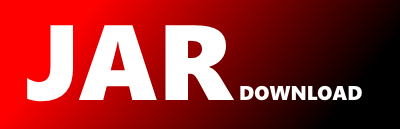
software.amazon.awssdk.services.iam.DefaultIamAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.iam.internal.IamServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.iam.model.AccountNotManagementOrDelegatedAdministratorException;
import software.amazon.awssdk.services.iam.model.AddClientIdToOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.AddClientIdToOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.AddRoleToInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.AddRoleToInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.AddUserToGroupRequest;
import software.amazon.awssdk.services.iam.model.AddUserToGroupResponse;
import software.amazon.awssdk.services.iam.model.AttachGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.AttachGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.AttachRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.AttachRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.AttachUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.AttachUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.CallerIsNotManagementAccountException;
import software.amazon.awssdk.services.iam.model.ChangePasswordRequest;
import software.amazon.awssdk.services.iam.model.ChangePasswordResponse;
import software.amazon.awssdk.services.iam.model.ConcurrentModificationException;
import software.amazon.awssdk.services.iam.model.CreateAccessKeyRequest;
import software.amazon.awssdk.services.iam.model.CreateAccessKeyResponse;
import software.amazon.awssdk.services.iam.model.CreateAccountAliasRequest;
import software.amazon.awssdk.services.iam.model.CreateAccountAliasResponse;
import software.amazon.awssdk.services.iam.model.CreateGroupRequest;
import software.amazon.awssdk.services.iam.model.CreateGroupResponse;
import software.amazon.awssdk.services.iam.model.CreateInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.CreateInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.CreateLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.CreateLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.CreateOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.CreateOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.CreatePolicyRequest;
import software.amazon.awssdk.services.iam.model.CreatePolicyResponse;
import software.amazon.awssdk.services.iam.model.CreatePolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.CreatePolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.CreateRoleRequest;
import software.amazon.awssdk.services.iam.model.CreateRoleResponse;
import software.amazon.awssdk.services.iam.model.CreateSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.CreateSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.CreateServiceLinkedRoleRequest;
import software.amazon.awssdk.services.iam.model.CreateServiceLinkedRoleResponse;
import software.amazon.awssdk.services.iam.model.CreateServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.CreateServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.CreateUserRequest;
import software.amazon.awssdk.services.iam.model.CreateUserResponse;
import software.amazon.awssdk.services.iam.model.CreateVirtualMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.CreateVirtualMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.CredentialReportExpiredException;
import software.amazon.awssdk.services.iam.model.CredentialReportNotPresentException;
import software.amazon.awssdk.services.iam.model.CredentialReportNotReadyException;
import software.amazon.awssdk.services.iam.model.DeactivateMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.DeactivateMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.DeleteAccessKeyRequest;
import software.amazon.awssdk.services.iam.model.DeleteAccessKeyResponse;
import software.amazon.awssdk.services.iam.model.DeleteAccountAliasRequest;
import software.amazon.awssdk.services.iam.model.DeleteAccountAliasResponse;
import software.amazon.awssdk.services.iam.model.DeleteAccountPasswordPolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteAccountPasswordPolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteConflictException;
import software.amazon.awssdk.services.iam.model.DeleteGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteGroupRequest;
import software.amazon.awssdk.services.iam.model.DeleteGroupResponse;
import software.amazon.awssdk.services.iam.model.DeleteInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.DeleteInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.DeleteLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.DeleteLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.DeleteOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.DeleteOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.DeletePolicyRequest;
import software.amazon.awssdk.services.iam.model.DeletePolicyResponse;
import software.amazon.awssdk.services.iam.model.DeletePolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.DeletePolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.DeleteRolePermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.DeleteRolePermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.DeleteRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteRoleRequest;
import software.amazon.awssdk.services.iam.model.DeleteRoleResponse;
import software.amazon.awssdk.services.iam.model.DeleteSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.DeleteSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.DeleteServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.DeleteServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.DeleteServiceLinkedRoleRequest;
import software.amazon.awssdk.services.iam.model.DeleteServiceLinkedRoleResponse;
import software.amazon.awssdk.services.iam.model.DeleteServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.DeleteServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.DeleteSigningCertificateRequest;
import software.amazon.awssdk.services.iam.model.DeleteSigningCertificateResponse;
import software.amazon.awssdk.services.iam.model.DeleteSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.DeleteSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.model.DeleteUserPermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.DeleteUserPermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.DeleteUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.DeleteUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.DeleteUserRequest;
import software.amazon.awssdk.services.iam.model.DeleteUserResponse;
import software.amazon.awssdk.services.iam.model.DeleteVirtualMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.DeleteVirtualMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.DetachGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.DetachGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.DetachRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.DetachRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.DetachUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.DetachUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.DisableOrganizationsRootCredentialsManagementRequest;
import software.amazon.awssdk.services.iam.model.DisableOrganizationsRootCredentialsManagementResponse;
import software.amazon.awssdk.services.iam.model.DisableOrganizationsRootSessionsRequest;
import software.amazon.awssdk.services.iam.model.DisableOrganizationsRootSessionsResponse;
import software.amazon.awssdk.services.iam.model.DuplicateCertificateException;
import software.amazon.awssdk.services.iam.model.DuplicateSshPublicKeyException;
import software.amazon.awssdk.services.iam.model.EnableMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.EnableMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.EnableOrganizationsRootCredentialsManagementRequest;
import software.amazon.awssdk.services.iam.model.EnableOrganizationsRootCredentialsManagementResponse;
import software.amazon.awssdk.services.iam.model.EnableOrganizationsRootSessionsRequest;
import software.amazon.awssdk.services.iam.model.EnableOrganizationsRootSessionsResponse;
import software.amazon.awssdk.services.iam.model.EntityAlreadyExistsException;
import software.amazon.awssdk.services.iam.model.EntityTemporarilyUnmodifiableException;
import software.amazon.awssdk.services.iam.model.GenerateCredentialReportRequest;
import software.amazon.awssdk.services.iam.model.GenerateCredentialReportResponse;
import software.amazon.awssdk.services.iam.model.GenerateOrganizationsAccessReportRequest;
import software.amazon.awssdk.services.iam.model.GenerateOrganizationsAccessReportResponse;
import software.amazon.awssdk.services.iam.model.GenerateServiceLastAccessedDetailsRequest;
import software.amazon.awssdk.services.iam.model.GenerateServiceLastAccessedDetailsResponse;
import software.amazon.awssdk.services.iam.model.GetAccessKeyLastUsedRequest;
import software.amazon.awssdk.services.iam.model.GetAccessKeyLastUsedResponse;
import software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsRequest;
import software.amazon.awssdk.services.iam.model.GetAccountAuthorizationDetailsResponse;
import software.amazon.awssdk.services.iam.model.GetAccountPasswordPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetAccountPasswordPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetAccountSummaryRequest;
import software.amazon.awssdk.services.iam.model.GetAccountSummaryResponse;
import software.amazon.awssdk.services.iam.model.GetContextKeysForCustomPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetContextKeysForCustomPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetContextKeysForPrincipalPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetContextKeysForPrincipalPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetCredentialReportRequest;
import software.amazon.awssdk.services.iam.model.GetCredentialReportResponse;
import software.amazon.awssdk.services.iam.model.GetGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetGroupRequest;
import software.amazon.awssdk.services.iam.model.GetGroupResponse;
