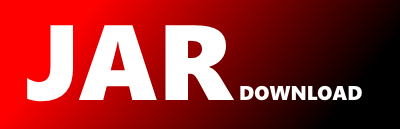
software.amazon.awssdk.services.iam.model.GenerateServiceLastAccessedDetailsRequest Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GenerateServiceLastAccessedDetailsRequest extends IamRequest implements
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(GenerateServiceLastAccessedDetailsRequest::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField GRANULARITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Granularity").getter(getter(GenerateServiceLastAccessedDetailsRequest::granularityAsString))
.setter(setter(Builder::granularity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Granularity").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, GRANULARITY_FIELD));
private final String arn;
private final String granularity;
private GenerateServiceLastAccessedDetailsRequest(BuilderImpl builder) {
super(builder);
this.arn = builder.arn;
this.granularity = builder.granularity;
}
/**
*
* The ARN of the IAM resource (user, group, role, or managed policy) used to generate information about when the
* resource was last used in an attempt to access an Amazon Web Services service.
*
*
* @return The ARN of the IAM resource (user, group, role, or managed policy) used to generate information about
* when the resource was last used in an attempt to access an Amazon Web Services service.
*/
public final String arn() {
return arn;
}
/**
*
* The level of detail that you want to generate. You can specify whether you want to generate information about the
* last attempt to access services or actions. If you specify service-level granularity, this operation generates
* only service data. If you specify action-level granularity, it generates service and action data. If you don't
* include this optional parameter, the operation generates service data.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #granularity} will
* return {@link AccessAdvisorUsageGranularityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #granularityAsString}.
*
*
* @return The level of detail that you want to generate. You can specify whether you want to generate information
* about the last attempt to access services or actions. If you specify service-level granularity, this
* operation generates only service data. If you specify action-level granularity, it generates service and
* action data. If you don't include this optional parameter, the operation generates service data.
* @see AccessAdvisorUsageGranularityType
*/
public final AccessAdvisorUsageGranularityType granularity() {
return AccessAdvisorUsageGranularityType.fromValue(granularity);
}
/**
*
* The level of detail that you want to generate. You can specify whether you want to generate information about the
* last attempt to access services or actions. If you specify service-level granularity, this operation generates
* only service data. If you specify action-level granularity, it generates service and action data. If you don't
* include this optional parameter, the operation generates service data.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #granularity} will
* return {@link AccessAdvisorUsageGranularityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #granularityAsString}.
*
*
* @return The level of detail that you want to generate. You can specify whether you want to generate information
* about the last attempt to access services or actions. If you specify service-level granularity, this
* operation generates only service data. If you specify action-level granularity, it generates service and
* action data. If you don't include this optional parameter, the operation generates service data.
* @see AccessAdvisorUsageGranularityType
*/
public final String granularityAsString() {
return granularity;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(granularityAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GenerateServiceLastAccessedDetailsRequest)) {
return false;
}
GenerateServiceLastAccessedDetailsRequest other = (GenerateServiceLastAccessedDetailsRequest) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(granularityAsString(), other.granularityAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GenerateServiceLastAccessedDetailsRequest").add("Arn", arn())
.add("Granularity", granularityAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Granularity":
return Optional.ofNullable(clazz.cast(granularityAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function