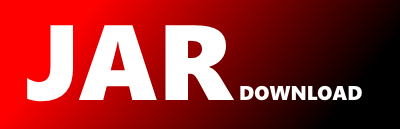
software.amazon.awssdk.services.iam.model.CreateOpenIdConnectProviderRequest Maven / Gradle / Ivy
Show all versions of iam Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateOpenIdConnectProviderRequest extends IamRequest implements
ToCopyableBuilder {
private static final SdkField URL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateOpenIdConnectProviderRequest::url)).setter(setter(Builder::url))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Url").build()).build();
private static final SdkField> CLIENT_ID_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateOpenIdConnectProviderRequest::clientIDList))
.setter(setter(Builder::clientIDList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientIDList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> THUMBPRINT_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateOpenIdConnectProviderRequest::thumbprintList))
.setter(setter(Builder::thumbprintList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ThumbprintList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(URL_FIELD,
CLIENT_ID_LIST_FIELD, THUMBPRINT_LIST_FIELD));
private final String url;
private final List clientIDList;
private final List thumbprintList;
private CreateOpenIdConnectProviderRequest(BuilderImpl builder) {
super(builder);
this.url = builder.url;
this.clientIDList = builder.clientIDList;
this.thumbprintList = builder.thumbprintList;
}
/**
*
* The URL of the identity provider. The URL must begin with https://
and should correspond to the
* iss
claim in the provider's OpenID Connect ID tokens. Per the OIDC standard, path components are
* allowed but query parameters are not. Typically the URL consists of only a hostname, like
* https://server.example.org
or https://example.com
.
*
*
* You cannot register the same provider multiple times in a single AWS account. If you try to submit a URL that has
* already been used for an OpenID Connect provider in the AWS account, you will get an error.
*
*
* @return The URL of the identity provider. The URL must begin with https://
and should correspond to
* the iss
claim in the provider's OpenID Connect ID tokens. Per the OIDC standard, path
* components are allowed but query parameters are not. Typically the URL consists of only a hostname, like
* https://server.example.org
or https://example.com
.
*
* You cannot register the same provider multiple times in a single AWS account. If you try to submit a URL
* that has already been used for an OpenID Connect provider in the AWS account, you will get an error.
*/
public String url() {
return url;
}
/**
*
* A list of client IDs (also known as audiences). When a mobile or web app registers with an OpenID Connect
* provider, they establish a value that identifies the application. (This is the value that's sent as the
* client_id
parameter on OAuth requests.)
*
*
* You can register multiple client IDs with the same provider. For example, you might have multiple applications
* that use the same OIDC provider. You cannot register more than 100 client IDs with a single IAM OIDC provider.
*
*
* There is no defined format for a client ID. The CreateOpenIDConnectProviderRequest
operation accepts
* client IDs up to 255 characters long.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of client IDs (also known as audiences). When a mobile or web app registers with an OpenID Connect
* provider, they establish a value that identifies the application. (This is the value that's sent as the
* client_id
parameter on OAuth requests.)
*
* You can register multiple client IDs with the same provider. For example, you might have multiple
* applications that use the same OIDC provider. You cannot register more than 100 client IDs with a single
* IAM OIDC provider.
*
*
* There is no defined format for a client ID. The CreateOpenIDConnectProviderRequest
operation
* accepts client IDs up to 255 characters long.
*/
public List clientIDList() {
return clientIDList;
}
/**
*
* A list of server certificate thumbprints for the OpenID Connect (OIDC) identity provider's server certificates.
* Typically this list includes only one entry. However, IAM lets you have up to five thumbprints for an OIDC
* provider. This lets you maintain multiple thumbprints if the identity provider is rotating certificates.
*
*
* The server certificate thumbprint is the hex-encoded SHA-1 hash value of the X.509 certificate used by the domain
* where the OpenID Connect provider makes its keys available. It is always a 40-character string.
*
*
* You must provide at least one thumbprint when creating an IAM OIDC provider. For example, assume that the OIDC
* provider is server.example.com
and the provider stores its keys at
* https://keys.server.example.com/openid-connect. In that case, the thumbprint string would be the hex-encoded
* SHA-1 hash value of the certificate used by https://keys.server.example.com.
*
*
* For more information about obtaining the OIDC provider's thumbprint, see Obtaining
* the Thumbprint for an OpenID Connect Provider in the IAM User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of server certificate thumbprints for the OpenID Connect (OIDC) identity provider's server
* certificates. Typically this list includes only one entry. However, IAM lets you have up to five
* thumbprints for an OIDC provider. This lets you maintain multiple thumbprints if the identity provider is
* rotating certificates.
*
* The server certificate thumbprint is the hex-encoded SHA-1 hash value of the X.509 certificate used by
* the domain where the OpenID Connect provider makes its keys available. It is always a 40-character
* string.