import software.amazon.awssdk.services.iam.model.GetInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.GetInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.GetLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.GetLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.GetMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.GetMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.GetOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.GetOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.GetOrganizationsAccessReportRequest;
import software.amazon.awssdk.services.iam.model.GetOrganizationsAccessReportResponse;
import software.amazon.awssdk.services.iam.model.GetPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetPolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.GetPolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.GetRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.GetRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.GetRoleRequest;
import software.amazon.awssdk.services.iam.model.GetRoleResponse;
import software.amazon.awssdk.services.iam.model.GetSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.GetSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.GetServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.GetServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsRequest;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsResponse;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsWithEntitiesRequest;
import software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsWithEntitiesResponse;
import software.amazon.awssdk.services.iam.model.GetServiceLinkedRoleDeletionStatusRequest;
import software.amazon.awssdk.services.iam.model.GetServiceLinkedRoleDeletionStatusResponse;
import software.amazon.awssdk.services.iam.model.GetSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.GetSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.model.GetUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.GetUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.GetUserRequest;
import software.amazon.awssdk.services.iam.model.GetUserResponse;
import software.amazon.awssdk.services.iam.model.IamException;
import software.amazon.awssdk.services.iam.model.InvalidAuthenticationCodeException;
import software.amazon.awssdk.services.iam.model.InvalidCertificateException;
import software.amazon.awssdk.services.iam.model.InvalidInputException;
import software.amazon.awssdk.services.iam.model.InvalidPublicKeyException;
import software.amazon.awssdk.services.iam.model.InvalidUserTypeException;
import software.amazon.awssdk.services.iam.model.KeyPairMismatchException;
import software.amazon.awssdk.services.iam.model.LimitExceededException;
import software.amazon.awssdk.services.iam.model.ListAccessKeysRequest;
import software.amazon.awssdk.services.iam.model.ListAccessKeysResponse;
import software.amazon.awssdk.services.iam.model.ListAccountAliasesRequest;
import software.amazon.awssdk.services.iam.model.ListAccountAliasesResponse;
import software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListAttachedGroupPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListAttachedRolePoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListAttachedUserPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyRequest;
import software.amazon.awssdk.services.iam.model.ListEntitiesForPolicyResponse;
import software.amazon.awssdk.services.iam.model.ListGroupPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListGroupPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListGroupsForUserRequest;
import software.amazon.awssdk.services.iam.model.ListGroupsForUserResponse;
import software.amazon.awssdk.services.iam.model.ListGroupsRequest;
import software.amazon.awssdk.services.iam.model.ListGroupsResponse;
import software.amazon.awssdk.services.iam.model.ListInstanceProfileTagsRequest;
import software.amazon.awssdk.services.iam.model.ListInstanceProfileTagsResponse;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleRequest;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesForRoleResponse;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesRequest;
import software.amazon.awssdk.services.iam.model.ListInstanceProfilesResponse;
import software.amazon.awssdk.services.iam.model.ListMfaDeviceTagsRequest;
import software.amazon.awssdk.services.iam.model.ListMfaDeviceTagsResponse;
import software.amazon.awssdk.services.iam.model.ListMfaDevicesRequest;
import software.amazon.awssdk.services.iam.model.ListMfaDevicesResponse;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProviderTagsRequest;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProviderTagsResponse;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProvidersRequest;
import software.amazon.awssdk.services.iam.model.ListOpenIdConnectProvidersResponse;
import software.amazon.awssdk.services.iam.model.ListOrganizationsFeaturesRequest;
import software.amazon.awssdk.services.iam.model.ListOrganizationsFeaturesResponse;
import software.amazon.awssdk.services.iam.model.ListPoliciesGrantingServiceAccessRequest;
import software.amazon.awssdk.services.iam.model.ListPoliciesGrantingServiceAccessResponse;
import software.amazon.awssdk.services.iam.model.ListPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListPolicyTagsRequest;
import software.amazon.awssdk.services.iam.model.ListPolicyTagsResponse;
import software.amazon.awssdk.services.iam.model.ListPolicyVersionsRequest;
import software.amazon.awssdk.services.iam.model.ListPolicyVersionsResponse;
import software.amazon.awssdk.services.iam.model.ListRolePoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListRolePoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListRoleTagsRequest;
import software.amazon.awssdk.services.iam.model.ListRoleTagsResponse;
import software.amazon.awssdk.services.iam.model.ListRolesRequest;
import software.amazon.awssdk.services.iam.model.ListRolesResponse;
import software.amazon.awssdk.services.iam.model.ListSamlProviderTagsRequest;
import software.amazon.awssdk.services.iam.model.ListSamlProviderTagsResponse;
import software.amazon.awssdk.services.iam.model.ListSamlProvidersRequest;
import software.amazon.awssdk.services.iam.model.ListSamlProvidersResponse;
import software.amazon.awssdk.services.iam.model.ListServerCertificateTagsRequest;
import software.amazon.awssdk.services.iam.model.ListServerCertificateTagsResponse;
import software.amazon.awssdk.services.iam.model.ListServerCertificatesRequest;
import software.amazon.awssdk.services.iam.model.ListServerCertificatesResponse;
import software.amazon.awssdk.services.iam.model.ListServiceSpecificCredentialsRequest;
import software.amazon.awssdk.services.iam.model.ListServiceSpecificCredentialsResponse;
import software.amazon.awssdk.services.iam.model.ListSigningCertificatesRequest;
import software.amazon.awssdk.services.iam.model.ListSigningCertificatesResponse;
import software.amazon.awssdk.services.iam.model.ListSshPublicKeysRequest;
import software.amazon.awssdk.services.iam.model.ListSshPublicKeysResponse;
import software.amazon.awssdk.services.iam.model.ListUserPoliciesRequest;
import software.amazon.awssdk.services.iam.model.ListUserPoliciesResponse;
import software.amazon.awssdk.services.iam.model.ListUserTagsRequest;
import software.amazon.awssdk.services.iam.model.ListUserTagsResponse;
import software.amazon.awssdk.services.iam.model.ListUsersRequest;
import software.amazon.awssdk.services.iam.model.ListUsersResponse;
import software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesRequest;
import software.amazon.awssdk.services.iam.model.ListVirtualMfaDevicesResponse;
import software.amazon.awssdk.services.iam.model.MalformedCertificateException;
import software.amazon.awssdk.services.iam.model.MalformedPolicyDocumentException;
import software.amazon.awssdk.services.iam.model.NoSuchEntityException;
import software.amazon.awssdk.services.iam.model.OpenIdIdpCommunicationErrorException;
import software.amazon.awssdk.services.iam.model.OrganizationNotFoundException;
import software.amazon.awssdk.services.iam.model.OrganizationNotInAllFeaturesModeException;
import software.amazon.awssdk.services.iam.model.PasswordPolicyViolationException;
import software.amazon.awssdk.services.iam.model.PolicyEvaluationException;
import software.amazon.awssdk.services.iam.model.PolicyNotAttachableException;
import software.amazon.awssdk.services.iam.model.PutGroupPolicyRequest;
import software.amazon.awssdk.services.iam.model.PutGroupPolicyResponse;
import software.amazon.awssdk.services.iam.model.PutRolePermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.PutRolePermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.PutRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.PutRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.PutUserPermissionsBoundaryRequest;
import software.amazon.awssdk.services.iam.model.PutUserPermissionsBoundaryResponse;
import software.amazon.awssdk.services.iam.model.PutUserPolicyRequest;
import software.amazon.awssdk.services.iam.model.PutUserPolicyResponse;
import software.amazon.awssdk.services.iam.model.RemoveClientIdFromOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.RemoveClientIdFromOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.RemoveRoleFromInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.RemoveRoleFromInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.RemoveUserFromGroupRequest;
import software.amazon.awssdk.services.iam.model.RemoveUserFromGroupResponse;
import software.amazon.awssdk.services.iam.model.ReportGenerationLimitExceededException;
import software.amazon.awssdk.services.iam.model.ResetServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.ResetServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.ResyncMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.ResyncMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.ServiceAccessNotEnabledException;
import software.amazon.awssdk.services.iam.model.ServiceFailureException;
import software.amazon.awssdk.services.iam.model.ServiceNotSupportedException;
import software.amazon.awssdk.services.iam.model.SetDefaultPolicyVersionRequest;
import software.amazon.awssdk.services.iam.model.SetDefaultPolicyVersionResponse;
import software.amazon.awssdk.services.iam.model.SetSecurityTokenServicePreferencesRequest;
