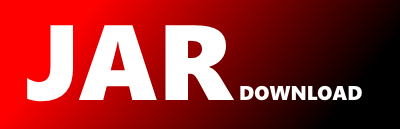
software.amazon.awssdk.services.iam.model.UpdateAccountPasswordPolicyRequest Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateAccountPasswordPolicyRequest extends IamRequest implements
ToCopyableBuilder {
private static final SdkField MINIMUM_PASSWORD_LENGTH_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MinimumPasswordLength").getter(getter(UpdateAccountPasswordPolicyRequest::minimumPasswordLength))
.setter(setter(Builder::minimumPasswordLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinimumPasswordLength").build())
.build();
private static final SdkField REQUIRE_SYMBOLS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RequireSymbols").getter(getter(UpdateAccountPasswordPolicyRequest::requireSymbols))
.setter(setter(Builder::requireSymbols))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireSymbols").build()).build();
private static final SdkField REQUIRE_NUMBERS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RequireNumbers").getter(getter(UpdateAccountPasswordPolicyRequest::requireNumbers))
.setter(setter(Builder::requireNumbers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireNumbers").build()).build();
private static final SdkField REQUIRE_UPPERCASE_CHARACTERS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("RequireUppercaseCharacters")
.getter(getter(UpdateAccountPasswordPolicyRequest::requireUppercaseCharacters))
.setter(setter(Builder::requireUppercaseCharacters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireUppercaseCharacters").build())
.build();
private static final SdkField REQUIRE_LOWERCASE_CHARACTERS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("RequireLowercaseCharacters")
.getter(getter(UpdateAccountPasswordPolicyRequest::requireLowercaseCharacters))
.setter(setter(Builder::requireLowercaseCharacters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireLowercaseCharacters").build())
.build();
private static final SdkField ALLOW_USERS_TO_CHANGE_PASSWORD_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("AllowUsersToChangePassword")
.getter(getter(UpdateAccountPasswordPolicyRequest::allowUsersToChangePassword))
.setter(setter(Builder::allowUsersToChangePassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowUsersToChangePassword").build())
.build();
private static final SdkField MAX_PASSWORD_AGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxPasswordAge").getter(getter(UpdateAccountPasswordPolicyRequest::maxPasswordAge))
.setter(setter(Builder::maxPasswordAge))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxPasswordAge").build()).build();
private static final SdkField PASSWORD_REUSE_PREVENTION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PasswordReusePrevention").getter(getter(UpdateAccountPasswordPolicyRequest::passwordReusePrevention))
.setter(setter(Builder::passwordReusePrevention))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PasswordReusePrevention").build())
.build();
private static final SdkField HARD_EXPIRY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("HardExpiry").getter(getter(UpdateAccountPasswordPolicyRequest::hardExpiry))
.setter(setter(Builder::hardExpiry))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HardExpiry").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MINIMUM_PASSWORD_LENGTH_FIELD,
REQUIRE_SYMBOLS_FIELD, REQUIRE_NUMBERS_FIELD, REQUIRE_UPPERCASE_CHARACTERS_FIELD, REQUIRE_LOWERCASE_CHARACTERS_FIELD,
ALLOW_USERS_TO_CHANGE_PASSWORD_FIELD, MAX_PASSWORD_AGE_FIELD, PASSWORD_REUSE_PREVENTION_FIELD, HARD_EXPIRY_FIELD));
private final Integer minimumPasswordLength;
private final Boolean requireSymbols;
private final Boolean requireNumbers;
private final Boolean requireUppercaseCharacters;
private final Boolean requireLowercaseCharacters;
private final Boolean allowUsersToChangePassword;
private final Integer maxPasswordAge;
private final Integer passwordReusePrevention;
private final Boolean hardExpiry;
private UpdateAccountPasswordPolicyRequest(BuilderImpl builder) {
super(builder);
this.minimumPasswordLength = builder.minimumPasswordLength;
this.requireSymbols = builder.requireSymbols;
this.requireNumbers = builder.requireNumbers;
this.requireUppercaseCharacters = builder.requireUppercaseCharacters;
this.requireLowercaseCharacters = builder.requireLowercaseCharacters;
this.allowUsersToChangePassword = builder.allowUsersToChangePassword;
this.maxPasswordAge = builder.maxPasswordAge;
this.passwordReusePrevention = builder.passwordReusePrevention;
this.hardExpiry = builder.hardExpiry;
}
/**
*
* The minimum number of characters allowed in an IAM user password.
