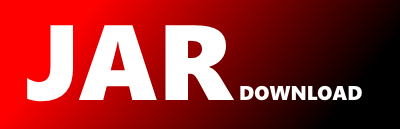
software.amazon.awssdk.services.iam.model.GetServiceLastAccessedDetailsResponse Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetServiceLastAccessedDetailsResponse extends IamResponse implements
ToCopyableBuilder {
private static final SdkField JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobStatus").getter(getter(GetServiceLastAccessedDetailsResponse::jobStatusAsString))
.setter(setter(Builder::jobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobStatus").build()).build();
private static final SdkField JOB_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobType").getter(getter(GetServiceLastAccessedDetailsResponse::jobTypeAsString))
.setter(setter(Builder::jobType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobType").build()).build();
private static final SdkField JOB_CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("JobCreationDate").getter(getter(GetServiceLastAccessedDetailsResponse::jobCreationDate))
.setter(setter(Builder::jobCreationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobCreationDate").build()).build();
private static final SdkField> SERVICES_LAST_ACCESSED_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ServicesLastAccessed")
.getter(getter(GetServiceLastAccessedDetailsResponse::servicesLastAccessed))
.setter(setter(Builder::servicesLastAccessed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServicesLastAccessed").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceLastAccessed::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField JOB_COMPLETION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("JobCompletionDate").getter(getter(GetServiceLastAccessedDetailsResponse::jobCompletionDate))
.setter(setter(Builder::jobCompletionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobCompletionDate").build()).build();
private static final SdkField IS_TRUNCATED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IsTruncated").getter(getter(GetServiceLastAccessedDetailsResponse::isTruncated))
.setter(setter(Builder::isTruncated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsTruncated").build()).build();
private static final SdkField MARKER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Marker")
.getter(getter(GetServiceLastAccessedDetailsResponse::marker)).setter(setter(Builder::marker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Marker").build()).build();
private static final SdkField ERROR_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Error").getter(getter(GetServiceLastAccessedDetailsResponse::error)).setter(setter(Builder::error))
.constructor(ErrorDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Error").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(JOB_STATUS_FIELD,
JOB_TYPE_FIELD, JOB_CREATION_DATE_FIELD, SERVICES_LAST_ACCESSED_FIELD, JOB_COMPLETION_DATE_FIELD, IS_TRUNCATED_FIELD,
MARKER_FIELD, ERROR_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String jobStatus;
private final String jobType;
private final Instant jobCreationDate;
private final List servicesLastAccessed;
private final Instant jobCompletionDate;
private final Boolean isTruncated;
private final String marker;
private final ErrorDetails error;
private GetServiceLastAccessedDetailsResponse(BuilderImpl builder) {
super(builder);
this.jobStatus = builder.jobStatus;
this.jobType = builder.jobType;
this.jobCreationDate = builder.jobCreationDate;
this.servicesLastAccessed = builder.servicesLastAccessed;
this.jobCompletionDate = builder.jobCompletionDate;
this.isTruncated = builder.isTruncated;
this.marker = builder.marker;
this.error = builder.error;
}
/**
*
* The status of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobStatus} will
* return {@link JobStatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #jobStatusAsString}.
*
*
* @return The status of the job.
* @see JobStatusType
*/
public final JobStatusType jobStatus() {
return JobStatusType.fromValue(jobStatus);
}
/**
*
* The status of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobStatus} will
* return {@link JobStatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #jobStatusAsString}.
*
*
* @return The status of the job.
* @see JobStatusType
*/
public final String jobStatusAsString() {
return jobStatus;
}
/**
*
* The type of job. Service jobs return information about when each service was last accessed. Action jobs also
* include information about when tracked actions within the service were last accessed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobType} will
* return {@link AccessAdvisorUsageGranularityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #jobTypeAsString}.
*
*
* @return The type of job. Service jobs return information about when each service was last accessed. Action jobs
* also include information about when tracked actions within the service were last accessed.
* @see AccessAdvisorUsageGranularityType
*/
public final AccessAdvisorUsageGranularityType jobType() {
return AccessAdvisorUsageGranularityType.fromValue(jobType);
}
/**
*
* The type of job. Service jobs return information about when each service was last accessed. Action jobs also
* include information about when tracked actions within the service were last accessed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobType} will
* return {@link AccessAdvisorUsageGranularityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #jobTypeAsString}.
*
*
* @return The type of job. Service jobs return information about when each service was last accessed. Action jobs
* also include information about when tracked actions within the service were last accessed.
* @see AccessAdvisorUsageGranularityType
*/
public final String jobTypeAsString() {
return jobType;
}
/**
*
* The date and time, in ISO 8601 date-time format, when the report job
* was created.
*
*
* @return The date and time, in ISO 8601 date-time format, when the
* report job was created.
*/
public final Instant jobCreationDate() {
return jobCreationDate;
}
/**
* For responses, this returns true if the service returned a value for the ServicesLastAccessed property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasServicesLastAccessed() {
return servicesLastAccessed != null && !(servicesLastAccessed instanceof SdkAutoConstructList);
}
/**
*
* A ServiceLastAccessed
object that contains details about the most recent attempt to access the
* service.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasServicesLastAccessed} method.
