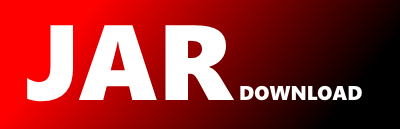
software.amazon.awssdk.services.inspector.model.AgentPreview Maven / Gradle / Ivy
Show all versions of inspector Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.inspector.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Used as a response element in the PreviewAgents action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AgentPreview implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField HOSTNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("hostname").getter(getter(AgentPreview::hostname)).setter(setter(Builder::hostname))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hostname").build()).build();
private static final SdkField AGENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("agentId").getter(getter(AgentPreview::agentId)).setter(setter(Builder::agentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("agentId").build()).build();
private static final SdkField AUTO_SCALING_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("autoScalingGroup").getter(getter(AgentPreview::autoScalingGroup))
.setter(setter(Builder::autoScalingGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("autoScalingGroup").build()).build();
private static final SdkField AGENT_HEALTH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("agentHealth").getter(getter(AgentPreview::agentHealthAsString)).setter(setter(Builder::agentHealth))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("agentHealth").build()).build();
private static final SdkField AGENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("agentVersion").getter(getter(AgentPreview::agentVersion)).setter(setter(Builder::agentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("agentVersion").build()).build();
private static final SdkField OPERATING_SYSTEM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("operatingSystem").getter(getter(AgentPreview::operatingSystem)).setter(setter(Builder::operatingSystem))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("operatingSystem").build()).build();
private static final SdkField KERNEL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kernelVersion").getter(getter(AgentPreview::kernelVersion)).setter(setter(Builder::kernelVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kernelVersion").build()).build();
private static final SdkField IPV4_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ipv4Address").getter(getter(AgentPreview::ipv4Address)).setter(setter(Builder::ipv4Address))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ipv4Address").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(HOSTNAME_FIELD,
AGENT_ID_FIELD, AUTO_SCALING_GROUP_FIELD, AGENT_HEALTH_FIELD, AGENT_VERSION_FIELD, OPERATING_SYSTEM_FIELD,
KERNEL_VERSION_FIELD, IPV4_ADDRESS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("hostname", HOSTNAME_FIELD);
put("agentId", AGENT_ID_FIELD);
put("autoScalingGroup", AUTO_SCALING_GROUP_FIELD);
put("agentHealth", AGENT_HEALTH_FIELD);
put("agentVersion", AGENT_VERSION_FIELD);
put("operatingSystem", OPERATING_SYSTEM_FIELD);
put("kernelVersion", KERNEL_VERSION_FIELD);
put("ipv4Address", IPV4_ADDRESS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String hostname;
private final String agentId;
private final String autoScalingGroup;
private final String agentHealth;
private final String agentVersion;
private final String operatingSystem;
private final String kernelVersion;
private final String ipv4Address;
private AgentPreview(BuilderImpl builder) {
this.hostname = builder.hostname;
this.agentId = builder.agentId;
this.autoScalingGroup = builder.autoScalingGroup;
this.agentHealth = builder.agentHealth;
this.agentVersion = builder.agentVersion;
this.operatingSystem = builder.operatingSystem;
this.kernelVersion = builder.kernelVersion;
this.ipv4Address = builder.ipv4Address;
}
/**
*
* The hostname of the EC2 instance on which the Amazon Inspector Agent is installed.
*
*
* @return The hostname of the EC2 instance on which the Amazon Inspector Agent is installed.
*/
public final String hostname() {
return hostname;
}
/**
*
* The ID of the EC2 instance where the agent is installed.
*
*
* @return The ID of the EC2 instance where the agent is installed.
*/
public final String agentId() {
return agentId;
}
/**
*
* The Auto Scaling group for the EC2 instance where the agent is installed.
*
*
* @return The Auto Scaling group for the EC2 instance where the agent is installed.