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of 6
.
*
*
* @return The minimum number of characters allowed in an IAM user password.
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* 6
.
*/
public final Integer minimumPasswordLength() {
return minimumPasswordLength;
}
/**
*
* Specifies whether IAM user passwords must contain at least one of the following non-alphanumeric characters:
*
*
* ! @ # $ % ^ & * ( ) _ + - = [ ] { } | '
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of false
* . The result is that passwords do not require at least one symbol character.
*
*
* @return Specifies whether IAM user passwords must contain at least one of the following non-alphanumeric
* characters:
*
* ! @ # $ % ^ & * ( ) _ + - = [ ] { } | '
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* false
. The result is that passwords do not require at least one symbol character.
*/
public final Boolean requireSymbols() {
return requireSymbols;
}
/**
*
* Specifies whether IAM user passwords must contain at least one numeric character (0 to 9).
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of false
* . The result is that passwords do not require at least one numeric character.
*
*
* @return Specifies whether IAM user passwords must contain at least one numeric character (0 to 9).
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* false
. The result is that passwords do not require at least one numeric character.
*/
public final Boolean requireNumbers() {
return requireNumbers;
}
/**
*
* Specifies whether IAM user passwords must contain at least one uppercase character from the ISO basic Latin
* alphabet (A to Z).
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of false
* . The result is that passwords do not require at least one uppercase character.
*
*
* @return Specifies whether IAM user passwords must contain at least one uppercase character from the ISO basic
* Latin alphabet (A to Z).
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* false
. The result is that passwords do not require at least one uppercase character.
*/
public final Boolean requireUppercaseCharacters() {
return requireUppercaseCharacters;
}
/**
*
* Specifies whether IAM user passwords must contain at least one lowercase character from the ISO basic Latin
* alphabet (a to z).
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of false
* . The result is that passwords do not require at least one lowercase character.
*
*
* @return Specifies whether IAM user passwords must contain at least one lowercase character from the ISO basic
* Latin alphabet (a to z).
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* false
. The result is that passwords do not require at least one lowercase character.
*/
public final Boolean requireLowercaseCharacters() {
return requireLowercaseCharacters;
}
/**
*
* Allows all IAM users in your account to use the Amazon Web Services Management Console to change their own
* passwords. For more information, see Permitting IAM users to change their own passwords in the IAM User Guide.
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of false
* . The result is that IAM users in the account do not automatically have permissions to change their own password.
*
*
* @return Allows all IAM users in your account to use the Amazon Web Services Management Console to change their
* own passwords. For more information, see Permitting IAM users to change their own passwords in the IAM User Guide.
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* false
. The result is that IAM users in the account do not automatically have permissions to
* change their own password.
*/
public final Boolean allowUsersToChangePassword() {
return allowUsersToChangePassword;
}
/**
*
* The number of days that an IAM user password is valid.
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of 0
.
* The result is that IAM user passwords never expire.
*
*
* @return The number of days that an IAM user password is valid.
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* 0
. The result is that IAM user passwords never expire.
*/
public final Integer maxPasswordAge() {
return maxPasswordAge;
}
/**
*
* Specifies the number of previous passwords that IAM users are prevented from reusing.
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of 0
.
* The result is that IAM users are not prevented from reusing previous passwords.
*
*
* @return Specifies the number of previous passwords that IAM users are prevented from reusing.
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* 0
. The result is that IAM users are not prevented from reusing previous passwords.
*/
public final Integer passwordReusePrevention() {
return passwordReusePrevention;
}
/**
*
* Prevents IAM users who are accessing the account via the Amazon Web Services Management Console from setting a
* new console password after their password has expired. The IAM user cannot access the console until an
* administrator resets the password.
*
*
* If you do not specify a value for this parameter, then the operation uses the default value of false
* . The result is that IAM users can change their passwords after they expire and continue to sign in as the user.
