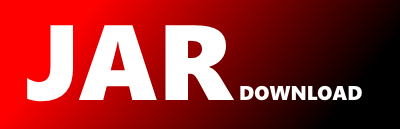
software.amazon.awssdk.services.inspector.model.AssessmentTemplate Maven / Gradle / Ivy
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.inspector.model;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import javax.annotation.Generated;
import software.amazon.awssdk.annotation.SdkInternalApi;
import software.amazon.awssdk.protocol.ProtocolMarshaller;
import software.amazon.awssdk.protocol.StructuredPojo;
import software.amazon.awssdk.runtime.StandardMemberCopier;
import software.amazon.awssdk.services.inspector.transform.AssessmentTemplateMarshaller;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about an Amazon Inspector assessment template. This data type is used as the response element in
* the DescribeAssessmentTemplates action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class AssessmentTemplate implements StructuredPojo, ToCopyableBuilder {
private final String arn;
private final String name;
private final String assessmentTargetArn;
private final Integer durationInSeconds;
private final List rulesPackageArns;
private final List userAttributesForFindings;
private final Date createdAt;
private AssessmentTemplate(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.assessmentTargetArn = builder.assessmentTargetArn;
this.durationInSeconds = builder.durationInSeconds;
this.rulesPackageArns = builder.rulesPackageArns;
this.userAttributesForFindings = builder.userAttributesForFindings;
this.createdAt = builder.createdAt;
}
/**
*
* The ARN of the assessment template.
*
*
* @return The ARN of the assessment template.
*/
public String arn() {
return arn;
}
/**
*
* The name of the assessment template.
*
*
* @return The name of the assessment template.
*/
public String name() {
return name;
}
/**
*
* The ARN of the assessment target that corresponds to this assessment template.
*
*
* @return The ARN of the assessment target that corresponds to this assessment template.
*/
public String assessmentTargetArn() {
return assessmentTargetArn;
}
/**
*
* The duration in seconds specified for this assessment tempate. The default value is 3600 seconds (one hour). The
* maximum value is 86400 seconds (one day).
*
*
* @return The duration in seconds specified for this assessment tempate. The default value is 3600 seconds (one
* hour). The maximum value is 86400 seconds (one day).
*/
public Integer durationInSeconds() {
return durationInSeconds;
}
/**
*
* The rules packages that are specified for this assessment template.
*
*
* @return The rules packages that are specified for this assessment template.
*/
public List rulesPackageArns() {
return rulesPackageArns;
}
/**
*
* The user-defined attributes that are assigned to every generated finding from the assessment run that uses this
* assessment template.
*
*
* @return The user-defined attributes that are assigned to every generated finding from the assessment run that
* uses this assessment template.
*/
public List userAttributesForFindings() {
return userAttributesForFindings;
}
/**
*
* The time at which the assessment template is created.
*
*
* @return The time at which the assessment template is created.
*/
public Date createdAt() {
return createdAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((arn() == null) ? 0 : arn().hashCode());
hashCode = 31 * hashCode + ((name() == null) ? 0 : name().hashCode());
hashCode = 31 * hashCode + ((assessmentTargetArn() == null) ? 0 : assessmentTargetArn().hashCode());
hashCode = 31 * hashCode + ((durationInSeconds() == null) ? 0 : durationInSeconds().hashCode());
hashCode = 31 * hashCode + ((rulesPackageArns() == null) ? 0 : rulesPackageArns().hashCode());
hashCode = 31 * hashCode + ((userAttributesForFindings() == null) ? 0 : userAttributesForFindings().hashCode());
hashCode = 31 * hashCode + ((createdAt() == null) ? 0 : createdAt().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AssessmentTemplate)) {
return false;
}
AssessmentTemplate other = (AssessmentTemplate) obj;
if (other.arn() == null ^ this.arn() == null) {
return false;
}
if (other.arn() != null && !other.arn().equals(this.arn())) {
return false;
}
if (other.name() == null ^ this.name() == null) {
return false;
}
if (other.name() != null && !other.name().equals(this.name())) {
return false;
}
if (other.assessmentTargetArn() == null ^ this.assessmentTargetArn() == null) {
return false;
}
if (other.assessmentTargetArn() != null && !other.assessmentTargetArn().equals(this.assessmentTargetArn())) {
return false;
}
if (other.durationInSeconds() == null ^ this.durationInSeconds() == null) {
return false;
}