*/
public final String autoScalingGroup() {
return autoScalingGroup;
}
/**
*
* The health status of the Amazon Inspector Agent.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #agentHealth} will
* return {@link AgentHealth#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #agentHealthAsString}.
*
*
* @return The health status of the Amazon Inspector Agent.
* @see AgentHealth
*/
public final AgentHealth agentHealth() {
return AgentHealth.fromValue(agentHealth);
}
/**
*
* The health status of the Amazon Inspector Agent.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #agentHealth} will
* return {@link AgentHealth#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #agentHealthAsString}.
*
*
* @return The health status of the Amazon Inspector Agent.
* @see AgentHealth
*/
public final String agentHealthAsString() {
return agentHealth;
}
/**
*
* The version of the Amazon Inspector Agent.
*
*
* @return The version of the Amazon Inspector Agent.
*/
public final String agentVersion() {
return agentVersion;
}
/**
*
* The operating system running on the EC2 instance on which the Amazon Inspector Agent is installed.
*
*
* @return The operating system running on the EC2 instance on which the Amazon Inspector Agent is installed.
*/
public final String operatingSystem() {
return operatingSystem;
}
/**
*
* The kernel version of the operating system running on the EC2 instance on which the Amazon Inspector Agent is
* installed.
*
*
* @return The kernel version of the operating system running on the EC2 instance on which the Amazon Inspector
* Agent is installed.
*/
public final String kernelVersion() {
return kernelVersion;
}
/**
*
* The IP address of the EC2 instance on which the Amazon Inspector Agent is installed.
*
*
* @return The IP address of the EC2 instance on which the Amazon Inspector Agent is installed.
*/
public final String ipv4Address() {
return ipv4Address;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hostname());
hashCode = 31 * hashCode + Objects.hashCode(agentId());
hashCode = 31 * hashCode + Objects.hashCode(autoScalingGroup());
hashCode = 31 * hashCode + Objects.hashCode(agentHealthAsString());
hashCode = 31 * hashCode + Objects.hashCode(agentVersion());
hashCode = 31 * hashCode + Objects.hashCode(operatingSystem());
hashCode = 31 * hashCode + Objects.hashCode(kernelVersion());
hashCode = 31 * hashCode + Objects.hashCode(ipv4Address());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AgentPreview)) {
return false;
}
AgentPreview other = (AgentPreview) obj;
return Objects.equals(hostname(), other.hostname()) && Objects.equals(agentId(), other.agentId())
&& Objects.equals(autoScalingGroup(), other.autoScalingGroup())
&& Objects.equals(agentHealthAsString(), other.agentHealthAsString())
&& Objects.equals(agentVersion(), other.agentVersion())
&& Objects.equals(operatingSystem(), other.operatingSystem())
&& Objects.equals(kernelVersion(), other.kernelVersion()) && Objects.equals(ipv4Address(), other.ipv4Address());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AgentPreview").add("Hostname", hostname()).add("AgentId", agentId())
.add("AutoScalingGroup", autoScalingGroup()).add("AgentHealth", agentHealthAsString())
.add("AgentVersion", agentVersion()).add("OperatingSystem", operatingSystem())
.add("KernelVersion", kernelVersion()).add("Ipv4Address", ipv4Address()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "hostname":
return Optional.ofNullable(clazz.cast(hostname()));
case "agentId":
return Optional.ofNullable(clazz.cast(agentId()));
case "autoScalingGroup":
return Optional.ofNullable(clazz.cast(autoScalingGroup()));
case "agentHealth":
return Optional.ofNullable(clazz.cast(agentHealthAsString()));
case "agentVersion":
return Optional.ofNullable(clazz.cast(agentVersion()));
case "operatingSystem":
return Optional.ofNullable(clazz.cast(operatingSystem()));
case "kernelVersion":
return Optional.ofNullable(clazz.cast(kernelVersion()));
case "ipv4Address":
return Optional.ofNullable(clazz.cast(ipv4Address()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function