import software.amazon.awssdk.services.iam.model.SetSecurityTokenServicePreferencesResponse;
import software.amazon.awssdk.services.iam.model.SimulateCustomPolicyRequest;
import software.amazon.awssdk.services.iam.model.SimulateCustomPolicyResponse;
import software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyRequest;
import software.amazon.awssdk.services.iam.model.SimulatePrincipalPolicyResponse;
import software.amazon.awssdk.services.iam.model.TagInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.TagInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.TagMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.TagMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.TagOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.TagOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.TagPolicyRequest;
import software.amazon.awssdk.services.iam.model.TagPolicyResponse;
import software.amazon.awssdk.services.iam.model.TagRoleRequest;
import software.amazon.awssdk.services.iam.model.TagRoleResponse;
import software.amazon.awssdk.services.iam.model.TagSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.TagSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.TagServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.TagServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.TagUserRequest;
import software.amazon.awssdk.services.iam.model.TagUserResponse;
import software.amazon.awssdk.services.iam.model.UnmodifiableEntityException;
import software.amazon.awssdk.services.iam.model.UnrecognizedPublicKeyEncodingException;
import software.amazon.awssdk.services.iam.model.UntagInstanceProfileRequest;
import software.amazon.awssdk.services.iam.model.UntagInstanceProfileResponse;
import software.amazon.awssdk.services.iam.model.UntagMfaDeviceRequest;
import software.amazon.awssdk.services.iam.model.UntagMfaDeviceResponse;
import software.amazon.awssdk.services.iam.model.UntagOpenIdConnectProviderRequest;
import software.amazon.awssdk.services.iam.model.UntagOpenIdConnectProviderResponse;
import software.amazon.awssdk.services.iam.model.UntagPolicyRequest;
import software.amazon.awssdk.services.iam.model.UntagPolicyResponse;
import software.amazon.awssdk.services.iam.model.UntagRoleRequest;
import software.amazon.awssdk.services.iam.model.UntagRoleResponse;
import software.amazon.awssdk.services.iam.model.UntagSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.UntagSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.UntagServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.UntagServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.UntagUserRequest;
import software.amazon.awssdk.services.iam.model.UntagUserResponse;
import software.amazon.awssdk.services.iam.model.UpdateAccessKeyRequest;
import software.amazon.awssdk.services.iam.model.UpdateAccessKeyResponse;
import software.amazon.awssdk.services.iam.model.UpdateAccountPasswordPolicyRequest;
import software.amazon.awssdk.services.iam.model.UpdateAccountPasswordPolicyResponse;
import software.amazon.awssdk.services.iam.model.UpdateAssumeRolePolicyRequest;
import software.amazon.awssdk.services.iam.model.UpdateAssumeRolePolicyResponse;
import software.amazon.awssdk.services.iam.model.UpdateGroupRequest;
import software.amazon.awssdk.services.iam.model.UpdateGroupResponse;
import software.amazon.awssdk.services.iam.model.UpdateLoginProfileRequest;
import software.amazon.awssdk.services.iam.model.UpdateLoginProfileResponse;
import software.amazon.awssdk.services.iam.model.UpdateOpenIdConnectProviderThumbprintRequest;
import software.amazon.awssdk.services.iam.model.UpdateOpenIdConnectProviderThumbprintResponse;
import software.amazon.awssdk.services.iam.model.UpdateRoleDescriptionRequest;
import software.amazon.awssdk.services.iam.model.UpdateRoleDescriptionResponse;
import software.amazon.awssdk.services.iam.model.UpdateRoleRequest;
import software.amazon.awssdk.services.iam.model.UpdateRoleResponse;
import software.amazon.awssdk.services.iam.model.UpdateSamlProviderRequest;
import software.amazon.awssdk.services.iam.model.UpdateSamlProviderResponse;
import software.amazon.awssdk.services.iam.model.UpdateServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.UpdateServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.UpdateServiceSpecificCredentialRequest;
import software.amazon.awssdk.services.iam.model.UpdateServiceSpecificCredentialResponse;
import software.amazon.awssdk.services.iam.model.UpdateSigningCertificateRequest;
import software.amazon.awssdk.services.iam.model.UpdateSigningCertificateResponse;
import software.amazon.awssdk.services.iam.model.UpdateSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.UpdateSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.model.UpdateUserRequest;
import software.amazon.awssdk.services.iam.model.UpdateUserResponse;
import software.amazon.awssdk.services.iam.model.UploadServerCertificateRequest;
import software.amazon.awssdk.services.iam.model.UploadServerCertificateResponse;
import software.amazon.awssdk.services.iam.model.UploadSigningCertificateRequest;
import software.amazon.awssdk.services.iam.model.UploadSigningCertificateResponse;
import software.amazon.awssdk.services.iam.model.UploadSshPublicKeyRequest;
import software.amazon.awssdk.services.iam.model.UploadSshPublicKeyResponse;
import software.amazon.awssdk.services.iam.transform.AddClientIdToOpenIdConnectProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.AddRoleToInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.AddUserToGroupRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.AttachGroupPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.AttachRolePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.AttachUserPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ChangePasswordRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateAccessKeyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateAccountAliasRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateGroupRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateLoginProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateOpenIdConnectProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreatePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreatePolicyVersionRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateSamlProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateServiceLinkedRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateServiceSpecificCredentialRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateUserRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.CreateVirtualMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeactivateMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteAccessKeyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteAccountAliasRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteAccountPasswordPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteGroupPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteGroupRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteLoginProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteOpenIdConnectProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeletePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeletePolicyVersionRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteRolePermissionsBoundaryRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteRolePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteSamlProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteServerCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteServiceLinkedRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteServiceSpecificCredentialRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteSigningCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteUserPermissionsBoundaryRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteUserPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteUserRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DeleteVirtualMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DetachGroupPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DetachRolePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DetachUserPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DisableOrganizationsRootCredentialsManagementRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.DisableOrganizationsRootSessionsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.EnableMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.EnableOrganizationsRootCredentialsManagementRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.EnableOrganizationsRootSessionsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GenerateCredentialReportRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GenerateOrganizationsAccessReportRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GenerateServiceLastAccessedDetailsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetAccessKeyLastUsedRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetAccountAuthorizationDetailsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetAccountPasswordPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetAccountSummaryRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetContextKeysForCustomPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetContextKeysForPrincipalPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetCredentialReportRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetGroupPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetGroupRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetLoginProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetOpenIdConnectProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetOrganizationsAccessReportRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetPolicyVersionRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetRolePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetSamlProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetServerCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetServiceLastAccessedDetailsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetServiceLastAccessedDetailsWithEntitiesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetServiceLinkedRoleDeletionStatusRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetUserPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.GetUserRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListAccessKeysRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListAccountAliasesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListAttachedGroupPoliciesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListAttachedRolePoliciesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListAttachedUserPoliciesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListEntitiesForPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListGroupPoliciesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListGroupsForUserRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListGroupsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListInstanceProfileTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListInstanceProfilesForRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListInstanceProfilesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListMfaDeviceTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListMfaDevicesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListOpenIdConnectProviderTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListOpenIdConnectProvidersRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListOrganizationsFeaturesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListPoliciesGrantingServiceAccessRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListPoliciesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListPolicyTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListPolicyVersionsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListRolePoliciesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListRoleTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListRolesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListSamlProviderTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListSamlProvidersRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListServerCertificateTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListServerCertificatesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListServiceSpecificCredentialsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListSigningCertificatesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListSshPublicKeysRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListUserPoliciesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListUserTagsRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListUsersRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ListVirtualMfaDevicesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.PutGroupPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.PutRolePermissionsBoundaryRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.PutRolePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.PutUserPermissionsBoundaryRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.PutUserPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.RemoveClientIdFromOpenIdConnectProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.RemoveRoleFromInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.RemoveUserFromGroupRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ResetServiceSpecificCredentialRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.ResyncMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.SetDefaultPolicyVersionRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.SetSecurityTokenServicePreferencesRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.SimulateCustomPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.SimulatePrincipalPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagOpenIdConnectProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagSamlProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagServerCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.TagUserRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagMfaDeviceRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagOpenIdConnectProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagSamlProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagServerCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UntagUserRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateAccessKeyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateAccountPasswordPolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateAssumeRolePolicyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateGroupRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateLoginProfileRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateOpenIdConnectProviderThumbprintRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateRoleDescriptionRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateRoleRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateSamlProviderRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateServerCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateServiceSpecificCredentialRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateSigningCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UpdateUserRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UploadServerCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UploadSigningCertificateRequestMarshaller;
import software.amazon.awssdk.services.iam.transform.UploadSshPublicKeyRequestMarshaller;
import software.amazon.awssdk.services.iam.waiters.IamAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link IamAsyncClient}.
*
* @see IamAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultIamAsyncClient implements IamAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultIamAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.QUERY).build();
private final AsyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultIamAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Adds a new client ID (also known as audience) to the list of client IDs already registered for the specified IAM
* OpenID Connect (OIDC) provider resource.
*
*
* This operation is idempotent; it does not fail or return an error if you add an existing client ID to the
* provider.
*
*
* @param addClientIdToOpenIdConnectProviderRequest
* @return A Java Future containing the result of the AddClientIDToOpenIDConnectProvider operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.AddClientIDToOpenIDConnectProvider
* @see AWS API Documentation
*/
@Override
public CompletableFuture addClientIDToOpenIDConnectProvider(
AddClientIdToOpenIdConnectProviderRequest addClientIdToOpenIdConnectProviderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addClientIdToOpenIdConnectProviderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
addClientIdToOpenIdConnectProviderRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddClientIDToOpenIDConnectProvider");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddClientIdToOpenIdConnectProviderResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddClientIDToOpenIDConnectProvider").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AddClientIdToOpenIdConnectProviderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addClientIdToOpenIdConnectProviderRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds the specified IAM role to the specified instance profile. An instance profile can contain only one role, and
* this quota cannot be increased. You can remove the existing role and then add a different role to an instance
* profile. You must then wait for the change to appear across all of Amazon Web Services because of eventual consistency. To force the change, you must
*
* disassociate the instance profile and then associate the
* instance profile, or you can stop your instance and then restart it.
*
*
*
* The caller of this operation must be granted the PassRole
permission on the IAM role by a
* permissions policy.
*
*
*
* For more information about roles, see IAM roles in the IAM User Guide.
* For more information about instance profiles, see Using
* instance profiles in the IAM User Guide.
*
*
* @param addRoleToInstanceProfileRequest
* @return A Java Future containing the result of the AddRoleToInstanceProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - UnmodifiableEntityException The request was rejected because service-linked roles are protected
* Amazon Web Services resources. Only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.AddRoleToInstanceProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture addRoleToInstanceProfile(
AddRoleToInstanceProfileRequest addRoleToInstanceProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addRoleToInstanceProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addRoleToInstanceProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddRoleToInstanceProfile");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddRoleToInstanceProfileResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddRoleToInstanceProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AddRoleToInstanceProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addRoleToInstanceProfileRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds the specified user to the specified group.
*
*
* @param addUserToGroupRequest
* @return A Java Future containing the result of the AddUserToGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.AddUserToGroup
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture addUserToGroup(AddUserToGroupRequest addUserToGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addUserToGroupRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addUserToGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddUserToGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddUserToGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddUserToGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AddUserToGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addUserToGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Attaches the specified managed policy to the specified IAM group.
*
*
* You use this operation to attach a managed policy to a group. To embed an inline policy in a group, use PutGroupPolicy
* .
