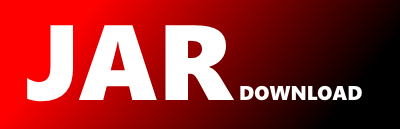
software.amazon.awssdk.services.iam.model.CreateRoleRequest Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateRoleRequest extends IamRequest implements
ToCopyableBuilder {
private static final SdkField PATH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Path")
.getter(getter(CreateRoleRequest::path)).setter(setter(Builder::path))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Path").build()).build();
private static final SdkField ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleName").getter(getter(CreateRoleRequest::roleName)).setter(setter(Builder::roleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleName").build()).build();
private static final SdkField ASSUME_ROLE_POLICY_DOCUMENT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AssumeRolePolicyDocument").getter(getter(CreateRoleRequest::assumeRolePolicyDocument))
.setter(setter(Builder::assumeRolePolicyDocument))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssumeRolePolicyDocument").build())
.build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateRoleRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField MAX_SESSION_DURATION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxSessionDuration").getter(getter(CreateRoleRequest::maxSessionDuration))
.setter(setter(Builder::maxSessionDuration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxSessionDuration").build())
.build();
private static final SdkField PERMISSIONS_BOUNDARY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PermissionsBoundary").getter(getter(CreateRoleRequest::permissionsBoundary))
.setter(setter(Builder::permissionsBoundary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PermissionsBoundary").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateRoleRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PATH_FIELD, ROLE_NAME_FIELD,
ASSUME_ROLE_POLICY_DOCUMENT_FIELD, DESCRIPTION_FIELD, MAX_SESSION_DURATION_FIELD, PERMISSIONS_BOUNDARY_FIELD,
TAGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String path;
private final String roleName;
private final String assumeRolePolicyDocument;
private final String description;
private final Integer maxSessionDuration;
private final String permissionsBoundary;
private final List tags;
private CreateRoleRequest(BuilderImpl builder) {
super(builder);
this.path = builder.path;
this.roleName = builder.roleName;
this.assumeRolePolicyDocument = builder.assumeRolePolicyDocument;
this.description = builder.description;
this.maxSessionDuration = builder.maxSessionDuration;
this.permissionsBoundary = builder.permissionsBoundary;
this.tags = builder.tags;
}
/**
*
* The path to the role. For more information about paths, see IAM Identifiers in the IAM
* User Guide.
*
*
* This parameter is optional. If it is not included, it defaults to a slash (/).
*
*
* This parameter allows (through its regex pattern) a string of
* characters consisting of either a forward slash (/) by itself or a string that must begin and end with forward
* slashes. In addition, it can contain any ASCII character from the ! (\u0021
) through the DEL
* character (\u007F
), including most punctuation characters, digits, and upper and lowercased letters.
*
*
* @return The path to the role. For more information about paths, see IAM Identifiers in the
* IAM User Guide.
*
* This parameter is optional. If it is not included, it defaults to a slash (/).
*
*
* This parameter allows (through its regex pattern) a string
* of characters consisting of either a forward slash (/) by itself or a string that must begin and end with
* forward slashes. In addition, it can contain any ASCII character from the ! (\u0021
) through
* the DEL character (\u007F
), including most punctuation characters, digits, and upper and
* lowercased letters.
*/
public final String path() {
return path;
}
/**
*
* The name of the role to create.
*
*
* IAM user, group, role, and policy names must be unique within the account. Names are not distinguished by case.
* For example, you cannot create resources named both "MyResource" and "myresource".
*
*
* This parameter allows (through its regex pattern) a string of
* characters consisting of upper and lowercase alphanumeric characters with no spaces. You can also include any of
* the following characters: _+=,.@-
*
*
* @return The name of the role to create.
*
* IAM user, group, role, and policy names must be unique within the account. Names are not distinguished by
* case. For example, you cannot create resources named both "MyResource" and "myresource".
*
*
* This parameter allows (through its regex pattern) a string
* of characters consisting of upper and lowercase alphanumeric characters with no spaces. You can also
* include any of the following characters: _+=,.@-
*/
public final String roleName() {
return roleName;
}
/**
*
* The trust relationship policy document that grants an entity permission to assume the role.
*
*
* In IAM, you must provide a JSON policy that has been converted to a string. However, for CloudFormation templates
* formatted in YAML, you can provide the policy in JSON or YAML format. CloudFormation always converts a YAML
* policy to JSON format before submitting it to IAM.
*
*
* The regex pattern used to validate this parameter is a string of
* characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of the ASCII
* character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (\u000D
* )
*
*
*
*
* Upon success, the response includes the same trust policy in JSON format.
*
*
* @return The trust relationship policy document that grants an entity permission to assume the role.
*
* In IAM, you must provide a JSON policy that has been converted to a string. However, for CloudFormation
* templates formatted in YAML, you can provide the policy in JSON or YAML format. CloudFormation always
* converts a YAML policy to JSON format before submitting it to IAM.
*
*
* The regex pattern used to validate this parameter is a
* string of characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character (\u0020
) through the end of
* the ASCII character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through
* \u00FF
)
*
*
* -
*
* The special characters tab (\u0009
), line feed (\u000A
), and carriage return (
* \u000D
)
*
*
*
*
* Upon success, the response includes the same trust policy in JSON format.