*
*
*
* In the Amazon Web Services Management Console, the custom password policy option Allow users to change their
* own password gives IAM users permissions to iam:ChangePassword
for only their user and to the
* iam:GetAccountPasswordPolicy
action. This option does not attach a permissions policy to each user,
* rather the permissions are applied at the account-level for all users by IAM. IAM users with
* iam:ChangePassword
permission and active access keys can reset their own expired console password
* using the CLI or API.
*
*
*
* @return Prevents IAM users who are accessing the account via the Amazon Web Services Management Console from
* setting a new console password after their password has expired. The IAM user cannot access the console
* until an administrator resets the password.
*
* If you do not specify a value for this parameter, then the operation uses the default value of
* false
. The result is that IAM users can change their passwords after they expire and
* continue to sign in as the user.
*
*
*
* In the Amazon Web Services Management Console, the custom password policy option Allow users to change
* their own password gives IAM users permissions to iam:ChangePassword
for only their user
* and to the iam:GetAccountPasswordPolicy
action. This option does not attach a permissions
* policy to each user, rather the permissions are applied at the account-level for all users by IAM. IAM
* users with iam:ChangePassword
permission and active access keys can reset their own expired
* console password using the CLI or API.
*
*/
public final Boolean hardExpiry() {
return hardExpiry;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(minimumPasswordLength());
hashCode = 31 * hashCode + Objects.hashCode(requireSymbols());
hashCode = 31 * hashCode + Objects.hashCode(requireNumbers());
hashCode = 31 * hashCode + Objects.hashCode(requireUppercaseCharacters());
hashCode = 31 * hashCode + Objects.hashCode(requireLowercaseCharacters());
hashCode = 31 * hashCode + Objects.hashCode(allowUsersToChangePassword());
hashCode = 31 * hashCode + Objects.hashCode(maxPasswordAge());
hashCode = 31 * hashCode + Objects.hashCode(passwordReusePrevention());
hashCode = 31 * hashCode + Objects.hashCode(hardExpiry());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateAccountPasswordPolicyRequest)) {
return false;
}
UpdateAccountPasswordPolicyRequest other = (UpdateAccountPasswordPolicyRequest) obj;
return Objects.equals(minimumPasswordLength(), other.minimumPasswordLength())
&& Objects.equals(requireSymbols(), other.requireSymbols())
&& Objects.equals(requireNumbers(), other.requireNumbers())
&& Objects.equals(requireUppercaseCharacters(), other.requireUppercaseCharacters())
&& Objects.equals(requireLowercaseCharacters(), other.requireLowercaseCharacters())
&& Objects.equals(allowUsersToChangePassword(), other.allowUsersToChangePassword())
&& Objects.equals(maxPasswordAge(), other.maxPasswordAge())
&& Objects.equals(passwordReusePrevention(), other.passwordReusePrevention())
&& Objects.equals(hardExpiry(), other.hardExpiry());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateAccountPasswordPolicyRequest").add("MinimumPasswordLength", minimumPasswordLength())
.add("RequireSymbols", requireSymbols()).add("RequireNumbers", requireNumbers())
.add("RequireUppercaseCharacters", requireUppercaseCharacters())
.add("RequireLowercaseCharacters", requireLowercaseCharacters())
.add("AllowUsersToChangePassword", allowUsersToChangePassword()).add("MaxPasswordAge", maxPasswordAge())
.add("PasswordReusePrevention", passwordReusePrevention()).add("HardExpiry", hardExpiry()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MinimumPasswordLength":
return Optional.ofNullable(clazz.cast(minimumPasswordLength()));
case "RequireSymbols":
return Optional.ofNullable(clazz.cast(requireSymbols()));
case "RequireNumbers":
return Optional.ofNullable(clazz.cast(requireNumbers()));
case "RequireUppercaseCharacters":
return Optional.ofNullable(clazz.cast(requireUppercaseCharacters()));
case "RequireLowercaseCharacters":
return Optional.ofNullable(clazz.cast(requireLowercaseCharacters()));
case "AllowUsersToChangePassword":
return Optional.ofNullable(clazz.cast(allowUsersToChangePassword()));
case "MaxPasswordAge":
return Optional.ofNullable(clazz.cast(maxPasswordAge()));
case "PasswordReusePrevention":
return Optional.ofNullable(clazz.cast(passwordReusePrevention()));
case "HardExpiry":
return Optional.ofNullable(clazz.cast(hardExpiry()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function