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param attachGroupPolicyRequest
* @return A Java Future containing the result of the AttachGroupPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - PolicyNotAttachableException The request failed because Amazon Web Services service role policies can
* only be attached to the service-linked role for that service.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.AttachGroupPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture attachGroupPolicy(AttachGroupPolicyRequest attachGroupPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(attachGroupPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, attachGroupPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AttachGroupPolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AttachGroupPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AttachGroupPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AttachGroupPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(attachGroupPolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Attaches the specified managed policy to the specified IAM role. When you attach a managed policy to a role, the
* managed policy becomes part of the role's permission (access) policy.
*
*
*
* You cannot use a managed policy as the role's trust policy. The role's trust policy is created at the same time
* as the role, using
* CreateRole
. You can update a role's trust policy using
* UpdateAssumerolePolicy
.
*
*
*
* Use this operation to attach a managed policy to a role. To embed an inline policy in a role, use PutRolePolicy
* . For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* @param attachRolePolicyRequest
* @return A Java Future containing the result of the AttachRolePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - UnmodifiableEntityException The request was rejected because service-linked roles are protected
* Amazon Web Services resources. Only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* - PolicyNotAttachableException The request failed because Amazon Web Services service role policies can
* only be attached to the service-linked role for that service.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.AttachRolePolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture attachRolePolicy(AttachRolePolicyRequest attachRolePolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(attachRolePolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, attachRolePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AttachRolePolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AttachRolePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AttachRolePolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AttachRolePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(attachRolePolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Attaches the specified managed policy to the specified user.
*
*
* You use this operation to attach a managed policy to a user. To embed an inline policy in a user, use PutUserPolicy
* .
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param attachUserPolicyRequest
* @return A Java Future containing the result of the AttachUserPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - PolicyNotAttachableException The request failed because Amazon Web Services service role policies can
* only be attached to the service-linked role for that service.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.AttachUserPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture attachUserPolicy(AttachUserPolicyRequest attachUserPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(attachUserPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, attachUserPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AttachUserPolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AttachUserPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AttachUserPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AttachUserPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(attachUserPolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Changes the password of the IAM user who is calling this operation. This operation can be performed using the
* CLI, the Amazon Web Services API, or the My Security Credentials page in the Amazon Web Services
* Management Console. The Amazon Web Services account root user password is not affected by this operation.
*
*
* Use UpdateLoginProfile to use the CLI, the Amazon Web Services API, or the Users page in the IAM
* console to change the password for any IAM user. For more information about modifying passwords, see Managing passwords in the
* IAM User Guide.
*
*
* @param changePasswordRequest
* @return A Java Future containing the result of the ChangePassword operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - InvalidUserTypeException The request was rejected because the type of user for the transaction was
* incorrect.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - EntityTemporarilyUnmodifiableException The request was rejected because it referenced an entity that
* is temporarily unmodifiable, such as a user name that was deleted and then recreated. The error indicates
* that the request is likely to succeed if you try again after waiting several minutes. The error message
* describes the entity.
* - PasswordPolicyViolationException The request was rejected because the provided password did not meet
* the requirements imposed by the account password policy.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.ChangePassword
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture changePassword(ChangePasswordRequest changePasswordRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(changePasswordRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, changePasswordRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ChangePassword");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ChangePasswordResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ChangePassword").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ChangePasswordRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(changePasswordRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new Amazon Web Services secret access key and corresponding Amazon Web Services access key ID for the
* specified user. The default status for new keys is Active
.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials. This is
* true even if the Amazon Web Services account has no associated users.
*
*
* For information about quotas on the number of keys you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* To ensure the security of your Amazon Web Services account, the secret access key is accessible only during key
* and user creation. You must save the key (for example, in a text file) if you want to be able to access it again.
* If a secret key is lost, you can delete the access keys for the associated user and then create new keys.
*
*
*
* @param createAccessKeyRequest
* @return A Java Future containing the result of the CreateAccessKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateAccessKey
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createAccessKey(CreateAccessKeyRequest createAccessKeyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAccessKeyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAccessKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAccessKey");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateAccessKeyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAccessKey").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAccessKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAccessKeyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an alias for your Amazon Web Services account. For information about using an Amazon Web Services account
* alias, see Creating,
* deleting, and listing an Amazon Web Services account alias in the Amazon Web Services Sign-In User
* Guide.
*
*
* @param createAccountAliasRequest
* @return A Java Future containing the result of the CreateAccountAlias operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateAccountAlias
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createAccountAlias(CreateAccountAliasRequest createAccountAliasRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAccountAliasRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAccountAliasRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAccountAlias");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateAccountAliasResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAccountAlias").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAccountAliasRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAccountAliasRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new group.
*
*
* For information about the number of groups you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @param createGroupRequest
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateGroup
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createGroup(CreateGroupRequest createGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createGroupRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new instance profile. For information about instance profiles, see Using roles for
* applications on Amazon EC2 in the IAM User Guide, and Instance profiles in the Amazon EC2 User Guide.
*
*
* For information about the number of instance profiles you can create, see IAM object quotas in the
* IAM User Guide.
*
*
* @param createInstanceProfileRequest
* @return A Java Future containing the result of the CreateInstanceProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateInstanceProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createInstanceProfile(
CreateInstanceProfileRequest createInstanceProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createInstanceProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createInstanceProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateInstanceProfile");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateInstanceProfileResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateInstanceProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateInstanceProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createInstanceProfileRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a password for the specified IAM user. A password allows an IAM user to access Amazon Web Services
* services through the Amazon Web Services Management Console.
*
*
* You can use the CLI, the Amazon Web Services API, or the Users page in the IAM console to create a
* password for any IAM user. Use ChangePassword to update your own existing password in the My Security
* Credentials page in the Amazon Web Services Management Console.
*
*
* For more information about managing passwords, see Managing passwords in the
* IAM User Guide.
*
*
* @param createLoginProfileRequest
* @return A Java Future containing the result of the CreateLoginProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - PasswordPolicyViolationException The request was rejected because the provided password did not meet
* the requirements imposed by the account password policy.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateLoginProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createLoginProfile(CreateLoginProfileRequest createLoginProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLoginProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLoginProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLoginProfile");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateLoginProfileResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLoginProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateLoginProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createLoginProfileRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an IAM entity to describe an identity provider (IdP) that supports OpenID Connect (OIDC).
*
*
* The OIDC provider that you create with this operation can be used as a principal in a role's trust policy. Such a
* policy establishes a trust relationship between Amazon Web Services and the OIDC provider.
*
*
* If you are using an OIDC identity provider from Google, Facebook, or Amazon Cognito, you don't need to create a
* separate IAM identity provider. These OIDC identity providers are already built-in to Amazon Web Services and are
* available for your use. Instead, you can move directly to creating new roles using your identity provider. To
* learn more, see Creating a role for web
* identity or OpenID connect federation in the IAM User Guide.