if (other.durationInSeconds() != null && !other.durationInSeconds().equals(this.durationInSeconds())) {
return false;
}
if (other.rulesPackageArns() == null ^ this.rulesPackageArns() == null) {
return false;
}
if (other.rulesPackageArns() != null && !other.rulesPackageArns().equals(this.rulesPackageArns())) {
return false;
}
if (other.userAttributesForFindings() == null ^ this.userAttributesForFindings() == null) {
return false;
}
if (other.userAttributesForFindings() != null
&& !other.userAttributesForFindings().equals(this.userAttributesForFindings())) {
return false;
}
if (other.createdAt() == null ^ this.createdAt() == null) {
return false;
}
if (other.createdAt() != null && !other.createdAt().equals(this.createdAt())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (arn() != null) {
sb.append("Arn: ").append(arn()).append(",");
}
if (name() != null) {
sb.append("Name: ").append(name()).append(",");
}
if (assessmentTargetArn() != null) {
sb.append("AssessmentTargetArn: ").append(assessmentTargetArn()).append(",");
}
if (durationInSeconds() != null) {
sb.append("DurationInSeconds: ").append(durationInSeconds()).append(",");
}
if (rulesPackageArns() != null) {
sb.append("RulesPackageArns: ").append(rulesPackageArns()).append(",");
}
if (userAttributesForFindings() != null) {
sb.append("UserAttributesForFindings: ").append(userAttributesForFindings()).append(",");
}
if (createdAt() != null) {
sb.append("CreatedAt: ").append(createdAt()).append(",");
}
sb.append("}");
return sb.toString();
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
AssessmentTemplateMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* The ARN of the assessment template.
*
*
* @param arn
* The ARN of the assessment template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder arn(String arn);
/**
*
* The name of the assessment template.
*
*
* @param name
* The name of the assessment template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
*
* The ARN of the assessment target that corresponds to this assessment template.
*
*
* @param assessmentTargetArn
* The ARN of the assessment target that corresponds to this assessment template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assessmentTargetArn(String assessmentTargetArn);
/**
*
* The duration in seconds specified for this assessment tempate. The default value is 3600 seconds (one hour).
* The maximum value is 86400 seconds (one day).
*
*
* @param durationInSeconds
* The duration in seconds specified for this assessment tempate. The default value is 3600 seconds (one
* hour). The maximum value is 86400 seconds (one day).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder durationInSeconds(Integer durationInSeconds);
/**
*
* The rules packages that are specified for this assessment template.
*
*
* @param rulesPackageArns
* The rules packages that are specified for this assessment template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder rulesPackageArns(Collection rulesPackageArns);
/**
*
* The rules packages that are specified for this assessment template.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRulesPackageArns(java.util.Collection)} or {@link #withRulesPackageArns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param rulesPackageArns
* The rules packages that are specified for this assessment template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder rulesPackageArns(String... rulesPackageArns);
/**
*
* The user-defined attributes that are assigned to every generated finding from the assessment run that uses
* this assessment template.
*
*
* @param userAttributesForFindings
* The user-defined attributes that are assigned to every generated finding from the assessment run that
* uses this assessment template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder userAttributesForFindings(Collection userAttributesForFindings);
/**
*
* The user-defined attributes that are assigned to every generated finding from the assessment run that uses
* this assessment template.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUserAttributesForFindings(java.util.Collection)} or
* {@link #withUserAttributesForFindings(java.util.Collection)} if you want to override the existing values.
*
*
* @param userAttributesForFindings
* The user-defined attributes that are assigned to every generated finding from the assessment run that
* uses this assessment template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder userAttributesForFindings(Attribute... userAttributesForFindings);
/**
*
* The time at which the assessment template is created.