*
*
* @return A ServiceLastAccessed
object that contains details about the most recent attempt to access
* the service.
*/
public final List servicesLastAccessed() {
return servicesLastAccessed;
}
/**
*
* The date and time, in ISO 8601 date-time format, when the generated
* report job was completed or failed.
*
*
* This field is null if the job is still in progress, as indicated by a job status value of
* IN_PROGRESS
.
*
*
* @return The date and time, in ISO 8601 date-time format, when the
* generated report job was completed or failed.
*
* This field is null if the job is still in progress, as indicated by a job status value of
* IN_PROGRESS
.
*/
public final Instant jobCompletionDate() {
return jobCompletionDate;
}
/**
*
* A flag that indicates whether there are more items to return. If your results were truncated, you can make a
* subsequent pagination request using the Marker
request parameter to retrieve more items. Note that
* IAM might return fewer than the MaxItems
number of results even when there are more results
* available. We recommend that you check IsTruncated
after every call to ensure that you receive all
* your results.
*
*
* @return A flag that indicates whether there are more items to return. If your results were truncated, you can
* make a subsequent pagination request using the Marker
request parameter to retrieve more
* items. Note that IAM might return fewer than the MaxItems
number of results even when there
* are more results available. We recommend that you check IsTruncated
after every call to
* ensure that you receive all your results.
*/
public final Boolean isTruncated() {
return isTruncated;
}
/**
*
* When IsTruncated
is true
, this element is present and contains the value to use for the
* Marker
parameter in a subsequent pagination request.
*
*
* @return When IsTruncated
is true
, this element is present and contains the value to use
* for the Marker
parameter in a subsequent pagination request.
*/
public final String marker() {
return marker;
}
/**
*
* An object that contains details about the reason the operation failed.
*
*
* @return An object that contains details about the reason the operation failed.
*/
public final ErrorDetails error() {
return error;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(jobStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(jobTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(jobCreationDate());
hashCode = 31 * hashCode + Objects.hashCode(hasServicesLastAccessed() ? servicesLastAccessed() : null);
hashCode = 31 * hashCode + Objects.hashCode(jobCompletionDate());
hashCode = 31 * hashCode + Objects.hashCode(isTruncated());
hashCode = 31 * hashCode + Objects.hashCode(marker());
hashCode = 31 * hashCode + Objects.hashCode(error());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetServiceLastAccessedDetailsResponse)) {
return false;
}
GetServiceLastAccessedDetailsResponse other = (GetServiceLastAccessedDetailsResponse) obj;
return Objects.equals(jobStatusAsString(), other.jobStatusAsString())
&& Objects.equals(jobTypeAsString(), other.jobTypeAsString())
&& Objects.equals(jobCreationDate(), other.jobCreationDate())
&& hasServicesLastAccessed() == other.hasServicesLastAccessed()
&& Objects.equals(servicesLastAccessed(), other.servicesLastAccessed())
&& Objects.equals(jobCompletionDate(), other.jobCompletionDate())
&& Objects.equals(isTruncated(), other.isTruncated()) && Objects.equals(marker(), other.marker())
&& Objects.equals(error(), other.error());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetServiceLastAccessedDetailsResponse").add("JobStatus", jobStatusAsString())
.add("JobType", jobTypeAsString()).add("JobCreationDate", jobCreationDate())
.add("ServicesLastAccessed", hasServicesLastAccessed() ? servicesLastAccessed() : null)
.add("JobCompletionDate", jobCompletionDate()).add("IsTruncated", isTruncated()).add("Marker", marker())
.add("Error", error()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "JobStatus":
return Optional.ofNullable(clazz.cast(jobStatusAsString()));
case "JobType":
return Optional.ofNullable(clazz.cast(jobTypeAsString()));
case "JobCreationDate":
return Optional.ofNullable(clazz.cast(jobCreationDate()));
case "ServicesLastAccessed":
return Optional.ofNullable(clazz.cast(servicesLastAccessed()));
case "JobCompletionDate":
return Optional.ofNullable(clazz.cast(jobCompletionDate()));
case "IsTruncated":
return Optional.ofNullable(clazz.cast(isTruncated()));
case "Marker":
return Optional.ofNullable(clazz.cast(marker()));
case "Error":
return Optional.ofNullable(clazz.cast(error()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("JobStatus", JOB_STATUS_FIELD);
map.put("JobType", JOB_TYPE_FIELD);
map.put("JobCreationDate", JOB_CREATION_DATE_FIELD);
map.put("ServicesLastAccessed", SERVICES_LAST_ACCESSED_FIELD);
map.put("JobCompletionDate", JOB_COMPLETION_DATE_FIELD);
map.put("IsTruncated", IS_TRUNCATED_FIELD);
map.put("Marker", MARKER_FIELD);
map.put("Error", ERROR_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function