*
*
* When you create the IAM OIDC provider, you specify the following:
*
*
* -
*
* The URL of the OIDC identity provider (IdP) to trust
*
*
* -
*
* A list of client IDs (also known as audiences) that identify the application or applications allowed to
* authenticate using the OIDC provider
*
*
* -
*
* A list of tags that are attached to the specified IAM OIDC provider
*
*
* -
*
* A list of thumbprints of one or more server certificates that the IdP uses
*
*
*
*
* You get all of this information from the OIDC IdP you want to use to access Amazon Web Services.
*
*
*
* Amazon Web Services secures communication with OIDC identity providers (IdPs) using our library of trusted root
* certificate authorities (CAs) to verify the JSON Web Key Set (JWKS) endpoint's TLS certificate. If your OIDC IdP
* relies on a certificate that is not signed by one of these trusted CAs, only then we secure communication using
* the thumbprints set in the IdP's configuration.
*
*
*
* The trust for the OIDC provider is derived from the IAM provider that this operation creates. Therefore, it is
* best to limit access to the CreateOpenIDConnectProvider operation to highly privileged users.
*
*
*
* @param createOpenIdConnectProviderRequest
* @return A Java Future containing the result of the CreateOpenIDConnectProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - OpenIdIdpCommunicationErrorException The request failed because IAM cannot connect to the OpenID
* Connect identity provider URL.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateOpenIDConnectProvider
* @see AWS API Documentation
*/
@Override
public CompletableFuture createOpenIDConnectProvider(
CreateOpenIdConnectProviderRequest createOpenIdConnectProviderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createOpenIdConnectProviderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createOpenIdConnectProviderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateOpenIDConnectProvider");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateOpenIdConnectProviderResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateOpenIDConnectProvider").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateOpenIdConnectProviderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createOpenIdConnectProviderRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new managed policy for your Amazon Web Services account.
*
*
* This operation creates a policy version with a version identifier of v1
and sets v1 as the policy's
* default version. For more information about policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
* As a best practice, you can validate your IAM policies. To learn more, see Validating IAM
* policies in the IAM User Guide.
*
*
* For more information about managed policies in general, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param createPolicyRequest
* @return A Java Future containing the result of the CreatePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - MalformedPolicyDocumentException The request was rejected because the policy document was malformed.
* The error message describes the specific error.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreatePolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createPolicy(CreatePolicyRequest createPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createPolicyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreatePolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreatePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreatePolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreatePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createPolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new version of the specified managed policy. To update a managed policy, you create a new policy
* version. A managed policy can have up to five versions. If the policy has five versions, you must delete an
* existing version using DeletePolicyVersion before you create a new version.
*
*
* Optionally, you can set the new version as the policy's default version. The default version is the version that
* is in effect for the IAM users, groups, and roles to which the policy is attached.
*
*
* For more information about managed policy versions, see Versioning for managed
* policies in the IAM User Guide.
*
*
* @param createPolicyVersionRequest
* @return A Java Future containing the result of the CreatePolicyVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - MalformedPolicyDocumentException The request was rejected because the policy document was malformed.
* The error message describes the specific error.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreatePolicyVersion
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createPolicyVersion(
CreatePolicyVersionRequest createPolicyVersionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createPolicyVersionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createPolicyVersionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreatePolicyVersion");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreatePolicyVersionResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreatePolicyVersion").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreatePolicyVersionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createPolicyVersionRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new role for your Amazon Web Services account.
*
*
* For more information about roles, see IAM roles in the IAM User Guide.
* For information about quotas for role names and the number of roles you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @param createRoleRequest
* @return A Java Future containing the result of the CreateRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - MalformedPolicyDocumentException The request was rejected because the policy document was malformed.
* The error message describes the specific error.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateRole
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createRole(CreateRoleRequest createRoleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createRoleRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRoleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRole");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateRoleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateRole")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateRoleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createRoleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an IAM resource that describes an identity provider (IdP) that supports SAML 2.0.
*
*
* The SAML provider resource that you create with this operation can be used as a principal in an IAM role's trust
* policy. Such a policy can enable federated users who sign in using the SAML IdP to assume the role. You can
* create an IAM role that supports Web-based single sign-on (SSO) to the Amazon Web Services Management Console or
* one that supports API access to Amazon Web Services.
*
*
* When you create the SAML provider resource, you upload a SAML metadata document that you get from your IdP. That
* document includes the issuer's name, expiration information, and keys that can be used to validate the SAML
* authentication response (assertions) that the IdP sends. You must generate the metadata document using the
* identity management software that is used as your organization's IdP.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* For more information, see Enabling SAML
* 2.0 federated users to access the Amazon Web Services Management Console and About SAML 2.0-based
* federation in the IAM User Guide.
*
*
* @param createSamlProviderRequest
* @return A Java Future containing the result of the CreateSAMLProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateSAMLProvider
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createSAMLProvider(CreateSamlProviderRequest createSamlProviderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSamlProviderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSamlProviderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSAMLProvider");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateSamlProviderResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSAMLProvider").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateSamlProviderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createSamlProviderRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an IAM role that is linked to a specific Amazon Web Services service. The service controls the attached
* policies and when the role can be deleted. This helps ensure that the service is not broken by an unexpectedly
* changed or deleted role, which could put your Amazon Web Services resources into an unknown state. Allowing the
* service to control the role helps improve service stability and proper cleanup when a service and its role are no
* longer needed. For more information, see Using service-linked
* roles in the IAM User Guide.
*
*
* To attach a policy to this service-linked role, you must make the request using the Amazon Web Services service
* that depends on this role.
*
*
* @param createServiceLinkedRoleRequest
* @return A Java Future containing the result of the CreateServiceLinkedRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateServiceLinkedRole
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createServiceLinkedRole(
CreateServiceLinkedRoleRequest createServiceLinkedRoleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createServiceLinkedRoleRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createServiceLinkedRoleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateServiceLinkedRole");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateServiceLinkedRoleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateServiceLinkedRole").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateServiceLinkedRoleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createServiceLinkedRoleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Generates a set of credentials consisting of a user name and password that can be used to access the service
* specified in the request. These credentials are generated by IAM, and can be used only for the specified service.
*
*
* You can have a maximum of two sets of service-specific credentials for each supported service per user.
*
*
* You can create service-specific credentials for CodeCommit and Amazon Keyspaces (for Apache Cassandra).
*
*
* You can reset the password to a new service-generated value by calling ResetServiceSpecificCredential.
*
*
* For more information about service-specific credentials, see Using IAM with CodeCommit:
* Git credentials, SSH keys, and Amazon Web Services access keys in the IAM User Guide.
