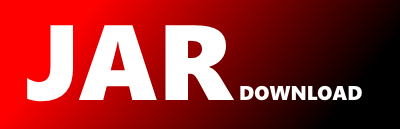
software.amazon.awssdk.services.iam.model.AccessDetail Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object that contains details about when a principal in the reported Organizations entity last attempted to access
* an Amazon Web Services service. A principal can be an IAM user, an IAM role, or the Amazon Web Services account root
* user within the reported Organizations entity.
*
*
* This data type is a response element in the GetOrganizationsAccessReport operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AccessDetail implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceName").getter(getter(AccessDetail::serviceName)).setter(setter(Builder::serviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceName").build()).build();
private static final SdkField SERVICE_NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceNamespace").getter(getter(AccessDetail::serviceNamespace))
.setter(setter(Builder::serviceNamespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceNamespace").build()).build();
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Region")
.getter(getter(AccessDetail::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Region").build()).build();
private static final SdkField ENTITY_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EntityPath").getter(getter(AccessDetail::entityPath)).setter(setter(Builder::entityPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EntityPath").build()).build();
private static final SdkField LAST_AUTHENTICATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastAuthenticatedTime").getter(getter(AccessDetail::lastAuthenticatedTime))
.setter(setter(Builder::lastAuthenticatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastAuthenticatedTime").build())
.build();
private static final SdkField TOTAL_AUTHENTICATED_ENTITIES_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("TotalAuthenticatedEntities")
.getter(getter(AccessDetail::totalAuthenticatedEntities))
.setter(setter(Builder::totalAuthenticatedEntities))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalAuthenticatedEntities").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_NAME_FIELD,
SERVICE_NAMESPACE_FIELD, REGION_FIELD, ENTITY_PATH_FIELD, LAST_AUTHENTICATED_TIME_FIELD,
TOTAL_AUTHENTICATED_ENTITIES_FIELD));
private static final long serialVersionUID = 1L;
private final String serviceName;
private final String serviceNamespace;
private final String region;
private final String entityPath;
private final Instant lastAuthenticatedTime;
private final Integer totalAuthenticatedEntities;
private AccessDetail(BuilderImpl builder) {
this.serviceName = builder.serviceName;
this.serviceNamespace = builder.serviceNamespace;
this.region = builder.region;
this.entityPath = builder.entityPath;
this.lastAuthenticatedTime = builder.lastAuthenticatedTime;
this.totalAuthenticatedEntities = builder.totalAuthenticatedEntities;
}
/**
*
* The name of the service in which access was attempted.
*
*
* @return The name of the service in which access was attempted.
*/
public final String serviceName() {
return serviceName;
}
/**
*
* The namespace of the service in which access was attempted.
*
*
* To learn the service namespace of a service, see Actions, resources, and condition keys for Amazon Web Services services in the Service Authorization
* Reference. Choose the name of the service to view details for that service. In the first paragraph, find the
* service prefix. For example, (service prefix: a4b)
. For more information about service namespaces,
* see Amazon Web Services service namespaces in the Amazon Web Services General Reference.
*
*
* @return The namespace of the service in which access was attempted.
*
* To learn the service namespace of a service, see Actions, resources, and condition keys for Amazon Web Services services in the Service
* Authorization Reference. Choose the name of the service to view details for that service. In the
* first paragraph, find the service prefix. For example, (service prefix: a4b)
. For more
* information about service namespaces, see Amazon Web Services service namespaces in the Amazon Web Services General Reference.
*/
public final String serviceNamespace() {
return serviceNamespace;
}
/**
*
* The Region where the last service access attempt occurred.
*
*
* This field is null if no principals in the reported Organizations entity attempted to access the service within
* the tracking period.
*
*
* @return The Region where the last service access attempt occurred.
*
* This field is null if no principals in the reported Organizations entity attempted to access the service
* within the tracking period.
*/
public final String region() {
return region;
}
/**
*
* The path of the Organizations entity (root, organizational unit, or account) from which an authenticated
* principal last attempted to access the service. Amazon Web Services does not report unauthenticated requests.
*
*
* This field is null if no principals (IAM users, IAM roles, or root user) in the reported Organizations entity
* attempted to access the service within the tracking period.
*
*
* @return The path of the Organizations entity (root, organizational unit, or account) from which an authenticated
* principal last attempted to access the service. Amazon Web Services does not report unauthenticated
* requests.
*
* This field is null if no principals (IAM users, IAM roles, or root user) in the reported Organizations
* entity attempted to access the service within the tracking period.
*/
public final String entityPath() {
return entityPath;
}
/**
*
* The date and time, in ISO 8601 date-time format, when an
* authenticated principal most recently attempted to access the service. Amazon Web Services does not report
* unauthenticated requests.
*
*
* This field is null if no principals in the reported Organizations entity attempted to access the service within
* the tracking period.
*
*
* @return The date and time, in ISO 8601 date-time format, when an
* authenticated principal most recently attempted to access the service. Amazon Web Services does not
* report unauthenticated requests.
*
* This field is null if no principals in the reported Organizations entity attempted to access the service
* within the tracking period.
*/
public final Instant lastAuthenticatedTime() {
return lastAuthenticatedTime;
}
/**
*
* The number of accounts with authenticated principals (root user, IAM users, and IAM roles) that attempted to
* access the service in the tracking period.
*
*
* @return The number of accounts with authenticated principals (root user, IAM users, and IAM roles) that attempted
* to access the service in the tracking period.
*/
public final Integer totalAuthenticatedEntities() {
return totalAuthenticatedEntities;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serviceName());
hashCode = 31 * hashCode + Objects.hashCode(serviceNamespace());
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(entityPath());
hashCode = 31 * hashCode + Objects.hashCode(lastAuthenticatedTime());
hashCode = 31 * hashCode + Objects.hashCode(totalAuthenticatedEntities());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AccessDetail)) {
return false;
}
AccessDetail other = (AccessDetail) obj;
return Objects.equals(serviceName(), other.serviceName()) && Objects.equals(serviceNamespace(), other.serviceNamespace())
&& Objects.equals(region(), other.region()) && Objects.equals(entityPath(), other.entityPath())
&& Objects.equals(lastAuthenticatedTime(), other.lastAuthenticatedTime())
&& Objects.equals(totalAuthenticatedEntities(), other.totalAuthenticatedEntities());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AccessDetail").add("ServiceName", serviceName()).add("ServiceNamespace", serviceNamespace())
.add("Region", region()).add("EntityPath", entityPath()).add("LastAuthenticatedTime", lastAuthenticatedTime())
.add("TotalAuthenticatedEntities", totalAuthenticatedEntities()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ServiceName":
return Optional.ofNullable(clazz.cast(serviceName()));
case "ServiceNamespace":
return Optional.ofNullable(clazz.cast(serviceNamespace()));
case "Region":
return Optional.ofNullable(clazz.cast(region()));
case "EntityPath":
return Optional.ofNullable(clazz.cast(entityPath()));
case "LastAuthenticatedTime":
return Optional.ofNullable(clazz.cast(lastAuthenticatedTime()));
case "TotalAuthenticatedEntities":
return Optional.ofNullable(clazz.cast(totalAuthenticatedEntities()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function