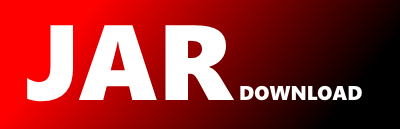
software.amazon.awssdk.services.inspector.model.AssessmentTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of inspector Show documentation
Show all versions of inspector Show documentation
The AWS Java SDK for Amazon Inspector Service module holds the client classes that are used for
communicating with Amazon Inspector Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.inspector.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about an Amazon Inspector application. This data type is used as the response element in the
* DescribeAssessmentTargets action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AssessmentTarget implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(AssessmentTarget::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(AssessmentTarget::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField RESOURCE_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceGroupArn").getter(getter(AssessmentTarget::resourceGroupArn))
.setter(setter(Builder::resourceGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceGroupArn").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(AssessmentTarget::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updatedAt").getter(getter(AssessmentTarget::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
RESOURCE_GROUP_ARN_FIELD, CREATED_AT_FIELD, UPDATED_AT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("arn", ARN_FIELD);
put("name", NAME_FIELD);
put("resourceGroupArn", RESOURCE_GROUP_ARN_FIELD);
put("createdAt", CREATED_AT_FIELD);
put("updatedAt", UPDATED_AT_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final String resourceGroupArn;
private final Instant createdAt;
private final Instant updatedAt;
private AssessmentTarget(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.resourceGroupArn = builder.resourceGroupArn;
this.createdAt = builder.createdAt;
this.updatedAt = builder.updatedAt;
}
/**
*
* The ARN that specifies the Amazon Inspector assessment target.
*
*
* @return The ARN that specifies the Amazon Inspector assessment target.
*/
public final String arn() {
return arn;
}
/**
*
* The name of the Amazon Inspector assessment target.
*
*
* @return The name of the Amazon Inspector assessment target.
*/
public final String name() {
return name;
}
/**
*
* The ARN that specifies the resource group that is associated with the assessment target.
*
*
* @return The ARN that specifies the resource group that is associated with the assessment target.
*/
public final String resourceGroupArn() {
return resourceGroupArn;
}
/**
*
* The time at which the assessment target is created.
*
*
* @return The time at which the assessment target is created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The time at which UpdateAssessmentTarget is called.
*
*
* @return The time at which UpdateAssessmentTarget is called.
*/
public final Instant updatedAt() {
return updatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(resourceGroupArn());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AssessmentTarget)) {
return false;
}
AssessmentTarget other = (AssessmentTarget) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(resourceGroupArn(), other.resourceGroupArn()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(updatedAt(), other.updatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AssessmentTarget").add("Arn", arn()).add("Name", name())
.add("ResourceGroupArn", resourceGroupArn()).add("CreatedAt", createdAt()).add("UpdatedAt", updatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "resourceGroupArn":
return Optional.ofNullable(clazz.cast(resourceGroupArn()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "updatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy