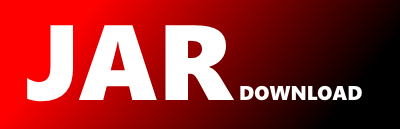
software.amazon.awssdk.services.iam.model.UserDetail Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about an IAM user, including all the user's policies and all the IAM groups the user is in.
*
*
* This data type is used as a response element in the GetAccountAuthorizationDetails operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UserDetail implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField PATH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Path")
.getter(getter(UserDetail::path)).setter(setter(Builder::path))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Path").build()).build();
private static final SdkField USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UserName").getter(getter(UserDetail::userName)).setter(setter(Builder::userName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserName").build()).build();
private static final SdkField USER_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("UserId")
.getter(getter(UserDetail::userId)).setter(setter(Builder::userId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserId").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(UserDetail::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField CREATE_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateDate").getter(getter(UserDetail::createDate)).setter(setter(Builder::createDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateDate").build()).build();
private static final SdkField> USER_POLICY_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserPolicyList")
.getter(getter(UserDetail::userPolicyList))
.setter(setter(Builder::userPolicyList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserPolicyList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PolicyDetail::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> GROUP_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("GroupList")
.getter(getter(UserDetail::groupList))
.setter(setter(Builder::groupList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GroupList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ATTACHED_MANAGED_POLICIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AttachedManagedPolicies")
.getter(getter(UserDetail::attachedManagedPolicies))
.setter(setter(Builder::attachedManagedPolicies))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttachedManagedPolicies").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AttachedPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PERMISSIONS_BOUNDARY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PermissionsBoundary")
.getter(getter(UserDetail::permissionsBoundary)).setter(setter(Builder::permissionsBoundary))
.constructor(AttachedPermissionsBoundary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PermissionsBoundary").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(UserDetail::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PATH_FIELD, USER_NAME_FIELD,
USER_ID_FIELD, ARN_FIELD, CREATE_DATE_FIELD, USER_POLICY_LIST_FIELD, GROUP_LIST_FIELD,
ATTACHED_MANAGED_POLICIES_FIELD, PERMISSIONS_BOUNDARY_FIELD, TAGS_FIELD));
private static final long serialVersionUID = 1L;
private final String path;
private final String userName;
private final String userId;
private final String arn;
private final Instant createDate;
private final List userPolicyList;
private final List groupList;
private final List attachedManagedPolicies;
private final AttachedPermissionsBoundary permissionsBoundary;
private final List tags;
private UserDetail(BuilderImpl builder) {
this.path = builder.path;
this.userName = builder.userName;
this.userId = builder.userId;
this.arn = builder.arn;
this.createDate = builder.createDate;
this.userPolicyList = builder.userPolicyList;
this.groupList = builder.groupList;
this.attachedManagedPolicies = builder.attachedManagedPolicies;
this.permissionsBoundary = builder.permissionsBoundary;
this.tags = builder.tags;
}
/**
*
* The path to the user. For more information about paths, see IAM identifiers in the IAM
* User Guide.
*
*
* @return The path to the user. For more information about paths, see IAM identifiers in the
* IAM User Guide.
*/
public final String path() {
return path;
}
/**
*
* The friendly name identifying the user.
*
*
* @return The friendly name identifying the user.
*/
public final String userName() {
return userName;
}
/**
*
* The stable and unique string identifying the user. For more information about IDs, see IAM identifiers in the IAM
* User Guide.
*
*
* @return The stable and unique string identifying the user. For more information about IDs, see IAM identifiers in the
* IAM User Guide.
*/
public final String userId() {
return userId;
}
/**
* Returns the value of the Arn property for this object.
*
* @return The value of the Arn property for this object.
*/
public final String arn() {
return arn;
}
/**
*
* The date and time, in ISO 8601 date-time format, when the user was
* created.
*
*
* @return The date and time, in ISO 8601 date-time format, when the
* user was created.
*/
public final Instant createDate() {
return createDate;
}
/**
* For responses, this returns true if the service returned a value for the UserPolicyList property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasUserPolicyList() {
return userPolicyList != null && !(userPolicyList instanceof SdkAutoConstructList);
}
/**
*
* A list of the inline policies embedded in the user.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasUserPolicyList} method.
