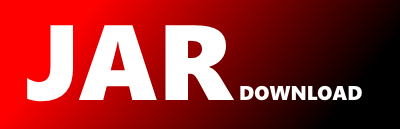
software.amazon.awssdk.services.iam.model.PolicyVersion Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about a version of a managed policy.
*
*
* This data type is used as a response element in the CreatePolicyVersion, GetPolicyVersion,
* ListPolicyVersions, and GetAccountAuthorizationDetails operations.
*
*
* For more information about managed policies, refer to Managed policies and inline
* policies in the IAM User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PolicyVersion implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DOCUMENT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Document").getter(getter(PolicyVersion::document)).setter(setter(Builder::document))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Document").build()).build();
private static final SdkField VERSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionId").getter(getter(PolicyVersion::versionId)).setter(setter(Builder::versionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionId").build()).build();
private static final SdkField IS_DEFAULT_VERSION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IsDefaultVersion").getter(getter(PolicyVersion::isDefaultVersion))
.setter(setter(Builder::isDefaultVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsDefaultVersion").build()).build();
private static final SdkField CREATE_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateDate").getter(getter(PolicyVersion::createDate)).setter(setter(Builder::createDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateDate").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOCUMENT_FIELD,
VERSION_ID_FIELD, IS_DEFAULT_VERSION_FIELD, CREATE_DATE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String document;
private final String versionId;
private final Boolean isDefaultVersion;
private final Instant createDate;
private PolicyVersion(BuilderImpl builder) {
this.document = builder.document;
this.versionId = builder.versionId;
this.isDefaultVersion = builder.isDefaultVersion;
this.createDate = builder.createDate;
}
/**
*
* The policy document.
*
*
* The policy document is returned in the response to the GetPolicyVersion and
* GetAccountAuthorizationDetails operations. It is not returned in the response to the
* CreatePolicyVersion or ListPolicyVersions operations.
*
*
* The policy document returned in this structure is URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the policy
* back to plain JSON text. For example, if you use Java, you can use the decode
method of the
* java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide similar
* functionality.
*
*
* @return The policy document.
*
* The policy document is returned in the response to the GetPolicyVersion and
* GetAccountAuthorizationDetails operations. It is not returned in the response to the
* CreatePolicyVersion or ListPolicyVersions operations.
*
*
* The policy document returned in this structure is URL-encoded compliant with RFC 3986. You can use a URL decoding method to convert the
* policy back to plain JSON text. For example, if you use Java, you can use the decode
method
* of the java.net.URLDecoder
utility class in the Java SDK. Other languages and SDKs provide
* similar functionality.
*/
public final String document() {
return document;
}
/**
*
* The identifier for the policy version.
*
*
* Policy version identifiers always begin with v
(always lowercase). When a policy is created, the
* first policy version is v1
.
*
*
* @return The identifier for the policy version.
*
* Policy version identifiers always begin with v
(always lowercase). When a policy is created,
* the first policy version is v1
.
*/
public final String versionId() {
return versionId;
}
/**
*
* Specifies whether the policy version is set as the policy's default version.
*
*
* @return Specifies whether the policy version is set as the policy's default version.
*/
public final Boolean isDefaultVersion() {
return isDefaultVersion;
}
/**
*
* The date and time, in ISO 8601 date-time format, when the policy
* version was created.
*
*
* @return The date and time, in ISO 8601 date-time format, when the
* policy version was created.
*/
public final Instant createDate() {
return createDate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(document());
hashCode = 31 * hashCode + Objects.hashCode(versionId());
hashCode = 31 * hashCode + Objects.hashCode(isDefaultVersion());
hashCode = 31 * hashCode + Objects.hashCode(createDate());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PolicyVersion)) {
return false;
}
PolicyVersion other = (PolicyVersion) obj;
return Objects.equals(document(), other.document()) && Objects.equals(versionId(), other.versionId())
&& Objects.equals(isDefaultVersion(), other.isDefaultVersion())
&& Objects.equals(createDate(), other.createDate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PolicyVersion").add("Document", document()).add("VersionId", versionId())
.add("IsDefaultVersion", isDefaultVersion()).add("CreateDate", createDate()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Document":
return Optional.ofNullable(clazz.cast(document()));
case "VersionId":
return Optional.ofNullable(clazz.cast(versionId()));
case "IsDefaultVersion":
return Optional.ofNullable(clazz.cast(isDefaultVersion()));
case "CreateDate":
return Optional.ofNullable(clazz.cast(createDate()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Document", DOCUMENT_FIELD);
map.put("VersionId", VERSION_ID_FIELD);
map.put("IsDefaultVersion", IS_DEFAULT_VERSION_FIELD);
map.put("CreateDate", CREATE_DATE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function