*
*
* You must provide at least one thumbprint when creating an IAM OIDC provider. For example, assume that the
* OIDC provider is server.example.com
and the provider stores its keys at
* https://keys.server.example.com/openid-connect. In that case, the thumbprint string would be the
* hex-encoded SHA-1 hash value of the certificate used by https://keys.server.example.com.
*
*
* For more information about obtaining the OIDC provider's thumbprint, see Obtaining the Thumbprint for an OpenID Connect Provider in the IAM User Guide.
*/
public List thumbprintList() {
return thumbprintList;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(url());
hashCode = 31 * hashCode + Objects.hashCode(clientIDList());
hashCode = 31 * hashCode + Objects.hashCode(thumbprintList());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateOpenIdConnectProviderRequest)) {
return false;
}
CreateOpenIdConnectProviderRequest other = (CreateOpenIdConnectProviderRequest) obj;
return Objects.equals(url(), other.url()) && Objects.equals(clientIDList(), other.clientIDList())
&& Objects.equals(thumbprintList(), other.thumbprintList());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CreateOpenIdConnectProviderRequest").add("Url", url()).add("ClientIDList", clientIDList())
.add("ThumbprintList", thumbprintList()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Url":
return Optional.ofNullable(clazz.cast(url()));
case "ClientIDList":
return Optional.ofNullable(clazz.cast(clientIDList()));
case "ThumbprintList":
return Optional.ofNullable(clazz.cast(thumbprintList()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* You cannot register the same provider multiple times in a single AWS account. If you try to submit a
* URL that has already been used for an OpenID Connect provider in the AWS account, you will get an
* error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder url(String url);
/**
*
* A list of client IDs (also known as audiences). When a mobile or web app registers with an OpenID Connect
* provider, they establish a value that identifies the application. (This is the value that's sent as the
* client_id
parameter on OAuth requests.)
*
*
* You can register multiple client IDs with the same provider. For example, you might have multiple
* applications that use the same OIDC provider. You cannot register more than 100 client IDs with a single IAM
* OIDC provider.
*
*
* There is no defined format for a client ID. The CreateOpenIDConnectProviderRequest
operation
* accepts client IDs up to 255 characters long.
*
*
* @param clientIDList
* A list of client IDs (also known as audiences). When a mobile or web app registers with an OpenID
* Connect provider, they establish a value that identifies the application. (This is the value that's
* sent as the client_id
parameter on OAuth requests.)
*
* You can register multiple client IDs with the same provider. For example, you might have multiple
* applications that use the same OIDC provider. You cannot register more than 100 client IDs with a
* single IAM OIDC provider.
*
*
* There is no defined format for a client ID. The CreateOpenIDConnectProviderRequest
* operation accepts client IDs up to 255 characters long.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientIDList(Collection clientIDList);
/**
*
* A list of client IDs (also known as audiences). When a mobile or web app registers with an OpenID Connect
* provider, they establish a value that identifies the application. (This is the value that's sent as the
* client_id
parameter on OAuth requests.)
*
*
* You can register multiple client IDs with the same provider. For example, you might have multiple
* applications that use the same OIDC provider. You cannot register more than 100 client IDs with a single IAM
* OIDC provider.
*
*
* There is no defined format for a client ID. The CreateOpenIDConnectProviderRequest
operation
* accepts client IDs up to 255 characters long.
*
*
* @param clientIDList
* A list of client IDs (also known as audiences). When a mobile or web app registers with an OpenID
* Connect provider, they establish a value that identifies the application. (This is the value that's
* sent as the client_id
parameter on OAuth requests.)
*
* You can register multiple client IDs with the same provider. For example, you might have multiple
* applications that use the same OIDC provider. You cannot register more than 100 client IDs with a
* single IAM OIDC provider.
*
*
* There is no defined format for a client ID. The CreateOpenIDConnectProviderRequest
* operation accepts client IDs up to 255 characters long.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientIDList(String... clientIDList);
/**
*
* A list of server certificate thumbprints for the OpenID Connect (OIDC) identity provider's server
* certificates. Typically this list includes only one entry. However, IAM lets you have up to five thumbprints
* for an OIDC provider. This lets you maintain multiple thumbprints if the identity provider is rotating
* certificates.
*
*
* The server certificate thumbprint is the hex-encoded SHA-1 hash value of the X.509 certificate used by the
* domain where the OpenID Connect provider makes its keys available. It is always a 40-character string.
*
*
* You must provide at least one thumbprint when creating an IAM OIDC provider. For example, assume that the
* OIDC provider is server.example.com
and the provider stores its keys at
* https://keys.server.example.com/openid-connect. In that case, the thumbprint string would be the hex-encoded
* SHA-1 hash value of the certificate used by https://keys.server.example.com.
