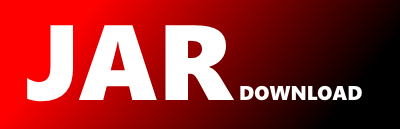
software.amazon.awssdk.services.iam.model.PasswordPolicy Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about the account password policy.
*
*
* This data type is used as a response element in the GetAccountPasswordPolicy operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PasswordPolicy implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField MINIMUM_PASSWORD_LENGTH_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(PasswordPolicy::minimumPasswordLength)).setter(setter(Builder::minimumPasswordLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinimumPasswordLength").build())
.build();
private static final SdkField REQUIRE_SYMBOLS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(PasswordPolicy::requireSymbols)).setter(setter(Builder::requireSymbols))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireSymbols").build()).build();
private static final SdkField REQUIRE_NUMBERS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(PasswordPolicy::requireNumbers)).setter(setter(Builder::requireNumbers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireNumbers").build()).build();
private static final SdkField REQUIRE_UPPERCASE_CHARACTERS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.getter(getter(PasswordPolicy::requireUppercaseCharacters))
.setter(setter(Builder::requireUppercaseCharacters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireUppercaseCharacters").build())
.build();
private static final SdkField REQUIRE_LOWERCASE_CHARACTERS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.getter(getter(PasswordPolicy::requireLowercaseCharacters))
.setter(setter(Builder::requireLowercaseCharacters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequireLowercaseCharacters").build())
.build();
private static final SdkField ALLOW_USERS_TO_CHANGE_PASSWORD_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.getter(getter(PasswordPolicy::allowUsersToChangePassword))
.setter(setter(Builder::allowUsersToChangePassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowUsersToChangePassword").build())
.build();
private static final SdkField EXPIRE_PASSWORDS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(PasswordPolicy::expirePasswords)).setter(setter(Builder::expirePasswords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpirePasswords").build()).build();
private static final SdkField MAX_PASSWORD_AGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(PasswordPolicy::maxPasswordAge)).setter(setter(Builder::maxPasswordAge))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxPasswordAge").build()).build();
private static final SdkField PASSWORD_REUSE_PREVENTION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(PasswordPolicy::passwordReusePrevention)).setter(setter(Builder::passwordReusePrevention))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PasswordReusePrevention").build())
.build();
private static final SdkField HARD_EXPIRY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(PasswordPolicy::hardExpiry)).setter(setter(Builder::hardExpiry))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HardExpiry").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MINIMUM_PASSWORD_LENGTH_FIELD,
REQUIRE_SYMBOLS_FIELD, REQUIRE_NUMBERS_FIELD, REQUIRE_UPPERCASE_CHARACTERS_FIELD, REQUIRE_LOWERCASE_CHARACTERS_FIELD,
ALLOW_USERS_TO_CHANGE_PASSWORD_FIELD, EXPIRE_PASSWORDS_FIELD, MAX_PASSWORD_AGE_FIELD,
PASSWORD_REUSE_PREVENTION_FIELD, HARD_EXPIRY_FIELD));
private static final long serialVersionUID = 1L;
private final Integer minimumPasswordLength;
private final Boolean requireSymbols;
private final Boolean requireNumbers;
private final Boolean requireUppercaseCharacters;
private final Boolean requireLowercaseCharacters;
private final Boolean allowUsersToChangePassword;
private final Boolean expirePasswords;
private final Integer maxPasswordAge;
private final Integer passwordReusePrevention;
private final Boolean hardExpiry;
private PasswordPolicy(BuilderImpl builder) {
this.minimumPasswordLength = builder.minimumPasswordLength;
this.requireSymbols = builder.requireSymbols;
this.requireNumbers = builder.requireNumbers;
this.requireUppercaseCharacters = builder.requireUppercaseCharacters;
this.requireLowercaseCharacters = builder.requireLowercaseCharacters;
this.allowUsersToChangePassword = builder.allowUsersToChangePassword;
this.expirePasswords = builder.expirePasswords;
this.maxPasswordAge = builder.maxPasswordAge;
this.passwordReusePrevention = builder.passwordReusePrevention;
this.hardExpiry = builder.hardExpiry;
}
/**
*
* Minimum length to require for IAM user passwords.
*
*
* @return Minimum length to require for IAM user passwords.
*/
public Integer minimumPasswordLength() {
return minimumPasswordLength;
}
/**
*
* Specifies whether to require symbols for IAM user passwords.
*
*
* @return Specifies whether to require symbols for IAM user passwords.
*/
public Boolean requireSymbols() {
return requireSymbols;
}
/**
*
* Specifies whether to require numbers for IAM user passwords.
*
*
* @return Specifies whether to require numbers for IAM user passwords.
*/
public Boolean requireNumbers() {
return requireNumbers;
}
/**
*
* Specifies whether to require uppercase characters for IAM user passwords.
*
*
* @return Specifies whether to require uppercase characters for IAM user passwords.