*
*
* @param createdAt
* The time at which the assessment template is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder createdAt(Date createdAt);
}
private static final class BuilderImpl implements Builder {
private String arn;
private String name;
private String assessmentTargetArn;
private Integer durationInSeconds;
private List rulesPackageArns;
private List userAttributesForFindings;
private Date createdAt;
private BuilderImpl() {
}
private BuilderImpl(AssessmentTemplate model) {
setArn(model.arn);
setName(model.name);
setAssessmentTargetArn(model.assessmentTargetArn);
setDurationInSeconds(model.durationInSeconds);
setRulesPackageArns(model.rulesPackageArns);
setUserAttributesForFindings(model.userAttributesForFindings);
setCreatedAt(model.createdAt);
}
public final String getArn() {
return arn;
}
@Override
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
public final void setArn(String arn) {
this.arn = arn;
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final String getAssessmentTargetArn() {
return assessmentTargetArn;
}
@Override
public final Builder assessmentTargetArn(String assessmentTargetArn) {
this.assessmentTargetArn = assessmentTargetArn;
return this;
}
public final void setAssessmentTargetArn(String assessmentTargetArn) {
this.assessmentTargetArn = assessmentTargetArn;
}
public final Integer getDurationInSeconds() {
return durationInSeconds;
}
@Override
public final Builder durationInSeconds(Integer durationInSeconds) {
this.durationInSeconds = durationInSeconds;
return this;
}
public final void setDurationInSeconds(Integer durationInSeconds) {
this.durationInSeconds = durationInSeconds;
}
public final Collection getRulesPackageArns() {
return rulesPackageArns;
}
@Override
public final Builder rulesPackageArns(Collection rulesPackageArns) {
this.rulesPackageArns = AssessmentTemplateRulesPackageArnListCopier.copy(rulesPackageArns);
return this;
}
@Override
@SafeVarargs
public final Builder rulesPackageArns(String... rulesPackageArns) {
if (this.rulesPackageArns == null) {
this.rulesPackageArns = new ArrayList<>(rulesPackageArns.length);
}
for (String e : rulesPackageArns) {
this.rulesPackageArns.add(e);
}
return this;
}
public final void setRulesPackageArns(Collection rulesPackageArns) {
this.rulesPackageArns = AssessmentTemplateRulesPackageArnListCopier.copy(rulesPackageArns);
}
@SafeVarargs
public final void setRulesPackageArns(String... rulesPackageArns) {
if (this.rulesPackageArns == null) {
this.rulesPackageArns = new ArrayList<>(rulesPackageArns.length);
}
for (String e : rulesPackageArns) {
this.rulesPackageArns.add(e);
}
}
public final Collection getUserAttributesForFindings() {
return userAttributesForFindings;
}
@Override
public final Builder userAttributesForFindings(Collection userAttributesForFindings) {
this.userAttributesForFindings = UserAttributeListCopier.copy(userAttributesForFindings);
return this;
}
@Override
@SafeVarargs
public final Builder userAttributesForFindings(Attribute... userAttributesForFindings) {
if (this.userAttributesForFindings == null) {
this.userAttributesForFindings = new ArrayList<>(userAttributesForFindings.length);
}
for (Attribute e : userAttributesForFindings) {
this.userAttributesForFindings.add(e);
}
return this;
}
public final void setUserAttributesForFindings(Collection userAttributesForFindings) {
this.userAttributesForFindings = UserAttributeListCopier.copy(userAttributesForFindings);
}
@SafeVarargs
public final void setUserAttributesForFindings(Attribute... userAttributesForFindings) {
if (this.userAttributesForFindings == null) {
this.userAttributesForFindings = new ArrayList<>(userAttributesForFindings.length);
}
for (Attribute e : userAttributesForFindings) {
this.userAttributesForFindings.add(e);
}
}
public final Date getCreatedAt() {
return createdAt;
}
@Override
public final Builder createdAt(Date createdAt) {
this.createdAt = StandardMemberCopier.copy(createdAt);
return this;
}
public final void setCreatedAt(Date createdAt) {
this.createdAt = StandardMemberCopier.copy(createdAt);
}
@Override
public AssessmentTemplate build() {
return new AssessmentTemplate(this);
}
}
}