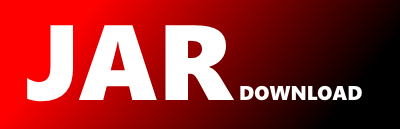
software.amazon.awssdk.services.inspector.model.CreateAssessmentTemplateRequest Maven / Gradle / Ivy
Show all versions of inspector Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.inspector.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateAssessmentTemplateRequest extends InspectorRequest implements
ToCopyableBuilder {
private static final SdkField ASSESSMENT_TARGET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("assessmentTargetArn").getter(getter(CreateAssessmentTemplateRequest::assessmentTargetArn))
.setter(setter(Builder::assessmentTargetArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assessmentTargetArn").build())
.build();
private static final SdkField ASSESSMENT_TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("assessmentTemplateName").getter(getter(CreateAssessmentTemplateRequest::assessmentTemplateName))
.setter(setter(Builder::assessmentTemplateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assessmentTemplateName").build())
.build();
private static final SdkField DURATION_IN_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("durationInSeconds").getter(getter(CreateAssessmentTemplateRequest::durationInSeconds))
.setter(setter(Builder::durationInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("durationInSeconds").build()).build();
private static final SdkField> RULES_PACKAGE_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("rulesPackageArns")
.getter(getter(CreateAssessmentTemplateRequest::rulesPackageArns))
.setter(setter(Builder::rulesPackageArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rulesPackageArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> USER_ATTRIBUTES_FOR_FINDINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("userAttributesForFindings")
.getter(getter(CreateAssessmentTemplateRequest::userAttributesForFindings))
.setter(setter(Builder::userAttributesForFindings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("userAttributesForFindings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attribute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ASSESSMENT_TARGET_ARN_FIELD,
ASSESSMENT_TEMPLATE_NAME_FIELD, DURATION_IN_SECONDS_FIELD, RULES_PACKAGE_ARNS_FIELD,
USER_ATTRIBUTES_FOR_FINDINGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("assessmentTargetArn", ASSESSMENT_TARGET_ARN_FIELD);
put("assessmentTemplateName", ASSESSMENT_TEMPLATE_NAME_FIELD);
put("durationInSeconds", DURATION_IN_SECONDS_FIELD);
put("rulesPackageArns", RULES_PACKAGE_ARNS_FIELD);
put("userAttributesForFindings", USER_ATTRIBUTES_FOR_FINDINGS_FIELD);
}
});
private final String assessmentTargetArn;
private final String assessmentTemplateName;
private final Integer durationInSeconds;
private final List rulesPackageArns;
private final List userAttributesForFindings;
private CreateAssessmentTemplateRequest(BuilderImpl builder) {
super(builder);
this.assessmentTargetArn = builder.assessmentTargetArn;
this.assessmentTemplateName = builder.assessmentTemplateName;
this.durationInSeconds = builder.durationInSeconds;
this.rulesPackageArns = builder.rulesPackageArns;
this.userAttributesForFindings = builder.userAttributesForFindings;
}
/**
*
* The ARN that specifies the assessment target for which you want to create the assessment template.
*
*
* @return The ARN that specifies the assessment target for which you want to create the assessment template.
*/
public final String assessmentTargetArn() {
return assessmentTargetArn;
}
/**
*
* The user-defined name that identifies the assessment template that you want to create. You can create several
* assessment templates for an assessment target. The names of the assessment templates that correspond to a
* particular assessment target must be unique.
*
*
* @return The user-defined name that identifies the assessment template that you want to create. You can create
* several assessment templates for an assessment target. The names of the assessment templates that
* correspond to a particular assessment target must be unique.
*/
public final String assessmentTemplateName() {
return assessmentTemplateName;
}
/**
*
* The duration of the assessment run in seconds.
*
*
* @return The duration of the assessment run in seconds.
*/
public final Integer durationInSeconds() {
return durationInSeconds;
}
/**
* For responses, this returns true if the service returned a value for the RulesPackageArns property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRulesPackageArns() {
return rulesPackageArns != null && !(rulesPackageArns instanceof SdkAutoConstructList);
}
/**
*
* The ARNs that specify the rules packages that you want to attach to the assessment template.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRulesPackageArns} method.
*
*
* @return The ARNs that specify the rules packages that you want to attach to the assessment template.
*/
public final List rulesPackageArns() {
return rulesPackageArns;
}
/**
* For responses, this returns true if the service returned a value for the UserAttributesForFindings property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasUserAttributesForFindings() {
return userAttributesForFindings != null && !(userAttributesForFindings instanceof SdkAutoConstructList);
}
/**
*
* The user-defined attributes that are assigned to every finding that is generated by the assessment run that uses
* this assessment template. An attribute is a key and value pair (an Attribute object). Within an assessment
* template, each key must be unique.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasUserAttributesForFindings} method.
*
*
* @return The user-defined attributes that are assigned to every finding that is generated by the assessment run
* that uses this assessment template. An attribute is a key and value pair (an Attribute object).
* Within an assessment template, each key must be unique.
*/
public final List userAttributesForFindings() {
return userAttributesForFindings;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(assessmentTargetArn());
hashCode = 31 * hashCode + Objects.hashCode(assessmentTemplateName());
hashCode = 31 * hashCode + Objects.hashCode(durationInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(hasRulesPackageArns() ? rulesPackageArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasUserAttributesForFindings() ? userAttributesForFindings() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateAssessmentTemplateRequest)) {
return false;
}
CreateAssessmentTemplateRequest other = (CreateAssessmentTemplateRequest) obj;
return Objects.equals(assessmentTargetArn(), other.assessmentTargetArn())
&& Objects.equals(assessmentTemplateName(), other.assessmentTemplateName())
&& Objects.equals(durationInSeconds(), other.durationInSeconds())
&& hasRulesPackageArns() == other.hasRulesPackageArns()
&& Objects.equals(rulesPackageArns(), other.rulesPackageArns())
&& hasUserAttributesForFindings() == other.hasUserAttributesForFindings()
&& Objects.equals(userAttributesForFindings(), other.userAttributesForFindings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateAssessmentTemplateRequest").add("AssessmentTargetArn", assessmentTargetArn())
.add("AssessmentTemplateName", assessmentTemplateName()).add("DurationInSeconds", durationInSeconds())
.add("RulesPackageArns", hasRulesPackageArns() ? rulesPackageArns() : null)
.add("UserAttributesForFindings", hasUserAttributesForFindings() ? userAttributesForFindings() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "assessmentTargetArn":
return Optional.ofNullable(clazz.cast(assessmentTargetArn()));
case "assessmentTemplateName":
return Optional.ofNullable(clazz.cast(assessmentTemplateName()));
case "durationInSeconds":
return Optional.ofNullable(clazz.cast(durationInSeconds()));
case "rulesPackageArns":
return Optional.ofNullable(clazz.cast(rulesPackageArns()));
case "userAttributesForFindings":
return Optional.ofNullable(clazz.cast(userAttributesForFindings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function