*
*
* @return A list of the inline policies embedded in the user.
*/
public final List userPolicyList() {
return userPolicyList;
}
/**
* For responses, this returns true if the service returned a value for the GroupList property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasGroupList() {
return groupList != null && !(groupList instanceof SdkAutoConstructList);
}
/**
*
* A list of IAM groups that the user is in.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasGroupList} method.
*
*
* @return A list of IAM groups that the user is in.
*/
public final List groupList() {
return groupList;
}
/**
* For responses, this returns true if the service returned a value for the AttachedManagedPolicies property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasAttachedManagedPolicies() {
return attachedManagedPolicies != null && !(attachedManagedPolicies instanceof SdkAutoConstructList);
}
/**
*
* A list of the managed policies attached to the user.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttachedManagedPolicies} method.
*
*
* @return A list of the managed policies attached to the user.
*/
public final List attachedManagedPolicies() {
return attachedManagedPolicies;
}
/**
*
* The ARN of the policy used to set the permissions boundary for the user.
*
*
* For more information about permissions boundaries, see Permissions boundaries
* for IAM identities in the IAM User Guide.
*
*
* @return The ARN of the policy used to set the permissions boundary for the user.
*
* For more information about permissions boundaries, see Permissions
* boundaries for IAM identities in the IAM User Guide.
*/
public final AttachedPermissionsBoundary permissionsBoundary() {
return permissionsBoundary;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags that are associated with the user. For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags that are associated with the user. For more information about tagging, see Tagging IAM resources in the
* IAM User Guide.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(path());
hashCode = 31 * hashCode + Objects.hashCode(userName());
hashCode = 31 * hashCode + Objects.hashCode(userId());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(createDate());
hashCode = 31 * hashCode + Objects.hashCode(hasUserPolicyList() ? userPolicyList() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasGroupList() ? groupList() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAttachedManagedPolicies() ? attachedManagedPolicies() : null);
hashCode = 31 * hashCode + Objects.hashCode(permissionsBoundary());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UserDetail)) {
return false;
}
UserDetail other = (UserDetail) obj;
return Objects.equals(path(), other.path()) && Objects.equals(userName(), other.userName())
&& Objects.equals(userId(), other.userId()) && Objects.equals(arn(), other.arn())
&& Objects.equals(createDate(), other.createDate()) && hasUserPolicyList() == other.hasUserPolicyList()
&& Objects.equals(userPolicyList(), other.userPolicyList()) && hasGroupList() == other.hasGroupList()
&& Objects.equals(groupList(), other.groupList())
&& hasAttachedManagedPolicies() == other.hasAttachedManagedPolicies()
&& Objects.equals(attachedManagedPolicies(), other.attachedManagedPolicies())
&& Objects.equals(permissionsBoundary(), other.permissionsBoundary()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UserDetail").add("Path", path()).add("UserName", userName()).add("UserId", userId())
.add("Arn", arn()).add("CreateDate", createDate())
.add("UserPolicyList", hasUserPolicyList() ? userPolicyList() : null)
.add("GroupList", hasGroupList() ? groupList() : null)
.add("AttachedManagedPolicies", hasAttachedManagedPolicies() ? attachedManagedPolicies() : null)
.add("PermissionsBoundary", permissionsBoundary()).add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Path":
return Optional.ofNullable(clazz.cast(path()));
case "UserName":
return Optional.ofNullable(clazz.cast(userName()));
case "UserId":
return Optional.ofNullable(clazz.cast(userId()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "CreateDate":
return Optional.ofNullable(clazz.cast(createDate()));
case "UserPolicyList":
return Optional.ofNullable(clazz.cast(userPolicyList()));
case "GroupList":
return Optional.ofNullable(clazz.cast(groupList()));
case "AttachedManagedPolicies":
return Optional.ofNullable(clazz.cast(attachedManagedPolicies()));
case "PermissionsBoundary":
return Optional.ofNullable(clazz.cast(permissionsBoundary()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function