*
*
* For more information about obtaining the OIDC provider's thumbprint, see Obtaining the Thumbprint for an OpenID Connect Provider in the IAM User Guide.
*
*
* @param thumbprintList
* A list of server certificate thumbprints for the OpenID Connect (OIDC) identity provider's server
* certificates. Typically this list includes only one entry. However, IAM lets you have up to five
* thumbprints for an OIDC provider. This lets you maintain multiple thumbprints if the identity provider
* is rotating certificates.
*
* The server certificate thumbprint is the hex-encoded SHA-1 hash value of the X.509 certificate used by
* the domain where the OpenID Connect provider makes its keys available. It is always a 40-character
* string.
*
*
* You must provide at least one thumbprint when creating an IAM OIDC provider. For example, assume that
* the OIDC provider is server.example.com
and the provider stores its keys at
* https://keys.server.example.com/openid-connect. In that case, the thumbprint string would be the
* hex-encoded SHA-1 hash value of the certificate used by https://keys.server.example.com.
*
*
* For more information about obtaining the OIDC provider's thumbprint, see Obtaining the Thumbprint for an OpenID Connect Provider in the IAM User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder thumbprintList(Collection thumbprintList);
/**
*
* A list of server certificate thumbprints for the OpenID Connect (OIDC) identity provider's server
* certificates. Typically this list includes only one entry. However, IAM lets you have up to five thumbprints
* for an OIDC provider. This lets you maintain multiple thumbprints if the identity provider is rotating
* certificates.
*
*
* The server certificate thumbprint is the hex-encoded SHA-1 hash value of the X.509 certificate used by the
* domain where the OpenID Connect provider makes its keys available. It is always a 40-character string.
*
*
* You must provide at least one thumbprint when creating an IAM OIDC provider. For example, assume that the
* OIDC provider is server.example.com
and the provider stores its keys at
* https://keys.server.example.com/openid-connect. In that case, the thumbprint string would be the hex-encoded
* SHA-1 hash value of the certificate used by https://keys.server.example.com.
*
*
* For more information about obtaining the OIDC provider's thumbprint, see Obtaining the Thumbprint for an OpenID Connect Provider in the IAM User Guide.
*
*
* @param thumbprintList
* A list of server certificate thumbprints for the OpenID Connect (OIDC) identity provider's server
* certificates. Typically this list includes only one entry. However, IAM lets you have up to five
* thumbprints for an OIDC provider. This lets you maintain multiple thumbprints if the identity provider
* is rotating certificates.
*
* The server certificate thumbprint is the hex-encoded SHA-1 hash value of the X.509 certificate used by
* the domain where the OpenID Connect provider makes its keys available. It is always a 40-character
* string.
*
*
* You must provide at least one thumbprint when creating an IAM OIDC provider. For example, assume that
* the OIDC provider is server.example.com
and the provider stores its keys at
* https://keys.server.example.com/openid-connect. In that case, the thumbprint string would be the
* hex-encoded SHA-1 hash value of the certificate used by https://keys.server.example.com.
*
*
* For more information about obtaining the OIDC provider's thumbprint, see Obtaining the Thumbprint for an OpenID Connect Provider in the IAM User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder thumbprintList(String... thumbprintList);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends IamRequest.BuilderImpl implements Builder {
private String url;
private List clientIDList = DefaultSdkAutoConstructList.getInstance();
private List thumbprintList = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(CreateOpenIdConnectProviderRequest model) {
super(model);
url(model.url);
clientIDList(model.clientIDList);
thumbprintList(model.thumbprintList);
}
public final String getUrl() {
return url;
}
@Override
public final Builder url(String url) {
this.url = url;
return this;
}
public final void setUrl(String url) {
this.url = url;
}
public final Collection getClientIDList() {
return clientIDList;
}
@Override
public final Builder clientIDList(Collection clientIDList) {
this.clientIDList = _clientIDListTypeCopier.copy(clientIDList);
return this;
}
@Override
@SafeVarargs
public final Builder clientIDList(String... clientIDList) {
clientIDList(Arrays.asList(clientIDList));
return this;
}
public final void setClientIDList(Collection clientIDList) {
this.clientIDList = _clientIDListTypeCopier.copy(clientIDList);
}
public final Collection getThumbprintList() {
return thumbprintList;
}
@Override
public final Builder thumbprintList(Collection thumbprintList) {
this.thumbprintList = _thumbprintListTypeCopier.copy(thumbprintList);
return this;
}
@Override
@SafeVarargs
public final Builder thumbprintList(String... thumbprintList) {
thumbprintList(Arrays.asList(thumbprintList));
return this;
}
public final void setThumbprintList(Collection thumbprintList) {
this.thumbprintList = _thumbprintListTypeCopier.copy(thumbprintList);
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateOpenIdConnectProviderRequest build() {
return new CreateOpenIdConnectProviderRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}