*/
public Boolean requireUppercaseCharacters() {
return requireUppercaseCharacters;
}
/**
*
* Specifies whether to require lowercase characters for IAM user passwords.
*
*
* @return Specifies whether to require lowercase characters for IAM user passwords.
*/
public Boolean requireLowercaseCharacters() {
return requireLowercaseCharacters;
}
/**
*
* Specifies whether IAM users are allowed to change their own password.
*
*
* @return Specifies whether IAM users are allowed to change their own password.
*/
public Boolean allowUsersToChangePassword() {
return allowUsersToChangePassword;
}
/**
*
* Indicates whether passwords in the account expire. Returns true if MaxPasswordAge
contains a value
* greater than 0. Returns false if MaxPasswordAge is 0 or not present.
*
*
* @return Indicates whether passwords in the account expire. Returns true if MaxPasswordAge
contains a
* value greater than 0. Returns false if MaxPasswordAge is 0 or not present.
*/
public Boolean expirePasswords() {
return expirePasswords;
}
/**
*
* The number of days that an IAM user password is valid.
*
*
* @return The number of days that an IAM user password is valid.
*/
public Integer maxPasswordAge() {
return maxPasswordAge;
}
/**
*
* Specifies the number of previous passwords that IAM users are prevented from reusing.
*
*
* @return Specifies the number of previous passwords that IAM users are prevented from reusing.
*/
public Integer passwordReusePrevention() {
return passwordReusePrevention;
}
/**
*
* Specifies whether IAM users are prevented from setting a new password after their password has expired.
*
*
* @return Specifies whether IAM users are prevented from setting a new password after their password has expired.
*/
public Boolean hardExpiry() {
return hardExpiry;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(minimumPasswordLength());
hashCode = 31 * hashCode + Objects.hashCode(requireSymbols());
hashCode = 31 * hashCode + Objects.hashCode(requireNumbers());
hashCode = 31 * hashCode + Objects.hashCode(requireUppercaseCharacters());
hashCode = 31 * hashCode + Objects.hashCode(requireLowercaseCharacters());
hashCode = 31 * hashCode + Objects.hashCode(allowUsersToChangePassword());
hashCode = 31 * hashCode + Objects.hashCode(expirePasswords());
hashCode = 31 * hashCode + Objects.hashCode(maxPasswordAge());
hashCode = 31 * hashCode + Objects.hashCode(passwordReusePrevention());
hashCode = 31 * hashCode + Objects.hashCode(hardExpiry());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PasswordPolicy)) {
return false;
}
PasswordPolicy other = (PasswordPolicy) obj;
return Objects.equals(minimumPasswordLength(), other.minimumPasswordLength())
&& Objects.equals(requireSymbols(), other.requireSymbols())
&& Objects.equals(requireNumbers(), other.requireNumbers())
&& Objects.equals(requireUppercaseCharacters(), other.requireUppercaseCharacters())
&& Objects.equals(requireLowercaseCharacters(), other.requireLowercaseCharacters())
&& Objects.equals(allowUsersToChangePassword(), other.allowUsersToChangePassword())
&& Objects.equals(expirePasswords(), other.expirePasswords())
&& Objects.equals(maxPasswordAge(), other.maxPasswordAge())
&& Objects.equals(passwordReusePrevention(), other.passwordReusePrevention())
&& Objects.equals(hardExpiry(), other.hardExpiry());
}
@Override
public String toString() {
return ToString.builder("PasswordPolicy").add("MinimumPasswordLength", minimumPasswordLength())
.add("RequireSymbols", requireSymbols()).add("RequireNumbers", requireNumbers())
.add("RequireUppercaseCharacters", requireUppercaseCharacters())
.add("RequireLowercaseCharacters", requireLowercaseCharacters())
.add("AllowUsersToChangePassword", allowUsersToChangePassword()).add("ExpirePasswords", expirePasswords())
.add("MaxPasswordAge", maxPasswordAge()).add("PasswordReusePrevention", passwordReusePrevention())
.add("HardExpiry", hardExpiry()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MinimumPasswordLength":
return Optional.ofNullable(clazz.cast(minimumPasswordLength()));
case "RequireSymbols":
return Optional.ofNullable(clazz.cast(requireSymbols()));
case "RequireNumbers":
return Optional.ofNullable(clazz.cast(requireNumbers()));
case "RequireUppercaseCharacters":
return Optional.ofNullable(clazz.cast(requireUppercaseCharacters()));
case "RequireLowercaseCharacters":
return Optional.ofNullable(clazz.cast(requireLowercaseCharacters()));
case "AllowUsersToChangePassword":
return Optional.ofNullable(clazz.cast(allowUsersToChangePassword()));
case "ExpirePasswords":
return Optional.ofNullable(clazz.cast(expirePasswords()));
case "MaxPasswordAge":
return Optional.ofNullable(clazz.cast(maxPasswordAge()));
case "PasswordReusePrevention":
return Optional.ofNullable(clazz.cast(passwordReusePrevention()));
case "HardExpiry":
return Optional.ofNullable(clazz.cast(hardExpiry()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function