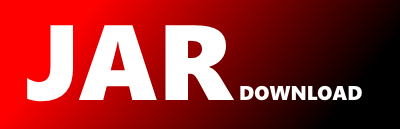
software.amazon.awssdk.services.iam.model.UpdateUserRequest Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateUserRequest extends IamRequest implements
ToCopyableBuilder {
private static final SdkField USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UserName").getter(getter(UpdateUserRequest::userName)).setter(setter(Builder::userName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserName").build()).build();
private static final SdkField NEW_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NewPath").getter(getter(UpdateUserRequest::newPath)).setter(setter(Builder::newPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewPath").build()).build();
private static final SdkField NEW_USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NewUserName").getter(getter(UpdateUserRequest::newUserName)).setter(setter(Builder::newUserName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewUserName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(USER_NAME_FIELD,
NEW_PATH_FIELD, NEW_USER_NAME_FIELD));
private final String userName;
private final String newPath;
private final String newUserName;
private UpdateUserRequest(BuilderImpl builder) {
super(builder);
this.userName = builder.userName;
this.newPath = builder.newPath;
this.newUserName = builder.newUserName;
}
/**
*
* Name of the user to update. If you're changing the name of the user, this is the original user name.
*
*
* This parameter allows (through its regex pattern) a string of
* characters consisting of upper and lowercase alphanumeric characters with no spaces. You can also include any of
* the following characters: _+=,.@-
*
*
* @return Name of the user to update. If you're changing the name of the user, this is the original user name.
*
* This parameter allows (through its regex pattern) a string
* of characters consisting of upper and lowercase alphanumeric characters with no spaces. You can also
* include any of the following characters: _+=,.@-
*/
public final String userName() {
return userName;
}
/**
*
* New path for the IAM user. Include this parameter only if you're changing the user's path.
*
*
* This parameter allows (through its regex pattern) a string of
* characters consisting of either a forward slash (/) by itself or a string that must begin and end with forward
* slashes. In addition, it can contain any ASCII character from the ! (\u0021
) through the DEL
* character (\u007F
), including most punctuation characters, digits, and upper and lowercased letters.
*
*
* @return New path for the IAM user. Include this parameter only if you're changing the user's path.
*
* This parameter allows (through its regex pattern) a string
* of characters consisting of either a forward slash (/) by itself or a string that must begin and end with
* forward slashes. In addition, it can contain any ASCII character from the ! (\u0021
) through
* the DEL character (\u007F
), including most punctuation characters, digits, and upper and
* lowercased letters.
*/
public final String newPath() {
return newPath;
}
/**
*
* New name for the user. Include this parameter only if you're changing the user's name.
*
*
* IAM user, group, role, and policy names must be unique within the account. Names are not distinguished by case.
* For example, you cannot create resources named both "MyResource" and "myresource".
*
*
* @return New name for the user. Include this parameter only if you're changing the user's name.
*
* IAM user, group, role, and policy names must be unique within the account. Names are not distinguished by
* case. For example, you cannot create resources named both "MyResource" and "myresource".
*/
public final String newUserName() {
return newUserName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(userName());
hashCode = 31 * hashCode + Objects.hashCode(newPath());
hashCode = 31 * hashCode + Objects.hashCode(newUserName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateUserRequest)) {
return false;
}
UpdateUserRequest other = (UpdateUserRequest) obj;
return Objects.equals(userName(), other.userName()) && Objects.equals(newPath(), other.newPath())
&& Objects.equals(newUserName(), other.newUserName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateUserRequest").add("UserName", userName()).add("NewPath", newPath())
.add("NewUserName", newUserName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UserName":
return Optional.ofNullable(clazz.cast(userName()));
case "NewPath":
return Optional.ofNullable(clazz.cast(newPath()));
case "NewUserName":
return Optional.ofNullable(clazz.cast(newUserName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* This parameter allows (through its regex pattern) a
* string of characters consisting of upper and lowercase alphanumeric characters with no spaces. You can
* also include any of the following characters: _+=,.@-
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder userName(String userName);
/**
*
* New path for the IAM user. Include this parameter only if you're changing the user's path.
*
*
* This parameter allows (through its regex pattern) a string of
* characters consisting of either a forward slash (/) by itself or a string that must begin and end with
* forward slashes. In addition, it can contain any ASCII character from the ! (\u0021
) through the
* DEL character (\u007F
), including most punctuation characters, digits, and upper and lowercased
* letters.
*
*
* @param newPath
* New path for the IAM user. Include this parameter only if you're changing the user's path.
*
* This parameter allows (through its regex pattern) a
* string of characters consisting of either a forward slash (/) by itself or a string that must begin
* and end with forward slashes. In addition, it can contain any ASCII character from the ! (
* \u0021
) through the DEL character (\u007F
), including most punctuation
* characters, digits, and upper and lowercased letters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder newPath(String newPath);
/**
*
* New name for the user. Include this parameter only if you're changing the user's name.
*
*
* IAM user, group, role, and policy names must be unique within the account. Names are not distinguished by
* case. For example, you cannot create resources named both "MyResource" and "myresource".
*
*
* @param newUserName
* New name for the user. Include this parameter only if you're changing the user's name.
*
* IAM user, group, role, and policy names must be unique within the account. Names are not distinguished
* by case. For example, you cannot create resources named both "MyResource" and "myresource".
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder newUserName(String newUserName);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends IamRequest.BuilderImpl implements Builder {
private String userName;
private String newPath;
private String newUserName;
private BuilderImpl() {
}
private BuilderImpl(UpdateUserRequest model) {
super(model);
userName(model.userName);
newPath(model.newPath);
newUserName(model.newUserName);
}
public final String getUserName() {
return userName;
}
public final void setUserName(String userName) {
this.userName = userName;
}
@Override
public final Builder userName(String userName) {
this.userName = userName;
return this;
}
public final String getNewPath() {
return newPath;
}
public final void setNewPath(String newPath) {
this.newPath = newPath;
}
@Override
public final Builder newPath(String newPath) {
this.newPath = newPath;
return this;
}
public final String getNewUserName() {
return newUserName;
}
public final void setNewUserName(String newUserName) {
this.newUserName = newUserName;
}
@Override
public final Builder newUserName(String newUserName) {
this.newUserName = newUserName;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateUserRequest build() {
return new UpdateUserRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}