*/
public final String assumeRolePolicyDocument() {
return assumeRolePolicyDocument;
}
/**
*
* A description of the role.
*
*
* @return A description of the role.
*/
public final String description() {
return description;
}
/**
*
* The maximum session duration (in seconds) that you want to set for the specified role. If you do not specify a
* value for this setting, the default value of one hour is applied. This setting can have a value from 1 hour to 12
* hours.
*
*
* Anyone who assumes the role from the CLI or API can use the DurationSeconds
API parameter or the
* duration-seconds
CLI parameter to request a longer session. The MaxSessionDuration
* setting determines the maximum duration that can be requested using the DurationSeconds
parameter.
* If users don't specify a value for the DurationSeconds
parameter, their security credentials are
* valid for one hour by default. This applies when you use the AssumeRole*
API operations or the
* assume-role*
CLI operations but does not apply when you use those operations to create a console
* URL. For more information, see Using
* IAM roles in the IAM User Guide.
*
*
* @return The maximum session duration (in seconds) that you want to set for the specified role. If you do not
* specify a value for this setting, the default value of one hour is applied. This setting can have a value
* from 1 hour to 12 hours.
*
* Anyone who assumes the role from the CLI or API can use the DurationSeconds
API parameter or
* the duration-seconds
CLI parameter to request a longer session. The
* MaxSessionDuration
setting determines the maximum duration that can be requested using the
* DurationSeconds
parameter. If users don't specify a value for the
* DurationSeconds
parameter, their security credentials are valid for one hour by default.
* This applies when you use the AssumeRole*
API operations or the assume-role*
* CLI operations but does not apply when you use those operations to create a console URL. For more
* information, see Using IAM
* roles in the IAM User Guide.
*/
public final Integer maxSessionDuration() {
return maxSessionDuration;
}
/**
*
* The ARN of the managed policy that is used to set the permissions boundary for the role.
*
*
* A permissions boundary policy defines the maximum permissions that identity-based policies can grant to an
* entity, but does not grant permissions. Permissions boundaries do not define the maximum permissions that a
* resource-based policy can grant to an entity. To learn more, see Permissions boundaries
* for IAM entities in the IAM User Guide.
*
*
* For more information about policy types, see Policy types
* in the IAM User Guide.
*
*
* @return The ARN of the managed policy that is used to set the permissions boundary for the role.
*
* A permissions boundary policy defines the maximum permissions that identity-based policies can grant to
* an entity, but does not grant permissions. Permissions boundaries do not define the maximum permissions
* that a resource-based policy can grant to an entity. To learn more, see Permissions
* boundaries for IAM entities in the IAM User Guide.
*
*
* For more information about policy types, see Policy
* types in the IAM User Guide.
*/
public final String permissionsBoundary() {
return permissionsBoundary;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags that you want to attach to the new role. Each tag consists of a key name and an associated value.
* For more information about tagging, see Tagging IAM resources in the IAM User
* Guide.
*
*
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire request
* fails and the resource is not created.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags that you want to attach to the new role. Each tag consists of a key name and an associated
* value. For more information about tagging, see Tagging IAM resources in the
* IAM User Guide.
*
* If any one of the tags is invalid or if you exceed the allowed maximum number of tags, then the entire
* request fails and the resource is not created.
*
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(path());
hashCode = 31 * hashCode + Objects.hashCode(roleName());
hashCode = 31 * hashCode + Objects.hashCode(assumeRolePolicyDocument());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(maxSessionDuration());
hashCode = 31 * hashCode + Objects.hashCode(permissionsBoundary());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateRoleRequest)) {
return false;
}
CreateRoleRequest other = (CreateRoleRequest) obj;
return Objects.equals(path(), other.path()) && Objects.equals(roleName(), other.roleName())
&& Objects.equals(assumeRolePolicyDocument(), other.assumeRolePolicyDocument())
&& Objects.equals(description(), other.description())
&& Objects.equals(maxSessionDuration(), other.maxSessionDuration())
&& Objects.equals(permissionsBoundary(), other.permissionsBoundary()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateRoleRequest").add("Path", path()).add("RoleName", roleName())
.add("AssumeRolePolicyDocument", assumeRolePolicyDocument()).add("Description", description())
.add("MaxSessionDuration", maxSessionDuration()).add("PermissionsBoundary", permissionsBoundary())
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Path":
return Optional.ofNullable(clazz.cast(path()));
case "RoleName":
return Optional.ofNullable(clazz.cast(roleName()));
case "AssumeRolePolicyDocument":
return Optional.ofNullable(clazz.cast(assumeRolePolicyDocument()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "MaxSessionDuration":
return Optional.ofNullable(clazz.cast(maxSessionDuration()));
case "PermissionsBoundary":
return Optional.ofNullable(clazz.cast(permissionsBoundary()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Path", PATH_FIELD);
map.put("RoleName", ROLE_NAME_FIELD);
map.put("AssumeRolePolicyDocument", ASSUME_ROLE_POLICY_DOCUMENT_FIELD);
map.put("Description", DESCRIPTION_FIELD);
map.put("MaxSessionDuration", MAX_SESSION_DURATION_FIELD);
map.put("PermissionsBoundary", PERMISSIONS_BOUNDARY_FIELD);
map.put("Tags", TAGS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function