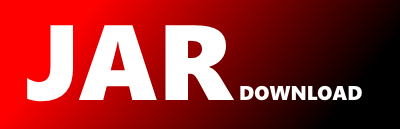
software.amazon.awssdk.services.iam.model.UploadServerCertificateRequest Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iam.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UploadServerCertificateRequest extends IamRequest implements
ToCopyableBuilder {
private static final SdkField PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UploadServerCertificateRequest::path)).setter(setter(Builder::path))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Path").build()).build();
private static final SdkField SERVER_CERTIFICATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UploadServerCertificateRequest::serverCertificateName)).setter(setter(Builder::serverCertificateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerCertificateName").build())
.build();
private static final SdkField CERTIFICATE_BODY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UploadServerCertificateRequest::certificateBody)).setter(setter(Builder::certificateBody))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CertificateBody").build()).build();
private static final SdkField PRIVATE_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UploadServerCertificateRequest::privateKey)).setter(setter(Builder::privateKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrivateKey").build()).build();
private static final SdkField CERTIFICATE_CHAIN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UploadServerCertificateRequest::certificateChain)).setter(setter(Builder::certificateChain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CertificateChain").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PATH_FIELD,
SERVER_CERTIFICATE_NAME_FIELD, CERTIFICATE_BODY_FIELD, PRIVATE_KEY_FIELD, CERTIFICATE_CHAIN_FIELD));
private final String path;
private final String serverCertificateName;
private final String certificateBody;
private final String privateKey;
private final String certificateChain;
private UploadServerCertificateRequest(BuilderImpl builder) {
super(builder);
this.path = builder.path;
this.serverCertificateName = builder.serverCertificateName;
this.certificateBody = builder.certificateBody;
this.privateKey = builder.privateKey;
this.certificateChain = builder.certificateChain;
}
/**
*
* The path for the server certificate. For more information about paths, see IAM Identifiers in the IAM
* User Guide.
*
*
* This parameter is optional. If it is not included, it defaults to a slash (/). This parameter allows (through its
* regex pattern) a string of characters consisting of either a
* forward slash (/) by itself or a string that must begin and end with forward slashes. In addition, it can contain
* any ASCII character from the ! (\u0021) through the DEL character (\u007F), including most punctuation
* characters, digits, and upper and lowercased letters.
*
*
*
* If you are uploading a server certificate specifically for use with Amazon CloudFront distributions, you must
* specify a path using the path
parameter. The path must begin with /cloudfront
and must
* include a trailing slash (for example, /cloudfront/test/
).
*
*
*
* @return The path for the server certificate. For more information about paths, see IAM Identifiers in the
* IAM User Guide.
*
* This parameter is optional. If it is not included, it defaults to a slash (/). This parameter allows
* (through its regex pattern) a string of characters
* consisting of either a forward slash (/) by itself or a string that must begin and end with forward
* slashes. In addition, it can contain any ASCII character from the ! (\u0021) through the DEL character
* (\u007F), including most punctuation characters, digits, and upper and lowercased letters.
*
*
*
* If you are uploading a server certificate specifically for use with Amazon CloudFront distributions, you
* must specify a path using the path
parameter. The path must begin with
* /cloudfront
and must include a trailing slash (for example, /cloudfront/test/
).
*
*/
public String path() {
return path;
}
/**
*
* The name for the server certificate. Do not include the path in this value. The name of the certificate cannot
* contain any spaces.
*
*
* This parameter allows (through its regex pattern) a string of
* characters consisting of upper and lowercase alphanumeric characters with no spaces. You can also include any of
* the following characters: _+=,.@-
*
*
* @return The name for the server certificate. Do not include the path in this value. The name of the certificate
* cannot contain any spaces.
*
* This parameter allows (through its regex pattern) a string
* of characters consisting of upper and lowercase alphanumeric characters with no spaces. You can also
* include any of the following characters: _+=,.@-
*/
public String serverCertificateName() {
return serverCertificateName;
}
/**
*
* The contents of the public key certificate in PEM-encoded format.
*
*
* The regex pattern used to validate this parameter is a string of
* characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character ( ) through the end of the ASCII character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF)
*
*
* -
*
* The special characters tab ( ), line feed ( ), and carriage return ( )
*
*
*
*
* @return The contents of the public key certificate in PEM-encoded format.
*
* The regex pattern used to validate this parameter is a
* string of characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character ( ) through the end of the ASCII character
* range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF)
*
*
* -
*
* The special characters tab ( ), line feed ( ), and carriage return ( )
*
*
*/
public String certificateBody() {
return certificateBody;
}
/**
*
* The contents of the private key in PEM-encoded format.
*
*
* The regex pattern used to validate this parameter is a string of
* characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character ( ) through the end of the ASCII character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF)
*
*
* -
*
* The special characters tab ( ), line feed ( ), and carriage return ( )
*
*
*
*
* @return The contents of the private key in PEM-encoded format.
*
* The regex pattern used to validate this parameter is a
* string of characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character ( ) through the end of the ASCII character
* range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF)
*
*
* -
*
* The special characters tab ( ), line feed ( ), and carriage return ( )
*
*
*/
public String privateKey() {
return privateKey;
}
/**
*
* The contents of the certificate chain. This is typically a concatenation of the PEM-encoded public key
* certificates of the chain.
*
*
* The regex pattern used to validate this parameter is a string of
* characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character ( ) through the end of the ASCII character range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF)
*
*
* -
*
* The special characters tab ( ), line feed ( ), and carriage return ( )
*
*
*
*
* @return The contents of the certificate chain. This is typically a concatenation of the PEM-encoded public key
* certificates of the chain.
*
* The regex pattern used to validate this parameter is a
* string of characters consisting of the following:
*
*
* -
*
* Any printable ASCII character ranging from the space character ( ) through the end of the ASCII character
* range
*
*
* -
*
* The printable characters in the Basic Latin and Latin-1 Supplement character set (through \u00FF)
*
*
* -
*
* The special characters tab ( ), line feed ( ), and carriage return ( )
*
*
*/
public String certificateChain() {
return certificateChain;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(path());
hashCode = 31 * hashCode + Objects.hashCode(serverCertificateName());
hashCode = 31 * hashCode + Objects.hashCode(certificateBody());
hashCode = 31 * hashCode + Objects.hashCode(privateKey());
hashCode = 31 * hashCode + Objects.hashCode(certificateChain());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UploadServerCertificateRequest)) {
return false;
}
UploadServerCertificateRequest other = (UploadServerCertificateRequest) obj;
return Objects.equals(path(), other.path()) && Objects.equals(serverCertificateName(), other.serverCertificateName())
&& Objects.equals(certificateBody(), other.certificateBody()) && Objects.equals(privateKey(), other.privateKey())
&& Objects.equals(certificateChain(), other.certificateChain());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("UploadServerCertificateRequest").add("Path", path())
.add("ServerCertificateName", serverCertificateName()).add("CertificateBody", certificateBody())
.add("PrivateKey", privateKey() == null ? null : "*** Sensitive Data Redacted ***")
.add("CertificateChain", certificateChain()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Path":
return Optional.ofNullable(clazz.cast(path()));
case "ServerCertificateName":
return Optional.ofNullable(clazz.cast(serverCertificateName()));
case "CertificateBody":
return Optional.ofNullable(clazz.cast(certificateBody()));
case "PrivateKey":
return Optional.ofNullable(clazz.cast(privateKey()));
case "CertificateChain":
return Optional.ofNullable(clazz.cast(certificateChain()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function