET when calling UploadServerCertificate
. For information about setting up
* signatures and authorization through the API, see Signing Amazon Web Services
* API requests in the Amazon Web Services General Reference. For general information about using the
* Query API with IAM, see Calling the
* API by making HTTP query requests in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UploadServerCertificateRequest.Builder} avoiding
* the need to create one manually via {@link UploadServerCertificateRequest#builder()}
*
*
* @param uploadServerCertificateRequest
* A {@link Consumer} that will call methods on {@link UploadServerCertificateRequest.Builder} to create a
* request.
* @return Result of the UploadServerCertificate operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedCertificateException
* The request was rejected because the certificate was malformed or expired. The error message describes
* the specific error.
* @throws KeyPairMismatchException
* The request was rejected because the public key certificate and the private key do not match.
* @throws ConcurrentModificationException
* The request was rejected because multiple requests to change this object were submitted simultaneously.
* Wait a few minutes and submit your request again.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UploadServerCertificate
* @see AWS
* API Documentation
*/
default UploadServerCertificateResponse uploadServerCertificate(
Consumer uploadServerCertificateRequest) throws LimitExceededException,
InvalidInputException, EntityAlreadyExistsException, MalformedCertificateException, KeyPairMismatchException,
ConcurrentModificationException, ServiceFailureException, AwsServiceException, SdkClientException, IamException {
return uploadServerCertificate(UploadServerCertificateRequest.builder().applyMutation(uploadServerCertificateRequest)
.build());
}
/**
*
* Uploads an X.509 signing certificate and associates it with the specified IAM user. Some Amazon Web Services
* services require you to use certificates to validate requests that are signed with a corresponding private key.
* When you upload the certificate, its default status is Active
.
*
*
* For information about when you would use an X.509 signing certificate, see Managing server
* certificates in IAM in the IAM User Guide.
*
*
* If the UserName
is not specified, the IAM user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. This operation works for access keys under the Amazon Web
* Services account. Consequently, you can use this operation to manage Amazon Web Services account root user
* credentials even if the Amazon Web Services account has no associated users.
*
*
*
* Because the body of an X.509 certificate can be large, you should use POST rather than GET when calling
* UploadSigningCertificate
. For information about setting up signatures and authorization through the
* API, see Signing Amazon Web
* Services API requests in the Amazon Web Services General Reference. For general information about
* using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* @param uploadSigningCertificateRequest
* @return Result of the UploadSigningCertificate operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedCertificateException
* The request was rejected because the certificate was malformed or expired. The error message describes
* the specific error.
* @throws InvalidCertificateException
* The request was rejected because the certificate is invalid.
* @throws DuplicateCertificateException
* The request was rejected because the same certificate is associated with an IAM user in the account.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UploadSigningCertificate
* @see AWS
* API Documentation
*/
default UploadSigningCertificateResponse uploadSigningCertificate(
UploadSigningCertificateRequest uploadSigningCertificateRequest) throws LimitExceededException,
EntityAlreadyExistsException, MalformedCertificateException, InvalidCertificateException,
DuplicateCertificateException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an X.509 signing certificate and associates it with the specified IAM user. Some Amazon Web Services
* services require you to use certificates to validate requests that are signed with a corresponding private key.
* When you upload the certificate, its default status is Active
.
*
*
* For information about when you would use an X.509 signing certificate, see Managing server
* certificates in IAM in the IAM User Guide.
*
*
* If the UserName
is not specified, the IAM user name is determined implicitly based on the Amazon Web
* Services access key ID used to sign the request. This operation works for access keys under the Amazon Web
* Services account. Consequently, you can use this operation to manage Amazon Web Services account root user
* credentials even if the Amazon Web Services account has no associated users.
*
*
*
* Because the body of an X.509 certificate can be large, you should use POST rather than GET when calling
* UploadSigningCertificate
. For information about setting up signatures and authorization through the
* API, see Signing Amazon Web
* Services API requests in the Amazon Web Services General Reference. For general information about
* using the Query API with IAM, see Making query requests in the
* IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UploadSigningCertificateRequest.Builder} avoiding
* the need to create one manually via {@link UploadSigningCertificateRequest#builder()}
*
*
* @param uploadSigningCertificateRequest
* A {@link Consumer} that will call methods on {@link UploadSigningCertificateRequest.Builder} to create a
* request.
* @return Result of the UploadSigningCertificate operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current Amazon Web Services
* account limits. The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedCertificateException
* The request was rejected because the certificate was malformed or expired. The error message describes
* the specific error.
* @throws InvalidCertificateException
* The request was rejected because the certificate is invalid.
* @throws DuplicateCertificateException
* The request was rejected because the same certificate is associated with an IAM user in the account.
* @throws NoSuchEntityException
* The request was rejected because it referenced a resource entity that does not exist. The error message
* describes the resource.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IamClient.UploadSigningCertificate
* @see AWS
* API Documentation
*/
default UploadSigningCertificateResponse uploadSigningCertificate(
Consumer uploadSigningCertificateRequest) throws LimitExceededException,
EntityAlreadyExistsException, MalformedCertificateException, InvalidCertificateException,
DuplicateCertificateException, NoSuchEntityException, ServiceFailureException, AwsServiceException,
SdkClientException, IamException {
return uploadSigningCertificate(UploadSigningCertificateRequest.builder().applyMutation(uploadSigningCertificateRequest)
.build());
}
/**
* Create an instance of {@link IamWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link IamWaiter}
*/
default IamWaiter waiter() {
throw new UnsupportedOperationException();
}
/**
* Create a builder that can be used to configure and create a {@link IamClient}.
*/
static IamClientBuilder builder() {
return new DefaultIamClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}