*
*
* @param createServiceSpecificCredentialRequest
* @return A Java Future containing the result of the CreateServiceSpecificCredential operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - ServiceNotSupportedException The specified service does not support service-specific credentials.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateServiceSpecificCredential
* @see AWS API Documentation
*/
@Override
public CompletableFuture createServiceSpecificCredential(
CreateServiceSpecificCredentialRequest createServiceSpecificCredentialRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createServiceSpecificCredentialRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createServiceSpecificCredentialRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateServiceSpecificCredential");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateServiceSpecificCredentialResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateServiceSpecificCredential").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateServiceSpecificCredentialRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createServiceSpecificCredentialRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new IAM user for your Amazon Web Services account.
*
*
* For information about quotas for the number of IAM users you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createUser(CreateUserRequest createUserRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUser");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateUserResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateUser")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createUserRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new virtual MFA device for the Amazon Web Services account. After creating the virtual MFA, use
* EnableMFADevice to attach the MFA device to an IAM user. For more information about creating and working
* with virtual MFA devices, see Using a virtual MFA device in
* the IAM User Guide.
*
*
* For information about the maximum number of MFA devices you can create, see IAM and STS quotas in the
* IAM User Guide.
*
*
*
* The seed information contained in the QR code and the Base32 string should be treated like any other secret
* access information. In other words, protect the seed information as you would your Amazon Web Services access
* keys or your passwords. After you provision your virtual device, you should ensure that the information is
* destroyed following secure procedures.
*
*
*
* @param createVirtualMfaDeviceRequest
* @return A Java Future containing the result of the CreateVirtualMFADevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - EntityAlreadyExistsException The request was rejected because it attempted to create a resource that
* already exists.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.CreateVirtualMFADevice
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createVirtualMFADevice(
CreateVirtualMfaDeviceRequest createVirtualMfaDeviceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createVirtualMfaDeviceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVirtualMfaDeviceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVirtualMFADevice");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateVirtualMfaDeviceResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateVirtualMFADevice").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateVirtualMfaDeviceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createVirtualMfaDeviceRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deactivates the specified MFA device and removes it from association with the user name for which it was
* originally enabled.
*
*
* For more information about creating and working with virtual MFA devices, see Enabling a virtual multi-factor
* authentication (MFA) device in the IAM User Guide.
*
*
* @param deactivateMfaDeviceRequest
* @return A Java Future containing the result of the DeactivateMFADevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityTemporarilyUnmodifiableException The request was rejected because it referenced an entity that
* is temporarily unmodifiable, such as a user name that was deleted and then recreated. The error indicates
* that the request is likely to succeed if you try again after waiting several minutes. The error message
* describes the entity.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeactivateMFADevice
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deactivateMFADevice(
DeactivateMfaDeviceRequest deactivateMfaDeviceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deactivateMfaDeviceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deactivateMfaDeviceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeactivateMFADevice");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeactivateMfaDeviceResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeactivateMFADevice").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeactivateMfaDeviceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deactivateMfaDeviceRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the access key pair associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the Amazon Web Services
* access key ID signing the request. This operation works for access keys under the Amazon Web Services account.
* Consequently, you can use this operation to manage Amazon Web Services account root user credentials even if the
* Amazon Web Services account has no associated users.
*
*
* @param deleteAccessKeyRequest
* @return A Java Future containing the result of the DeleteAccessKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteAccessKey
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAccessKey(DeleteAccessKeyRequest deleteAccessKeyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAccessKeyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAccessKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccessKey");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteAccessKeyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccessKey").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAccessKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAccessKeyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified Amazon Web Services account alias. For information about using an Amazon Web Services
* account alias, see Creating, deleting, and
* listing an Amazon Web Services account alias in the Amazon Web Services Sign-In User Guide.
*
*
* @param deleteAccountAliasRequest
* @return A Java Future containing the result of the DeleteAccountAlias operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteAccountAlias
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAccountAlias(DeleteAccountAliasRequest deleteAccountAliasRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAccountAliasRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAccountAliasRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccountAlias");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteAccountAliasResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccountAlias").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAccountAliasRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAccountAliasRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the password policy for the Amazon Web Services account. There are no parameters.
*
*
* @param deleteAccountPasswordPolicyRequest
* @return A Java Future containing the result of the DeleteAccountPasswordPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteAccountPasswordPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteAccountPasswordPolicy(
DeleteAccountPasswordPolicyRequest deleteAccountPasswordPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAccountPasswordPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAccountPasswordPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccountPasswordPolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteAccountPasswordPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccountPasswordPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAccountPasswordPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAccountPasswordPolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified IAM group. The group must not contain any users or have any attached policies.
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - DeleteConflictException The request was rejected because it attempted to delete a resource that has
* attached subordinate entities. The error message describes these entities.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteGroup
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteGroup(DeleteGroupRequest deleteGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteGroupRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM group.
*
*
* A group can also have managed policies attached to it. To detach a managed policy from a group, use
* DetachGroupPolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param deleteGroupPolicyRequest
* @return A Java Future containing the result of the DeleteGroupPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteGroupPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteGroupPolicy(DeleteGroupPolicyRequest deleteGroupPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteGroupPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGroupPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGroupPolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteGroupPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteGroupPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteGroupPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteGroupPolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified instance profile. The instance profile must not have an associated role.
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the instance profile you are about to
* delete. Deleting a role or instance profile that is associated with a running instance will break any
* applications running on the instance.
*
*
*
* For more information about instance profiles, see Using
* instance profiles in the IAM User Guide.
*
*
* @param deleteInstanceProfileRequest
* @return A Java Future containing the result of the DeleteInstanceProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - DeleteConflictException The request was rejected because it attempted to delete a resource that has
* attached subordinate entities. The error message describes these entities.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteInstanceProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteInstanceProfile(
DeleteInstanceProfileRequest deleteInstanceProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteInstanceProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInstanceProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInstanceProfile");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteInstanceProfileResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteInstanceProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteInstanceProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteInstanceProfileRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the password for the specified IAM user, For more information, see Managing
* passwords for IAM users.
*
*
* You can use the CLI, the Amazon Web Services API, or the Users page in the IAM console to delete a
* password for any IAM user. You can use ChangePassword to update, but not delete, your own password in the
* My Security Credentials page in the Amazon Web Services Management Console.
*
*
*
* Deleting a user's password does not prevent a user from accessing Amazon Web Services through the command line
* interface or the API. To prevent all user access, you must also either make any access keys inactive or delete
* them. For more information about making keys inactive or deleting them, see UpdateAccessKey and
* DeleteAccessKey.
*
*
*
* @param deleteLoginProfileRequest
* @return A Java Future containing the result of the DeleteLoginProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EntityTemporarilyUnmodifiableException The request was rejected because it referenced an entity that
* is temporarily unmodifiable, such as a user name that was deleted and then recreated. The error indicates
* that the request is likely to succeed if you try again after waiting several minutes. The error message
* describes the entity.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteLoginProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteLoginProfile(DeleteLoginProfileRequest deleteLoginProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteLoginProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLoginProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLoginProfile");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteLoginProfileResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLoginProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteLoginProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteLoginProfileRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an OpenID Connect identity provider (IdP) resource object in IAM.
*
*
* Deleting an IAM OIDC provider resource does not update any roles that reference the provider as a principal in
* their trust policies. Any attempt to assume a role that references a deleted provider fails.
*
*
* This operation is idempotent; it does not fail or return an error if you call the operation for a provider that
* does not exist.
*
*
* @param deleteOpenIdConnectProviderRequest
* @return A Java Future containing the result of the DeleteOpenIDConnectProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteOpenIDConnectProvider
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteOpenIDConnectProvider(
DeleteOpenIdConnectProviderRequest deleteOpenIdConnectProviderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteOpenIdConnectProviderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteOpenIdConnectProviderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteOpenIDConnectProvider");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteOpenIdConnectProviderResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteOpenIDConnectProvider").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteOpenIdConnectProviderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteOpenIdConnectProviderRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified managed policy.
*
*
* Before you can delete a managed policy, you must first detach the policy from all users, groups, and roles that
* it is attached to. In addition, you must delete all the policy's versions. The following steps describe the
* process for deleting a managed policy:
*
*
* -
*
* Detach the policy from all users, groups, and roles that the policy is attached to, using
* DetachUserPolicy, DetachGroupPolicy, or DetachRolePolicy. To list all the users, groups, and
* roles that a policy is attached to, use ListEntitiesForPolicy.
*
*
* -
*
* Delete all versions of the policy using DeletePolicyVersion. To list the policy's versions, use
* ListPolicyVersions. You cannot use DeletePolicyVersion to delete the version that is marked as the
* default version. You delete the policy's default version in the next step of the process.
*
*
* -
*
* Delete the policy (this automatically deletes the policy's default version) using this operation.
*
*
*
*
* For information about managed policies, see Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param deletePolicyRequest
* @return A Java Future containing the result of the DeletePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - DeleteConflictException The request was rejected because it attempted to delete a resource that has
* attached subordinate entities. The error message describes these entities.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeletePolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deletePolicy(DeletePolicyRequest deletePolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePolicyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeletePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeletePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deletePolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified version from the specified managed policy.
*
*
* You cannot delete the default version from a policy using this operation. To delete the default version from a
* policy, use DeletePolicy. To find out which version of a policy is marked as the default version, use
* ListPolicyVersions.
*
*
* For information about versions for managed policies, see Versioning for managed
* policies in the IAM User Guide.
*
*
* @param deletePolicyVersionRequest
* @return A Java Future containing the result of the DeletePolicyVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - DeleteConflictException The request was rejected because it attempted to delete a resource that has
* attached subordinate entities. The error message describes these entities.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeletePolicyVersion
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deletePolicyVersion(
DeletePolicyVersionRequest deletePolicyVersionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePolicyVersionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePolicyVersionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePolicyVersion");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeletePolicyVersionResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePolicyVersion").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeletePolicyVersionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deletePolicyVersionRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified role. Unlike the Amazon Web Services Management Console, when you delete a role
* programmatically, you must delete the items attached to the role manually, or the deletion fails. For more
* information, see Deleting an IAM role. Before attempting to delete a role, remove the following attached items:
*
*
* -
*
* Inline policies (DeleteRolePolicy)
*
*
* -
*
* Attached managed policies (DetachRolePolicy)
*
*
* -
*
* Instance profile (RemoveRoleFromInstanceProfile)
*
*
* -
*
* Optional – Delete instance profile after detaching from role for resource clean up (DeleteInstanceProfile)
*
*
*
*
*
* Make sure that you do not have any Amazon EC2 instances running with the role you are about to delete. Deleting a
* role or instance profile that is associated with a running instance will break any applications running on the
* instance.
*
*
*
* @param deleteRoleRequest
* @return A Java Future containing the result of the DeleteRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - DeleteConflictException The request was rejected because it attempted to delete a resource that has
* attached subordinate entities. The error message describes these entities.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - UnmodifiableEntityException The request was rejected because service-linked roles are protected
* Amazon Web Services resources. Only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* - ConcurrentModificationException The request was rejected because multiple requests to change this
* object were submitted simultaneously. Wait a few minutes and submit your request again.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteRole
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteRole(DeleteRoleRequest deleteRoleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteRoleRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRoleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRole");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteRoleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteRole")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteRoleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRoleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the permissions boundary for the specified IAM role.
*
*
* You cannot set the boundary for a service-linked role.
*
*
*
* Deleting the permissions boundary for a role might increase its permissions. For example, it might allow anyone
* who assumes the role to perform all the actions granted in its permissions policies.
*
*
*
* @param deleteRolePermissionsBoundaryRequest
* @return A Java Future containing the result of the DeleteRolePermissionsBoundary operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - UnmodifiableEntityException The request was rejected because service-linked roles are protected
* Amazon Web Services resources. Only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteRolePermissionsBoundary
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteRolePermissionsBoundary(
DeleteRolePermissionsBoundaryRequest deleteRolePermissionsBoundaryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteRolePermissionsBoundaryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteRolePermissionsBoundaryRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRolePermissionsBoundary");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteRolePermissionsBoundaryResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRolePermissionsBoundary").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteRolePermissionsBoundaryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRolePermissionsBoundaryRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM role.
*
*
* A role can also have managed policies attached to it. To detach a managed policy from a role, use
* DetachRolePolicy. For more information about policies, refer to Managed policies and
* inline policies in the IAM User Guide.
*
*
* @param deleteRolePolicyRequest
* @return A Java Future containing the result of the DeleteRolePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - UnmodifiableEntityException The request was rejected because service-linked roles are protected
* Amazon Web Services resources. Only the service that depends on the service-linked role can modify or
* delete the role on your behalf. The error message includes the name of the service that depends on this
* service-linked role. You must request the change through that service.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteRolePolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteRolePolicy(DeleteRolePolicyRequest deleteRolePolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteRolePolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRolePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRolePolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteRolePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRolePolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteRolePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRolePolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a SAML provider resource in IAM.
*
*
* Deleting the provider resource from IAM does not update any roles that reference the SAML provider resource's ARN
* as a principal in their trust policies. Any attempt to assume a role that references a non-existent provider
* resource ARN fails.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param deleteSamlProviderRequest
* @return A Java Future containing the result of the DeleteSAMLProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidInputException The request was rejected because an invalid or out-of-range value was supplied
* for an input parameter.
* - LimitExceededException The request was rejected because it attempted to create resources beyond the
* current Amazon Web Services account limits. The error message describes the limit exceeded.
* - NoSuchEntityException The request was rejected because it referenced a resource entity that does not
* exist. The error message describes the resource.
* - ServiceFailureException The request processing has failed because of an unknown error, exception or
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IamAsyncClient.DeleteSAMLProvider
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteSAMLProvider(DeleteSamlProviderRequest deleteSamlProviderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteSamlProviderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSamlProviderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IAM");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSAMLProvider");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteSamlProviderResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams